文章目录
- 树基础知识
- [104. 二叉树的最大深度](https://leetcode-cn.com/problems/maximum-depth-of-binary-tree/)
- [102. 二叉树的层序遍历](https://leetcode-cn.com/problems/binary-tree-level-order-traversal/)
- [94. 二叉树的中序遍历](https://leetcode-cn.com/problems/binary-tree-inorder-traversal/)
- [101. 对称二叉树](https://leetcode-cn.com/problems/symmetric-tree/)
- [98. 验证二叉搜索树](https://leetcode-cn.com/problems/validate-binary-search-tree/)
- [100. 相同的树](https://leetcode-cn.com/problems/same-tree/)
- [226. 翻转二叉树](https://leetcode-cn.com/problems/invert-binary-tree/)
- [144. 二叉树的前序遍历](https://leetcode-cn.com/problems/binary-tree-preorder-traversal/)
- [111. 二叉树的最小深度](https://leetcode-cn.com/problems/minimum-depth-of-binary-tree/)
- [112. 路径总和](https://leetcode-cn.com/problems/path-sum/)
- [145. 二叉树的后序遍历](https://leetcode-cn.com/problems/binary-tree-postorder-traversal/)
- [110. 平衡二叉树](https://leetcode-cn.com/problems/balanced-binary-tree/)
- [114. 二叉树展开为链表](https://leetcode-cn.com/problems/flatten-binary-tree-to-linked-list/)
树基础知识
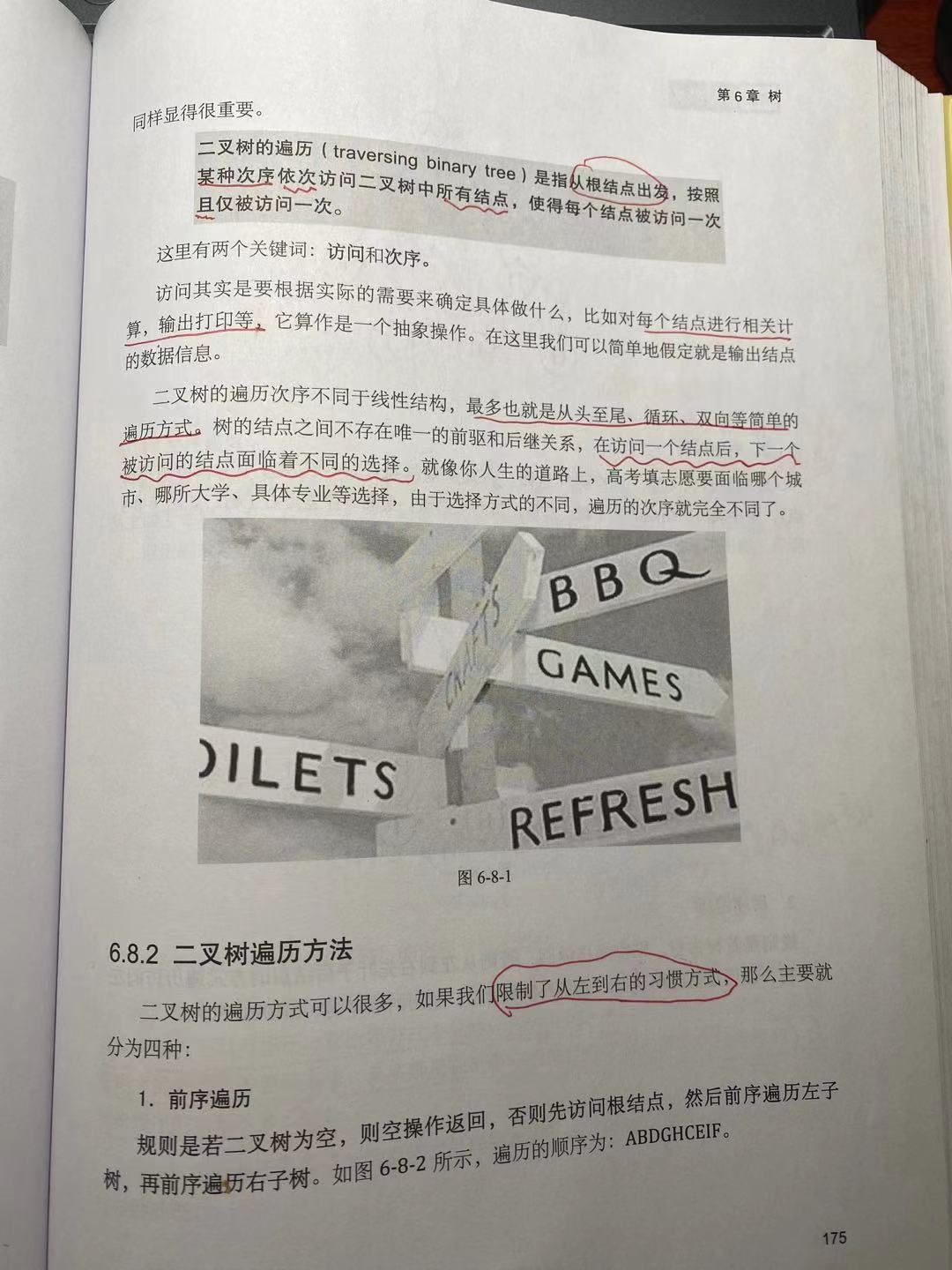

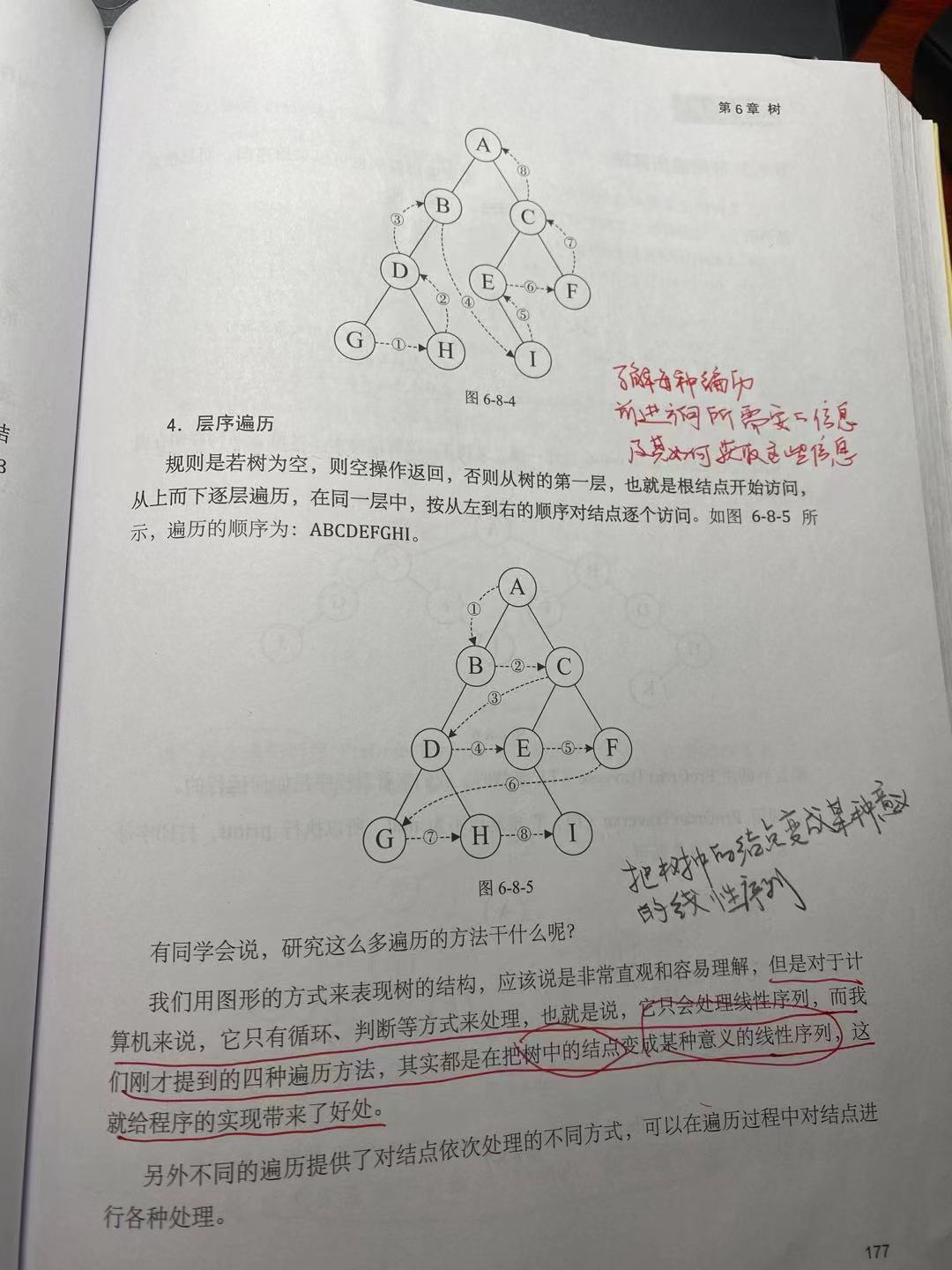
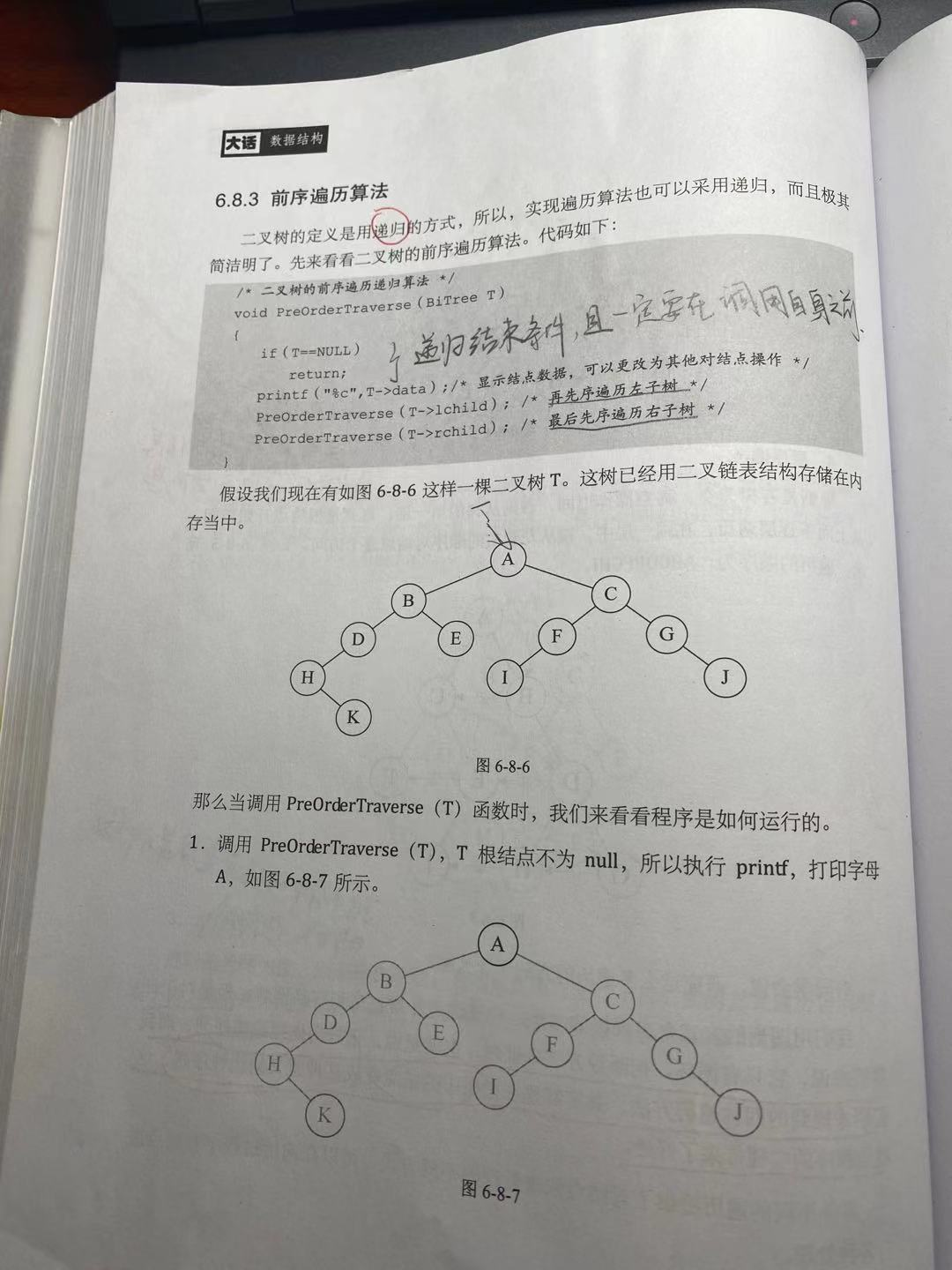
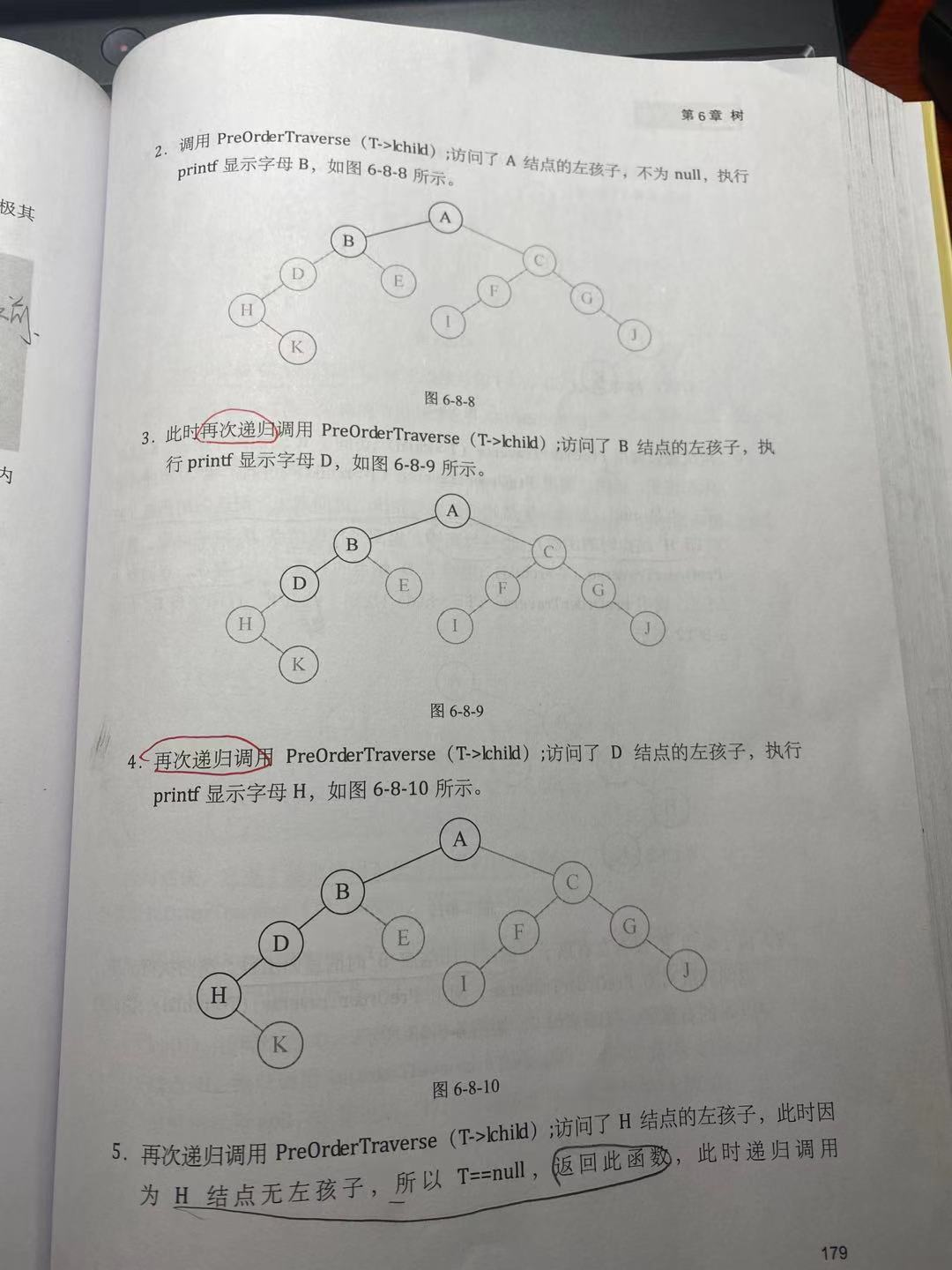

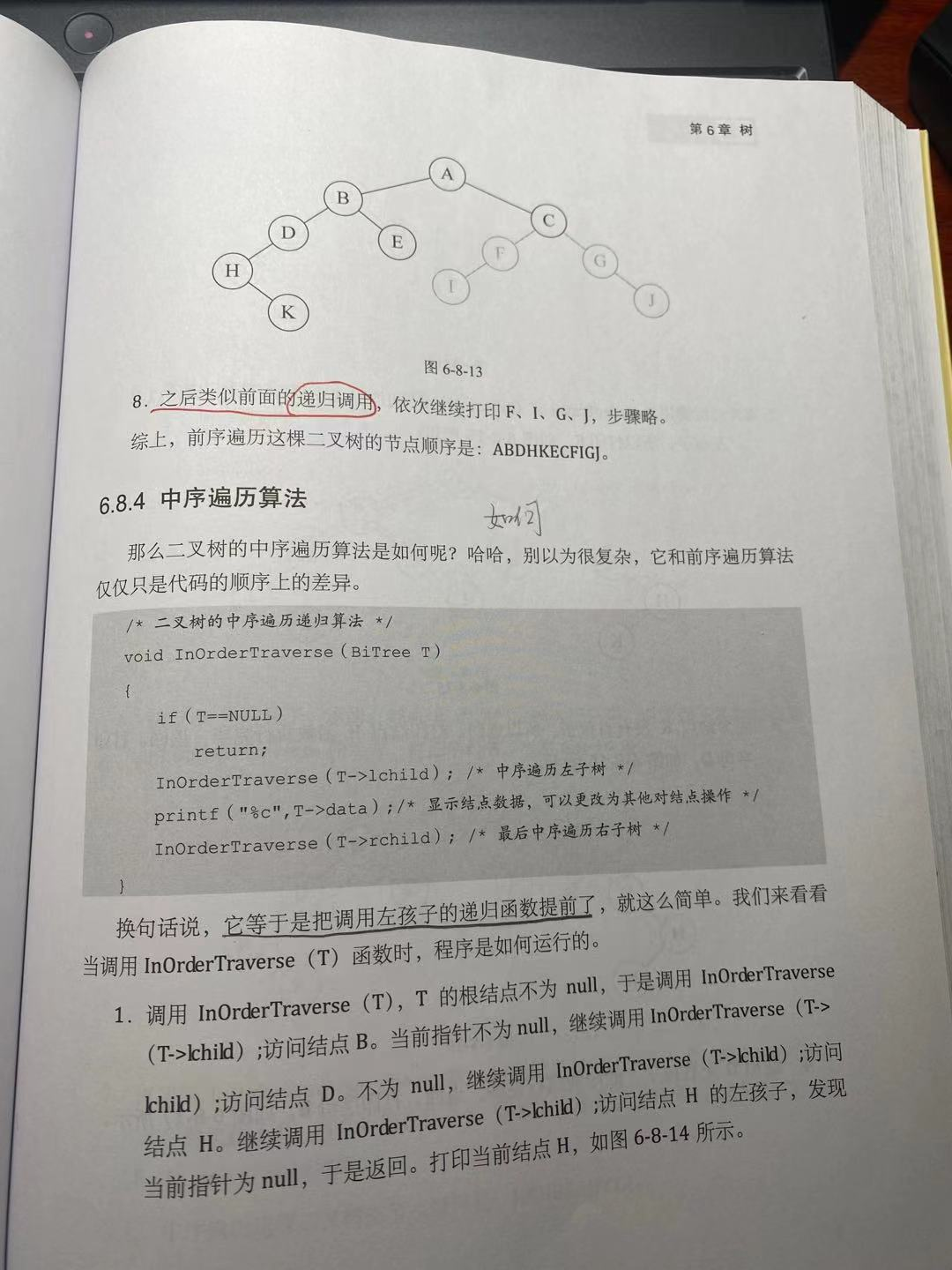
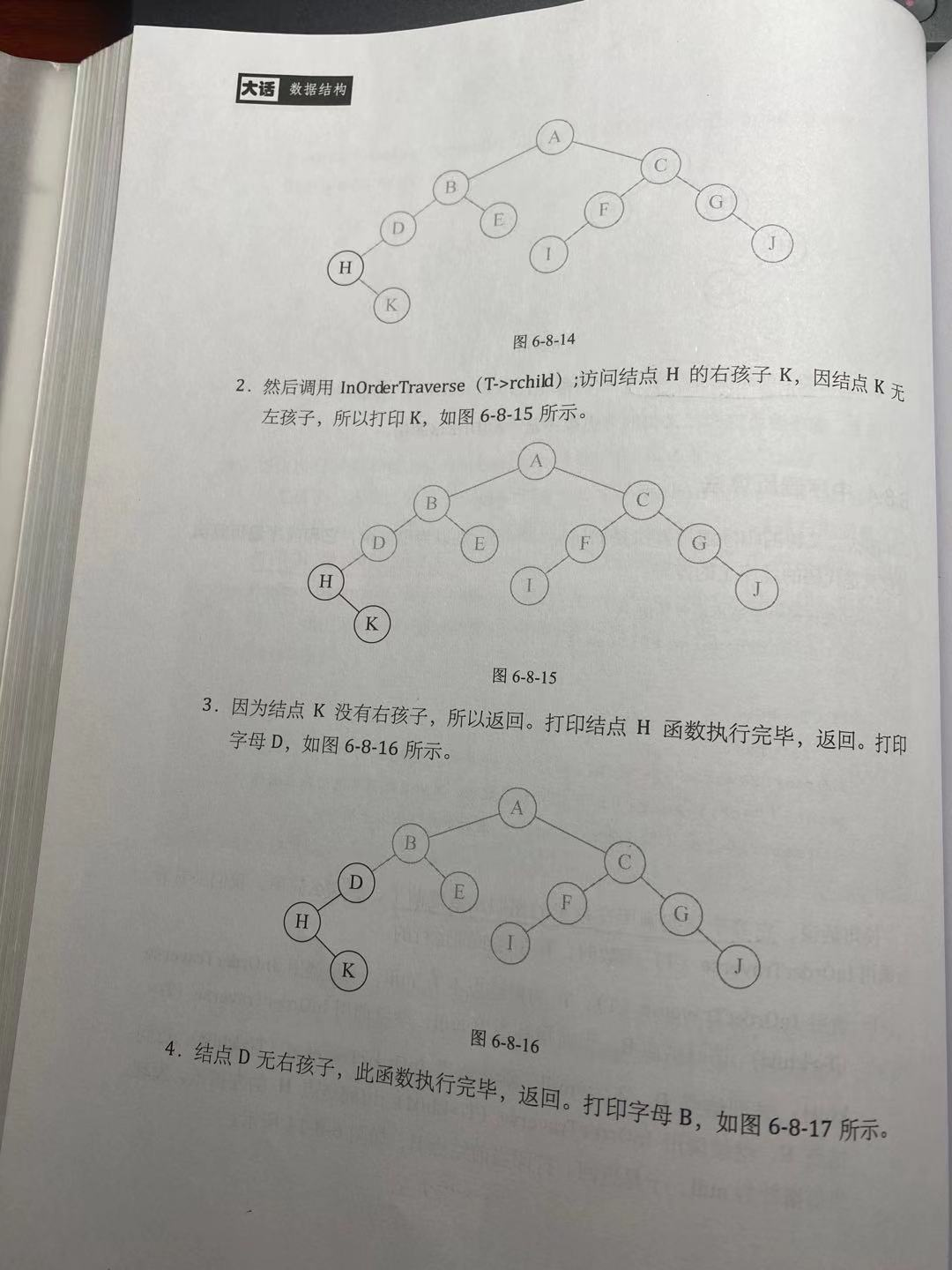
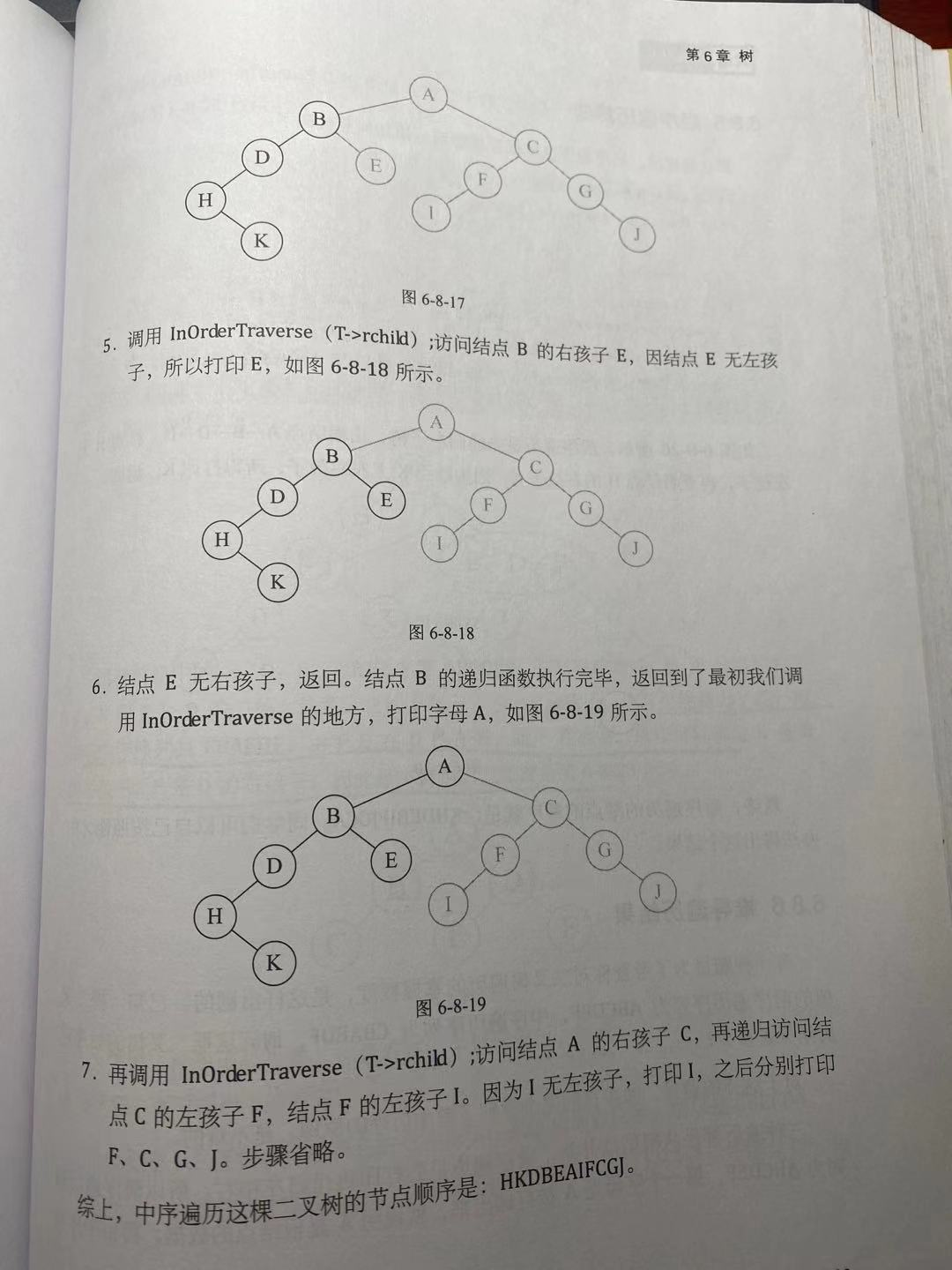
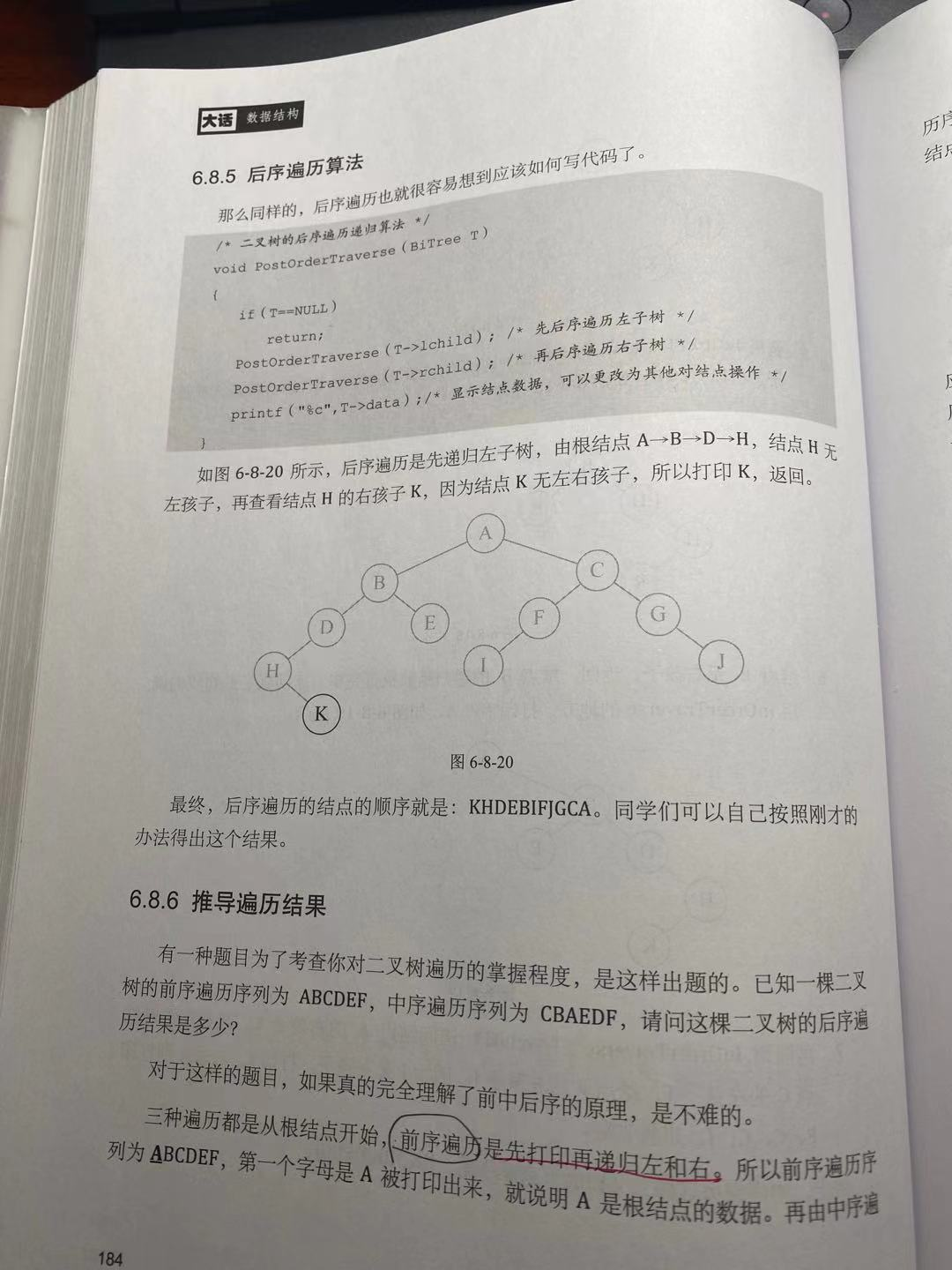
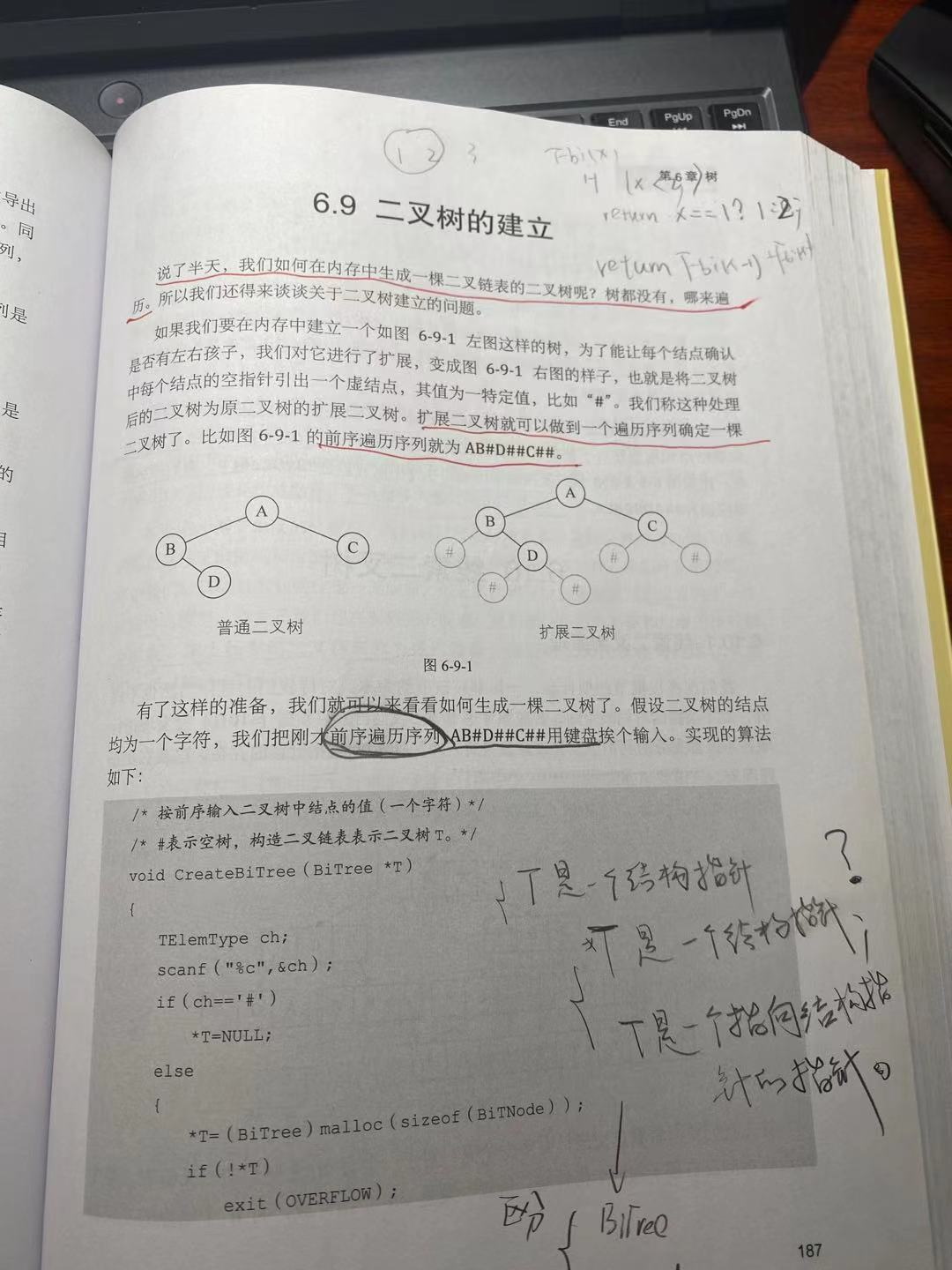

下面用图解来说明一下前序遍历算法的递归过程。
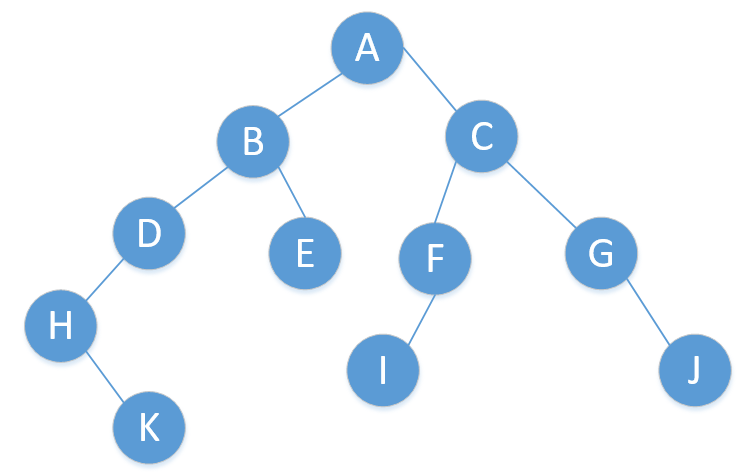
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-t649bQ1H-1631410485856)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906222707569.png#pic_center)]
)]
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-WJP8QBir-1631410165737)(
104. 二叉树的最大深度
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-hokROzkn-1631410165737)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906225439631.png)]
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-c6vkvJPE-1631410165738)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906230105322.png)]
int maxDepth(struct TreeNode *root) {
if (root == NULL) return 0;
return fmax(maxDepth(root->left), maxDepth(root->right)) + 1;
}
-------------------------------------------------------------------
class Solution {
public:
int maxDepth(TreeNode* root) {
if (root == nullptr) return 0;
return max(maxDepth(root->left), maxDepth(root->right)) + 1;
}
};
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-K6grKsYv-1631410165738)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906230159673.png)]
struct QueNode {
struct TreeNode *p;
struct QueNode *next;
};
void init(struct QueNode **p, struct TreeNode *t) {
(*p) = (struct QueNode *)malloc(sizeof(struct QueNode));
(*p)->p = t;
(*p)->next = NULL;
}
int maxDepth(struct TreeNode *root) {
if (root == NULL) return 0;
struct QueNode *left, *right;
init(&left, root);
right = left;
int ans = 0, sz = 1, tmp = 0;
while (left != NULL) {
tmp = 0;
while (sz > 0) {
if (left->p->left != NULL) {
init(&right->next, left->p->left);
right = right->next;
tmp++;
}
if (left->p->right != NULL) {
init(&right->next, left->p->right);
right = right->next;
tmp++;
}
left = left->next;
sz--;
}
sz += tmp;
ans++;
}
return ans;
}
---------------------------------------------------------------------
class Solution {
public:
int maxDepth(TreeNode* root) {
if (root == nullptr) return 0;
queue<TreeNode*> Q;
Q.push(root);
int ans = 0;
while (!Q.empty()) {
int sz = Q.size();
while (sz > 0) {
TreeNode* node = Q.front();Q.pop();
if (node->left) Q.push(node->left);
if (node->right) Q.push(node->right);
sz -= 1;
}
ans += 1;
}
return ans;
}
};
102. 二叉树的层序遍历
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-FSQWBJz6-1631410165739)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906230630256.png)]
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-hVrPvVav-1631410165739)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906230809581.png)]
class Solution {
public:
vector<vector<int>> levelOrder(TreeNode* root) {
vector <vector <int>> ret;
if (!root) {
return ret;
}
queue <TreeNode*> q;
q.push(root);
while (!q.empty()) {
int currentLevelSize = q.size();
ret.push_back(vector <int> ());
for (int i = 1; i <= currentLevelSize; ++i) {
auto node = q.front(); q.pop();
ret.back().push_back(node->val);
if (node->left) q.push(node->left);
if (node->right) q.push(node->right);
}
}
return ret;
}
};
94. 二叉树的中序遍历
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-haY1LbiS-1631410165739)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906231012868.png)]
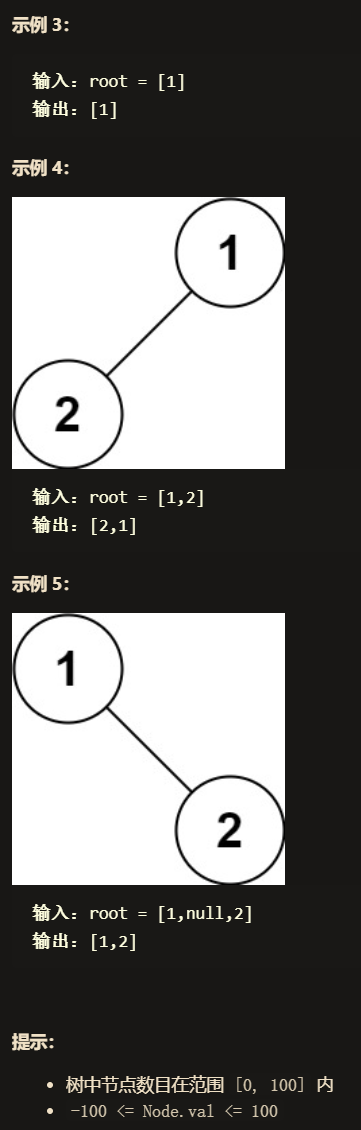
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-eKamUhz6-1631410165740)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906231108467.png)]
void inorder(struct TreeNode* root, int* res, int* resSize) {
if (!root) {
return;
}
inorder(root->left, res, resSize);
res[(*resSize)++] = root->val;
inorder(root->right, res, resSize);
}
int* inorderTraversal(struct TreeNode* root, int* returnSize) {
int* res = malloc(sizeof(int) * 501);
*returnSize = 0;
inorder(root, res, returnSize);
return res;
}
---------------------------------------------------------------------
class Solution {
public:
void inorder(TreeNode* root, vector<int>& res) {
if (!root) {
return;
}
inorder(root->left, res);
res.push_back(root->val);
inorder(root->right, res);
}
vector<int> inorderTraversal(TreeNode* root) {
vector<int> res;
inorder(root, res);
return res;
}
};
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-1ykLuVUp-1631410165741)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906231203588.png)]
int* inorderTraversal(struct TreeNode* root, int* returnSize) {
*returnSize = 0;
int* res = malloc(sizeof(int) * 501);
struct TreeNode** stk = malloc(sizeof(struct TreeNode*) * 501);
int top = 0;
while (root != NULL || top > 0) {
while (root != NULL) {
stk[top++] = root;
root = root->left;
}
root = stk[--top];
res[(*returnSize)++] = root->val;
root = root->right;
}
return res;
}
---------------------------------------------------------------
Solution {
public:
vector<int> inorderTraversal(TreeNode* root) {
vector<int> res;
stack<TreeNode*> stk;
while (root != nullptr || !stk.empty()) {
while (root != nullptr) {
stk.push(root);
root = root->left;
}
root = stk.top();
stk.pop();
res.push_back(root->val);
root = root->right;
}
return res;
}
};
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-dOsyM3uB-1631410165741)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906231316031.png)]
int* inorderTraversal(struct TreeNode* root, int* returnSize) {
int* res = malloc(sizeof(int) * 501);
*returnSize = 0;
struct TreeNode* predecessor = NULL;
while (root != NULL) {
if (root->left != NULL) {
predecessor = root->left;
while (predecessor->right != NULL && predecessor->right != root) {
predecessor = predecessor->right;
}
if (predecessor->right == NULL) {
predecessor->right = root;
root = root->left;
}
else {
res[(*returnSize)++] = root->val;
predecessor->right = NULL;
root = root->right;
}
}
else {
res[(*returnSize)++] = root->val;
root = root->right;
}
}
return res;
}
-----------------------------------------------------------------
class Solution {
public:
vector<int> inorderTraversal(TreeNode* root) {
vector<int> res;
TreeNode *predecessor = nullptr;
while (root != nullptr) {
if (root->left != nullptr) {
predecessor = root->left;
while (predecessor->right != nullptr && predecessor->right != root) {
predecessor = predecessor->right;
}
if (predecessor->right == nullptr) {
predecessor->right = root;
root = root->left;
}
else {
res.push_back(root->val);
predecessor->right = nullptr;
root = root->right;
}
}
else {
res.push_back(root->val);
root = root->right;
}
}
return res;
}
};
101. 对称二叉树
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-DDaijCXA-1631410165741)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906231723650.png)]
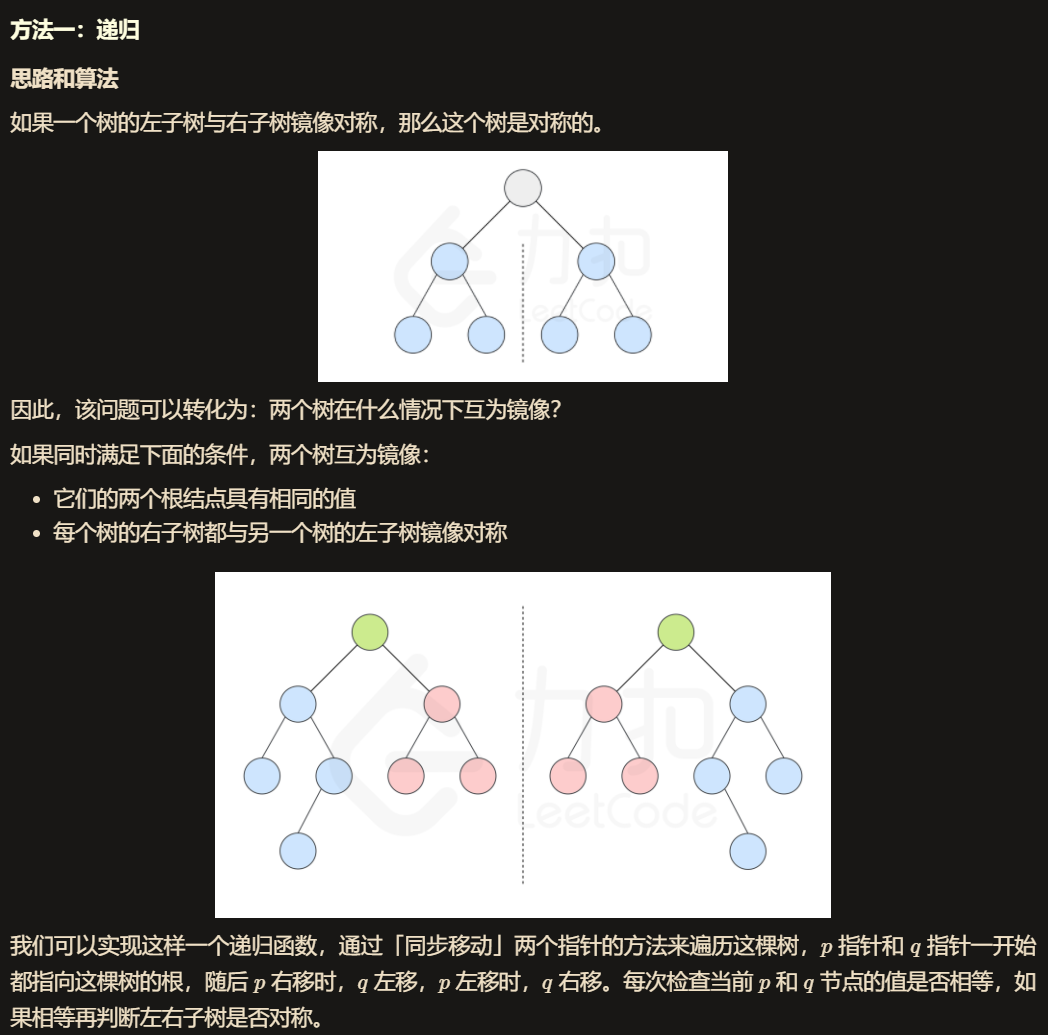
class Solution {
public:
bool check(TreeNode *p, TreeNode *q) {
if (!p && !q) return true;
if (!p || !q) return false;
return p->val == q->val && check(p->left, q->right) && check(p->right, q->left);
}
bool isSymmetric(TreeNode* root) {
return check(root, root);
}
};
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-TJOn4n2k-1631410165742)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906231838027.png)]
class Solution {
public:
bool check(TreeNode *u, TreeNode *v) {
queue <TreeNode*> q;
q.push(u); q.push(v);
while (!q.empty()) {
u = q.front(); q.pop();
v = q.front(); q.pop();
if (!u && !v) continue;
if ((!u || !v) || (u->val != v->val)) return false;
q.push(u->left);
q.push(v->right);
q.push(u->right);
q.push(v->left);
}
return true;
}
bool isSymmetric(TreeNode* root) {
return check(root, root);
}
};
98. 验证二叉搜索树
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-XEZkgoUx-1631410165742)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906232046142.png)]
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-VP8vQGV3-1631410165743)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906232733702.png)]
class Solution {
public:
bool helper(TreeNode* root, long long lower, long long upper) {
if (root == nullptr) {
return true;
}
if (root -> val <= lower || root -> val >= upper) {
return false;
}
return helper(root -> left, lower, root -> val) && helper(root -> right, root -> val, upper);
}
bool isValidBST(TreeNode* root) {
return helper(root, LONG_MIN, LONG_MAX);
}
};
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-YXztDcbC-1631410165743)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906232758657.png)]
class Solution {
public:
bool isValidBST(TreeNode* root) {
stack<TreeNode*> stack;
long long inorder = (long long)INT_MIN - 1;
while (!stack.empty() || root != nullptr) {
while (root != nullptr) {
stack.push(root);
root = root -> left;
}
root = stack.top();
stack.pop();
if (root -> val <= inorder) {
return false;
}
inorder = root -> val;
root = root -> right;
}
return true;
}
};
100. 相同的树
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-9x97yxnb-1631410165743)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906232905376.png)]
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-tpraHkvi-1631410165744)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906232942970.png)]
bool isSameTree(struct TreeNode* p, struct TreeNode* q) {
if (p == NULL && q == NULL) {
return true;
} else if (p == NULL || q == NULL) {
return false;
} else if (p->val != q->val) {
return false;
} else {
return isSameTree(p->left, q->left) && isSameTree(p->right, q->right);
}
}
class Solution {
public:
bool isSameTree(TreeNode* p, TreeNode* q) {
if (p == nullptr && q == nullptr) {
return true;
} else if (p == nullptr || q == nullptr) {
return false;
} else if (p->val != q->val) {
return false;
} else {
return isSameTree(p->left, q->left) && isSameTree(p->right, q->right);
}
}
};
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-7oaAoo5M-1631410165744)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906233029546.png)]
bool isSameTree(struct TreeNode* p, struct TreeNode* q) {
if (p == NULL && q == NULL) {
return true;
} else if (p == NULL || q == NULL) {
return false;
}
struct TreeNode** que1 = (struct TreeNode**)malloc(sizeof(struct TreeNode*));
struct TreeNode** que2 = (struct TreeNode**)malloc(sizeof(struct TreeNode*));
int queleft1 = 0, queright1 = 0;
int queleft2 = 0, queright2 = 0;
que1[queright1++] = p;
que2[queright2++] = q;
while (queleft1 < queright1 && queleft2 < queright2) {
struct TreeNode* node1 = que1[queleft1++];
struct TreeNode* node2 = que2[queleft2++];
if (node1->val != node2->val) {
return false;
}
struct TreeNode* left1 = node1->left;
struct TreeNode* right1 = node1->right;
struct TreeNode* left2 = node2->left;
struct TreeNode* right2 = node2->right;
if ((left1 == NULL) ^ (left2 == NULL)) {
return false;
}
if ((right1 == NULL) ^ (right2 == NULL)) {
return false;
}
if (left1 != NULL) {
queright1++;
que1 = realloc(que1, sizeof(struct TreeNode*) * queright1);
que1[queright1 - 1] = left1;
}
if (right1 != NULL) {
queright1++;
que1 = realloc(que1, sizeof(struct TreeNode*) * queright1);
que1[queright1 - 1] = right1;
}
if (left2 != NULL) {
queright2++;
que2 = realloc(que2, sizeof(struct TreeNode*) * queright2);
que2[queright2 - 1] = left2;
}
if (right2 != NULL) {
queright2++;
que2 = realloc(que2, sizeof(struct TreeNode*) * queright2);
que2[queright2 - 1] = right2;
}
}
return queleft1 == queright1 && queleft2 == queright2;
}
--------------------------------------------------------------------
class Solution {
public:
bool isSameTree(TreeNode* p, TreeNode* q) {
if (p == nullptr && q == nullptr) {
return true;
} else if (p == nullptr || q == nullptr) {
return false;
}
queue <TreeNode*> queue1, queue2;
queue1.push(p);
queue2.push(q);
while (!queue1.empty() && !queue2.empty()) {
auto node1 = queue1.front();
queue1.pop();
auto node2 = queue2.front();
queue2.pop();
if (node1->val != node2->val) {
return false;
}
auto left1 = node1->left, right1 = node1->right, left2 = node2->left, right2 = node2->right;
if ((left1 == nullptr) ^ (left2 == nullptr)) {
return false;
}
if ((right1 == nullptr) ^ (right2 == nullptr)) {
return false;
}
if (left1 != nullptr) {
queue1.push(left1);
}
if (right1 != nullptr) {
queue1.push(right1);
}
if (left2 != nullptr) {
queue2.push(left2);
}
if (right2 != nullptr) {
queue2.push(right2);
}
}
return queue1.empty() && queue2.empty();
}
};
226. 翻转二叉树
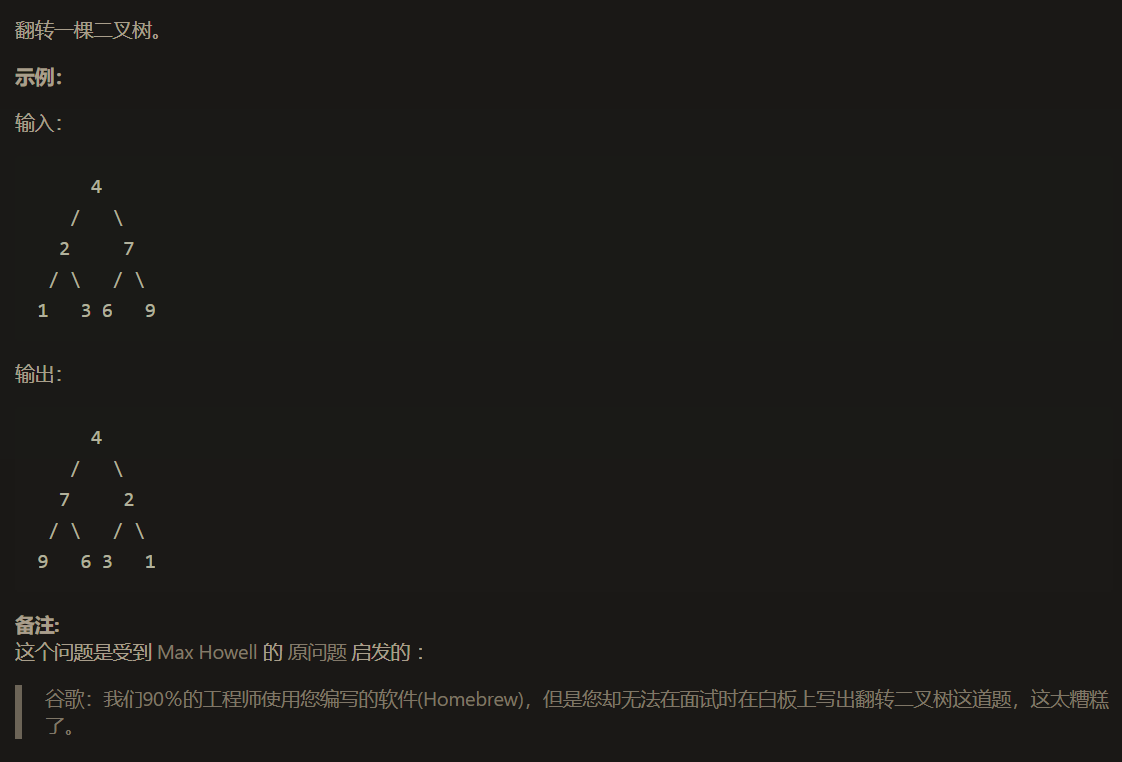
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-eHkkuHEY-1631410165745)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906233211449.png)]
struct TreeNode* invertTree(struct TreeNode* root) {
if (root == NULL) {
return NULL;
}
struct TreeNode* left = invertTree(root->left);
struct TreeNode* right = invertTree(root->right);
root->left = right;
root->right = left;
return root;
}
--------------------------------------------------------
class Solution {
public:
TreeNode* invertTree(TreeNode* root) {
if (root == nullptr) {
return nullptr;
}
TreeNode* left = invertTree(root->left);
TreeNode* right = invertTree(root->right);
root->left = right;
root->right = left;
return root;
}
};
144. 二叉树的前序遍历
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-IVY2Xsww-1631410165745)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906233405175.png)]
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-VqSyQqMO-1631410165746)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906233442557.png)]
void preorder(struct TreeNode* root, int* res, int* resSize) {
if (root == NULL) {
return;
}
res[(*resSize)++] = root->val;
preorder(root->left, res, resSize);
preorder(root->right, res, resSize);
}
int* preorderTraversal(struct TreeNode* root, int* returnSize) {
int* res = malloc(sizeof(int) * 2000);
*returnSize = 0;
preorder(root, res, returnSize);
return res;
}
------------------------------------------------------------------
class Solution {
public:
void preorder(TreeNode *root, vector<int> &res) {
if (root == nullptr) {
return;
}
res.push_back(root->val);
preorder(root->left, res);
preorder(root->right, res);
}
vector<int> preorderTraversal(TreeNode *root) {
vector<int> res;
preorder(root, res);
return res;
}
};
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-4Ui2W7OE-1631410165746)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906233539153.png)]
int* preorderTraversal(struct TreeNode* root, int* returnSize) {
int* res = malloc(sizeof(int) * 2000);
*returnSize = 0;
if (root == NULL) {
return res;
}
struct TreeNode* stk[2000];
struct TreeNode* node = root;
int stk_top = 0;
while (stk_top > 0 || node != NULL) {
while (node != NULL) {
res[(*returnSize)++] = node->val;
stk[stk_top++] = node;
node = node->left;
}
node = stk[--stk_top];
node = node->right;
}
return res;
}
----------------------------------------------------------------------
class Solution {
public:
vector<int> preorderTraversal(TreeNode* root) {
vector<int> res;
if (root == nullptr) {
return res;
}
stack<TreeNode*> stk;
TreeNode* node = root;
while (!stk.empty() || node != nullptr) {
while (node != nullptr) {
res.emplace_back(node->val);
stk.emplace(node);
node = node->left;
}
node = stk.top();
stk.pop();
node = node->right;
}
return res;
}
};
111. 二叉树的最小深度
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-ccT0q5XO-1631410165747)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906233645598.png)]
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-x0EskIlp-1631410165747)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906233711706.png)]
int minDepth(struct TreeNode *root) {
if (root == NULL) {
return 0;
}
if (root->left == NULL && root->right == NULL) {
return 1;
}
int min_depth = INT_MAX;
if (root->left != NULL) {
min_depth = fmin(minDepth(root->left), min_depth);
}
if (root->right != NULL) {
min_depth = fmin(minDepth(root->right), min_depth);
}
return min_depth + 1;
}
------------------------------------------------------------------------
class Solution {
public:
int minDepth(TreeNode *root) {
if (root == nullptr) {
return 0;
}
if (root->left == nullptr && root->right == nullptr) {
return 1;
}
int min_depth = INT_MAX;
if (root->left != nullptr) {
min_depth = min(minDepth(root->left), min_depth);
}
if (root->right != nullptr) {
min_depth = min(minDepth(root->right), min_depth);
}
return min_depth + 1;
}
};
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-ydP328lE-1631410165748)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906233802403.png)]
typedef struct {
int val;
struct TreeNode *node;
struct queNode *next;
} queNode;
void init(queNode **p, int val, struct TreeNode *node) {
(*p) = (queNode *)malloc(sizeof(queNode));
(*p)->val = val;
(*p)->node = node;
(*p)->next = NULL;
}
int minDepth(struct TreeNode *root) {
if (root == NULL) {
return 0;
}
queNode *queLeft, *queRight;
init(&queLeft, 1, root);
queRight = queLeft;
while (queLeft != NULL) {
struct TreeNode *node = queLeft->node;
int depth = queLeft->val;
if (node->left == NULL && node->right == NULL) {
return depth;
}
if (node->left != NULL) {
init(&queRight->next, depth + 1, node->left);
queRight = queRight->next;
}
if (node->right != NULL) {
init(&queRight->next, depth + 1, node->right);
queRight = queRight->next;
}
queLeft = queLeft->next;
}
return false;
}
-------------------------------------------------------------------------
class Solution {
public:
int minDepth(TreeNode *root) {
if (root == nullptr) {
return 0;
}
queue<pair<TreeNode *, int> > que;
que.emplace(root, 1);
while (!que.empty()) {
TreeNode *node = que.front().first;
int depth = que.front().second;
que.pop();
if (node->left == nullptr && node->right == nullptr) {
return depth;
}
if (node->left != nullptr) {
que.emplace(node->left, depth + 1);
}
if (node->right != nullptr) {
que.emplace(node->right, depth + 1);
}
}
return 0;
}
};
112. 路径总和
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-00hgieFP-1631410165748)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906233938807.png)]
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-H8hDaV3s-1631410165748)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906234012608.png)]
typedef struct queNode {
int val;
struct TreeNode *node;
struct queNode *next;
} queNode;
void init(struct queNode **p, int val, struct TreeNode *node) {
(*p) = (struct queNode *)malloc(sizeof(struct queNode));
(*p)->val = val;
(*p)->node = node;
(*p)->next = NULL;
}
bool hasPathSum(struct TreeNode *root, int sum) {
if (root == NULL) {
return false;
}
struct queNode *queLeft, *queRight;
init(&queLeft, root->val, root);
queRight = queLeft;
while (queLeft != NULL) {
struct TreeNode *now = queLeft->node;
int temp = queLeft->val;
if (now->left == NULL && now->right == NULL) {
if (temp == sum) return true;
}
if (now->left != NULL) {
init(&queRight->next, now->left->val + temp, now->left);
queRight = queRight->next;
}
if (now->right != NULL) {
init(&queRight->next, now->right->val + temp, now->right);
queRight = queRight->next;
}
queLeft = queLeft->next;
}
return false;
}
---------------------------------------------------------------------------
class Solution {
public:
bool hasPathSum(TreeNode *root, int sum) {
if (root == nullptr) {
return false;
}
queue<TreeNode *> que_node;
queue<int> que_val;
que_node.push(root);
que_val.push(root->val);
while (!que_node.empty()) {
TreeNode *now = que_node.front();
int temp = que_val.front();
que_node.pop();
que_val.pop();
if (now->left == nullptr && now->right == nullptr) {
if (temp == sum) {
return true;
}
continue;
}
if (now->left != nullptr) {
que_node.push(now->left);
que_val.push(now->left->val + temp);
}
if (now->right != nullptr) {
que_node.push(now->right);
que_val.push(now->right->val + temp);
}
}
return false;
}
};
145. 二叉树的后序遍历
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-SEiyjvXK-1631410165749)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906234156320.png)]
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-EAUHEPx9-1631410165749)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906234226463.png)]
void postorder(struct TreeNode *root, int *res, int *resSize) {
if (root == NULL) {
return;
}
postorder(root->left, res, resSize);
postorder(root->right, res, resSize);
res[(*resSize)++] = root->val;
}
int *postorderTraversal(struct TreeNode *root, int *returnSize) {
int *res = malloc(sizeof(int) * 2001);
*returnSize = 0;
postorder(root, res, returnSize);
return res;
}
--------------------------------------------------------------------
class Solution {
public:
void postorder(TreeNode *root, vector<int> &res) {
if (root == nullptr) {
return;
}
postorder(root->left, res);
postorder(root->right, res);
res.push_back(root->val);
}
vector<int> postorderTraversal(TreeNode *root) {
vector<int> res;
postorder(root, res);
return res;
}
};
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-tHyQn7hP-1631410165750)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906234322640.png)]
int *postorderTraversal(struct TreeNode *root, int *returnSize) {
int *res = malloc(sizeof(int) * 2001);
*returnSize = 0;
if (root == NULL) {
return res;
}
struct TreeNode **stk = malloc(sizeof(struct TreeNode *) * 2001);
int top = 0;
struct TreeNode *prev = NULL;
while (root != NULL || top > 0) {
while (root != NULL) {
stk[top++] = root;
root = root->left;
}
root = stk[--top];
if (root->right == NULL || root->right == prev) {
res[(*returnSize)++] = root->val;
prev = root;
root = NULL;
} else {
stk[top++] = root;
root = root->right;
}
}
return res;
}
-----------------------------------------------------------------------
class Solution {
public:
vector<int> postorderTraversal(TreeNode *root) {
vector<int> res;
if (root == nullptr) {
return res;
}
stack<TreeNode *> stk;
TreeNode *prev = nullptr;
while (root != nullptr || !stk.empty()) {
while (root != nullptr) {
stk.emplace(root);
root = root->left;
}
root = stk.top();
stk.pop();
if (root->right == nullptr || root->right == prev) {
res.emplace_back(root->val);
prev = root;
root = nullptr;
} else {
stk.emplace(root);
root = root->right;
}
}
return res;
}
};
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-XExe5TJK-1631410165750)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906234403833.png)]
void addPath(int *vec, int *vecSize, struct TreeNode *node) {
int count = 0;
while (node != NULL) {
++count;
vec[(*vecSize)++] = node->val;
node = node->right;
}
for (int i = (*vecSize) - count, j = (*vecSize) - 1; i < j; ++i, --j) {
int t = vec[i];
vec[i] = vec[j];
vec[j] = t;
}
}
int *postorderTraversal(struct TreeNode *root, int *returnSize) {
int *res = malloc(sizeof(int) * 2001);
*returnSize = 0;
if (root == NULL) {
return res;
}
struct TreeNode *p1 = root, *p2 = NULL;
while (p1 != NULL) {
p2 = p1->left;
if (p2 != NULL) {
while (p2->right != NULL && p2->right != p1) {
p2 = p2->right;
}
if (p2->right == NULL) {
p2->right = p1;
p1 = p1->left;
continue;
} else {
p2->right = NULL;
addPath(res, returnSize, p1->left);
}
}
p1 = p1->right;
}
addPath(res, returnSize, root);
return res;
}
--------------------------------------------------------------------------
class Solution {
public:
void addPath(vector<int> &vec, TreeNode *node) {
int count = 0;
while (node != nullptr) {
++count;
vec.emplace_back(node->val);
node = node->right;
}
reverse(vec.end() - count, vec.end());
}
vector<int> postorderTraversal(TreeNode *root) {
vector<int> res;
if (root == nullptr) {
return res;
}
TreeNode *p1 = root, *p2 = nullptr;
while (p1 != nullptr) {
p2 = p1->left;
if (p2 != nullptr) {
while (p2->right != nullptr && p2->right != p1) {
p2 = p2->right;
}
if (p2->right == nullptr) {
p2->right = p1;
p1 = p1->left;
continue;
} else {
p2->right = nullptr;
addPath(res, p1->left);
}
}
p1 = p1->right;
}
addPath(res, root);
return res;
}
};
110. 平衡二叉树
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-5Ck6xuXv-1631410165751)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906234611310.png)]
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-efecf3g3-1631410165751)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906234654446.png)]
int height(struct TreeNode* root) {
if (root == NULL) {
return 0;
} else {
return fmax(height(root->left), height(root->right)) + 1;
}
}
bool isBalanced(struct TreeNode* root) {
if (root == NULL) {
return true;
} else {
return fabs(height(root->left) - height(root->right)) <= 1 && isBalanced(root->left) && isBalanced(root->right);
}
}
------------------------------------------------------------------------
class Solution {
public:
int height(TreeNode* root) {
if (root == NULL) {
return 0;
} else {
return max(height(root->left), height(root->right)) + 1;
}
}
bool isBalanced(TreeNode* root) {
if (root == NULL) {
return true;
} else {
return abs(height(root->left) - height(root->right)) <= 1 && isBalanced(root->left) && isBalanced(root->right);
}
}
};
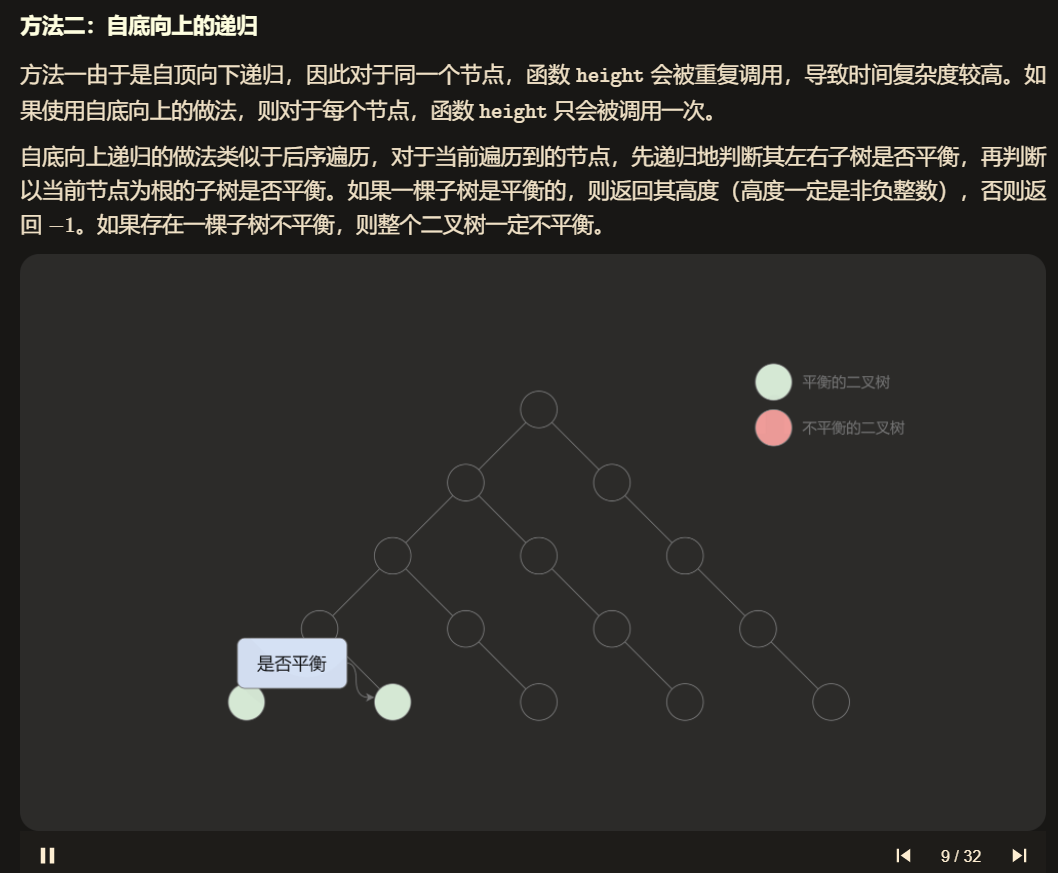
int height(struct TreeNode* root) {
if (root == NULL) {
return 0;
}
int leftHeight = height(root->left);
int rightHeight = height(root->right);
if (leftHeight == -1 || rightHeight == -1 || fabs(leftHeight - rightHeight) > 1) {
return -1;
} else {
return fmax(leftHeight, rightHeight) + 1;
}
}
bool isBalanced(struct TreeNode* root) {
return height(root) >= 0;
}
---------------------------------------------------------------------
class Solution {
public:
int height(TreeNode* root) {
if (root == NULL) {
return 0;
}
int leftHeight = height(root->left);
int rightHeight = height(root->right);
if (leftHeight == -1 || rightHeight == -1 || abs(leftHeight - rightHeight) > 1) {
return -1;
} else {
return max(leftHeight, rightHeight) + 1;
}
}
bool isBalanced(TreeNode* root) {
return height(root) >= 0;
}
};
114. 二叉树展开为链表
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-7hIa3TA8-1631410165752)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906235203849.png)]
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-AQjmOXnn-1631410165752)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906235425413.png)]
int num;
void flatten(struct TreeNode* root) {
num = 0;
struct TreeNode** l = (struct TreeNode**)malloc(0);
preorderTraversal(root, &l);
for (int i = 1; i < num; i++) {
struct TreeNode *prev = l[i - 1], *curr = l[i];
prev->left = NULL;
prev->right = curr;
}
free(l);
}
void preorderTraversal(struct TreeNode* root, struct TreeNode*** l) {
if (root != NULL) {
num++;
(*l) = (struct TreeNode**)realloc((*l), sizeof(struct TreeNode*) * num);
(*l)[num - 1] = root;
preorderTraversal(root->left, l);
preorderTraversal(root->right, l);
}
}
------------------------------------------------------------------------
class Solution {
public:
void flatten(TreeNode* root) {
vector<TreeNode*> l;
preorderTraversal(root, l);
int n = l.size();
for (int i = 1; i < n; i++) {
TreeNode *prev = l.at(i - 1), *curr = l.at(i);
prev->left = nullptr;
prev->right = curr;
}
}
void preorderTraversal(TreeNode* root, vector<TreeNode*> &l) {
if (root != NULL) {
l.push_back(root);
preorderTraversal(root->left, l);
preorderTraversal(root->right, l);
}
}
};
void flatten(struct TreeNode* root) {
struct TreeNode** stk = (struct TreeNode**)malloc(0);
int stk_top = 0;
struct TreeNode** vec = (struct TreeNode**)malloc(0);
int vec_len = 0;
struct TreeNode* node = root;
while (node != NULL || stk_top != 0) {
while (node != NULL) {
vec_len++;
vec = (struct TreeNode**)realloc(vec, sizeof(struct TreeNode*) * vec_len);
vec[vec_len - 1] = node;
stk_top++;
stk = (struct TreeNode**)realloc(stk, sizeof(struct TreeNode*) * stk_top);
stk[stk_top - 1] = node;
node = node->left;
}
node = stk[--stk_top];
node = node->right;
}
for (int i = 1; i < vec_len; i++) {
struct TreeNode *prev = vec[i - 1], *curr = vec[i];
prev->left = NULL;
prev->right = curr;
}
free(stk);
free(vec);
}
class Solution {
public:
void flatten(TreeNode* root) {
auto v = vector<TreeNode*>();
auto stk = stack<TreeNode*>();
TreeNode *node = root;
while (node != nullptr || !stk.empty()) {
while (node != nullptr) {
v.push_back(node);
stk.push(node);
node = node->left;
}
node = stk.top(); stk.pop();
node = node->right;
}
int size = v.size();
for (int i = 1; i < size; i++) {
auto prev = v.at(i - 1), curr = v.at(i);
prev->left = nullptr;
prev->right = curr;
}
}
};
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-s1hNkuMD-1631410165752)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906235425413.png)]
void flatten(struct TreeNode *root) {
if (root == NULL) {
return;
}
struct TreeNode **stk = (struct TreeNode **)malloc(sizeof(struct TreeNode *));
int stk_top = 1;
stk[0] = root;
struct TreeNode *prev = NULL;
while (stk_top != 0) {
struct TreeNode *curr = stk[--stk_top];
if (prev != NULL) {
prev->left = NULL;
prev->right = curr;
}
struct TreeNode *left = curr->left, *right = curr->right;
if (right != NULL) {
stk_top++;
stk = (struct TreeNode **)realloc(stk, sizeof(struct TreeNode *) * stk_top);
stk[stk_top - 1] = right;
}
if (left != NULL) {
stk_top++;
stk = (struct TreeNode **)realloc(stk, sizeof(struct TreeNode *) * stk_top);
stk[stk_top - 1] = left;
}
prev = curr;
}
free(stk);
}
--------------------------------------------------------------------
class Solution {
public:
void flatten(TreeNode* root) {
if (root == nullptr) {
return;
}
auto stk = stack<TreeNode*>();
stk.push(root);
TreeNode *prev = nullptr;
while (!stk.empty()) {
TreeNode *curr = stk.top(); stk.pop();
if (prev != nullptr) {
prev->left = nullptr;
prev->right = curr;
}
TreeNode *left = curr->left, *right = curr->right;
if (right != nullptr) {
stk.push(right);
}
if (left != nullptr) {
stk.push(left);
}
prev = curr;
}
}
};
本文内容由网友自发贡献,版权归原作者所有,本站不承担相应法律责任。如您发现有涉嫌抄袭侵权的内容,请联系:hwhale#tublm.com(使用前将#替换为@)