接着上一篇 Spring Cloud实战(四)-配置中心 现在开始搭建api模块
一.声明式接口模块api
1.pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<parent>
<artifactId>cloud-action</artifactId>
<groupId>com.example</groupId>
<version>0.0.1-SNAPSHOT</version>
</parent>
<modelVersion>4.0.0</modelVersion>
<artifactId>api</artifactId>
<properties>
<fastjson.version>1.2.80</fastjson.version>
</properties>
<dependencies>
<!-- eureka客户端-->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-client</artifactId>
</dependency>
<!-- 配置中心客户端-->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-config</artifactId>
</dependency>
<!-- 消息总线 -->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-bus-amqp</artifactId>
</dependency>
<!-- 配置文件自动刷新[https://docs.spring.io/spring-cloud-config/docs/current/reference/html/#_embedding_the_config_server] -->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-config-monitor</artifactId>
</dependency>
<!-- 声明式调用框架-->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-openfeign</artifactId>
</dependency>
<!-- 负载均衡器-->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-loadbalancer</artifactId>
</dependency>
<!-- 服务容错框架-->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-circuitbreaker-resilience4j</artifactId>
</dependency>
<!-- java校验 hibernate校验-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-validation</artifactId>
</dependency>
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
<version>${fastjson.version}</version>
</dependency>
</dependencies>
</project>
2.业务功能定义
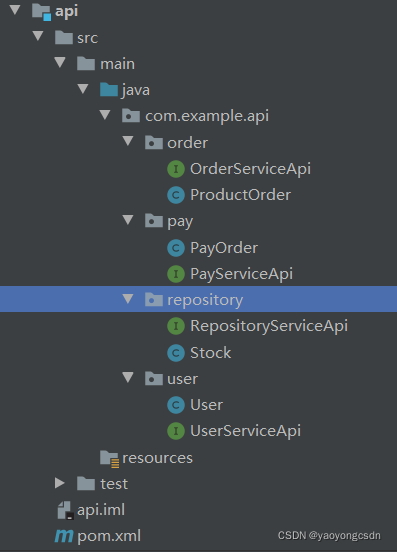
UserServiceApi.java
package com.example.api.user;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
/**
* @author 86188
*/
@RequestMapping(value = "/user")
public interface UserServiceApi {
/**
* 新增用户
* @param phone 手机号
* @return
*/
@RequestMapping(value = "/createUserByPhone",method = RequestMethod.POST)
User createUserByPhone(String phone);
}
OrderServiceApi.java
package com.example.api.order;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
/**
* @author 86188
*/
@RequestMapping(value = "/order")
public interface OrderServiceApi {
/**
* 新增订单
*
* @param order 订单
* @return
*/
@RequestMapping(value = "/createOrder", method = RequestMethod.POST)
ProductOrder createOrder(ProductOrder order);
}
RepositoryServiceApi.java
package com.example.api.repository;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
/**
* @author 86188
*/
@RequestMapping(value = "/repository")
public interface RepositoryServiceApi {
/**
* 减库存
*
* @param productId 商品id
* @param num 数量
* @return
*/
@RequestMapping(value = "/reduceProduct",method = RequestMethod.PUT)
Boolean reduceProduct(String productId, int num);
}
PayServiceApi.java
package com.example.api.pay;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
/**
* @author 86188
*/
@RequestMapping(value = "/pay")
public interface PayServiceApi {
/**
* 创建支付订单
*
* @return
*/
@RequestMapping(value = "/createPayOrder",method = RequestMethod.POST)
PayOrder createPayOrder();
}
3.说明
这个模块非常简单,主要用于其他业务模块接口声明,可以把一些公共依赖放在这里,或者单独再建一个commons模块.
好了,这个模块到这里就先结束了,后面会逐步实现具体业务作为服务的提供者,预计使用manage-server服务作为消费者,将会使用openfeign实现声明式调用.