Java 根据word doc文档模板导出
maven依赖
<dependency>
<groupId>commons-beanutils</groupId>
<artifactId>commons-beanutils</artifactId>
<version>1.9.4</version>
</dependency>
<dependency>
<groupId>commons-digester</groupId>
<artifactId>commons-digester</artifactId>
<version>2.1</version>
</dependency>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-jexl</artifactId>
<version>2.1.1</version>
</dependency>
<dependency>
<groupId>net.sourceforge.jexcelapi</groupId>
<artifactId>jxl</artifactId>
<version>2.6.12</version>
</dependency>
<dependency>
<groupId>net.sf.jxls</groupId>
<artifactId>jxls-core</artifactId>
<version>1.0.6</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>3.15</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>3.15</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-scratchpad</artifactId>
<version>3.15</version>
</dependency>
导出模板
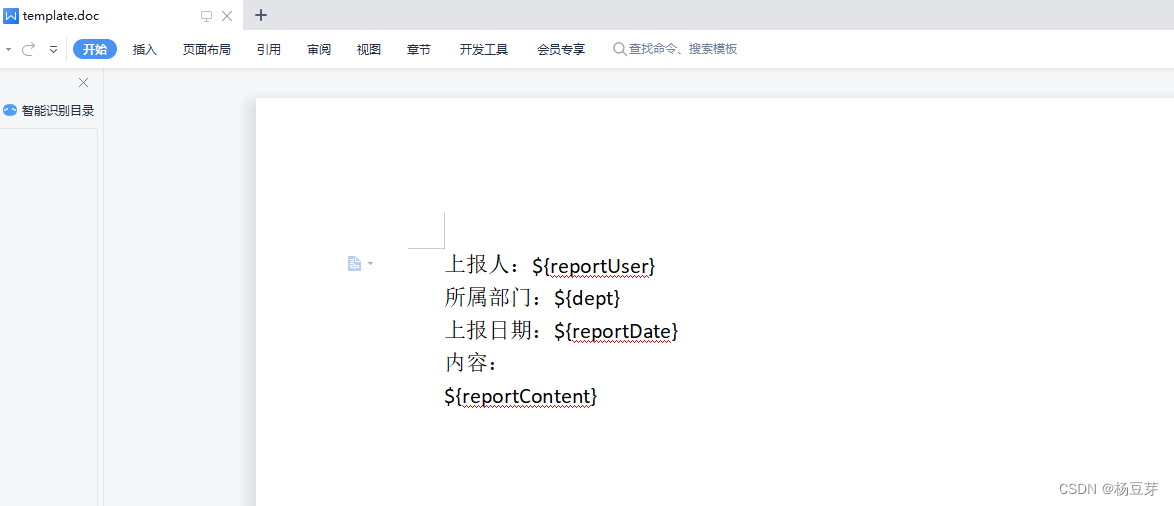
代码工具
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
import org.apache.poi.hwpf.HWPFDocument;
import org.apache.poi.hwpf.usermodel.Range;
/**
* 根据doc模板导出
*
* @author Yang xt
* @Mar 22, 2017
*/
public class POIExportDoc {
public static void main(String[] args) {
String templatePath = "E:\\tmp\\excel\\template.doc";
// ."D:\\export-office\\doc\\write.doc"
// exportDoc();
Map<String, String> map = new HashMap<>();
map.put("${reportUser}", "杨豆芽");
map.put("${dept}", "研发部");
map.put("${reportDate}", new SimpleDateFormat("yyyy年MM月dd日").format(new Date()));
map.put("${reportContent}", "曾梦想仗剑走天涯,看一看世界的繁华");
Map<String, Object> maps = exportDoc(map, templatePath, "E:\\tmp\\excel\\", "export-doc");
System.out.println(maps.toString());
}
/**
* 使用doc模板导出数据 注:导出类型为文本类型,其他字符类型必须自行控制
*
* @param paramMap 导入doc参数 key-value 如: map.put("${userName}", "杨秀腾"); doc模板上同是匹配参数 ${userName}
* @param templateFilePath 模板文件路径 -- 绝对路径
* @param savePath 导出文件存储路径
* @param saveDocFileName 导出文件文件名 后缀默认 .doc 可写可不写
* @return Map<String, Object> 返回程序状态 key(msgCode) - value(msgContent) 3 -- 导出doc成功
*/
public static Map<String, Object> exportDoc(Map<String, String> paramMap, String templateFilePath, String savePath, String saveDocFileName) {
Map<String, Object> resMap = new HashMap<String, Object>();
File file = null;
if (null != paramMap && paramMap.size() > 0) {
if (templateFilePath.endsWith(".doc")) {
file = new File(templateFilePath);
if (file.isFile()) {
InputStream is = null;
OutputStream os = null;
HWPFDocument doc = null;
try {
is = new FileInputStream(templateFilePath);
doc = new HWPFDocument(is);
Range range = doc.getRange();
// 把range范围内的${xx}替换为当前的日期
Set<String> mapKeys = paramMap.keySet();
for (String string : mapKeys) {
range.replaceText(string, String.valueOf((null == paramMap.get(string)) ? "" : paramMap.get(string)));
}
file = new File(savePath);
if (!file.exists()) {
file.mkdirs();
}
if (!saveDocFileName.endsWith(".doc")) {
saveDocFileName = saveDocFileName.concat(".doc");
}
if (!savePath.endsWith("/")) {
savePath = savePath.concat("/");
}
os = new FileOutputStream(savePath.concat(saveDocFileName));
// 把doc输出到输出流中
doc.write(os);
doc.close();
os.flush();
os.close();
is.close();
resMap.put("msgCode", 3);
resMap.put("msgContent", "export doc success");
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} finally {
if (null != doc) {
try {
doc.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (null != os) {
try {
os.flush();
os.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (null != is) {
try {
is.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
} else {
resMap.put("msgCode", 2);
resMap.put("msgContent", "template is not found");
}
} else {
resMap.put("msgCode", 1);
resMap.put("msgContent", "template type error,support Microsoft Word 97 - 2003 (*.doc)");
}
} else {
resMap.put("msgCode", 0);
resMap.put("msgContent", "parameters cannot be empty");
}
return resMap;
}
}
导出结果
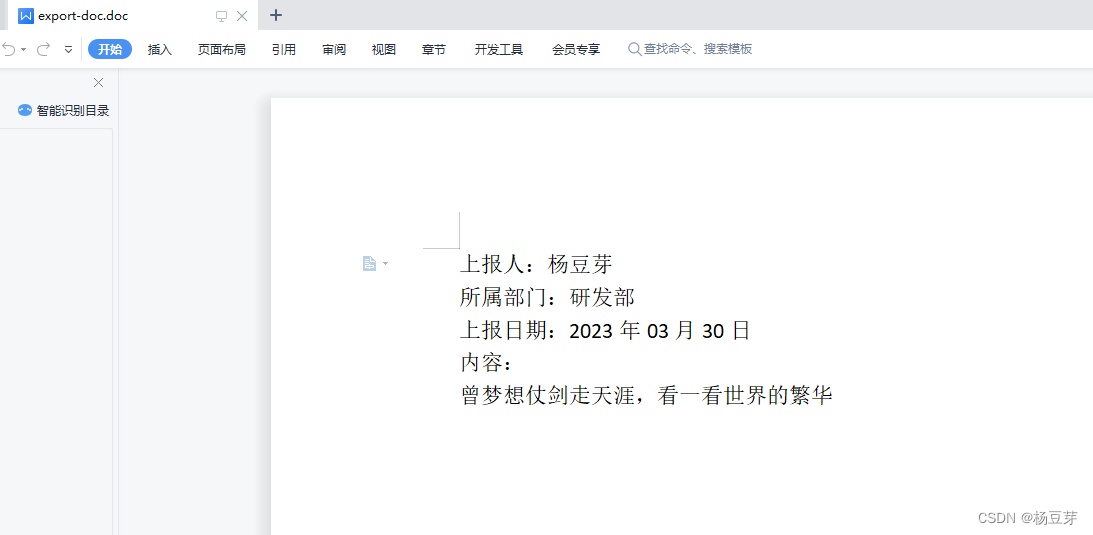