1、编写系统全局异常处理类要求:
1、定义全局异常处理类,使用@ControllerAdvice 注解标签修饰
2、处理指定异常类信息,通过@ExceptionHandler 注解标签修饰
3、处理指定异常类是否向前端返回错误信息,如果需要向调用端返回错误信息,通过@ResponseBody 注解标签修饰
4、编写指定处理异常类的方法,注意定义方法的属性参数必须包含错误异常类。
1. 1、编写系统全局异常示例
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.ResponseBody;
import com.zzg.common.entity.Result;
import com.zzg.sms.exception.SMSException;
/**
*
* @ClassName: GlobalException
* @Description: 全局异常处理
*/
@ControllerAdvice
public class GlobalException {
// 日志记录
public static final Logger logger = LoggerFactory.getLogger(GlobalException.class);
@ExceptionHandler(value = SMSException.class)
@ResponseBody
public Result exceptionHandler(SMSException e) {
logger.error("error: {}", e.getMessage(), e);
return Result.error(e.getMessage());
}
}
注意:上述示例中,我们对捕获的异常进行简单的二次处理,向前端返回异常的信息,并由前端提示用户相关错误信息的原因。
1.2 、编写自定义异常对象
package com.zzg.sms.exception;
public class SMSException extends RuntimeException {
/**
* @Fields serialVersionUID : TODO(用一句话描述这个变量表示什么)
*/
private static final long serialVersionUID = 1L;
private String message;
public String getMessage() {
return message;
}
public void setMessage(String message) {
this.message = message;
}
public SMSException(String message) {
super();
this.message = message;
}
}
1.3、编写自定义请求响应对象
package com.zzg.common.entity;
import java.io.Serializable;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
@SuppressWarnings("serial")
public class Result implements Serializable {
// 成功返回
public static String RESULT_CODE_SUCCESS = "0";
// 异常返回
public static String RESULT_CODE_ERROR = "500";
// 返回码
protected String code;
// 返回提示信息
protected String message;
// 返回消息类型
protected Integer type;
// 返回数据
protected Map<String, Object> datas = new HashMap<String, Object>();
public Result() {
this.code = RESULT_CODE_SUCCESS;
this.message = "success";
}
public static Result ok() {
return new Result();
}
public static Result ok(String msg) {
return ok(RESULT_CODE_SUCCESS, msg);
}
public static Result ok(String code, String msg) {
Result r = new Result();
r.code = code;
r.message = msg;
return r;
}
public static Result error(String msg) {
return error(RESULT_CODE_ERROR, msg);
}
public static Result error(String code, String msg) {
Result r = new Result();
r.code = code;
r.message = msg;
return r;
}
public static Result error(String code, String msg, Integer type) {
Result r = new Result();
r.code = code;
r.message = msg;
r.type = type;
return r;
}
/**
*
* @Title: setData @Description: 添加返回数据到默认 data 节点 @param: @param
* obj @param: @return @return: Result @throws
*/
public Result setDatas(Object obj) {
datas.put("data", obj);
return this;
}
public Result setDatas(Map rs) {
datas.putAll(rs);
return this;
}
public Result setDatas(List list) {
datas.put("list", list);
return this;
}
public Result setDatas(String name, Object value) {
datas.put(name, value);
return this;
}
public Result setSid(Long sid) {
setDatas("sid", sid);
return this;
}
public Result setSid(String sid) {
setDatas("sid", sid);
return this;
}
public String getCode() {
return code;
}
public void setCode(String code) {
this.code = code;
}
public String getMessage() {
return message;
}
public void setMessage(String message) {
this.message = message;
}
public Object getDatas() {
return datas;
}
public Integer getType() {
return type;
}
public void setType(Integer type) {
this.type = type;
}
}
1.4、编写功能代码抛出自定义异常信息
1.4.1、 Controller 功能调用
// 短信实时发送
// 获取request 对象
ServletRequestAttributes servletRequestAttributes = (ServletRequestAttributes) RequestContextHolder
.getRequestAttributes();
HttpServletRequest request = servletRequestAttributes.getRequest();
chain.exect(phone, IpUtils.getIpAddr(request));
注意:chain 是自定义拦截链组件,主要用户判断:指定手机号码发送短信是否符合拦截要求,否则抛出自定义异常类SMSException 异常类型
具体请参考:SpringBoot + Redis+ 拦截链实现用户短信发送规则匹配。
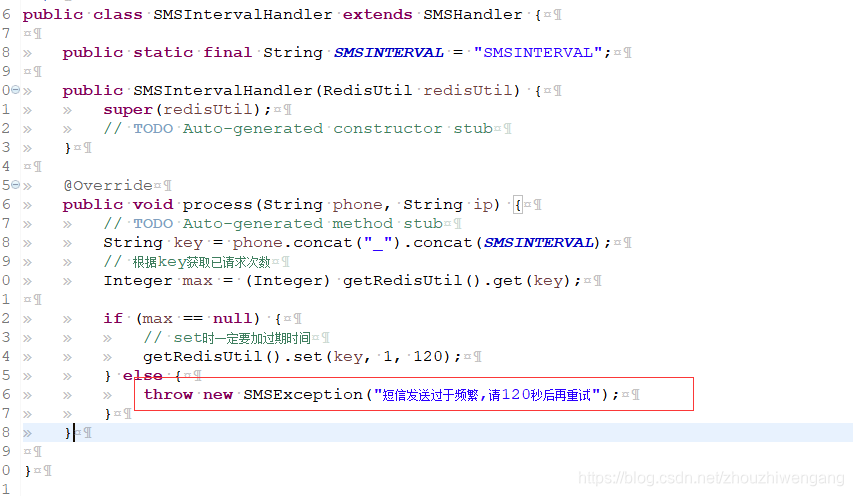