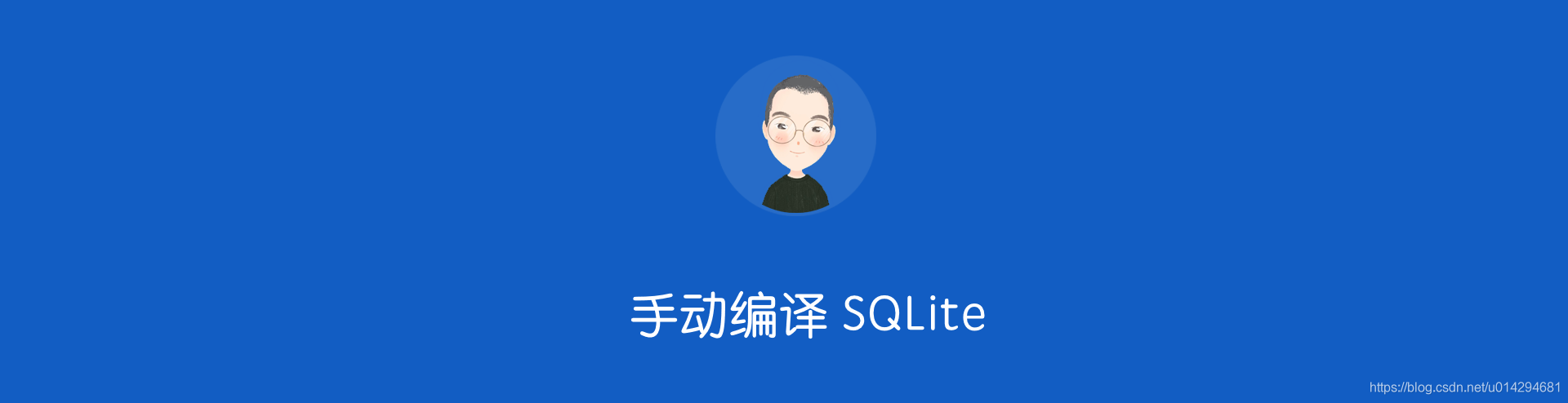
今天来写 NDK 的最后一篇 - SQLite,下一篇文章将进入 Framework 的世界。
SQLite,是一款轻型的数据库,是遵守 ACID 的关系型数据库,它包含在一个相对小的 C 库中。它是 D.RichardHipp 建立的公有领域项目。它的设计目标是嵌入式的,而且目前已经在很多嵌入式产品中使用了它,它占用资源非常的低,在嵌入式中,可能只需要几百K的内存就够了。它能够支持 Windows/Linux/Unix 等主流的操作系统,同时能够跟很多程序语言相结合,比如 C#、PHP、Java 等。还有 ODBC 接口,比起 Mysql、PostgreSQL 这两款开源的世界著名数据库管理系统来讲,它的处理速度比它们都快。SQLite 第一个版本诞生于2000年5月,至2015年已经有15个年头,SQLite 也迎来了新版本 SQLite 3 的发布。
目录:
- 获取 SQLite3 源代码
- 编写 Java 接口
- 编写 JNI 类
- Android.mk 和 Application.mk
- 编译生成 so 库
1. 获取 SQLite3 源代码
可以上官网下载源码,SQLite 的源码没有选择使用 github 托管,下载地址:https://sqlite.org/download.html
如果需要快速获取源码调试,可以使用这份:https://github.com/HuiLi/Sqlite3.07.14/tree/master/Sqlite3.07.14-run,不过版本比较旧。
我这边使用的是:SQLite 3.18.0:

sqlite3.h 的代码有一万行,sqlite3.c 的代码有二十多万行。
2. 编写 Java 接口
SQLiteDatabase.java:
package io.kzw.sqlite3native;
public class SQLiteDatabase {
native int opendb(String fileName, String tempDir) throws SQLiteException;
native void closedb(int sqliteHandle) throws SQLiteException;
native void beginTransaction(int sqliteHandle);
native void commitTransaction(int sqliteHandle);
}
SQLiteCursor.java:
package io.kzw.sqlite3native;
public class SQLiteCursor {
native int columnType(int statementHandle, int columnIndex);
native int columnIsNull(int statementHandle, int columnIndex);
native int columnIntValue(int statementHandle, int columnIndex);
native long columnLongValue(int statementHandle, int columnIndex);
native double columnDoubleValue(int statementHandle, int columnIndex);
native String columnStringValue(int statementHandle, int columnIndex);
native byte[] columnByteArrayValue(int statementHandle, int columnIndex);
native int columnByteBufferValue(int statementHandle, int columnIndex);
}
SQLitePreparedStatement:
package io.kzw.sqlite3native;
public class SQLitePreparedStatement {
native void bindByteBuffer(int statementHandle, int index, ByteBuffer value, int length) throws SQLiteException;
native void bindString(int statementHandle, int index, String value) throws SQLiteException;
native void bindInt(int statementHandle, int index, int value) throws SQLiteException;
native void bindLong(int statementHandle, int index, long value) throws SQLiteException;
native void bindDouble(int statementHandle, int index, double value) throws SQLiteException;
native void bindNull(int statementHandle, int index) throws SQLiteException;
native void reset(int statementHandle) throws SQLiteException;
native int prepare(int sqliteHandle, String sql) throws SQLiteException;
native void finalize(int statementHandle) throws SQLiteException;
native int step(int statementHandle) throws SQLiteException;
}
SQLiteException:
package io.kzw.sqlite3native;
public class SQLiteException extends Exception {
private static final long serialVersionUID = -2398298479089615621L;
public final int errorCode;
public SQLiteException(int errcode, String msg) {
super(msg);
errorCode = errcode;
}
public SQLiteException(String msg) {
this(0, msg);
}
public SQLiteException() {
errorCode = 0;
}
}
3. 编写 JNI 类
SqliteWrapper.cpp:
#include "sqlite3.h"
#include <jni.h>
void throw_sqlite3_exception(JNIEnv *env, sqlite3 *handle, int errcode) {
if (SQLITE_OK == errcode) {
errcode = sqlite3_errcode(handle);
}
const char *errmsg = sqlite3_errmsg(handle);
jclass exClass = env->FindClass("io/kzw/sqlite3native/SQLiteException");
env->ThrowNew(exClass, errmsg);
}
extern "C" {
int Java_io_kzw_sqlite3native_SQLitePreparedStatement_step(JNIEnv *env, jobject object, int statementHandle) {
sqlite3_stmt *handle = (sqlite3_stmt *) statementHandle;
int errcode = sqlite3_step(handle);
if (errcode == SQLITE_ROW) {
return 0;
} else if(errcode == SQLITE_DONE) {
return 1;
} else if(errcode == SQLITE_BUSY) {
return -1;
}
throw_sqlite3_exception(env, sqlite3_db_handle(handle), errcode);
}
int Java_io_kzw_sqlite3native_SQLitePreparedStatement_prepare(JNIEnv *env, jobject object, int sqliteHandle, jstring sql) {
sqlite3 *handle = (sqlite3 *) sqliteHandle;
char const *sqlStr = env->GetStringUTFChars(sql, 0);
sqlite3_stmt *stmt_handle;
int errcode = sqlite3_prepare_v2(handle, sqlStr, -1, &stmt_handle, 0);
if (SQLITE_OK != errcode) {
throw_sqlite3_exception(env, handle, errcode);
}
if (sqlStr != 0) {
env->ReleaseStringUTFChars(sql, sqlStr);
}
return (int) stmt_handle;
}
void Java_io_kzw_sqlite3native_SQLitePreparedStatement_reset(JNIEnv *env, jobject object, int statementHandle) {
sqlite3_stmt *handle = (sqlite3_stmt *) statementHandle;
int errcode = sqlite3_reset(handle);
if (SQLITE_OK != errcode) {
throw_sqlite3_exception(env, sqlite3_db_handle(handle), errcode);
}
}
void Java_io_kzw_sqlite3native_SQLitePreparedStatement_finalize(JNIEnv *env, jobject object, int statementHandle) {
sqlite3_finalize((sqlite3_stmt *) statementHandle);
}
void Java_io_kzw_sqlite3native_SQLitePreparedStatement_bindByteBuffer(JNIEnv *env, jobject object, int statementHandle, int index, jobject value, int length) {
sqlite3_stmt *handle = (sqlite3_stmt *) statementHandle;
void *buf = env->GetDirectBufferAddress(value);
int errcode = sqlite3_bind_blob(handle, index, buf, length, SQLITE_STATIC);
if (SQLITE_OK != errcode) {
throw_sqlite3_exception(env, sqlite3_db_handle(handle), errcode);
}
}
void Java_io_kzw_sqlite3native_SQLitePreparedStatement_bindString(JNIEnv *env, jobject object, int statementHandle, int index, jstring value) {
sqlite3_stmt *handle = (sqlite3_stmt *) statementHandle;
char const *valueStr = env->GetStringUTFChars(value, 0);
int errcode = sqlite3_bind_text(handle, index, valueStr, -1, SQLITE_TRANSIENT);
if (SQLITE_OK != errcode) {
throw_sqlite3_exception(env, sqlite3_db_handle(handle), errcode);
}
if (valueStr != 0) {
env->ReleaseStringUTFChars(value, valueStr);
}
}
void Java_io_kzw_sqlite3native_SQLitePreparedStatement_bindInt(JNIEnv *env, jobject object, int statementHandle, int index, int value) {
sqlite3_stmt *handle = (sqlite3_stmt *) statementHandle;
int errcode = sqlite3_bind_int(handle, index, value);
if (SQLITE_OK != errcode) {
throw_sqlite3_exception(env, sqlite3_db_handle(handle), errcode);
}
}
void Java_io_kzw_sqlite3native_SQLitePreparedStatement_bindLong(JNIEnv *env, jobject object, int statementHandle, int index, long long value) {
sqlite3_stmt *handle = (sqlite3_stmt *) statementHandle;
int errcode = sqlite3_bind_int64(handle, index, value);
if (SQLITE_OK != errcode) {
throw_sqlite3_exception(env, sqlite3_db_handle(handle), errcode);
}
}
void Java_io_kzw_sqlite3native_SQLitePreparedStatement_bindDouble(JNIEnv *env, jobject object, int statementHandle, int index, double value) {
sqlite3_stmt *handle = (sqlite3_stmt *) statementHandle;
int errcode = sqlite3_bind_double(handle, index, value);
if (SQLITE_OK != errcode) {
throw_sqlite3_exception(env, sqlite3_db_handle(handle), errcode);
}
}
void Java_io_kzw_sqlite3native_SQLitePreparedStatement_bindNull(JNIEnv *env, jobject object, int statementHandle, int index) {
sqlite3_stmt *handle = (sqlite3_stmt *) statementHandle;
int errcode = sqlite3_bind_null(handle, index);
if (SQLITE_OK != errcode) {
throw_sqlite3_exception(env, sqlite3_db_handle(handle), errcode);
}
}
void Java_io_kzw_sqlite3native_SQLiteDatabase_closedb(JNIEnv *env, jobject object, int sqliteHandle) {
sqlite3 *handle = (sqlite3 *)sqliteHandle;
int err = sqlite3_close(handle);
if (SQLITE_OK != err) {
throw_sqlite3_exception(env, handle, err);
}
}
void Java_io_kzw_sqlite3native_SQLiteDatabase_beginTransaction(JNIEnv *env, jobject object, int sqliteHandle) {
sqlite3 *handle = (sqlite3 *)sqliteHandle;
sqlite3_exec(handle, "BEGIN", 0, 0, 0);
}
void Java__io_kzw_sqlite3native_SQLiteDatabase_commitTransaction(JNIEnv *env, jobject object, int sqliteHandle) {
sqlite3 *handle = (sqlite3 *)sqliteHandle;
sqlite3_exec(handle, "COMMIT", 0, 0, 0);
}
int Java_io_kzw_sqlite3native_SQLiteDatabase_opendb(JNIEnv *env, jobject object, jstring fileName, jstring tempDir) {
char const *fileNameStr = env->GetStringUTFChars(fileName, 0);
char const *tempDirStr = env->GetStringUTFChars(tempDir, 0);
if (sqlite3_temp_directory != 0) {
sqlite3_free(sqlite3_temp_directory);
}
sqlite3_temp_directory = sqlite3_mprintf("%s", tempDirStr);
sqlite3 *handle = 0;
int err = sqlite3_open(fileNameStr, &handle);
if (SQLITE_OK != err) {
throw_sqlite3_exception(env, handle, err);
}
if (fileNameStr != 0) {
env->ReleaseStringUTFChars(fileName, fileNameStr);
}
if (tempDirStr != 0) {
env->ReleaseStringUTFChars(tempDir, tempDirStr);
}
return (int)handle;
}
int Java_io_kzw_sqlite3native_SQLiteCursor_columnType(JNIEnv *env, jobject object, int statementHandle, int columnIndex) {
sqlite3_stmt *handle = (sqlite3_stmt *)statementHandle;
return sqlite3_column_type(handle, columnIndex);
}
int Java_io_kzw_sqlite3native_SQLiteCursor_columnIsNull(JNIEnv *env, jobject object, int statementHandle, int columnIndex) {
sqlite3_stmt *handle = (sqlite3_stmt *)statementHandle;
int valType = sqlite3_column_type(handle, columnIndex);
return SQLITE_NULL == valType;
}
int Java_io_kzw_sqlite3native_SQLiteCursor_columnIntValue(JNIEnv *env, jobject object, int statementHandle, int columnIndex) {
sqlite3_stmt *handle = (sqlite3_stmt *)statementHandle;
int valType = sqlite3_column_type(handle, columnIndex);
if (SQLITE_NULL == valType) {
return 0;
}
return sqlite3_column_int(handle, columnIndex);
}
long long Java_io_kzw_sqlite3native_SQLiteCursor_columnLongValue(JNIEnv *env, jobject object, int statementHandle, int columnIndex) {
sqlite3_stmt *handle = (sqlite3_stmt *)statementHandle;
int valType = sqlite3_column_type(handle, columnIndex);
if (SQLITE_NULL == valType) {
return 0;
}
return sqlite3_column_int64(handle, columnIndex);
}
double Java_io_kzw_sqlite3native_SQLiteCursor_columnDoubleValue(JNIEnv *env, jobject object, int statementHandle, int columnIndex) {
sqlite3_stmt *handle = (sqlite3_stmt *)statementHandle;
int valType = sqlite3_column_type(handle, columnIndex);
if (SQLITE_NULL == valType) {
return 0;
}
return sqlite3_column_double(handle, columnIndex);
}
jstring Java_io_kzw_sqlite3native_SQLiteCursor_columnStringValue(JNIEnv *env, jobject object, int statementHandle, int columnIndex) {
sqlite3_stmt *handle = (sqlite3_stmt *)statementHandle;
const char *str = (const char *) sqlite3_column_text(handle, columnIndex);
if (str != 0) {
return env->NewStringUTF(str);
}
return 0;
}
jbyteArray Java_io_kzw_sqlite3native_SQLiteCursor_columnByteArrayValue(JNIEnv *env, jobject object, int statementHandle, int columnIndex) {
sqlite3_stmt *handle = (sqlite3_stmt *)statementHandle;
const jbyte *buf = (const jbyte *) sqlite3_column_blob(handle, columnIndex);
int length = sqlite3_column_bytes(handle, columnIndex);
if (buf != nullptr && length > 0) {
jbyteArray result = env->NewByteArray(length);
env->SetByteArrayRegion(result, 0, length, buf);
return result;
}
return nullptr;
}
}
4. Android.mk 和 Application.mk
Android.mk:
LOCAL_PATH := $(call my-dir)
ifeq ($(TARGET_ARCH_ABI),armeabi)
LOCAL_ARM_MODE := thumb
else
LOCAL_ARM_MODE := arm
endif
LOCAL_MODULE := sqlite3
LOCAL_CFLAGS := -w -std=c11 -Os -DNULL=0 -DSOCKLEN_T=socklen_t -DLOCALE_NOT_USED -D_LARGEFILE_SOURCE=1 -D_FILE_OFFSET_BITS=32
LOCAL_CFLAGS += -DANDROID_NDK -DDISABLE_IMPORTGL -fno-strict-aliasing -fprefetch-loop-arrays -DAVOID_TABLES -DANDROID_TILE_BASED_DECODE -DANDROID_ARMV6_IDCT -DHAVE_STRCHRNUL=0
LOCAL_SRC_FILES := \
./sqlite3.c
include $(BUILD_STATIC_LIBRARY)
include $(CLEAR_VARS)
LOCAL_MODULE := sqlite_wrapper
LOCAL_CPPFLAGS := -std=c++11
LOCAL_LDLIBS := -llog -lz
LOCAL_STATIC_LIBRARIES := sqlite3
LOCAL_SRC_FILES += \
./SqliteWrapper.cpp \
include $(BUILD_SHARED_LIBRARY)
这边编译的是 armeabi 和 armeabi-v7a 平台,先编译 sqlite3 静态库,然后作为 sqlite_wrapper 共享库的依赖库。由于我使用的是 NDK r15 编译的,需要设置 -D_FILE_OFFSET_BITS=32,否则会报错:mmap unknown identifier,具体可以看这篇文章:https://github.com/couchbaselabs/couchbase-lite-libsqlcipher/pull/23
Application.mk:
APP_PLATFORM := android-14
APP_ABI := armeabi armeabi-v7a
NDK_TOOLCHAIN_VERSION := 4.9
APP_STL := gnustl_static
5. 编译生成 so 库
MacBook-Pro-3:jni kuangzhongwen$ ndk-build
[armeabi] Compile++ thumb: sqlite_wrapper <= SqliteWrapper.cpp
[armeabi] Compile thumb : sqlite3 <= sqlite3.c
[armeabi] StaticLibrary : libgnustl_shared.a
[armeabi] SharedLibrary : libsqlite_wrapper.so
[armeabi] Install : libsqlite_wrapper.so => libs/armeabi/libsqlite_wrapper.so
[armeabi-v7a] Compile++ thumb: sqlite_wrapper <= SqliteWrapper.cpp
ndk-build,可以看到依次生成了两个平台的 so 库,都是先打出 .a 静态库,再打出 so 共享库,最终 so 库会打包进 apk 中。
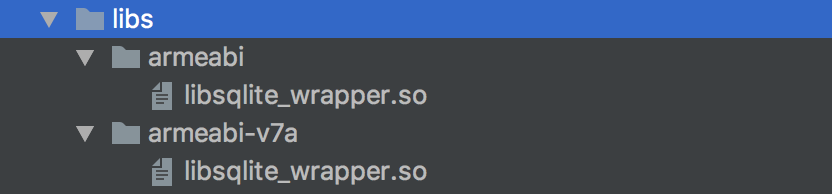
使用时加载库即可:
static {
System.loadLibrary("sqlite_wrapper");
}
时间有限,就不写 demo 运行了,感兴趣的同学可以自行编译运行一下。明天开始,将开写 40-50 篇的 Framework 文章,也是对自己的一个查漏补缺。
本文内容由网友自发贡献,版权归原作者所有,本站不承担相应法律责任。如您发现有涉嫌抄袭侵权的内容,请联系:hwhale#tublm.com(使用前将#替换为@)