目录
1、背景
2、代码
3、idea配置
4、服务端远程开启debug服务
5、远程调试
5.1 服务端
5.2 本地启动
6、注意
1、背景
线上出了问题,我们一般是通过日志来定位问题。在没有日志的情况下,往往定位问题是比较困难的。
这时我们希望线上可以和本地环境一样可以debug。可以用线上的环境,本地工具。幸运的是Java是有远程DEBUG的支持的,而且IDEA也实现了相关的功能。
2、代码
spring boot项目
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.example</groupId>
<artifactId>remote.debug</artifactId>
<version>1.0</version>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.1.7.RELEASE</version>
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
<fastjson.version>1.2.24</fastjson.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- https://mvnrepository.com/artifact/com.alibaba/fastjson -->
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
<version>${fastjson.version}</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-starter-logging -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-logging</artifactId>
</dependency>
<!-- https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-starter-test -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
</dependency>
<!-- https://mvnrepository.com/artifact/org.apache.commons/commons-lang3 -->
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<scope>provided</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
import lombok.Builder;
import lombok.Data;
/**
* @Title: User.java
* @ProjectName com.spring.pro.test.controller
* @Description:
* @author ybwei
* @date 2020年3月6日 下午6:18:36
*/
@Data
@Builder
public class User {
private Long id;
private String name;
}
import javax.annotation.Resource;
/**
* @Description:
* @Param:
* @Return:
* @Author: ybwei
* @Date: 2020/10/27
**/
@RestController
@Slf4j
public class TestController {
/**
* @Description:测试延迟加载
* @Author: ybwei
* @Date: 2020/7/31
**/
@Resource
private UserService userService;
/**
* @Description:
* @Param: []
* @Return: com.cloud.model.User
* @Author: ybwei
* @Date: 2020/7/31
**/
@GetMapping("/getUser")
public User getUser(Long id){
return userService.getUser(id);
}
}
import com.cloud.model.User;
/**
* @ClassName UserService
* @Description:
* @Author ybwei
* @Date 2020/7/31
* @Version V1.0
**/
public interface UserService {
/**
* @Description:
* @Param: [id]
* @Return: com.cloud.model.User
* @Author: ybwei
* @Date: 2020/7/31
**/
User getUser(Long id);
}
import com.cloud.model.User;
import com.cloud.service.UserService;
import lombok.extern.slf4j.Slf4j;
import org.springframework.stereotype.Component;
/**
* @ClassName UserServiceImpl
* @Description:
* @Author ybwei
* @Date 2020/7/31
* @Version V1.0
**/
@Slf4j
@Component
public class UserServiceImpl implements UserService {
@Override
public User getUser(Long id) {
log.info("id:{}",id);
return User.builder()
.id(id)
.name("张三"+id)
.build();
}
}
3、idea配置
点击主窗口菜单 Run / Edit Configurations,打开“Run/Debug Configurations”窗口
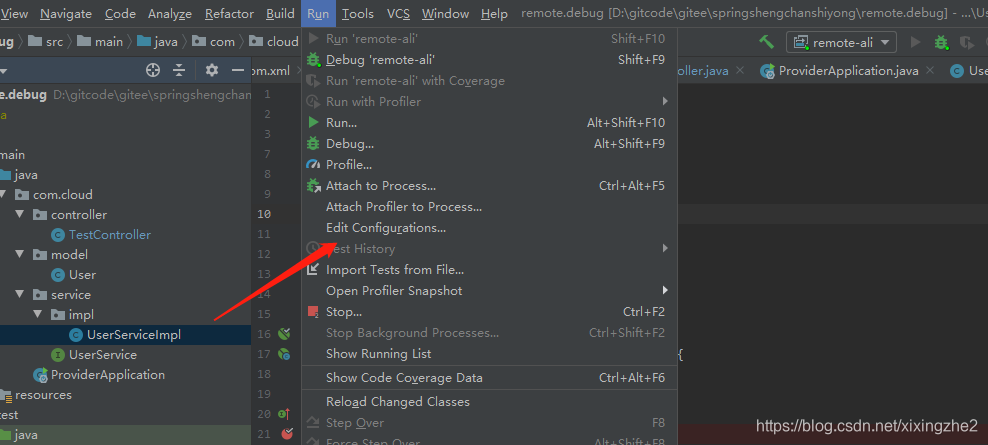
点击工具栏上的“+”按钮,下拉菜单中选择“Remote”
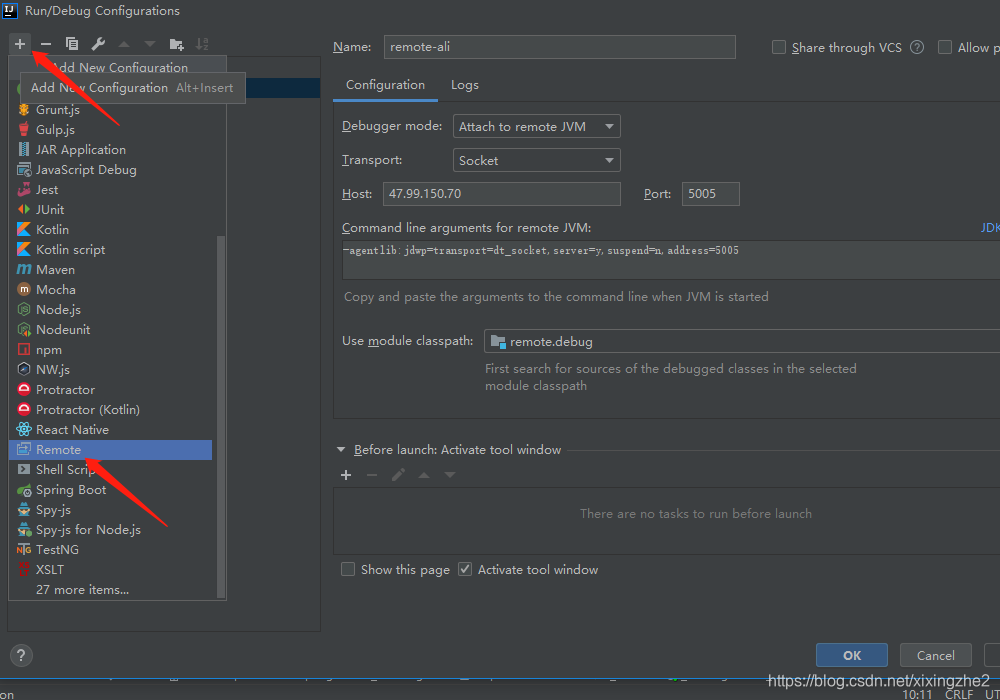
设置 Host 为远程服务器的域名或IP,保持 Port=5005 无需调整。如果Command line没有,一般默认会有值,复制命令行参数,形如 -agentlib:jdwp=transport=dt_socket,server=y,suspend=n,address=5005。
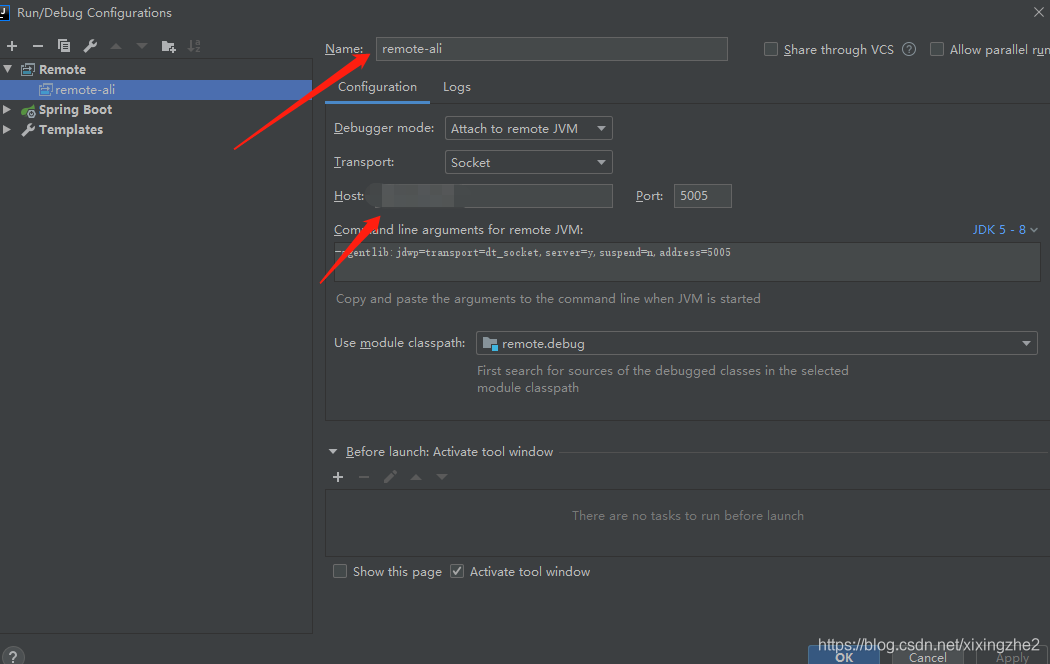
4、服务端远程开启debug服务
编写启动脚本start.sh
java -Xdebug -Xnoagent -Djava.compiler=NONE -Xrunjdwp:transport=dt_socket,server=y,suspend=n,address=5005 -jar remote.debug-1.0.jar
参数说明
-
-Xdebug:JVM在DEBUG模式下工作;
-
-Xrunjdwp:JVM使用(java debug wire protocol)来运行调试环境;
-
transport:监听Socket端口连接方式,常用的dt_socket表示使用socket连接.
-
server:=y表示当前是调试服务端,=n表示当前是调试客户端;
-
suspend:=n表示启动时不中断.
-
address:=5005表示本地监听5005端口。调试时防火墙要打开这个端口。
5、远程调试
5.1 服务端
执行start.sh
Listening for transport dt_socket at address: 5005
14:51:04,804 |-INFO in ch.qos.logback.classic.LoggerContext[default] - Could NOT find resource [logback-test.xml]
14:51:04,804 |-INFO in ch.qos.logback.classic.LoggerContext[default] - Could NOT find resource [logback.groovy]
14:51:04,804 |-INFO in ch.qos.logback.classic.LoggerContext[default] - Found resource [logback.xml] at [jar:file:/root/remote/remote.debug-1.0.jar!/BOOT-INF/classes!/logback.xml]
14:51:04,847 |-INFO in ch.qos.logback.core.joran.spi.ConfigurationWatchList@74294adb - URL [jar:file:/root/remote/remote.debug-1.0.jar!/BOOT-INF/classes!/logback.xml] is not of type file
14:51:04,952 |-INFO in ch.qos.logback.classic.joran.action.ConfigurationAction - debug attribute not set
14:51:04,954 |-INFO in ch.qos.logback.core.joran.action.AppenderAction - About to instantiate appender of type [ch.qos.logback.core.ConsoleAppender]
14:51:04,976 |-INFO in ch.qos.logback.core.joran.action.AppenderAction - Naming appender as [stdout]
14:51:04,991 |-INFO in ch.qos.logback.core.joran.action.NestedComplexPropertyIA - Assuming default type [ch.qos.logback.classic.encoder.PatternLayoutEncoder] for [encoder] property
14:51:05,083 |-INFO in ch.qos.logback.core.joran.action.AppenderAction - About to instantiate appender of type [ch.qos.logback.core.rolling.RollingFileAppender]
14:51:05,089 |-INFO in ch.qos.logback.core.joran.action.AppenderAction - Naming appender as [InfoFile]
14:51:05,121 |-INFO in c.q.l.core.rolling.TimeBasedRollingPolicy@1890187342 - No compression will be used
14:51:05,123 |-INFO in c.q.l.core.rolling.TimeBasedRollingPolicy@1890187342 - Will use the pattern d:/logs/info/info-%d{yyyy-MM-dd}.%i.log for the active file
14:51:05,127 |-INFO in ch.qos.logback.core.rolling.SizeAndTimeBasedFNATP@130f889 - The date pattern is 'yyyy-MM-dd' from file name pattern 'd:/logs/info/info-%d{yyyy-MM-dd}.%i.log'.
14:51:05,127 |-INFO in ch.qos.logback.core.rolling.SizeAndTimeBasedFNATP@130f889 - Roll-over at midnight.
14:51:05,131 |-INFO in ch.qos.logback.core.rolling.SizeAndTimeBasedFNATP@130f889 - Setting initial period to Tue Oct 27 14:51:05 CST 2020
14:51:05,131 |-WARN in ch.qos.logback.core.rolling.SizeAndTimeBasedFNATP@130f889 - SizeAndTimeBasedFNATP is deprecated. Use SizeAndTimeBasedRollingPolicy instead
14:51:05,131 |-WARN in ch.qos.logback.core.rolling.SizeAndTimeBasedFNATP@130f889 - For more information see http://logback.qos.ch/manual/appenders.html#SizeAndTimeBasedRollingPolicy
14:51:05,135 |-WARN in ch.qos.logback.core.rolling.RollingFileAppender[InfoFile] - This appender no longer admits a layout as a sub-component, set an encoder instead.
14:51:05,135 |-WARN in ch.qos.logback.core.rolling.RollingFileAppender[InfoFile] - To ensure compatibility, wrapping your layout in LayoutWrappingEncoder.
14:51:05,135 |-WARN in ch.qos.logback.core.rolling.RollingFileAppender[InfoFile] - See also http://logback.qos.ch/codes.html#layoutInsteadOfEncoder for details
14:51:05,142 |-INFO in ch.qos.logback.core.rolling.RollingFileAppender[InfoFile] - Active log file name: d:/logs/info/info.log
14:51:05,142 |-INFO in ch.qos.logback.core.rolling.RollingFileAppender[InfoFile] - File property is set to [d:/logs/info/info.log]
14:51:05,144 |-INFO in ch.qos.logback.core.joran.action.AppenderAction - About to instantiate appender of type [ch.qos.logback.core.rolling.RollingFileAppender]
14:51:05,144 |-INFO in ch.qos.logback.core.joran.action.AppenderAction - Naming appender as [ErrorFile]
14:51:05,147 |-INFO in c.q.l.core.rolling.TimeBasedRollingPolicy@294184992 - No compression will be used
14:51:05,147 |-INFO in c.q.l.core.rolling.TimeBasedRollingPolicy@294184992 - Will use the pattern d:/logs/error/error-%d{yyyy-MM-dd}.%i.log for the active file
14:51:05,147 |-INFO in ch.qos.logback.core.rolling.SizeAndTimeBasedFNATP@2f490758 - The date pattern is 'yyyy-MM-dd' from file name pattern 'd:/logs/error/error-%d{yyyy-MM-dd}.%i.log'.
14:51:05,147 |-INFO in ch.qos.logback.core.rolling.SizeAndTimeBasedFNATP@2f490758 - Roll-over at midnight.
14:51:05,148 |-INFO in ch.qos.logback.core.rolling.SizeAndTimeBasedFNATP@2f490758 - Setting initial period to Tue Oct 27 14:51:05 CST 2020
14:51:05,148 |-WARN in ch.qos.logback.core.rolling.SizeAndTimeBasedFNATP@2f490758 - SizeAndTimeBasedFNATP is deprecated. Use SizeAndTimeBasedRollingPolicy instead
14:51:05,148 |-WARN in ch.qos.logback.core.rolling.SizeAndTimeBasedFNATP@2f490758 - For more information see http://logback.qos.ch/manual/appenders.html#SizeAndTimeBasedRollingPolicy
14:51:05,148 |-WARN in ch.qos.logback.core.rolling.RollingFileAppender[ErrorFile] - This appender no longer admits a layout as a sub-component, set an encoder instead.
14:51:05,148 |-WARN in ch.qos.logback.core.rolling.RollingFileAppender[ErrorFile] - To ensure compatibility, wrapping your layout in LayoutWrappingEncoder.
14:51:05,148 |-WARN in ch.qos.logback.core.rolling.RollingFileAppender[ErrorFile] - See also http://logback.qos.ch/codes.html#layoutInsteadOfEncoder for details
14:51:05,149 |-INFO in ch.qos.logback.core.rolling.RollingFileAppender[ErrorFile] - Active log file name: d:/logs/error/error.log
14:51:05,149 |-INFO in ch.qos.logback.core.rolling.RollingFileAppender[ErrorFile] - File property is set to [d:/logs/error/error.log]
14:51:05,149 |-INFO in ch.qos.logback.core.joran.action.AppenderAction - About to instantiate appender of type [ch.qos.logback.core.rolling.RollingFileAppender]
14:51:05,149 |-INFO in ch.qos.logback.core.joran.action.AppenderAction - Naming appender as [AllFile]
14:51:05,150 |-INFO in c.q.l.core.rolling.TimeBasedRollingPolicy@270397815 - No compression will be used
14:51:05,150 |-INFO in c.q.l.core.rolling.TimeBasedRollingPolicy@270397815 - Will use the pattern d:/logs/all/all-%d{yyyy-MM-dd}.%i.log for the active file
14:51:05,151 |-INFO in ch.qos.logback.core.rolling.SizeAndTimeBasedFNATP@166fa74d - The date pattern is 'yyyy-MM-dd' from file name pattern 'd:/logs/all/all-%d{yyyy-MM-dd}.%i.log'.
14:51:05,151 |-INFO in ch.qos.logback.core.rolling.SizeAndTimeBasedFNATP@166fa74d - Roll-over at midnight.
14:51:05,152 |-INFO in ch.qos.logback.core.rolling.SizeAndTimeBasedFNATP@166fa74d - Setting initial period to Tue Oct 27 14:51:05 CST 2020
14:51:05,152 |-WARN in ch.qos.logback.core.rolling.SizeAndTimeBasedFNATP@166fa74d - SizeAndTimeBasedFNATP is deprecated. Use SizeAndTimeBasedRollingPolicy instead
14:51:05,152 |-WARN in ch.qos.logback.core.rolling.SizeAndTimeBasedFNATP@166fa74d - For more information see http://logback.qos.ch/manual/appenders.html#SizeAndTimeBasedRollingPolicy
14:51:05,152 |-WARN in ch.qos.logback.core.rolling.RollingFileAppender[AllFile] - This appender no longer admits a layout as a sub-component, set an encoder instead.
14:51:05,152 |-WARN in ch.qos.logback.core.rolling.RollingFileAppender[AllFile] - To ensure compatibility, wrapping your layout in LayoutWrappingEncoder.
14:51:05,152 |-WARN in ch.qos.logback.core.rolling.RollingFileAppender[AllFile] - See also http://logback.qos.ch/codes.html#layoutInsteadOfEncoder for details
14:51:05,153 |-INFO in ch.qos.logback.core.rolling.RollingFileAppender[AllFile] - Active log file name: d:/logs/all/all.log
14:51:05,153 |-INFO in ch.qos.logback.core.rolling.RollingFileAppender[AllFile] - File property is set to [d:/logs/all/all.log]
14:51:05,153 |-INFO in ch.qos.logback.classic.joran.action.LoggerAction - Setting level of logger [org.mybatis] to TRACE
14:51:05,154 |-INFO in ch.qos.logback.classic.joran.action.LoggerAction - Setting level of logger [com.cloud.mapper] to DEBUG
14:51:05,154 |-INFO in ch.qos.logback.classic.joran.action.RootLoggerAction - Setting level of ROOT logger to INFO
14:51:05,154 |-INFO in ch.qos.logback.core.joran.action.AppenderRefAction - Attaching appender named [stdout] to Logger[ROOT]
14:51:05,155 |-INFO in ch.qos.logback.core.joran.action.AppenderRefAction - Attaching appender named [InfoFile] to Logger[ROOT]
14:51:05,155 |-INFO in ch.qos.logback.core.joran.action.AppenderRefAction - Attaching appender named [ErrorFile] to Logger[ROOT]
14:51:05,155 |-INFO in ch.qos.logback.core.joran.action.AppenderRefAction - Attaching appender named [AllFile] to Logger[ROOT]
14:51:05,155 |-INFO in ch.qos.logback.classic.joran.action.ConfigurationAction - End of configuration.
14:51:05,156 |-INFO in ch.qos.logback.classic.joran.JoranConfigurator@40f08448 - Registering current configuration as safe fallback point
. ____ _ __ _ _
/\\ / ___'_ __ _ _(_)_ __ __ _ \ \ \ \
( ( )\___ | '_ | '_| | '_ \/ _` | \ \ \ \
\\/ ___)| |_)| | | | | || (_| | ) ) ) )
' |____| .__|_| |_|_| |_\__, | / / / /
=========|_|==============|___/=/_/_/_/
:: Spring Boot :: (v2.1.7.RELEASE)
[INFO ] 2020-10-27 14:51:05.963 [main] com.cloud.ProviderApplication - Starting ProviderApplication v1.0 on iZbp12v151n660twhkl8xvZ with PID 1827 (/root/remote/remote.debug-1.0.jar started by root in /root/remote)
[INFO ] 2020-10-27 14:51:05.969 [main] com.cloud.ProviderApplication - No active profile set, falling back to default profiles: default
[INFO ] 2020-10-27 14:51:07.807 [main] o.s.b.w.e.tomcat.TomcatWebServer - Tomcat initialized with port(s): 8080 (http)
[INFO ] 2020-10-27 14:51:07.834 [main] o.a.coyote.http11.Http11NioProtocol - Initializing ProtocolHandler ["http-nio-8080"]
[INFO ] 2020-10-27 14:51:07.856 [main] o.a.catalina.core.StandardService - Starting service [Tomcat]
[INFO ] 2020-10-27 14:51:07.864 [main] o.a.catalina.core.StandardEngine - Starting Servlet engine: [Apache Tomcat/9.0.22]
[INFO ] 2020-10-27 14:51:07.980 [main] o.a.c.c.C.[Tomcat].[localhost].[/] - Initializing Spring embedded WebApplicationContext
[INFO ] 2020-10-27 14:51:07.980 [main] o.s.web.context.ContextLoader - Root WebApplicationContext: initialization completed in 1908 ms
[INFO ] 2020-10-27 14:51:08.258 [main] o.s.s.c.ThreadPoolTaskExecutor - Initializing ExecutorService 'applicationTaskExecutor'
[INFO ] 2020-10-27 14:51:08.633 [main] o.a.coyote.http11.Http11NioProtocol - Starting ProtocolHandler ["http-nio-8080"]
[INFO ] 2020-10-27 14:51:08.658 [main] o.s.b.w.e.tomcat.TomcatWebServer - Tomcat started on port(s): 8080 (http) with context path ''
[INFO ] 2020-10-27 14:51:08.664 [main] com.cloud.ProviderApplication - Started ProviderApplication in 3.356 seconds (JVM running for 4.269)
5.2 本地启动
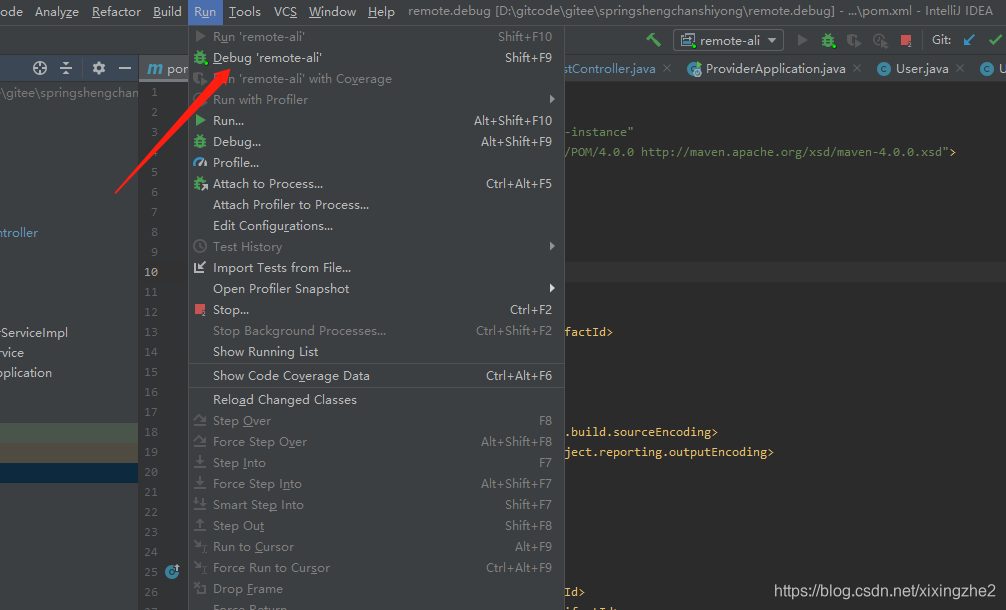
打断点
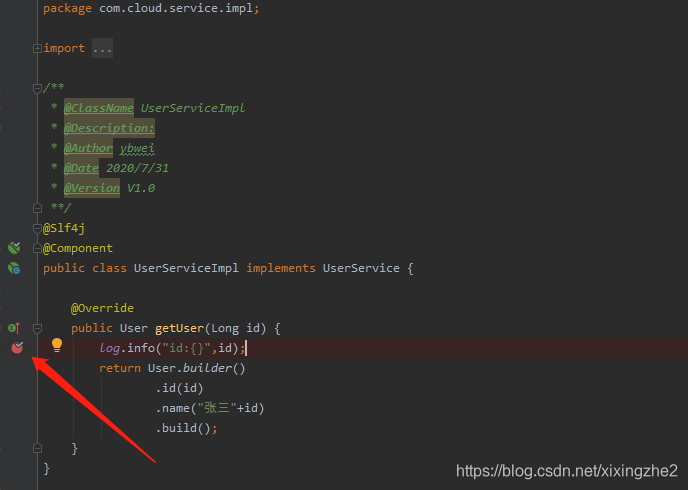
访问:http://IP:8080/getUser?id=2
IP:远程地址
就可以本地debug远程了。
6、注意
- 远程部署和本地代码一致。
- 服务端防火墙要打开address端口。服务器上多开放个端口是不安全的,调试完毕后可恢复防火墙设置。