项目介绍
- 本项目实现广度优先搜索算法。
- 读取txt文件中第一行表示图中顶点数的单个整数N
- 读取txt文件中第二行开始是一对对的整数。每一对表示图中某条边两端的两个顶点。
- 图是无向的,因此节点编号为0 - N‐1。
- 程序先遍历图的顶点,从节点0开始,输出从这个节点可以到达的所有节点。
- 输出包含从算法获得的生成树。它应该被打印成树中有序的边列表。每条边的顶点应该按照BFS遍历的顺序列出。
输入的txt文件如下:
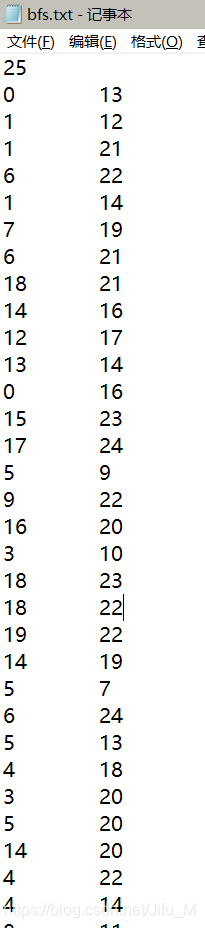
其中第一行是总的顶点数,后面的两列组成的对表示一条边上的两个顶点。
代码实现
#include <iostream>
#include <fstream>
using namespace std;
//Two lines' array with maximum 200 rows
int VerticesEdge[200][200];
int Vertices[200];
int queue[200];
int QueueNumber = 0;
int Index = 0;// Vertices index
void AddVertices(int i);
void Inqueue(int i);
void Dequeue();
bool Check(int x);//check repeat
int main()
{
int EdgeNumbers = 0;//used for edge counting
int TotalVertices;//read the number of vertex
int LeftVertices;// Left column vertex
int RightVertices;//Right column vertex
ifstream read;
string a;
cout << "Please enter the filename: " << endl;
cin >>a;
read.open(a.c_str());
if (!read)
{
cout << "Can not find the file" << endl;
exit(1);
}
read >> TotalVertices;
while (!read.eof())
{
read >> LeftVertices;
read >> RightVertices;
VerticesEdge[LeftVertices][RightVertices] = 1;
VerticesEdge[RightVertices][LeftVertices] = 1;
EdgeNumbers++;
}
read.close();
EdgeNumbers--;//There is a space line at the end of the file
Inqueue( VerticesEdge[0][0]);//write root node into queue
AddVertices( VerticesEdge[0][0]);
while ( QueueNumber != 0)
{
for (int x = 0; x < TotalVertices; x++)
{
if ( VerticesEdge[queue[0]][x] == 1)
{
if (Check(x) ==false)
{
AddVertices(x);
Inqueue(x);
cout << queue[0] << " " << x << endl;
}
}
}
Dequeue();
}
return 0;
}
void AddVertices(int a)
{
Vertices[Index] = a;
Index++;
}
void Inqueue(int a)
{
queue[QueueNumber] = a;
QueueNumber++;
}
void Dequeue()
{
for (int i = 0; i < QueueNumber; i++)
{
queue[i] = queue[i + 1];
}
QueueNumber--;
}
bool Check(int x)
{
int a = 0;
while (a < Index)
{
if (x == Vertices[a])
{
return true;
}
if (a == Index - 1)
{
return false;
}
a++;
}
}
输出结果如下所示:
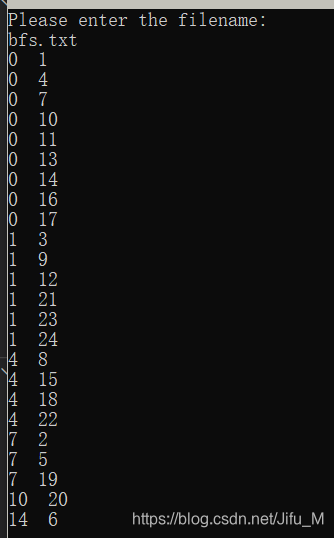