源码地址
4.1)通过ServletAPI获取
将HttpServletRequest作为控制器方法的形参,此时HttpServletRequest类型的参数表示封装了当前请求的请求报文的对象,代码如下:
@Controller
public class ParamController {
@RequestMapping("/testServletAPI")
//形参位置的request表示当前请求
public String testServletAPI(HttpServletRequest request){
HttpSession session = request.getSession();
String username = request.getParameter("username");
String password = request.getParameter("password");
System.out.println("username:"+username+",password:"+password);
return "success";
}
}
新建 test_param.html ,代码如下:
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>测试请求参数</title>
</head>
<body>
<h1>测试请求参数</h1>
<a th:href="@{/testServletAPI(username='admin',password=123456)}">1)测试使用servletAPI获取请求参数</a><br>
</body>
</html>
输出:【配置Tomacat后启动工程,浏览器访问:http://localhost:8080/SpringMvcDemo2/param 】页面如下:
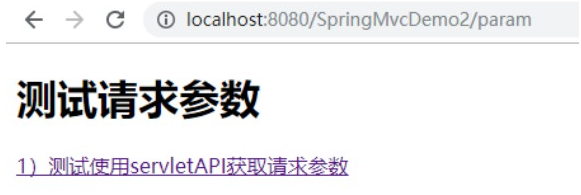
点击链接1,页面跳转至:http://localhost:8080/SpringMvcDemo2/testServletAPI?username=admin&password=123456,页面如下:

控制台输出参数:
14:14:25.454 [http-nio-8080-exec-7] DEBUG org.springframework.web.servlet.DispatcherServlet - GET "/SpringMvcDemo2/testServletAPI?username=admin&password=123456", parameters={masked}
14:14:25.455 [http-nio-8080-exec-7] DEBUG org.springframework.web.servlet.mvc.method.annotation.RequestMappingHandlerMapping - Mapped to com.study.mvc.controller.ParamController#testServletAPI(HttpServletRequest)
username:admin,password:123456
14:14:25.514 [http-nio-8080-exec-7] DEBUG org.springframework.web.servlet.DispatcherServlet - Completed 200 OK
4.2)通过控制器方法的形参获取请求参数
在控制器方法的形参位置,设置和请求参数同名的形参,当浏览器发送请求,匹配到请求映射时,在DispatcherServlet中就会将请求参数赋值给相应的形参,代码如下:
@Controller
public class ParamController {
@RequestMapping("/testParam")
public String testParam(String username, String password, String[] hobby) {
//若请求参数中出现多个同名的请求参数,可以再控制器方法的形参位置设置字符串类型或字符串数组接收此请求参数
//若使用字符串类型的形参,最终结果为请求参数的每一个值之间使用逗号进行拼接
System.out.println("username:" + username + ",password:" + password + ",hobby:" + Arrays.toString(hobby));
return "success";
}
}
修改 test_param.html ,代码如下:
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>测试请求参数</title>
</head>
<body>
<h1>测试请求参数</h1>
<a th:href="@{/testServletAPI(username='admin',password=123456)}">1)测试使用servletAPI获取请求参数</a><br>
<a th:href="@{/testParam(username='admin',password=123456)}">2)测试使用控制器的形参获取请求参数</a><br>
<form th:action="@{/testParam}" method="get">
用户名:<input type="text" name="user_name"><br>
密码:<input type="password" name="password"><br>
爱好:<input type="checkbox" name="hobby" value="a">a
<input type="checkbox" name="hobby" value="b">b
<input type="checkbox" name="hobby" value="c">c<br>
<input type="submit" value="测试使用控制器的形参获取请求参数">
</form>
</body>
</html>
输出:【配置Tomacat后启动工程,浏览器访问:http://localhost:8080/SpringMvcDemo2/param 】,输入相关信息,点击“测试使用控制器的形参获取请求参数”,页面跳转至:http://localhost:8080/SpringMvcDemo2/testParam?user_name=admin1&password=654321&hobby=a&hobby=c,页面如下:
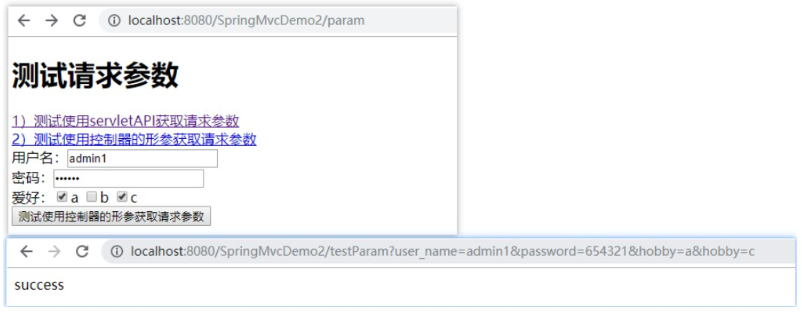
控制台输出参数:
14:19:08.688 [http-nio-8080-exec-4] DEBUG org.springframework.web.servlet.DispatcherServlet - GET "/SpringMvcDemo2/testParam?user_name=admin1&password=654321&hobby=a&hobby=c", parameters={masked}
14:19:08.689 [http-nio-8080-exec-4] DEBUG org.springframework.web.servlet.mvc.method.annotation.RequestMappingHandlerMapping - Mapped to com.study.mvc.controller.ParamController#testParam(String, String, String[])
username:null,password:654321,hobby:[a, c]
14:19:08.690 [http-nio-8080-exec-4] DEBUG org.springframework.web.servlet.DispatcherServlet - Completed 200 OK
说明:【请求参数与页面参数名称不一致则无法获取到该参数】
若请求所传输的请求参数中有多个同名的请求参数,此时可以在控制器方法的形参中设置字符串数组或者字符串类型的形参接收此请求参数;
若使用字符串数组类型的形参,此参数的数组中包含了每一个数据;
若使用字符串类型的形参,此参数的值为每个数据中间使用逗号拼接的结果;
4.3)@RequestParam
@RequestParam注解是将请求参数和控制器方法的形参创建映射关系,一共有三个属性:
-
value:指定为形参赋值的请求参数的参数名;
-
required:设置是否必须传输此请求参数,默认值为true;
若设置为true时,则当前请求必须传输value所指定的请求参数,若没有传输该请求参数,且没有设置defaultValue属性,则页面报错400:Required String parameter 'xxx' is not present;
若设置为false,则当前请求不是必须传输value所指定的请求参数,若没有传输,则注解所标识的形参的值为null
-
defaultValue:不管required属性值为true或false,当value所指定的请求参数没有传输或传输的值为""时,则使用默认值为形参赋值;
代码如下:
@RequestMapping("/requestParam")
public String requestParam(
@RequestParam(value = "user_name", required = false, defaultValue = "hehe") String username,
String password,
String[] hobby){
//若请求参数中出现多个同名的请求参数,可以再控制器方法的形参位置设置字符串类型或字符串数组接收此请求参数
//若使用字符串类型的形参,最终结果为请求参数的每一个值之间使用逗号进行拼接
System.out.println("username:"+username+",password:"+password+",hobby:"+ Arrays.toString(hobby));
return "success";
}
修改 test_param.html ,代码如下:
<form th:action="@{/requestParam}" method="get">
用户名:<input type="text" name="user_name"><br>
密码:<input type="password" name="password"><br>
爱好:<input type="checkbox" name="hobby" value="a">a
<input type="checkbox" name="hobby" value="b">b
<input type="checkbox" name="hobby" value="c">c<br>
<input type="submit" value="测试控制器的RequestParam注解">
</form>
【配置Tomacat后启动工程,浏览器访问:http://localhost:8080/SpringMvcDemo2/param 】,输入相关信息,点击“测试控制器的RequestParam注解”,页面跳转至:http://localhost:8080/SpringMvcDemo2/requestParam?user_name=&password=98765&hobby=b&hobby=c,页面如下:
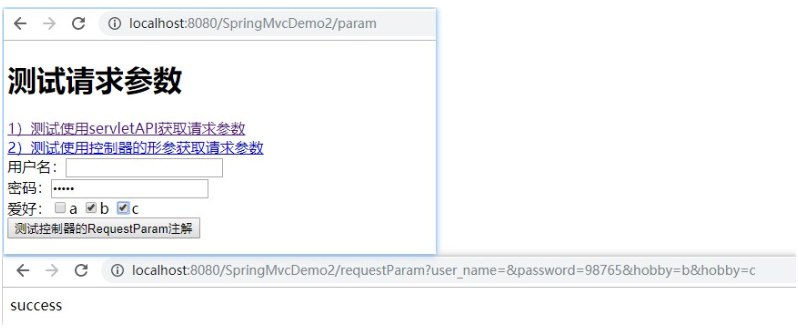
控制台输出参数:【页面没有传送user_name参数则取默认值 username:hehe】
14:32:52.988 [http-nio-8080-exec-8] DEBUG org.springframework.web.servlet.DispatcherServlet - GET "/SpringMvcDemo2/requestParam?user_name=&password=98765&hobby=b&hobby=c", parameters={masked}
14:32:52.989 [http-nio-8080-exec-8] DEBUG org.springframework.web.servlet.mvc.method.annotation.RequestMappingHandlerMapping - Mapped to com.study.mvc.controller.ParamController#requestParam(String, String, String[])
username:hehe,password:98765,hobby:[b, c]
14:32:53.067 [http-nio-8080-exec-8] DEBUG org.springframework.web.servlet.DispatcherServlet - Completed 200 OK
4.4)@RequestHeader
@RequestHeader是将请求头信息和控制器方法的形参创建映射关系
@RequestHeader注解一共有三个属性:value、required、defaultValue,用法同@RequestParam
代码如下:
@RequestMapping("/requestHeader")
public String RequestHeader(
@RequestParam(value = "user_name", required = false, defaultValue = "hehe") String username,
String password,
String[] hobby,
@RequestHeader(value = "sayHaha", required = true, defaultValue = "haha") String host) {
//若请求参数中出现多个同名的请求参数,可以再控制器方法的形参位置设置字符串类型或字符串数组接收此请求参数
//若使用字符串类型的形参,最终结果为请求参数的每一个值之间使用逗号进行拼接
System.out.println("username:" + username + ",password:" + password + ",hobby:" + Arrays.toString(hobby));
System.out.println("host:" + host);
return "success";
}
修改 test_param.html ,代码如下:
<form th:action="@{/requestHeader}" method="get">
用户名:<input type="text" name="user_name"><br>
密码:<input type="password" name="password"><br>
爱好:<input type="checkbox" name="hobby" value="a">a
<input type="checkbox" name="hobby" value="b">b
<input type="checkbox" name="hobby" value="c">c<br>
<input type="submit" value="测试控制器的RequestHeader注解">
</form>
【配置Tomacat后启动工程,浏览器访问:http://localhost:8080/SpringMvcDemo2/param 】,输入相关信息,点击“测试控制器的RequestParam注解”,页面跳转至:http://localhost:8080/SpringMvcDemo2/requestParam?user_name=&password=98765&hobby=b&hobby=c,页面如下:
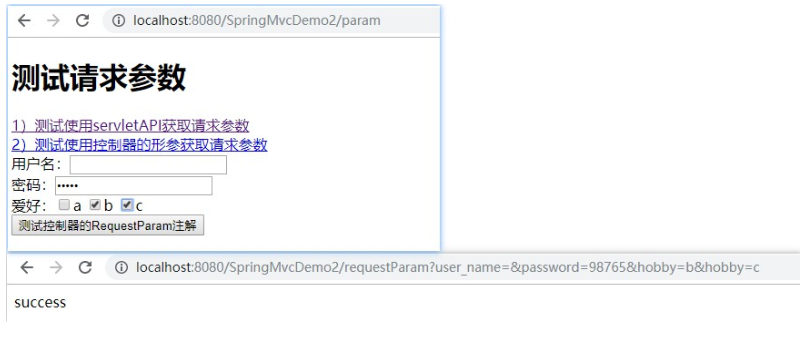
控制台输出参数:【请求头信息没有传送host参数则取默认值 host:haha】
14:55:54.096 [http-nio-8080-exec-5] DEBUG org.springframework.web.servlet.DispatcherServlet - GET "/SpringMvcDemo2/requestHeader?user_name=&password=98765&hobby=b&hobby=c", parameters={masked}
14:55:54.096 [http-nio-8080-exec-5] DEBUG org.springframework.web.servlet.mvc.method.annotation.RequestMappingHandlerMapping - Mapped to com.study.mvc.controller.ParamController#RequestHeader(String, String, String[], String)
username:hehe,password:98765,hobby:[b, c]
host:haha
14:55:54.099 [http-nio-8080-exec-5] DEBUG org.springframework.web.servlet.DispatcherServlet - Completed 200 OK
4.5)@CookieValue
@CookieValue是将cookie数据和控制器方法的形参创建映射关系
@CookieValue注解一共有三个属性:value、required、defaultValue,用法同@RequestParam
代码如下:
@RequestMapping("/cookieValue")
public String CookieValue(
@RequestParam(value = "user_name", required = false, defaultValue = "hehe") String username,
String password,
String[] hobby,
@RequestHeader(value = "sayHaha", required = true, defaultValue = "haha") String host,
@CookieValue("JSESSIONID") String JSESSIONID) {
//若请求参数中出现多个同名的请求参数,可以再控制器方法的形参位置设置字符串类型或字符串数组接收此请求参数
//若使用字符串类型的形参,最终结果为请求参数的每一个值之间使用逗号进行拼接
System.out.println("username:" + username + ",password:" + password + ",hobby:" + Arrays.toString(hobby));
System.out.println("host:" + host);
System.out.println("JSESSIONID:"+JSESSIONID);
return "success";
}
修改 test_param.html ,代码如下:
<form th:action="@{/cookieValue}" method="get">
用户名:<input type="text" name="user_name"><br>
密码:<input type="password" name="password"><br>
爱好:<input type="checkbox" name="hobby" value="a">a
<input type="checkbox" name="hobby" value="b">b
<input type="checkbox" name="hobby" value="c">c<br>
<input type="submit" value="测试控制器的cookieValue注解">
</form>
【配置Tomacat后启动工程,浏览器访问:http://localhost:8080/SpringMvcDemo2/param 】,输入相关信息,点击“测试控制器的RequestParam注解”,页面跳转至:http://localhost:8080/SpringMvcDemo2/requestParam?user_name=&password=98765&hobby=b&hobby=c,页面如下:
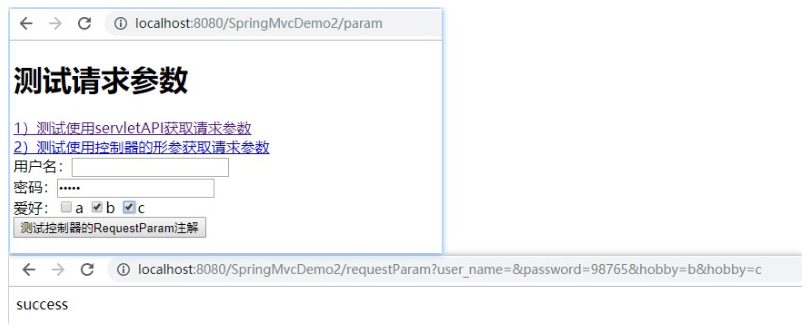
控制台输出参数:【cookie数据中取值--JSESSIONID:81755E5DE0D3E5631DC7C7A3EE0C9C79】
15:25:43.432 [http-nio-8080-exec-8] DEBUG org.springframework.web.servlet.DispatcherServlet - GET "/SpringMvcDemo2/cookieValue?user_name=&password=98765&hobby=b&hobby=c", parameters={masked}
15:25:43.432 [http-nio-8080-exec-8] DEBUG org.springframework.web.servlet.mvc.method.annotation.RequestMappingHandlerMapping - Mapped to com.study.mvc.controller.ParamController#CookieValue(String, String, String[], String, String)
username:hehe,password:98765,hobby:[b, c]
host:haha
JSESSIONID:81755E5DE0D3E5631DC7C7A3EE0C9C79
15:25:43.508 [http-nio-8080-exec-8] DEBUG org.springframework.web.servlet.DispatcherServlet - Completed 200 OK
4.6)通过POJO获取请求参数
可以在控制器方法的形参位置设置一个实体类类型的形参,此时若浏览器传输的请求参数的参数名和实体类中的属性名一致,那么请求参数就会为此属性赋值。代码如下:
// 通过POJO获取请求参数
@RequestMapping("/testBean")
public String testBean(User user){
System.out.println(user);
return "success";
}
修改 test_param.html ,代码如下:
<form th:action="@{/testBean}" method="post">
用户名:<input type="text" name="username"><br>
密码:<input type="password" name="password"><br>
性别:<input type="radio" name="sex" value="男">男<input type="radio" name="sex" value="女">女<br>
年龄:<input type="text" name="age"><br>
邮箱:<input type="text" name="email"><br>
<input type="submit" value="使用实体类接收请求参数">
</form>
新增 User.java,代码如下:
public class User {
private Integer id;
private String username;
private String password;
private Integer age;
private String sex;
private String email;
public User() {
}
public User(Integer id, String username, String password, Integer age, String sex, String email) {
this.id = id;
this.username = username;
this.password = password;
this.age = age;
this.sex = sex;
this.email = email;
}
@Override
public String toString() {
return "User{" +
"id=" + id +
", username='" + username + '\'' +
", password='" + password + '\'' +
", age=" + age +
", sex='" + sex + '\'' +
", email='" + email + '\'' +
'}';
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
public String getSex() {
return sex;
}
public void setSex(String sex) {
this.sex = sex;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
}
【配置Tomacat后启动工程,浏览器访问:http://localhost:8080/SpringMvcDemo2/param 】,输入相关信息,点击“使用实体类接收请求参数”,页面跳转至:http://localhost:8080/SpringMvcDemo2/testBean,页面如下:
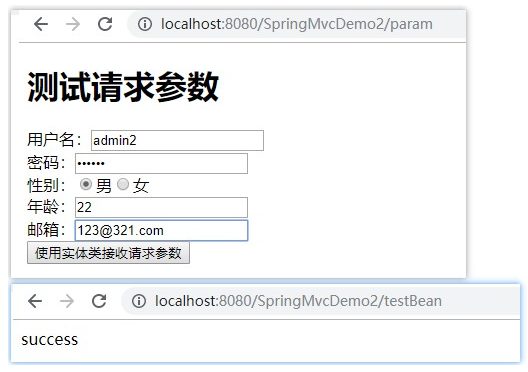
控制台输出参数:【通过实体类对象成功获取到参数,但存在乱码情况】
15:37:35.487 [http-nio-8080-exec-10] DEBUG org.springframework.web.servlet.mvc.method.annotation.RequestMappingHandlerMapping - Mapped to com.study.mvc.controller.ParamController#testBean(User)
User{id=null, username='admin2', password='654377', age=22, sex='??·', email='123@321.com'}
15:37:35.556 [http-nio-8080-exec-10] DEBUG org.springframework.web.servlet.DispatcherServlet - Completed 200 OK
4.6.1)解决获取请求参数的乱码问题
4.6.1.1)get方法
修改上述 test_param.html 中的方法为get,代码如下:
<form th:action="@{/testBean}" method="get">
用户名:<input type="text" name="username"><br>
密码:<input type="password" name="password"><br>
性别:<input type="radio" name="sex" value="男">男<input type="radio" name="sex" value="女">女<br>
年龄:<input type="text" name="age"><br>
邮箱:<input type="text" name="email"><br>
<input type="submit" value="使用实体类接收请求参数">
</form>
修改本地Tomcat的配置文件:【添加URIEncoding="UTF-8"】
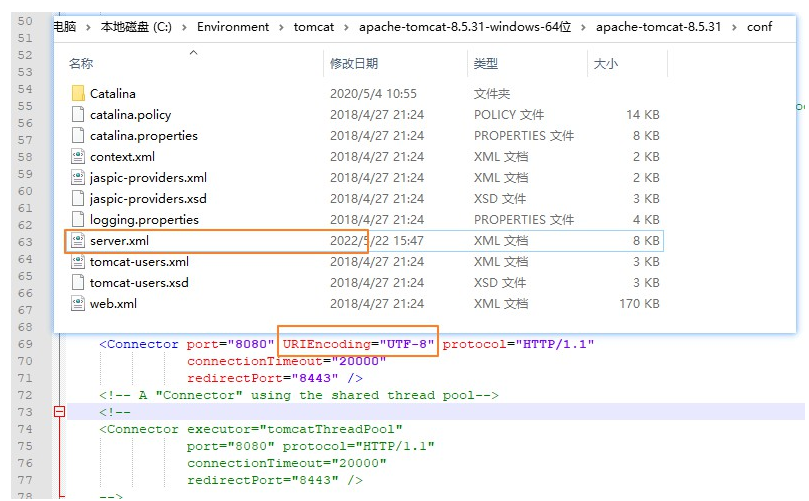
再次重启服务,控制台输出参数:【通过实体类对象成功获取到参数,不存在乱码情况】
15:51:10.102 [http-nio-8080-exec-10] DEBUG org.springframework.web.servlet.DispatcherServlet - GET "/SpringMvcDemo2/testBean?username=admin3&password=325634&sex=%E7%94%B7&age=15&email=123%40321.com", parameters={masked}
15:51:10.103 [http-nio-8080-exec-10] DEBUG org.springframework.web.servlet.mvc.method.annotation.RequestMappingHandlerMapping - Mapped to com.study.mvc.controller.ParamController#testBean(User)
User{id=null, username='admin3', password='325634', age=15, sex='男', email='123@321.com'}
15:51:10.171 [http-nio-8080-exec-10] DEBUG org.springframework.web.servlet.DispatcherServlet - Completed 200 OK
4.6.1.2)post方法
解决获取请求参数的乱码问题,可以使用SpringMVC提供的编码过滤器CharacterEncodingFilter,但是必须在web.xml中进行注册,修改web.xml代码如下:
<!-- 编码过滤器CharacterEncodingFilter解决乱码问题,filter 会在 servlet之前加载-->
<filter>
<filter-name>CharacterEncodingFilter</filter-name>
<filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class>
<init-param>
<param-name>encoding</param-name>
<param-value>UTF-8</param-value>
</init-param>
<init-param>
<param-name>forceResponseEncoding</param-name>
<param-value>true</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>CharacterEncodingFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
再次重启服务,控制台输出参数:【通过实体类对象成功获取到参数,不存在乱码情况】
16:03:41.682 [http-nio-8080-exec-8] DEBUG org.springframework.web.servlet.DispatcherServlet - POST "/SpringMvcDemo2/testBean", parameters={masked}
16:03:41.682 [http-nio-8080-exec-8] DEBUG org.springframework.web.servlet.mvc.method.annotation.RequestMappingHandlerMapping - Mapped to com.study.mvc.controller.ParamController#testBean(User)
User{id=null, username='admin3', password='543678', age=23, sex='男', email='123@435.com'}
16:03:41.753 [http-nio-8080-exec-8] DEBUG org.springframework.web.servlet.DispatcherServlet - Completed 200 OK
本文内容由网友自发贡献,版权归原作者所有,本站不承担相应法律责任。如您发现有涉嫌抄袭侵权的内容,请联系:hwhale#tublm.com(使用前将#替换为@)