1.Stepper,Step
Stepper({
Key key,
@required this.steps, //step类型的子集
this.physics,
this.type = StepperType.vertical, //方向
this.currentStep = 0, //当前位置
this.onStepTapped, //点击事件
this.onStepContinue, //继续事件
this.onStepCancel, //返回
this.controlsBuilder, //操作的控制builder
})
const Step({
@required this.title, //标题
this.subtitle, //副标题
@required this.content, //内容
this.state = StepState.indexed, //图标的状态
this.isActive = false, //是否是active
})
import 'package:flutter/material.dart';
class MyComponent extends StatefulWidget {
@override
State<StatefulWidget> createState() {
// TODO: implement createState
return _MyComponent();
}
}
class _MyComponent extends State<MyComponent> {
int _step = 0;
@override
Widget build(BuildContext context) {
// TODO: implement build
return Scaffold(
appBar: AppBar(
title: Text("Component"),
),
body: Column(
children: <Widget>[
Stepper(
currentStep: _step,
//继续的回调
onStepContinue: () {
if (_step == 3) {
setState(() {
_step = 0;
});
} else {
setState(() {
_step += 1;
});
}
},
//控制按钮的builder
controlsBuilder: (BuildContext context,
{VoidCallback onStepContinue, VoidCallback onStepCancel}) {
return Row(
children: <Widget>[
RaisedButton(
onPressed: onStepContinue,
child: const Text('继续'),
)
],
);
},
steps: [
Step(
title: Text("标题"),
content: Container(
width: 150.0,
height: 50.0,
color: Colors.black12,
),
state: StepState.complete,
isActive: true),
Step(
title: Text("标题"),
content: Text("内容"),
state: StepState.disabled,
),
Step(
title: Text("标题"),
content: Text("内容"),
state: StepState.editing,
),
Step(
title: Text("标题"),
subtitle: Text("副标题"),
content: Text("内容"),
isActive: true)
],
)
],
),
);
}
}
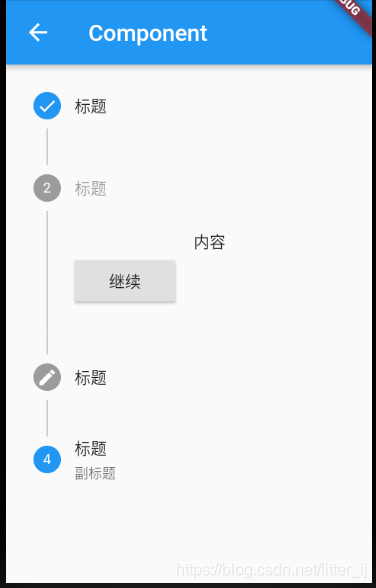
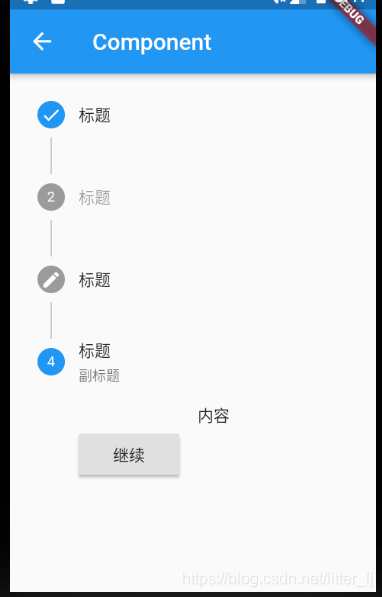
2.Padding,Align,Center
const Padding({
Key key,
@required this.padding, //间距padding
Widget child, //子集
})
//居中
//当widthFactor和heightFactor为null的时候,
//当其有限制条件的时候,Align会根据限制条件尽量的扩展自己的尺寸,
//当没有限制条件的时候,会调整到child的尺寸;
//当widthFactor或者heightFactor不为null的时候,
//Aligin会根据factor属性,扩展自己的尺寸,
//例如设置widthFactor为2.0的时候,那么,Align的宽度将会是child的两倍
const Center({
Key key,
double widthFactor,
double heightFactor,
Widget child
})
const Align({
Key key,
this.alignment = Alignment.center, //对齐方式
this.widthFactor, //同上
this.heightFactor, //同上
Widget child, //子集
})
import 'package:flutter/material.dart';
class MyIos extends StatefulWidget {
@override
State<StatefulWidget> createState() {
// TODO: implement createState
return _MyIos();
}
}
class _MyIos extends State<MyIos> {
@override
Widget build(BuildContext context) {
// TODO: implement build
return Scaffold(
appBar: AppBar(
title: Text("Layout"),
),
body: Column(
children: <Widget>[
//Container有对齐方式,这里只是测试
//Align
Container(
width: 200.0,
height: 100.0,
color: Colors.black12,
child: Padding(
padding: EdgeInsets.all(10.0),
child: Align(
alignment: Alignment.bottomRight,
child: Text("内容很少"),
),
),
),
//Container有对齐方式,这里只是测试
//Center
Container(
margin: EdgeInsets.only(top: 15.0),
width: 200.0,
height: 100.0,
color: Colors.black12,
child: Padding(
padding: EdgeInsets.all(10.0),
child: Center(
child: Text("内容"),
),
),
)
],
),
);
}
}
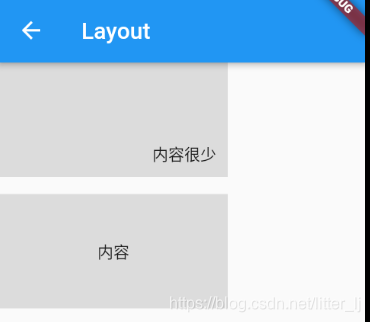
3.FittedBox
//类似于图片的那种缩放满足容器的大小的那种盒模型
//如果外部有约束的话,按照外部约束调整自身尺寸,然后缩放调整child,按照指定的条件进行布局
//如果没有外部约束条件,则跟child尺寸一致,指定的缩放以及位置属性将不起作用
const FittedBox({
Key key,
this.fit = BoxFit.contain, //类似图片的fit
this.alignment = Alignment.center, //对齐方式
Widget child, //子集
})
import 'package:flutter/material.dart';
class MyIos extends StatefulWidget {
@override
State<StatefulWidget> createState() {
// TODO: implement createState
return _MyIos();
}
}
class _MyIos extends State<MyIos> {
@override
Widget build(BuildContext context) {
// TODO: implement build
return Scaffold(
appBar: AppBar(
title: Text("Layout"),
),
body: Column(
children: <Widget>[
Container(
width: 200.0,
height: 200.0,
color: Colors.black12,
child: FittedBox(
fit: BoxFit.contain,
child: Text("567"),
),
),
Container(
width: 200.0,
margin: EdgeInsets.only(top: 15.0),
height: 200.0,
color: Colors.black12,
child: FittedBox(
alignment: Alignment.bottomRight,
fit: BoxFit.scaleDown,
child: Text("567"),
),
)
],
),
);
}
}
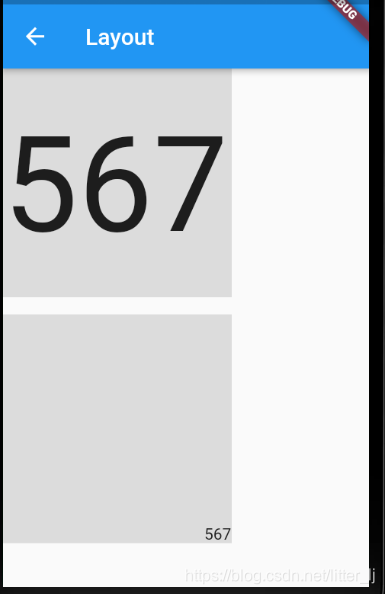
4.AspectRatio
//场景是相机,相机的预览尺寸都是固定的几个值,因此不能随意去设置相机的显示区域,必须按照比率进行显示
//AspectRatio首先会在布局限制条件允许的范围内尽可能的扩展,widget的高度是由宽度和比率决定的,类似于BoxFit中的contain,按照固定比率去尽量占满区域
//如果在满足所有限制条件过后无法找到一个可行的尺寸,AspectRatio最终将会去优先适应布局限制条件,而忽略所设置的比率
const AspectRatio({
Key key,
@required this.aspectRatio, //宽高比
Widget child,
})
Container(
height: 200.0,
child: new AspectRatio(
aspectRatio: 1.5,
child: new Container(
color: Colors.red,
),
),
)
// 300 x 200的区域
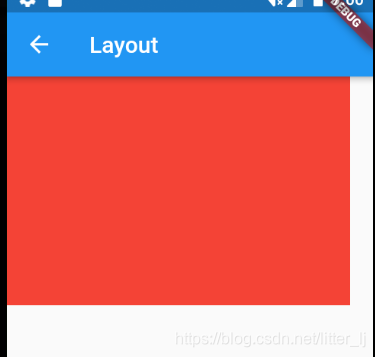
5.ConstrainedBox
//约束条件
ConstrainedBox({
Key key,
@required this.constraints,
Widget child,
})
const BoxConstraints({
this.minWidth = 0.0,
this.maxWidth = double.infinity,
this.minHeight = 0.0,
this.maxHeight = double.infinity,
});
//最小宽度和最小高度
//Container宽度全屏,高度只有150
ConstrainedBox(
constraints: BoxConstraints(
minWidth: 100.0,
minHeight: 150.0
),
child: Container(
color: Colors.black12,
),
)
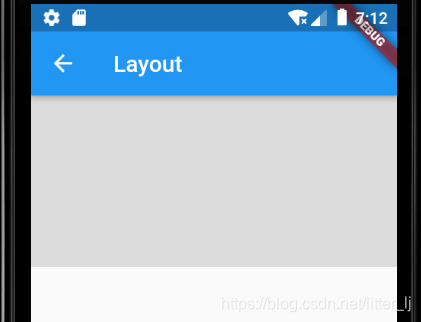