前期准备
1.开发环境及框架的搭建。(基于SSH开发框架)
2.数据库建表,表应该有一个字段用来存储文件在服务区上的存储路径。
3.map.xml文件
4.Action.xml文件
5.写好实体类及get()set()方法
6.DAO层
7.Action()实现,Action()里面需要实现文件的上传服务器功能和路径存储数据库功能。
8.前端页面,前端页面需要实现文件的选择功能。
具体实例展示
1.数据库建表
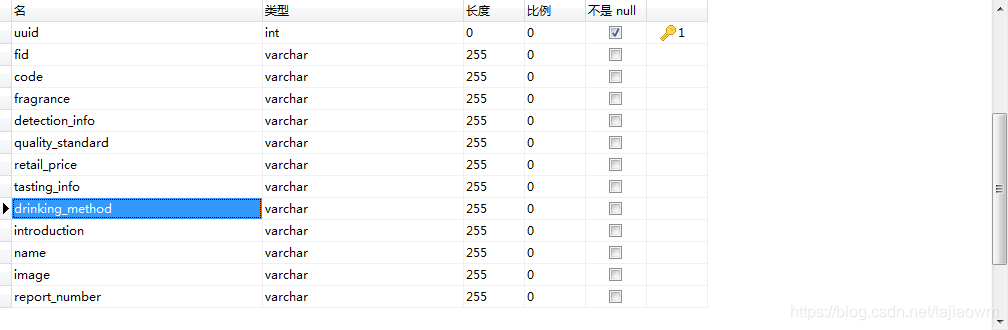
其中image字段用来存储文件在服务器上的存储路径。
2.增删查改(map.xml文件)
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE sqlMap PUBLIC "-//iBATIS.com//DTD SQL Map 2.0//EN" "sql-map-2.dtd">
<sqlMap>
<!-- Start -->
<typeAlias alias="finishwinewl" type="com.qkj.finishwinewl.bean.finishwinewlBean" />
<select id="finishwinewl_getfinishwinewl" resultClass="finishwinewl" parameterClass="java.util.Map">
<![CDATA[
Select * From qkj_t_finishwinewl
]]>
<dynamic prepend="WHERE"><!--所有查询项目-->
<isNotNull prepend="AND" property="uuid"><![CDATA[ uuid=#uuid# ]]></isNotNull>
</dynamic>
</select>
<insert id="finishwinewl_addfinishwinewl" parameterClass="finishwinewl">
<![CDATA[
Insert Into qkj_t_finishwinewl (fid,code,fragrance,detection_info,quality_standard,retail_price,tasting_info,drinking_method,introduction,name,image,report_number)
Values (#fid#,#code#,#fragrance#,#detection_info#,#quality_standard#,#retail_price#,#tasting_info#,#drinking_method#,#introduction#,#name#,#image#,#report_number#)
]]>
<selectKey resultClass="int" keyProperty="uuid">
<![CDATA[
Select @@IDENTITY
]]>
</selectKey>
</insert>
<update id="finishwinewl_mdyfinishwinewl" parameterClass="finishwinewl">
<![CDATA[
Update qkj_t_finishwinewl
Set
fid=#fid#,
code=#code#,
fragrance=#fragrance#,
detection_info=#detection_info#,
quality_standard=#quality_standard#,
retail_price=#retail_price#,
tasting_info=#tasting_info#,
drinking_method=#drinking_method#,
introduction=#introduction#,
report_number=#report_number#,
name=#name#,
image=#image# Where uuid = #uuid#
]]>
</update>
<delete id="finishwinewl_delfinishwinewl" parameterClass="finishwinewl">
<![CDATA[
Delete From qkj_t_finishwinewl Where uuid=#uuid#
]]>
</delete>
<!-- End -->
</sqlMap>
3.dao层
package com.qkj.finishwinewl.dao;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.iweb.sys.AbstractDAO;
/**
* @author xiaoyan
*
*/
public class finishwinewlDao extends AbstractDAO{
public List list(Map<String, Object> map) {
return super.list("finishwinewl_getfinishwinewl", map);
}
public Object get(Object uuid) {
Map<String, Object> map = new HashMap<String, Object>();
map.put("uuid", uuid);
return super.get("finishwinewl_getfinishwinewl", map);
}
public Object add(Object parameters) {
return super.add("finishwinewl_addfinishwinewl", parameters);
}
public int save(Object parameters) {
return super.save("finishwinewl_mdyfinishwinewl", parameters);
}
public int delete(Object parameters) {
return super.delete("finishwinewl_delfinishwinewl", parameters);
}
public int getResultCount() {
return super.getResultCount();
}
}
4.Action实现
package com.qkj.finishwinewl.action;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.InputStream;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.apache.struts2.ServletActionContext;
import org.iweb.sys.ContextHelper;
import org.iweb.sys.Parameters;
import org.iweb.sys.ToolsUtil;
import com.opensymphony.xwork2.ActionSupport;
import com.qkj.finishwinewl.dao.finishwinewlDao;
import com.qkj.finishwinewl.bean.finishwinewlBean;
/**
* @author yanluhai
*
*/
public class finishwinewlAction extends ActionSupport{
private static final long serialVersionUID = 1L;
private static Log log = LogFactory.getLog(finishwinewlAction.class);
private Map<String, Object> map = new HashMap<String, Object>();
private finishwinewlDao dao = new finishwinewlDao();
private finishwinewlBean finishwinewl;
private List<finishwinewlBean> finishwinewls;
private String message;
private String viewFlag;
private int recCount;
private int pageSize;
private int currPage;
private String path = "<a href='/manager/default'>首页</a> > 列表";
private String [] imageUpload;
private File fileUpload;
private String fileUploadFileName; // 文件名+FileName
public File getFileUpload() {
return fileUpload;
}
public void setFileUpload(File fileUpload) {
this.fileUpload = fileUpload;
}
public static Log getLog() {
return log;
}
public static void setLog(Log log) {
finishwinewlAction.log = log;
}
public Map<String, Object> getMap() {
return map;
}
public void setMap(Map<String, Object> map) {
this.map = map;
}
public finishwinewlDao getDao() {
return dao;
}
public void setDao(finishwinewlDao dao) {
this.dao = dao;
}
public finishwinewlBean getFinishwinewl() {
return finishwinewl;
}
public void setFinishwinewl(finishwinewlBean finishwinewl) {
this.finishwinewl = finishwinewl;
}
public List<finishwinewlBean> getFinishwinewls() {
return finishwinewls;
}
public void setFinishwinewls(List<finishwinewlBean> finishwinewls) {
this.finishwinewls = finishwinewls;
}
public String getMessage() {
return message;
}
public void setMessage(String message) {
this.message = message;
}
public String getViewFlag() {
return viewFlag;
}
public void setViewFlag(String viewFlag) {
this.viewFlag = viewFlag;
}
public int getRecCount() {
return recCount;
}
public void setRecCount(int recCount) {
this.recCount = recCount;
}
public int getPageSize() {
return pageSize;
}
public void setPageSize(int pageSize) {
this.pageSize = pageSize;
}
public int getCurrPage() {
return currPage;
}
public void setCurrPage(int currPage) {
this.currPage = currPage;
}
public String getPath() {
return path;
}
public void setPath(String path) {
this.path = path;
}
public String[] getImageUpload() {
return imageUpload;
}
public void setImageUpload(String[] imageUpload) {
this.imageUpload = imageUpload;
}
public String getFileUploadFileName() {
return fileUploadFileName;
}
public void setFileUploadFileName(String fileUploadFileName) {
this.fileUploadFileName = fileUploadFileName;
}
public static long getSerialversionuid() {
return serialVersionUID;
}
public String list() throws Exception {
ContextHelper.isPermit("QKJ_FINISHWINEWL_FINISHWINEWL_LIST");
try {
map.clear();
if (finishwinewl == null) finishwinewl = new finishwinewlBean();
ContextHelper.setSearchDeptPermit4Search("QKJ_FINISHWINEWL_FINISHWINEWL_LIST", map, "apply_depts", "apply_user");
ContextHelper.SimpleSearchMap4Page("QKJ_FINISHWINEWL_FINISHWINEWL_LIST", map, finishwinewl, viewFlag);
this.setPageSize(ContextHelper.getPageSize(map));
this.setCurrPage(ContextHelper.getCurrPage(map));
this.setFinishwinewls(dao.list(map));
this.setRecCount(dao.getResultCount());
path = "<a href='/manager/default'>首页</a> > 列表";
} catch (Exception e) {
log.error(this.getClass().getName() + "!list 读取数据错误:", e);
throw new Exception(this.getClass().getName() + "!list 读取数据错误:", e);
}
return SUCCESS;
}
public String load() throws Exception {
try {
if (null == viewFlag) {
this.setFinishwinewl(null);
setMessage("你没有选择任何操作!");
} else if ("add".equals(viewFlag)) {
this.setFinishwinewl(null);
path = "<a href='/manager/default'>首页</a> > <a href='/finishwinewl/finishwinewl_list?viewFlag=relist'>列表</a> > 增加";
} else if ("mdy".equals(viewFlag)) {
if (!(finishwinewl == null || finishwinewl.getUuid() == null)) {
this.setFinishwinewl((finishwinewlBean) dao.get(finishwinewl.getUuid()));
System.out.println(finishwinewl.getImage());
} else {
this.setFinishwinewl(null);
}
path = "<a href='/manager/default'>首页</a> > <a href='/finishwinewl/finishwinewl_list?viewFlag=relist'>列表</a> > 修改产品";
} else {
this.setFinishwinewl(null);
setMessage("无操作类型!");
}
} catch (Exception e) {
log.error(this.getClass().getName() + "!load 读取数据错误:", e);
throw new Exception(this.getClass().getName() + "!load 读取数据错误:", e);
}
return SUCCESS;
}
public String add() throws Exception {
ContextHelper.isPermit("QKJ_FINISHWINEWL_FINISHWINEWL_ADD");
try {
/*finishwinewl.setAdd_user(ContextHelper.getUserLoginUuid());
finishwinewl.setAdd_time(new Date());*/
//String c = fileUpload.getParent();
//File mm = new File(c + File.separator + fileUploadFileName);
//copyFile(fileUpload.toString(), mm.toString());
fileUploadFileName="9FBA2651-8851-4a92-88C7-36BDA03856E9.png";
String UploadRoot="";
String t=Thread.currentThread().getContextClassLoader().getResource("").getPath();
//int num=t.indexOf(".metadata");
//String realpath=t.substring(1,num).replace('/', '\\')+"yxzx\\WebContent\\Imageupload";
// String realpath = ServletActionContext.getServletContext().getRealPath("./") + File.separator + "Imageupload";
// String realpath = "u01/wwwhome/Imageupload";
//String realpath = "u01" + File.separator +"wwwhome" + File.separator +"Imageupload";
//String realpath = "E:\\hhh\\kk";
String realpath = ServletActionContext.getServletContext().getRealPath("./") + File.separator + "Imageupload";
if (fileUploadFileName != null)
{
//imageUpload = fileUploadFileName.split(",");
/*for(int i=0;i<imageUpload.length;i++)
{*/
//if (imageUpload !=null)
//{
String datetime=new SimpleDateFormat("yyyyMMddhhmmss").format(new Date());
int x=(int)(Math.random()*100000);
while(x<10000 || !handle(x))
{
x=(int)(Math.random()*100000);
}
int ii= fileUploadFileName.indexOf(".");
String sExt = fileUploadFileName.substring(ii,fileUploadFileName.length());
String fileNamel = datetime+String.valueOf(x)+sExt;
File mm = new File(realpath + File.separator + fileNamel);
copyFile(fileUpload.toString(), mm.toString());
UploadRoot=UploadRoot+"\\Imageupload\\" + fileNamel;
//File myFile = new File("C:" + File.separator + "tmp" + File.separator, "test.txt");
//}
//}
}
finishwinewl.setImage(UploadRoot);
dao.add(finishwinewl);
} catch (Exception e) {
log.error(this.getClass().getName() + "!add 数据添加失败:", e);
throw new Exception(this.getClass().getName() + "!add 数据添加失败:", e);
}
return SUCCESS;
}
public String save() throws Exception {
ContextHelper.isPermit("QKJ_FINISHWINEWL_FINISHWINEWL_MDY");
try {
System.out.println("aaa");
/*finishwinewl.setLm_user(ContextHelper.getUserLoginUuid());
finishwinewl.setLm_time(new Date());*/
fileUploadFileName="9FBA2651-8851-4a92-88C7-36BDA03856E9.png";
String UploadRoot="";
String t=Thread.currentThread().getContextClassLoader().getResource("").getPath();
//int num=t.indexOf(".metadata");
//String realpath=t.substring(1,num).replace('/', '\\')+"yxzx\\WebContent\\Imageupload";
// String realpath = ServletActionContext.getServletContext().getRealPath("./") + File.separator + "Imageupload";
// String realpath = "u01/wwwhome/Imageupload";
//String realpath = "u01" + File.separator +"wwwhome" + File.separator +"Imageupload";
String realpath = ServletActionContext.getServletContext().getRealPath("./") + File.separator + "Imageupload";
if (fileUploadFileName != null)
{
//imageUpload = fileUploadFileName.split(",");
/*for(int i=0;i<imageUpload.length;i++)
{*/
//if (imageUpload !=null)
//{
String datetime=new SimpleDateFormat("yyyyMMddhhmmss").format(new Date());
int x=(int)(Math.random()*100000);
while(x<10000 || !handle(x))
{
x=(int)(Math.random()*100000);
}
int ii= fileUploadFileName.indexOf(".");
String sExt = fileUploadFileName.substring(ii,fileUploadFileName.length());
String fileNamel = datetime+String.valueOf(x)+sExt;
File mm = new File(realpath + File.separator + fileNamel);
copyFile(fileUpload.toString(), mm.toString());
UploadRoot=UploadRoot+"\\Imageupload\\" + fileNamel;
//File myFile = new File("C:" + File.separator + "tmp" + File.separator, "test.txt");
//}
//}
}
finishwinewl.setImage(UploadRoot);
dao.save(finishwinewl);
} catch (Exception e) {
log.error(this.getClass().getName() + "!save 数据更新失败:", e);
throw new Exception(this.getClass().getName() + "!save 数据更新失败:", e);
}
return SUCCESS;
}
public String del() throws Exception {
ContextHelper.isPermit("QKJ_FINISHWINEWL_FINISHWINEWL_DEL");
try {
dao.delete(finishwinewl);
setMessage("删除成功!ID=" + finishwinewl.getUuid());
} catch (Exception e) {
log.error(this.getClass().getName() + "!del 数据删除失败:", e);
throw new Exception(this.getClass().getName() + "!del 数据删除失败:", e);
}
return SUCCESS;
}
public static void copyFile(String oldPath, String newPath)
{
try {
int bytesum = 0;
int byteread = 0;
File oldfile = new File(oldPath);
System.out.println("oldPath:"+oldPath);
System.out.println("newPath:"+newPath);
if (oldfile.exists())
{ // 文件存在时
InputStream inStream = new FileInputStream(oldPath); // 读入原文件
FileOutputStream fs = new FileOutputStream(newPath);
byte[] buffer = new byte[1444];
int length;
while ((byteread = inStream.read(buffer)) != -1)
{
bytesum += byteread; // 字节数 文件大小
System.out.println(bytesum);
fs.write(buffer, 0, byteread);
}
inStream.close();
}
} catch (Exception e)
{
System.out.println("复制单个文件操作出错");
e.printStackTrace();
}
}
public static boolean handle(int n)
{
int[] list=new int[5];
for(int i=0;i<5;i++)
{
list[i]=n%10;
n=n/10;
}
for(int i=0;i<5;i++)
{
for(int j=i+1;j<5;j++)
{
if(list[i]==list[j]) return false;
}
}
return true;
}
}
5.前端页面
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<%@ taglib prefix="s" uri="/struts-tags"%>
<%@ taglib prefix="it" uri="http://qkjchina.com/iweb/iwebTags" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>列表--<s:text name="APP_NAME" /></title>
<s:action name="ref_head" namespace="/manager" executeResult="true" />
</head>
<body>
<!-- 顶部和左侧菜单导航 -->
<s:action name="nav" namespace="/manage" executeResult="true" />
<div class="tab_right">
<div class="tab_warp main" >
<div class="dq_step">
${path}
<c:if test="${it:checkPermit('QKJ_FINISHWINEWL_FINISHWINEWL_ADD',null)==true}">
<span class="opb lb op-area"><a href="<s:url namespace="/finishwinewl" action="finishwinewl_load"><s:param name="viewFlag">add</s:param></s:url>">添加单据</a></span>
</c:if>
</div>
<s:form id="serachForm" name="serachForm" action="finishwinewl_list" method="get" namespace="/finishwinewl" theme="simple">
<div class="label_con">
<div class="label_main">
<div class="label_hang tac">
<s:submit value="搜索" /> <s:reset value="重置" />
</div>
</div>
</div>
</s:form>
<div class="tab_warp">
<table>
<tr id="coltr">
<th>物料编码</th>
<th>物料名称</th>
<th>香型</th>
<th>检测信息</th>
<th>质量标准</th>
<th>零售价</th>
<th>品鉴信息</th>
<th>建议饮用方式</th>
<th>产品介绍</th>
<th>检测报告编号</th>
<th>操作</th>
</tr>
<s:iterator value="finishwinewls" status="sta">
<tr id="showtr${uuid}">
<td>${code}</td>
<td>${name}</td>
<td>${fragrance}</td>
<td>${introduction}</td>
<td>${detection_info}</td>
<td>${quality_standard }</td>
<td>${retail_price}</td>
<td>${tasting_info}</td>
<td>${drinking_method}</td>
<td>${report_number}</td>
<td class="td4 op-area">
<c:if test="${it:checkPermit('QKJ_FINISHWINEWL_FINISHWINEWL_MDY',null)==true}">
<a class="input-blue" href="<s:url namespace="/finishwinewl" action="finishwinewl_load"><s:param name="viewFlag">mdy</s:param><s:param name="finishwinewl.uuid" value="uuid"></s:param></s:url>">修改</a>
</c:if>
<c:if test="${it:checkPermit('QKJ_FINISHWINEWL_FINISHWINEWL_DEL',null)==true}">
<a class="input-red" href="<s:url namespace="/finishwinewl" action="finishwinewl_del"><s:param name="finishwinewl.uuid" value="uuid"></s:param></s:url>" onclick="return isDel();">删除</a>
</c:if>
</td>
<td>
</td>
<td class="td0 op-area"><a onClick="showDetail('showtr${uuid}');" href="javascript:;" class="input-nostyle">查看</a></td>
</tr>
</s:iterator>
</table>
</div>
<div id="listpage" class="pagination"></div>
</div>
</div>
<!-- HIDDEN AREA END -->
<s:action name="ref_foot" namespace="/manager" executeResult="true" />
<script type="text/javascript">
$(function(){
printPagination("listpage",'${currPage}','${recCount}','${pageSize}');
});
</body>
</html>
此页用列表的方式展示相应信息
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<%@ taglib prefix="s" uri="/struts-tags"%>
<%@ taglib prefix="it" uri="http://qkjchina.com/iweb/iwebTags"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>产品管理--<s:text name="APP_NAME" /></title>
<s:action name="ref_head" namespace="/manager" executeResult="true" />
</head>
<body>
<s:action name="nav" namespace="/manage" executeResult="true" />
<div class="tab_right">
<div class="tab_warp main">
<div class="dq_step">
${path}
<span class="opb lb op-area"><a href="<s:url namespace="/finishwinewl" action="finishwinewl_list"></s:url>">返回列表</a></span>
</div>
<s:form id="editForm" name="editForm" cssClass="validForm"
action="finishwinewl_load" namespace="/finishwinewl" method="post"
theme="simple" enctype="multipart/form-data">
<!--<form action="/sysvip/uploadFile" method="post" enctype ="multipart/form-data">-->
<div class="label_hang">
<div class="label_ltit">fid:</div>
<div class="label_rwben label_rwb">
<s:textfield name="finishwinewl.fid" title="fid"/>
</div>
</div>
<div class="label_hang">
<div class="label_ltit">物料编码:</div>
<div class="label_rwben label_rwb">
<s:textfield name="finishwinewl.code" title="物料编码"/>
</div>
</div>
<div class="label_hang">
<div class="label_ltit">香型:</div>
<div class="label_rwben label_rwb">
<s:textfield name="finishwinewl.fragrance" title="香型"/>
</div>
</div>
<div class="label_hang">
<div class="label_ltit">检测信息:</div>
<div class="label_rwben label_rwb">
<s:textfield name="finishwinewl.detection_info" title="检测信息"/>
</div>
</div>
<div class="label_hang">
<div class="label_ltit">质量标准:</div>
<div class="label_rwben label_rwb">
<s:textfield name="finishwinewl.quality_standard " title="质量标准"/>
</div>
</div>
<div class="label_hang">
<div class="label_ltit">零售价:</div>
<div class="label_rwben label_rwb">
<s:textfield name="finishwinewl.retail_price" title="零售价"/>
</div>
</div>
<div class="label_hang">
<div class="label_ltit">品鉴信息:</div>
<div class="label_rwben label_rwb">
<s:textfield name="finishwinewl.tasting_info" title="品鉴信息"/>
</div>
</div>
<div class="label_hang">
<div class="label_ltit">建议饮用方式:</div>
<div class="label_rwben label_rwb">
<s:textfield name="finishwinewl.drinking_method" title="建议饮用方式"/>
</div>
</div>
<div class="label_hang">
<div class="label_ltit">产品介绍:</div>
<div class="label_rwben label_rwb">
<s:textfield name="finishwinewl.introduction" title="产品介绍"/>
</div>
</div>
<div class="label_hang">
<div class="label_ltit">检测报告编号:</div>
<div class="label_rwben label_rwb">
<s:textfield name="finishwinewl.report_number" title="产品介绍"/>
</div>
</div>
<s:if test="'mdy' == viewFlag">
<div class="label_main">
<div class="label_hang">
<s:hidden name="finishwinewl.image" title="产品图片路径" />
<div class="label_ltit">产品图片:
</div>
<img src="${finishwinewl.image }" border="0" width="300" height="300" class="imgbox_img" />${image}
</div>
</div>
</s:if>
<div class="label_main">
<div class="label_hang" id="more">
<div class="label_ltit">产品图片:</div>
<div class="label_rwben">
<input type="file" name="fileUpload" title="上传产品图片" />
</div>
</div>
</div>
<div class="label_main">
<div class="label_hang">
<div class="label_ltit" style="font-size: 14px;">相关操作:</div>
<div class="label_rwbenx">
<span id="message"><s:property value="message" /></span>
<s:if test="null == finishwinewl && 'add' == viewFlag">
<c:if test="${it:checkPermit('QKJ_FINISHWINEWL_FINISHWINEWL_ADD',null)==true}">
<s:submit id="add" name="add" value="确定" action="finishwinewl_add"
class="input-blue" />
</c:if>
</s:if>
<s:elseif test="null != finishwinewl && 'mdy' == viewFlag">
<c:if
test="${it:checkPermit('QKJ_FINISHWINEWL_FINISHWINEWL_MDY',null)==true}">
<s:submit id="save" name="save" value="保存"
action="finishwinewl_save" class="input-blue" />
</c:if>
<c:if
test="${it:checkPermit('QKJ_FINISHWINEWL_FINISHWINEWL_DEL',null)==true}">
<s:submit id="delete" name="delete" value="删除"
action="finishwinewl_del" class="input-red"
onclick="return isDel();" />
</c:if>
</s:elseif>
<input type="button" class="input-gray" value="返回"
onclick="linkurl('<s:url action="product_list" namespace="/qkjmanage" />');" />
</div>
</div>
</div>
<!-- </form>-->
</s:form>
</div>
</div>
<s:action name="ref_foot" namespace="/manager" executeResult="true" />
</body>
</html>
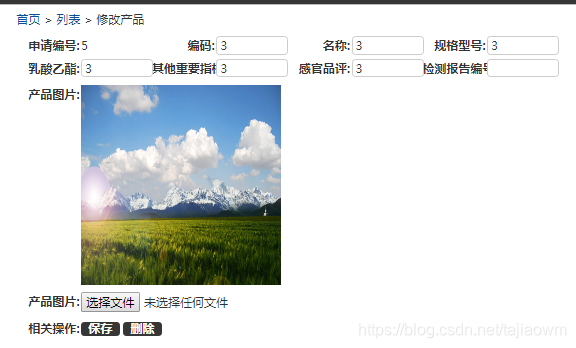
最终可以看到我们上传的各项数据及文件(以图片为例)展示在页面上。
明细页实现前端收集数据和展示文件的功能
接下来介绍实现文件上传的几个关键点
1.前端文件如何选择:
<div class="label_main">
<div class="label_hang" id="more">
<div class="label_ltit">产品图片:</div>
<div class="label_rwben">
<input type="file" name="fileUpload" title="上传产品图片" />
</div>
</div>
</div>
其中fileUpload暂存上传的文件,
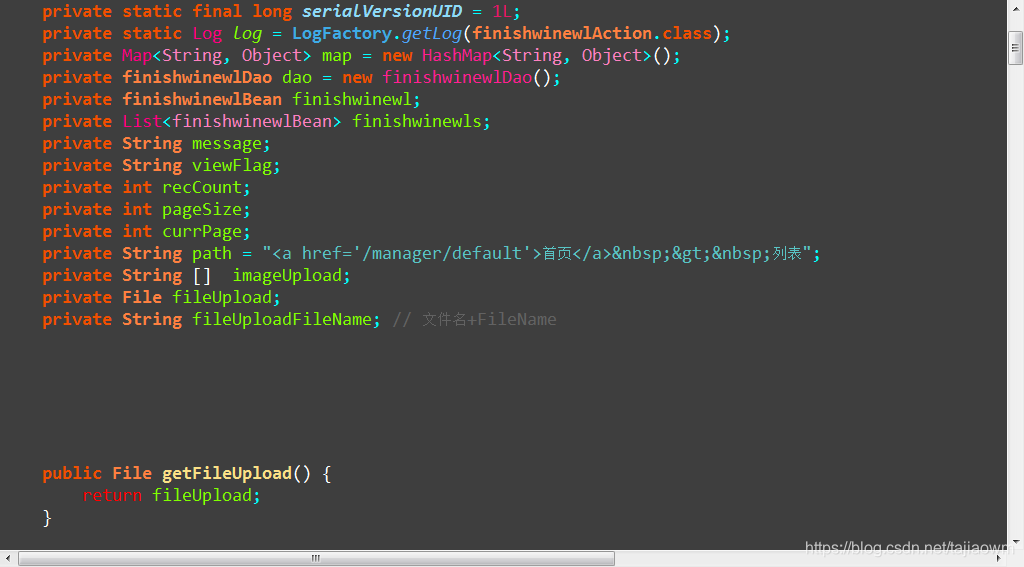
在后端代码中定义file 类型的fileUpload,写好它的get(),set()方法,想象一下我们已经在前端将一个文件传到现在的后端fileUpload中,现在要做的是将文件上传到服务器指定文件夹或者项目的某个文件夹中,要将这个路径存放到我们的数据库相应字段下面。
下面实现的就是将文件上传到项目文件夹以及将路径存储到数据库的过程。
public String add() throws Exception {
ContextHelper.isPermit("QKJ_FINISHWINEWL_FINISHWINEWL_ADD");
try {
/*finishwinewl.setAdd_user(ContextHelper.getUserLoginUuid());
finishwinewl.setAdd_time(new Date());*/
//String c = fileUpload.getParent();
//File mm = new File(c + File.separator + fileUploadFileName);
//copyFile(fileUpload.toString(), mm.toString());
fileUploadFileName="9FBA2651-8851-4a92-88C7-36BDA03856E9.png";
String UploadRoot="";
String t=Thread.currentThread().getContextClassLoader().getResource("").getPath();
String realpath = ServletActionContext.getServletContext().getRealPath("./") + File.separator + "Imageupload";
if (fileUploadFileName != null)
{
//imageUpload = fileUploadFileName.split(",");
/*for(int i=0;i<imageUpload.length;i++)
{*/
//if (imageUpload !=null)
//{
String datetime=new SimpleDateFormat("yyyyMMddhhmmss").format(new Date());
int x=(int)(Math.random()*100000);
while(x<10000 || !handle(x))
{
x=(int)(Math.random()*100000);
}
int ii= fileUploadFileName.indexOf(".");
String sExt = fileUploadFileName.substring(ii,fileUploadFileName.length());
String fileNamel = datetime+String.valueOf(x)+sExt;
File mm = new File(realpath + File.separator + fileNamel);
copyFile(fileUpload.toString(), mm.toString());
UploadRoot=UploadRoot+"\\Imageupload\\" + fileNamel;
//File myFile = new File("C:" + File.separator + "tmp" + File.separator, "test.txt");
//}
//}
}
finishwinewl.setImage(UploadRoot);
dao.add(finishwinewl);
} catch (Exception e) {
log.error(this.getClass().getName() + "!add 数据添加失败:", e);
throw new Exception(this.getClass().getName() + "!add 数据添加失败:", e);
}
return SUCCESS;
}
我们随便写一个固定的文件名,主要是后面的.png等文件类型,并获取文件即将上传的项目文件夹的路径,并在该文件夹下面建一个图片,将我们上传的图片通过copfile函数覆盖创建在项目文件夹下面的那个图片
public static void copyFile(String oldPath, String newPath)
{
try {
int bytesum = 0;
int byteread = 0;
File oldfile = new File(oldPath);
System.out.println("oldPath:"+oldPath);
System.out.println("newPath:"+newPath);
if (oldfile.exists())
{ // 文件存在时
InputStream inStream = new FileInputStream(oldPath); // 读入原文件
FileOutputStream fs = new FileOutputStream(newPath);
byte[] buffer = new byte[1444];
int length;
while ((byteread = inStream.read(buffer)) != -1)
{
bytesum += byteread; // 字节数 文件大小
System.out.println(bytesum);
fs.write(buffer, 0, byteread);
}
inStream.close();
}
} catch (Exception e)
{
System.out.println("复制单个文件操作出错");
e.printStackTrace();
}
}
public static boolean handle(int n)//这里只是得到一个随机的数字
{
int[] list=new int[5];
for(int i=0;i<5;i++)
{
list[i]=n%10;
n=n/10;
}
for(int i=0;i<5;i++)
{
for(int j=i+1;j<5;j++)
{
if(list[i]==list[j]) return false;
}
}
return true;
}
UploadRoot=UploadRoot+"\Imageupload\" + fileNamel;得到相对路径并将其存到数据库中。