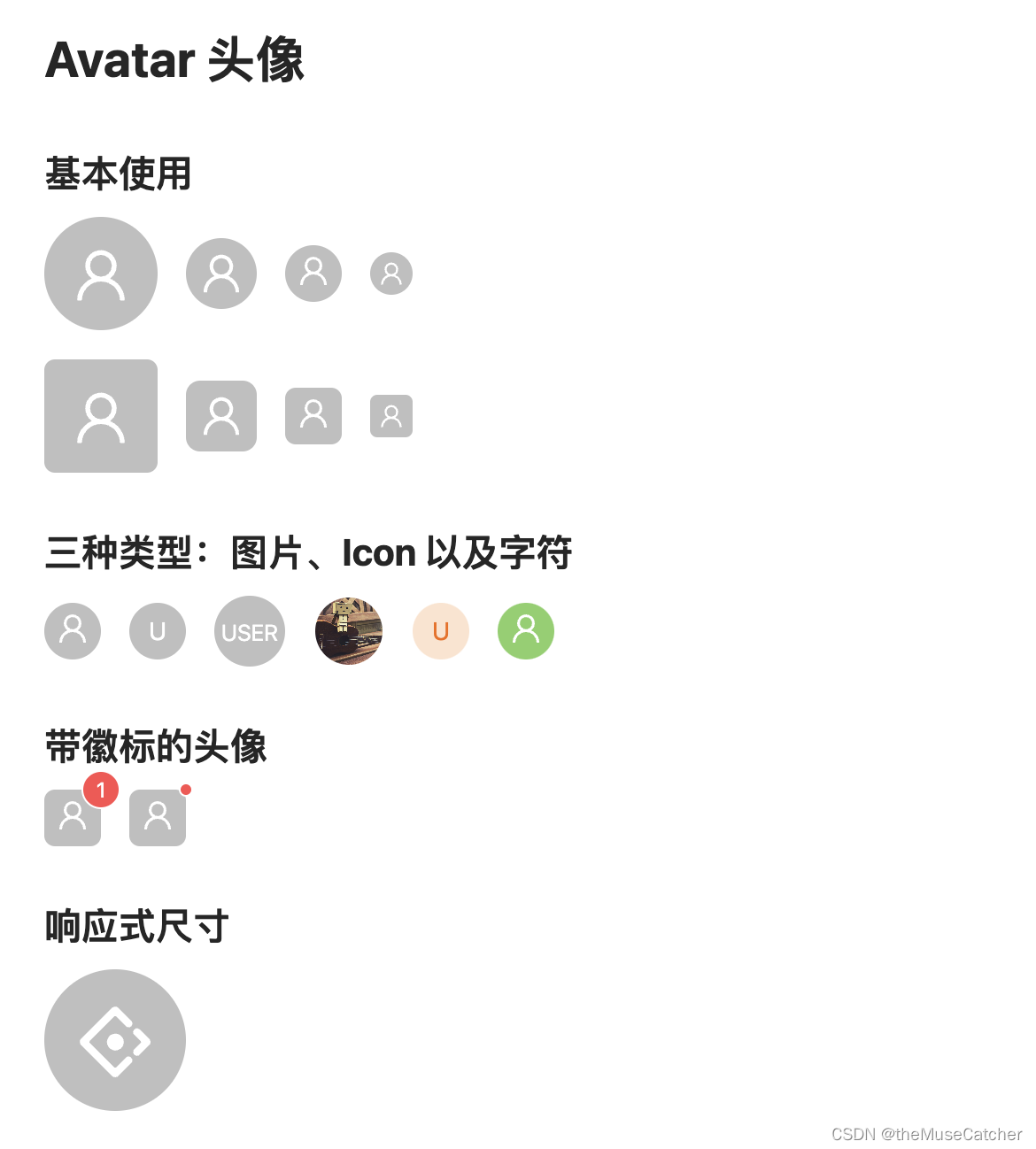
APIs
参数 |
说明 |
类型 |
默认值 |
必传 |
shape |
指定头像的形状 |
‘circle’ | ‘square’ |
‘circle’ |
false |
size |
设置头像的大小 |
number | ‘large’ | ‘small’ | ‘default’ | Responsive |
‘default’ |
false |
src |
图片类头像资源地址 |
string |
‘’ |
false |
alt |
图片无法显示时的替代文本 |
string |
‘’ |
false |
icon |
设置头像的图标 |
slot |
undefined |
false |
Responsive Type
名称 |
说明 |
类型 |
必传 |
xs |
<576px 响应式栅格 |
number |
false |
sm |
≥576px 响应式栅格 |
number |
false |
md |
≥768px 响应式栅格 |
number |
false |
lg |
≥992px 响应式栅格 |
number |
false |
xl |
≥1200px 响应式栅格 |
number |
false |
xxl |
≥1600px 响应式栅格 |
number |
false |
创建头像组件Avatar.vue
<script setup lang="ts">
import { ref, computed, onMounted, onUnmounted, nextTick } from 'vue'
import type { Slot } from 'vue'
interface Responsive {
xs?: number // <576px 响应式栅格
sm?: number // ≥576px 响应式栅格
md?: number // ≥768px 响应式栅格
lg?: number // ≥992px 响应式栅格
xl?: number // ≥1200px 响应式栅格
xxl?: number // ≥1600px 响应式栅格
}
interface Props {
shape?: 'circle'|'square' // 指定头像的形状
size?: number|'large'|'small'|'default'|Responsive // 设置头像的大小
src?: string // 图片类头像资源地址
alt?: string // 图片无法显示时的替代文本
icon?: Slot // 设置头像的图标
}
const props = withDefaults(defineProps<Props>(), {
shape: 'circle',
size: 'default',
src: '',
alt: '',
icon: undefined
})
const clientWidth = ref(document.documentElement.clientWidth)
const iconRef = ref()
const showIcon = ref(1)
const strRef = ref()
const showStr = ref(1)
onMounted(() => {
window.addEventListener('resize', getBrowserSize)
if (!props.src) {
showIcon.value = iconRef.value.offsetHeight
nextTick(() => {
if (!showIcon.value) {
showStr.value = strRef.value.offsetHeight
}
})
}
})
onUnmounted(() => {
window.removeEventListener('resize', getBrowserSize)
})
function getBrowserSize () {
// document.documentElement返回<html>元素
clientWidth.value = document.documentElement.clientWidth
}
const avatarStyle = computed(() => {
if (typeof props.size === 'string') {
return null
}
if (typeof props.size === 'number') {
if (showIcon.value) {
return {
width: props.size + 'px',
height: props.size + 'px',
lineHeight: props.size + 'px',
fontSize: (props.size as number / 2) + 'px'
}
} else {
return {
width: props.size + 'px',
height: props.size + 'px',
lineHeight: props.size + 'px',
fontSize: '18px'
}
}
}
if (typeof props.size === 'object') {
let size = 32
if (clientWidth.value >= 1600 && props.size.xxl) {
size = props.size.xxl
} else if (clientWidth.value >= 1200 && props.size.xl) {
size = props.size.xl
} else if (clientWidth.value >= 992 && props.size.lg) {
size = props.size.lg
} else if (clientWidth.value >= 768 && props.size.md) {
size = props.size.md
} else if (clientWidth.value >= 576 && props.size.sm) {
size = props.size.sm
} else if (clientWidth.value < 576 && props.size.xs) {
size = props.size.xs
}
return {
width: size + 'px',
height: size + 'px',
lineHeight: size + 'px',
fontSize: (size / 2) + 'px'
}
}
})
const strStyle = computed(() => {
if (typeof props.size === 'string') {
return {
transform: `scale(1) translateX(-50%)`
}
}
if (typeof props.size === 'number') {
const scale = Math.min(1, Math.max(1/45, (1 + (props.size - 9) * 1) / 45))
return {
lineHeight: props.size + 'px',
transform: `scale(${scale}) translateX(-50%)`
}
}
})
</script>
<template>
<div
class="m-avatar"
:class="[avatarStyle === null ? 'avatar-' + size: '', 'avatar-' + shape, {'avatar-image': src}]"
:style="avatarStyle || {}">
<img class="u-image" :src="src" :alt="alt" v-if="src" />
<span class="m-icon" ref="iconRef" v-if="!src && showIcon">
<slot name="icon"></slot>
</span>
<span class="m-string" :style="strStyle" ref="strRef" v-if="!src && !showIcon && showStr">
<slot></slot>
</span>
</div>
</template>
<style lang="less" scoped>
.m-avatar {
color: #fff;
font-size: 14px;
line-height: 30px;
position: relative;
display: inline-block;
overflow: hidden;
white-space: nowrap;
text-align: center;
vertical-align: middle;
background: rgba(0, 0, 0, .25);
border: 1px solid transparent;
width: 32px;
height: 32px;
border-radius: 50%;
&.avatar-square {
border-radius: 6px;
}
.u-image {
display: block;
width: 100%;
height: 100%;
object-fit: cover;
}
.m-icon {
display: inline-flex;
align-items: center;
color: inherit;
line-height: 0;
text-align: center;
vertical-align: -0.125em;
}
.m-string {
position: absolute;
left: 50%;
transform-origin: 0 center;
}
}
.avatar-large {
font-size: 24px;
width: 40px;
height: 40px;
line-height: 38px;
border-radius: 50%;
.m-icon {
font-size: 24px;
}
&.avatar-square {
border-radius: 8px;
}
}
.avatar-default {
.m-icon {
font-size: 18px;
}
}
.avatar-small {
font-size: 14px;
width: 24px;
height: 24px;
line-height: 22px;
border-radius: 50%;
.m-icon {
font-size: 14px;
}
&.avatar-square {
border-radius: 4px;
}
}
.avatar-image {
background: transparent;
}
</style>
在要使用的页面引入
其中引入使用了 Vue3间距(Space)、Vue3徽标数(Badge)
<script setup lang="ts">
import Avatar from './Avatar.vue'
import Space from './Space.vue'
import Badge from './Badge.vue'
</script>
<template>
<div>
<h1>Avatar 头像</h1>
<h2 class="mt30 mb10">基本使用</h2>
<Space :size="16" align="center">
<Avatar :size="64">
<template #icon>
<svg focusable="false" class="u-icon" data-icon="user" width="1em" height="1em" fill="currentColor" aria-hidden="true" viewBox="64 64 896 896"><path d="M858.5 763.6a374 374 0 00-80.6-119.5 375.63 375.63 0 00-119.5-80.6c-.4-.2-.8-.3-1.2-.5C719.5 518 760 444.7 760 362c0-137-111-248-248-248S264 225 264 362c0 82.7 40.5 156 102.8 201.1-.4.2-.8.3-1.2.5-44.8 18.9-85 46-119.5 80.6a375.63 375.63 0 00-80.6 119.5A371.7 371.7 0 00136 901.8a8 8 0 008 8.2h60c4.4 0 7.9-3.5 8-7.8 2-77.2 33-149.5 87.8-204.3 56.7-56.7 132-87.9 212.2-87.9s155.5 31.2 212.2 87.9C779 752.7 810 825 812 902.2c.1 4.4 3.6 7.8 8 7.8h60a8 8 0 008-8.2c-1-47.8-10.9-94.3-29.5-138.2zM512 534c-45.9 0-89.1-17.9-121.6-50.4S340 407.9 340 362c0-45.9 17.9-89.1 50.4-121.6S466.1 190 512 190s89.1 17.9 121.6 50.4S684 316.1 684 362c0 45.9-17.9 89.1-50.4 121.6S557.9 534 512 534z"></path></svg></template>
</Avatar>
<Avatar size="large">
<template #icon>
<svg focusable="false" class="u-icon" data-icon="user" width="1em" height="1em" fill="currentColor" aria-hidden="true" viewBox="64 64 896 896"><path d="M858.5 763.6a374 374 0 00-80.6-119.5 375.63 375.63 0 00-119.5-80.6c-.4-.2-.8-.3-1.2-.5C719.5 518 760 444.7 760 362c0-137-111-248-248-248S264 225 264 362c0 82.7 40.5 156 102.8 201.1-.4.2-.8.3-1.2.5-44.8 18.9-85 46-119.5 80.6a375.63 375.63 0 00-80.6 119.5A371.7 371.7 0 00136 901.8a8 8 0 008 8.2h60c4.4 0 7.9-3.5 8-7.8 2-77.2 33-149.5 87.8-204.3 56.7-56.7 132-87.9 212.2-87.9s155.5 31.2 212.2 87.9C779 752.7 810 825 812 902.2c.1 4.4 3.6 7.8 8 7.8h60a8 8 0 008-8.2c-1-47.8-10.9-94.3-29.5-138.2zM512 534c-45.9 0-89.1-17.9-121.6-50.4S340 407.9 340 362c0-45.9 17.9-89.1 50.4-121.6S466.1 190 512 190s89.1 17.9 121.6 50.4S684 316.1 684 362c0 45.9-17.9 89.1-50.4 121.6S557.9 534 512 534z"></path></svg>
</template>
</Avatar>
<Avatar>
<template #icon>
<svg focusable="false" class="u-icon" data-icon="user" width="1em" height="1em" fill="currentColor" aria-hidden="true" viewBox="64 64 896 896"><path d="M858.5 763.6a374 374 0 00-80.6-119.5 375.63 375.63 0 00-119.5-80.6c-.4-.2-.8-.3-1.2-.5C719.5 518 760 444.7 760 362c0-137-111-248-248-248S264 225 264 362c0 82.7 40.5 156 102.8 201.1-.4.2-.8.3-1.2.5-44.8 18.9-85 46-119.5 80.6a375.63 375.63 0 00-80.6 119.5A371.7 371.7 0 00136 901.8a8 8 0 008 8.2h60c4.4 0 7.9-3.5 8-7.8 2-77.2 33-149.5 87.8-204.3 56.7-56.7 132-87.9 212.2-87.9s155.5 31.2 212.2 87.9C779 752.7 810 825 812 902.2c.1 4.4 3.6 7.8 8 7.8h60a8 8 0 008-8.2c-1-47.8-10.9-94.3-29.5-138.2zM512 534c-45.9 0-89.1-17.9-121.6-50.4S340 407.9 340 362c0-45.9 17.9-89.1 50.4-121.6S466.1 190 512 190s89.1 17.9 121.6 50.4S684 316.1 684 362c0 45.9-17.9 89.1-50.4 121.6S557.9 534 512 534z"></path></svg>
</template>
</Avatar>
<Avatar size="small">
<template #icon>
<svg focusable="false" class="u-icon" data-icon="user" width="1em" height="1em" fill="currentColor" aria-hidden="true" viewBox="64 64 896 896"><path d="M858.5 763.6a374 374 0 00-80.6-119.5 375.63 375.63 0 00-119.5-80.6c-.4-.2-.8-.3-1.2-.5C719.5 518 760 444.7 760 362c0-137-111-248-248-248S264 225 264 362c0 82.7 40.5 156 102.8 201.1-.4.2-.8.3-1.2.5-44.8 18.9-85 46-119.5 80.6a375.63 375.63 0 00-80.6 119.5A371.7 371.7 0 00136 901.8a8 8 0 008 8.2h60c4.4 0 7.9-3.5 8-7.8 2-77.2 33-149.5 87.8-204.3 56.7-56.7 132-87.9 212.2-87.9s155.5 31.2 212.2 87.9C779 752.7 810 825 812 902.2c.1 4.4 3.6 7.8 8 7.8h60a8 8 0 008-8.2c-1-47.8-10.9-94.3-29.5-138.2zM512 534c-45.9 0-89.1-17.9-121.6-50.4S340 407.9 340 362c0-45.9 17.9-89.1 50.4-121.6S466.1 190 512 190s89.1 17.9 121.6 50.4S684 316.1 684 362c0 45.9-17.9 89.1-50.4 121.6S557.9 534 512 534z"></path></svg>
</template>
</Avatar>
</Space>
<br />
<br />
<Space :size="16" align="center">
<Avatar shape="square" :size="64">
<template #icon>
<svg focusable="false" class="u-icon" data-icon="user" width="1em" height="1em" fill="currentColor" aria-hidden="true" viewBox="64 64 896 896"><path d="M858.5 763.6a374 374 0 00-80.6-119.5 375.63 375.63 0 00-119.5-80.6c-.4-.2-.8-.3-1.2-.5C719.5 518 760 444.7 760 362c0-137-111-248-248-248S264 225 264 362c0 82.7 40.5 156 102.8 201.1-.4.2-.8.3-1.2.5-44.8 18.9-85 46-119.5 80.6a375.63 375.63 0 00-80.6 119.5A371.7 371.7 0 00136 901.8a8 8 0 008 8.2h60c4.4 0 7.9-3.5 8-7.8 2-77.2 33-149.5 87.8-204.3 56.7-56.7 132-87.9 212.2-87.9s155.5 31.2 212.2 87.9C779 752.7 810 825 812 902.2c.1 4.4 3.6 7.8 8 7.8h60a8 8 0 008-8.2c-1-47.8-10.9-94.3-29.5-138.2zM512 534c-45.9 0-89.1-17.9-121.6-50.4S340 407.9 340 362c0-45.9 17.9-89.1 50.4-121.6S466.1 190 512 190s89.1 17.9 121.6 50.4S684 316.1 684 362c0 45.9-17.9 89.1-50.4 121.6S557.9 534 512 534z"></path></svg>
</template>
</Avatar>
<Avatar shape="square" size="large">
<template #icon>
<svg focusable="false" class="u-icon" data-icon="user" width="1em" height="1em" fill="currentColor" aria-hidden="true" viewBox="64 64 896 896"><path d="M858.5 763.6a374 374 0 00-80.6-119.5 375.63 375.63 0 00-119.5-80.6c-.4-.2-.8-.3-1.2-.5C719.5 518 760 444.7 760 362c0-137-111-248-248-248S264 225 264 362c0 82.7 40.5 156 102.8 201.1-.4.2-.8.3-1.2.5-44.8 18.9-85 46-119.5 80.6a375.63 375.63 0 00-80.6 119.5A371.7 371.7 0 00136 901.8a8 8 0 008 8.2h60c4.4 0 7.9-3.5 8-7.8 2-77.2 33-149.5 87.8-204.3 56.7-56.7 132-87.9 212.2-87.9s155.5 31.2 212.2 87.9C779 752.7 810 825 812 902.2c.1 4.4 3.6 7.8 8 7.8h60a8 8 0 008-8.2c-1-47.8-10.9-94.3-29.5-138.2zM512 534c-45.9 0-89.1-17.9-121.6-50.4S340 407.9 340 362c0-45.9 17.9-89.1 50.4-121.6S466.1 190 512 190s89.1 17.9 121.6 50.4S684 316.1 684 362c0 45.9-17.9 89.1-50.4 121.6S557.9 534 512 534z"></path></svg>
</template>
</Avatar>
<Avatar shape="square">
<template #icon>
<svg focusable="false" class="u-icon" data-icon="user" width="1em" height="1em" fill="currentColor" aria-hidden="true" viewBox="64 64 896 896"><path d="M858.5 763.6a374 374 0 00-80.6-119.5 375.63 375.63 0 00-119.5-80.6c-.4-.2-.8-.3-1.2-.5C719.5 518 760 444.7 760 362c0-137-111-248-248-248S264 225 264 362c0 82.7 40.5 156 102.8 201.1-.4.2-.8.3-1.2.5-44.8 18.9-85 46-119.5 80.6a375.63 375.63 0 00-80.6 119.5A371.7 371.7 0 00136 901.8a8 8 0 008 8.2h60c4.4 0 7.9-3.5 8-7.8 2-77.2 33-149.5 87.8-204.3 56.7-56.7 132-87.9 212.2-87.9s155.5 31.2 212.2 87.9C779 752.7 810 825 812 902.2c.1 4.4 3.6 7.8 8 7.8h60a8 8 0 008-8.2c-1-47.8-10.9-94.3-29.5-138.2zM512 534c-45.9 0-89.1-17.9-121.6-50.4S340 407.9 340 362c0-45.9 17.9-89.1 50.4-121.6S466.1 190 512 190s89.1 17.9 121.6 50.4S684 316.1 684 362c0 45.9-17.9 89.1-50.4 121.6S557.9 534 512 534z"></path></svg>
</template>
</Avatar>
<Avatar shape="square" size="small">
<template #icon>
<svg focusable="false" class="u-icon" data-icon="user" width="1em" height="1em" fill="currentColor" aria-hidden="true" viewBox="64 64 896 896"><path d="M858.5 763.6a374 374 0 00-80.6-119.5 375.63 375.63 0 00-119.5-80.6c-.4-.2-.8-.3-1.2-.5C719.5 518 760 444.7 760 362c0-137-111-248-248-248S264 225 264 362c0 82.7 40.5 156 102.8 201.1-.4.2-.8.3-1.2.5-44.8 18.9-85 46-119.5 80.6a375.63 375.63 0 00-80.6 119.5A371.7 371.7 0 00136 901.8a8 8 0 008 8.2h60c4.4 0 7.9-3.5 8-7.8 2-77.2 33-149.5 87.8-204.3 56.7-56.7 132-87.9 212.2-87.9s155.5 31.2 212.2 87.9C779 752.7 810 825 812 902.2c.1 4.4 3.6 7.8 8 7.8h60a8 8 0 008-8.2c-1-47.8-10.9-94.3-29.5-138.2zM512 534c-45.9 0-89.1-17.9-121.6-50.4S340 407.9 340 362c0-45.9 17.9-89.1 50.4-121.6S466.1 190 512 190s89.1 17.9 121.6 50.4S684 316.1 684 362c0 45.9-17.9 89.1-50.4 121.6S557.9 534 512 534z"></path></svg>
</template>
</Avatar>
</Space>
<h2 class="mt30 mb10">三种类型:图片、Icon 以及字符</h2>
<Space :size="16" align="center">
<Avatar>
<template #icon>
<svg focusable="false" class="u-icon" data-icon="user" width="1em" height="1em" fill="currentColor" aria-hidden="true" viewBox="64 64 896 896"><path d="M858.5 763.6a374 374 0 00-80.6-119.5 375.63 375.63 0 00-119.5-80.6c-.4-.2-.8-.3-1.2-.5C719.5 518 760 444.7 760 362c0-137-111-248-248-248S264 225 264 362c0 82.7 40.5 156 102.8 201.1-.4.2-.8.3-1.2.5-44.8 18.9-85 46-119.5 80.6a375.63 375.63 0 00-80.6 119.5A371.7 371.7 0 00136 901.8a8 8 0 008 8.2h60c4.4 0 7.9-3.5 8-7.8 2-77.2 33-149.5 87.8-204.3 56.7-56.7 132-87.9 212.2-87.9s155.5 31.2 212.2 87.9C779 752.7 810 825 812 902.2c.1 4.4 3.6 7.8 8 7.8h60a8 8 0 008-8.2c-1-47.8-10.9-94.3-29.5-138.2zM512 534c-45.9 0-89.1-17.9-121.6-50.4S340 407.9 340 362c0-45.9 17.9-89.1 50.4-121.6S466.1 190 512 190s89.1 17.9 121.6 50.4S684 316.1 684 362c0 45.9-17.9 89.1-50.4 121.6S557.9 534 512 534z"></path></svg>
</template>
</Avatar>
<Avatar>U</Avatar>
<Avatar :size="40">USER</Avatar>
<Avatar :size="40" src="https://cdn.jsdelivr.net/gh/themusecatcher/resources@0.0.3/1.jpg" />
<Avatar style="color: #f56a00; background-color: #fde3cf">U</Avatar>
<Avatar style="background-color: #87d068">
<template #icon>
<svg focusable="false" class="u-icon" data-icon="user" width="1em" height="1em" fill="currentColor" aria-hidden="true" viewBox="64 64 896 896"><path d="M858.5 763.6a374 374 0 00-80.6-119.5 375.63 375.63 0 00-119.5-80.6c-.4-.2-.8-.3-1.2-.5C719.5 518 760 444.7 760 362c0-137-111-248-248-248S264 225 264 362c0 82.7 40.5 156 102.8 201.1-.4.2-.8.3-1.2.5-44.8 18.9-85 46-119.5 80.6a375.63 375.63 0 00-80.6 119.5A371.7 371.7 0 00136 901.8a8 8 0 008 8.2h60c4.4 0 7.9-3.5 8-7.8 2-77.2 33-149.5 87.8-204.3 56.7-56.7 132-87.9 212.2-87.9s155.5 31.2 212.2 87.9C779 752.7 810 825 812 902.2c.1 4.4 3.6 7.8 8 7.8h60a8 8 0 008-8.2c-1-47.8-10.9-94.3-29.5-138.2zM512 534c-45.9 0-89.1-17.9-121.6-50.4S340 407.9 340 362c0-45.9 17.9-89.1 50.4-121.6S466.1 190 512 190s89.1 17.9 121.6 50.4S684 316.1 684 362c0 45.9-17.9 89.1-50.4 121.6S557.9 534 512 534z"></path></svg>
</template>
</Avatar>
</Space>
<h2 class="mt30 mb10">带徽标的头像</h2>
<Space :size="16">
<Badge :count="1">
<Avatar shape="square">
<template #icon>
<svg focusable="false" class="u-icon" data-icon="user" width="1em" height="1em" fill="currentColor" aria-hidden="true" viewBox="64 64 896 896"><path d="M858.5 763.6a374 374 0 00-80.6-119.5 375.63 375.63 0 00-119.5-80.6c-.4-.2-.8-.3-1.2-.5C719.5 518 760 444.7 760 362c0-137-111-248-248-248S264 225 264 362c0 82.7 40.5 156 102.8 201.1-.4.2-.8.3-1.2.5-44.8 18.9-85 46-119.5 80.6a375.63 375.63 0 00-80.6 119.5A371.7 371.7 0 00136 901.8a8 8 0 008 8.2h60c4.4 0 7.9-3.5 8-7.8 2-77.2 33-149.5 87.8-204.3 56.7-56.7 132-87.9 212.2-87.9s155.5 31.2 212.2 87.9C779 752.7 810 825 812 902.2c.1 4.4 3.6 7.8 8 7.8h60a8 8 0 008-8.2c-1-47.8-10.9-94.3-29.5-138.2zM512 534c-45.9 0-89.1-17.9-121.6-50.4S340 407.9 340 362c0-45.9 17.9-89.1 50.4-121.6S466.1 190 512 190s89.1 17.9 121.6 50.4S684 316.1 684 362c0 45.9-17.9 89.1-50.4 121.6S557.9 534 512 534z"></path></svg>
</template>
</Avatar>
</Badge>
<Badge dot>
<Avatar shape="square">
<template #icon>
<svg focusable="false" class="u-icon" data-icon="user" width="1em" height="1em" fill="currentColor" aria-hidden="true" viewBox="64 64 896 896"><path d="M858.5 763.6a374 374 0 00-80.6-119.5 375.63 375.63 0 00-119.5-80.6c-.4-.2-.8-.3-1.2-.5C719.5 518 760 444.7 760 362c0-137-111-248-248-248S264 225 264 362c0 82.7 40.5 156 102.8 201.1-.4.2-.8.3-1.2.5-44.8 18.9-85 46-119.5 80.6a375.63 375.63 0 00-80.6 119.5A371.7 371.7 0 00136 901.8a8 8 0 008 8.2h60c4.4 0 7.9-3.5 8-7.8 2-77.2 33-149.5 87.8-204.3 56.7-56.7 132-87.9 212.2-87.9s155.5 31.2 212.2 87.9C779 752.7 810 825 812 902.2c.1 4.4 3.6 7.8 8 7.8h60a8 8 0 008-8.2c-1-47.8-10.9-94.3-29.5-138.2zM512 534c-45.9 0-89.1-17.9-121.6-50.4S340 407.9 340 362c0-45.9 17.9-89.1 50.4-121.6S466.1 190 512 190s89.1 17.9 121.6 50.4S684 316.1 684 362c0 45.9-17.9 89.1-50.4 121.6S557.9 534 512 534z"></path></svg>
</template>
</Avatar>
</Badge>
</Space>
<h2 class="mt30 mb10">响应式尺寸</h2>
<Avatar :size="{ xs: 24, sm: 32, md: 40, lg: 64, xl: 80, xxl: 100 }">
<template #icon>
<svg focusable="false" class="u-icon" data-icon="ant-design" width="1em" height="1em" fill="currentColor" aria-hidden="true" viewBox="64 64 896 896"><path d="M716.3 313.8c19-18.9 19-49.7 0-68.6l-69.9-69.9.1.1c-18.5-18.5-50.3-50.3-95.3-95.2-21.2-20.7-55.5-20.5-76.5.5L80.9 474.2a53.84 53.84 0 000 76.4L474.6 944a54.14 54.14 0 0076.5 0l165.1-165c19-18.9 19-49.7 0-68.6a48.7 48.7 0 00-68.7 0l-125 125.2c-5.2 5.2-13.3 5.2-18.5 0L189.5 521.4c-5.2-5.2-5.2-13.3 0-18.5l314.4-314.2c.4-.4.9-.7 1.3-1.1 5.2-4.1 12.4-3.7 17.2 1.1l125.2 125.1c19 19 49.8 19 68.7 0zM408.6 514.4a106.3 106.2 0 10212.6 0 106.3 106.2 0 10-212.6 0zm536.2-38.6L821.9 353.5c-19-18.9-49.8-18.9-68.7.1a48.4 48.4 0 000 68.6l83 82.9c5.2 5.2 5.2 13.3 0 18.5l-81.8 81.7a48.4 48.4 0 000 68.6 48.7 48.7 0 0068.7 0l121.8-121.7a53.93 53.93 0 00-.1-76.4z"></path></svg>
</template>
</Avatar>
</div>
</template>
<style lang="less" scoped>
.u-icon {
display: inline-block;
fill: #fff;
}
</style>