Linear Layout
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:gravity="center">
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="North"
android:layout_marginBottom="100dp">
</Button>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="25dp"
android:text="WES">
</Button>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="50dp"
android:layout_marginEnd="50dp"
android:text="Center">
</Button>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="East">
</Button>
</LinearLayout>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="South"
android:layout_marginTop="100dp">
</Button>
</LinearLayout>
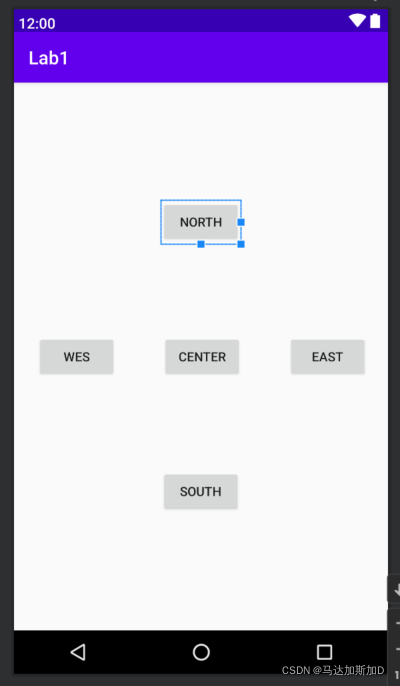
Relative Layout
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center">
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text = "NORTH"
android:layout_centerHorizontal="true"
android:layout_alignParentTop="true"
android:layout_marginTop="100dp"
android:id="@+id/north">
</Button>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_toStartOf="@+id/center"
android:layout_marginTop="30dp"
android:layout_below="@+id/north"
android:text="NW">
</Button>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_toEndOf="@+id/center"
android:layout_marginTop="30dp"
android:layout_below="@+id/north"
android:text="NE">
</Button>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerVertical="true"
android:layout_alignParentStart="true"
android:layout_marginStart="20dp"
android:text="WEST"
android:id="@+id/west">
</Button>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerInParent="true"
android:text="CENTER"
android:id="@+id/center">
</Button>
<Button
android:id="@+id/east"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentEnd="true"
android:layout_centerVertical="true"
android:layout_marginEnd="20dp"
android:text="EAST">
</Button>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_toStartOf="@+id/center"
android:layout_above="@+id/south"
android:layout_marginBottom="30dp"
android:text="SW">
</Button>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_toEndOf="@+id/center"
android:layout_above="@+id/south"
android:layout_marginBottom="30dp"
android:text="SE">
</Button>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
android:layout_alignParentBottom="true"
android:layout_marginBottom="100dp"
android:text = "SOUTH"
android:id="@+id/south">
</Button>
</RelativeLayout>
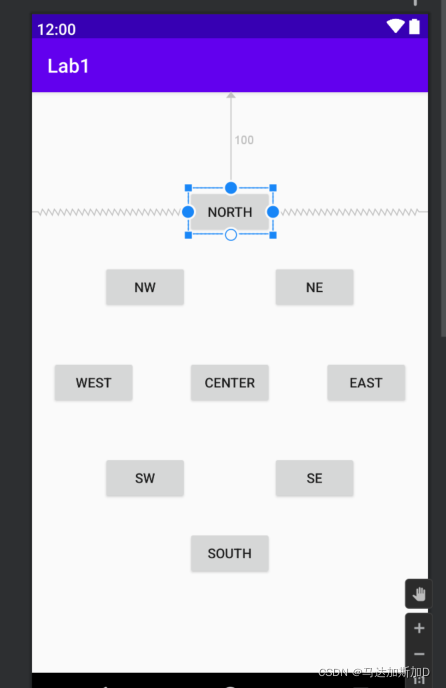
Table Layout
<?xml version="1.0" encoding="utf-8"?>
<TableLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_column="3"
android:layout_gravity="center">
<TableRow
android:id="@+id/tr1"
android:layout_width="match_parent"
android:layout_height="match_parent">
<Button
android:id="@+id/north_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_column="1"
android:text="North" />
</TableRow>
<TableRow
android:id="@+id/tr2"
android:layout_width="match_parent"
android:layout_height="match_parent">
<Button
android:id="@+id/west_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_column="0"
android:text="WEST" />
<Button
android:id="@+id/center_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_column="1"
android:text="CENTER" />
<Button
android:id="@+id/east_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_column="2"
android:text="EAST" />
</TableRow>
<TableRow
android:id="@+id/t3"
android:layout_width="match_parent"
android:layout_height="match_parent">
<Button
android:id="@+id/south_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_column="1"
android:text="SOUTH" />
</TableRow>
</TableLayout>
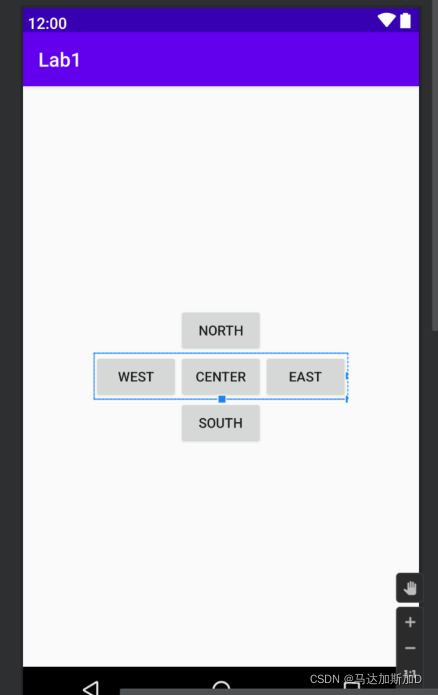
Grid Layout
<?xml version="1.0" encoding="utf-8"?>
<GridLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:columnCount="3"
android:rowCount="3">
<Button
android:id="@+id/north_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_row="0"
android:layout_column="1"
android:layout_rowWeight="1"
android:layout_columnWeight="1"
android:text="north" />
<Button
android:id="@+id/west_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_row="1"
android:layout_column="0"
android:layout_rowWeight="1"
android:layout_columnWeight="1"
android:text="west" />
<Button
android:id="@+id/center_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_row="1"
android:layout_column="1"
android:layout_rowWeight="1"
android:layout_columnWeight="1"
android:text="center" />
<Button
android:id="@+id/east_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_row="1"
android:layout_column="2"
android:layout_rowWeight="1"
android:layout_columnWeight="1"
android:text="east" />
<Button
android:id="@+id/south_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_row="2"
android:layout_column="1"
android:layout_rowWeight="1"
android:layout_columnWeight="1"
android:text="south" />
</GridLayout>
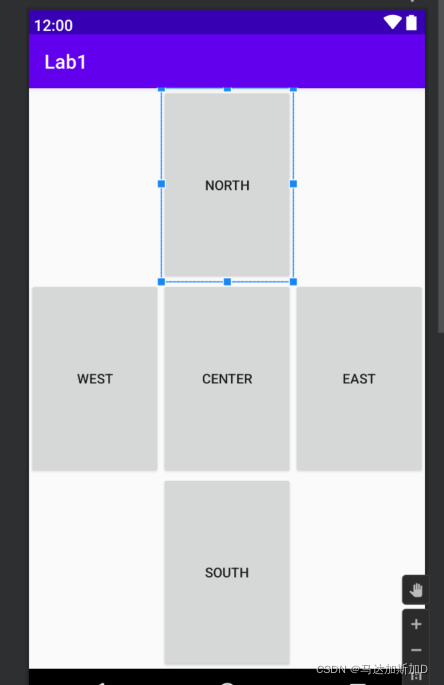
Frame Layout
<?xml version="1.0" encoding="utf-8"?>
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/framelayout"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@drawable/worldmap"
android:scaleType="centerCrop">
<ImageView
android:layout_width="100dp"
android:layout_height="100dp"
android:layout_marginTop="30dp"
android:background="@drawable/scu_copy"
android:layout_gravity="center_horizontal">
</ImageView>
</FrameLayout>
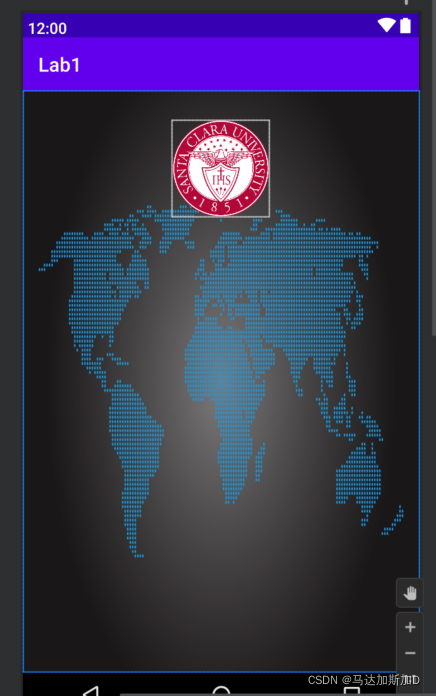
Constraint Layout
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
xmlns:app="http://schemas.android.com/apk/res-auto">
<androidx.constraintlayout.widget.Guideline
android:layout_height="wrap_content"
android:layout_width="wrap_content"
android:orientation="horizontal"
app:layout_constraintGuide_begin="0dp"
android:id="@+id/TopGuideline">
</androidx.constraintlayout.widget.Guideline>
<androidx.constraintlayout.widget.Guideline
android:layout_height="wrap_content"
android:layout_width="wrap_content"
android:orientation="horizontal"
app:layout_constraintGuide_end="0dp"
android:id="@+id/bottomGuideline">
</androidx.constraintlayout.widget.Guideline>
<androidx.constraintlayout.widget.Guideline
android:layout_height="wrap_content"
android:layout_width="wrap_content"
android:orientation="vertical"
app:layout_constraintGuide_begin="0dp"
android:id="@+id/leftGuideline">
</androidx.constraintlayout.widget.Guideline>
<androidx.constraintlayout.widget.Guideline
android:layout_height="wrap_content"
android:layout_width="wrap_content"
android:orientation="vertical"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintGuide_end="0dp"
android:id="@+id/rightGuideline">
</androidx.constraintlayout.widget.Guideline>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:layout_constraintBottom_toTopOf="@+id/center"
app:layout_constraintTop_toBottomOf="@+id/TopGuideline"
app:layout_constraintStart_toStartOf="@+id/center"
android:text="north">
</Button>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:layout_constraintStart_toStartOf="@+id/leftGuideline"
app:layout_constraintEnd_toStartOf="@+id/center"
app:layout_constraintTop_toBottomOf="@+id/TopGuideline"
app:layout_constraintBottom_toTopOf="@+id/bottomGuideline"
android:text="West">
</Button>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:layout_constraintBottom_toTopOf="@+id/bottomGuideline"
app:layout_constraintTop_toBottomOf="@+id/TopGuideline"
app:layout_constraintStart_toEndOf="@+id/leftGuideline"
app:layout_constraintEnd_toStartOf="@+id/rightGuideline"
android:id="@+id/center"
android:text="center">
</Button>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:layout_constraintEnd_toStartOf="@+id/rightGuideline"
app:layout_constraintStart_toEndOf="@+id/center"
app:layout_constraintTop_toBottomOf="@+id/TopGuideline"
app:layout_constraintBottom_toTopOf="@+id/bottomGuideline"
android:text="East">
</Button>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:layout_constraintBottom_toTopOf="@+id/bottomGuideline"
app:layout_constraintTop_toBottomOf="@+id/center"
app:layout_constraintStart_toStartOf="@+id/center"
android:text="south">
</Button>
</androidx.constraintlayout.widget.ConstraintLayout>
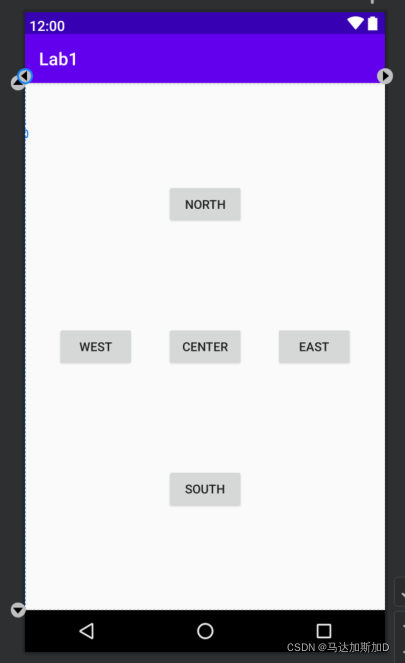
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
xmlns:app="http://schemas.android.com/apk/res-auto">
<androidx.constraintlayout.widget.Guideline
android:layout_height="wrap_content"
android:layout_width="wrap_content"
android:orientation="horizontal"
app:layout_constraintGuide_begin="0dp"
android:id="@+id/TopGuideline">
</androidx.constraintlayout.widget.Guideline>
<androidx.constraintlayout.widget.Guideline
android:layout_height="wrap_content"
android:layout_width="wrap_content"
android:orientation="horizontal"
app:layout_constraintGuide_end="0dp"
android:id="@+id/bottomGuideline">
</androidx.constraintlayout.widget.Guideline>
<androidx.constraintlayout.widget.Guideline
android:layout_height="wrap_content"
android:layout_width="wrap_content"
android:orientation="vertical"
app:layout_constraintGuide_begin="0dp"
android:id="@+id/leftGuideline">
</androidx.constraintlayout.widget.Guideline>
<androidx.constraintlayout.widget.Guideline
android:layout_height="wrap_content"
android:layout_width="wrap_content"
android:orientation="vertical"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintGuide_end="0dp"
android:id="@+id/rightGuideline">
</androidx.constraintlayout.widget.Guideline>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:layout_constraintBottom_toTopOf="@+id/bottomGuideline"
app:layout_constraintTop_toBottomOf="@+id/TopGuideline"
app:layout_constraintStart_toEndOf="@+id/leftGuideline"
app:layout_constraintEnd_toStartOf="@+id/rightGuideline"
android:id="@+id/center"
android:text="center">
</Button>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:layout_constraintCircle="@+id/center"
app:layout_constraintCircleAngle="0"
app:layout_constraintCircleRadius="150dp"
android:text="North">
</Button>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:layout_constraintCircle="@+id/center"
app:layout_constraintCircleAngle="-45"
app:layout_constraintCircleRadius="150dp"
android:text="NE">
</Button>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:layout_constraintCircle="@+id/center"
app:layout_constraintCircleAngle="-90"
app:layout_constraintCircleRadius="150dp"
android:text="East">
</Button>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:layout_constraintCircle="@+id/center"
app:layout_constraintCircleAngle="-135"
app:layout_constraintCircleRadius="150dp"
android:text="SE">
</Button>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:layout_constraintCircle="@+id/center"
app:layout_constraintCircleAngle="-180"
app:layout_constraintCircleRadius="150dp"
android:text="South">
</Button>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:layout_constraintCircle="@+id/center"
app:layout_constraintCircleAngle="-225"
app:layout_constraintCircleRadius="150dp"
android:text="SW">
</Button>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:layout_constraintCircle="@+id/center"
app:layout_constraintCircleAngle="-270"
app:layout_constraintCircleRadius="150dp"
android:text="West">
</Button>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:layout_constraintCircle="@+id/center"
app:layout_constraintCircleAngle="-315"
app:layout_constraintCircleRadius="150dp"
android:text="NW">
</Button>
</androidx.constraintlayout.widget.ConstraintLayout>
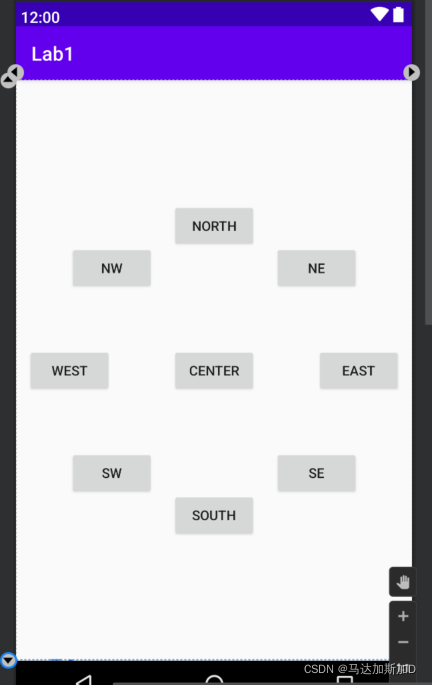