环境配置
delve仓库,含有教程:https://github.com/go-delve/delve
golang的debugging教程:https://github.com/golang/vscode-go/wiki/debugging
> go version
go version go1.20 windows/amd64
> go install github.com/go-delve/delve/cmd/dlv@latest
快捷键
F8 下一步
F7 进入函数
Shift+F8 退出函数
F9 下一个断点
golang的调试有三种模式
1、Launch package
// Launch package: Debug/test the package of the open file
{
"name": "Launch Package",
"type": "go",
"request": "launch",
"mode": "auto",
"args": ["new", " conda"],
"program": "${fileDirname}"
}
从说明上看出这是调试当前打开的文件所在的包,所以当前打开的文件需要是main包,里面有main函数,或者是测试文件,也就是需要可直接运行的文件。类比于我们直接进入该目录执行go run
可以带上运行参数 args
测试程序
ctl/
ctl.go
package main
import (
"fmt"
"voteapi/ctl/cmd"
)
func main() {
fmt.Println("xxx")
cmd.Execute()
}
在fmt.Println("xxx")
打上断点,因为调试器会直接跳到第一个断点的地方,如果一个断点都没有那就直接结束了。
控制台输出
Starting: D:\dev\php\magook\trunk\server\golang\path\bin\dlv.exe dap --listen=127.0.0.1:20155 from D:\dev\php\magook\trunk\server\voteapi\ctl
DAP server listening at: 127.0.0.1:20155
Type 'dlv help' for list of commands.
mode
参数的值
-
debug
: build and debug a main package
-
test
: build and debug a test
-
exec
: debug a precompiled binary
- The binary must be built with
go build -gcflags=all="-N -l"
to disable inlining and optimizations that can interfere with debugging.
-
auto
: automatically choose between debug
and test
depending on the open file
2、Attach to process
// Attach to local process: Attach to an existing process by process ID
{
"name": "Attach to Process",
"type": "go",
"request": "attach",
"mode": "local",
"processId": 0
}
attach: You can use this configuration to attach to a running process or a running debug session.
mode
参数的值
- local: attaches to a local process.
- The binary must be built with
go build -gcflags=all="-N -l"
to disable inlining and optimizations that can interfere with debugging.
-
remote
: attaches to an in-progress debug session run by an external server.
You can debug an already running program using the local
mode type configuration. The Go extension will start dlv dap
and configure it to attach to the specified process. Users can select the process to debug with one of the following options:
- Specifying the numeric process id (PID) with the
processId
attribute.
- Specifying the target program name in the
processId
attribute. If there are multiple processes matching the specified program name, the extension will show the list of matching processes at the start of the debug session.
- Specifying
0
in the processId
attribute and selecting the process from the drop-down menu at the start of the debug session.
attach 的 local 模式的应用场景是常驻内存的程序,比如 http server,我们编译运行程序后,来到VScode,主要配置 processId 属性,可以是进程ID,可以是进程名称,也可以给0,然后编辑器会弹出下拉框让你选择要attach上的程序。
示例,还是 ctl.go 文件,改一下
package main
import (
"fmt"
"net/http"
)
func main() {
http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) {
fmt.Fprintf(w, "%s", "hello")
})
http.ListenAndServe(":9999", nil)
}
go build ctl.go
ctl.exe
我们选择Attach to Process
,点击运行调试三角形按钮,在弹出的下拉框输入ctl.exe
,启动调试,同样的要先打几个断点。

此时调试工具栏是这样的

是出于暂停状态的,我们在浏览器请求一下这个服务,它就被激活了,就可以逐步调试了。
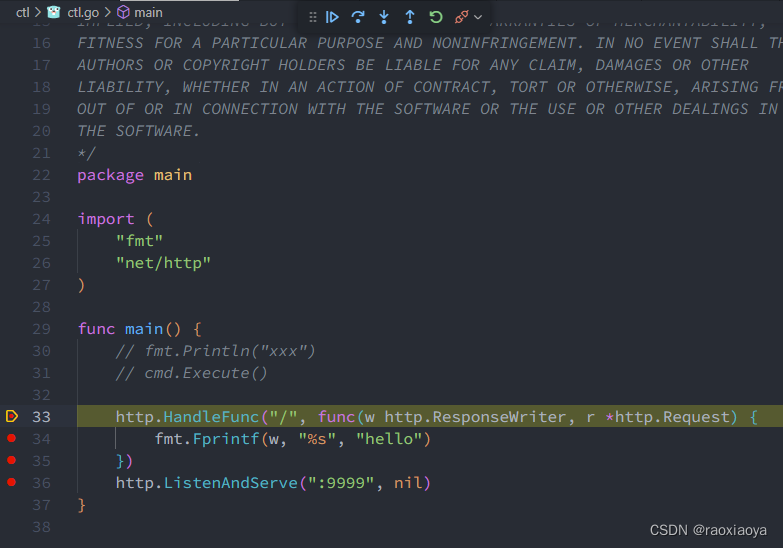
一个请求被调试完之后,调试不会结束,而是暂停了,等待下一个请求来触发。
3、Connect to server
说明 https://github.com/golang/vscode-go/wiki/debugging#remote-debugging
这是 vscode 的 Remote debugging 功能,他是基于 Remote Development,即远程开发,远程调试,这还需要安装额外的vscode扩展,将远程代码映射到本地,然后本地代码能时时推送到远程服务器,最终在远程服务器上运行代码。
我是直接在本地来的,发现也可以调试。
package main
import (
"fmt"
"voteapi/ctl/cmd"
)
func main() {
fmt.Println("xxx")
cmd.Execute()
}
// Connect to server: Connect to a remote headless debug server
{
"name": "Connect to server",
"type": "go",
"debugAdapter": "dlv-dap",
"request": "attach",
"mode": "remote",
"remotePath": "${workspaceFolder}",
"port": 2345,
"host": "127.0.0.1"
}
dlv debug --headless --listen=:2345
launch.json配置项
文档 https://github.com/golang/vscode-go/wiki/debugging#configuration
// 命令行参数,要自己打空格
"args": ["-config", " server.json"],
// 编译参数
"buildFlags": "-tags 'server'",
// key:value
"env": {},