新建Spring boot项目
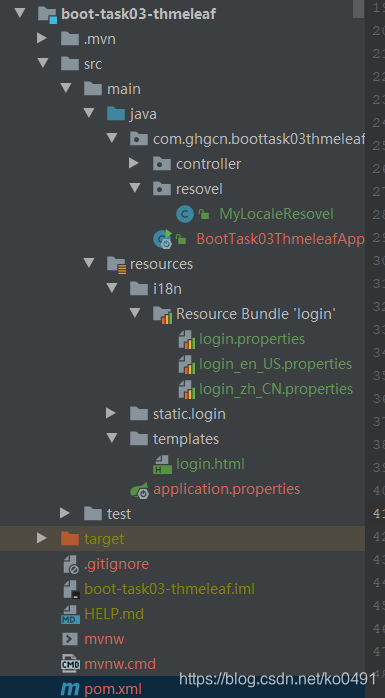
pom.xml文件
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.ghgcn</groupId>
<artifactId>boot-task03-thmeleaf</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>boot-task03-thmeleaf</name>
<description>Demo project for Spring Boot</description>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<maven.compiler.source>1.8</maven.compiler.source>
<maven.compiler.target>1.8</maven.compiler.target>
</properties>
<!--不使用spring-boot-starter-parent-->
<dependencyManagement>
<dependencies>
<dependency>
<!-- Import dependency management from Spring Boot -->
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-dependencies</artifactId>
<version>2.2.8.RELEASE</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
<exclusions>
<exclusion>
<groupId>org.junit.vintage</groupId>
<artifactId>junit-vintage-engine</artifactId>
</exclusion>
</exclusions>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
Controller与启动类
@Controller
public class LoginController {
@RequestMapping("/toLoginPage")
public String toLoginPage(Model model){
model.addAttribute("currentYear", Calendar.getInstance().get(Calendar.YEAR));
return "login";
}
}
@SpringBootApplication
public class BootTask03ThmeleafApplication {
public static void main(String[] args) {
SpringApplication.run(BootTask03ThmeleafApplication.class, args);
}
}
自定义LocaleResolver
@Configuration
public class MyLocaleResovel implements LocaleResolver {
@Override
public Locale resolveLocale(HttpServletRequest request) {
//获取语言参数 -自定义传的参数
String language = request.getParameter("language");
//Accept-Language: zh-CN,zh;q=0.9 浏览器请求头中的参数
String header = request.getHeader("Accept-Language");
Locale locale=null;
if(!StringUtils.isEmpty(language)){
String[] split = language.split("_");
locale = new Locale(split[0],split[1] );
}else {
//Accept-Language: zh-CN,zh;q=0.9
//zh-CN,zh;q=0.9
String[] splits = header.split(",");
//zh,CN,
String[] split = splits[0].split("-");
locale = new Locale(split[0],split[1] );
}
return locale;
}
@Override
public void setLocale(HttpServletRequest httpServletRequest, HttpServletResponse httpServletResponse, Locale locale) {
System.out.println(locale);
}
//用来覆盖Spring默认的
@Bean
public MyLocaleResovel localeResolver(){
return new MyLocaleResovel();
}
}
i18n文件
在resources目录下新建i18n文件夹
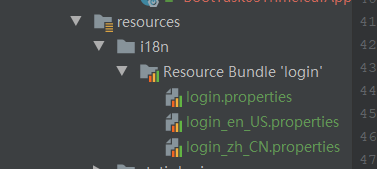
login.tip=请登录
login.username=用户名
login.password=密码
login.rememberme=记住我
login.button=登录
login.chinese=中文
login.en=English
login.tip=Please sign in
login.username=Username
login.password=password
login.rememberme=Remember me
login.button=Login
login.chinese=Chinese
login.en=English
login.tip=请登录
login.username=用户名
login.password=密码
login.rememberme=记住我
login.button=登录
login.chinese=中文
login.en=English
login.properties 是自定义的默认语言配置文件
login_en_US.properties 是英文
login_zh_CN.properties 是中文
Spring Boot默认识别的语言文件为类路径resources下的messages.properties,其他语言国际化文件的名称必须严格按照
文件名前缀名_语言代码_国家代码.properties
形式命名
上面是在resources下自定义了一个i18n文件夹下统一配置管理多语言配置文件,进行国际化文件文件基础名配置,才可以引用国际化文件
在application.properties全局配置文件中添加国际化文件基础名设置
spring.thymeleaf.cache=false
spring.thymeleaf.encoding=utf-8
spring.thymeleaf.prefix=classpath:/templates/
spring.thymeleaf.mode=HTML
spring.thymeleaf.enabled=true
spring.thymeleaf.suffix=.html
server.port=8099
server.servlet.context-path=/
spring.messages.encoding=UTF-8
spring.messages.basename=i18n.login
建立html页面
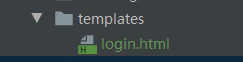
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1,shrink-to-fit=no">
<title>用户登录界面</title>
<link th:href="@{/login/css/bootstrap.min.css}" rel="stylesheet">
<link th:href="@{/login/css/signin.css}" rel="stylesheet">
</head>
<body class="text-center">
<!-- 用户登录form表单 -->
<form class="form-signin">
<!-- <img class="mb-4" th:src="@{/login/img/login.jpg}" width="72" height="72">-->
<h1 class="h3 mb-3 font-weight-normal" th:text="#{login.tip}" >请登录</h1>
<input type="text" class="form-control"
th:placeholder="#{login.username}" required="" autofocus="">
<input type="password" class="form-control"
th:placeholder="#{login.password}" required="">
<div class="checkbox mb-3">
<label>
<input type="checkbox" value="remember-me"> [[#{login.rememberme}]]
</label>
</div>
<button class="btn btn-lg btn-primary btn-block" type="submit" th:text="#{login.button}">登录</button>
<p class="mt-5 mb-3 text-muted">© <span th:text="${currentYear}">2019</span>-<span th:text="${currentYear}+1">2020</span></p>
<a class="btn btn-sm" th:href="@{/toLoginPage(language='zh_CN')}" th:text="#{login.chinese}">中文</a>
<a class="btn btn-sm" th:href="@{/toLoginPage(language='en_US')}" th:text="#{login.en}">English</a>
</form>
</body>
</html>
启动项目
http://localhost:8099/toLoginPage
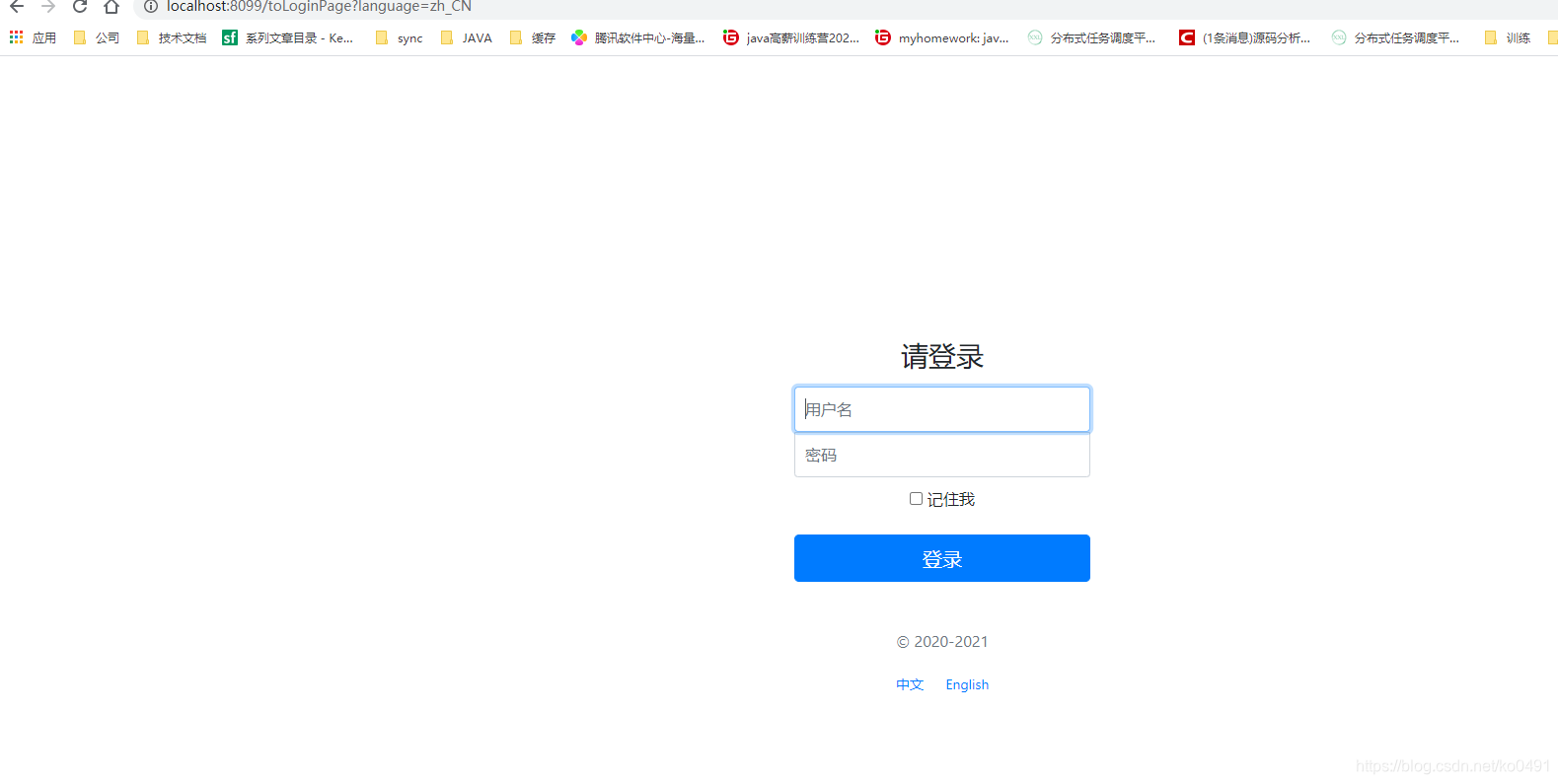
点击英文
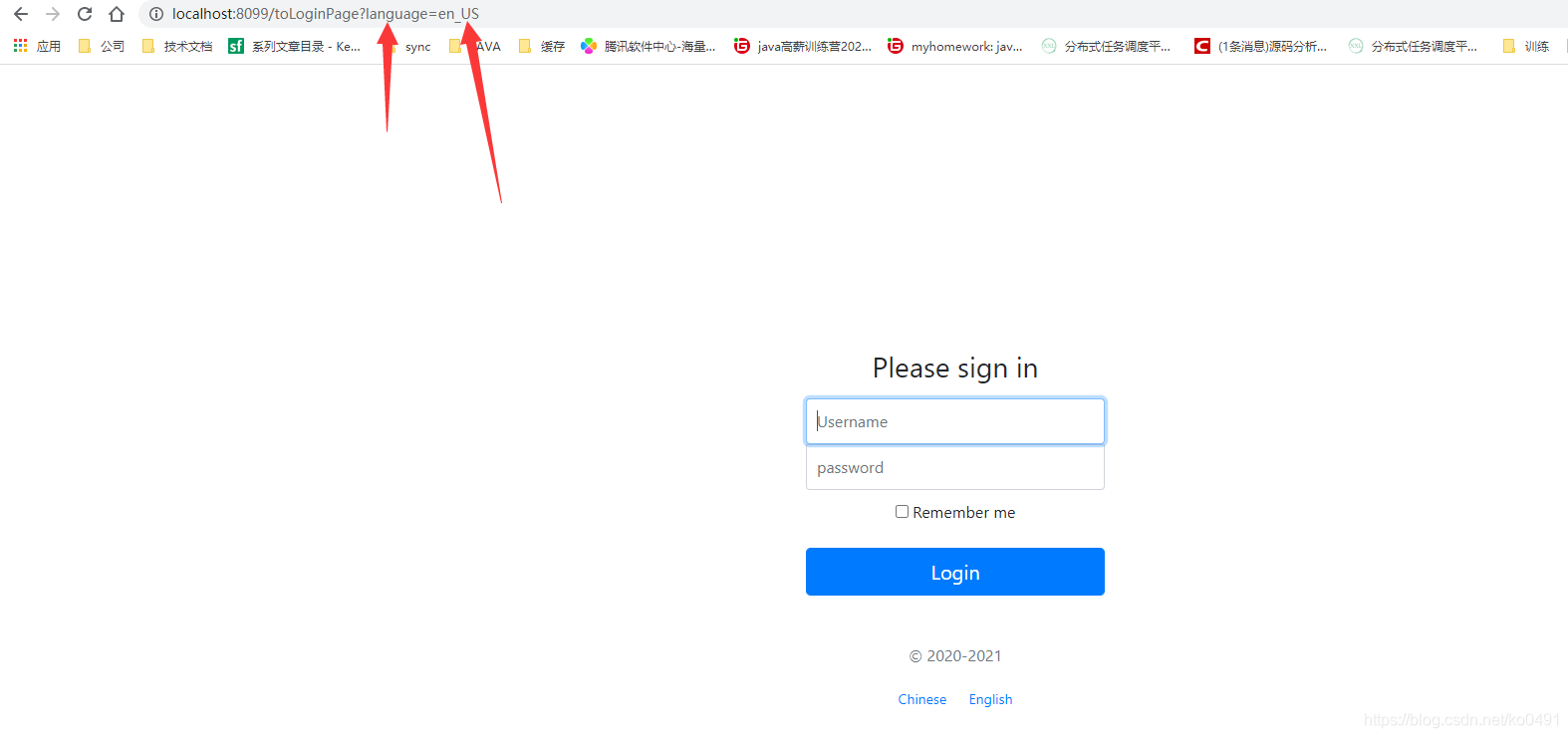
- 点中文
到这里基本完成
https://gitee.com/null_631_9084/myhomework/tree/master/stage01-springboot/boot-task03-thmeleaf