C# —— 面向对象编程练习
基础题

代码如下:
class Program
{
static void Main(string[] args)
{
rectangle r = new rectangle();
Console.WriteLine("调用不含参构造函数初始化后矩形的长为{0},宽为{1}", r.GetLength().ToString(), r.GetWidth().ToString());
Console.WriteLine("请输入矩形长、宽:");
rectangle R = new rectangle(float.Parse(Console.ReadLine()), float.Parse(Console.ReadLine()));
Console.WriteLine("调用不含参构造函数初始化后矩形的长为{0},宽为{1}", R.GetLength().ToString(), R.GetWidth().ToString());
Console.WriteLine("此矩形的周长为{0},面积为{1}", R.GetPerimeter().ToString(), R.GetArea().ToString());
Console.WriteLine("修改矩形的长为:");
R.ChangeLength(float.Parse(Console.ReadLine()));
Console.WriteLine("修改矩形的宽为:");
R.ChangeWidth(float.Parse(Console.ReadLine()));
Console.WriteLine("修改后的矩形的周长为{0},面积为{1}", R.GetPerimeter().ToString(), R.GetArea().ToString());
}
}
class rectangle
{
//私有访问。只限于本类成员访问,子类,实例都不能访问
private float len;
private float wid;
//无参构造函数
public rectangle()
{
this.len = 0;
this.wid = 0;
}
//含参构造函数
public rectangle(float length,float width)
{
this.len = length;
this.wid = width;
}
//求周长
public float GetPerimeter()
{
return (this.len + this.wid) * 2;
}
//求面积
public float GetArea()
{
return this.len * this.wid;
}
//取矩形长度
public float GetLength()
{
return this.len;
}
//取矩形宽度
public float GetWidth()
{
return this.wid;
}
//修改矩形长度
public void ChangeLength(float length)
{
this.len = length;
}
// 修改矩形宽度
public void ChangeWidth(float width)
{
this.wid = width;
}
}
运行结果:
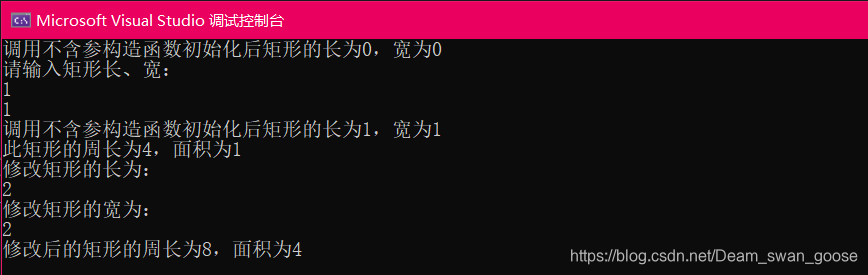
解析:
- this代表当前类的实例对象,所以this.len 和 this.wid 都是全局变量。

考察点:继承,构造函数的多种方法
代码如下:
class Program
{
static void Main(string[] args)
{
Student s1 = new Student();
s1.Display();
Student s2 = new Student("张大磊", "男", 40, new int[] { 10, 20, 30, 40, 50 });
s2.Display();
Student s3 = new Student(new int[] { 60, 70, 80, 90, 100 });
s3.Display();
}
}
class Person
{
//保护访问。只限于本类和子类访问,实例不能访问
protected string Name;
protected string Sex;
protected int Age;
/*此处必须写一个无参构造函数。
因为如果你的父类定义了带参数的构造函数同时没有无参重载的情况下,
那么在子类中,你必须对父类的带参数的构造进行赋值
*/
public Person() { }
public Person(string name , string sex ,int age)
{
this.Name = name;
this.Sex = sex;
this.Age = age;
}
}
class Student : Person
{
private int[] Sorce;
//构造函数1
public Student()
{
this.Name = "张磊";
this.Sex = "男";
this.Age = 30;
this.Sorce = new int[] { 60, 60, 60, 60, 60 };
}
//构造函数2
public Student(string name,string sex,int age,int[] sorce)
:base(name, sex, age)//调用父类的含参构造函数
{
this.Sorce = sorce;
}
//构造函数3
public Student(int[] sorce)
{
this.Name = "张小磊";
this.Sex = "男";
this.Age = 30;
this.Sorce = sorce;
}
//求平均成绩
public float Avg()
{
float sum = 0;
foreach (int i in Sorce)
{
sum += i;
}
return sum / Sorce.Length;
}
public void Display()
{
Console.WriteLine($"姓名:{this.Name}");
Console.WriteLine($"性别:{this.Sex}");
Console.WriteLine($"年龄:{this.Age}");
Console.Write("成绩:");
foreach (int i in this.Sorce)
{
Console.Write(i + " ");
}
Console.WriteLine($"平均成绩为:{Avg()}\n");
}
}
运行结果:
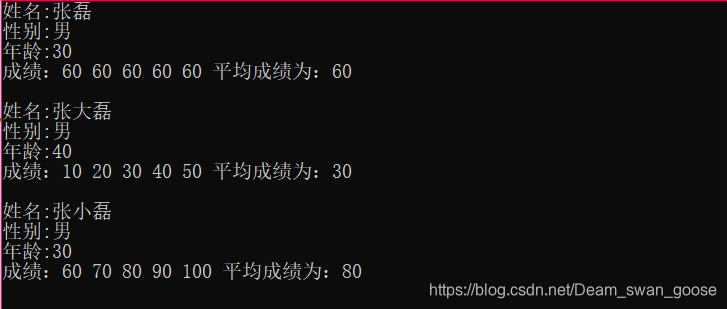
3.
(1)定义一个接口CanCry,描述会吼叫的方法public void cry()。
(2)分别定义狗类(Dog)和猫类(Cat),实现CanCry接口。实现方法的功能分别为:打印输出“我是狗,我的叫声是汪汪汪”、“我是猫,我的叫声是喵喵喵”。
(3)定义一个主类G,①定义一个void makeCry(CanCry c)方法,其中让会吼叫的事物吼叫。 ②在main方法中创建狗类对象(dog)、猫类对象(cat)、G类对象(g),用g调用makecry方法,让狗和猫吼叫。
考察点:接口,多继承。
代码如下:
class Program
{
static void Main(string[] args)
{
Cancry dog = new Dog();
Cancry cat = new Cat();
G g = new G();
g.makeCry(dog);
g.makeCry(cat);
}
}
//接口用来实现多继承是让一个类具有两个以上基类的唯一方式。
interface Cancry
{
public void cry();
}
class Dog : Cancry
{
public void cry()
{
Console.WriteLine("我是狗,我的叫声是汪汪汪");
}
}
class Cat : Cancry
{
public void cry()
{
Console.WriteLine("我是猫,我的叫声是喵喵喵");
}
}
class G
{
public void makeCry(Cancry c)
{
c.cry();
}
}
运行结果:

4.定义一个人类,包括属性:姓名、性别、年龄、国籍;包括方法:吃饭、睡觉,工作。 (1)根据人类,派生一个学生类,增加属性:学校、学号;重写工作方法(学生的工作是学习)。 (2)根据人类,派生一个工人类,增加属性:单位、工龄;重写工作方法(工人的工作是做工)。 (3)根据学生类,派生一个学生干部类,增加属性:职务;增加方法:开会。 (4)编写主函数分别对上述3类具体人物进行测试。
考点:继承,方法的重写,属性。
代码如下:
class Program
{
static void Main(string[] args)
{
Student student = new Student("张磊", "男", 18, "中国", "河南大学", "s123");
student.Work();
Worker worker = new Worker("张小磊", "男", 28, "中国", "河南大学", 4);
worker.Work();
Student_cadres student_cadres = new Student_cadres("张大磊", "男", 20, "河南大学","s456", "班长");
student_cadres.Meeting();
}
}
class Person
{
public string Name { get; set; } = "";
public string Sex { get; protected set; } = "";
public int Age { get; protected set; } = 0;
public string Country { get; protected set; } = "";
public virtual void Eat() { }
public virtual void Ship() { }
public virtual void Work() { }
}
class Student : Person
{
public Student() { }
public Student(string name,string sex,int age ,string country,string school,string stu_num)
{
Name = name;
Sex = sex;
Age = age;
Country = country;
School = school;
Stu_Num = stu_num;
}
public string School { get; protected set; } = "";
public string Stu_Num { get; protected set; } = "";
public override void Work()
{
Console.WriteLine($"{Name}是{School}{Age}岁的{Country}{Sex}学生,{Name}的学号是{Stu_Num}。{Name}有好好学习哦\n");
}
}
class Worker: Person
{
public Worker(string name, string sex, int age, string country, string company, int working_years)
{
Name = name;
Sex = sex;
Age = age;
Country = country;
Company = company;
Working_years = working_years;
}
public string Company { get; protected set; } = "";
public int Working_years { get; protected set; } = 0;
public override void Work()
{
Console.WriteLine($"{Name}是{Company}{Age}岁的{Country}{Sex}工人,{Name}的工龄是{Working_years}。{Name}工作超努力的哦\n");
}
}
class Student_cadres : Student
{
public Student_cadres(string name, string sex, int age, string country, string stu_num, string post)
{
Name = name;
Sex = sex;
Age = age;
Country = country;
Stu_Num = stu_num;
Post = post;
}
public string Post { get; protected set; } = "";
public void Meeting()
{
Console.WriteLine($"{Name}是{School}{Age}岁的{Country}{Sex}学生,{Name}的学号是{Stu_Num},职务是{Post}。{Name}有认真开会哦\n");
}
}
运行结果:
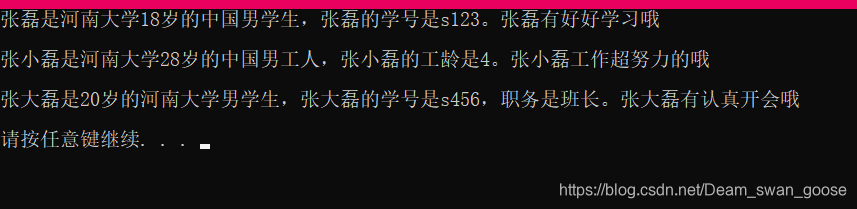
5.Employee:这是所有员工总的父类,属性:员工的姓名,员工的生日月份。方法:double getSalary(int month) 根据参数月份来确定工资,如果该月员工过生日,则公司会额外奖励100元。
SalariedEmployee:Employee的子类,拿固定工资的员工。属性:月薪HourlyEmployee:Employee的子类,按小时拿工资的员工,每月工作超出160小时的部分按照1.5倍工资发放。属性:每小时的工资、每月工作的小时数
SalesEmployee:Employee的子类,销售人员,工资由月销售额和提成率决定。属性:月销售额、提成率
BasePlusSalesEmployee:SalesEmployee的子类,有固定底薪的销售人员,工资由底薪加上销售提成部分。属性:底薪。
写一个程序,把若干各种类型的员工放在一个Employee数组里,
写一个方法,打印出某月每个员工的工资数额。
注意:要求把每个类都做成完全封装,不允许非私有化属性。
考察点:抽象,私有化属性。
代码如下:
class Program
{
public void PrintSalary(Employee[] woker)
{
Console.Write("请输入现在的月份:");
int month = int.Parse(Console.ReadLine());
for (int i = 0; i < woker.Length; i++)
{
Console.WriteLine($"我是{woker[i].GetName()},我在{month}月的工资是{woker[i].getSalary(month)}\n");
}
}
static void Main(string[] args)
{
SalariedEmployee employee1 = new SalariedEmployee("张磊",1,5000);
HourlyEmployee employee2 = new HourlyEmployee("张小磊", 2, 20, 200);
SalesEmployee employee3 = new SalesEmployee("张大磊", 3, 10000, 0.7);
BasePlusSalesEmployee emloyee4 = new BasePlusSalesEmployee("张磊磊", 4, 5000, 0.7, 3000);
Employee[] woker = { employee1, employee2, employee3, emloyee4 };
Program p = new Program();
p.PrintSalary(woker);
}
}
abstract class Employee
{
private string Name { get; set; } = "";
private int BirthMonth { get; set; } = 0;
public void SetName(string name)
{
Name = name;
}
public void SetBirthMonth(int birthmonth)
{
BirthMonth = birthmonth;
}
public string GetName()
{
return Name;
}
public int GetBirthMonth()
{
return BirthMonth;
}
public abstract double getSalary(int month) ;
}
class SalariedEmployee : Employee
{
private int Monthly_Salary { get; set; } = 0;
public SalariedEmployee (string name,int birthmonth,int monthly_salary)
{
SetName(name);
SetBirthMonth(birthmonth);
Monthly_Salary = monthly_salary;
}
public override double getSalary(int month)
{
if (GetBirthMonth() == month)
{
return Monthly_Salary + 100;
}
else
return Monthly_Salary;
}
}
class HourlyEmployee : Employee
{
private int Hourly_Salary { get; set; } = 0;
private int Hour { get; set; } = 0;
public HourlyEmployee(string name, int birthmonth, int hourly_salary,int hour)
{
SetName(name);
SetBirthMonth(birthmonth);
Hourly_Salary = hourly_salary;
Hour = hour;
}
public override double getSalary(int month)
{
double money = 0;
if (Hour > 160)
{
money = 160 * Hourly_Salary + (Hour - 160) * Hourly_Salary * 1.5;
}
else
money = Hourly_Salary * Hour;
if (GetBirthMonth() == month)
return money + 100;
else
return money;
}
}
class SalesEmployee : Employee
{
private int Monthly_Sales { get; set; } = 0;
private double Royalty_Rate { get; set; } = 0;
public int GetMonthly_Sales() { return Monthly_Sales; }
public double GetRoyalty_Rate() { return Royalty_Rate; }
public SalesEmployee() { }
public SalesEmployee(string name, int birthmonth,int monthly_slaes,double royalty_rate)
{
SetName(name);
SetBirthMonth(birthmonth);
Monthly_Sales = monthly_slaes;
Royalty_Rate = royalty_rate;
}
public override double getSalary(int month)
{
double money = Monthly_Sales * Royalty_Rate;
if (GetBirthMonth() == month)
return money + 100;
else
return money;
}
}
class BasePlusSalesEmployee : SalesEmployee
{
private int Base_Salary { get; set; } = 0;
public BasePlusSalesEmployee(string name, int birthmonth, int monthly_slaes, double royalty_rate, int base_salary)
:base(name,birthmonth,monthly_slaes,royalty_rate)
{
Base_Salary = base_salary;
}
public override double getSalary(int month)
{
double money = GetMonthly_Sales() * GetRoyalty_Rate() + Base_Salary;
if (GetBirthMonth() == month)
return money + 100;
else
return money;
}
}
运行结果:
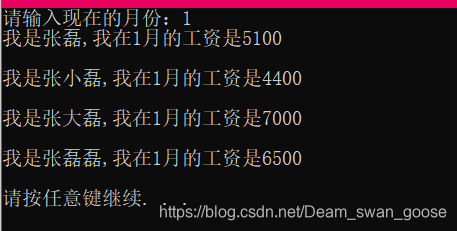
解析:
本题特殊点在于要求属性全是私有的,那么子类想要修改和获取父类的属性时就需要通过父类的Get()和Set()函数来进行操作。
6.图书买卖类库,该系统中必须包括三个类,类名及属性设置如下。
图书类(Book)
- 图书编号(bookId)
- 图书名称(bookName)
- 图书单价(price)
- 库存数量(storage)
订单项类(OrderItem)
- 图书名称(bookName)
- 图书单价(price)
- 购买数量(num)
订单类(Order):
- 订单号(orderId)
- 订单总额(total)
- 订单日期(date)
- 订单项列表(items)
具体要求及推荐实现步骤
1、创建图书类,根据业务需要提供需要的构造方法和setter/getter方法。
2、创建订单项类,根据业务需要提供需要的构造方法和setter/getter方法。
3、创建订单类,根据业务需要提供需要的构造方法和setter/getter方法。
4、创建测试类Test,实现顾客购买图书。
A、获取所有图书信息并输出:创建至少三个图书对象并输出即可。
B、顾客购买图书:顾客通过输入图书编号来购买图书,并输入购买数量。
C、输出订单信息:包括订单号、订单明细、订单总额、订单日期。
考察点:类之间的相互协同
代码如下:
class Program
{
static void Main(string[] args)
{
Book book1 = new Book(1, "Java教程", 30.6, 30);
Book book2 = new Book(2, "JSP 指南", 42.1, 40);
Book book3 = new Book(3, "SSH 架构", 47.3, 15);
Book[] book = { book1, book2, book3 };
Test test = new Test();
test.BookItem(book);
Order order = new Order();
test.PrintBookItems(test.Buy(book), order);
}
}
class Test
{
public void BookItem(Book[] book)
{
Console.WriteLine("\t图书列表");
Console.WriteLine(string.Format(
"{0,-10}{1,-10}{2,-10}{3,5}",
"图书编号", "图书名称", "图书单价", "库存数量"));
Console.WriteLine("--------------------------------------------------");
for (int i = 0; i < book.Length; i++)
{
Console.WriteLine($"{book[i].GetBookId()}\t{book[i].GetBookName()}\t{book[i].GetPrice()}\t{book[i].GetStock()}");
}
Console.WriteLine("--------------------------------------------------");
}
public List<OrderItem> Buy(Book[] book)
{
List<OrderItem> OrderItemList = new List<OrderItem>();
int i = 1;
while (i <= 3)
{
i++;
Console.Write("请输入图书编号选择图书:");
int bookid = int.Parse(Console.ReadLine())-1;
Console.Write("请输入购买图书的数量:");
int num = int.Parse(Console.ReadLine());
Console.WriteLine("请继续购买图书。");
OrderItemList.Add(new OrderItem(num, book[bookid].GetBookName(), book[bookid].GetPrice()));
}
return OrderItemList;
}
public void PrintBookItems(List<OrderItem> OrderItemList, Order order)
{
Console.WriteLine("\n\t图书订单");
Console.WriteLine($"图书订单号:{order.GetOrderId()}");
Console.WriteLine(string.Format("{0,-10}{1,-10}{2,-10}", "图书名称", "购买数量", "图书单价"));
Console.WriteLine("---------------------------------");
order.PrintItems(OrderItemList);
Console.WriteLine("---------------------------------");
Console.WriteLine($"订单总额:\t\t\t{order.GetTotal(OrderItemList)}");
Console.WriteLine($"日期:{order.GetData()}");
}
}
class Book
{
private int BookId { get; set; } = 0;
private string BookName { get; set; } = "";
private double Price { get; set; } = 0;
private int Stock { get; set; } = 0;
public Book(int bookid, string bookname, double price, int stock)
{
BookId = bookid;
BookName = bookname;
Price = price;
Stock = stock;
}
public void SetStock(int stock)
{
Stock = stock;
}
public void SetPrice(double price)
{
Price = price;
}
public int GetBookId()
{
return BookId;
}
public string GetBookName()
{
return BookName;
}
public double GetPrice()
{
return Price;
}
public int GetStock()
{
return Stock;
}
}
class OrderItem
{
private int Num { get; set; } = 0;
private string BookName { get; set; } = "";
private double Price { get; set; } = 0;
public OrderItem(int num, string bookname, double price)
{
Num = num;
BookName = bookname;
Price = price;
}
public void SetNum(int num)
{
Num = num;
}
public int GetNum()
{
return Num;
}
public string GetBookName()
{
return BookName;
}
public double GetPrice()
{
return Price;
}
}
class Order
{
private string OrderId = "00001";
private double Total { get; set; } = 0;
private string Data = DateTime.Now.ToString();
private string Items { get; set; } = "";
public double GetTotal(List<OrderItem> OrderItemList)
{
foreach (var orderitem in OrderItemList)
{
Total += orderitem.GetNum() * orderitem.GetPrice();
}
return Total;
}
public string GetData()
{
return Data;
}
public void PrintItems(List<OrderItem> OrderItemList)
{
foreach (var orderitem in OrderItemList)
{
Console.WriteLine($"{orderitem.GetBookName()}\t{orderitem.GetNum()}\t{orderitem.GetPrice()}");
}
}
public string GetOrderId() { return OrderId; }
}
运行结果:
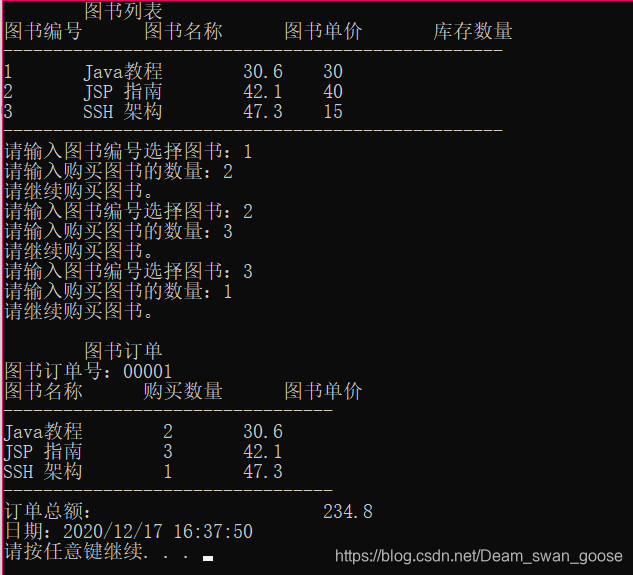
解析:
感觉这道题没用多少类的知识,但是写完感觉对函数用法更加深刻了。建议闲了动手写写,还挺好玩的。
7.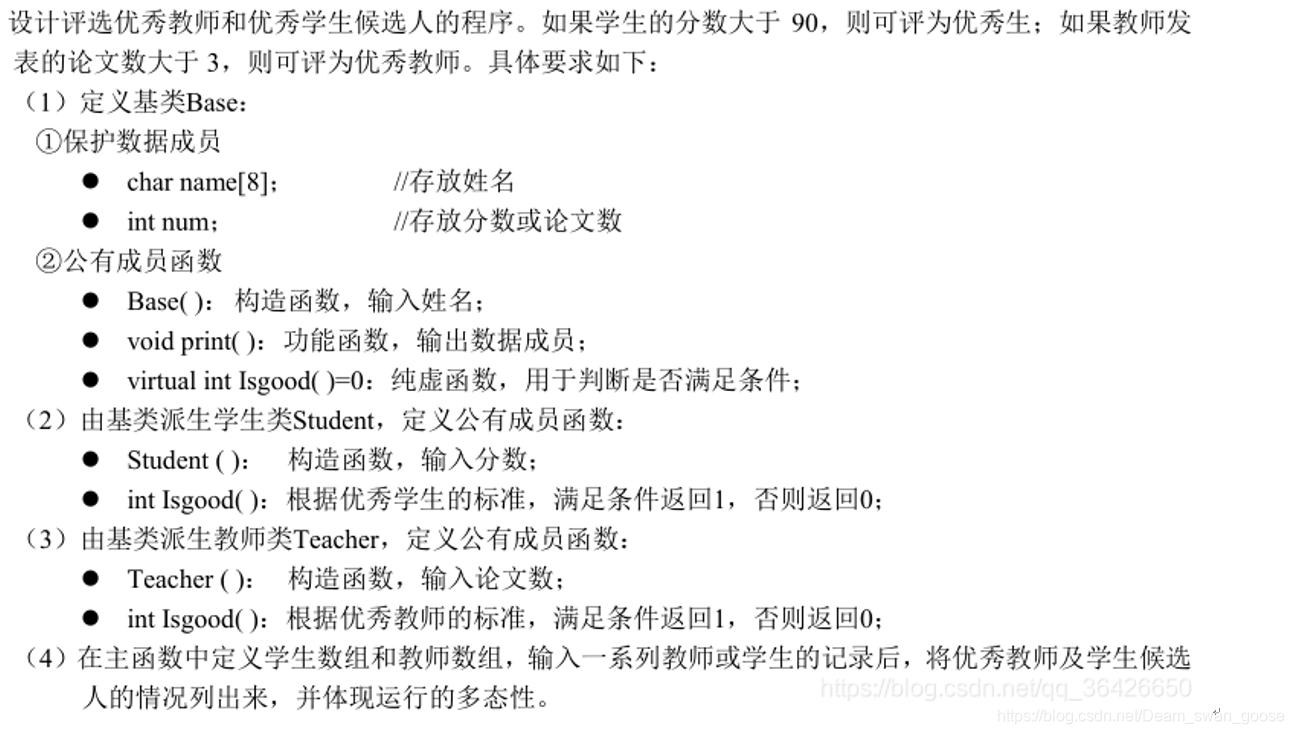
考察点:虚方法的实现,二维数组。
代码如下:
class Program
{
static void Main(string[] args)
{
Console.Write("请输入本次评优的学生数量:");
string[,] s = new string[int.Parse(Console.ReadLine()),2];
for (int i = 0; i < s.GetLength(0); i++)
{
Console.Write("请输入学生姓名:");
s[i, 0] = Console.ReadLine();
Console.Write("请输入学生的成绩:");
s[i, 1] = Console.ReadLine();
Console.WriteLine();
}
Console.Write("请输入本次评优的老师数量:");
string[,] t = new string[int.Parse(Console.ReadLine()),2];
for (int i = 0; i < s.GetLength(0); i++)
{
Console.Write("请输入老师姓名:");
t[i, 0] = Console.ReadLine();
Console.Write("请输入老师的论文数量:");
t[i, 1] = Console.ReadLine();
Console.WriteLine();
}
Console.WriteLine("----优秀学生榜----");
for (int i = 0; i < s.GetLength(0); i++)
{
Student student = new Student(s[i, 0].ToCharArray(), int.Parse(s[i, 1]));
if (student.Isgood() == 1)
Console.WriteLine(student.print());
}
Console.WriteLine("\n----优秀老师榜----");
for (int i = 0; i < s.GetLength(0); i++)
{
Teacher teacher = new Teacher(t[i, 0].ToCharArray(), int.Parse(t[i, 1]));
if (teacher.Isgood() == 1)
Console.WriteLine(teacher.print());
}
}
class Base
{
protected char[] Name = new char[8];
protected int Num;
public Base() { }
public Base(char[] name)
{
Name = name;
}
public string print()
{
return $"我是{string.Join("",Name)},我的成果是{Num}(分/篇),所以我很优秀哦!";
}
public virtual int Isgood() { return 0; }
}
class Student : Base
{
public Student(char[] name,int num)
:base(name)
{
Num = num;
}
public override int Isgood()
{
if (Num > 90)
return 1;
else
return 0;
}
}
class Teacher : Base
{
public Teacher(char[] name,int num)
:base (name)
{
Num = num;
}
public override int Isgood()
{
if (Num > 3)
return 1;
else
return 0;
}
}
}
运行结果:
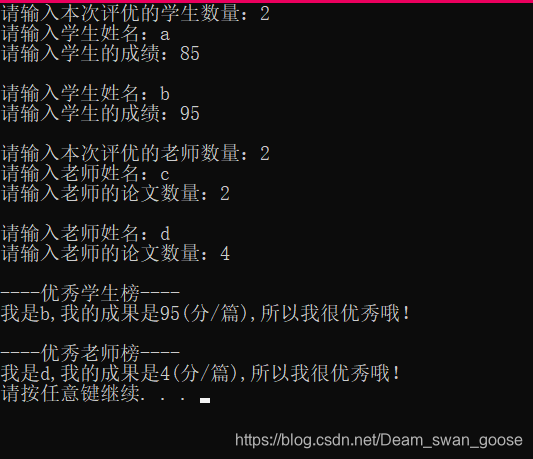
8.
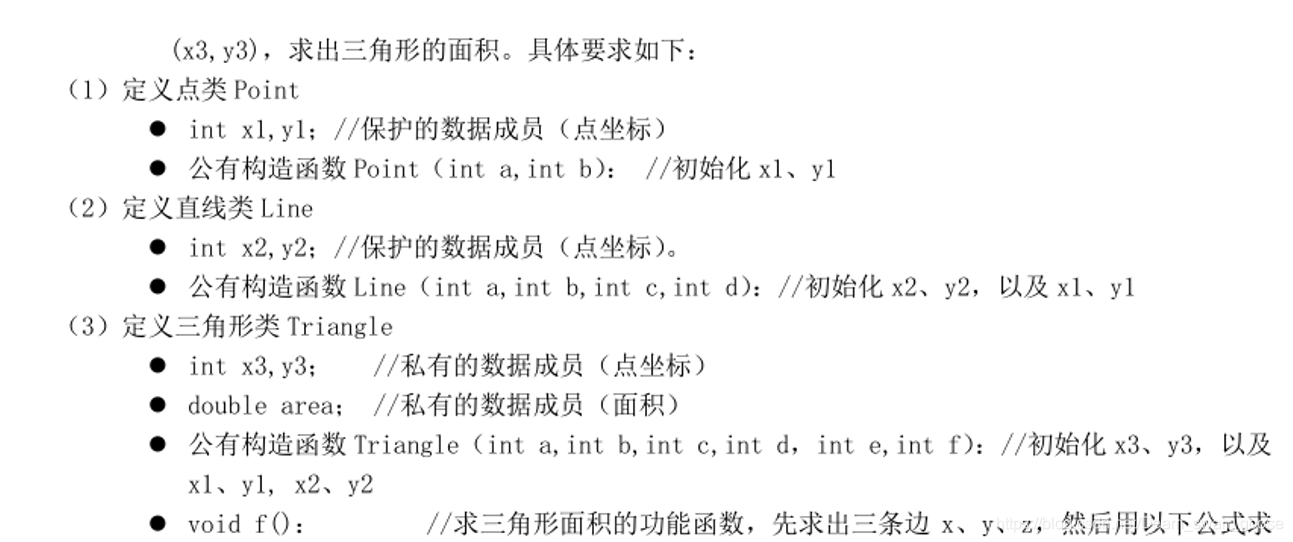
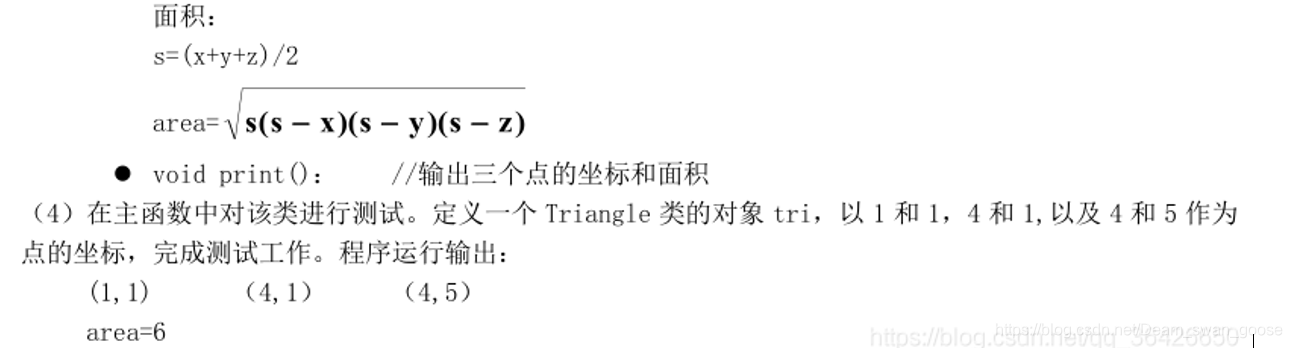
基础题不难。
代码如下:
class Program
{
static void Main(string[] args)
{
Triangle tri = new Triangle(1, 1, 4, 1, 4, 5);
tri.f();
tri.Print();
}
}
class Point
{
protected int x1 { get; set; } = 0;
protected int y1 { get; set; } = 0;
public Point() { }
public Point(int a ,int b)
{
x1 = a;
y1 = b;
}
}
class Line : Point
{
protected int x2 { get; set; } = 0;
protected int y2 { get; set; } = 0;
public Line() { }
public Line(int a,int b,int c,int d)
:base(a, b)
{
x2 = c;
y2 = d;
}
}
class Triangle : Line
{
private int x3 { get; set; } = 0;
private int y3 { get; set; } = 0;
private double area { get; set; } = 0;
public Triangle(int a, int b, int c, int d, int e,int f)
:base(a, b, c, d)
{
x3 = e;
y3 = f;
}
public void f()
{
double x = Math.Sqrt((x1 - x2) * (x1 - x2) + (y1 - y2) * (y1 - y2));
double y = Math.Sqrt((x1 - x3) * (x1 - x3) + (y1 - y3) * (y1 - y3));
double z = Math.Sqrt((x3 - x2) * (x3 - x2) + (y3 - y2) * (y3 - y2));
double s = (x + y + z) / 2;
area = Math.Sqrt(s * (s - x) * (s - y) * (s - z));
}
public void Print()
{
Console.WriteLine($"({x1},{y1})\t({x2},{y2})\t({x3},{y3})");
Console.WriteLine($"area = {area}");
}
}
运行结果:
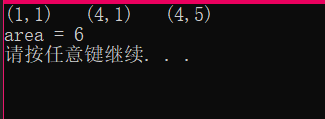