Common Java APIs and complexity analysis
1 String Common Methods
1.1 String’s Common Methods
Scanner scanner = new Scanner(System.in);
if (scanner.hasNext()) {
String currentLineString = scanner.next();
}
String addedString = 1 + 2 + "test" + 1 + 2 + 'a' + 3.4;
StringBuffer appendedStringBuffer = new StringBuffer().append(1 + 2).append("test").append(1).append(2).append('a').append(3.4);
String[] splittedStringArray = "how-r-u".split("-");
String[] splittedStringArray2 = "how-r-u".split(":");
String joinedString = String.join(",", Arrays.asList("a", "bb", "cd"));
String joinedString2 = String.join(",", new String[]{"a", "b"});
StringJoiner stringJoiner = new StringJoiner(",", "Prefix:", ":Suffix");
String outputtedStringJoiner = stringJoiner.add("a").add("b").add("c").toString();
boolean ifContains = "abcd".contains("ab");
boolean isStartWithCat = "CatVSDog".startsWith("Cat");
boolean isEndWithCat = "CatVSDog".endsWith("Dog");
boolean isEqual = "abc".equals("abc");
boolean isEqualIgnoringCase = "abc".equalsIgnoreCase("aBc");
String substring1ToEnd = "abcd".substring(1);
String substring1To3 = "abcd".substring(1,3);
String stringToLowerCase = "abCD".toLowerCase();
String stringToUpperCase = "abCD".toUpperCase();
int firstIndexOfString = "abcabcab".indexOf("ab");
int lastIndexOfString = "abcabcab".lastIndexOf("ab");
int firstIndexOfSubstring = "abcabcab".indexOf("ab",1);
int lastIndexOfSubstring = "abcabcab".lastIndexOf("ab",5);
int positiveCompareToString = "cde".compareTo("ab");
int negativeCompareToString = "aab".compareTo("acde");
int negativeCompareToString2 = "ab".compareTo("abcde");
int negativeCompareToString3 = "ab".compareTo(null);
int zeroCompareToString = "ab".compareTo("ab");
1.2 StringBuffer and StringBuilder
StringBuilder stringBuilder = new StringBuilder();
stringBuilder.append(new StringBuffer("abc")).append('1').append(2.3);
stringBuilder.deleteCharAt(1);
stringBuilder.delete(1,2);
stringBuilder.insert(1, 'd');
stringBuilder.reverse();
stringBuilder.setCharAt(1, 'e');
stringBuilder.replace(1, 3, "xyz");
String transferToString = stringBuilder.toString();
int compareResult = stringBuilder.compareTo(new StringBuilder("ab"));
int firstIndexOfSubstring = stringBuilder.indexOf("a");
int lastIndexOfSubstring = stringBuilder.lastIndexOf("a");
int stringBuilderLength = stringBuilder.length();
char charAtStringBuilder = stringBuilder.charAt(2);
String substring = stringBuilder.substring(2);
1.3 String’s Mutual Type Conversion
String integerString = String.valueOf(123);
String decimalString = String.valueOf(12.34);
String booleanString = String.valueOf(true);
String characterString = String.valueOf('a');
String characterArrayString = new String(new char[]{'a', 'b', 'c'});
char characterAtAString = "string".charAt(2);
char[] characterArray = "string".toCharArray();
int parsedInteger = Integer.parseInt("123");
boolean parsedBoolean = Boolean.parseBoolean("true");
String bigIntegerAndBigDecimalString = new BigInteger("1").toString() + new BigDecimal("0.1").toString() + new BigDecimal("0.1").toPlainString();
1.4 String’s Regex Methods
String replacedString = "abbbc".replace("bb","o");
String firstReplacedString = "abbbbc".replaceFirst("bb","o");
String replacedAllString = "abbbbbc".replaceAll("(.)\\1\\1*","$1$1");
boolean isRegexMatched = Pattern.matches("^\\w{2}$", "ab");
1.5 Remove leading and trailing space去除空白字符
String trimmedSentence = " Hi, my friend! ".trim();
String stripedSentence = " How are you? ".strip();
System.out.println("".trim());
System.out.println("".strip());
boolean isStringEmpty = "".isEmpty();
boolean isStringBlank = " ".isBlank();
String stringAfterReplaceBlankAsEmpty = " How are you? ".replace(" ", "");
String stringAfterReplaceAllBlankAsEmpty = " How r u ".replaceAll("\\s+", "");
2 Math, Operator and Big Numbers
2.1 Java Binary Operator /
and%
2.1.1 Java Binary Operator /
According to Oracle Java SE Specifications - 15.17.2. Division Operator /. The binary operator /
performs division, producing the quotient of its operands. The left-hand operand is the dividend and the right-hand operand is the divisor.
The quotient produced by the divisible symbol /
is the result of normal division followed by subtracting the decimal part, rather than the result of rounding. The positive and negative sign of the quotient is determined by both signs of the dividend and the divisor.
整除符号/
产生的最终数值是普通除法后再去除小数位的数值,而不是四舍五入的结果。整除符号/
产生的最终数值的正负符号由除数和被除数共同决定。
System.out.println(4 / 3);
System.out.println(5 / 3);
System.out.println(-4 / 3);
System.out.println(-5 / 3);
System.out.println(4 / -3);
System.out.println(5 / -3);
System.out.println(-4 / -3);
System.out.println(-5 / -3);
magnitude /ˈmæɡnɪtuːd/ n量级,程度(在下文中可以近似理解为绝对值)
Absolute value, Measure of the magnitude of a real number, complex number, or vector.
There is one special case that does not satisfy this rule: if the dividend is the negative integer of largest possible magnitude for its type, and the divisor is -1
, then integer overflow occurs and the result is equal to the dividend. Despite the overflow, no exception is thrown in this case.
System.out.println(Integer.MIN_VALUE/-1);
2.1.2 Java Binary Operator %
According to Oracle Java SE Specifications - 15.17.3. Remainder Operator %
. The binary %
operator is said to yield the remainder of its operands from an implied division; the left-hand operand is the dividend and the right-hand operand is the divisor.
It follows from this rule that the result of the remainder operation can be negative only if the dividend is negative, and can be positive only if the dividend is positive.
模运算符号%
产生的最终数值是被模数和模数的绝对值取模后再复用模数正负号的数值,即其运算结果的符号始终和被模数的符号一致。
System.out.println(4 % 3);
System.out.println(5 % 3);
System.out.println(-4 % 3);
System.out.println(-5 % 3);
System.out.println(4 % -3);
System.out.println(5 % -3);
System.out.println(-4 % -3);
System.out.println(-5 % -3);
2.2 Java Array, String and Collection’s Length
.length
is for getting the length of an array, such as int arrayLength = new int[2].length;
..length()
is for getting the length of a string, such as int stringLength = "string".length();
..size()
is for getting the length of a collection, such as int collectionLength = new ArrayList<>(Arrays.asList(1, 2)).size();
. int size();
is one of Java Collection
Interface’s interface methods.
int arrayLength = (new int[]{1, 2, 3}).length;
String[][][] multiDimensionalArray = new String[4][5][6];
int firstDimensionArrayLength = multiDimensionalArray.length;
int secondDimensionArrayLength = multiDimensionalArray[0].length;
int thirdDimensionArrayLength = multiDimensionalArray[0][0].length;
int stringLength = "string".length();
int collectionLength = new ArrayList<>(Arrays.asList(1, 2)).size();
2.3 Math Utility
double ceilingDoubleValue = Math.ceil(-1.99);
double flooredDoubleValue = Math.floor(-1.01);
long roundedPositiveValue = Math.round(1.49) + Math.round(1.5) + Math.round(1.51);
long roundedNegativeValue = Math.round(-1.49) + Math.round(-1.5) + Math.round(-1.51);
System.out.println(roundedPositiveValue + " "+roundedNegativeValue);
double poweredValue = Math.pow(1.2, 3.4);
double squareRoot = Math.sqrt(9.0);
double absoluteValue = Math.abs(-1.5);
double minAndMaxValue = Math.min(1, 2) + Math.max(2.5, 4.5);
double randomDecimalValue = Math.random();
new Random().nextInt();
new Random().nextInt(10);
new Random().nextDouble();
2.4 BigInteger
BigInteger bigInteger = BigInteger.valueOf(-12);
bigInteger = new BigInteger("-000012");
double bigIntegerValues = bigInteger.floatValue() + bigInteger.doubleValue() + bigInteger.byteValueExact() + bigInteger.intValueExact() + bigInteger.longValueExact() + bigInteger.shortValueExact();
double doubleConvertedByBigInteger = BigInteger.valueOf(9999999999L).doubleValue();
int intConvertedByBigInteger = BigInteger.valueOf(Integer.MAX_VALUE + 1L).intValue();
try {
int intExactConvertedByBigInteger = BigInteger.valueOf(Integer.MAX_VALUE + 1L).intValueExact();
new BigInteger("00123.4a5");
} catch (Exception ignored) {
}
int comparedResult = bigInteger.compareTo(new BigInteger("0"));
BigInteger addedBigInteger = bigInteger.add(new BigInteger(String.valueOf(10)));
BigInteger subtractedBigInteger = bigInteger.subtract(new BigInteger("1"));
BigInteger multipliedBigInteger = bigInteger.multiply(new BigInteger("2"));
BigInteger dividedBigInteger = bigInteger.divide(new BigInteger("5"));
BigInteger remainedBigInteger = bigInteger.remainder(new BigInteger("7"));
BigInteger absoluteBigInteger = bigInteger.abs();
BigInteger exponentialBigInteger = bigInteger.pow(2);
BigInteger squareRootedBigInteger = new BigInteger("16").sqrt();
2.5 BigDecimal
String bigDecimalString = new BigDecimal(0.125).toString();
String bigDecimalPlainString = new BigDecimal(0.1).toPlainString();
BigDecimal bigDecimal = new BigDecimal("0.125");
int scaleSum = new BigDecimal("-0.001").scale() + new BigDecimal("1200.0100").scale();
int positiveScale = new BigDecimal("12000.0100").stripTrailingZeros().scale();
int negativeScale = new BigDecimal("12000.0000").stripTrailingZeros().scale();
new BigDecimal("1234.5678").setScale(2);
new BigDecimal("1234.5678").setScale(2, RoundingMode.HALF_EVEN);
new BigDecimal("1234.5678").setScale(-2, RoundingMode.CEILING);
new BigDecimal("1234.5678").setScale(6);
BigDecimal addedBigDecimal = new BigDecimal("1.23").add(new BigDecimal("4.5670000"));
BigDecimal subtractedBigDecimal = new BigDecimal("1.23").subtract(new BigDecimal("4.5670000"));
BigDecimal multipliedBigDecimal = new BigDecimal("1.23").multiply(new BigDecimal("2.0000"));
BigDecimal dividedIntegralBigDecimal = new BigDecimal("35.99").divideToIntegralValue(new BigDecimal("2.00000"));
BigDecimal dividedBigDecimalRemainder = new BigDecimal("35.99").remainder(new BigDecimal("2"));
BigDecimal dividedBigDecimal2 = new BigDecimal("2.46").divide(new BigDecimal("2.0000"), 1, RoundingMode.HALF_EVEN);
boolean isBigDecimalEqual = new BigDecimal("1.2").equals(new BigDecimal("1.20"));
boolean isBigDecimalEqual2 = new BigDecimal("1.2").equals(new BigDecimal("1.2"));
boolean isBigDecimalEqual3 = new BigDecimal("1.2").equals(new BigDecimal("1.20").stripTrailingZeros());
int isBigDecimalSameValue = new BigDecimal("1.2").compareTo(new BigDecimal("1.20"));
int isBigDecimalBigger = new BigDecimal("1.3").compareTo(new BigDecimal("1.20"));
BigDecimal poweredBigDecimal = new BigDecimal(-2).pow(3);
BigDecimal absoluteBigDecimal = new BigDecimal(-2).abs();
int intValue = new BigDecimal("12.3").intValue();
int intValueExact = new BigDecimal("1.2").intValueExact();
BigInteger convertedBigInteger = new BigDecimal("12.3").toBigInteger();
BigInteger convertedExactBigInteger = new BigDecimal("1.2").toBigIntegerExact();
3 Collections
3.1 List and Set(ArrayList and HashSet)
Collection<Integer> collection = new ArrayList<>();
int collectionSize = collection.size();
boolean isAddSuccessfully = collection.add(1);
boolean isAllAddSuccessfully = collection.addAll(Arrays.asList(1, 1, 2));
boolean isRemovedSuccessfully = collection.remove(1);
collection.addAll(Arrays.asList(1, 2, 2, 4));
boolean isAllRemovedSuccessfully = collection.removeAll(Arrays.asList(2, 3));
boolean isEmpty = collection.isEmpty();
boolean isContain = collection.contains(1);
boolean isAllContain = collection.containsAll(Arrays.asList(1, 2));
boolean isAllContain2 = collection.containsAll(Arrays.asList(1, 4));
Object[] objectArray = collection.toArray();
Integer[] intArray = collection.toArray(new Integer[0]);
collection.clear();
ArrayList<Integer> arrayList = new ArrayList<>();
arrayList.add(1);
arrayList.add(2);
arrayList = new ArrayList<>(new HashSet<>());
arrayList = new ArrayList<>() {{
this.add(1);
add(2);
}};
arrayList.add(3);
arrayList = new ArrayList<>(Arrays.asList(1, 2, 3));
arrayList.add(4);
arrayList = new ArrayList<>(Collections.nCopies(5, 666));
arrayList.add(5);
arrayList = new ArrayList<>(List.of(1, 2, 3));
arrayList.add(6);
List<Integer> immutableArrayList = Arrays.asList(1, 2, 3);
immutableArrayList.add(4);
immutableArrayList = Collections.nCopies(5, 666);
immutableArrayList.add(6);
immutableArrayList = List.of(1, 2, 3);
immutableArrayList.add(7);
arrayList = new ArrayList<Integer>(Arrays.asList(1, 2, 3));
Integer specifiedIndexElement = arrayList.get(-1);
specifiedIndexElement = arrayList.get(2);
arrayList.add(4);
arrayList.add(4, 5);
arrayList.add(0, 0);
arrayList.addAll(6, Arrays.asList(6));
arrayList.remove(5);
arrayList.set(5, 5);
int indexOfElement3 = arrayList.indexOf(3);
int lastIndexOfElement2 = arrayList.lastIndexOf(2);
List<Integer> subArrayList = arrayList.subList(0, 2);
HashSet<String> hashSet = new HashSet<>() {{
add("element1");
add("element2");
}};
hashSet = new HashSet<>(Arrays.asList("element1", "element2"));
hashSet = new HashSet<>(Set.of("element1", "element2"));
hashSet.add("element3");
Set<String> immutableSet = Set.of("element1", "element2");
immutableSet.add("element3");
3.2 Map and HashMap
HashMap<String, String> hashMap = new HashMap<>() {{
put("key1", "value1");
put("key2", "value2");
}};
String hashMapString = hashMap.toString();
hashMap.put("key3", "value3");
hashMap = new HashMap<>(Map.of("key1", "value1", "key2", "value2"));
Map<String, String> immutableMap = Map.of("key1", "value1", "key2", "value2");
hashMap.clear();
boolean isEmpty = hashMap.isEmpty();
String previousAssociatedValue = hashMap.put("key1", "value1");
hashMap.putAll(Map.of("key2", "value2", "key3", "value3"));
int hashMapSize = hashMap.size();
String associatedValue = hashMap.get("key2");
String removedPreviousAssociatedValue = hashMap.remove("key3");
Set<String> hashMapKeySet = hashMap.keySet();
Collection<String> hashMapValueCollection = hashMap.values();
Set<Map.Entry<String, String>> hashMapEntrySet = hashMap.entrySet();
boolean ifContainsKey = hashMap.containsKey("key1");
boolean ifContainsValue = hashMap.containsValue("value1");
hashMap.replace("key1", "new_value1");
4 Utility
4.1 java.util.Arrays
4.1.1 Mind Mapping of Java Array’s common methods
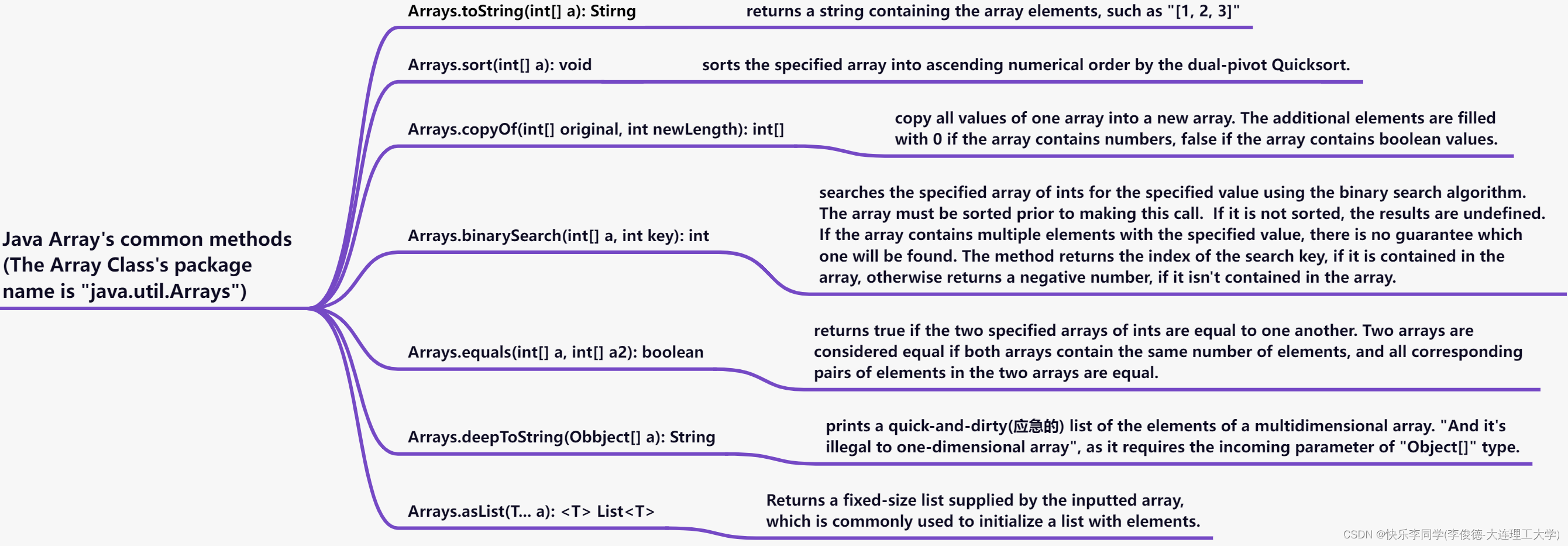
4.1.2 Practical Code Demo
package com.test;
import java.util.Arrays;
public class JavaArrayUsage {
static int[] nullIntArray;
public static void main(String[] args) {
System.out.println(nullIntArray);
int tempArray[] = null;
int[] emptyIntArray = new int[0];
int[] emptyIntArray2 = new int[]{};
System.out.println(Arrays.toString(emptyIntArray) + " " + emptyIntArray.length);
System.out.println(Arrays.toString(emptyIntArray2) + " " + emptyIntArray2.length);
int[] intArray = {1, 2, 3};
int[] intArray2 = new int[]{1, 2, 3};
int[] intArray3 = new int[3];
int arrayLength = 3;
int[] intArray4 = new int[arrayLength];
for (int i = 0; i < intArray.length; i++) {
System.out.println(intArray[i]);
}
for (int j : intArray) {
System.out.println(j);
}
System.out.println(intArray);
System.out.println(Arrays.toString(intArray));
int[] luckyNumbers = {1, 2};
luckyNumbers = new int[]{1, 3};
int[] copiedLuckyNumbers = Arrays.copyOf(luckyNumbers, luckyNumbers.length * 2);
System.out.println(Arrays.toString(copiedLuckyNumbers));
int[] unsortedArray = {4, 3, 5, 2};
Arrays.sort(unsortedArray);
System.out.println(Arrays.toString(unsortedArray));
int[] intArray7 = {1, 2, 3, 5};
int searchedNumber3Index = Arrays.binarySearch(intArray7, 3);
System.out.println("the index of value 3 in array [1, 2, 3, 5] is " + searchedNumber3Index);
int searchedNumber4Index = Arrays.binarySearch(intArray7, 4);
System.out.println("the index of value 4 in array [1, 2, 3, 5] is " + searchedNumber4Index);
int[] intArray8 = {1, 3, 5};
int[] intArray9 = {1, 3, 5};
System.out.println(intArray8 == intArray9);
System.out.println(Arrays.equals(intArray8, intArray9));
int[][] twoDimenArray = {
{1, 3, 2},
{4, 8, 6}
};
for (int[] eachRow : twoDimenArray) {
for (int eachItem : eachRow) {
System.out.print(eachItem + " ");
}
}
System.out.println(Arrays.deepToString(twoDimenArray));
int[][] raggedArray = new int[3][];
int[] firstRow = raggedArray[0];
raggedArray[0] = new int[]{1, 4};
raggedArray[2] = new int[]{5, 7, 9};
System.out.println(Arrays.deepToString(raggedArray));
ArrayList<Integer> integerArrayList = new ArrayList<>(Arrays.asList(1, 2));
System.out.println(integerArrayList);
}
}
4.2 Character
Character newLineCharacter = new Character('\n');
boolean isLetter = Character.isLetter('a');
boolean isDigit = Character.isDigit('1');
boolean isWhitespace = Character.isWhitespace(' ');
boolean isUpperCase = Character.isUpperCase('A');
boolean isLowerCase = Character.isLowerCase('a');
char upperCaseLetter = Character.toUpperCase('a');
Character lowerCaseLetter = Character.toLowerCase('A');
String characterString = Character.toString('s');
4.3 Collections
ArrayList<Integer> arrayList = new ArrayList<>(Arrays.asList(1, 2));
Collections.addAll(arrayList, Arrays.asList(4, 5).toArray(new Integer[0]));
Collections.addAll(arrayList, 4, 5);
Collections.addAll(arrayList, new Integer[]{4, 5});
Collections.addAll(new HashSet<>(), 1, 2);
Collections.reverse(arrayList);
Collections.swap(arrayList, 1, 2);
int sum = Collections.min(arrayList) + Collections.max(arrayList);
boolean isAtLeastReplaceOnce = Collections.replaceAll(arrayList, 2, 6);
Collections.shuffle(arrayList);
Collections.sort(arrayList);
Collections.sort(arrayList, Collections.reverseOrder());
4.4 Iterable and Iterator
4.4.1 Definitions and difference
java.lang.Iterable represents a collection that can be iterated over using a for-each loop. When implementing an Iterable, we need to override the iterator() method.
public interface Iterable<T> {
Iterator<T> iterator();
default void forEach(Consumer<? super T> action) {
Objects.requireNonNull(action);
for (T t : this) {
action.accept(t);
}
}
}
java.util.Iterator represents an interface that can be used to iterate over a collection. When implementing an Iterator, we need to override the hasNext() and next() methods.
package java.util;
public interface Iterator<E> {
boolean hasNext();
E next();
default void remove() {
throw new UnsupportedOperationException("remove");
}
}
4.4.2 Examples from Source Codes
java.util.Collection
extends Iterable
, so ArrayList and HashSet implemented the interface method Iterable.iterator()
. Here is the implementation of Iterable.iterator()
from ArrayList:
public class ArrayList<E> extends AbstractList<E>
implements List<E>, RandomAccess, Cloneable, java.io.Serializable
{
public Iterator<E> iterator() {
return new Itr();
}
private class Itr implements Iterator<E> {
int cursor;
int lastRet = -1;
int expectedModCount = modCount;
Itr() {}
public boolean hasNext() {
return cursor != size;
}
@SuppressWarnings("unchecked")
public E next() {
checkForComodification();
int i = cursor;
if (i >= size)
throw new NoSuchElementException();
Object[] elementData = ArrayList.this.elementData;
if (i >= elementData.length)
throw new ConcurrentModificationException();
cursor = i + 1;
return (E) elementData[lastRet = i];
}
public void remove() {
if (lastRet < 0)
throw new IllegalStateException();
checkForComodification();
try {
ArrayList.this.remove(lastRet);
cursor = lastRet;
lastRet = -1;
expectedModCount = modCount;
} catch (IndexOutOfBoundsException ex) {
throw new ConcurrentModificationException();
}
}
@Override
public void forEachRemaining(Consumer<? super E> action) {
}
final void checkForComodification() {
if (modCount != expectedModCount)
throw new ConcurrentModificationException();
}
}
}
4.4.3 Common Usage
ArrayList<Integer> arrayList = new ArrayList<>(Arrays.asList(1, 2));
for (int listIndex = 0; listIndex < arrayList.size(); listIndex++) {
Integer listElement = arrayList.get(listIndex);
}
for (Integer listElement : arrayList) {
Integer tempListElement = listElement;
}
Integer[] arrayListArray = arrayList.toArray(new Integer[0]);
for (Iterator<Integer> iterator = arrayList.iterator(); iterator.hasNext(); ) {
Integer listElement = iterator.next();
iterator.remove();
}
HashSet<Integer> hashSet = new HashSet<>(Arrays.asList(1, 2));
for (Integer setElement : hashSet) {
Integer tempSetElement = setElement;
}
Integer[] setArray = hashSet.toArray(new Integer[0]);
for (Iterator<Integer> iterator = hashSet.iterator(); iterator.hasNext(); ) {
Integer listElement = iterator.next();
iterator.remove();
}
HashMap<String, String> hashMap = new HashMap<>(Map.of("key1", "value1", "key2", "value2"));
for (Iterator<Map.Entry<String, String>> hashMapIterator = hashMap.entrySet().iterator(); hashMapIterator.hasNext(); ) {
Map.Entry<String, String> hashMapEntry = hashMapIterator.next();
String hashMapKey = hashMapEntry.getKey();
String hashMapValue = hashMapEntry.getValue();
hashMapIterator.remove();
}
Iterator<String> hashMapKeyIterator = hashMap.keySet().iterator();
Iterator<String> hashMapValueIterator = hashMap.values().iterator();
for (Map.Entry<String, String> hashMapEntry : hashMap.entrySet()) {
String hashMapKeyAndValue = hashMapEntry.getKey() + "=" + hashMapEntry.getValue();
}
hashMap.forEach((key, value) -> {
String hashMapKeyAndValue = key + "=" + value;
});
4.5 Comparable and Comparator
4.5.1 Definitions and difference
java.lang.Comparable
interface imposes a total ordering on the objects of each class that implements it. This ordering is referred to as the class’s natural ordering, and the class’s compareTo method is referred to as its natural comparison method. Lists (and arrays) of objects that implement this interface can be sorted automatically by Collections. sort (and Arrays.sort). Objects that implement this interface can be used as keys in a sorted map or as elements in a sorted set, without the need to specify a comparator.
public interface Comparable<T> {
public int compareTo(T o);
}
java.util.Comparator
is a comparison function, which imposes a total ordering on some collection of objects. Comparators can be passed to a sort method (such as Collections.sort or Arrays.sort) to allow precise control over the sort order. Comparators can also be used to control the order of certain data structures (such as sorted sets or sorted maps), or to provide an ordering for collections of objects that don’t have a natural ordering.
public interface Comparator<T> {
int compare(T o1, T o2);
boolean equals(Object obj);
}
4.5.2 Examples from Source Codes
public final class Integer extends Number implements Comparable<Integer> {
public int compareTo(Integer anotherInteger) {
return compare(this.value, anotherInteger.value);
}
public static int compare(int x, int y) {
return (x < y) ? -1 : ((x == y) ? 0 : 1);
}
}
public class Collections {
public static <T> Comparator<T> reverseOrder() {
return (Comparator<T>) ReverseComparator.REVERSE_ORDER;
}
private static class ReverseComparator implements Comparator<Comparable<Object>>, Serializable {
static final ReverseComparator REVERSE_ORDER = new ReverseComparator();
public int compare(Comparable<Object> c1, Comparable<Object> c2) {
return c2.compareTo(c1);
}
}
}
4.5.3 Common Usage
public static void comparableAndComparatorUsage() {
Integer[] integerArray = new Integer[]{1, 3, 2};
Arrays.sort(integerArray);
Arrays.sort(integerArray, Collections.reverseOrder());
Arrays.sort(integerArray, new Comparator<Integer>() {
@Override
public int compare(Integer o1, Integer o2) {
return o1.compareTo(o2);
}
});
ArrayList<Integer> arrayList = new ArrayList<>(Arrays.asList(1, 3, 2));
Collections.sort(arrayList);
Collections.sort(arrayList, Collections.reverseOrder());
Collections.sort(arrayList, new Comparator<Integer>() {
@Override
public int compare(Integer o1, Integer o2) {
return (o1 < o2) ? -1 : ((o1.equals(o2)) ? 0 : 1);
}
});
ArrayList<ComparableBook> comparableBookArrayList = new ArrayList<>() {{
add(new ComparableBook("Book1", 10));
add(new ComparableBook("Book3", 30));
add(new ComparableBook("Book2", 20));
}};
Collections.sort(comparableBookArrayList);
Arrays.sort(comparableBookArrayList.toArray());
ArrayList<Book> bookArrayList = new ArrayList<>() {{
add(new Book("Book1", 10));
add(new Book("Book3", 30));
add(new Book("Book2", 20));
}};
Collections.sort(bookArrayList, new Comparator<Book>() {
@Override
public int compare(Book book1, Book book2) {
if (book1.price > book2.price) {
return 1;
} else if (book1.price < book2.price) {
return -1;
} else {
return 0;
}
}
});
}
public static class Book {
public String name;
public double price;
public Book(String name, double price) {
this.name = name;
this.price = price;
}
@Override
public String toString() {
return "Book{" + "name='" + name + '\'' + ", price=" + price + '}';
}
}
public static class ComparableBook implements Comparable<ComparableBook> {
public String name;
public double price;
public ComparableBook(String name, double price) {
this.name = name;
this.price = price;
}
@Override
public int compareTo(ComparableBook comparedBook) {
if (this.price > comparedBook.price) {
return 1;
} else if (this.price == comparedBook.price) {
return 0;
} else {
return -1;
}
}
@Override
public String toString() {
return "ComparableBook{" + "name='" + name + '\'' + ", price=" + price + '}';
}
}
References:
CSDN-LeetCode和牛客网的编程题中常用的Java方法和API
简书-java 整数除法
廖雪峰-Java核心类-字符串和编码
掘金-从String中移除空白字符的多种方式!?
简书-code point & code unit
简书-Java中List与数组互相转换
掘金-《java基础》集合类的toArray方法你会用吗?
JianShu-Java常用类笔记
Regex Reference:
正则表达式自学网站:https://regexlearn.com/
正则表达式30分钟入门教程:https://deerchao.cn/tutorials/regex/regex.htm
正则表达式可视化及实例代码:http://tool.rbtree.cn/
正则表达式可视化解析树及动画:https://blog.robertelder.org/regular-expression-visualizer/
正则表达式时间复杂度为什么为O(n):StackOverFlow-What’s the Time Complexity of Average Regex algorithms?
BigDecimal Reference:
LiaoXueFeng-BigDecimal
简书-BigDecimal用法
ZhiHu-BigDecimal的浮点数运算能保证精度的原理是什么?
StackOverFlow-Setting scale to a negative number with BigDecimal
StackOverFlow-Why scale() method of bigDecimal returning negative value when combining with stripTrailingZeros() method?
Collection Reference:
CSDN-Java创建初始化List集合的几种方式
ZhiHu-ArrayList源码方法小结(一) 常用方法
CSDN-java-List集合初始化的几种方式与一些常用操作-持续更新
CSDN-java常用知识点整理
Java工具类Collections方法详解
CSDN-Java中的Collections类
WeChat-HashMap 的 7 种遍历方式与性能分析!(强烈推荐)
ZhiHu-HashMap和HashSet有多少种遍历方式
CSDN-遍历Arraylist的几种方法
ZhiHu-Java 中iterator和iterable的关系是怎样的?有何意义?
本文内容由网友自发贡献,版权归原作者所有,本站不承担相应法律责任。如您发现有涉嫌抄袭侵权的内容,请联系:hwhale#tublm.com(使用前将#替换为@)