1.编写程序,定义整型指针变量p,初始化整型一维数组a的首地址(数组a的长度为10),利用指针变量p实现从键盘输入10个整型数据到一维数组a中,并输出该数组中最大值和最大值元素的下标。
1.Write a C program that declares an integer type pointer variable initialized the initial address of array a (the length is 10). You’re your program use the pointer variable p to store the numbers to the one-dimensional array a form the keyboard inputting and output the maximum and it’s subscript of the array a.
#include<stdio.h>
int main()
{
int i,max,sub=0,a[10];
int *p=a;
for(i=0;i<10;i++)
scanf("%d",p+i);
max=a[0];
for(i=0;i<10;i++,p++)
{
if(*p>max)
{
max=*p;
sub=i+1;
}
}
printf("MAX=%d,subscript=%d",max,sub);
return 0;
}
2.编写程序,实现删除字符串s中的所有空格,其中实现字符串空格删除功能要求定义一个形式参数为字符指针的函数。
2.Write a C program that defines a function with a character pointer as formal parameter to delete the blank space in the string s.
#include<stdio.h>
void offspace(char *p)
{
int i,j;
for(i=0,j=0;p[i];i++)
{
if(p[i]!=' ')
{
p[j]=p[i];
j++;
}
}
p[j]='\0';
}
int main()
{
char s[101];
gets(s);
offspace(s);
puts(s);
return 0;
}
3.编写程序,定义字符指针变量p,实现输入一个字符串,按相反次序输出其中的所有字符。
3.Write a C program that initially accepts a string by defining the character pointer variable p. Have your program output every character in reverse order.
#include<stdio.h>
int main()
{
char s[101],*p=s;
int i=0,j;
gets(s);
for(;*p;){
i++;
p++;
}
p=s;
for(j=i-1;j>=0;j--)
putchar(*(p+j));
return 0;
}
#include<stdio.h>
int main()
{
char s[101],*p=s;
gets(s);
for(;*p;){
p++;
}
p--;
for(;p>=s;p--)
putchar(*p);
return 0;
}
4.指针与二维数组。下面程序的功能是求行列长度为5的二维数组方阵m的主对角线元素之和以及次对角线元素之和,请完善以下程序,并上机调试。
4.Pointer and Two-dimensional array. The function of the following C program that calculates the sums of the main diagonal line and the secondary diagonal line of the two-dimensional array (the lengths of the row and column are 5). Fill the vacant positions and debug the following program.
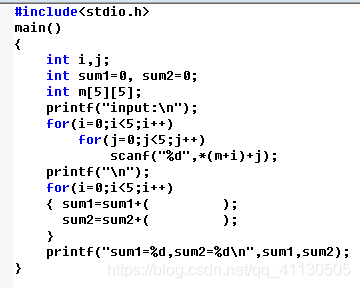
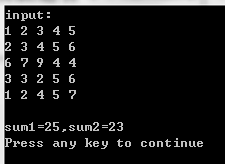
#include<stdio.h>
main()
{
int i,j;
int sum1=0,sum2=0;
int m[5][5];
printf("input:\n");
for(i=0;i<5;i++)
for(j=0;j<5;j++)
scanf("%d",*(m+i)+j);
printf("\n");
for(i=0;i<5;i++)
{
sum1=sum1+*(*(m+i)+i);
sum2=sum2+*(*(m+4-i)+i);
}
printf("sum1=%d,sum2=%d\n",sum1,sum2);
}
5.指针数组示例。使用指针数组求解组合问题,已知1个不透明的布袋里装有红、蓝、黄同样大小的圆球各1个,现从中1次抓出2个,问可能抓出的球颜色组合有哪些,完善程序,并上机调试,将输出结果截图。
5.Pointer arrays. Write a C program that calculates the combinatorial problem using the pointer array. There are the same size balls with red, blue and yellow in a nontransparent bag. Every color includes only one ball. When take out two balls at a time, how many combinations of possible ball colors? Fill the vacant positions and debug the following program.
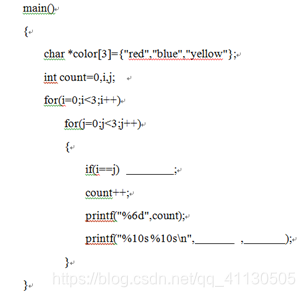
#include<stdio.h>
main()
{
char *color[3]={"red","blue","yellow"};
int count=0,i,j;
for(i=0;i<3;i++)
for(j=0;j<3;j++)
{
if(i==j)
continue;
count++;
printf("%6d",count);
printf("%10s%10s\n",color[i],color[j]);
}
}
因时间仓促,后三题是在CSDN用户qq_52027107的指导下完成,特此表示感谢。
本文内容由网友自发贡献,版权归原作者所有,本站不承担相应法律责任。如您发现有涉嫌抄袭侵权的内容,请联系:hwhale#tublm.com(使用前将#替换为@)