对一些特殊的应用需要对纹理坐标进行处理,主要包括纹理坐标自动生成和纹理坐标变换。下图显示了纹理坐标的来源、处理过程以及到达光栅处理器的过程。
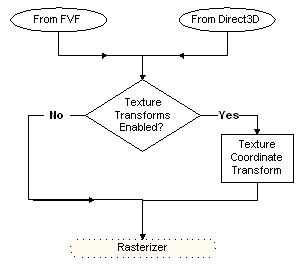
纹理坐标自动生成
在Direct3D程序中,不仅可以在模型载入阶段或渲染阶段指定物体的纹理坐标,还可以通过Direct3D渲染引擎自动生成纹理坐标,用于诸如环境映射等特殊的视觉效果。与手动设置纹理坐标相比,纹理坐标自动生成在Direct3D坐标 变换和光照流水线中完成,执行速度更快。
Direct3D系统可以使用经过变换的摄像机空间顶点位置坐标、法线信息来生成纹理坐标。如果使用纹理坐标自动生成,那么在顶点中就可以不用包含纹理坐标数据,从而可以降低图形渲染时的数据传输量。纹理坐标自动生成主要用于产生一些特殊效果,在大多数情况下还是手工为每个顶点指定纹理坐标。
通过调用SetTextureStageState()并将第二个参数设置为D3DTSS_TEXCOORDINDEX来控制Direct3D系统如何自动生成纹理坐标。
D3DTSS_TEXCOORDINDEX
-
Index of the texture coordinate set to use with this texture stage. You can specify up to eight sets of texture coordinates per vertex. If a vertex does not include a set of texture coordinates at the specified index, the system defaults to the u and v coordinates (0,0).
When rendering using vertex shaders, each stage's texture coordinate index must be set to its default value. The default index for each stage is equal to the stage index. Set this state to the zero-based index of the coordinate set for each vertex that this texture stage uses.
Additionally, applications can include, as logical OR with the index being set, one of the constants to request that Direct3D automatically generate the input texture coordinates for a texture transformation. For a list of all the constants, see D3DTSS_TCI.
With the exception of D3DTSS_TCI_PASSTHRU, which resolves to zero, if any of the following values is included with the index being set, the system uses the index strictly to determine texture wrapping mode. These flags are most useful when performing environment mapping.
其中第三个参数可以设为下列列表中的成员:
D3DTSS_TCI
Driver texture coordinate capability flags.
#define |
Value |
Description |
D3DTSS_TCI_PASSTHRU
|
0x00000000L
|
Use the specified texture coordinates contained within the vertex format. This value resolves to zero.
|
D3DTSS_TCI_CAMERASPACENORMAL
|
0x00010000L
|
Use the vertex normal, transformed to camera space, as the input texture coordinates for this stage's texture transformation.
|
D3DTSS_TCI_CAMERASPACEPOSITION
|
0x00020000L
|
Use the vertex position, transformed to camera space, as the input texture coordinates for this stage's texture transformation.
|
D3DTSS_TCI_CAMERASPACEREFLECTIONVECTOR
|
0x00030000L
|
Use the reflection vector, transformed to camera space, as the input texture coordinate for this stage's texture transformation. The reflection vector is computed from the input vertex position and normal vector.
|
D3DTSS_TCI_SPHEREMAP
|
0x00040000L
|
Use the specified texture coordinates for sphere mapping.
|
These constants are used by D3DTSS_TEXCOORDINDEX.
D3DTSS_TEXCOORDINDEX用于指定特定纹理层使用顶点中的第几组纹理坐标,但如果指定了上表中的成员值,Direct3D将忽略顶点中的纹理坐标,转而使用自动生成的纹理坐标。
D3DTSS_TEXTURETRANSFORMFLAGS用来控制生成的纹理坐标的输出,在大多数情况下纹理坐标是二维的,即将D3DTSS_TEXTURETRANSFORMFLAGS设置为D3DTTFF_COUNT2。但当绘制线段或三维纹理时,纹理坐标可能是一维或三维的。
D3DTSS_TEXTURETRANSFORMFLAGS
-
Member of the D3DTEXTURETRANSFORMFLAGS enumerated type that controls the transformation of texture coordinates for this texture stage. The default value is D3DTTFF_DISABLE.
输出的纹理坐标维数由枚举类型D3DTSS_TEXTURETRANSFORMFLAGS指定,其定义如下:
Defines texture coordinate transformation values.
typedef enum D3DTEXTURETRANSFORMFLAGS
{
D3DTTFF_DISABLE = 0,
D3DTTFF_COUNT1 = 1,
D3DTTFF_COUNT2 = 2,
D3DTTFF_COUNT3 = 3,
D3DTTFF_COUNT4 = 4,
D3DTTFF_PROJECTED = 256,
D3DTTFF_FORCE_DWORD = 0x7fffffff,
} D3DTEXTURETRANSFORMFLAGS, *LPD3DTEXTURETRANSFORMFLAGS;
Constants
D3DTTFF_DISABLE
-
Texture coordinates are passed directly to the rasterizer.
D3DTTFF_COUNT1
-
The rasterizer should expect 1D texture coordinates. This value is used by fixed function vertex processing; it should be set to 0 when using a programmable vertex shader.
D3DTTFF_COUNT2
-
The rasterizer should expect 2D texture coordinates. This value is used by fixed function vertex processing; it should be set to 0 when using a programmable vertex shader.
D3DTTFF_COUNT3
-
The rasterizer should expect 3D texture coordinates. This value is used by fixed function vertex processing; it should be set to 0 when using a programmable vertex shader.
D3DTTFF_COUNT4
-
The rasterizer should expect 4D texture coordinates. This value is used by fixed function vertex processing; it should be set to 0 when using a programmable vertex shader.
D3DTTFF_PROJECTED
-
This flag is honored by the fixed function pixel pipeline, as well as the programmable pixel pipeline in versions ps_1_1 to ps_1_3. When texture projection is enabled for a texture stage, all four floating point values must be written to the corresponding texture register. Each texture coordinate is divided by the last element before being passed to the rasterizer. For example, if this flag is specified with the D3DTTFF_COUNT3 flag, the first and second texture coordinates are divided by the third coordinate before being passed to the rasterizer.
D3DTTFF_FORCE_DWORD
-
Forces this enumeration to compile to 32 bits in size. Without this value, some compilers would allow this enumeration to compile to a size other than 32 bits. This value is not used.
Remarks
Texture coordinates can be transformed using a 4 x 4 matrix before the results are passed to the rasterizer. The texture coordinate transforms are set by calling IDirect3DDevice9::SetTextureStageState, and by passing in the D3DTSS_TEXTURETRANSFORMFLAGS texture stage state and one of the values from D3DTEXTURETRANSFORMFLAGS. For more information about texture transforms, see Texture Coordinate Transformations (Direct3D 9).
首先,我们定义顶点结构和格式:
struct sCustomVertex
{
float x, y, z;
};
#define D3DFVF_CUSTOM_VERTEX D3DFVF_XYZ
接着生成顶点数据,顶点数据中没有包含纹理坐标:
// create vertex buffer and fill data
sCustomVertex vertices[] =
{
{ -1.0f, -1.0f, 0.0f},
{ -1.0f, 1.0f, 0.0f},
{ 1.0f, -1.0f, 0.0f},
{ 1.0f, 1.0f, 0.0f}
};
pd3dDevice->CreateVertexBuffer(sizeof(vertices), 0, D3DFVF_CUSTOM_VERTEX, D3DPOOL_MANAGED, &g_vertex_buffer, NULL);
void* ptr;
g_vertex_buffer->Lock(0, sizeof(vertices), (void**)&ptr, 0);
memcpy(ptr, vertices, sizeof(vertices));
g_vertex_buffer->Unlock();
然后让Direct3D自动生成纹理坐标:
// create texture coordinate using vertex position in camera space
pd3dDevice->SetTextureStageState(0, D3DTSS_TEXCOORDINDEX, D3DTSS_TCI_CAMERASPACEPOSITION);
pd3dDevice->SetTextureStageState(0, D3DTSS_TEXTURETRANSFORMFLAGS, D3DTTFF_COUNT2);
运行效果:
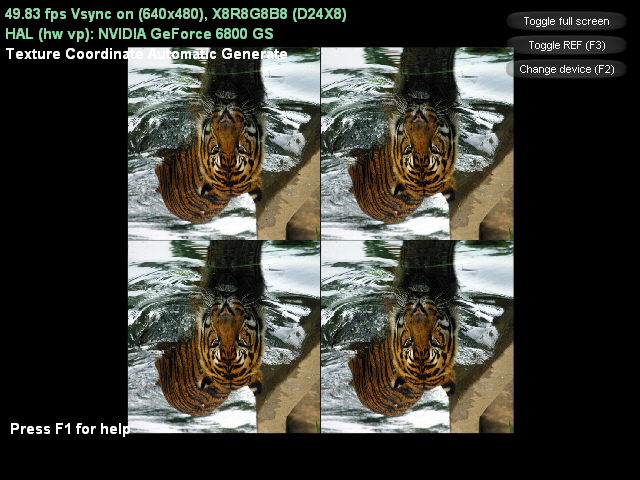
若设置D3DTSS_TEXCOORDINDEX为以下D3DTSS_TCI_CAMERASPACEREFLECTIONVECTOR,则效果为:
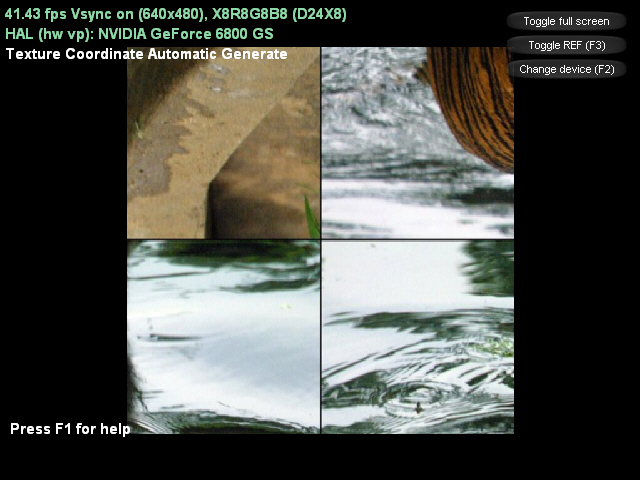
若设置D3DTSS_TEXCOORDINDEX为以下D3DTSS_TCI_SPHEREMAP,则效果为:
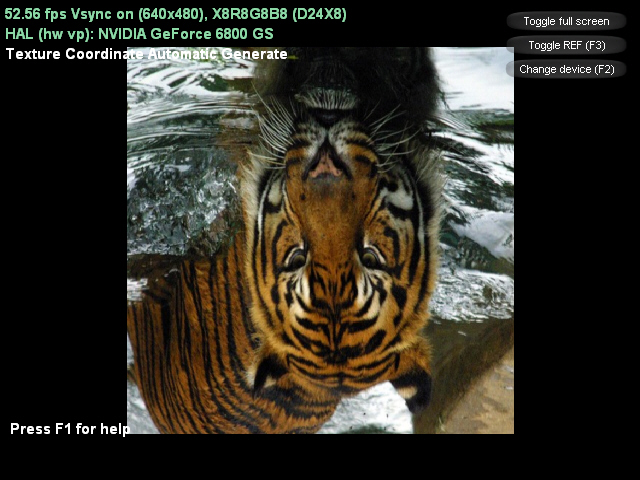
主程序:
#include
"
dxstdafx.h
"
#include
"
resource.h
"
#pragma warning(disable :
4127
4995
4996
)
#define
release_com(p) do { if(p) { (p)->Release(); (p) = NULL; } } while(0)
#define
IDC_TOGGLE_FULLSCREEN 1
#define
IDC_TOGGLE_REF 2
#define
IDC_CHANGE_DEVICE 3
struct
sCustomVertex
{
float
x, y, z;
};
#define
D3DFVF_CUSTOM_VERTEX D3DFVF_XYZ
const
D3DXCOLOR FONT_COLOR(
0.55f
,
0.85f
,
0.65f
,
1.0f
);
CDXUTDialogResourceManager g_dlg_resource_manager;
CD3DSettingsDlg g_settings_dlg;
CDXUTDialog g_button_dlg;
IDirect3DVertexBuffer9
*
g_vertex_buffer;
IDirect3DTexture9
*
g_texture;
ID3DXFont
*
g_font;
ID3DXSprite
*
g_text_sprite;
bool
g_show_help;
//
--------------------------------------------------------------------------------------
//
Rejects any devices that aren't acceptable by returning false
//
--------------------------------------------------------------------------------------
bool
CALLBACK IsDeviceAcceptable( D3DCAPS9
*
pCaps, D3DFORMAT AdapterFormat,
D3DFORMAT BackBufferFormat,
bool
bWindowed,
void
*
pUserContext )
{
//
Typically want to skip backbuffer formats that don't support alpha blending
IDirect3D9
*
pD3D
=
DXUTGetD3DObject();
if
( FAILED( pD3D
->
CheckDeviceFormat( pCaps
->
AdapterOrdinal, pCaps
->
DeviceType, AdapterFormat,
D3DUSAGE_QUERY_POSTPIXELSHADER_BLENDING, D3DRTYPE_TEXTURE, BackBufferFormat ) ) )
return
false
;
return
true
;
}
//
--------------------------------------------------------------------------------------
//
Before a device is created, modify the device settings as needed.
//
--------------------------------------------------------------------------------------
bool
CALLBACK ModifyDeviceSettings( DXUTDeviceSettings
*
pDeviceSettings,
const
D3DCAPS9
*
pCaps,
void
*
pUserContext )
{
//
If video card does not support hardware vertex processing, then uses sofaware vertex processing.
if
((pCaps
->
DevCaps
&
D3DDEVCAPS_HWTRANSFORMANDLIGHT)
==
0
)
pDeviceSettings
->
BehaviorFlags
=
D3DCREATE_SOFTWARE_VERTEXPROCESSING;
static
bool
is_first_time
=
true
;
if
(is_first_time)
{
is_first_time
=
false
;
//
if using reference device, then pop a warning message box.
if
(pDeviceSettings
->
DeviceType
==
D3DDEVTYPE_REF)
DXUTDisplaySwitchingToREFWarning();
}
return
true
;
}
//
--------------------------------------------------------------------------------------
//
Create any D3DPOOL_MANAGED resources here
//
--------------------------------------------------------------------------------------
HRESULT CALLBACK OnCreateDevice( IDirect3DDevice9
*
pd3dDevice,
const
D3DSURFACE_DESC
*
pBackBufferSurfaceDesc,
void
*
pUserContext )
{
HRESULT hr;
V_RETURN(g_dlg_resource_manager.OnCreateDevice(pd3dDevice));
V_RETURN(g_settings_dlg.OnCreateDevice(pd3dDevice));
D3DXCreateFont(pd3dDevice,
18
,
0
, FW_BOLD,
1
, FALSE, DEFAULT_CHARSET, OUT_DEFAULT_PRECIS, DEFAULT_QUALITY,
DEFAULT_PITCH
|
FF_DONTCARE, L
"
Arial
"
,
&
g_font);
V_RETURN(D3DXCreateTextureFromFile(pd3dDevice, L
"
texture.jpg
"
,
&
g_texture));
//
create vertex buffer and fill data
sCustomVertex vertices[]
=
{
{
-
1.0f
,
-
1.0f
,
0.0f
},
{
-
1.0f
,
1.0f
,
0.0f
},
{
1.0f
,
-
1.0f
,
0.0f
},
{
1.0f
,
1.0f
,
0.0f
}
};
pd3dDevice
->
CreateVertexBuffer(
sizeof
(vertices),
0
, D3DFVF_CUSTOM_VERTEX, D3DPOOL_MANAGED,
&
g_vertex_buffer, NULL);
void
*
ptr;
g_vertex_buffer
->
Lock(
0
,
sizeof
(vertices), (
void
**
)
&
ptr,
0
);
memcpy(ptr, vertices,
sizeof
(vertices));
g_vertex_buffer
->
Unlock();
return
S_OK;
}
//
--------------------------------------------------------------------------------------
//
Create any D3DPOOL_DEFAULT resources here
//
--------------------------------------------------------------------------------------
HRESULT CALLBACK OnResetDevice( IDirect3DDevice9
*
pd3dDevice,
const
D3DSURFACE_DESC
*
pBackBufferSurfaceDesc,
void
*
pUserContext )
{
HRESULT hr;
V_RETURN(g_dlg_resource_manager.OnResetDevice());
V_RETURN(g_settings_dlg.OnResetDevice());
V_RETURN(g_font
->
OnResetDevice());
V_RETURN(D3DXCreateSprite(pd3dDevice,
&
g_text_sprite));
//
set dialog position and size
g_button_dlg.SetLocation(pBackBufferSurfaceDesc
->
Width
-
170
,
0
);
g_button_dlg.SetSize(
170
,
170
);
//
setup world matrix
D3DXMATRIX mat_world;
D3DXMatrixIdentity(
&
mat_world);
pd3dDevice
->
SetTransform(D3DTS_WORLD,
&
mat_world);
//
setup view matrix
D3DXMATRIX mat_view;
D3DXVECTOR3 eye(
0.0f
,
0.0f
,
-
3.0f
);
D3DXVECTOR3 at(
0.0f
,
0.0f
,
0.0f
);
D3DXVECTOR3 up(
0.0f
,
1.0f
,
0.0f
);
D3DXMatrixLookAtLH(
&
mat_view,
&
eye,
&
at,
&
up);
pd3dDevice
->
SetTransform(D3DTS_VIEW,
&
mat_view);
//
set projection matrix
D3DXMATRIX mat_proj;
float
aspect
=
(
float
)pBackBufferSurfaceDesc
->
Width
/
pBackBufferSurfaceDesc
->
Height;
D3DXMatrixPerspectiveFovLH(
&
mat_proj, D3DX_PI
/
4
, aspect,
1.0f
,
100.0f
);
pd3dDevice
->
SetTransform(D3DTS_PROJECTION,
&
mat_proj);
pd3dDevice
->
SetRenderState(D3DRS_LIGHTING, FALSE);
//
set texture color blend method, disalbe alpha blend.
pd3dDevice
->
SetTexture(
0
, g_texture);
pd3dDevice
->
SetTextureStageState(
0
, D3DTSS_COLORARG1, D3DTA_TEXTURE);
pd3dDevice
->
SetTextureStageState(
0
, D3DTSS_COLOROP, D3DTOP_SELECTARG1);
pd3dDevice
->
SetTextureStageState(
0
, D3DTSS_ALPHAOP, D3DTOP_DISABLE);
//
create texture coordinate using vertex position in camera space
pd3dDevice
->
SetTextureStageState(
0
, D3DTSS_TEXCOORDINDEX, D3DTSS_TCI_CAMERASPACEPOSITION);
//
pd3dDevice->SetTextureStageState(0, D3DTSS_TEXCOORDINDEX, D3DTSS_TCI_CAMERASPACEREFLECTIONVECTOR);
//
pd3dDevice->SetTextureStageState(0, D3DTSS_TEXCOORDINDEX, D3DTSS_TCI_SPHEREMAP);
pd3dDevice
->
SetTextureStageState(
0
, D3DTSS_TEXTURETRANSFORMFLAGS, D3DTTFF_COUNT2);
return
S_OK;
}
//
--------------------------------------------------------------------------------------
//
Release resources created in the OnResetDevice callback here
//
--------------------------------------------------------------------------------------
void
CALLBACK OnLostDevice(
void
*
pUserContext )
{
g_dlg_resource_manager.OnLostDevice();
g_settings_dlg.OnLostDevice();
g_font
->
OnLostDevice();
release_com(g_text_sprite);
}
//
--------------------------------------------------------------------------------------
//
Release resources created in the OnCreateDevice callback here
//
--------------------------------------------------------------------------------------
void
CALLBACK OnDestroyDevice(
void
*
pUserContext )
{
g_dlg_resource_manager.OnDestroyDevice();
g_settings_dlg.OnDestroyDevice();
release_com(g_font);
release_com(g_vertex_buffer);
release_com(g_texture);
}
//
--------------------------------------------------------------------------------------
//
Handle updates to the scene
//
--------------------------------------------------------------------------------------
void
CALLBACK OnFrameMove( IDirect3DDevice9
*
pd3dDevice,
double
fTime,
float
fElapsedTime,
void
*
pUserContext )
{
}
//
--------------------------------------------------------------------------------------
//
Render the helper information
//
--------------------------------------------------------------------------------------
void
RenderText()
{
CDXUTTextHelper text_helper(g_font, g_text_sprite,
20
);
text_helper.Begin();
//
show frame and device states
text_helper.SetInsertionPos(
5
,
5
);
text_helper.SetForegroundColor(FONT_COLOR);
text_helper.DrawTextLine( DXUTGetFrameStats(
true
) );
text_helper.DrawTextLine( DXUTGetDeviceStats() );
//
show other simple information
text_helper.SetForegroundColor( D3DXCOLOR(
1.0f
,
1.0f
,
1.0f
,
1.0f
) );
text_helper.DrawTextLine(L
"
Texture Coordinate Automatic Generate
"
);
//
show helper information
const
D3DSURFACE_DESC
*
surface_desc
=
DXUTGetBackBufferSurfaceDesc();
if
(g_show_help)
{
text_helper.SetInsertionPos(
10
, surface_desc
->
Height
-
18
*
5
);
text_helper.SetForegroundColor(FONT_COLOR);
text_helper.DrawTextLine(L
"
Controls (F1 to hide):
"
);
text_helper.SetInsertionPos(
40
, surface_desc
->
Height
-
18
*
4
);
text_helper.DrawTextLine(L
"
Quit: ESC
"
);
}
else
{
text_helper.SetInsertionPos(
10
, surface_desc
->
Height
-
15
*
4
);
text_helper.SetForegroundColor( D3DXCOLOR(
1.0f
,
1.0f
,
1.0f
,
1.0f
) );
text_helper.DrawTextLine(L
"
Press F1 for help
"
);
}
text_helper.End();
}
//
--------------------------------------------------------------------------------------
//
Render the scene
//
--------------------------------------------------------------------------------------
void
CALLBACK OnFrameRender( IDirect3DDevice9
*
pd3dDevice,
double
fTime,
float
fElapsedTime,
void
*
pUserContext )
{
HRESULT hr;
if
(g_settings_dlg.IsActive())
{
g_settings_dlg.OnRender(fElapsedTime);
return
;
}
//
Clear the render target and the zbuffer
V( pd3dDevice
->
Clear(
0
, NULL, D3DCLEAR_TARGET
|
D3DCLEAR_ZBUFFER, D3DCOLOR_ARGB(
0
,
0
,
0
,
0
),
1.0f
,
0
) );
//
Render the scene
if
( SUCCEEDED( pd3dDevice
->
BeginScene() ) )
{
pd3dDevice
->
SetStreamSource(
0
, g_vertex_buffer,
0
,
sizeof
(sCustomVertex));
pd3dDevice
->
SetFVF(D3DFVF_CUSTOM_VERTEX);
pd3dDevice
->
DrawPrimitive(D3DPT_TRIANGLESTRIP,
0
,
2
);
RenderText();
V(g_button_dlg.OnRender(fElapsedTime));
V( pd3dDevice
->
EndScene() );
}
}
//
--------------------------------------------------------------------------------------
//
Handle messages to the application
//
--------------------------------------------------------------------------------------
LRESULT CALLBACK MsgProc( HWND hWnd, UINT uMsg, WPARAM wParam, LPARAM lParam,
bool
*
pbNoFurtherProcessing,
void
*
pUserContext )
{
*
pbNoFurtherProcessing
=
g_dlg_resource_manager.MsgProc(hWnd, uMsg, wParam, lParam);
if
(
*
pbNoFurtherProcessing)
return
0
;
if
(g_settings_dlg.IsActive())
{
g_settings_dlg.MsgProc(hWnd, uMsg, wParam, lParam);
return
0
;
}
*
pbNoFurtherProcessing
=
g_button_dlg.MsgProc(hWnd, uMsg, wParam, lParam);
if
(
*
pbNoFurtherProcessing)
return
0
;
return
0
;
}
//
--------------------------------------------------------------------------------------
//
Handle keybaord event
//
--------------------------------------------------------------------------------------
void
CALLBACK OnKeyboardProc(UINT charater,
bool
is_key_down,
bool
is_alt_down,
void
*
user_context)
{
if
(is_key_down)
{
switch
(charater)
{
case
VK_F1:
g_show_help
=
!
g_show_help;
break
;
}
}
}
//
--------------------------------------------------------------------------------------
//
Handle events for controls
//
--------------------------------------------------------------------------------------
void
CALLBACK OnGUIEvent(UINT
event
,
int
control_id, CDXUTControl
*
control,
void
*
user_context)
{
switch
(control_id)
{
case
IDC_TOGGLE_FULLSCREEN:
DXUTToggleFullScreen();
break
;
case
IDC_TOGGLE_REF:
DXUTToggleREF();
break
;
case
IDC_CHANGE_DEVICE:
g_settings_dlg.SetActive(
true
);
break
;
}
}
//
--------------------------------------------------------------------------------------
//
Initialize dialogs
//
--------------------------------------------------------------------------------------
void
InitDialogs()
{
g_settings_dlg.Init(
&
g_dlg_resource_manager);
g_button_dlg.Init(
&
g_dlg_resource_manager);
g_button_dlg.SetCallback(OnGUIEvent);
int
x
=
35
, y
=
10
, width
=
125
, height
=
22
;
g_button_dlg.AddButton(IDC_TOGGLE_FULLSCREEN, L
"
Toggle full screen
"
, x, y, width, height);
g_button_dlg.AddButton(IDC_TOGGLE_REF, L
"
Toggle REF (F3)
"
, x, y
+=
24
, width, height);
g_button_dlg.AddButton(IDC_CHANGE_DEVICE, L
"
Change device (F2)
"
, x, y
+=
24
, width, height, VK_F2);
}
//
--------------------------------------------------------------------------------------
//
Initialize everything and go into a render loop
//
--------------------------------------------------------------------------------------
INT WINAPI WinMain( HINSTANCE, HINSTANCE, LPSTR,
int
)
{
//
Enable run-time memory check for debug builds.
#if
defined(DEBUG) | defined(_DEBUG)
_CrtSetDbgFlag( _CRTDBG_ALLOC_MEM_DF
|
_CRTDBG_LEAK_CHECK_DF );
#endif
//
Set the callback functions
DXUTSetCallbackDeviceCreated( OnCreateDevice );
DXUTSetCallbackDeviceReset( OnResetDevice );
DXUTSetCallbackDeviceLost( OnLostDevice );
DXUTSetCallbackDeviceDestroyed( OnDestroyDevice );
DXUTSetCallbackMsgProc( MsgProc );
DXUTSetCallbackFrameRender( OnFrameRender );
DXUTSetCallbackFrameMove( OnFrameMove );
DXUTSetCallbackKeyboard(OnKeyboardProc);
//
TODO: Perform any application-level initialization here
InitDialogs();
//
Initialize DXUT and create the desired Win32 window and Direct3D device for the application
DXUTInit(
true
,
true
,
true
);
//
Parse the command line, handle the default hotkeys, and show msgboxes
DXUTSetCursorSettings(
true
,
true
);
//
Show the cursor and clip it when in full screen
DXUTCreateWindow( L
"
Texture Color And Alpha Blend
"
);
DXUTCreateDevice( D3DADAPTER_DEFAULT,
true
,
640
,
480
, IsDeviceAcceptable, ModifyDeviceSettings );
//
Start the render loop
DXUTMainLoop();
//
TODO: Perform any application-level cleanup here
return
DXUTGetExitCode();
}
下载示例工程