根据输入的字符串精确查找位置,用GeocodeQuery查找坐标,然后根据获取到的坐标,用RegeocodeQuery查询地址。例子中用了两个页面,一个是显示地址信息及定位的页面,另一个是搜索页面,点击搜索结果返回显示页面,显示信息并定位:
显示并定位的页面:
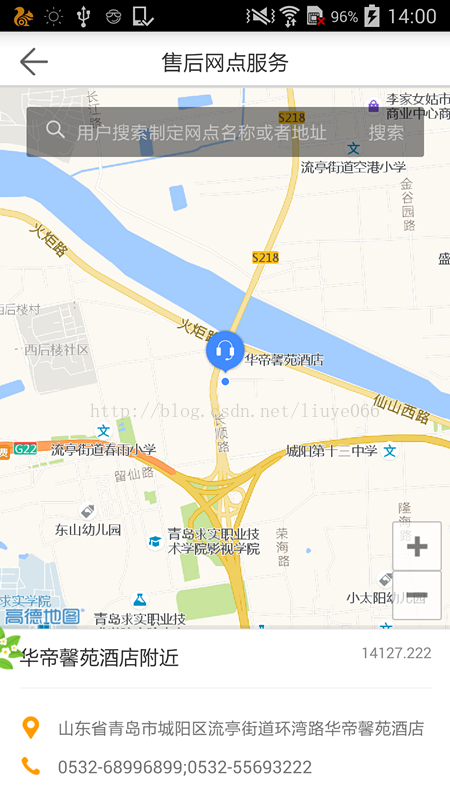
public class SaleMapActivity extends AppCompatActivity implements GeocodeSearch.OnGeocodeSearchListener {
private static final String TAG = "SaleMapActivity";
@BindView(R.id.iv_mapBack)
ImageView ivMapBack;
@BindView(R.id.afterSaleMap)
MapView afterSaleMap;
@BindView(R.id.iv_saleSear)
ImageView ivSaleSear;
@BindView(R.id.rl_sale_search)
RelativeLayout rlSaleSearch;
@BindView(R.id.tv_pointName)
TextView tvPointName;
@BindView(R.id.tv_distance)
TextView tvDistance;
@BindView(R.id.iv_location)
ImageView ivLocation;
@BindView(R.id.tv_location)
TextView tvLocation;
@BindView(R.id.ll_location)
LinearLayout llLocation;
@BindView(R.id.iv_phone)
ImageView ivPhone;
@BindView(R.id.tv_phone)
TextView tvPhone;
@BindView(R.id.ll_phone)
LinearLayout llPhone;
@BindView(R.id.map_bottom)
LinearLayout mapBottom;
AMap aMap;
//声明mLocationOption对象
public AMapLocationClientOption mLocationOption = null;
//声明AMapLocationClient类对象
public AMapLocationClient mLocationClient = null;
private Marker geoMarker;
private Marker regeoMarker;
private Double lat;
private Double lon;
private String mCity = "";
private String areaInfo="";
private String adCode = "";
Context mContext;
private GeocodeSearch geocoderSearch;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_sale_map);
ButterKnife.bind(this);
afterSaleMap.onCreate(savedInstanceState);
mContext = SaleMapActivity.this;
//初始化定位
mLocationClient = new AMapLocationClient(getApplicationContext());
//设置定位回调监听
mLocationClient.setLocationListener(mLocationListener);
initAmap();
}
/**
* * 初始化AMap对象
*/
private void initAmap() {
if (aMap == null) {
aMap = afterSaleMap.getMap();
}
setUpMap();
geocoderSearch = new GeocodeSearch(mContext);
geocoderSearch.setOnGeocodeSearchListener(this);
}
private void setUpMap() {
//初始化定位参数
mLocationOption = new AMapLocationClientOption();
//设置定位模式为高精度模式,Battery_Saving为低功耗模式,Device_Sensors是仅设备模式
mLocationOption.setLocationMode(AMapLocationClientOption.AMapLocationMode.Hight_Accuracy);
//设置是否返回地址信息(默认返回地址信息)
mLocationOption.setNeedAddress(true);
//设置是否只定位一次,默认为false
mLocationOption.setOnceLocation(true);
//设置是否强制刷新WIFI,默认为强制刷新
mLocationOption.setWifiActiveScan(true);
//设置是否允许模拟位置,默认为false,不允许模拟位置
mLocationOption.setMockEnable(false);
//设置定位间隔,单位毫秒,默认为2000ms
// mLocationOption.setInterval(2000);
//给定位客户端对象设置定位参数
mLocationClient.setLocationOption(mLocationOption);
//启动定位
mLocationClient.startLocation();
//重新定位时移除红气泡,再重新初始化
geoMarker = aMap.addMarker(new MarkerOptions().anchor(0.5f, 0.5f)
.icon(BitmapDescriptorFactory
.defaultMarker(BitmapDescriptorFactory.HUE_BLUE)));
// geoMarker = aMap.addMarker(new MarkerOptions().anchor(0.5f, 0.5f)
// .icon(BitmapDescriptorFactory.fromResource(R.drawable.cur_position)));
// regeoMarker = aMap.addMarker(new MarkerOptions().anchor(0.5f, 0.5f)
// .icon(BitmapDescriptorFactory
// .defaultMarker(BitmapDescriptorFactory.HUE_RED)));
regeoMarker = aMap.addMarker(new MarkerOptions().anchor(0.5f, 0.5f)
.icon(BitmapDescriptorFactory.fromResource(R.drawable.icon_map_search)));
}
//声明定位回调监听器
public AMapLocationListener mLocationListener = new AMapLocationListener() {
@Override
public void onLocationChanged(AMapLocation amapLocation) {
if (amapLocation != null) {
if (amapLocation.getErrorCode() == 0) {
//定位成功回调信息,设置相关消息
amapLocation.getLocationType();//获取当前定位结果来源,如网络定位结果,详见定位类型表
amapLocation.getLatitude();//获取纬度
amapLocation.getLongitude();//获取经度
amapLocation.getAccuracy();//获取精度信息
SimpleDateFormat df = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
Date date = new Date(amapLocation.getTime());
df.format(date);//定位时间
amapLocation.getAddress();//地址,如果option中设置isNeedAddress为false,则没有此结果,网络定位结果中会有地址信息,GPS定位不返回地址信息。
amapLocation.getCountry();//国家信息
amapLocation.getProvince();//省信息
mCity = amapLocation.getCity();//城市信息
amapLocation.getDistrict();//城区信息
amapLocation.getStreet();//街道信息
amapLocation.getStreetNum();//街道门牌号信息
amapLocation.getCityCode();//城市编码
adCode = amapLocation.getAdCode();//地区编码
amapLocation.getAoiName();//获取当前定位点的AOI信息
lat = amapLocation.getLatitude();
lon = amapLocation.getLongitude();
areaInfo = amapLocation.getProvince()+" "+amapLocation.getCity() +" "+amapLocation.getDistrict();
Log.v("pcw", "lat : " + lat + " lon : " + lon+" adCode: "+adCode+","+areaInfo);
Log.v("pcw", "amapLocation="+amapLocation.toString());
// etEAddr.setText(amapLocation.getAoiName());
tvPointName.setText(amapLocation.getAoiName());
tvLocation.setText(amapLocation.getAddress());
// 设置当前地图显示为当前位置
aMap.moveCamera(CameraUpdateFactory.newLatLngZoom(new LatLng(lat, lon), 16));
MarkerOptions markerOptions = new MarkerOptions();
markerOptions.position(new LatLng(lat, lon));
markerOptions.title("当前位置");
markerOptions.visible(true);
// BitmapDescriptor bitmapDescriptor = BitmapDescriptorFactory.fromBitmap(BitmapFactory.decodeResource(getResources(), R.drawable.location_marker));
// BitmapDescriptor bitmapDescriptor = BitmapDescriptorFactory.fromResource(R.drawable.cur_position);
//设置当前位置的marker图标
ImageView imageView = new ImageView(SaleMapActivity.this);
imageView.setImageResource(R.drawable.cur_position);
BitmapDescriptor bitmapDescriptor = BitmapDescriptorFactory.fromView(imageView);
markerOptions.icon(bitmapDescriptor);
aMap.addMarker(markerOptions);
// mLocationListener.onLocationChanged(amapLocation);
} else {
//显示错误信息ErrCode是错误码,errInfo是错误信息,详见错误码表。
Log.e("AmapError", "location Error, ErrCode:"
+ amapLocation.getErrorCode() + ", errInfo:"
+ amapLocation.getErrorInfo());
Toast.makeText(mContext,amapLocation.getErrorInfo(),Toast.LENGTH_LONG).show();
}
}
}
};
@Override
protected void onActivityResult(int requestCode,int resultCode,Intent data){
LogUtil.d("SaleMapActivity","sdjflksdfjdlskf");
if(requestCode == 1){
if(resultCode == RESULT_OK){
LogUtil.d("SaleMapActivity","onActivityResult===========");
Bundle bundle = data.getBundleExtra("searAddr");
GeocodeAddress address = bundle.getParcelable("addr");
//根据传回来的地址坐标获取该地点的位置详细信息
//通过 RegeocodeQuery(LatLonPoint point, float radius, java.lang.String latLonType) 设置查询参数
RegeocodeQuery rQuery = new RegeocodeQuery(address.getLatLonPoint(),200,GeocodeSearch.AMAP);
//调用 GeocodeSearch 的 getFromLocationAsyn(RegeocodeQuery regeocodeQuery) 方法发起请求。
//通过回调接口 onRegeocodeSearched 解析返回的结果
geocoderSearch.getFromLocationAsyn(rQuery);
}
}
}
@OnClick({R.id.iv_mapBack, R.id.rl_sale_search, R.id.ll_phone})
public void onClick(View view) {
switch (view.getId()) {
case R.id.iv_mapBack:
finish();
break;
case R.id.rl_sale_search:
Intent searchIntent = new Intent(SaleMapActivity.this, SearchSaleActivity.class);
startActivityForResult(searchIntent,1);
// startActivity(searchIntent);
break;
case R.id.ll_phone:
break;
}
}
@Override
public void onRegeocodeSearched(RegeocodeResult regeocodeResult, int i) {
if(i== 1000){
if(regeocodeResult != null && regeocodeResult.getRegeocodeAddress()!= null
&& regeocodeResult.getRegeocodeAddress().getFormatAddress() != null){
adCode = regeocodeResult.getRegeocodeAddress().getAdCode();
LatLng latLng= convertToLatLng(regeocodeResult.getRegeocodeQuery().getPoint());
aMap.animateCamera(CameraUpdateFactory.newLatLngZoom(latLng,15));
regeoMarker.setPosition(latLng);
RegeocodeAddress address = regeocodeResult.getRegeocodeAddress();
List<PoiItem> poiItems = address.getPois();
for(int j = 0;j<poiItems.size();j++){
PoiItem item = poiItems.get(j);
LogUtil.d(TAG,"Title="+item.getTitle()+",Distance="+item.getDistance()+",AdName="+item.getAdName()+",Tel"+item.getTel()
+",BusinessArea="+item.getBusinessArea()+",Snippet="+item.getSnippet()+",Direction"+item.getDirection()
+",TypeDes"+item.getTypeDes());
}
tvPointName.setText(poiItems.get(0).getTitle()+"附近");
//我的当前位置与搜索到的位置的距离
LatLng latLng1 = new LatLng(lat,lon);
float distance = AMapUtils.calculateLineDistance(latLng1,regeoMarker.getPosition());
tvDistance.setText(String.valueOf(distance));
tvLocation.setText(address.getFormatAddress());
tvPhone.setText(poiItems.get(0).getTel());
Toast.makeText(mContext, "定位成功", Toast.LENGTH_LONG).show();
}else{
Toast.makeText(mContext, "定位失败", Toast.LENGTH_LONG).show();
}
}else{
Toast.makeText(mContext, i, Toast.LENGTH_LONG).show();
}
}
public LatLng convertToLatLng(LatLonPoint point){
return new LatLng(point.getLatitude(),point.getLongitude());
}
@Override
public void onGeocodeSearched(GeocodeResult geocodeResult, int i) {
}
}
2. 搜索页面:
public class SearchSaleActivity extends AppCompatActivity {
private static final String TAG = "SearchSaleActivity";
@BindView(R.id.salesearch_edit)
TextField salesearchEdit;
@BindView(R.id.tv_saleCancel)
TextView tvSaleCancel;
@BindView(R.id.lvResult)
ListView lvResult;
GeocodeSearch geocodeSearch;
Context mContext;
SearchMapAdapter adapter;
List<GeocodeAddress> addrList;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_search_sale);
ButterKnife.bind(this);
mContext = SearchSaleActivity.this;
addrList = new ArrayList<GeocodeAddress>();
//构造 GeocodeSearch 对象,并设置监听。
geocodeSearch = new GeocodeSearch(mContext);
geocodeSearch.setOnGeocodeSearchListener(new GeocodeSearch.OnGeocodeSearchListener() {
@Override
public void onRegeocodeSearched(RegeocodeResult regeocodeResult, int i) {
}
@Override
public void onGeocodeSearched(GeocodeResult geocodeResult, int i) {
//地理编码;根据地址获取坐标
if(i == 1000){
LogUtil.d(TAG,"success");
if(geocodeResult != null && geocodeResult.getGeocodeAddressList() != null
&& geocodeResult.getGeocodeAddressList().size() != 0){
LogUtil.d(TAG,""+geocodeResult.getGeocodeQuery().getLocationName());
addrList = geocodeResult.getGeocodeAddressList();
adapter = new SearchMapAdapter(mContext,addrList);
lvResult.setAdapter(adapter);
}
}
}
});
salesearchEdit.addTextChangedListener(new MyTextChangeListener());
lvResult.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
Intent intent = new Intent(mContext,SaleMapActivity.class);
Bundle bundle = new Bundle();
bundle.putParcelable("addr",addrList.get(position));
intent.putExtra("searAddr",bundle);
setResult(RESULT_OK,intent);
finish();
}
});
}
//文本框的改变监听
class MyTextChangeListener implements TextWatcher{
@Override
public void beforeTextChanged(CharSequence s, int start, int count, int after) {
}
@Override
public void onTextChanged(CharSequence s, int start, int before, int count) {
String newText = s.toString().trim();
if(newText != null && !newText.equals("")){
//通过 GeocodeQuery(java.lang.String locationName, java.lang.String city) 设置查询参数
//name表示地址,第二个参数表示查询城市,中文或者中文全拼,citycode、adcode
GeocodeQuery query = new GeocodeQuery(newText,"0532");
//调用 GeocodeSearch 的 getFromLocationNameAsyn(GeocodeQuery geocodeQuery) 方法发起请求。
//通过回调接口 onGeocodeSearched 解析返回的结果
geocodeSearch.getFromLocationNameAsyn(query);
}
}
@Override
public void afterTextChanged(Editable s) {
}
}
@OnClick(R.id.tv_saleCancel)
public void onClick() {
}
}