Spring和Mybatis整合
在mybatis中,操作数据库需要获取到SQLSession对象,而该对象的实例过程在mybatis是通过SQLSessionFactoryBuilder读取全局配置文件来实例化一个SQLSessionFactory,通过SQLSessionFactory来获取到SQLSession对象,而和spring整合过程中,可以通过单例的形式来管理SQLSessionFactory对象,mybatis-spring中提供了一个SQLSessionFactoryBean类来实例化SQLSessionFactory,SQLSessionFactory中需要必须的属性是DataSource与Mappers。
整合步骤如下
1、引入mybatis-spring
为了完成spring和mybatis的整合,由mybatis提供了一个整合jar包:mybatis-spring
<!--mybatis和spring整合依赖-->
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis-spring</artifactId>
<version>1.3.0</version>
</dependency>
完整的依赖文件如下:
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.example</groupId>
<artifactId>springTest</artifactId>
<version>1.0-SNAPSHOT</version>
<name>springTest</name>
<!-- FIXME change it to the project's website -->
<url>http://www.example.com</url>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<maven.compiler.source>1.7</maven.compiler.source>
<maven.compiler.target>1.7</maven.compiler.target>
</properties>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.11</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>5.2.8.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.2.8.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-beans</artifactId>
<version>5.2.8.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-expression</artifactId>
<version>5.2.8.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-aop</artifactId>
<version>5.2.8.RELEASE</version>
</dependency>
<dependency>
<groupId>org.aspectj</groupId>
<artifactId>aspectjweaver</artifactId>
<version>1.7.4</version>
</dependency>
<dependency>
<groupId>aopalliance</groupId>
<artifactId>aopalliance</artifactId>
<version>1.0</version>
</dependency>
<dependency>
<groupId>log4j</groupId>
<artifactId>log4j</artifactId>
<version>1.2.12</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-jdbc</artifactId>
<version>5.2.8.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-tx</artifactId>
<version>5.2.8.RELEASE</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.39</version>
</dependency>
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis-spring</artifactId>
<version>1.3.0</version>
</dependency>
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
<version>3.4.1</version>
</dependency>
<dependency>
<groupId>com.mchange</groupId>
<artifactId>c3p0</artifactId>
<version>0.9.5.2</version>
</dependency>
</dependencies>
<build>
<pluginManagement><!-- lock down plugins versions to avoid using Maven defaults (may be moved to parent pom) -->
<plugins>
<!-- clean lifecycle, see https://maven.apache.org/ref/current/maven-core/lifecycles.html#clean_Lifecycle -->
<plugin>
<artifactId>maven-clean-plugin</artifactId>
<version>3.1.0</version>
</plugin>
<!-- default lifecycle, jar packaging: see https://maven.apache.org/ref/current/maven-core/default-bindings.html#Plugin_bindings_for_jar_packaging -->
<plugin>
<artifactId>maven-resources-plugin</artifactId>
<version>3.0.2</version>
</plugin>
<plugin>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.0</version>
</plugin>
<plugin>
<artifactId>maven-surefire-plugin</artifactId>
<version>2.22.1</version>
</plugin>
<plugin>
<artifactId>maven-jar-plugin</artifactId>
<version>3.0.2</version>
</plugin>
<plugin>
<artifactId>maven-install-plugin</artifactId>
<version>2.5.2</version>
</plugin>
<plugin>
<artifactId>maven-deploy-plugin</artifactId>
<version>2.8.2</version>
</plugin>
<!-- site lifecycle, see https://maven.apache.org/ref/current/maven-core/lifecycles.html#site_Lifecycle -->
<plugin>
<artifactId>maven-site-plugin</artifactId>
<version>3.7.1</version>
</plugin>
<plugin>
<artifactId>maven-project-info-reports-plugin</artifactId>
<version>3.0.0</version>
</plugin>
</plugins>
</pluginManagement>
</build>
</project>
2.开发过程
mybatis全局配置文件(可以忽略)、Pojo类、Mapper.java接口文件和Mapper.xml配置文件
StudentMapper.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="org.example.dao.StudentMapper">
<select id="selectStudentById" parameterType="int" resultType="org.example.bean.Student1">
select * from Student where SID = #{SID}
</select>
</mapper>
Student1
package org.example.bean;
/**
* Description :
* Created by Resumebb
* Date :2021/3/26
*/
public class Student1 {
/**
* 和数据库中的Student表对应
*/
private Integer SID;
private String Sname;
private String Ssex;
private Integer Sage;
//省略的getter和setter方法
public Integer getSID() {
return SID;
}
public void setSID(Integer SID) {
this.SID = SID;
}
public String getSname() {
return Sname;
}
public void setSname(String sname) {
Sname = sname;
}
public String getSsex() {
return Ssex;
}
public void setSsex(String ssex) {
Ssex = ssex;
}
public Integer getSage() {
return Sage;
}
public void setSage(Integer sage) {
Sage = sage;
}
@Override
public String toString() {
return "com.pojo{" +
"SID=" + SID +
", Sname='" + Sname + '\'' +
", Ssex='" + Ssex + '\'' +
", Sage=" + Sage +
'}';
}
}
3.spring-mybatis.xml配置
根据数据库信息注入数据源,并将相应的映射文件加载至xml中。
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-3.0.xsd">
<bean id="dataSource" class="com.mchange.v2.c3p0.DriverManagerDataSource">
<property name="driverClass" value="com.mysql.jdbc.Driver"></property>
<property name="jdbcUrl" value="jdbc:mysql://localhost:3306/test"></property>
<property name="user" value="root"></property>
<property name="password" value="123456"></property>
</bean>
<bean id="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean">
<!-- 数据源配置-->
<property name="dataSource" ref="dataSource"></property>
<!-- 加载mybatis配置文件-->
<property name="configLocation" value="mybatis-config.xml"></property>
<property name="mapperLocations" value="mapper/*.xml"></property>
</bean>
<!-- 获取Studentapper的代理对象-->
<bean id="studentMapper" class="org.mybatis.spring.mapper.MapperFactoryBean">
<property name="sqlSessionFactory" ref="sqlSessionFactory"></property>
<property name="mapperInterface" value="org.example.dao.StudentMapper"></property>
</bean>
</beans>
4.测试
public class MybatisDemo {
public static void main(String[] args) {
String path = "spring-mybatis.xml";
ClassPathXmlApplicationContext classContext = new ClassPathXmlApplicationContext(path);
StudentMapper studentMapper = (StudentMapper)classContext.getBean("studentMapper");
Student1 student1 = studentMapper.selectStudentById(1);
System.out.println(student1);
}
}
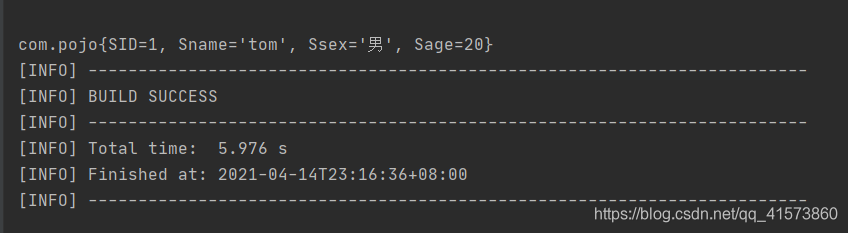
Spring中JDBCTemplate的使用
jdbcTemplate操作步骤
导入相关依赖
<!--spring支持jdbc模板操作-->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-jdbc</artifactId>
<version>5.2.8.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-tx</artifactId>
<version>5.2.8.RELEASE</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.39</version>
</dependency>
JDBCTemplate的使用
public class JDBCTemplateDemo {
public static void main(String[] args) {
//创建对象,设置数据源信息
DriverManagerDataSource driverManagerDataSource = new DriverManagerDataSource();
driverManagerDataSource.setDriverClassName("com.mysql.jdbc.Driver");
driverManagerDataSource.setUrl("jdbc:mysql://localhost:3306/test");
driverManagerDataSource.setUsername("root");
driverManagerDataSource.setPassword("123456");
//获取jdbc模板实例
JdbcTemplate jdbcTemplate = new JdbcTemplate(driverManagerDataSource);
//通过模板对象调用方法来执行相关操作
//通过jdbcTemplate提供的update方法来完成变更操作(修改、新增、删除)
//删除操作SQL
String deleteSql = "delete from Student where SID = ?";
int update = jdbcTemplate.update(deleteSql, new Object[]{22});
System.out.println(update);
//查询操作(单个对象) 使用queryForObject方法
String selectSql1 = "select * from Student where SID = ?";
Student1 student1 = jdbcTemplate.queryForObject(selectSql1, new Object[]{1}, new StudentMapper());
System.out.println(student1);
//查询操作(多个结果集对象) 使用query方法
String selectSql2 = "select * from Student";
List <Student1> student1List = jdbcTemplate.query(selectSql2, new StudentMapper());
Iterator <Student1> iterator = student1List.iterator();
while (iterator.hasNext()){
System.out.println(iterator.next());
}
}
}
JDBCTemplate解决了JDBC结果集合Java代码的耦合性问题
实现一个RowMapper接口的实现类,手动完成数据库结果集和Java对象的映射
public class StudentMapper implements RowMapper<Student1> {
@Override
/**
* jdbc模板能够完成自动映射为Java对象关键点在于实现RowMapper接口
* 实现mapRow方法
* ResultSet:数据库结果集
* int :类似数据库数据序号,从0开始
* 返回类型:指定的Java对象
* 该方法主要是完成ResultSet结果集手动映射为Java对象
*
*/
public Student1 mapRow(ResultSet resultSet, int i) throws SQLException {
Student1 student1 = new Student1();
student1.setSID(resultSet.getInt("SID"));
student1.setSage(resultSet.getString("Sage"));
student1.setSname(resultSet.getString("Sname"));
student1.setSsex(resultSet.getString("Ssex"));
return student1;
}
}
不管是单个结果集还是多个结果集都会代用到在RowMapper接口的实现类,数据库中每一条记录都会执行到mapRow方法。
本文内容由网友自发贡献,版权归原作者所有,本站不承担相应法律责任。如您发现有涉嫌抄袭侵权的内容,请联系:hwhale#tublm.com(使用前将#替换为@)