这里写目录标题
- 一.昨日回顾
- 二.今日内容
- 1.跨域问题
-
- 2.头部组件,尾部组件
- components/Header.vue
- components/Footer.vue
- 3.首页组件,轮播图组件
- components/Banner.vue
- views/Home.vue
- views/ActualCourse.vue
- views/FreeCourse.vue
- views/LightCourse.vue
- App.vue
- router/index.js
- 4.git入门
一.昨日回顾
1 前端配置
-cnpm install jQuery --save
-vue create 项目名字(eslint不使用,vuex,router,bable)
-npm run serve
-npm run build
-每个组件都有三部分
-template
-script
-style
2 后端配置
-全局异常
-全局响应对象Response对象
-记录日志
3 跨域问题解决
-前端使用代理(什么叫反向代理,什么是正向代理)
-自己处理(CORS:跨资源共享)(csrf:跨站请求伪造,xss:跨站脚本攻击)
-借助第三方 django-cors-headers
-注册app
-写中间件
-一堆配置
二.今日内容
1.跨域问题
1 同源策略:浏览器的安全策略,不允许去另一个域加载数据
2 域:ip或者端口都必须一致
3 前后端分离项目会出现跨域
4 不使用第三方,自己处理
5 CORS:后端技术,跨域资源共享,服务端只要做配置,(本身浏览器已经支持了),就支持跨域
-access-control-allow-origin: *
6 浏览器将CORS请求分成两类:
-简单请求(simple request)
-非简单请求(not-so-simple request)
7 满足以下两大条件就是简单请求
(1)请求方法是以下三种方法之一:
HEAD
GET
POST
(2)HTTP的头信息不超出以下几种字段
Accept
Accept-Language
Content-Language
Last-Event-ID
Content-Type:只限于三个值application/x-www-form-urlencoded、multipart/form-data、text/plain
8 简单请求,只发送一次,如果后端写了CORS,浏览器就不禁止了
9 非简单请求,发两次,第一次是OPTIONS(预检请求),看后端是否允许,如果允许再发真正的请求
1.1后端自己处理跨域
简单请求
from django.http import JsonResponse
def test(request):
obj=JsonResponse({'name':'lqz','age':18})
obj['Access-Control-Allow-Origin']='*'
return obj
from django.urls import path
from user import views
urlpatterns = [
path('test/',views.test),
]
from django.shortcuts import render
def index(request):
return render(request, 'index.html')
from django.urls import path
from app01 import views
urlpatterns = [
path('test/', views.index),
]
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<title>Title</title>
</head>
<body>
<button id="btn">点我</button>
</body>
<script>
$('#btn').click(function(){
$.ajax({
url:'http://127.0.0.1:8000/user/test/',
method:'get',
success:function(data){
console.log(data)
}
})
})
</script>
</html>
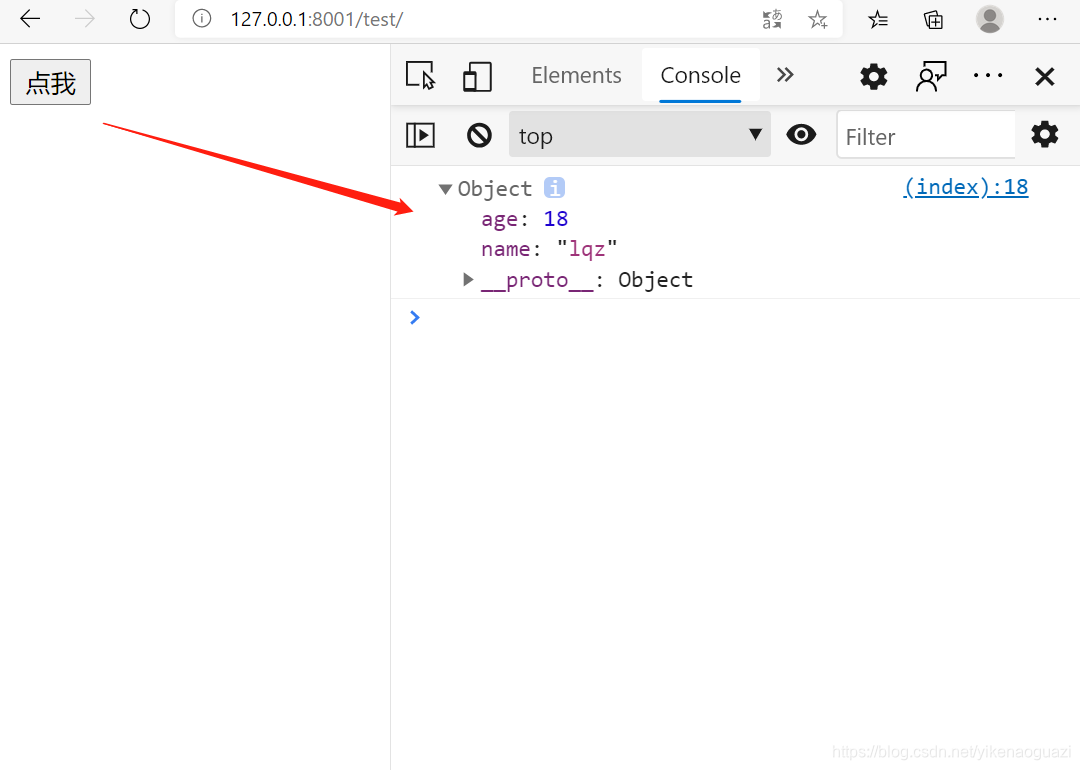
非简单请求
原django项目apps/user/views.py
from django.http import JsonResponse
def test(request):
obj = JsonResponse({'name': 'Lisa', 'age': '18'})
if request.method == 'OPTIONS':
obj['Access-Control-Allow-Headers'] = 'Content-Type,authorization'
obj['Access-Control-Allow-Origin'] = 'http://127.0.0.1:8001'
return obj
新django项目templates/index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
</head>
<body>
<button id="btn">点我</button>
</body>
<script>
$('#btn').click(function () {
let obj = {name: 'Lisa'}
$.ajax({
url: 'http://127.0.0.1:8000/user/test/',
method: 'post',
contentType: 'application/json',
headers: {authorization: 'jwt'},
data: JSON.stringify(obj),
success: function (data) {
console.log(data)
}
})
})
</script>
</html>
中间件处理
在原django项目的根路径创建middle.py
from django.utils.deprecation import MiddlewareMixin
class CoreMiddle(MiddlewareMixin):
def process_response(self, request, response):
if request.method == 'OPTIONS':
response['Access-Control-Allow-Headers'] = 'Content-Type,authorization'
response['Access-Control-Allow-Origin'] = '*'
return response
MIDDLEWARE = [
...
'middle.CoreMiddle',
...
]
from django.http import JsonResponse
def test(request):
return JsonResponse({'name': 'lqz', 'age': '18'})
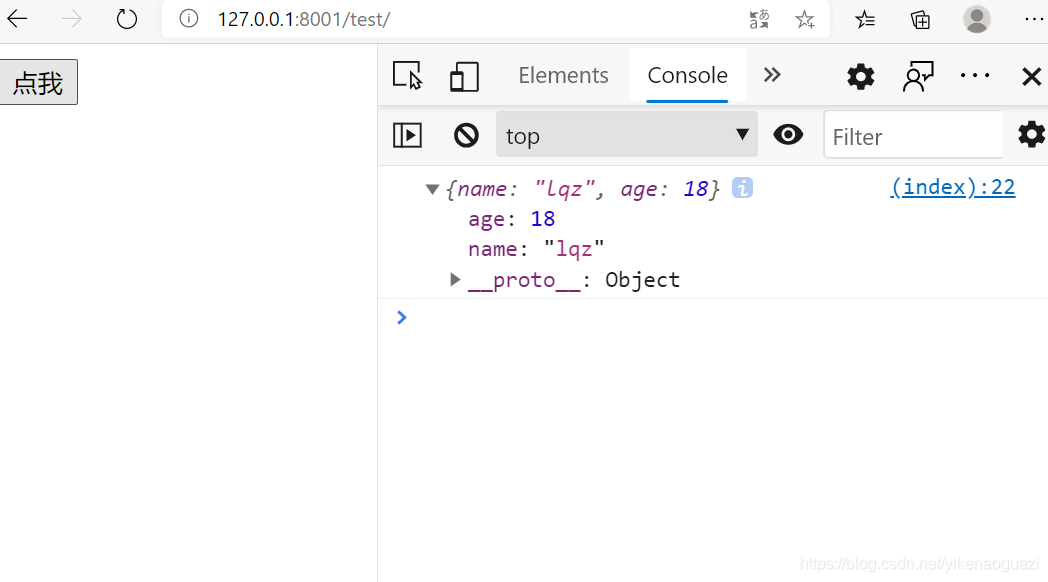
1.2前端处理跨域
App.vue
<template>
<div id="app">
<h1>我是主页</h1>
<div v-html="ret"></div>
</div>
</template>
<script>
export default {
data () {
return {
ret: ''
}
},
mounted () {
this.$axios.get('/asgard/app/video/').then(res => {
this.ret = res.data
})
}
}
</script>
vue.config.js
const webpack = require('webpack')
module.exports = {
devServer: {
proxy: {
'/asgard': {
target: 'https://m.maoyan.com/',
changeOrigin: true
},
'/user': {
target: 'http://127.0.0.1:8000/',
changeOrigin: true
}
}
}
}
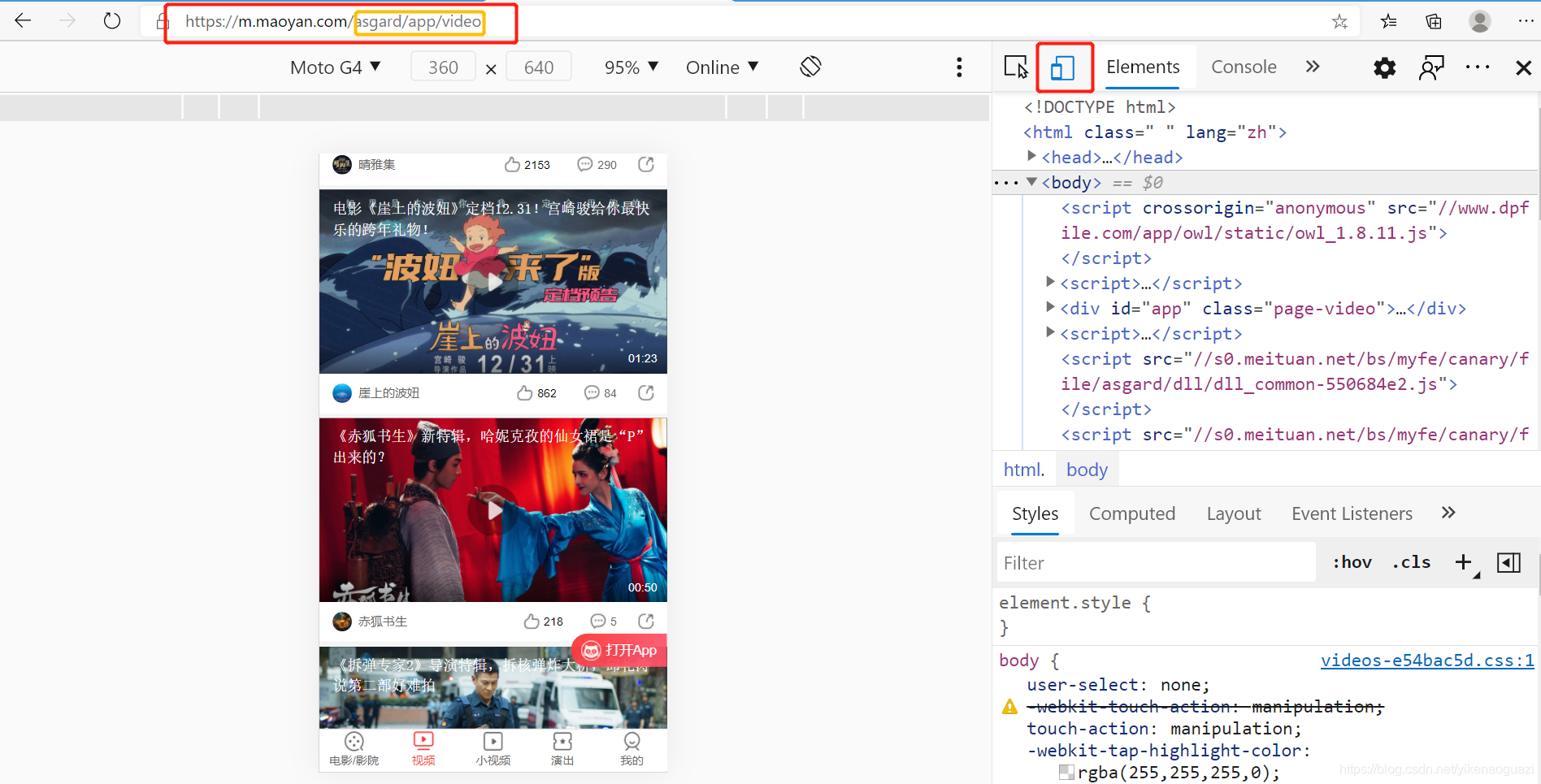
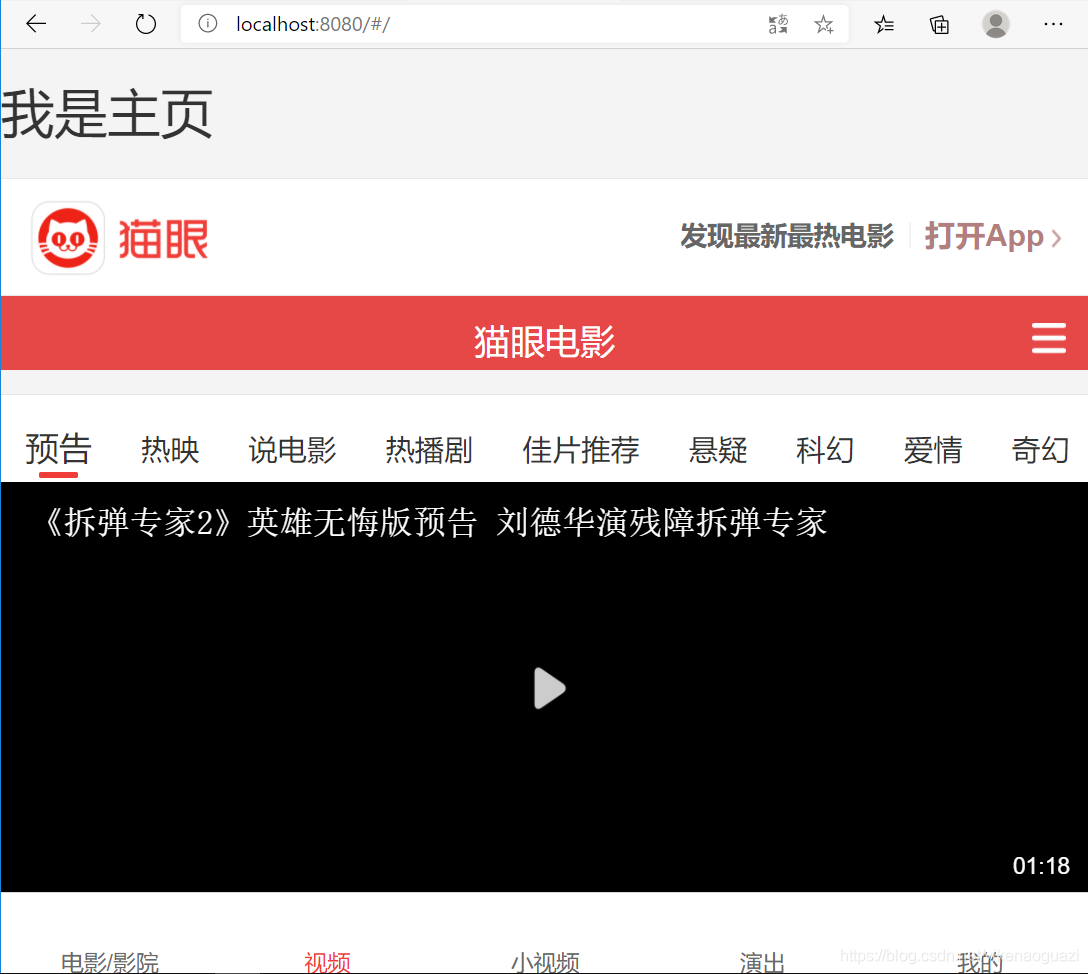
2.头部组件,尾部组件
components/Header.vue
<template>
<div class="header">
<div class="slogan">
<p>老男孩IT教育 | 帮助有志向的年轻人通过努力学习获得体面的工作和生活</p>
</div>
<div class="nav">
<ul class="left-part">
<li class="logo">
<router-link to="/">
<img src="../assets/img/head-logo.svg" alt="">
</router-link>
</li>
<li class="ele">
<span @click="goPage('/free-course')" :class="{active: url_path === '/free-course'}">免费课</span>
</li>
<li class="ele">
<span @click="goPage('/actual-course')" :class="{active: url_path === '/actual-course'}">实战课</span>
</li>
<li class="ele">
<span @click="goPage('/light-course')" :class="{active: url_path === '/light-course'}">轻课</span>
</li>
</ul>
<div class="right-part">
<div>
<span>登录</span>
<span class="line">|</span>
<span>注册</span>
</div>
</div>
</div>
</div>
</template>
<script>
export default {
name: "Header",
data() {
return {
url_path: sessionStorage.url_path || '/',
}
},
methods: {
goPage(url_path) {
if (this.url_path !== url_path) {
this.$router.push(url_path);
}
sessionStorage.url_path = url_path;
},
},
}
</script>
<style scoped>
.header {
background-color: white;
box-shadow: 0 0 5px 0 #aaa;
}
.header:after {
content: "";
display: block;
clear: both;
}
.slogan {
background-color: #eee;
height: 40px;
}
.slogan p {
width: 1200px;
margin: 0 auto;
color: #aaa;
font-size: 13px;
line-height: 40px;
}
.nav {
background-color: white;
user-select: none;
width: 1200px;
margin: 0 auto;
}
.nav ul {
padding: 15px 0;
float: left;
}
.nav ul:after {
clear: both;
content: '';
display: block;
}
.nav ul li {
float: left;
}
.logo {
margin-right: 20px;
}
.ele {
margin: 0 20px;
}
.ele span {
display: block;
font: 15px/36px '微软雅黑';
border-bottom: 2px solid transparent;
cursor: pointer;
}
.ele span:hover {
border-bottom-color: orange;
}
.ele span.active {
color: orange;
border-bottom-color: orange;
}
.right-part {
float: right;
}
.right-part .line {
margin: 0 10px;
}
.right-part span {
line-height: 68px;
cursor: pointer;
}
</style>
components/Footer.vue
<template>
<div class="footer">
<ul>
<li>关于我们</li>
<li>联系我们</li>
<li>商务合作</li>
<li>帮助中心</li>
<li>意见反馈</li>
<li>新手指南</li>
</ul>
<p>Copyright © luffycity.com版权所有 | 京ICP备17072161号-1</p>
</div>
</template>
<script>
export default {
name: "Footer"
}
</script>
<style scoped>
.footer {
width: 100%;
height: 128px;
background: #25292e;
color: #fff;
}
.footer ul {
margin: 0 auto 16px;
padding-top: 38px;
width: 810px;
}
.footer ul li {
float: left;
width: 112px;
margin: 0 10px;
text-align: center;
font-size: 14px;
}
.footer ul::after {
content: "";
display: block;
clear: both;
}
.footer p {
text-align: center;
font-size: 12px;
}
</style>
3.首页组件,轮播图组件
components/Banner.vue
<template>
<div>
<el-carousel height="400px">
<el-carousel-item v-for="item in 4" :key="item">
<img src="../assets/img/banner4.png" alt="">
</el-carousel-item>
</el-carousel>
</div>
</template>
<script>
export default {
name: "Banner",
}
</script>
<style scoped>
.el-carousel__item {
height: 400px;
min-width: 1200px;
}
.el-carousel__item img {
height: 400px;
margin-left: calc(50% - 1920px / 2);
}
</style>
views/Home.vue
<template>
<div id="home">
<Header></Header>
<Banner></Banner>
<br><br><br><br><br><br>
<br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br>
内容部分
<br><br><br><br>
<Footer></Footer>
</div>
</template>
<script>
import Header from "../components/Header";
import Footer from "../components/Footer";
import Banner from "../components/Banner";
export default {
name: "Home",
data(){
return {
}
},
components:{
Header,
Footer,
Banner
}
}
</script>
<style scoped>
</style>
views/ActualCourse.vue
<template>
<div>
<Header></Header>
<h1>实战课</h1>
</div>
</template>
<script>
import Header from '../components/Header'
export default {
name: 'ActualCourse',
data () {
return {
currentDate: new Date()
}
},
components: {
Header
}
}
</script>
<style scoped>
</style>
views/FreeCourse.vue
<template>
<div>
<Header></Header>
<h1>免费课</h1>
</div>
</template>
<script>
import Header from '../components/Header'
export default {
name: 'FreeCourse',
data () {
return {
currentDate: new Date()
}
},
components: {
Header
}
}
</script>
<style scoped>
</style>
views/LightCourse.vue
<template>
<div>
<Header></Header>
<h1>轻课</h1>
</div>
</template>
<script>
import Header from '../components/Header'
export default {
name: 'LightCourse',
data () {
return {
currentDate: new Date()
}
},
components: {
Header
}
}
</script>
<style scoped>
</style>
App.vue
<template>
<div id="app">
<router-view/>
</div>
</template>
<script>
</script>
router/index.js
import Vue from 'vue'
import VueRouter from 'vue-router'
import Home from '../views/Home.vue'
import ActualCourse from '../views/ActualCourse.vue'
import LightCourse from '../views/LightCourse.vue'
import FreeCourse from '../views/FreeCourse.vue'
Vue.use(VueRouter)
const routes = [
{
path: '/',
name: 'Home',
component: Home
},
{
path: '/actual-course',
name: 'ActualCourse',
component: ActualCourse
},
{
path: '/light-course',
name: 'LightCourse',
component: LightCourse
},
{
path: '/free-course',
name: 'FreeCourse',
component: FreeCourse
}
]
const router = new VueRouter({
routes
})
export default router
4.git入门
1 协同开发:版本管理工具
-svn
-git
2 git能干什么
完成协同开发项目,帮助程序员整合代码
i)帮助开发者合并开发的代码
ii)如果出现冲突代码的合并,会提示后提交合并代码的开发者,让其解决冲突
软件:SVN 、 GIT(都是同一个人的个人项目)
github、gitee(两个采用git版本控制器管理代码的公共平台)
github,gitee,gitlab:区别
-github:一般开源的代码放再github,代码托管平台(公有仓库,私有仓库),公司代码不会放 在这上面
-gitee(码云):中国的github,开源代码放在共有仓库,有一部分公司的公司代码托管到gitee 的私有仓库(你们公司代码放在码云上)
-gitlab:公司自己的搭建github,公司内部自己访问(docker拉一个gitlab镜像,跑起来即可)
git:集群化、多分支
3 安装git客户端
一路下一步
4 右键---》git bash(terminal窗口)
git init :初始化仓库
本文内容由网友自发贡献,版权归原作者所有,本站不承担相应法律责任。如您发现有涉嫌抄袭侵权的内容,请联系:hwhale#tublm.com(使用前将#替换为@)