欢迎大家扫码关注我的微信公众号:
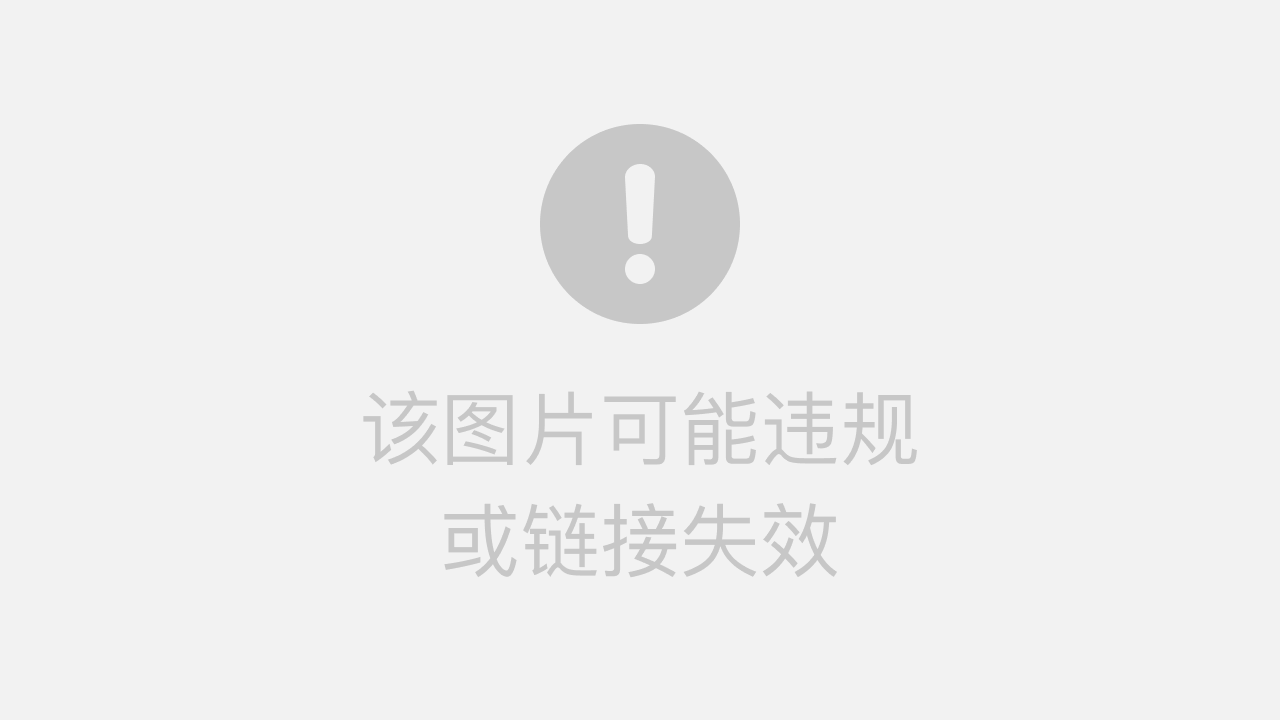
一、插入数据
MySQL 中使用 insert 语句来向数据库表中插入新的数据记录。
☆ 为表的所有字段插入数据
insert into tb_name (col_list) values (value_list)
创建一个数据表 person 如下:
>>> create table person(
id int unsigned not null primary key auto_increment,
name char(40) not null default "",
age int not null default 0,
info char(50) null
);
>>> show tables;
+-----------------+
| Tables_in_learn |
+-----------------+
| person |
+-----------------+
1 row in set (0.00 sec)
>>> select * from person:
Empty set (0.03 sec)
我们查看当前数据表显示已经创建成功,查看数据表中的数据,显示当前数据表为空。
接下来我们向表中插入数据:
>>> insert into person (id, name, age, info)
values (1, 'Green', 21, 'Lawyer');
Query OK, 1 row affected (0.00 sec)
插入数据时,不需要按照表定义的顺序插入,只要保证值的顺序与列字段的顺序相同就可以。这里不再演示。
在插入数据时,允许列名称列表 col_list 为空,值列表中需要为列的每一个字段指定值,并且值的顺序必须和数据表中字段定义时的顺序相同。如:
>>> insert into person
values (2, 'Lily', 18, 'teacher');
Query OK, 1 row affected (0.00 sec)
>>> select * from person;
+----+-------+-----+---------+
| id | name | age | info |
+----+-------+-----+---------+
| 1 | Green | 21 | Lawyer |
| 2 | Lily | 18 | teacher |
+----+-------+-----+---------+
2 rows in set (0.00 sec)
☆ 为表的指定字段插入数据
>>> insert into person (name, age, info)
values ('Lilei', 20, 'sports man');
Query OK, 1 row affected (0.00 sec)
>>> select * from person;
+----+-------+-----+------------+
| id | name | age | info |
+----+-------+-----+------------+
| 1 | Green | 21 | Lawyer |
| 2 | Lily | 18 | teacher |
| 3 | Lilei | 20 | sports man |
+----+-------+-----+------------+
3 rows in set (0.00 sec)
在 person 表中,id 字段为主键,不能为空,所以系统会自动为该字段插入自增的序列值。在插入数据时,如果某些字段没有指定插入值,MySQL 将插入该字段在 定义时的默认值。
如:
>>> insert into person (name, age)
values ('Xiaohua', 18);
Query OK, 1 row affected (0.01 sec)
>>> mysql> select * from person;
+----+---------+-----+------------+
| id | name | age | info |
+----+---------+-----+------------+
| 1 | Green | 21 | Lawyer |
| 2 | Lily | 18 | teacher |
| 3 | Lilei | 20 | sports man |
| 4 | Xiaohua | 18 | NULL |
+----+---------+-----+------------+
4 rows in set (0.00 sec)
可以看到,插入语句中没有指定 info 的值,所以 MySQL 自动为该字段插入了定义表时定义的默认值——空值。
☆ 同时插入多条数据
insert 语句可以同时向数据表中插入多条记录,插入时指定多个值列表,每个值列表之间用逗号隔开:
insert into tb_name (col_list) values (values_list1), (values_list2), ...
eg:
>>> insert into person (name, age, info)
values ('Evans', 22, 'student'),
('Jack', 27, 'singer'),
('Dawei', 33, 'cook');
Query OK, 3 rows affected (0.00 sec)
Records: 3 Duplicates: 0 Warnings: 0
>>> select * from person;
+----+---------+-----+------------+
| id | name | age | info |
+----+---------+-----+------------+
| 1 | Green | 21 | Lawyer |
| 2 | Lily | 18 | teacher |
| 3 | Lilei | 20 | sports man |
| 4 | Xiaohua | 18 | NULL |
| 5 | Evans | 22 | student |
| 6 | Jack | 27 | singer |
| 7 | Dawei | 33 | cook |
+----+---------+-----+------------+
7 rows in set (0.00 sec)
我们同样可以不指定插入字段列表,直接插入数据记录,但此时,我们插入的值列表的顺序及数据类型必须和表定义时的字段类型及数据类型匹配。
【注意】由于 MySQL 执行单条 insert 语句插入多行数据,比用多条 insert 语句要快,所以在插入多条记录时,最好选择使用单条 insert 语句。
☆ 将查询结果插入表中
如果我们想要把另外一个表合并个人信息到 person 表中,不需要把每条记录的值逐一输入,我们只需要一个 insert 语句和一个 select 语句组合在一起即可:
insert into tb_name1 (col_list1) select (col_list2) from tb_name2 where (condition)
即:我们根据 condition 条件从 tb_name2 的表中 select 出 col_list2 中指定的字段的值,然后 insert 到 tb_name1 的 col_list1 中相应的字段中。
首先,我们先新建一个 people 表,它具有和 person 表相同的字段名和数据类型,并向其内插入两条数据:
>>> create table people(
id int unsigned not null primary key auto_increment,
name char(40) not null default '',
age int not null default 0,
info char(50) null
);
Query OK, 0 rows affected (0.01 sec)
>>> insert into people
values (8, 'Xiaogang', 45, 'Doctor'),
(9, 'Eva', 35, 'police');
Query OK, 2 rows affected (0.01 sec)
Records: 2 Duplicates: 0 Warnings: 0
>>> select * from people;
+----+----------+-----+--------+
| id | name | age | info |
+----+----------+-----+--------+
| 8 | Xiaogang | 45 | Doctor |
| 9 | Eva | 35 | police |
+----+----------+-----+--------+
2 rows in set (0.00 sec)
现在,我们将 people 表中的数据插入到 person 表中:
>>> insert into person (id, name, age, info)
select id, name, age, info from people;
Query OK, 2 rows affected (0.00 sec)
Records: 2 Duplicates: 0 Warnings: 0
>>> select * from person;
+----+----------+-----+------------+
| id | name | age | info |
+----+----------+-----+------------+
| 1 | Green | 21 | Lawyer |
| 2 | Lily | 18 | teacher |
| 3 | Lilei | 20 | sports man |
| 4 | Xiaohua | 18 | NULL |
| 5 | Evans | 22 | student |
| 6 | Jack | 27 | singer |
| 7 | Dawei | 33 | cook |
| 8 | Xiaogang | 45 | Doctor |
| 9 | Eva | 35 | police |
+----+----------+-----+------------+
9 rows in set (0.01 sec)
成功!
id 字段为主键字段,在插入的时候要保证该字段值的唯一性,如果不能确定,可以忽略该字段,系统会自动为我们加上正确的值。
【注意】MySQL 并不关心 select 返回的列名,它其实是根据列的位置进行插入操作,第一列对应插入第一列,第二列对应插入第二列,以此类推,所以我们只要保证 select 出来的数据的 字段数量 与 insert 到的表的 col_list1 字段数量 相同即可,同时别忘了具有相同的数据类型。
二、更新数据
MySQL 使用 update 语句更新表中的记录, 可以更新特定的行或者同时更新所有的行。
update tb_name set col_name1 = value1, cul_name2 = value2, ... where condition
如:我们将 id 为 8 的记录中的 name 更新为 Harry,age 更新为 22:
>>> select * from person where id = 8;
+----+----------+-----+--------+
| id | name | age | info |
+----+----------+-----+--------+
| 8 | Xiaogang | 45 | Doctor |
+----+----------+-----+--------+
1 row in set (0.00 sec)
>>> update person set name='Harry', age=22 where id = 8;
Query OK, 1 row affected (0.01 sec)
Rows matched: 1 Changed: 1 Warnings: 0
>>> select * from person where id = 8;
+----+-------+-----+--------+
| id | name | age | info |
+----+-------+-----+--------+
| 8 | Harry | 22 | Doctor |
+----+-------+-----+--------+
1 row in set (0.00 sec)
现在我们要更新年龄在 18 ~ 22 之间的所有人的 info 为 student:
>>> select * from person where age between 18 and 22;
+----+---------+-----+------------+
| id | name | age | info |
+----+---------+-----+------------+
| 1 | Green | 21 | Lawyer |
| 2 | Lily | 18 | teacher |
| 3 | Lilei | 20 | sports man |
| 4 | Xiaohua | 18 | NULL |
| 5 | Evans | 22 | student |
| 8 | Harry | 22 | Doctor |
+----+---------+-----+------------+
6 rows in set (0.00 sec)
>>> update person set info = 'student' where age between 18 and 22;
Query OK, 5 rows affected (0.01 sec)
Rows matched: 6 Changed: 5 Warnings: 0
>>> select * from person where age between 18 and 22;
+----+---------+-----+---------+
| id | name | age | info |
+----+---------+-----+---------+
| 1 | Green | 21 | student |
| 2 | Lily | 18 | student |
| 3 | Lilei | 20 | student |
| 4 | Xiaohua | 18 | student |
| 5 | Evans | 22 | student |
| 8 | Harry | 22 | student |
+----+---------+-----+---------+
6 rows in set (0.00 sec)
成功!
三、删除数据
MySQL 使用 delete 语句进行数据的删除,同时允许 where 子句指定删除条件:
delete from tb_name [where condition]
其中,where condition 为可选的删除条件,如果没有这条子句,将删除整个表。
我们现在将 person 表中的 id 为 8 的数据删除:
>>> select * from person where id = 8;
+----+-------+-----+---------+
| id | name | age | info |
+----+-------+-----+---------+
| 8 | Harry | 22 | student |
+----+-------+-----+---------+
1 row in set (0.00 sec)
>>> delete from person where id = 8;
Query OK, 1 row affected (0.00 sec)
>>> select * from person where id = 8;
Empty set (0.00 sec)
再次查询显示记录为空,说明我们已经成功将 id 为 8 的数据记录删除。
我们现在删除 age 在 18 ~ 22 之间的数据记录:
>>> select * from person where age between 18 and 22;
+----+---------+-----+---------+
| id | name | age | info |
+----+---------+-----+---------+
| 1 | Green | 21 | student |
| 2 | Lily | 18 | student |
| 3 | Lilei | 20 | student |
| 4 | Xiaohua | 18 | student |
| 5 | Evans | 22 | student |
+----+---------+-----+---------+
5 rows in set (0.00 sec)
>>> delete from person where age between 18 and 22;
Query OK, 5 rows affected (0.00 sec)
>>> select * from person where age between 18 and 22;
Empty set (0.00 sec)
再次查询显示记录为空,说明已经成功删除响应数据记录。
假如我们现在要删除整个数据表的所有记录,那我们可以这样操作:
>>> select * from person;
+----+-------+-----+--------+
| id | name | age | info |
+----+-------+-----+--------+
| 6 | Jack | 27 | singer |
| 7 | Dawei | 33 | cook |
| 9 | Eva | 35 | police |
+----+-------+-----+--------+
3 rows in set (0.00 sec)
>>> delete from person ;
Query OK, 3 rows affected (0.00 sec)
>>> select * from person;
Empty set (0.00 sec)
现在只是将 person 整个表的数据清空了,但是表还存在:
>>> show tables;
+-----------------+
| Tables_in_learn |
+-----------------+
| people |
| person |
+-----------------+
2 rows in set (0.00 sec)
关于数据的删除,如果我们想删除一个数据表中的所有数据记录,我们还可以使用truncate table tb_name
语句。它的原理是直接将表删除,然后重新建一张跟原表一模一样的表,但是此时表中没有数据,相当于清空表中的所有数据:
>>> select * from people;
+----+----------+-----+--------+
| id | name | age | info |
+----+----------+-----+--------+
| 8 | Xiaogang | 45 | Doctor |
| 9 | Eva | 35 | police |
+----+----------+-----+--------+
2 rows in set (0.00 sec)
>>> truncate table people;
Query OK, 0 rows affected (0.01 sec)
>>> select * from people;
Empty set (0.00 sec)
>>> show tables;
+-----------------+
| Tables_in_learn |
+-----------------+
| people |
| person |
+-----------------+
2 rows in set (0.00 sec)