import org.apache.commons.codec.binary.Base32;
import org.apache.commons.codec.binary.Base64;
import javax.crypto.Mac;
import javax.crypto.spec.SecretKeySpec;
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
import java.security.SecureRandom;
/**
* google身份验证器,java服务端实现
*/
public class GoogleAuthenticator {
// 生成的key长度( Generate secret key length)
public static final int SECRET_SIZE = 10;
public static final String SEED = "g8GjEvTbW5oVSV7avL47357438reyhreyuryetredLDVKs2m0QN7vxRs2im5MDaNCWGmcD2rvcZx";
// Java实现随机数算法
public static final String RANDOM_NUMBER_ALGORITHM = "SHA1PRNG";
// 最多可偏移的时间
int window_size = 3; // default 3 - max 17
/**
* set the windows size. This is an integer value representing the number of
* 30 second windows we allow The bigger the window, the more tolerant of
* clock skew we are.
*
* @param s
* window size - must be >=1 and <=17. Other values are ignored
*/
public void setWindowSize(int s) {
if (s >= 1 && s <= 17)
window_size = s;
}
/**
* Generate a random secret key. This must be saved by the server and
* associated with the users account to verify the code displayed by Google
* Authenticator. The user must register this secret on their device.
* 生成一个随机秘钥
*
* @return secret key
*/
public static String generateSecretKey() {
SecureRandom sr = null;
try {
sr = SecureRandom.getInstance(RANDOM_NUMBER_ALGORITHM);
sr.setSeed(Base64.decodeBase64(SEED));
byte[] buffer = sr.generateSeed(SECRET_SIZE);
Base32 codec = new Base32();
byte[] bEncodedKey = codec.encode(buffer);
String encodedKey = new String(bEncodedKey);
return encodedKey;
} catch (NoSuchAlgorithmException e) {
// should never occur... configuration error
}
return null;
}
/**
* Return a URL that generates and displays a QR barcode. The user scans
* this bar code with the Google Authenticator application on their
* smartphone to register the auth code. They can also manually enter the
* secret if desired
*
* @param user
* user id (e.g. fflinstone)
* @param host
* host or system that the code is for (e.g. myapp.com)
* @param secret
* the secret that was previously generated for this user
* @return the URL for the QR code to scan
*/
public static String getQRBarcodeURL(String user, String host, String secret) {
String format = "http://www.google.com/chart?chs=200x200&chld=M%%7C0&cht=qr&chl=otpauth://totp/%s@%s?secret=%s";
return String.format(format, user, host, secret);
}
/**
* 生成一个google身份验证器,识别的字符串,只需要把该方法返回值生成二维码扫描就可以了。
*
* @param user
* 账号
* @param secret
* 密钥
* @return
*/
public static String getQRBarcode(String user, String secret) {
String format = "otpauth://totp/%s?secret=%s";
return String.format(format, user, secret);
}
/**
* Check the code entered by the user to see if it is valid 验证code是否合法
*
* @param secret
* The users secret.
* @param code
* The code displayed on the users device
* @param timeMsec
* The time in msec (System.currentTimeMillis() for example)
* @return
*/
public boolean check_code(String secret, long code, long timeMsec) {
Base32 codec = new Base32();
byte[] decodedKey = codec.decode(secret);
// convert unix msec time into a 30 second "window"
// this is per the TOTP spec (see the RFC for details)
long t = (timeMsec / 1000L) / 30L;
// Window is used to check codes generated in the near past.
// You can use this value to tune how far you're willing to go.
for (int i = -window_size; i <= window_size; ++i) {
long hash;
try {
hash = verify_code(decodedKey, t + i);
} catch (Exception e) {
// Yes, this is bad form - but
// the exceptions thrown would be rare and a static
// configuration problem
e.printStackTrace();
throw new RuntimeException(e.getMessage());
// return false;
}
if (hash == code) {
return true;
}
}
// The validation code is invalid.
return false;
}
private static int verify_code(byte[] key, long t) throws NoSuchAlgorithmException, InvalidKeyException {
byte[] data = new byte[8];
long value = t;
for (int i = 8; i-- > 0; value >>>= 8) {
data[i] = (byte) value;
}
SecretKeySpec signKey = new SecretKeySpec(key, "HmacSHA1");
Mac mac = Mac.getInstance("HmacSHA1");
mac.init(signKey);
byte[] hash = mac.doFinal(data);
int offset = hash[20 - 1] & 0xF;
// We're using a long because Java hasn't got unsigned int.
long truncatedHash = 0;
for (int i = 0; i < 4; ++i) {
truncatedHash <<= 8;
// We are dealing with signed bytes:
// we just keep the first byte.
truncatedHash |= (hash[offset + i] & 0xFF);
}
truncatedHash &= 0x7FFFFFFF;
truncatedHash %= 1000000;
return (int) truncatedHash;
}
}
测试类实现
import org.junit.Test;
import java.util.HashSet;
import java.util.Set;
public class AuthTest {
//当测试authTest时候,把genSecretTest生成的secret值赋值给它
private static String secret="BYJBZYGU2AO4ZKK7";
@Test
public void genSecretTest() {// 生成密钥
secret = GoogleAuthenticator.generateSecretKey();
// 把这个qrcode生成二维码,用google身份验证器扫描二维码就能添加成功
String qrcode = GoogleAuthenticator.getQRBarcode("2816661736@qq.com", secret);
System.out.println("qrcode:" + qrcode + ",key:" + secret);
}
/**
* 对app的随机生成的code,输入并验证
*/
@Test
public void verifyTest() {
long code = 936171;
long t = System.currentTimeMillis();
GoogleAuthenticator ga = new GoogleAuthenticator();
ga.setWindowSize(2);
boolean r = ga.check_code(secret, code, t);
System.out.println("检查code是否正确?" + r);
}
}
运行下面链接中下载的demo中的AuthTest的genSecretTest方法,控制台打印的结果如下图:
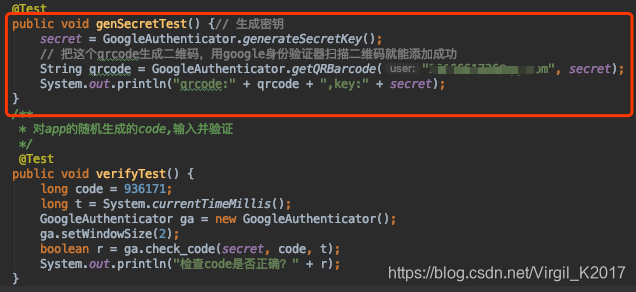
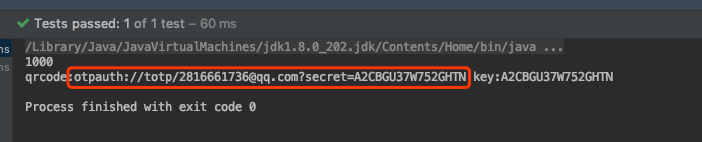
key:为app与服务端约定的秘钥,用于双方的认证。
qrcode:是app扫码能够识别的就是二维码值,把它生成二维码如下图:
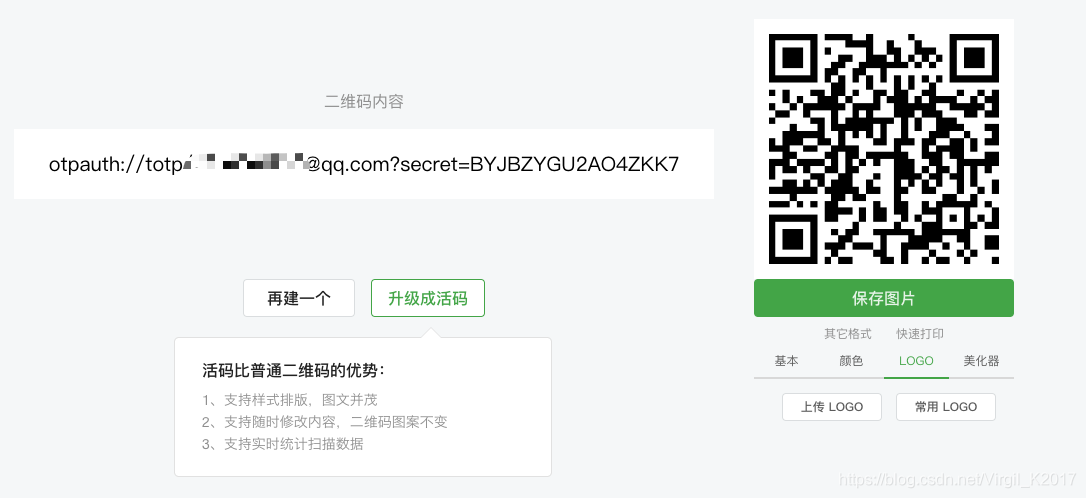
打开google authenticator app软件选择扫描条形码按扭打开相机对二维码扫描加入账号,如下图:
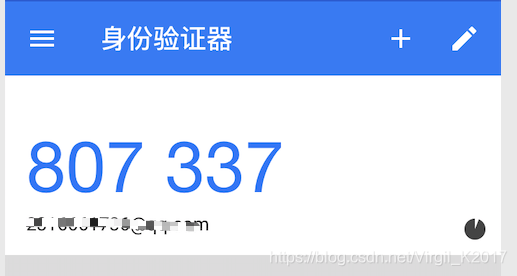
把app中的数字,在AuthTest的verifyTest进行验证,如下图:
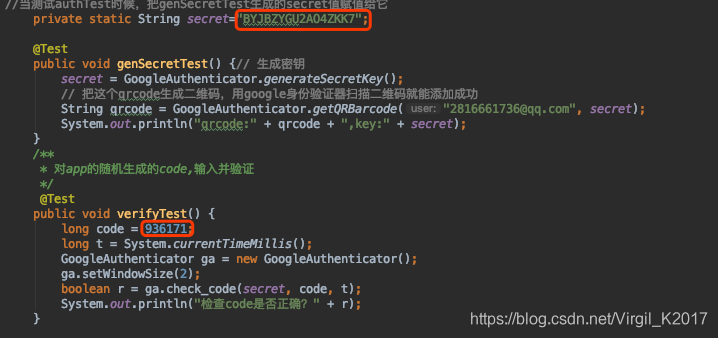

本文内容由网友自发贡献,版权归原作者所有,本站不承担相应法律责任。如您发现有涉嫌抄袭侵权的内容,请联系:hwhale#tublm.com(使用前将#替换为@)