介绍
Spring Cache是一个框架,实现了基于注解的缓存功能,只需要简单地加一个注解,就能实现缓存功能,大大简化我们在业务中操作缓存的代码。
Spring Cache只是提供了一层抽象,底层可以切换不同的cache实现。具体就是通过CacheManager接口来统一不同的缓存技术。CacheManager是Spring提供的各种缓存技术抽象接口。
针对不同的缓存技术需要实现不同的CacheManager:
CacheManager |
描述 |
EhCacheCacheManager |
使用EhCache作为缓存技术 |
GuavaCacheManager |
使用Google的GuavaCache作为缓存技术 |
RedisCacheManager |
使用Redis作为缓存技术 |
注解
在SpringCache中提供了很多缓存操作的注解,常见的是以下的几个:
注解 |
说明 |
@EnableCaching |
开启缓存注解功能 , 在启动类上添加 |
@Cacheable |
在方法执行前spring先查看缓存中是否有数据,如果有数据,则直接返回缓存数据;若没有数据,调用方法并将方法返回值放到缓存中 |
@CachePut |
将方法的返回值放到缓存中 |
@CacheEvict |
将一条或多条数据从缓存中删除 |
在spring boot项目中,使用缓存技术只需在项目中导入相关缓存技术的依赖包,并在启动类上使用@EnableCaching开启缓存支持即可。
例如,使用Redis作为缓存技术,只需要导入Spring data Redis的maven坐标即可。
使用SpringCach主要注意key的设计, 因为缓存数据,可能要缓存多份,这些数据不能混了, 就需要根据key去区分,而这个key往往都是动态的, 比如说我动态的使用当前用户的id作为key, 那这个id怎么获得呢, 它就给我们提供了一种SPEL表达式语言, 通过这种方式就可以动态的获取用户di
比如获取对象的 id 的 key的写法如下: 都可以用 , 推荐使用 user.id , 因为id是唯一的
#user.id : #user指的是方法形参的名称, id指的是user的id属性 , 也就是使用user的id属性作为key ;
#user.name: #user指的是方法形参的名称, name指的是user的name属性 ,也就是使用user的name属性作为key ;
#result.id : #result代表方法返回值,该表达式 代表以返回对象的id属性作为key ;
#result.name : #result代表方法返回值,该表达式 代表以返回对象的name属性作为key ;
使用redis来做缓存,主要需要操作以下几步:
在Spring Boot项目中使用 Spring Cache 的操作步骤 ( 使用redis缓存技术)
1. 导入maven坐标 : spring-boot-starter-cache , spring-boot-starter-data-redis
2. 配置application.yml 设置缓存有效期
3. 编写Redis的配置类RedisConfig,定义RedisTemplate
4. 在启动类上加入 @EnableCaching 注解 , 开启缓存注解功能
5. 在Controller的方法上加入@Cacheable . @CacheEvict , @CachePut 等注解 , 进行缓存操作
1). pom.xml 导入2个maven坐标 spring-boot-starter-cache , spring-boot-starter-data-redis
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-cache</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
2). 配置application.yml 加入redis相关配置 , 设置缓存有效期
spring:
redis:
host: 172.0.0.1
port: 6379
password: 123456 #自己的密码
database: 0
cache:
redis:
time-to-live: 1800000 #设置缓存过期时间30分钟,可选
3). 编写Redis的配置类RedisConfig,定义RedisTemplate
框架默认声明的RedisTemplate用的key和value的序列化方式是默认的 JdkSerializationRedisSerializer,如果key采用这种方式序列化,最终我们在测试时通过redis的图形化界面查询不是很方便, 所以使用我们自定义的RedisTemplate, key的序列化方式使用的是StringRedisSerializer, 也就是字符串形式


import org.springframework.cache.annotation.CachingConfigurerSupport;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.data.redis.connection.RedisConnectionFactory;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.data.redis.serializer.StringRedisSerializer;
@Configuration
public class RedisConfig extends CachingConfigurerSupport {
@Bean
public RedisTemplate<Object, Object> redisTemplate(RedisConnectionFactory connectionFactory) {
RedisTemplate<Object, Object> redisTemplate = new RedisTemplate<>();
//默认的Key序列化器为:JdkSerializationRedisSerializer
redisTemplate.setKeySerializer(new StringRedisSerializer());
redisTemplate.setConnectionFactory(connectionFactory);
return redisTemplate;
}
}
4). 在启动类上加入 @EnableCaching 注解 , 开启缓存注解功能
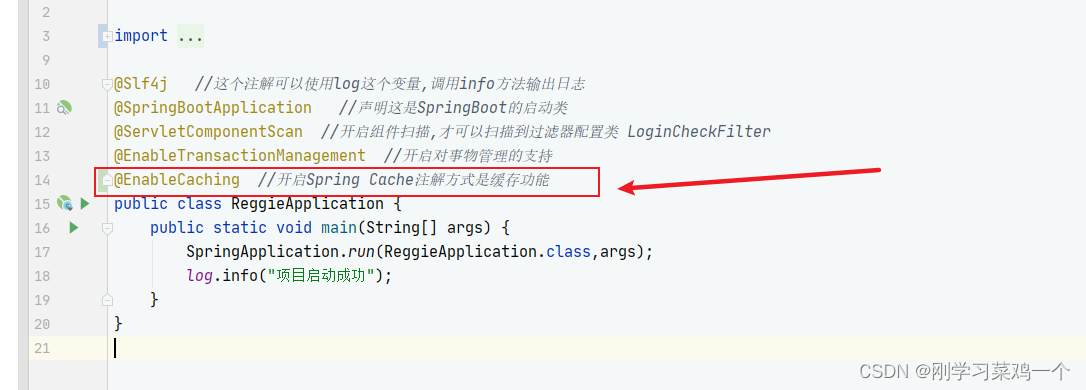
5). 在Controller的方法上加入@Cacheable . @CacheEvict , @CachePut 等注解 , 进行缓存操作
1. @Cacheble a注解说明:
作用: 在方法执行前,spring先查看缓存中是否有数据,如果有数据,则直接返回缓存数据;若没有数据,调用方法并将方法返回值放到缓存中
value: 缓存的名称,每个缓存名称下面可以有多个key
key: 缓存的key ----------> 支持Spring的表达式语言SPEL语法
condition : 表示满足什么条件, 再进行缓存 ;
unless : 表示满足条件则不缓存 ; 与上述的condition是反向的 ;
/**
* Cacheable:在方法执行前spring先查看缓存中是否有数据,如果有数据,则直接返回缓存数据;若没有数据,调用方法并将方法返回值放到缓存中
* value:缓存的名称,每个缓存名称下面可以有多个key
* key:缓存的key
* condition:条件,满足条件时才缓存数据 , 不能使用result里面是没有result对象的,
* unless:满足条件则不缓存
*/
@Cacheable(value = "userCache",key = "#id", unless = "#result == null")
@GetMapping("/{id}")
public User getById(@PathVariable Long id){
User user = userService.getById(id);
return user;
}
在list方法上加注解@Cacheable
在list方法中进行查询时,有两个查询条件,如果传递了id,根据id查询; 如果传递了name, 根据name查询,那么我们缓存的key在设计的时候,就需要既包含id,又包含name。
@Cacheable(value = "userCache",key = "#user.id + '_' + #user.name")
@GetMapping("/list")
public List<User> list(User user){
LambdaQueryWrapper<User> queryWrapper = new LambdaQueryWrapper<>();
queryWrapper.eq(user.getId() != null,User::getId,user.getId());
queryWrapper.eq(user.getName() != null,User::getName,user.getName());
List<User> list = userService.list(queryWrapper);
return list;
}
2. @CacheEvict 注解说明:
作用: 清理指定缓存
value: 缓存的名称,每个缓存名称下面可以有多个key
key: 缓存的key ----------> 支持Spring的表达式语言SPEL语法 , 可以动态的计算key的值
1). 在 delete 方法上加注解@CacheEvict 当我们在删除数据库user表的数据的时候,我们需要删除缓存中对应的数据,此时就可以使用@CacheEvict注解, 具体的使用方式如下:
* CacheEvict:清理指定缓存
* value:缓存的名称,每个缓存名称下面可以有多个key
* key:缓存的key
*/
//@CacheEvict(value = "userCache",key = "#p0") //#p0 代表第一个参数
//@CacheEvict(value = "userCache",key = "#root.args[0]") //#root.args[0] 代表第一个参数
@CacheEvict(value = "userCache",key = "#id") //#id 代表变量名为id的参数 推荐使用
@DeleteMapping("/{id}")
public void delete(@PathVariable Long id){
userService.removeById(id);
}
2). 在 update 方法上加注解@CacheEvict 在更新数据之后,数据库的数据已经发生了变更,我们需要将缓存中对应的数据删除掉,避免出现数据库数据与缓存数据不一致的情况。
//@CacheEvict(value = "userCache",key = "#p0.id") //第一个参数的id属性
//@CacheEvict(value = "userCache",key = "#root.args[0].id") //第一个参数的id属性
//@CacheEvict(value = "userCache",key = "#result.id") //返回值的id属性
@CacheEvict(value = "userCache",key = "#user.id") //参数名为user参数的id属性
@PutMapping
public User update(User user){
userService.updateById(user);
return user;
}
3) 新增套餐 删除套装的清除缓存 allEntries = true
/**
* 删除套餐
* @param ids
* @return
*/
@DeleteMapping
@CacheEvict(value = "setmealCache",allEntries = true) //清除setmealCache名称下,所有的缓存数据
public R<String> delete(@RequestParam List<Long> ids){
log.info("ids:{}",ids);
setmealService.removeWithDish(ids);
return R.success("套餐数据删除成功");
}
**
* 新增套餐
* @param setmealDto
* @return
*/
@PostMapping
@CacheEvict(value = "setmealCache",allEntries = true) //清除setmealCache名称下,所有的缓存数据
public R<String> save(@RequestBody SetmealDto setmealDto){
log.info("套餐信息:{}",setmealDto);
setmealService.saveWithDish(setmealDto);
return R.success("新增套餐成功");
}
3 . @CachePut 注解说明:
作用: 将方法返回值,放入缓存
value: 缓存的名称, 每个缓存名称下面可以有很多key
key: 缓存的key ----------> 支持Spring的表达式语言SPEL语法
1). 在save方法上加注解@CachePut
save方法是用来保存用户信息的,我们希望在该用户信息保存到数据库的同时,也往缓存中缓存一份数据,可以在save方法上加上注解 @CachePut
/**
* CachePut:将方法返回值放入缓存 现在使用的缓存底层是Map
* value:缓存的名称,每个缓存名称下面可以有多个key
* key:缓存的key
*/
@CachePut(value = "userCache", key = "#user.id")
@PostMapping
public User save(User user){
userService.save(user);
return user;
}