0 概述
线程池,从字面的含义上来看,是指管理一组工作线程(Worker Thread)的资源池。线程池是与工作队列(Work Queue)密切相关的,其中在工作队列中保存了需要执行的任务。工作线程很简单:从任务队列中取出一个任务,执行任务,然后返回线程池并等待下一个任务。前面提到了线程池基本概念,那么为什么要使用线程池呢?创建线程要花费一定的的资源和时间,如果任务来了才创建线程那么响应时间会变长,而且一个进程能创建的线程数有限的。本文主要主要来分析JDK线程池的源码实现。以便我们更好的理解和使用线程池。
1 使用实例
public class ThreadPool {
//线程池初始化corePoolSize=2,maximumPoolSize=10,keepAliveTime=600s
private static Executor executor=new ThreadPoolExecutor(2, 10,
600, TimeUnit.SECONDS,
new LinkedBlockingQueue<Runnable>(10));
private static AtomicInteger atomicInteger=new AtomicInteger();
public static void main(String[] args) {
for(int i=0;i<20;i++) {
executor.execute(new Runnable() {
@Override
public void run() {
System.out.println(Thread.currentThread().getName());
}
});
}
}
}
输出:
pool-1-thread-1
pool-1-thread-2
pool-1-thread-1
pool-1-thread-2
pool-1-thread-1
pool-1-thread-2
pool-1-thread-2
pool-1-thread-2
pool-1-thread-1
pool-1-thread-2
pool-1-thread-3
pool-1-thread-2
pool-1-thread-1
pool-1-thread-2
pool-1-thread-3
pool-1-thread-2
pool-1-thread-1
pool-1-thread-1
pool-1-thread-2
pool-1-thread-3
线程池几个关键的参数说明,
1.corePoolSize 核心线程数
2.最大线程数
3.当线程池中线程数量大于corePoolSize(核心线程数量)或设置了allowCoreThreadTimeOut(是否允许空闲核心线程超时)时,线程会根据keepAliveTime的值进行活性检查,一旦超时便销毁线程
4.时间单位
5.任务队列
6.线程工厂,用于创建线程使用,建议复写,返回特定线程名,方便出问题容易追溯
7.线程池丢弃策略,异常处理的方式,可以打印出日志或者记录日志到DB、缓存、消息等
//核心线程数
int corePoolSize = 2;
//最大线程数
int maximumPoolSize = 4;
// 60s没有使用归还给OS
long keepAliveTime = 60;
ThreadPoolExecutor executor = new ThreadPoolExecutor(
corePoolSize,
maximumPoolSize,
keepAliveTime,
TimeUnit.SECONDS,
new ArrayBlockingQueue<>(97),
Executors.defaultThreadFactory(),
new ThreadPoolExecutor.DiscardOldestPolicy()
);
2 线程池工作原理图
下图给出了线程池的工作原理图,可以看出当线程数量小于corePoolSize(核心数量)数量的时候会创建新的线程;当任务队列满时候且线程数量没有达到maximumPoolSize(最大数量)时候也会创建新的线程。
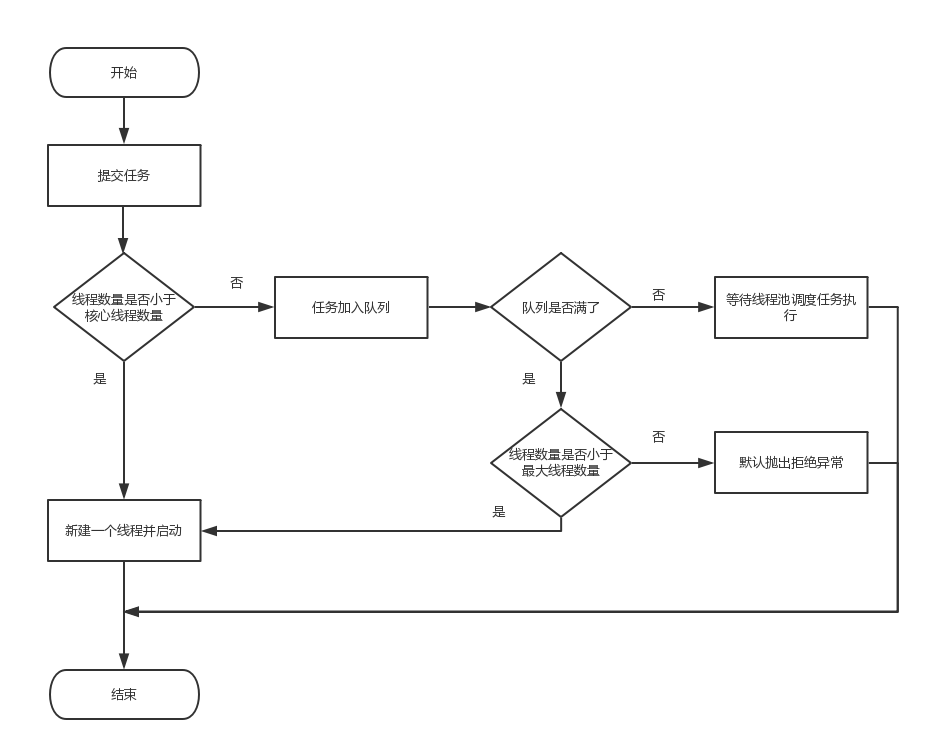
3 ThreadPoolExecutor源码分析
线程池几个重要的成员变量
- AtomicInteger类型的ctl代表了ThreadPoolExecutor中的控制状态,是一个原子整数,通过高低位包装了两个字段:1)workerCount:线程池中当前活动的线程数量,占据ctl的低29位;2)runState:线程池运行状态,占据ctl的高3位,其包含RUNNING、SHUTDOWN、STOP、TIDYING、TERMINATED五种状态,不难发现其值递增的。
private final AtomicInteger ctl = new AtomicInteger(ctlOf(RUNNING, 0));
//32-3
private static final int COUNT_BITS = Integer.SIZE - 3;
private static final int CAPACITY = (1 << COUNT_BITS) - 1;
// runState is stored in the high-order bits
//-1 左移29 位 111 00000 00000000 00000000 00000000
private static final int RUNNING = -1 << COUNT_BITS;
// 0 左移29位还是0
private static final int SHUTDOWN = 0 << COUNT_BITS;
// 1左移29位 001 00000 00000000 00000000 00000000
private static final int STOP = 1 << COUNT_BITS;
// 2左移29位 010 00000 00000000 00000000 00000000
private static final int TIDYING = 2 << COUNT_BITS;
// 3左移29位 011 00000 00000000 00000000 00000000
private static final int TERMINATED = 3 << COUNT_BITS;
//工作队列,用于存放任务
private final BlockingQueue<Runnable> workQueue;
//工作线程组,存放工作线程
private final HashSet<Worker> workers = new HashSet<Worker>();
构造函数(只是初始化赋值)
public ThreadPoolExecutor(int corePoolSize,
int maximumPoolSize,
long keepAliveTime,
TimeUnit unit,
BlockingQueue<Runnable> workQueue) {
this(corePoolSize, maximumPoolSize, keepAliveTime, unit, workQueue,
Executors.defaultThreadFactory(), defaultHandler);
}
public ThreadPoolExecutor(int corePoolSize,
int maximumPoolSize,
long keepAliveTime,
TimeUnit unit,
BlockingQueue<Runnable> workQueue,
ThreadFactory threadFactory,
RejectedExecutionHandler handler) {
if (corePoolSize < 0 ||
maximumPoolSize <= 0 ||
maximumPoolSize < corePoolSize ||
keepAliveTime < 0)
throw new IllegalArgumentException();
if (workQueue == null || threadFactory == null || handler == null)
throw new NullPointerException();
this.corePoolSize = corePoolSize;
this.maximumPoolSize = maximumPoolSize;
this.workQueue = workQueue;
this.keepAliveTime = unit.toNanos(keepAliveTime);
this.threadFactory = threadFactory;
this.handler = handler;
}
public void execute(Runnable command) {
if (command == null)
throw new NullPointerException();
/*
* Proceed in 3 steps:
*
* 1. If fewer than corePoolSize threads are running, try to
* start a new thread with the given command as its first
* task. The call to addWorker atomically checks runState and
* workerCount, and so prevents false alarms that would add
* threads when it shouldn't, by returning false.
*
* 2. If a task can be successfully queued, then we still need
* to double-check whether we should have added a thread
* (because existing ones died since last checking) or that
* the pool shut down since entry into this method. So we
* recheck state and if necessary roll back the enqueuing if
* stopped, or start a new thread if there are none.
*
* 3. If we cannot queue task, then we try to add a new
* thread. If it fails, we know we are shut down or saturated
* and so reject the task.
*/
int c = ctl.get();
//判断线程数量是否小于核心线程数量
if (workerCountOf(c) < corePoolSize) {
//创建新的线程成功后直接返回
if (addWorker(command, true))
return;
c = ctl.get();
}
//程序走到这里说明数量是小于核心线程数量或新建线程失败了
//线程池是运行状态且任务加入队列成功
if (isRunning(c) && workQueue.offer(command)) {
int recheck = ctl.get();
//如果线程不是运行状态然后移除任务
if (! isRunning(recheck) && remove(command))
reject(command);
//线程数量为0(还有任务需要执行,这时候创建一个新的线程)
else if (workerCountOf(recheck) == 0)
//重新创建一个线程(空任务)放到线程池中
addWorker(null, false);
}
//队列满了,尝试重新创建线程,注意addWorker第二参数为false了
else if (!addWorker(command, false))
//添加失败拒绝任务
reject(command);
}
addWorker 方法分析(创建一个新的线程并启动)
private boolean addWorker(Runnable firstTask, boolean core) {
retry:
for (;;) {
int c = ctl.get();
int rs = runStateOf(c);
// Check if queue empty only if necessary.
if (rs >= SHUTDOWN &&
! (rs == SHUTDOWN &&
firstTask == null &&
! workQueue.isEmpty()))
return false;
for (;;) {
//线程数量
int wc = workerCountOf(c);
//core是true 和corePoolSize比较,core是false 和 maximumPoolSize比较
if (wc >= CAPACITY ||
wc >= (core ? corePoolSize : maximumPoolSize))
return false;
//增加线程个数成功,退出循环
if (compareAndIncrementWorkerCount(c))
break retry;
c = ctl.get(); // Re-read ctl
if (runStateOf(c) != rs)
continue retry;
// else CAS failed due to workerCount change; retry inner loop
}
}
boolean workerStarted = false;
boolean workerAdded = false;
Worker w = null;
try {
//创建新建线程
w = new Worker(firstTask);
final Thread t = w.thread;
if (t != null) {
final ReentrantLock mainLock = this.mainLock;
mainLock.lock();
try {
// Recheck while holding lock.
// Back out on ThreadFactory failure or if
// shut down before lock acquired.
int rs = runStateOf(ctl.get());
if (rs < SHUTDOWN ||
(rs == SHUTDOWN && firstTask == null)) {
if (t.isAlive()) // precheck that t is startable
throw new IllegalThreadStateException();
workers.add(w);
int s = workers.size();
if (s > largestPoolSize)
largestPoolSize = s;
workerAdded = true;
}
} finally {
mainLock.unlock();
}
if (workerAdded) {
//启动线程,会执行worker 对象的run方法
t.start();
workerStarted = true;
}
}
} finally {
if (! workerStarted)
addWorkerFailed(w);
}
return workerStarted;
}
可以看出线程启动后会执行Woker类中run方法
Worker(Runnable firstTask) {
setState(-1); // inhibit interrupts until runWorker
this.firstTask = firstTask;
//线程传的Runnable接口是this对象
this.thread = getThreadFactory().newThread(this);
}
/** Delegates main run loop to outer runWorker */
public void run() {
runWorker(this);
}
runWorker 线程启动,执行的方法,从代码上可有看出,1)如果线程数量大于核心线程数量且keepAliveTime时间内任务队列没有任务的时候线程会被回收;2)如果allowCoreThreadTimeOut设置为true的时候,keepAliveTime时间内任务队列没有任务时候即使线程数量小于核心线程数量也会被回收。3)如果线程数量小于等于核心线程数量将会阻塞等待任务上,也就不会出现死循环的情况(allowCoreThreadTimeOut=false)。
final void runWorker(Worker w) {
Thread wt = Thread.currentThread();
Runnable task = w.firstTask;
w.firstTask = null;
w.unlock(); // allow interrupts
boolean completedAbruptly = true;
try {
//task为null 且getTask()==null时候退出循环
while (task != null || (task = getTask()) != null) {
w.lock();
// If pool is stopping, ensure thread is interrupted;
// if not, ensure thread is not interrupted. This
// requires a recheck in second case to deal with
// shutdownNow race while clearing interrupt
if ((runStateAtLeast(ctl.get(), STOP) ||
(Thread.interrupted() &&
runStateAtLeast(ctl.get(), STOP))) &&
!wt.isInterrupted())
wt.interrupt();
try {
beforeExecute(wt, task);
Throwable thrown = null;
try {
task.run();
} catch (RuntimeException x) {
thrown = x; throw x;
} catch (Error x) {
thrown = x; throw x;
} catch (Throwable x) {
thrown = x; throw new Error(x);
} finally {
afterExecute(task, thrown);
}
} finally {
task = null;
w.completedTasks++;
w.unlock();
}
}
completedAbruptly = false;
} finally {
//线程退出,从woker中移除
processWorkerExit(w, completedAbruptly);
}
}
private Runnable getTask() {
boolean timedOut = false; // Did the last poll() time out?
for (;;) {
int c = ctl.get();
int rs = runStateOf(c);
// Check if queue empty only if necessary.
if (rs >= SHUTDOWN && (rs >= STOP || workQueue.isEmpty())) {
decrementWorkerCount();
return null;
}
int wc = workerCountOf(c);
// allowCoreThreadTimeOut 默认false,也就是说当线程数量大于corePoolSize 时候 timed=true
boolean timed = allowCoreThreadTimeOut || wc > corePoolSize;
if ((wc > maximumPoolSize || (timed && timedOut))
&& (wc > 1 || workQueue.isEmpty())) {
if (compareAndDecrementWorkerCount(c))
return null;
continue;
}
try {
// 如果timed 为true 就会按照keepAliveTime时间进行等待,如果等待不到直接返回null,也就是上面的runWorker 会退出,线程就会被释放,当如果timed 为
false 会一直阻塞等待
Runnable r = timed ?
workQueue.poll(keepAliveTime, TimeUnit.NANOSECONDS) ;
workQueue.take();
if (r != null)
return r;
timedOut = true;
} catch (InterruptedException retry) {
timedOut = false;
}
}
}