1.string基本概念
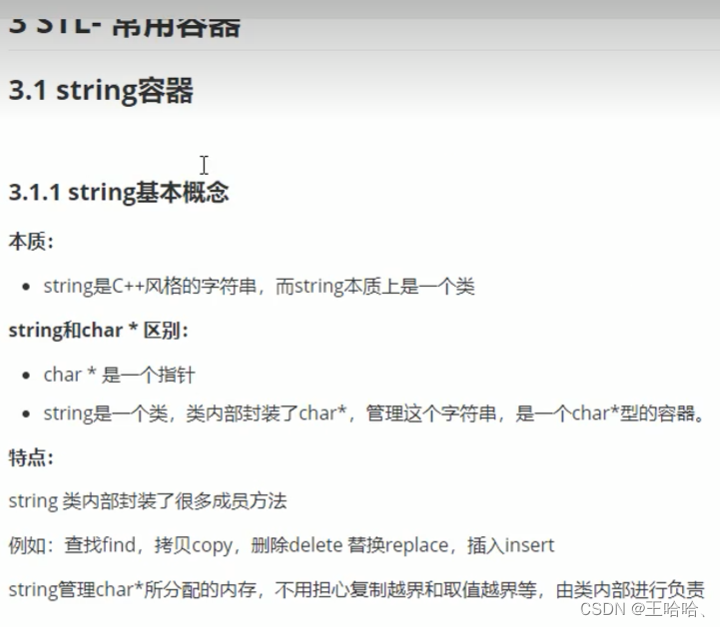
2.string构造函数
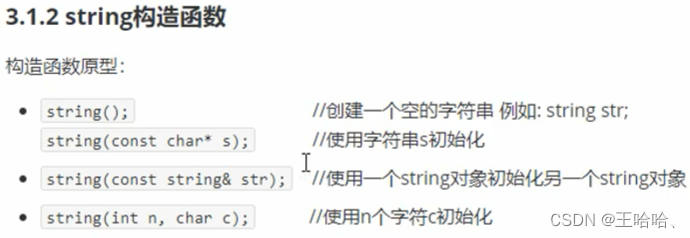

#include <iostream>
using namespace std;
//string容器
void test01()
{
string s1;//创建空字符串,调用无参构造函数
cout << "str1 = " << s1 << endl;//什么都不输出
const char* str = "hello world";
string s2(str); //c_string转换成了string
cout << "str2 = " << s2 << endl;
string s3(s2); //调用拷贝构造函数
cout << "str3 = " << s3 << endl;
string s4(10, 'a'); //使用n个字符c初始化
cout << "str4 = " << s4 << endl;
}
//*************************************
int main() {
test01();
//test02();
//**************************************
system("pause");
return 0;
}
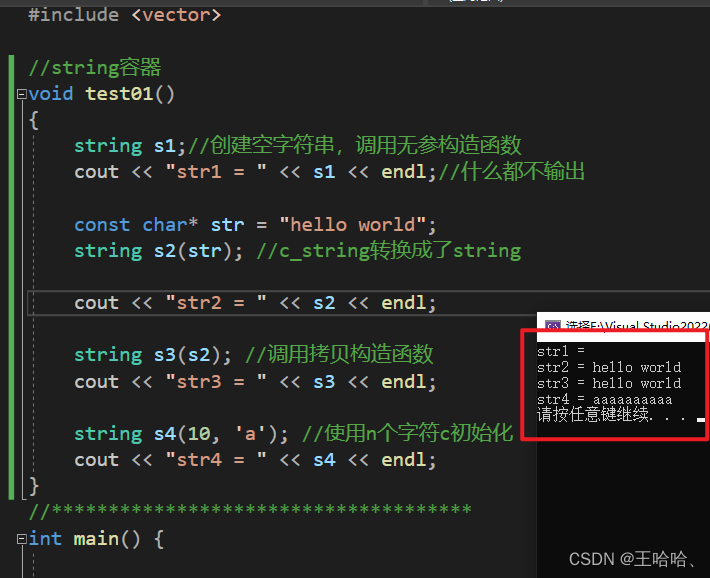
3.string赋值操作
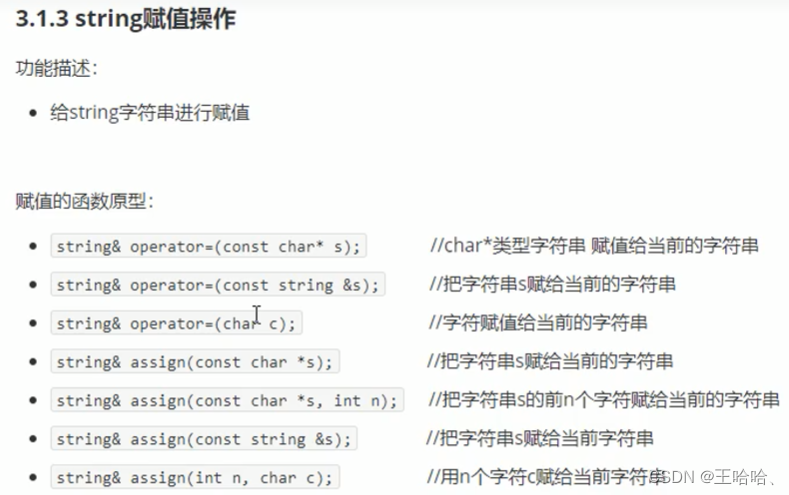
#include <iostream>
using namespace std;
void test01()
{
string str1;
str1 = "hello world";
cout << "str1 = " << str1 << endl;
string str2;
str2 = str1;
cout << "str2 = " << str2 << endl;
string str3;
str3 = 'a';
cout << "str3 = " << str3 << endl;
string str4;
str4.assign("hello c++");
cout << "str4 = " << str4 << endl;
string str5;
str5.assign("hello c++", 5);
cout << "str5 = " << str5 << endl;
string str6;
str6.assign(str5);
cout << "str6 = " << str6 << endl;
string str7;
str7.assign(5, 'x');
cout << "str7 = " << str7 << endl;
}
//*************************************
int main() {
test01();
//test02();
//**************************************
system("pause");
return 0;
}
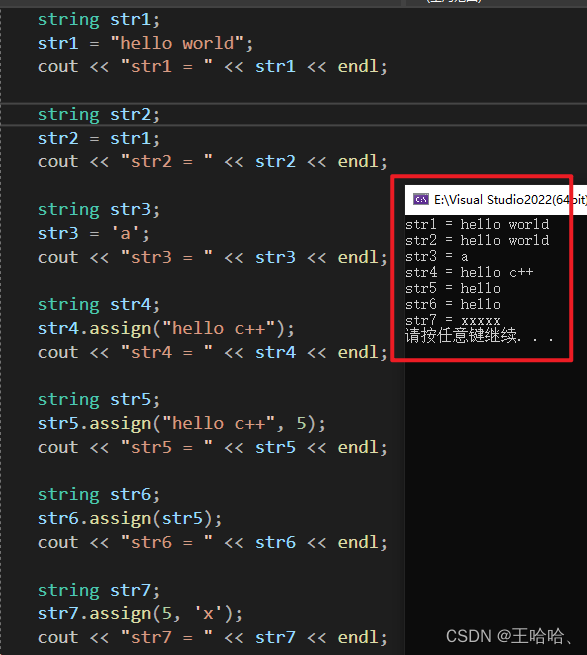
4.string字符串拼接
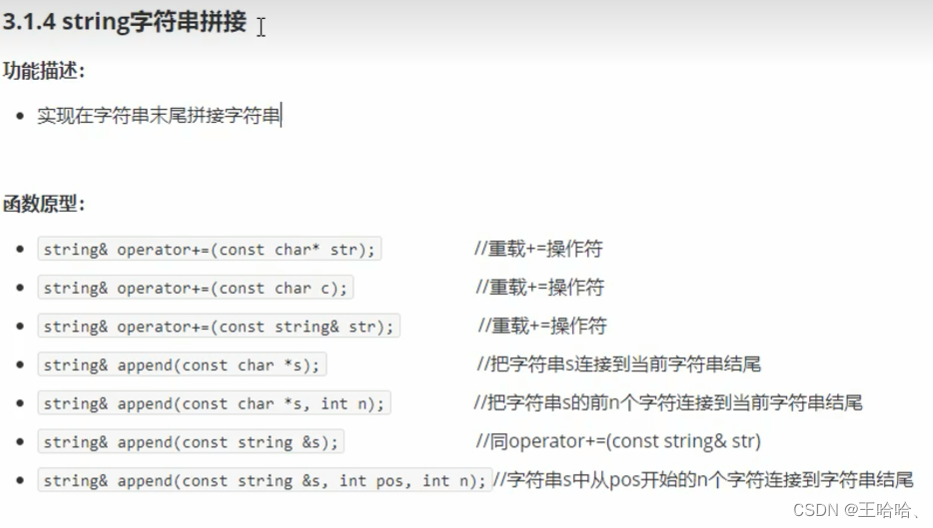
#include <iostream>
using namespace std;
//string字符串拼接
//函数原型:
// string& operator+=(const char* str); //重载+=操作符
// string& operator+=(const char c); //重载+=操作符
// string& operator+=(const string& str); //重载+=操作符
// string& append(const char *s); //把字符串S连接到当前字符串结尾
// string& append(const char *s,int n); //把字符串S的前n个字符串连接到当前字符串结尾
// string& append(const string &s); //同operator+=(const string& str)
// string& append(const string &s, int pos, int n);//字符串S中从pos开始的n个字符连接到字符串结尾
//********************************************************************************************
void test01()
{
// string& operator+=(const char* str); //重载+=操作符
string str1 = "我";
str1 += "爱陶子";
cout << "str1 = " << str1 << endl;
// string& operator+=(const char c); //重载+=操作符
str1 += '!';
cout << "str1 = " << str1 << endl;
// string& operator+=(const string& str); //重载+=操作符
string str2 = " Miss Tao!";
str1 += str2;
cout << "str1 = " << str1 << endl;
// string& append(const char *s); //把字符串S连接到当前字符串结尾
string str3 = "I";
str3.append(" love ");
cout << "str3 = " << str3 << endl;
// string& append(const char *s,int n); //把字符串S的前n个字符串连接到当前字符串结尾
str3.append("Tao! taozi", 4);
cout << "str3 = " << str3 << endl;
// string& append(const string &s); //同operator+=(const string& str)
str3.append(str2);
cout << "str3 = " << str3 << endl;
// string& append(const string &s, int pos, int n);//字符串S中从pos开始的n个字符连接到字符串结尾
str3.append(str2, 6, 4);
cout << "str3 = " << str3 << endl;
}
int main() {
test01();
//test02();
//**************************************
system("pause");
return 0;
}
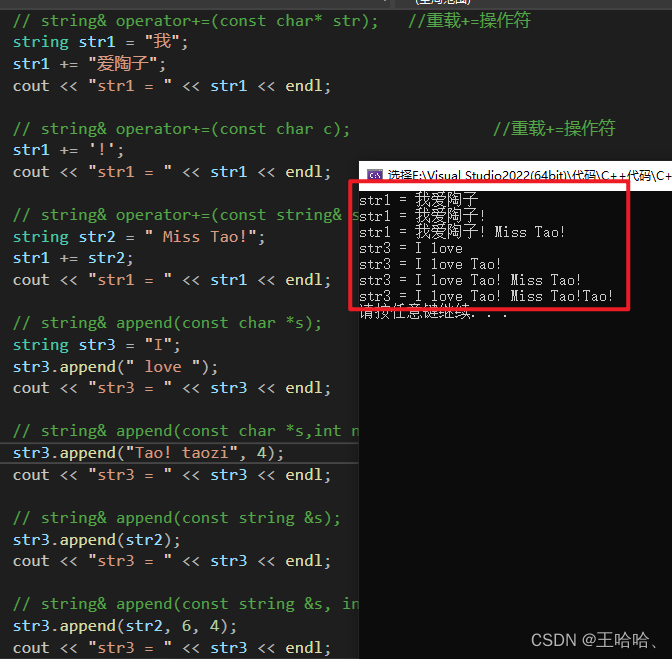

不知道再查就好啦
5.string查找和替换
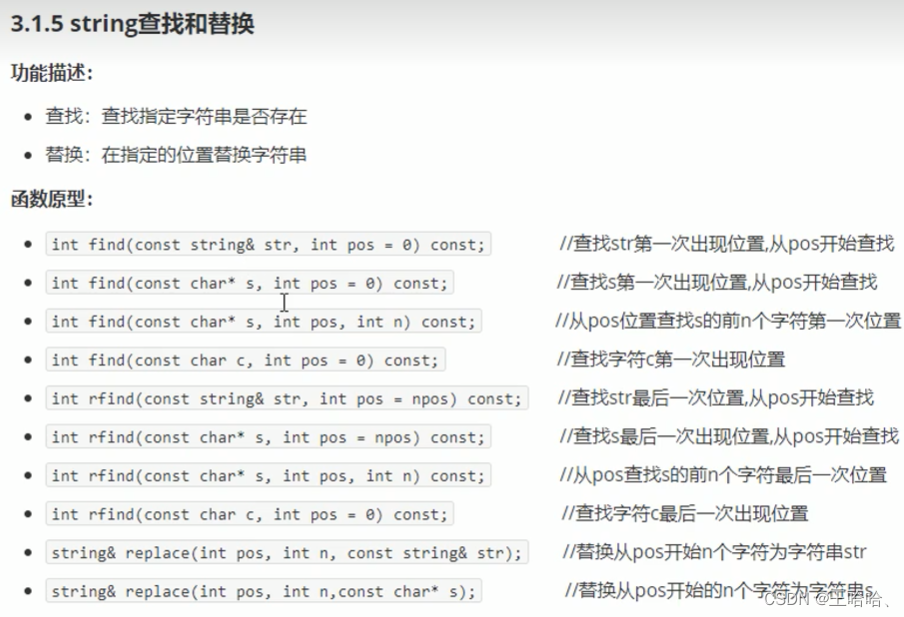
#include <iostream>
using namespace std;
//string字符串的查找和替换
//查找
void test01()
{
string str1 = "abcdefgde";
//find
int pos = str1.find("de");
if (pos == -1)
{
cout << "未找到" << endl;
}
else
{
cout << "pos = " << pos << endl;
}
//rfind
pos = str1.rfind("de");
cout << "pos = " << pos << endl;
//find和rfind的区别
//find从左往右查找 rfind从右往左查找
}
//替换
void test02()
{
string str1 = "abcdefgde";
str1.replace(1, 3, "1111"); //把第1到3位全部移除,将1111全部插入
cout << "str1 = " << str1 << endl;
}
int main() {
//test01();
test02();
//**************************************
system("pause");
return 0;
}
《查找》的结果
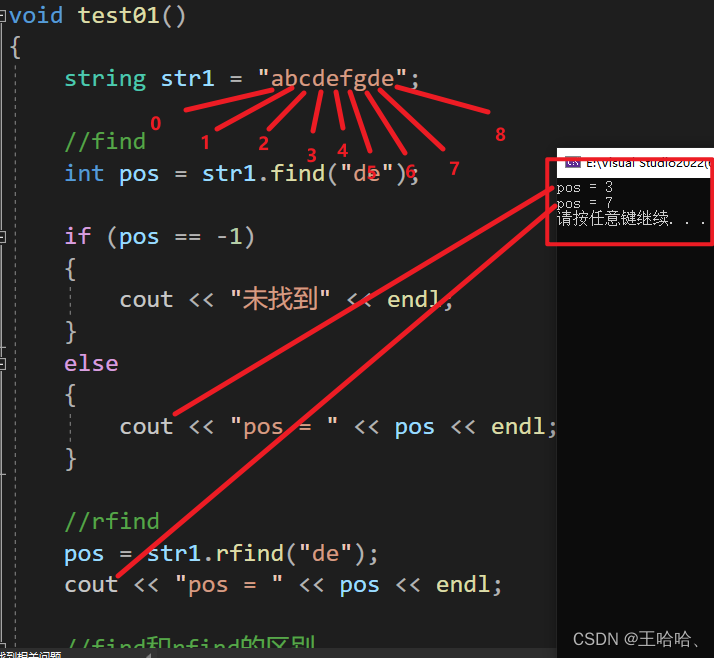
《替换》的结果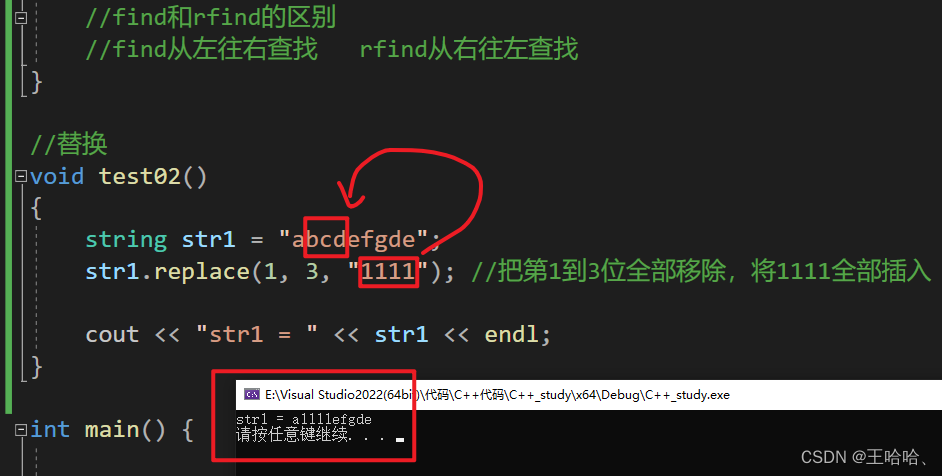
6.string字符串比较
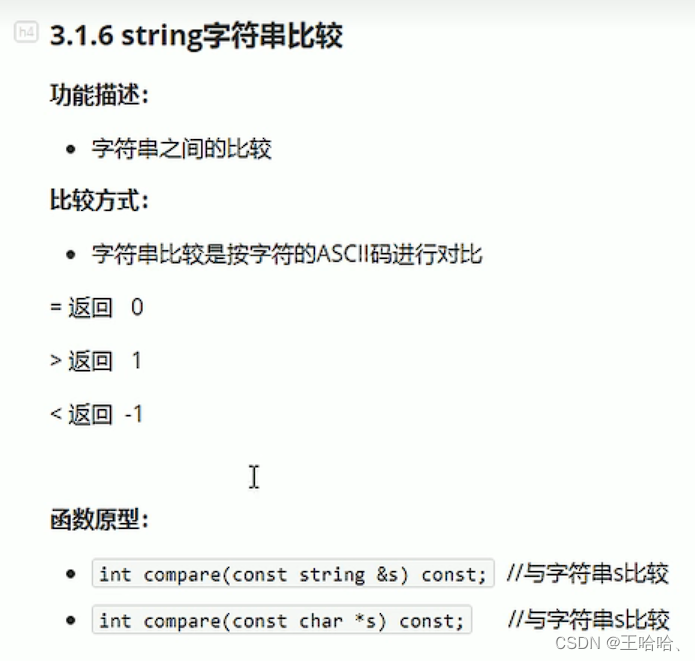
#include <iostream>
using namespace std;
//string字符串比较
void test01()
{
string str1 = "hello";
string str2 = "delao";
int ret = str1.compare(str2);
if (ret == 0)
{
cout << "str1等于str2" << endl;
}
else if (ret > 0)
{
cout << "str1大于str2" << endl;
}
else
{
cout << "str1小于str2" << endl;
}
}
int main() {
test01();
//test02();
//**************************************
system("pause");
return 0;
}
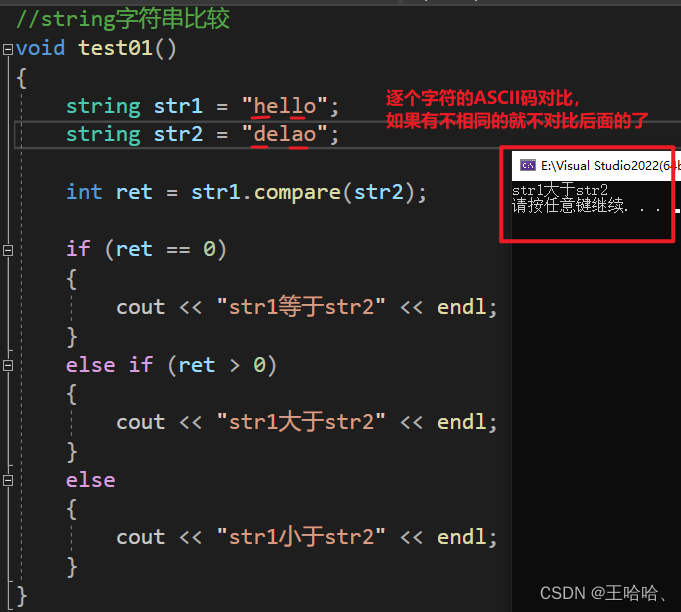

7.string字符存取
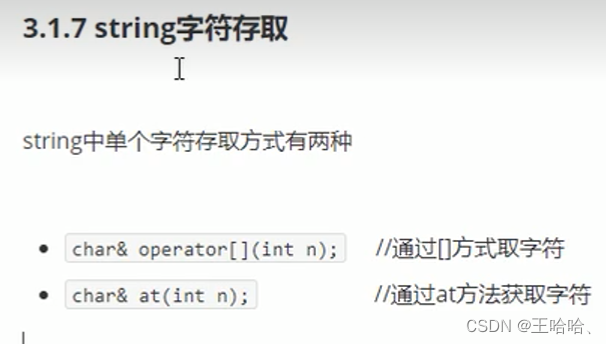
#include <iostream>
using namespace std;
//string字符存取
void test01()
{
string str = "hello";
cout << "str = " << str << endl;
//1、通过[]访问单个字符
for (int i = 0; i < str.size(); i++)
{
cout << str[i] << " ";
}
cout << endl;
//通过at方式访问单个字符
for (int i = 0; i < str.size(); i++)
{
cout << str.at(i) << " ";
}
cout << endl;
//修改单个字符
str[0] = 'x';
//xello
cout << "str = " << str << endl;
str.at(1) = 'x';
//xxlo
cout << "str = " << str << endl;
}
int main() {
test01();
//test02();
//**************************************
system("pause");
return 0;
}
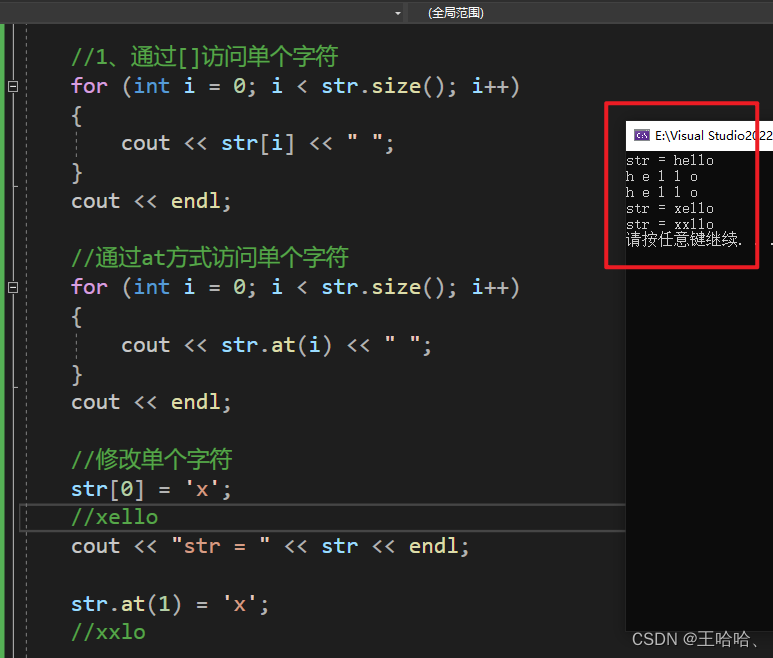

8.string插入和删除
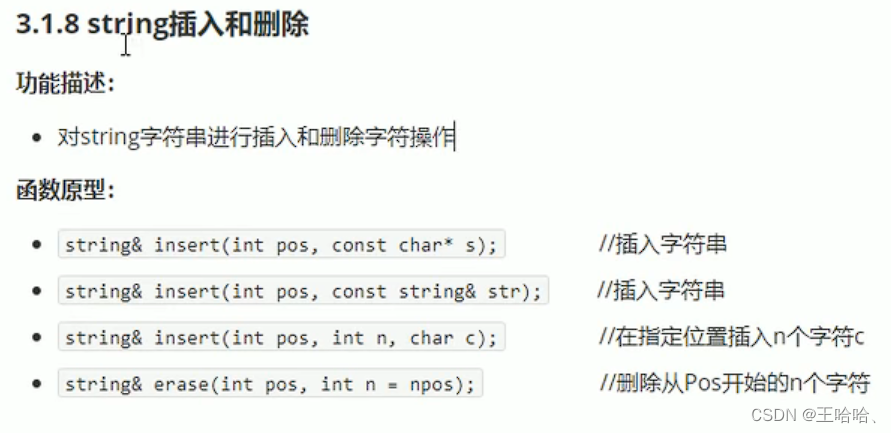
#include <iostream>
using namespace std;
//字符串插入和删除
void test01()
{
string str = "hello";
//插入
str.insert(1, "111");
//h111ello
cout << str << endl;
//删除
str.erase(1, 3); //从1号位置开始的3个字符
cout << str << endl;
}
int main() {
test01();
//test02();
//**************************************
system("pause");
return 0;
}
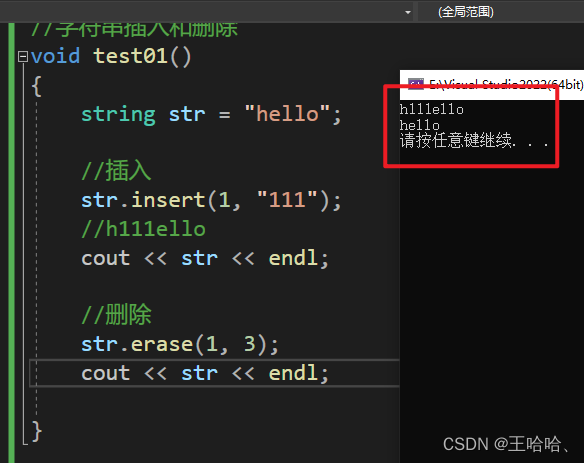
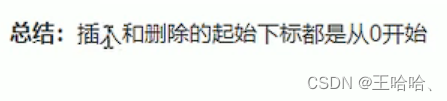
9.string子串
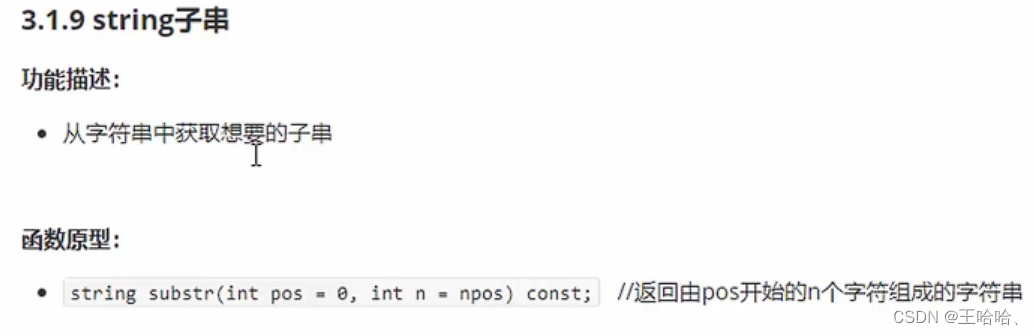

#include <iostream>
using namespace std;
//string子串截取
void test01()
{
string str = "123456";
string subStr = str.substr(1, 3);
cout << "subStr = " << subStr << endl;
//实用例子-从邮箱名提取用户信息
string email = "wanghaha@163.com";
int pos = email.find('@');
string usrname = email.substr(0, pos);
cout << "Name = " << usrname << endl;
}
int main() {
test01();
//test02();
//**************************************
system("pause");
return 0;
}
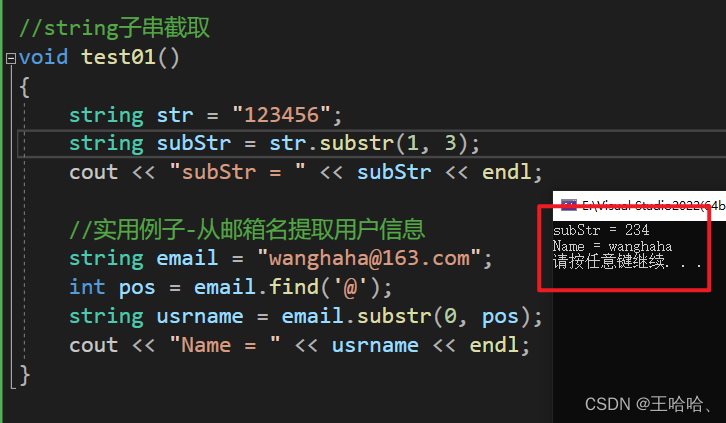