HTTP上传文件的时候,需要设置Content-Type为multipart/form-data;它包括一个类似标志性质的名为boundary的标志,它可以是随便输入的字符串。如boundary=----fasjdflkj23r8uffsdl, 对后面的具体内容也是必须的。它用来分辨一段内容的开始,用于将后面的数据分成数据块。数据可以文本,也可以是文件等。数据内容前面需要有Content-Disposition, Content-Type以及Content-Transfer-Encoding等说明字段。最后结束加入结束标记。
下面是一个发送给服务器的数据内容示例:
POST /upload_file/UploadFile HTTP/1.1
Accept: text/plain, */*
Accept-Language: zh-cn
Host: 192.168.29.65:80
Content-Type:multipart/form-data;boundary=---------------------------7d33a816d302b6
User-Agent: Mozilla/4.0 (compatible; OpenOffice.org)
Content-Length: 424
Connection: Keep-Alive
-----------------------------7d33a816d302b6
Content-Disposition: form-data; name="userfile1"; filename="E:\s"
Content-Type: application/octet-stream
a
bb
XXX
ccc
-----------------------------7d33a816d302b6
Content-Disposition: form-data; name="password1"
bar
-----------------------------7d33a816d302b6—
Content-Dispostion:form-data; name="submitted"
Submit
-----------------------------7d33a816d302b6—
实例的红色字体部分就是协议的头。给服务器上传数据时,并非协议头每个字段都得说明,其中,content-type是必须的,它包括一个类似标志性质的名为boundary的标志,它可以是随便输入的字符串。对后面的具体内容也是必须的。它用来分辨一段内容的开始。绿色字体部分就是需要上传的数据,可以是文本,也可以是图片等。最后的紫色部分就是协议的结尾了。
下面给出关键的VC实现代码及相关说明:
首先,根据HTTP的POST协议,封装协议头。
// strBoundary 为协议中的boundary
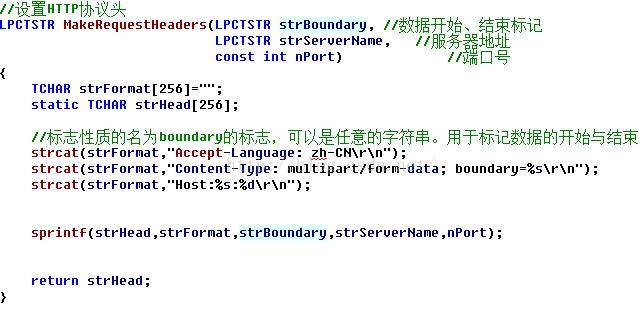
其次,封装数据前面的描述部分:
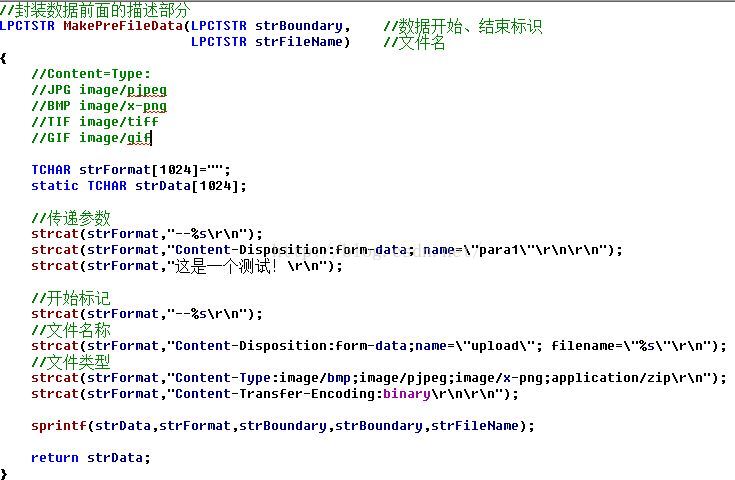
第三,封装协议尾
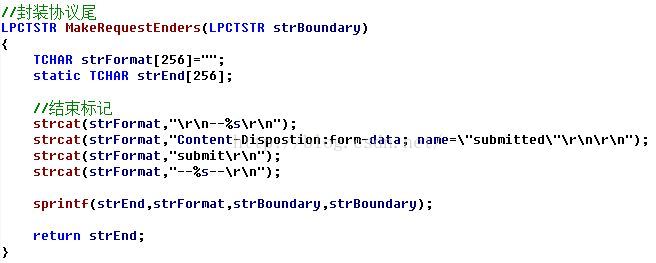
注意,其中的\r\n换行必不可少,否则会报错。
经过上面这些工作,http协议这部分工作差不多完成了,需要注意的是传输文件时,form-data中的name字段要和服务器中的名字一致。
既然协议已经封装好了,那么接下来就要解决与服务器的连接工作了,在VC 中,可以使用类CInternetSession来创建并初始化一个简单的Internet对话。首先,在应用程序中,建立一个 CIternetSession类对象,然后,利用GetHttpConnection函数来建立一个HTTP连接并且它返回一个 CHttpConnection对象的指针。其次,再利用OpenRequest方法来打开一个HTTP连接,接着可以用 AddRequestHeaders方法来添加一个或多个HTTP请求的头,即HTTP协议头。然后再利用SendRequestEx发送一个请求至 HTTP服务器,同时,利用该函数,加上CInternetFile的方法Write和WriterString可以发送各种类型的数据至服务器,但我们必需知道整个文件的大小=协议头大小+数据描述字段字节大小+实际数据字节大小+协议尾大小。当发送完数据后,需用EndRequest方法来关闭连接。具体代码如下:
BOOL SendTrack(CString strURL,CString m_strFilePath)
{
int startp = m_strFilePath.ReverseFind('\\');
int namelen = m_strFilePath.GetLength()-startp-1;
CString strFileName = m_strFilePath.Mid(startp+1,namelen);
//UpdateData(TRUE);
BOOL bResult = FALSE;
DWORD dwType = 0;
CString strServer;
CString strObject;
INTERNET_PORT wPort = 0;
bResult = AfxParseURL(strURL, dwType, strServer, strObject, wPort);
CString defServerName =strServer;
CString defObjectName =strObject;
// USES_CONVERSION;
CInternetSession Session;
CHttpConnection *pHttpConnection = NULL;
INTERNET_PORT nPort = wPort;
CFile fTrack;
CHttpFile* pHTTP;
CString strRequestHeader=_T("");
CString strHTTPBoundary=_T("");
CString strPreFileData=_T("");
CString strPostFileData=_T("");
CString strResponse =_T("");
DWORD dwTotalRequestLength;
DWORD dwChunkLength;
DWORD dwReadLength;
DWORD dwResponseLength;
TCHAR szError[MAX_PATH];
void* pBuffer =NULL;
LPSTR szResponse;
BOOL bSuccess = TRUE;
CString strDebugMessage =_T("");
if (FALSE == fTrack.Open(m_strFilePath, CFile::modeRead | CFile::shareDenyWrite))
{
AfxMessageBox(_T("Unable to open the file."));
return FALSE;
}
strHTTPBoundary = _T("-----------------------------7d86d16250370");
strRequestHeader =MakeRequestHeaders(strHTTPBoundary,strServer,wPort);
strPreFileData = MakePreFileData(strHTTPBoundary, strFileName);
strPostFileData = MakeRequestEnders(strHTTPBoundary);
MessageBox(NULL,strRequestHeader,"RequestHeader",MB_OK | MB_ICONINFORMATION);
MessageBox(NULL,strPreFileData,"PreFileData",MB_OK | MB_ICONINFORMATION);
MessageBox(NULL,strPostFileData,"PostFileData",MB_OK | MB_ICONINFORMATION);
dwTotalRequestLength = strPreFileData.GetLength() + strPostFileData.GetLength() + fTrack.GetLength();
dwChunkLength = 64 * 1024;
pBuffer = malloc(dwChunkLength);
if (NULL == pBuffer)
{
return FALSE;
}
try
{
pHttpConnection = Session.GetHttpConnection(defServerName,nPort);
pHTTP = pHttpConnection->OpenRequest(CHttpConnection::HTTP_VERB_POST, defObjectName);
pHTTP->AddRequestHeaders(strRequestHeader);
pHTTP->SendRequestEx(dwTotalRequestLength, HSR_SYNC | HSR_INITIATE);
#ifdef _UNICODE
pHTTP->Write(W2A(strPreFileData), strPreFileData.GetLength());
#else
pHTTP->Write((LPSTR)(LPCSTR)strPreFileData, strPreFileData.GetLength());
#endif
dwReadLength = -1;
while (0 != dwReadLength)
{
strDebugMessage.Format(_T("%u / %u\n"), fTrack.GetPosition(), fTrack.GetLength());
TRACE(strDebugMessage);
dwReadLength = fTrack.Read(pBuffer, dwChunkLength);
if (0 != dwReadLength)
{
pHTTP->Write(pBuffer, dwReadLength);
}
}
#ifdef _UNICODE
pHTTP->Write(W2A(strPostFileData), strPostFileData.GetLength());
#else
pHTTP->Write((LPSTR)(LPCSTR)strPostFileData, strPostFileData.GetLength());
#endif
pHTTP->EndRequest(HSR_SYNC);
dwResponseLength = pHTTP->GetLength();
while (0 != dwResponseLength)
{
szResponse = (LPSTR)malloc(dwResponseLength + 1);
szResponse[dwResponseLength] = '\0';
pHTTP->Read(szResponse, dwResponseLength);
strResponse += szResponse;
free(szResponse);
dwResponseLength = pHTTP->GetLength();
}
MessageBox(NULL,strResponse,"Response",MB_OK | MB_ICONINFORMATION);
}
catch (CException* e)
{
e->GetErrorMessage(szError, MAX_PATH);
e->Delete();
AfxMessageBox(szError);
bSuccess = FALSE;
}
pHTTP->Close();
delete pHTTP;
fTrack.Close();
if (NULL != pBuffer)
{
free(pBuffer);
}
return bSuccess;
}
我测试将文件上传到java服务器上,由自己写的severlet上,后来使用sturts2,发现上传文件的目录会被sturts2去掉,还有就是C++提交的参数中不能使用中文,试过各种转,但没一种好用,但是java返回参数却没有问题。C++编码转java不知道如何转,其他一切正常。
下面我测试用的JAVA代码