系列文章目录
基于PHP的旅游管理系统
基于SSM的毕业设计管理系统
基于SpringBoot的高校在线答疑管理系统
目录
系列文章目录
一、相关技术
二、系统功能
三、系统页面
1.管理员功能页面
2.学生功能页面
3.党组织功能页面
4.党员功能页面
5.前台功能页面
四、核心代码
一、相关技术
末尾获取源码
开发语言:Java
Java开发工具:JDK1.8
后端框架:SpringBoot
前端:采用Vue和HTML技术开发
数据库:MySQL5.7和Navicat管理工具结合
服务器:Tomcat8.5
开发软件:IDEA / Eclipse
是否Maven项目:是
二、系统功能
本党务管理系统主要包括五大功能模块,即管理员模块、学生模块、积极分子模块、党员、党建组织。
(1)管理员模块:主要功能有:首页、个人中心、学生管理、学院管理、专业管理、班级管理、积极分子管理、党员管理、党建组织管理、党员转入管理、党员转出管理、入党资料管理、党团活动管理、公告信息管理、系统管理。
(2)党员:首页、个人中心、党员转入管理、党员转出管理。
(3)学生:首页、个人中心、入党资料管理。
(4)党建组织:首页、个人中心、党员管理、党员转入管理、党员转出管理。
三、系统页面
1.管理员功能页面
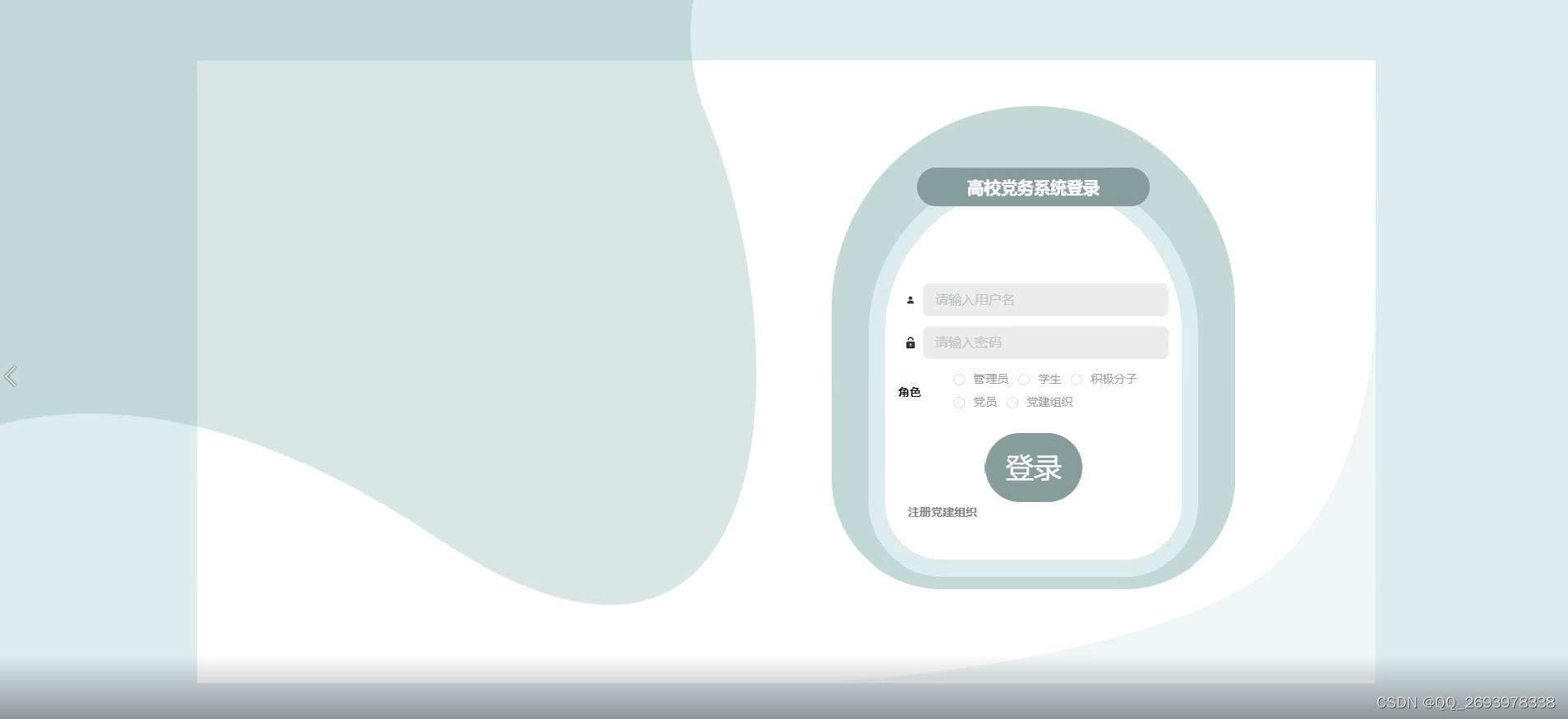
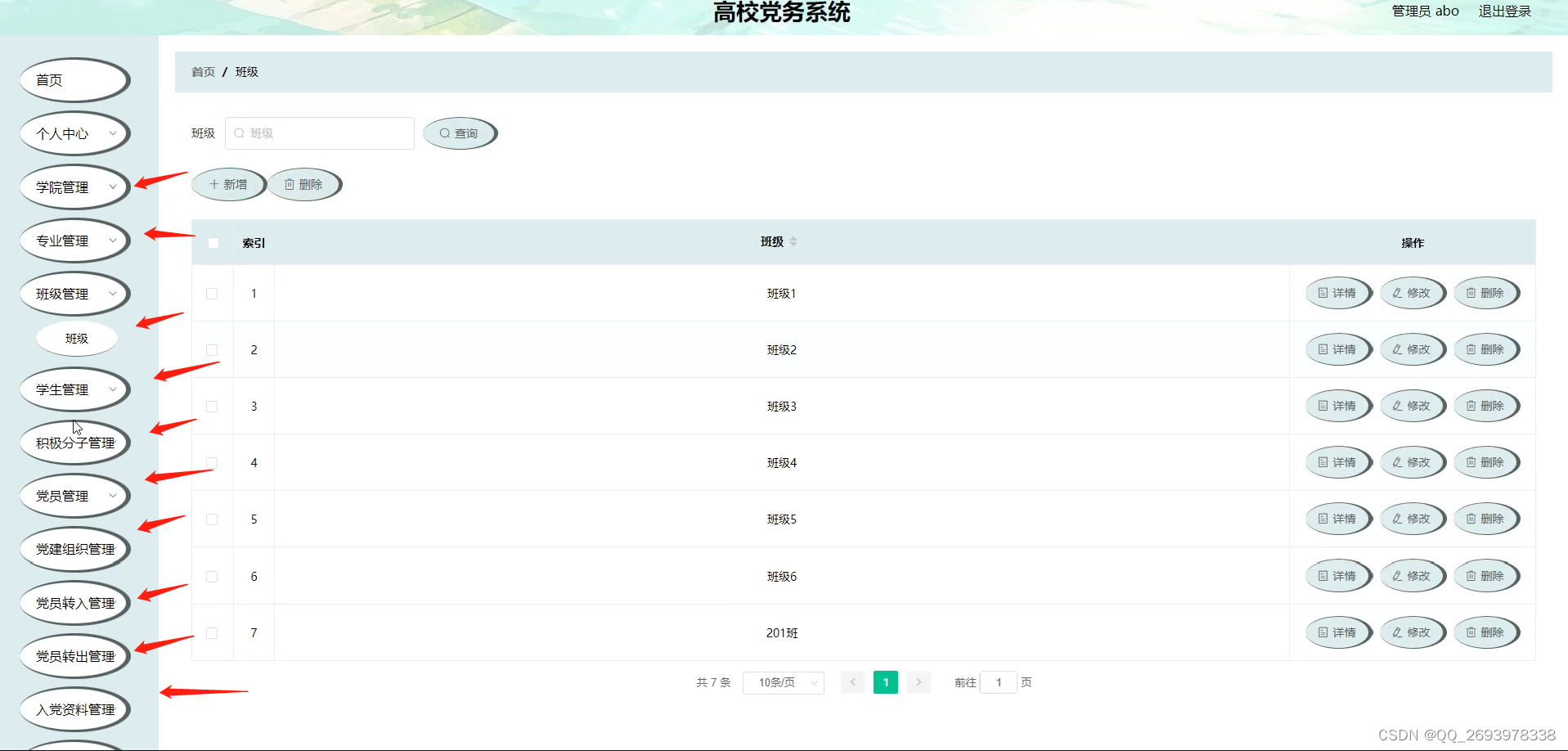
2.学生功能页面
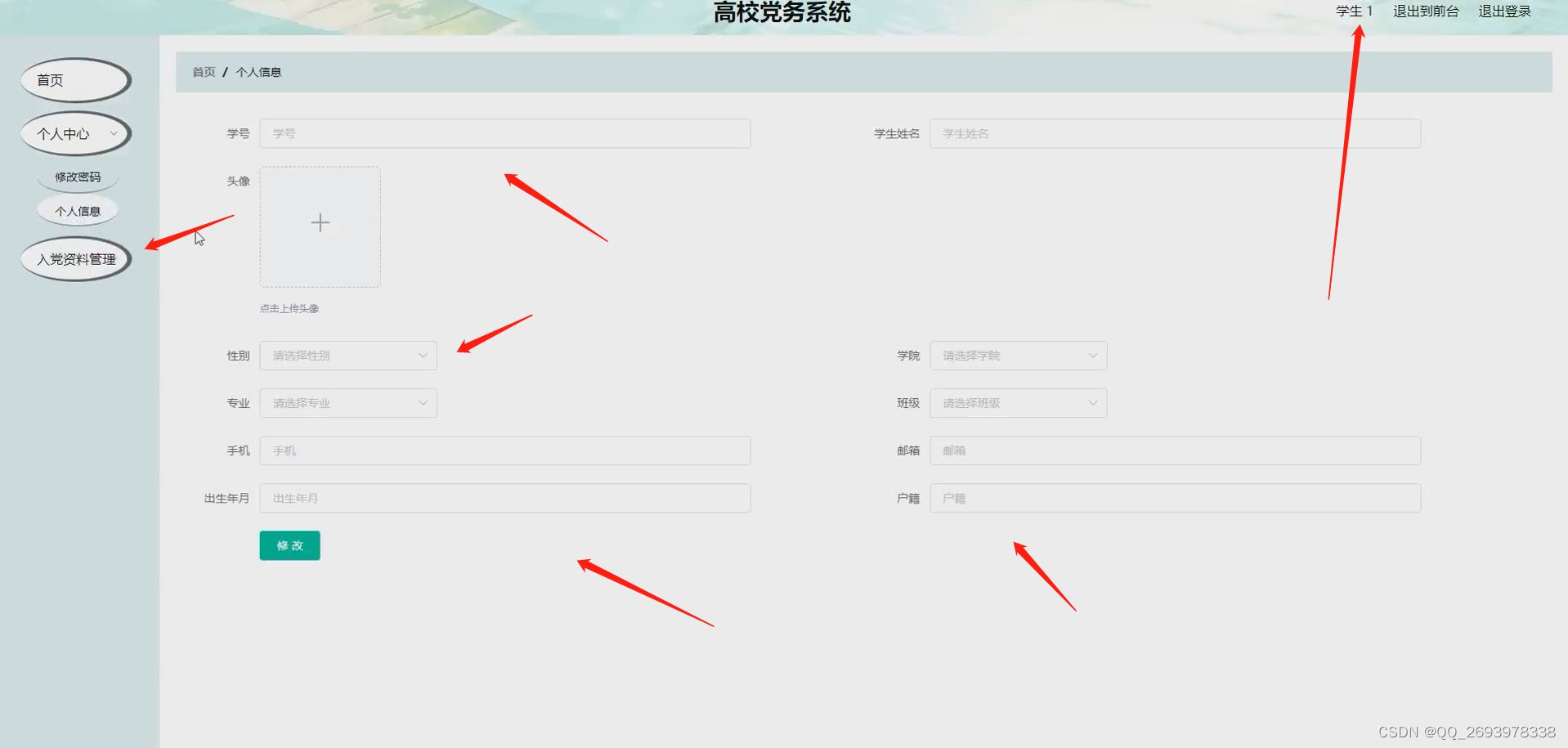
3.党组织功能页面
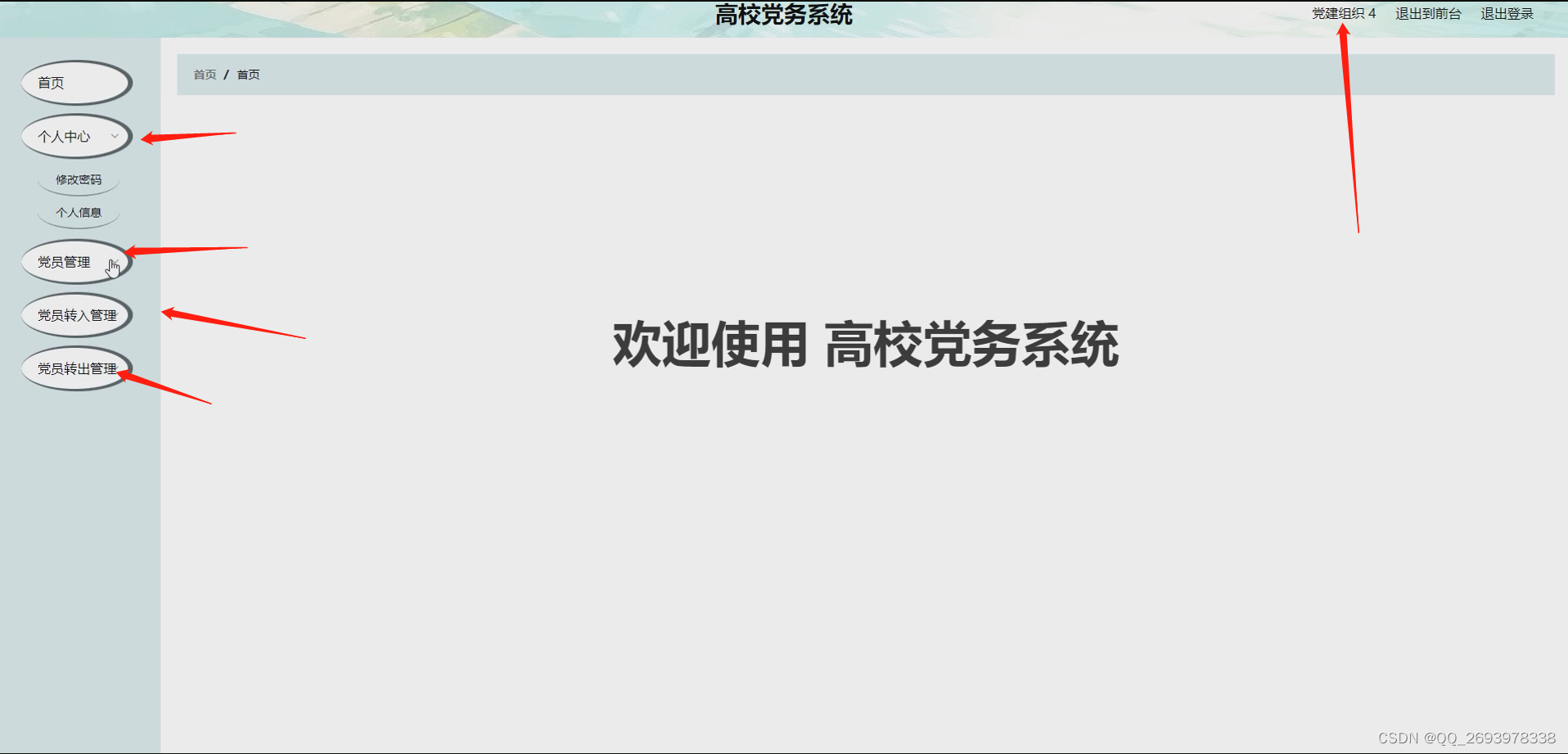
4.党员功能页面
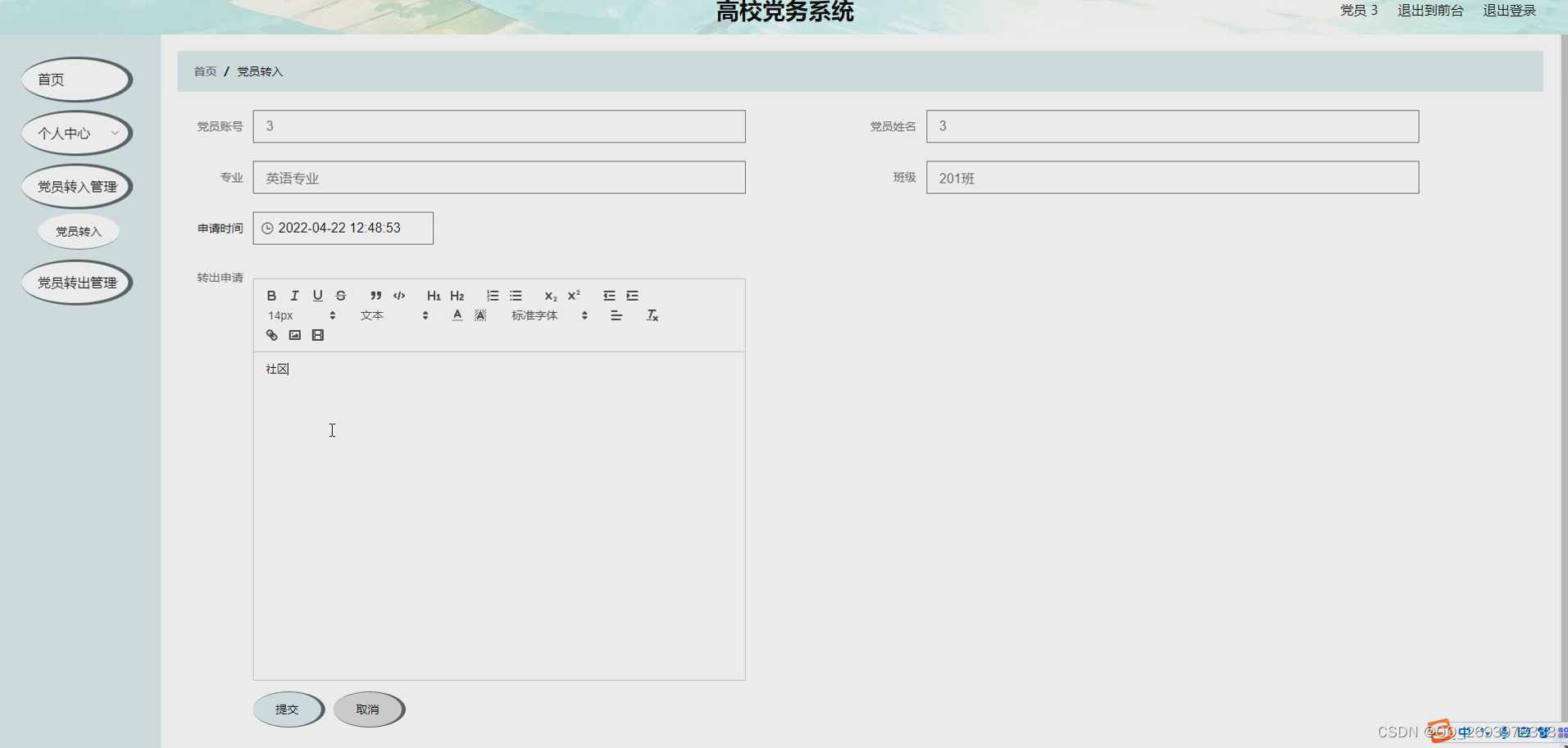
5.前台功能页面
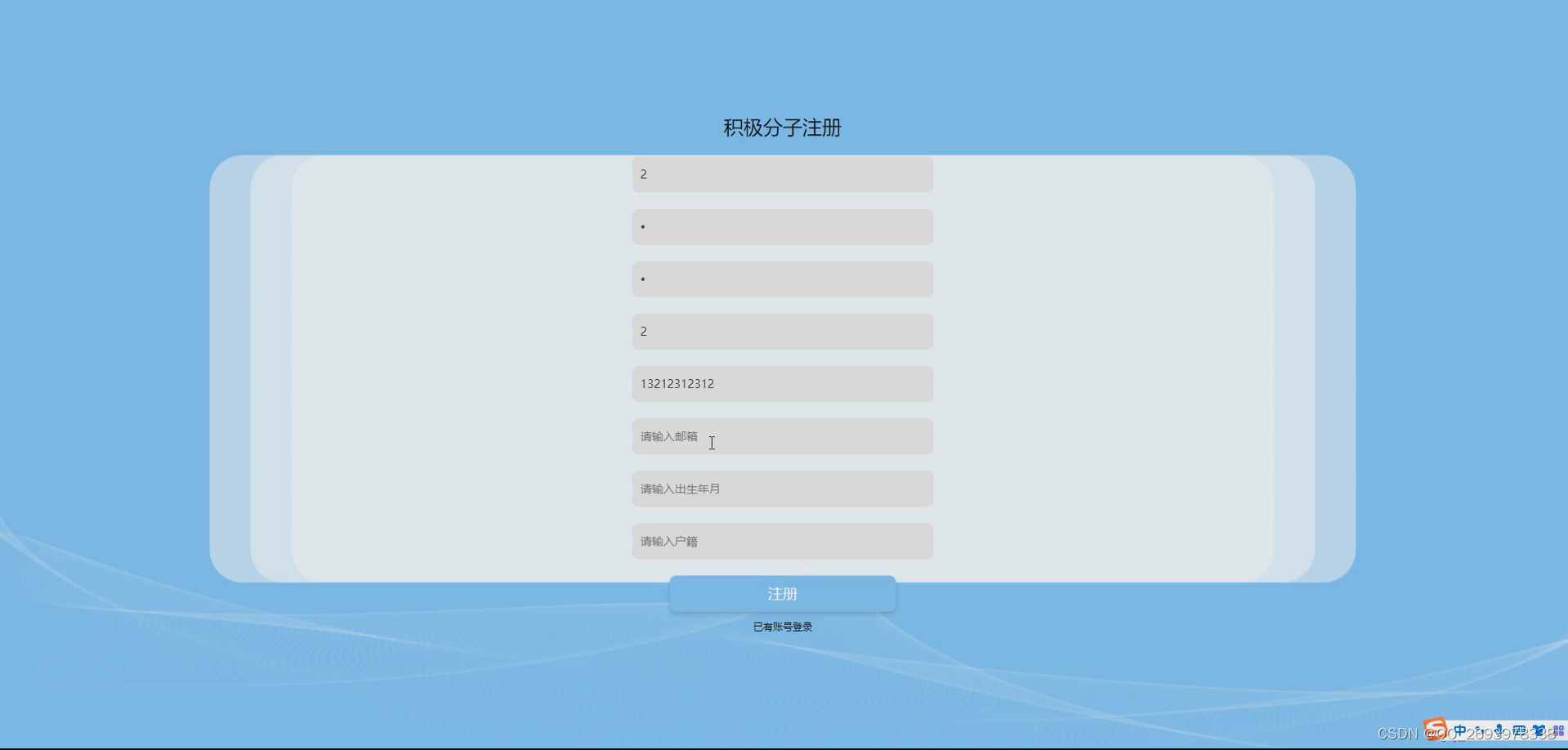
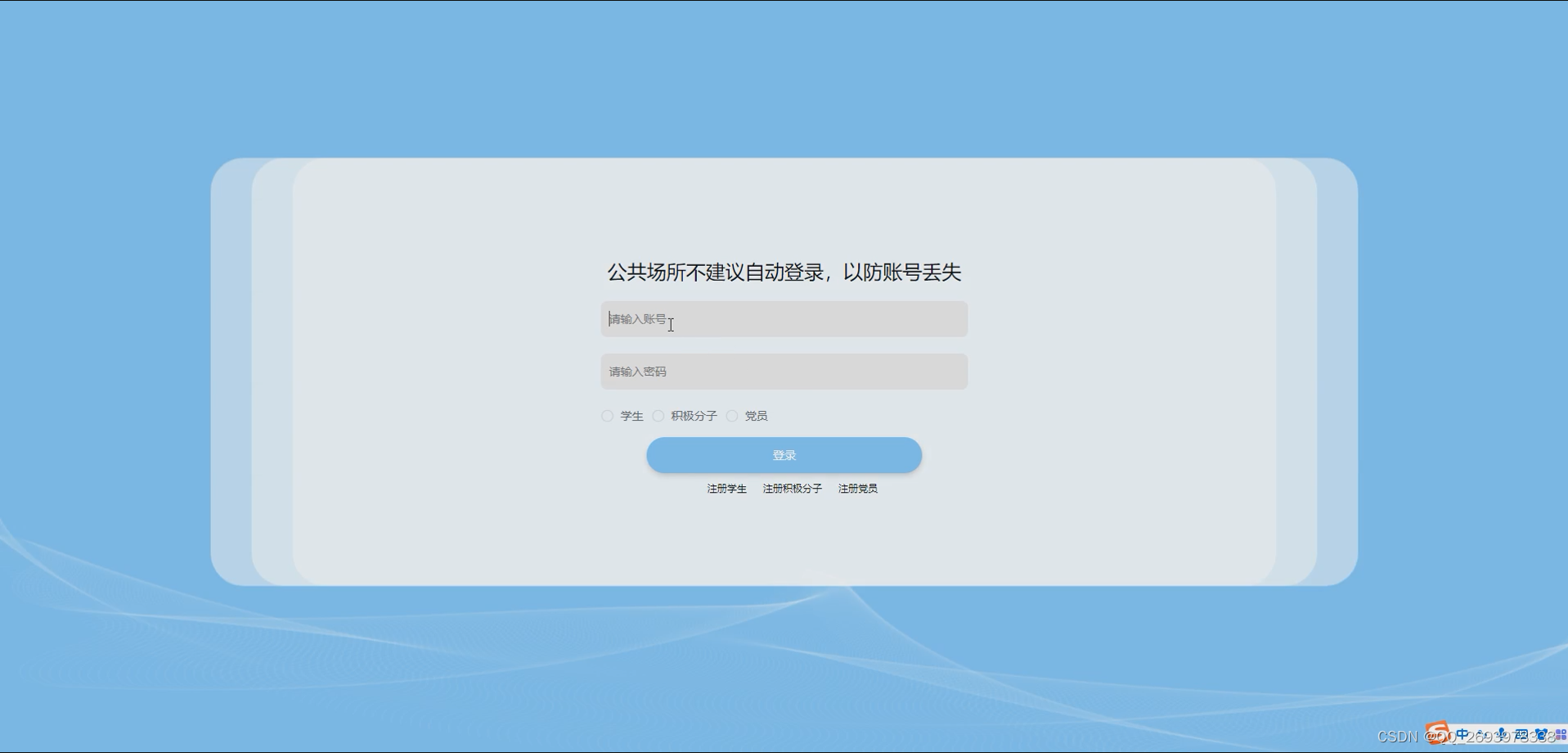
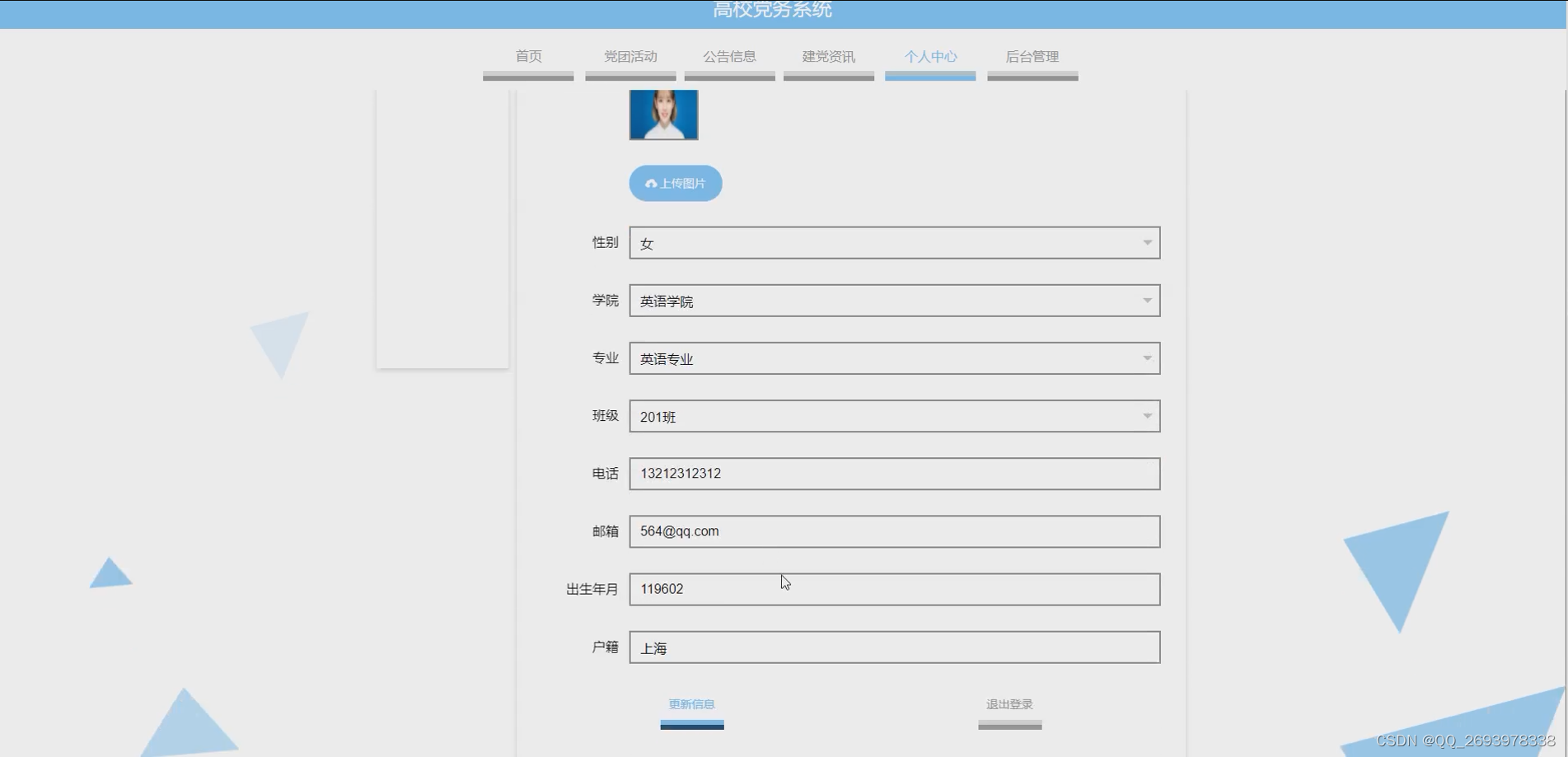
四、核心代码
1、登录核心代码
package com.controller;
import java.util.Arrays;
import java.util.Calendar;
import java.util.Date;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.bind.annotation.RestController;
import com.annotation.IgnoreAuth;
import com.baomidou.mybatisplus.mapper.EntityWrapper;
import com.entity.TokenEntity;
import com.entity.UserEntity;
import com.service.TokenService;
import com.service.UserService;
import com.utils.CommonUtil;
import com.utils.MPUtil;
import com.utils.PageUtils;
import com.utils.R;
import com.utils.ValidatorUtils;
/**
* 登录相关
*/
@RequestMapping("users")
@RestController
public class UserController{
@Autowired
private UserService userService;
@Autowired
private TokenService tokenService;
/**
* 登录
*/
@IgnoreAuth
@PostMapping(value = "/login")
public R login(String username, String password, String captcha, HttpServletRequest request) {
UserEntity user = userService.selectOne(new EntityWrapper<UserEntity>().eq("username", username));
if(user==null || !user.getPassword().equals(password)) {
return R.error("账号或密码不正确");
}
String token = tokenService.generateToken(user.getId(),username, "users", user.getRole());
return R.ok().put("token", token);
}
/**
* 注册
*/
@IgnoreAuth
@PostMapping(value = "/register")
public R register(@RequestBody UserEntity user){
// ValidatorUtils.validateEntity(user);
if(userService.selectOne(new EntityWrapper<UserEntity>().eq("username", user.getUsername())) !=null) {
return R.error("用户已存在");
}
userService.insert(user);
return R.ok();
}
/**
* 退出
*/
@GetMapping(value = "logout")
public R logout(HttpServletRequest request) {
request.getSession().invalidate();
return R.ok("退出成功");
}
/**
* 密码重置
*/
@IgnoreAuth
@RequestMapping(value = "/resetPass")
public R resetPass(String username, HttpServletRequest request){
UserEntity user = userService.selectOne(new EntityWrapper<UserEntity>().eq("username", username));
if(user==null) {
return R.error("账号不存在");
}
user.setPassword("123456");
userService.update(user,null);
return R.ok("密码已重置为:123456");
}
/**
* 列表
*/
@RequestMapping("/page")
public R page(@RequestParam Map<String, Object> params,UserEntity user){
EntityWrapper<UserEntity> ew = new EntityWrapper<UserEntity>();
PageUtils page = userService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.allLike(ew, user), params), params));
return R.ok().put("data", page);
}
/**
* 列表
*/
@RequestMapping("/list")
public R list( UserEntity user){
EntityWrapper<UserEntity> ew = new EntityWrapper<UserEntity>();
ew.allEq(MPUtil.allEQMapPre( user, "user"));
return R.ok().put("data", userService.selectListView(ew));
}
/**
* 信息
*/
@RequestMapping("/info/{id}")
public R info(@PathVariable("id") String id){
UserEntity user = userService.selectById(id);
return R.ok().put("data", user);
}
/**
* 获取用户的session用户信息
*/
@RequestMapping("/session")
public R getCurrUser(HttpServletRequest request){
Long id = (Long)request.getSession().getAttribute("userId");
UserEntity user = userService.selectById(id);
return R.ok().put("data", user);
}
/**
* 保存
*/
@PostMapping("/save")
public R save(@RequestBody UserEntity user){
// ValidatorUtils.validateEntity(user);
if(userService.selectOne(new EntityWrapper<UserEntity>().eq("username", user.getUsername())) !=null) {
return R.error("用户已存在");
}
userService.insert(user);
return R.ok();
}
/**
* 修改
*/
@RequestMapping("/update")
public R update(@RequestBody UserEntity user){
// ValidatorUtils.validateEntity(user);
UserEntity u = userService.selectOne(new EntityWrapper<UserEntity>().eq("username", user.getUsername()));
if(u!=null && u.getId()!=user.getId() && u.getUsername().equals(user.getUsername())) {
return R.error("用户名已存在。");
}
userService.updateById(user);//全部更新
return R.ok();
}
/**
* 删除
*/
@RequestMapping("/delete")
public R delete(@RequestBody Long[] ids){
userService.deleteBatchIds(Arrays.asList(ids));
return R.ok();
}
}
2、文件上传
package com.controller;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.util.Arrays;
import java.util.Date;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Random;
import java.util.UUID;
import org.apache.commons.io.FileUtils;
import org.apache.commons.lang3.StringUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpStatus;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.util.ResourceUtils;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.multipart.MultipartFile;
import com.annotation.IgnoreAuth;
import com.baomidou.mybatisplus.mapper.EntityWrapper;
import com.entity.ConfigEntity;
import com.entity.EIException;
import com.service.ConfigService;
import com.utils.R;
/**
* 上传文件映射表
*/
@RestController
@RequestMapping("file")
@SuppressWarnings({"unchecked","rawtypes"})
public class FileController{
@Autowired
private ConfigService configService;
/**
* 上传文件
*/
@RequestMapping("/upload")
public R upload(@RequestParam("file") MultipartFile file,String type) throws Exception {
if (file.isEmpty()) {
throw new EIException("上传文件不能为空");
}
String fileExt = file.getOriginalFilename().substring(file.getOriginalFilename().lastIndexOf(".")+1);
File path = new File(ResourceUtils.getURL("classpath:static").getPath());
if(!path.exists()) {
path = new File("");
}
File upload = new File(path.getAbsolutePath(),"/upload/");
if(!upload.exists()) {
upload.mkdirs();
}
String fileName = new Date().getTime()+"."+fileExt;
File dest = new File(upload.getAbsolutePath()+"/"+fileName);
file.transferTo(dest);
/**
* 如果使用idea或者eclipse重启项目,发现之前上传的图片或者文件丢失,将下面一行代码注释打开
* 请将以下的"D:\\springbootq33sd\\src\\main\\resources\\static\\upload"替换成你本地项目的upload路径,
* 并且项目路径不能存在中文、空格等特殊字符
*/
// FileUtils.copyFile(dest, new File("D:\\springbootq33sd\\src\\main\\resources\\static\\upload"+"/"+fileName)); /**修改了路径以后请将该行最前面的//注释去掉**/
if(StringUtils.isNotBlank(type) && type.equals("1")) {
ConfigEntity configEntity = configService.selectOne(new EntityWrapper<ConfigEntity>().eq("name", "faceFile"));
if(configEntity==null) {
configEntity = new ConfigEntity();
configEntity.setName("faceFile");
configEntity.setValue(fileName);
} else {
configEntity.setValue(fileName);
}
configService.insertOrUpdate(configEntity);
}
return R.ok().put("file", fileName);
}
/**
* 下载文件
*/
@IgnoreAuth
@RequestMapping("/download")
public ResponseEntity<byte[]> download(@RequestParam String fileName) {
try {
File path = new File(ResourceUtils.getURL("classpath:static").getPath());
if(!path.exists()) {
path = new File("");
}
File upload = new File(path.getAbsolutePath(),"/upload/");
if(!upload.exists()) {
upload.mkdirs();
}
File file = new File(upload.getAbsolutePath()+"/"+fileName);
if(file.exists()){
/*if(!fileService.canRead(file, SessionManager.getSessionUser())){
getResponse().sendError(403);
}*/
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_OCTET_STREAM);
headers.setContentDispositionFormData("attachment", fileName);
return new ResponseEntity<byte[]>(FileUtils.readFileToByteArray(file),headers, HttpStatus.CREATED);
}
} catch (IOException e) {
e.printStackTrace();
}
return new ResponseEntity<byte[]>(HttpStatus.INTERNAL_SERVER_ERROR);
}
}
3、token验证
package com.annotation;
import java.lang.annotation.*;
/**
* 忽略Token验证
*/
@Target(ElementType.METHOD)
@Retention(RetentionPolicy.RUNTIME)
@Documented
public @interface IgnoreAuth {
}