java图形化 图书管理系统 使用mysql数据库
此博客是本人一次Java实验课作业:
1、管理员操作:能够实现图书的增、删、改、查操作
2、普通注册用户操作:
(1)借阅图书(借阅成功后,对应图书在馆数量相应减1)
(2)归还图书(归还成功后,对应图书在馆数量加1)
(3)查阅自己借阅的图书
(4)续借图书(借阅图书以3个月为限,可以在期间内续借1个月)
(5)如果预借图书在馆数量为零,或者图书不存在,则无法借阅
(6)可以为所借阅的图书进行评论
3自行设计数据库表
此项目已经更新,修改了所有反馈的bug,增加了新功能,增加可玩性,调整了代码结构。欢迎访问另一篇博客
https://blog.csdn.net/m0_52889702/article/details/127230912
结果展示:
普通用户
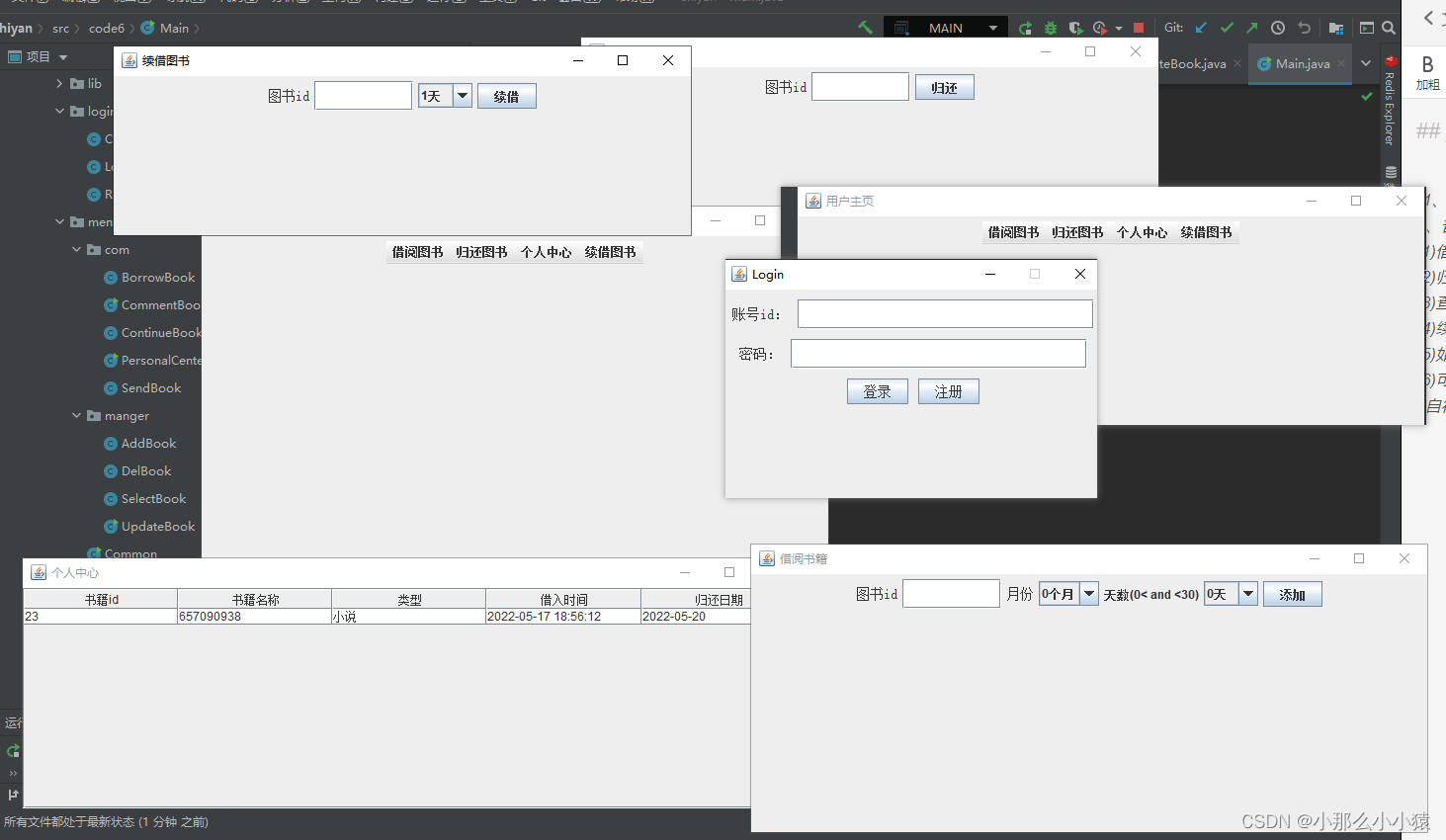
管理员:
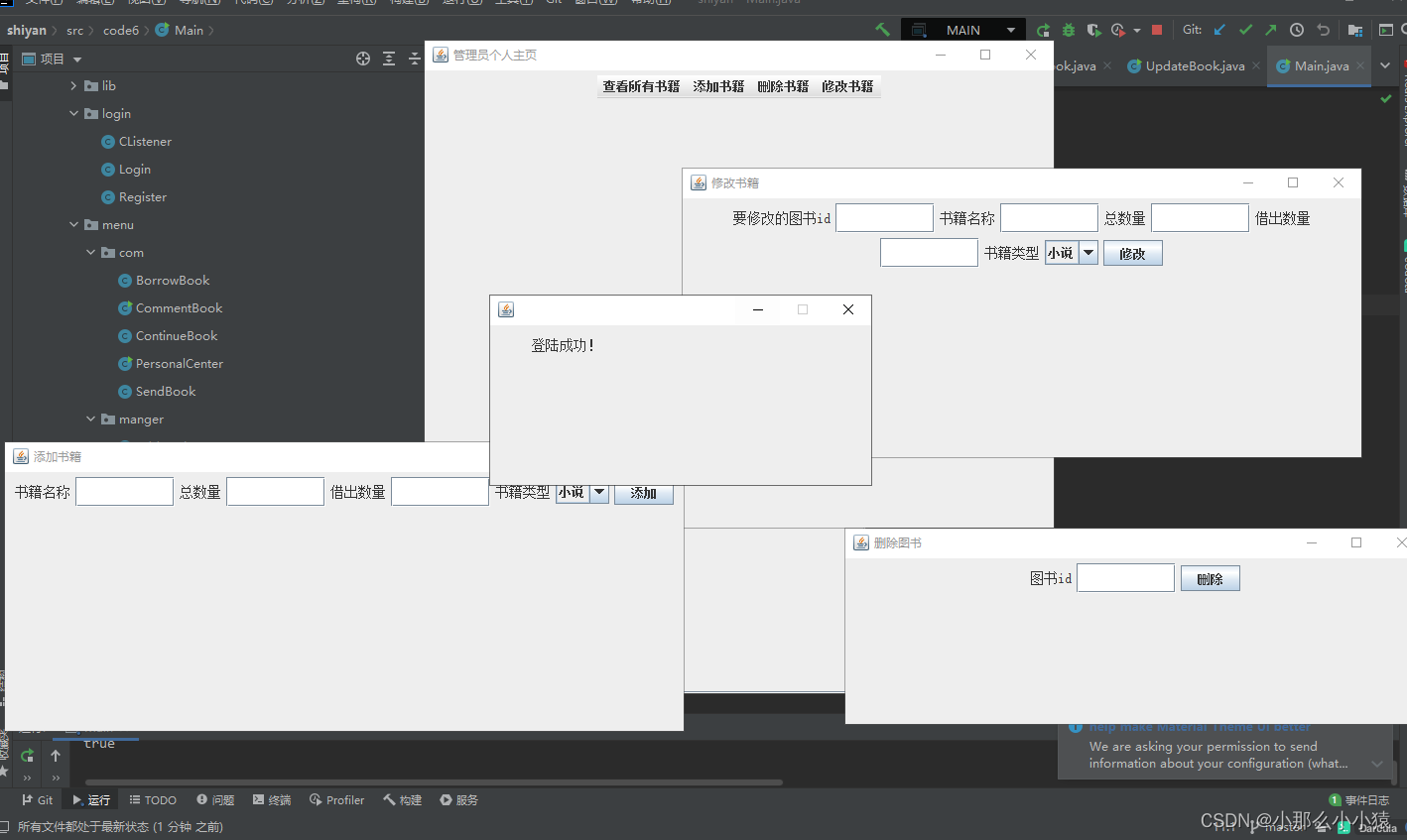
源码包地址:
https://gitee.com/wang-yongyan188/experimental-code.git
库表设计:




代码:引入jdbc-mysql的jar包 添加到库
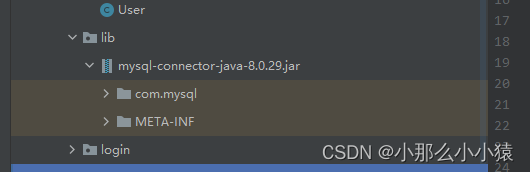
创建实体类:目的是将数据库记录映射为Java对象
book:
public class Book {
String bname;
Integer bid;
Integer allnum;
Integer borrownum;
String type;
public Book() {
}
public Book(String bname, Integer bid, Integer allnum, Integer borrownum, String type) {
this.bname = bname;
this.bid = bid;
this.allnum = allnum;
this.borrownum = borrownum;
this.type = type;
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public String getBname() {
return bname;
}
public void setBname(String bname) {
this.bname = bname;
}
public Integer getBid() {
return bid;
}
public void setBid(Integer bid) {
this.bid = bid;
}
public Integer getAllnum() {
return allnum;
}
public void setAllnum(Integer allnum) {
this.allnum = allnum;
}
public Integer getBorrownum() {
return borrownum;
}
public void setBorrownum(Integer borrownum) {
this.borrownum = borrownum;
}
@Override
public String toString() {
return "Book{" +
"bname='" + bname + '\'' +
", bid=" + bid +
", allnum=" + allnum +
", borrownum=" + borrownum +
'}';
}
}
User:
public class User {
Integer id;
String passwd;
String uname;
Integer ismanger;
public User(Integer id, String passwd, String uname, Integer ismanger) {
this.id = id;
this.passwd = passwd;
this.uname = uname;
this.ismanger = ismanger;
}
public User() {
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getPasswd() {
return passwd;
}
public Integer getIsmanger() {
return ismanger;
}
public void setIsmanger(Integer ismanger) {
this.ismanger = ismanger;
}
public void setPasswd(String passwd) {
this.passwd = passwd;
}
public String getUname() {
return uname;
}
public void setUname(String uname) {
this.uname = uname;
}
@Override
public String toString() {
return "User{" +
"id=" + id +
", passwd='" + passwd + '\'' +
", uname='" + uname + '\'' +
", ismanger=" + ismanger +
'}';
}
}
Rent:
public class Rent {
Integer rid;
String btime;
String days;
Integer uid;
Integer bid;
public Rent() {
}
public Rent(Integer rid, String btime, String days, Integer uid, Integer bid) {
this.rid = rid;
this.btime = btime;
this.days = days;
this.uid = uid;
this.bid = bid;
}
public Integer getRid() {
return rid;
}
public void setRid(Integer rid) {
this.rid = rid;
}
public String getBtime() {
return btime;
}
public void setBtime(String btime) {
this.btime = btime;
}
public String getDays() {
return days;
}
public void setDays(String days) {
this.days = days;
}
public Integer getUid() {
return uid;
}
public void setUid(Integer uid) {
this.uid = uid;
}
public Integer getBid() {
return bid;
}
public void setBid(Integer bid) {
this.bid = bid;
}
@Override
public String toString() {
return "Rent{" +
"rid=" + rid +
", btime='" + btime + '\'' +
", days='" + days + '\'' +
", uid=" + uid +
", bid=" + bid +
'}';
}
}
与数据库进行交互 封装成的工具类:
import code6.entity.Book;
import code6.entity.Rent;
import code6.entity.User;
import java.sql.*;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.*;
import java.util.Date;
public class SqlUtils {
public static Connection getCon() throws ClassNotFoundException, SQLException {
Class.forName("com.mysql.cj.jdbc.Driver");
Connection con = DriverManager.getConnection("jdbc:mysql://localhost:3306/code6", "root", "1234");// 注意:这里要修改为自己的!!!
return con;
}
public static int AutoId() {
int orderId = UUID.randomUUID().toString().hashCode();
orderId = orderId < 0 ? -orderId : orderId;
return orderId;
}
public static User getUserById(int id) throws SQLException, ClassNotFoundException {
Connection con = getCon();
Statement statement = con.createStatement();
String sql = "SELECT * from `user` where uid=" + id;
ResultSet resultSet = statement.executeQuery(sql);
System.out.println(resultSet.next());
User user = new User();
user.setId(resultSet.getInt(1));
user.setPasswd(resultSet.getString(2));
user.setUname(resultSet.getString(3));
user.setIsmanger(resultSet.getInt(4));
statement.close();
con.close();
return user;
}
public static List<Book> getAll() throws SQLException, ClassNotFoundException {
Connection con = getCon();
Statement statement = con.createStatement();
String sql = "SELECT * FROM book";
ResultSet resultSet = statement.executeQuery(sql);
ArrayList<Book> books = new ArrayList<>();
Book book = new Book();
while (resultSet.next()) {
book.setBname(resultSet.getString(1));
book.setBid(resultSet.getInt(2));
book.setAllnum(resultSet.getInt(3));
book.setBorrownum(resultSet.getInt(4));
book.setType(resultSet.getString(5));
books.add(book);
}
statement.close();
con.close();
return books;
}
public static int addBook(Book book) throws SQLException, ClassNotFoundException {
Connection con = getCon();
String sql = "INSERT book VALUES(?,?,?,?,?);";
PreparedStatement prepareStatement = con.prepareStatement(sql);
prepareStatement.setString(1, book.getBname());
prepareStatement.setInt(2, AutoId());
prepareStatement.setInt(3, book.getAllnum());
prepareStatement.setInt(4, book.getBorrownum());
prepareStatement.setString(5, book.getType());
int i = prepareStatement.executeUpdate();
prepareStatement.close();
con.close();
return i;
}
public static int delBook(int bid) throws SQLException, ClassNotFoundException {
Connection con = getCon();
String sql="DELETE from book WHERE bid=?";
PreparedStatement prepareStatement = con.prepareStatement(sql);
prepareStatement.setInt(1,bid);
return prepareStatement.executeUpdate();
}
public static int upBook(Book book) throws SQLException, ClassNotFoundException {
Connection con = getCon();
String sql="UPDATE book SET bname=?,allnum=?,borrownum=?,type=? WHERE bid=?";
PreparedStatement prepareStatement = con.prepareStatement(sql);
prepareStatement.setString(1,book.getBname());
prepareStatement.setInt(2,book.getAllnum());
prepareStatement.setInt(3,book.getBorrownum());
prepareStatement.setString(4,book.getType());
prepareStatement.setInt(5,book.getBid());
int i = prepareStatement.executeUpdate();
prepareStatement.close();
con.close();
return i;
}
public static int addRent(int uid,int bid,int days,int month) throws SQLException, ClassNotFoundException {
Connection con = getCon();
con.setAutoCommit(false);
String sql="INSERT INTO rent VALUES(?,?,?,?,?)";
PreparedStatement prepareStatement = con.prepareStatement(sql);
prepareStatement.setInt(1,AutoId());
Date date = new Date();
SimpleDateFormat format = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
String sbegin= format.format(date);
prepareStatement.setString(2,sbegin);
Calendar cal = Calendar.getInstance();
cal.setTime(date);
cal.add(Calendar.MONTH,month);
Date temptime = cal.getTime();
SimpleDateFormat format2 = new SimpleDateFormat("yyyy-MM-dd");
String endday = format2.format(temptime.getTime() + days * 24 * 60 * 60 * 1000);
prepareStatement.setString(3,endday);
prepareStatement.setInt(4,uid);
prepareStatement.setInt(5,bid);
String sql2="UPDATE book set borrownum=borrownum+1 where bid="+bid;
int i1=0;
int i=0;
Statement statement = con.createStatement();
try{
i1= statement.executeUpdate(sql2);
i= prepareStatement.executeUpdate();
con.commit();
}
catch (Exception e){
con.rollback();
}
finally {
statement.close();
prepareStatement.close();
con.close();
}
return (i==1&&i==1)?1:0;
}
public static Boolean isAddRend(int bid) throws SQLException, ClassNotFoundException {
Connection con = getCon();
String sql="SELECT allnum,borrownum from book WHERE bid="+bid;
Statement statement = con.createStatement();
ResultSet resultSet = statement.executeQuery(sql);
resultSet.next();
int all=resultSet.getInt(1);
int rent=resultSet.getInt(2);
statement.close();
con.close();
return all>rent?true:false;
}
public static List<Rent> getAllRentByUid(int uid) throws SQLException, ClassNotFoundException {
Connection con = getCon();
String sql="SELECT * from rent where uid="+uid;
Statement statement = con.createStatement();
ResultSet resultSet = statement.executeQuery(sql);
ArrayList<Rent> rents = new ArrayList<>(5);
while (resultSet.next()){
Rent rent = new Rent();
rent.setRid(resultSet.getInt(1));
rent.setBtime(resultSet.getString(2));
rent.setDays(resultSet.getString(3));
rent.setUid(resultSet.getInt(4));
rent.setBid(resultSet.getInt(5));
rents.add(rent);
}
return rents;
}
public static Book getBookByBid(int bid) throws SQLException, ClassNotFoundException {
Connection con = getCon();
String sql="SELECT * from book where bid="+bid;
Statement statement = con.createStatement();
ResultSet resultSet = statement.executeQuery(sql);
Book book = new Book();
while (resultSet.next()){
book.setBname(resultSet.getString(1));
book.setBid(resultSet.getInt(2));
book.setAllnum(resultSet.getInt(3));
book.setBorrownum(resultSet.getInt(4));
book.setType(resultSet.getString(5));
}
return book;
}
public static List<Map> getCentosByUid(int uid) throws SQLException, ClassNotFoundException {
Connection con = getCon();
Statement statement = con.createStatement();
String sql="SELECT bname,b.bid,type,btime,days from book b ,rent r WHERE b.bid=r.bid and r.uid="+uid;
ResultSet resultSet = statement.executeQuery(sql);
ArrayList<Map> maps = new ArrayList<>();
while (resultSet.next()){
HashMap<String, String> map = new HashMap<>();
map.put("bname",resultSet.getString(1));
map.put("bid", String.valueOf(resultSet.getInt(2)));
map.put("type",resultSet.getString(3));
map.put("btime",resultSet.getString(4));
map.put("days",resultSet.getString(5));
maps.add(map);
}
return maps;
}
public static Boolean IsRent(int uid,int bid) throws SQLException, ClassNotFoundException {
Connection con = getCon();
String sql="SELECT bid from rent WHERE uid="+uid;
Statement statement = con.createStatement();
ArrayList<Object> list = new ArrayList<>();
ResultSet resultSet = statement.executeQuery(sql);
while (resultSet.next()){
list.add(resultSet.getInt(1));
}
System.out.println(list);
for (int i=0;i<list.size();i++){
if(list.get(i).equals(bid)){
return true;
}
}
return false;
}
public static Boolean decRend(int uid,int bid) throws SQLException, ClassNotFoundException {
Connection con = getCon();
con.setAutoCommit(false);
String sql="DELETE from rent WHERE uid=? and bid=?";
PreparedStatement prepareStatement = con.prepareStatement(sql);
prepareStatement.setInt(1,uid);
prepareStatement.setInt(2,bid);
int i =0;
String sql2="UPDATE book set borrownum=borrownum-1 where bid="+bid;
Statement statement = con.createStatement();
int i1 =0;
try { i=prepareStatement.executeUpdate();
i1=statement.executeUpdate(sql2);
con.commit();
}
catch (Exception e){
System.out.println("失败");
con.rollback();
}
return (i1>0 &&i>0)? true:false;
}
public static int contineDays(int bid,int uid,int days) throws SQLException, ClassNotFoundException, ParseException {
Connection con = getCon();
String sql1="SELECT days from rent WHERE uid="+uid+" and bid="+bid;
Statement statement = con.createStatement();
ResultSet resultSet = statement.executeQuery(sql1);
resultSet.next();
String nowtime= resultSet.getString(1);
SimpleDateFormat format = new SimpleDateFormat("yyyy-MM-dd");
Date parse = format.parse(nowtime);
Calendar instance = Calendar.getInstance();
instance.setTime(parse);
instance.add(Calendar.DAY_OF_MONTH,days);
String afterdays = format.format(instance.getTime());
String sql2="UPDATE rent SET days=? WHERE uid=? and bid=?";
PreparedStatement prepareStatement = con.prepareStatement(sql2);
prepareStatement.setString(1,afterdays);
prepareStatement.setInt(2,uid);
prepareStatement.setInt(3,bid);
int i = prepareStatement.executeUpdate();
return i;
}
public static int addCom(String content,int bid,int uid) throws SQLException, ClassNotFoundException {
Connection con = getCon();
String sql="INSERT `comment`VALUES(?,?,?,?)";
PreparedStatement prepareStatement = con.prepareStatement(sql);
prepareStatement.setInt(1,AutoId());
prepareStatement.setString(2,content);
prepareStatement.setInt(3,uid);
prepareStatement.setInt(4,bid);
int i = prepareStatement.executeUpdate();
prepareStatement.close();
con.close();
return i;
}
public static List<String> getComById(int bid) throws SQLException, ClassNotFoundException {
ArrayList<String> list = new ArrayList<>();
Connection con = getCon();
Statement statement = con.createStatement();
String sql="SELECT * from `comment` WHERE bid="+bid;
ResultSet resultSet = statement.executeQuery(sql);
while (resultSet.next()){
String content = resultSet.getString(2);
int uid = resultSet.getInt(3);
String str=content+" By [user:"+uid+"]";
list.add(str);
}
statement.close();
con.close();
return list;
}
public static int RegisterUser(String uname,String passwd) throws SQLException, ClassNotFoundException {
Connection con = getCon();
String sql="insert user values(?,?,?,?)";
PreparedStatement prepareStatement = con.prepareStatement(sql);
prepareStatement.setString(1,passwd);
prepareStatement.setString(2,uname);
int i = prepareStatement.executeUpdate();
return i;
}
}
登录页面类:
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class Login {
public void loginUi(){
JFrame frame = new JFrame();
//设置窗体对象的属性值
frame.setTitle("Login");//设置窗体标题
frame.setSize(400, 250);//设置窗体大小,只对顶层容器生效
frame.setDefaultCloseOperation(3);//设置窗体关闭操作,3表示关闭窗体退出程序
frame.setLocationRelativeTo(null);//设置窗体相对于另一组间的居中位置,参数null表示窗体相对于屏幕的中央位置
frame.setResizable(false);//禁止调整窗体大小
frame.setFont(new Font("宋体",Font.PLAIN,14));//设置字体,显示格式正常,大小
FlowLayout fl = new FlowLayout(FlowLayout.CENTER,10,10);
//实例化流式布局类的对象
frame.setLayout(fl);
//实例化JLabel标签对象,该对象显示“账号”
JLabel labname = new JLabel("账号id:");
labname.setFont(new Font("宋体",Font.PLAIN,14));
frame.add(labname);
JTextField text_name = new JTextField();
Dimension dim1 = new Dimension(300,30);
text_name.setPreferredSize(dim1);//设置除顶级容器组件以外其他组件的大小
frame.add(text_name);
JLabel labpass = new JLabel("密码:");
labpass.setFont(new Font("宋体",Font.PLAIN,14));
frame.add(labpass);
JPasswordField text_password = new JPasswordField();
//设置大小
text_password.setPreferredSize(dim1);
frame.add(text_password);
JButton button1 = new JButton();
JButton button2 = new JButton("注册");
Dimension dim2 = new Dimension(100,30);
button1.setText("登录");
button1.setFont(new Font("宋体",Font.PLAIN,14));
button2.setFont(new Font("宋体",Font.PLAIN,14));
button1.setSize(dim2);
button2.setSize(dim2);
button2.addActionListener(
new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
new Register().reg();
}
}
);
frame.add(button1);
frame.add(button2);
frame.setVisible(true);
CListener listener = new CListener(frame, text_name, text_password);
button1.addActionListener(listener);
}
}
效果:
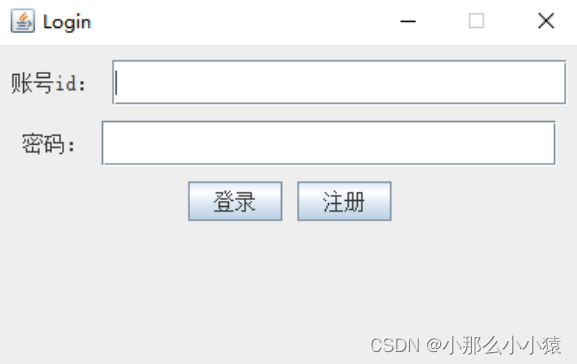
登录按钮的监听类:
import code6.entity.User;
import code6.menu.Common;
import code6.menu.MangerMenu;
import code6.utils.SqlUtils;
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.sql.SQLException;
public class CListener implements ActionListener {
JFrame login;
JTextField text_name;
JPasswordField text_passwd;
public CListener() {
}
public CListener(JFrame login, JTextField text_name, JPasswordField text_passwd) {
this.login = login;
this.text_name = text_name;
this.text_passwd = text_passwd;
}
@Override
public void actionPerformed(ActionEvent e) {
Dimension dim3 = new Dimension(300,30);
JFrame login2 = new JFrame();
login2.setSize(400,200);
login2.setDefaultCloseOperation(3);
login2.setLocationRelativeTo(null);
login2.setFont(new Font("宋体",Font.PLAIN,14)); //宋体,正常风格,14号字体
JPanel jp1 = new JPanel();
JPanel jp2 = new JPanel();
User user = new User();
try {
user= SqlUtils.getUserById(Integer.parseInt(text_name.getText()));
} catch (SQLException throwables) {
throwables.printStackTrace();
} catch (ClassNotFoundException classNotFoundException) {
classNotFoundException.printStackTrace();
}
String passwd=user.getPasswd();
if(text_passwd.getText().equals(passwd)) {
JLabel message = new JLabel("登陆成功!");
message.setFont(new Font("宋体", Font.PLAIN, 14)); //宋体,正常风格,14号字体
message.setPreferredSize(dim3);
jp1.add(message);
login2.add(jp1, BorderLayout.CENTER);
login2.setResizable(false);
login2.setVisible(true);
login.dispose();
if(user.getIsmanger()==1){
new MangerMenu().ui(user.getId());
}else
{
new Common().ui(user.getId());
}
}
else {
JLabel message = new JLabel("账号或密码错误");
message.setFont(new Font("宋体",Font.PLAIN,14));
message.setPreferredSize(dim3);
jp1.add(message);
login2.add(jp1,BorderLayout.CENTER);
JButton close = new JButton("确定");
close.setFont(new Font("宋体",Font.PLAIN,14));
close.setSize(dim3);
jp2.add(close);
login2.add(jp2,BorderLayout.SOUTH);
close.addActionListener(new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
login2.dispose();
}
});
login2.setResizable(false);
login2.setVisible(true);
login.dispose();
}
}
}
注册按钮监听类:
import code6.utils.SqlUtils;
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.sql.SQLException;
public class Register {
public static void reg(){
JFrame f = new JFrame("注册普通用户");
JPanel jPanel = new JPanel();
JTextField tname = new JTextField();
JTextField tpasswd = new JTextField();
Dimension dimension = new Dimension(100, 30);
JLabel lname= new JLabel("用户名");
JLabel lpassd= new JLabel("密码");
lname.setFont(new Font("宋体",Font.PLAIN,14));
lpassd.setFont(new Font("宋体",Font.PLAIN,14));
tname.setPreferredSize(dimension);
tpasswd.setPreferredSize(dimension);
JButton jbutton = new JButton("注册");
jbutton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String bname=null;
String passwd=null;
bname=tname.getText();
passwd= tpasswd.getText();
int i=0;
try {
i=SqlUtils.RegisterUser(bname,passwd);
} catch (SQLException throwables) {
throwables.printStackTrace();
} catch (ClassNotFoundException classNotFoundException) {
classNotFoundException.printStackTrace();
}
if(i>0){
System.out.println("注册成功");
}else
{
System.out.println("注册失败");
}
}
});
jPanel.add(lname);
jPanel.add(tname);
jPanel.add(lpassd);
jPanel.add(tpasswd);
jPanel.add(jbutton);
f.add(jPanel);
f.setSize(700,300);
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
f.setVisible(true);
}
}
登录成功后 普通用户的菜单界面类:
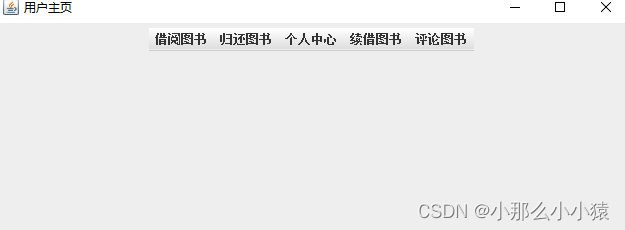
import code6.menu.com.BorrowBook;
import code6.menu.com.ContinueBook;
import code6.menu.com.PersonalCenter;
import code6.menu.com.SendBook;
import javax.swing.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.sql.SQLException;
public class Common {
public void ui(int uid) {
JFrame jframe = new JFrame("用户主页");
jframe.setBounds(300, 180, 650, 500);
JPanel jPanel = new JPanel();
JMenuBar jmenuBar=new JMenuBar();
JMenu sf = new JMenu("借阅图书");
JMenu af = new JMenu("归还图书");
JMenu df = new JMenu("个人中心");
JMenu uf = new JMenu("续借图书");
JMenuItem d1 = new JMenuItem("借阅");
JMenuItem d2 = new JMenuItem("归还");
JMenuItem d3 = new JMenuItem("租借记录");
JMenuItem d4 = new JMenuItem("续借");
d1.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
try {
new BorrowBook().add(uid);
} catch (SQLException throwables) {
throwables.printStackTrace();
} catch (ClassNotFoundException classNotFoundException) {
classNotFoundException.printStackTrace();
}
}
});
d2.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
new SendBook().send();
}
});
d3.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
try {
new PersonalCenter().center(uid);
} catch (SQLException throwables) {
throwables.printStackTrace();
} catch (ClassNotFoundException classNotFoundException) {
classNotFoundException.printStackTrace();
}
}
});
d4.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
new ContinueBook().con(uid);
}
});
af.add(d2);
df.add(d3);
sf.add(d1);
uf.add(d4);
jmenuBar.add(sf);
jmenuBar.add(af);
jmenuBar.add(df);
jmenuBar.add(uf);
jPanel.add(jmenuBar);
jframe.add(jPanel);
jframe.setVisible(true);
jframe.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
public static void main(String[] args) {
new Common().ui(1);
}
}
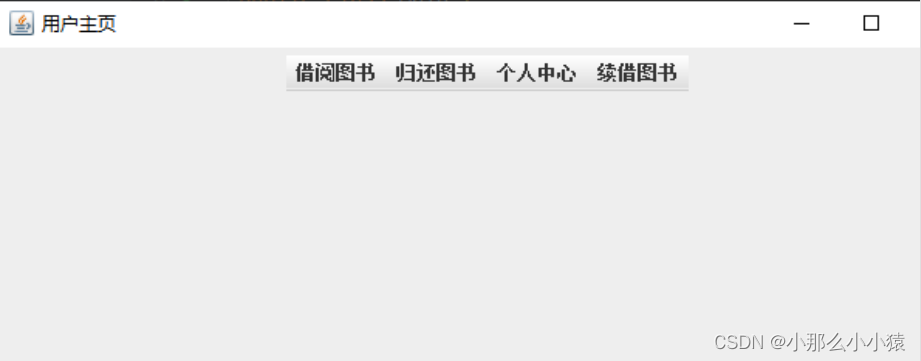
借书功能类:

import code6.menu.manger.SelectBook;
import code6.utils.SqlUtils;
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.sql.SQLException;
public class BorrowBook {
public void add(int uid) throws SQLException, ClassNotFoundException {
JFrame f = new JFrame("借阅书籍");
JPanel jPanel = new JPanel();
JTextField tid = new JTextField();
Dimension dimension = new Dimension(100, 30);
new SelectBook().comTable();
JLabel lid= new JLabel("图书id");
JLabel lgroup= new JLabel("月份");
lid.setFont(new Font("宋体",Font.PLAIN,14));
lgroup.setFont(new Font("宋体",Font.PLAIN,14));
tid.setPreferredSize(dimension);
JLabel lday= new JLabel("天数(0< and <30)");
JComboBox<String> box2 = new JComboBox<>();
for (int i=0;i<30;i++){
box2.addItem(i+"天");
}
JComboBox<String> box1 = new JComboBox<>();
box1.addItem("0个月");
box1.addItem("1个月");
box1.addItem("2个月");
JButton jbutton = new JButton("添加");
jbutton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
int id= Integer.parseInt(tid.getText());
System.out.println(id);
int months= Integer.parseInt(box1.getSelectedItem().toString().substring(0,1));
int days= Integer.parseInt(box2.getSelectedItem().toString().substring(0,1));
int i=0;
try {
if(SqlUtils.IsRent(uid,id))
{
if(SqlUtils.isAddRend(id)){
i=SqlUtils.addRent(uid,id,days,months);
}
}
} catch (SQLException throwables) {
throwables.printStackTrace();
} catch (ClassNotFoundException classNotFoundException) {
classNotFoundException.printStackTrace();
}
String res= i>0?"借阅成功":"借阅失败";
System.out.println(res);
}
});
jPanel.add(lid);
jPanel.add(tid);
jPanel.add(lgroup);
jPanel.add(box1);
jPanel.add(lday);
jPanel.add(box2);
jPanel.add(jbutton);
f.add(jPanel);
f.setSize(700,300);
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
f.setVisible(true);
}
}
评论书籍类:
!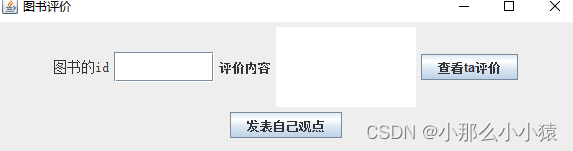
import code6.utils.SqlUtils;
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.sql.SQLException;
import java.text.ParseException;
import java.util.List;
public class CommentBook {
public void comment(int uid){
JFrame f = new JFrame("图书评价");
JPanel jPanel = new JPanel();
JLabel lid= new JLabel("图书的id");
lid.setFont(new Font("宋体",Font.PLAIN,14));
JTextField tid = new JTextField();
Dimension dimension = new Dimension(100, 30);
tid.setPreferredSize(dimension);
JLabel lcon = new JLabel("评价内容");
JTextArea tcon = new JTextArea(5,20);
JTextArea tta = new JTextArea(10, 20);
tta.setFont(new Font("宋体",Font.PLAIN,14));
tta.setVisible(false);
// JTextField tcon = new JTextField();
JButton jbutton = new JButton("发表自己观点");
tcon.setFont(new Font("宋体",Font.PLAIN,14));
// lcon.setFont(new Font("宋体",Font.PLAIN,14));
tcon.setPreferredSize(dimension);
JButton jbutton2 = new JButton("查看ta评价");
jbutton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
int i = 0;
int bid= Integer.parseInt(tid.getText());
try {
if(SqlUtils.IsRent(uid,bid)){
i=SqlUtils.addCom(tcon.getText(),bid,uid);
}
} catch (SQLException throwables) {
throwables.printStackTrace();
} catch (ClassNotFoundException classNotFoundException) {
classNotFoundException.printStackTrace();
}
if(i>0){
System.out.println("评价成功");
}
else {
System.out.println("评价失败");
}
}
});
jbutton2.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
int bid= Integer.parseInt(tid.getText());
try {
System.out.println(SqlUtils.IsRent(uid, bid));
List<String> com = SqlUtils.getComById(bid);
tta.setText("");
for (int i=0;i<com.size();i++){
String con=com.get(i);
tta.append(con+"\n");
}
if(com.size()==0){
tta.setText("当前书籍暂无评价");
}
tta.setVisible(true);
} catch (SQLException throwables) {
throwables.printStackTrace();
} catch (ClassNotFoundException classNotFoundException) {
classNotFoundException.printStackTrace();
}
}
});
jPanel.add(lid);
jPanel.add(tid);
jPanel.add(lcon);
jPanel.add(tcon);
jPanel.add(jbutton2);
jPanel.add(jbutton);
jPanel.add(tta);
f.add(jPanel);
f.setSize(600,200);
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
f.setVisible(true);
}
public static void main(String[] args) {
new CommentBook().comment(1);
}
}
续借功能类:
import code6.utils.SqlUtils;
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.sql.SQLException;
import java.text.ParseException;
public class ContinueBook {
public void con(int uid){
JFrame f = new JFrame("续借图书");
JPanel jPanel = new JPanel();
JLabel lid= new JLabel("图书id");
lid.setFont(new Font("宋体",Font.PLAIN,14));
JTextField tid = new JTextField();
Dimension dimension = new Dimension(100, 30);
tid.setPreferredSize(dimension);
JButton jbutton = new JButton("续借");
JComboBox<String> box1 = new JComboBox<>();
for (int i=1;i<=30;i++){
box1.addItem(i+"天");
}
jbutton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
int i = 0;
int days= Integer.parseInt(box1.getSelectedItem().toString().substring(0,1));
int bid= Integer.parseInt(tid.getText());
try {
i = SqlUtils.contineDays(bid, uid, days);
} catch (SQLException throwables) {
throwables.printStackTrace();
} catch (ClassNotFoundException classNotFoundException) {
classNotFoundException.printStackTrace();
} catch (ParseException parseException) {
parseException.printStackTrace();
}
if(i>0){
System.out.println("续借成功");
}
else {
System.out.println("续借失败");
}
}
});
jPanel.add(lid);
jPanel.add(tid);
jPanel.add(box1);
jPanel.add(jbutton);
f.add(jPanel);
f.setSize(600,200);
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
f.setVisible(true);
}
}
个人中心 负责查看自己所借书的功能类:

import code6.utils.SqlUtils;
import javax.swing.*;
import java.awt.*;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class PersonalCenter {
public void center(int uid) throws SQLException, ClassNotFoundException {
JFrame f = new JFrame("个人中心");
f.setSize(800, 300);
f.setLocation(200, 200);
f.setLayout(new BorderLayout());
String[] th = new String[]{ "书籍id", "书籍名称","类型", "借入时间","归还日期"};
List<Map> centosByUid = SqlUtils.getCentosByUid(uid);
HashMap<String, String> map = new HashMap<>();
String[][] td=new String[centosByUid.size()][];
for (int i=0;i<td.length;i++) {
map= (HashMap<String, String>) centosByUid.get(i);
td[i] = new String[5];
td[i][0] = map.get("bname");
td[i][1] = map.get("bid");
td[i][2] = map.get("type");
td[i][3] = map.get("btime");
td[i][4] = map.get("days");
}
JTable t = new JTable(td, th);
JScrollPane sp = new JScrollPane(t);
f.add(sp, BorderLayout.CENTER);
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
f.setVisible(true);
}
public static void main(String[] args) {
try {
new PersonalCenter().center(1);
} catch (SQLException throwables) {
throwables.printStackTrace();
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
}
还书功能类:
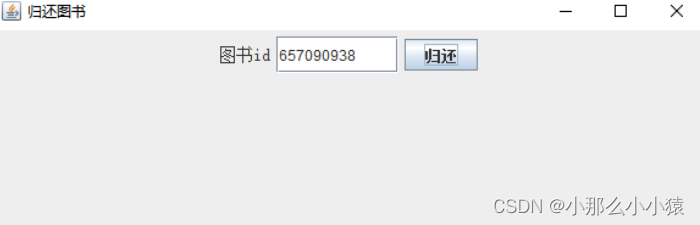
import code6.utils.SqlUtils;
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.sql.SQLException;
public class SendBook {
public void send(){
JFrame f = new JFrame("归还图书");
JPanel jPanel = new JPanel();
JLabel lid= new JLabel("图书id");
lid.setFont(new Font("宋体",Font.PLAIN,14));
JTextField tid = new JTextField();
Dimension dimension = new Dimension(100, 30);
tid.setPreferredSize(dimension);
JButton jbutton = new JButton("归还");
jbutton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
int i = 0;
try {
i= SqlUtils.delBook(Integer.parseInt(tid.getText()));
} catch (SQLException throwables) {
throwables.printStackTrace();
} catch (ClassNotFoundException classNotFoundException) {
classNotFoundException.printStackTrace();
}
if(i>0){
System.out.println("归还成功");
}
else {
System.out.println("归还失败");
}
}
});
jPanel.add(lid);
jPanel.add(tid);
jPanel.add(jbutton);
f.add(jPanel);
f.setSize(600,200);
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
f.setVisible(true);
}
}
管理员页面:

import code6.menu.manger.AddBook;
import code6.menu.manger.DelBook;
import code6.menu.manger.SelectBook;
import code6.menu.manger.UpdateBook;
import javax.swing.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.sql.SQLException;
public class MangerMenu {
public void ui(int uid) {
JFrame jframe = new JFrame("管理员个人主页");
jframe.setBounds(300, 180, 650, 500);
JPanel jPanel = new JPanel();
JMenuBar jmenuBar=new JMenuBar();
JMenu sf = new JMenu("查看所有书籍");
JMenu af = new JMenu("添加书籍");
JMenu df = new JMenu("删除书籍");
JMenu uf = new JMenu("修改书籍");
JMenuItem d1 = new JMenuItem("查询");
JMenuItem d2 = new JMenuItem("添加");
JMenuItem d3 = new JMenuItem("删除");
JMenuItem d4 = new JMenuItem("修改");
d1.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
try {
new SelectBook().comTable();
} catch (SQLException throwables) {
throwables.printStackTrace();
} catch (ClassNotFoundException classNotFoundException) {
classNotFoundException.printStackTrace();
}
}
});
d2.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
new AddBook().add();
}
});
d3.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
new DelBook().del();
}
});
d4.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
new UpdateBook().update();
}
});
af.add(d2);
df.add(d3);
sf.add(d1);
uf.add(d4);
jmenuBar.add(sf);
jmenuBar.add(af);
jmenuBar.add(df);
jmenuBar.add(uf);
jPanel.add(jmenuBar);
jframe.add(jPanel);
jframe.setVisible(true);
jframe.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
添加书籍功能类:

import code6.entity.Book;
import code6.utils.SqlUtils;
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.sql.SQLException;
public class AddBook {
public static void add(){
JFrame f = new JFrame("添加书籍");
JPanel jPanel = new JPanel();
JTextField textfield = new JTextField();
JTextField tnum = new JTextField();
JTextField tbrrow = new JTextField();
Dimension dimension = new Dimension(100, 30);
JLabel lname= new JLabel("书籍名称");
JLabel lnum= new JLabel("总数量");
JLabel lbrrow= new JLabel("借出数量");
JLabel lgroup= new JLabel("书籍类型");
lname.setFont(new Font("宋体",Font.PLAIN,14));
lnum.setFont(new Font("宋体",Font.PLAIN,14));
lgroup.setFont(new Font("宋体",Font.PLAIN,14));
lbrrow.setFont(new Font("宋体",Font.PLAIN,14));
tnum.setPreferredSize(dimension);
tbrrow.setPreferredSize(dimension);
textfield.setPreferredSize(dimension);
JButton jbutton = new JButton("添加");
JComboBox<String> box = new JComboBox<>();
box.addItem("小说");
box.addItem("教材");
box.addItem("科普");
box.addItem("其他");
jbutton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String bname=null;
int allnum=0;
int brrow=0;
String type=null;
bname=textfield.getText();
allnum= Integer.parseInt(tnum.getText());
brrow= Integer.parseInt(tbrrow.getText());
type=box.getSelectedItem().toString();
Book book = new Book(bname,null,allnum,brrow,type);
int i=0;
try {
i=SqlUtils.addBook(book);
} catch (SQLException throwables) {
throwables.printStackTrace();
} catch (ClassNotFoundException classNotFoundException) {
classNotFoundException.printStackTrace();
}
if(i>0){
System.out.println("添加成功");
}else
{
System.out.println("添加失败");
}
}
});
jPanel.add(lname);
jPanel.add(textfield);
jPanel.add(lnum);
jPanel.add(tnum);
jPanel.add(lbrrow);
jPanel.add(tbrrow);
jPanel.add(lgroup);
jPanel.add(box);
jPanel.add(jbutton);
f.add(jPanel);
f.setSize(700,300);
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
f.setVisible(true);
}
}
点击查看所有书籍:
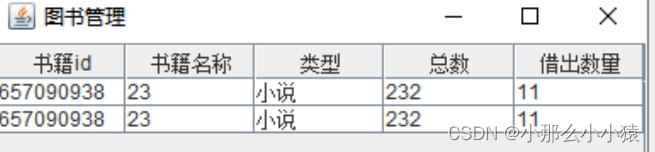
import code6.entity.Book;
import code6.utils.SqlUtils;
import javax.swing.*;
import java.awt.*;
import java.sql.SQLException;
import java.util.List;
public class SelectBook {
public static void comTable() throws SQLException, ClassNotFoundException {
JFrame f = new JFrame("图书管理");
f.setSize(400, 300);
f.setLocation(200, 200);
f.setLayout(new BorderLayout());
String[] th = new String[]{ "书籍id", "书籍名称","类型", "总数","借出数量"};
List<Book> all = SqlUtils.getAll();
String[][] td=new String[all.size()][];
Book book = new Book();
for (int i=0;i<td.length;i++){
td[i]=new String[5];
book=all.get(i);
td[i][0]= String.valueOf(book.getBid());
td[i][1]=book.getBname();
td[i][2]=book.getType();
td[i][3]= String.valueOf(book.getAllnum());
td[i][4]= String.valueOf(book.getBorrownum());
}
JTable t = new JTable(td, th);
JScrollPane sp = new JScrollPane(t);
f.add(sp, BorderLayout.CENTER);
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
f.setVisible(true);
}
}
删除书籍

import code6.utils.SqlUtils;
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.sql.SQLException;
public class DelBook {
public void del(){
JFrame f = new JFrame("删除图书");
JPanel jPanel = new JPanel();
JLabel lid= new JLabel("图书id");
lid.setFont(new Font("宋体",Font.PLAIN,14));
JTextField tid = new JTextField();
Dimension dimension = new Dimension(100, 30);
tid.setPreferredSize(dimension);
JButton jbutton = new JButton("删除");
jbutton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
int i = 0;
try {
i= SqlUtils.delBook(Integer.parseInt(tid.getText()));
} catch (SQLException throwables) {
throwables.printStackTrace();
} catch (ClassNotFoundException classNotFoundException) {
classNotFoundException.printStackTrace();
}
if(i>0){
System.out.println("删除成功");
}
else {
System.out.println("删除失败");
}
}
});
jPanel.add(lid);
jPanel.add(tid);
jPanel.add(jbutton);
f.add(jPanel);
f.setSize(600,200);
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
f.setVisible(true);
}
}
更新书籍:

import code6.entity.Book;
import code6.utils.SqlUtils;
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.sql.SQLException;
public class UpdateBook {
public void update(){
JFrame f = new JFrame("修改书籍");
JPanel jPanel = new JPanel();
JTextField textfield = new JTextField();
JTextField tnum = new JTextField();
JTextField tid = new JTextField();
JTextField tbrrow = new JTextField();
Dimension dimension = new Dimension(100, 30);
JLabel lbid = new JLabel("要修改的图书id");
JLabel lname= new JLabel("书籍名称");
JLabel lnum= new JLabel("总数量");
JLabel lbrrow= new JLabel("借出数量");
JLabel lgroup= new JLabel("书籍类型");
lbid.setFont(new Font("宋体",Font.PLAIN,14));
lname.setFont(new Font("宋体",Font.PLAIN,14));
lnum.setFont(new Font("宋体",Font.PLAIN,14));
lgroup.setFont(new Font("宋体",Font.PLAIN,14));
lbrrow.setFont(new Font("宋体",Font.PLAIN,14));
tnum.setPreferredSize(dimension);
tbrrow.setPreferredSize(dimension);
textfield.setPreferredSize(dimension);
tid.setPreferredSize(dimension);
JButton jbutton = new JButton("修改");
JComboBox<String> box = new JComboBox<>();
box.addItem("小说");
box.addItem("教材");
box.addItem("科普");
box.addItem("其他");
jbutton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
int bid=0;
String bname=null;
int allnum=0;
int brrow=0;
String type=null;
bid= Integer.parseInt(tid.getText());
bname=textfield.getText();
allnum= Integer.parseInt(tnum.getText());
brrow= Integer.parseInt(tbrrow.getText());
type=box.getSelectedItem().toString();
Book book = new Book();
book.setBid(bid);
book.setBorrownum(brrow);
book.setType(type);
book.setBname(bname);
book.setAllnum(allnum);
System.out.println(book);
int i=0;
try {
SqlUtils.upBook(book);
} catch (SQLException throwables) {
throwables.printStackTrace();
} catch (ClassNotFoundException classNotFoundException) {
classNotFoundException.printStackTrace();
}
if(i>0){
System.out.println("修改成功");
}else
{
System.out.println("修改失败");
}
}
});
jPanel.add(lbid);
jPanel.add(tid);
jPanel.add(lname);
jPanel.add(textfield);
jPanel.add(lnum);
jPanel.add(tnum);
jPanel.add(lbrrow);
jPanel.add(tbrrow);
jPanel.add(lgroup);
jPanel.add(box);
jPanel.add(jbutton);
f.add(jPanel);
f.setSize(700,300);
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
f.setVisible(true);
}
public static void main(String[] args) {
new UpdateBook().update();
}
}
到此完成