Arc类是JavaxFX的一部分。圆弧类在指定的某些给定值上创建圆弧,例如圆弧的中心,起始角度,圆弧的长度(长度)和半径。弧形类扩展了形状类。
该类的构造函数是
Arc():创建弧类的空实例
Arc(double centerX, double centerY, double radiusX, double radiusY, double startAngle, double length):创建具有给定坐标和值的弧
常用方法
方法
说明
getCenterX()
返回圆弧中心的x坐标
getCenterY()
返回圆弧中心的y坐标
getRadiusX()
返回圆弧半径的x坐标
getRadiusY()
返回圆弧半径的y坐标
getStartAngle()
返回弧的起始角度
getType()
获取属性类型的值。
setCenterX(double v)
设置圆弧中心的x坐标
setCenterY(double v)
设置圆弧中心的y坐标
setLength(double v)
设置圆弧的长度
setRadiusX(double v)
设置圆弧的X半径
setRadiusY(double v)
设置圆弧的y半径
setStartAngle(double v)
设置圆弧的起始角度
setType(ArcType v)
设置圆弧的圆弧类型
以下程序将说明Arc类的用法
Java程序创建弧
该程序将创建一个名为arc的圆弧(将中心,半径,起始角度和圆弧长度作为参数传递)。弧将在场景内创建,而场景又将在场景内托管。函数setTitle()用于为舞台提供标题。然后创建一个组,并将弧附加到该组上。最后,调用show()方法以显示最终结果。
// Java program to create a arc
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.shape.DrawMode;
import javafx.scene.layout.*;
import javafx.event.ActionEvent;
import javafx.scene.shape.Arc;
import javafx.scene.control.*;
import javafx.stage.Stage;
import javafx.scene.Group;
public class arc_0 extends Application {
// launch the application
public void start(Stage stage)
{
// set title for the stage
stage.setTitle("creating arc");
// create a arc
Arc arc = new Arc(100.0f, 100.0f, 100.0f, 100.0f, 0.0f, 100.0f);
// create a Group
Group group = new Group(arc);
// translate the arc to a position
arc.setTranslateX(100);
arc.setTranslateY(100);
// create a scene
Scene scene = new Scene(group, 500, 300);
// set the scene
stage.setScene(scene);
stage.show();
}
public static void main(String args[])
{
// launch the application
launch(args);
}
}
输出:
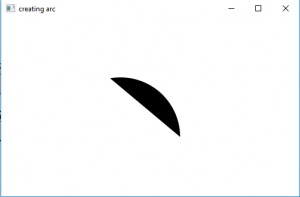
Java程序创建弧并为弧设置填充
该程序创建一个以名称arc表示的弧(使用setCenterX(),setCenterY(),setRadiusX(),setRadiusY(),setLength()设置中心,半径,起始角度和弧长)。弧将在场景内创建,而场景又将在场景内托管。函数setTitle()用于为舞台提供标题。然后创建一个组,并将弧附加到该组上。最后,调用show()方法以显示最终结果。功能setFill()用于设置圆弧的填充。
// Java program to create a arc
// and set fill for the arc
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.shape.DrawMode;
import javafx.scene.layout.*;
import javafx.event.ActionEvent;
import javafx.scene.shape.Arc;
import javafx.scene.control.*;
import javafx.stage.Stage;
import javafx.scene.Group;
import javafx.scene.shape.ArcType;
import javafx.scene.paint.Color;
public class arc_1 extends Application {
// launch the application
public void start(Stage stage)
{
// set title for the stage
stage.setTitle("creating arc");
// create a arc
Arc arc = new Arc();
// set center
arc.setCenterX(100.0f);
arc.setCenterY(100.0f);
// set radius
arc.setRadiusX(100.0f);
arc.setRadiusY(100.0f);
// set start angle and length
arc.setStartAngle(0.0f);
arc.setLength(270.0f);
// create a Group
Group group = new Group(arc);
// translate the arc to a position
arc.setTranslateX(100);
arc.setTranslateY(100);
// set fill for the arc
arc.setFill(Color.BLUE);
// create a scene
Scene scene = new Scene(group, 500, 300);
// set the scene
stage.setScene(scene);
stage.show();
}
public static void main(String args[])
{
// launch the application
launch(args);
}
}
输出:
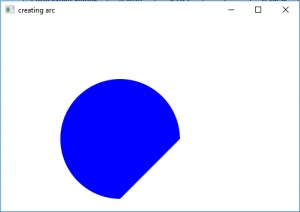
Java程序创建圆弧并指定其填充和圆弧类型
该程序创建一个以名称arc表示的弧(使用setCenterX(),setCenterY(),setRadiusX(),setRadiusY(),setLength()设置中心,半径,起始角度和弧长)。弧将在场景内创建,而场景又将在场景内托管。函数setTitle()用于为舞台提供标题。然后创建一个组,并将弧附加到该组上。最后,调用show()方法以显示最终结果。功能setFill()用于设置圆弧的填充,功能setArcType()用于设置圆弧的ArcType。
// Java Program to create a arc and specify its fill and arc type
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.shape.DrawMode;
import javafx.scene.layout.*;
import javafx.event.ActionEvent;
import javafx.scene.shape.Arc;
import javafx.scene.control.*;
import javafx.stage.Stage;
import javafx.scene.Group;
import javafx.scene.shape.ArcType;
import javafx.scene.paint.Color;
public class arc_2 extends Application {
// launch the application
public void start(Stage stage)
{
// set title for the stage
stage.setTitle("creating arc");
// create a arc
Arc arc = new Arc();
// set center
arc.setCenterX(100.0f);
arc.setCenterY(100.0f);
// set radius
arc.setRadiusX(100.0f);
arc.setRadiusY(100.0f);
// set start angle and length
arc.setStartAngle(0.0f);
arc.setLength(270.0f);
// create a Group
Group group = new Group(arc);
// translate the arc to a position
arc.setTranslateX(100);
arc.setTranslateY(100);
// set fill for the arc
arc.setFill(Color.BLUE);
// set Type of Arc
arc.setType(ArcType.ROUND);
// create a scene
Scene scene = new Scene(group, 500, 300);
// set the scene
stage.setScene(scene);
stage.show();
}
public static void main(String args[])
{
// launch the application
launch(args);
}
}