文章目录
- 一、性能分析插件
-
- 二、条件构造器
- 1、ge、gt、le、It、isNull、isNotNull
- 2、eq、ne
- 3、between、notBetween
- 4、like、noLike、likeLeft、likeRight
- 5、orderBy、orderByDesc、orderByAsc
- 6、inSql
- 7、last
- 8、指定查询的列
- 三、代码生成器
- 1、导入MP 坐标
- 2、导入数据库配置
- 3、MyBatisPlusConfig配置类
- 4、代码生成器类
一、性能分析插件
我们在平时的开发汇总,会遇到一些慢SQL。
作用:性能分析拦截器,用于输出每条SQL语句及其执行时间。
MP也提供了性能分析插件,如果超过这个时间就停止运行。
1、导入插件
@Bean
@Profile({"dev","test"})
public PerformanceInterceptor performanceInterceptor(){
PerformanceInterceptor performanceInterceptor =new PerformanceInterceptor();
performanceInterceptor.setMaxTime(100);
performanceInterceptor.setFormat(true);
return performanceInterceptor;
}
注意: 要在SprinBoot 中配置环境为 dev
或者 test
环境。
# 设置开发环境 dev、test、prod
spring.profiles.active=dev
2、 测试使用
@Test
void contextLoads() {
List<User> users = userMapper.selectList(null);
users.forEach(System.out::println);
}
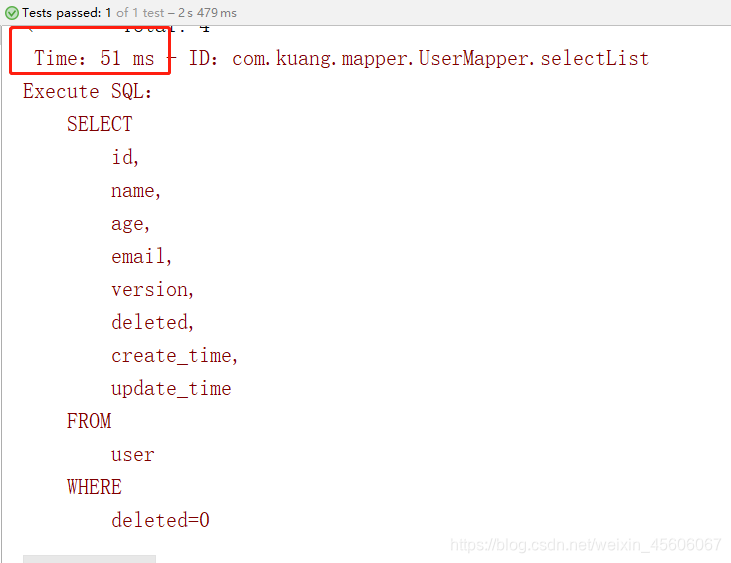
使用性能分析插件,可以帮助我们提高效率。
二、条件构造器
十分重要:Wrapper
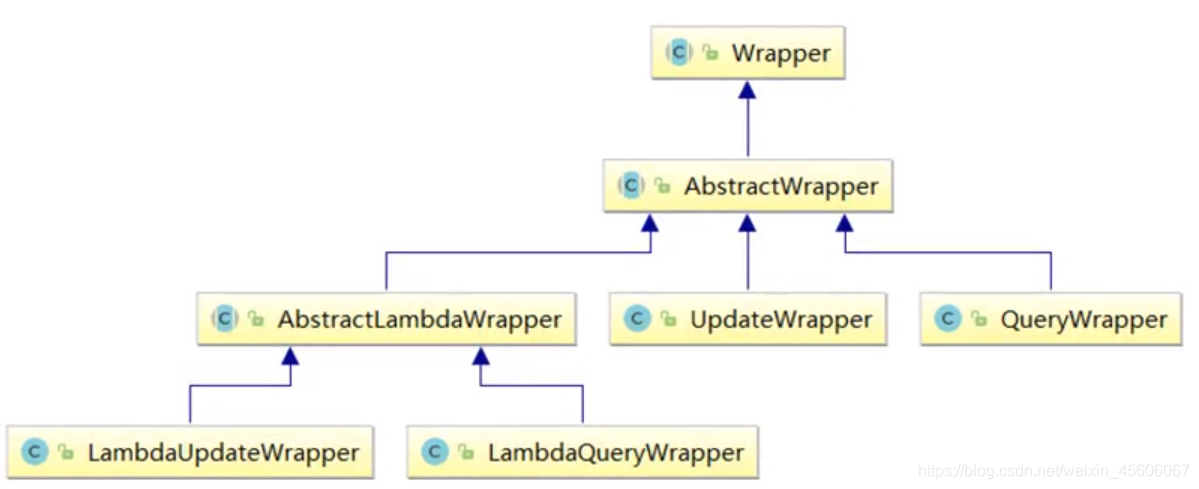
Wrapper:条件构造抽象类,最顶端父类
- AbstractWrapper:用于查询条件封装,生成sql的 where条件
- QueryWrapper:Entity对象封装操作类,不是用lambda语法
- UpdateWrapper : Update条件封装,用于Entity对象更新操作
- AbstractLambdaWrapper:Lambda语法使用Wrapper统一处理解析lambda获取column
- LambdaQueryWrapper:看名称也能明白就是用于Lambda语法使用的查询Wrapper
- LambdaUpdateWrapper:Lambda更新封装Wrapper
我们写一些复杂的SQL就可以使用它们来替代。
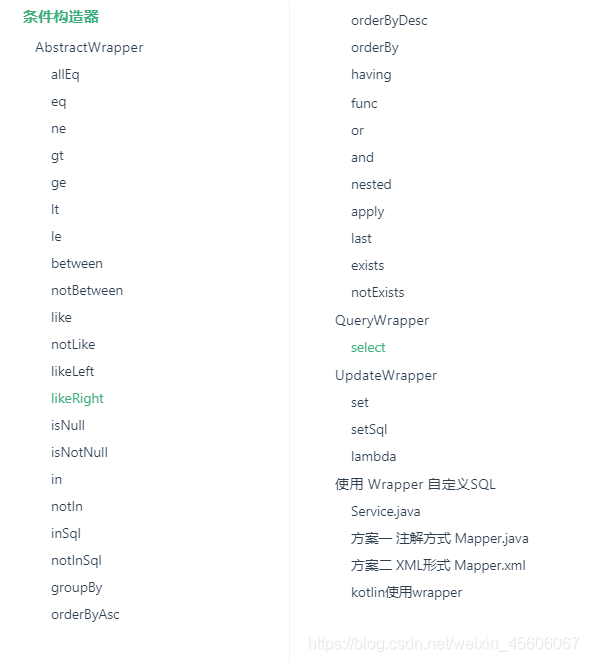
1、ge、gt、le、It、isNull、isNotNull
@SpringBootTest
public class WrapperTest {
@Autowired
private UserMapper userMapper;
@Test
void contextLoads() {
QueryWrapper<User> wrapper = new QueryWrapper<>();
wrapper
.isNotNull("name")
.isNotNull("email")
.ge("age","12");
userMapper.selectList(wrapper).forEach(System.out::println);
}
}
SQL语句:
SELECT id,name,age,email,version,deleted,create_time,update_time
FROM user
WHERE deleted=0
AND name IS NOT NULL
AND email IS NOT NULL
AND age > ‘12’
2、eq、ne
注意:selectOne返回的是一条实体记录,当出现多条记录会报错
@Test
public void test2() {
QueryWrapper<User> wrapper = new QueryWrapper<>();
wrapper.eq("name","Billie");
User user = userMapper.selectOne(wrapper);
System.out.println(user);
}
SQL语句:
SELECT id,name,age,email,version,deleted,create_time,update_time
FROM user
WHERE deleted=0
AND name = ‘Billie’
3、between、notBetween
@Test
public void test3() {
QueryWrapper<User> wrapper = new QueryWrapper<>();
wrapper.between("age",20,30);
Integer count = userMapper.selectCount(wrapper);
System.out.println(count);
}
SQL:
SELECT COUNT(1)
FROM user
WHERE deleted=0
AND age BETWEEN 20 AND 30
4、like、noLike、likeLeft、likeRight
@Test
public void test4() {
QueryWrapper<User> wrapper = new QueryWrapper<>();
wrapper
.notLike("name","e")
.likeRight("email","t");
List<Map<String, Object>> maps = userMapper.selectMaps(wrapper);
maps.forEach(System.out::println);
}
SQL语句:
SELECT id,name,age,email,version,deleted,create_time,update_time
FROM user
WHERE deleted=0
AND name NOT LIKE ‘%e%’
AND name LIKE ‘t%’
5、orderBy、orderByDesc、orderByAsc
@Test
public void test7() {
QueryWrapper<User> wrapper = new QueryWrapper<>();
wrapper.orderByDesc("id");
List<User> users = userMapper.selectList(wrapper);
users.forEach(System.out::println);
}
6、inSql
@Test
public void test6() {
QueryWrapper<User> wrapper = new QueryWrapper<>();
wrapper.inSql("id","select id from user where id <3");
List<Object> objects = userMapper.selectObjs(wrapper);
objects.forEach(System.out::println);
}
SQL语句:
SELECT id,name,age,email,version,deleted,create_time,update_time
FROM user
WHERE deleted=0
AND id IN(select id from user where id < 3)
7、last
直接拼接到sql的最后
注意:只能调用一次,多次调用以最后一次为准,有sql注入的风险,请谨慎使用
@Test
public void test7() {
QueryWrapper<User> wrapper = new QueryWrapper<>();
wrapper.last("limit 1");
List<User> users = userMapper.selectList(wrapper);
users.forEach(System.out::println);
}
SQL:
SELECT id,name,age,email,version,deleted,create_time,update_time
FROM user WHERE deleted 0 limit 1
8、指定查询的列
@Test
public void test7() {
QueryWrapper<User> wrapper = new QueryWrapper<>();
wrapper.select("id","name","age");
List<User> users = userMapper.selectList(wrapper);
users.forEach(System.out::println);
}
SQL:
SELECT id,name,age
FROM user
WHERE deleted=0
三、代码生成器
dao、pojo、service、controller都给我们自己编写完成。
AutoGenerator 是 MyBatis-Plus 的代码生成器,通过 AutoGenerator 可以快速生成 Entity、Mapper、Mapper XML、Service、Controller 等各个模块的代码,极大的提升了开发效率。
1、导入MP 坐标
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.0.5</version>
</dependency>
2、导入数据库配置
# 服务端口
server.port=9000
# 设置开发环境
spring.profiles.active=dev
# 禁止模板缓存
spring.thymeleaf.cache=false
# mysql 5 驱动不同 com.mysql.jdbc.Driver
spring.datasource.username=root
spring.datasource.password=123456
spring.datasource.url=jdbc:mysql://localhost:3306/starSea99_community?useSSL=false&useUnicode=true&characterEncoding=utf-8
spring.datasource.driver-class-name=com.mysql.jdbc.Driver
# mysql 8 驱动不同 com.mysql.cj.jdbc.Driver,需要增加时区的配置 serverTimezone=GMT%2B8
# 配置日志
mybatis-plus.configuration.log-impl=org.apache.ibatis.logging.stdout.StdOutImpl
# 配置逻辑删除
mybatis-plus.global-config.db-config.logic-delete-value=1
mybatis-plus.global-config.db-config.logic-not-delete-value=0
3、MyBatisPlusConfig配置类
@MapperScan("com.kuang.mapper")
@EnableTransactionManagement
@Configuration
public class MyBatisPlusConfig {
@Bean
public OptimisticLockerInterceptor optimisticLockerInterceptor() {
return new OptimisticLockerInterceptor();
}
@Bean
public PaginationInterceptor paginationInterceptor(){
return new PaginationInterceptor();
}
@Bean
public ISqlInjector sqlInjector(){
return new LogicSqlInjector();
}
@Bean
@Profile({"dev","test"})
public PerformanceInterceptor performanceInterceptor(){
PerformanceInterceptor performanceInterceptor =new PerformanceInterceptor();
performanceInterceptor.setMaxTime(1000);
performanceInterceptor.setFormat(true);
return performanceInterceptor;
}
}
4、代码生成器类
public class KuangCode {
public static void main(String[] args) {
AutoGenerator mpg = new AutoGenerator();
GlobalConfig gc = new GlobalConfig();
String projectPath = System.getProperty("user.dir");
gc.setOutputDir(projectPath + "/src/main/java");
gc.setAuthor("StarSea99");
gc.setOpen(false);
gc.setFileOverride(false);
gc.setServiceName("%sService");
gc.setIdType(IdType.ID_WORKER);
gc.setDateType(DateType.ONLY_DATE);
gc.setSwagger2(true);
mpg.setGlobalConfig(gc);
DataSourceConfig dsc = new DataSourceConfig();
dsc.setUrl("jdbc:mysql://localhost:3306/mybatis_plus?useSSL=false&useUnicode=true&characterEncoding=utf-8");
dsc.setDriverName("com.mysql.jdbc.Driver");
dsc.setUsername("root");
dsc.setPassword("123456");
dsc.setDbType(DbType.MYSQL);
mpg.setDataSource(dsc);
PackageConfig pc = new PackageConfig();
pc.setModuleName("blog");
pc.setParent("com.kuang");
pc.setEntity("entity");
pc.setMapper("mapper");
pc.setService("service");
pc.setController("controller");
mpg.setPackageInfo(pc);
StrategyConfig strategy = new StrategyConfig();
strategy.setInclude("user");
strategy.setNaming(NamingStrategy.underline_to_camel);
strategy.setColumnNaming(NamingStrategy.underline_to_camel);
strategy.setEntityLombokModel(true);
strategy.setLogicDeleteFieldName("deleted");
TableFill gmtCreate = new TableFill("gmt_create", FieldFill.INSERT);
TableFill gmtModified = new TableFill("gmt_modified", FieldFill.INSERT_UPDATE);
ArrayList<TableFill> tableFills = new ArrayList<>();
tableFills.add(gmtCreate);
tableFills.add(gmtModified);
strategy.setTableFillList(tableFills);
strategy.setVersionFieldName("version");
strategy.setRestControllerStyle(true);
strategy.setControllerMappingHyphenStyle(true);
mpg.setStrategy(strategy);
mpg.execute();
}
}
部分效果图
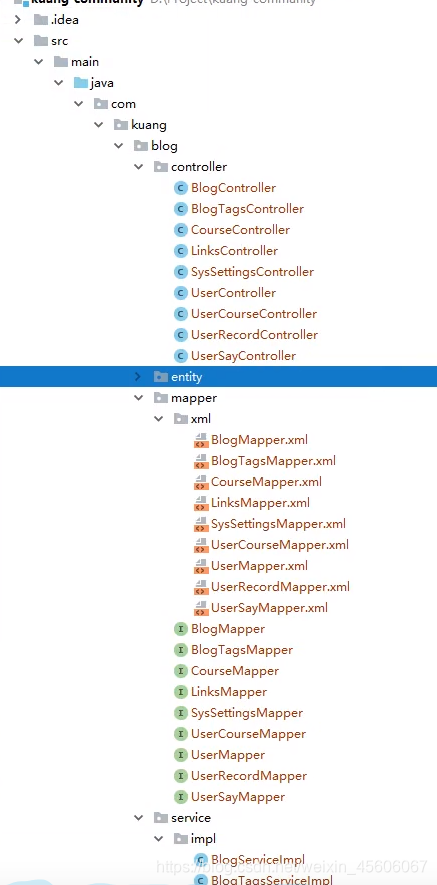
如果有收获!!! 希望老铁们来个三连,点赞、收藏、转发。
创作不易,别忘点个赞,可以让更多的人看到这篇文章,顺便鼓励我写出更好的博客
本文内容由网友自发贡献,版权归原作者所有,本站不承担相应法律责任。如您发现有涉嫌抄袭侵权的内容,请联系:hwhale#tublm.com(使用前将#替换为@)