/*****************************************************************//**
* \file GoldDir.h
* \brief 组合模式CompositePattern 亦称: 对象树、Object Tree、Composite c++14
* 2023年5月28日 涂聚文 Geovin Du Visual Studio 2022 edit.
* \author geovindu
* \date May 2023
*********************************************************************/
#pragma once
#ifndef GOLDDIR_H
#define GOLDDIR_H
#include <iostream>
#include <sstream>
#include <vector>
#include <list>
#include "GoldFile.h"
using namespace std;
/**
* @brief 类库空间名
* geovindu edit
*
*/
namespace DuCompositePattern
{
/// <summary>
/// 目录相关类
/// </summary>
class GoldDir
{
public:
/// <summary>
/// 构造函数
/// </summary>
/// <param name="name"></param>
GoldDir(string name) :mSname(name) {}
public:
/// <summary>
/// 目录中可以增加其他文件
/// </summary>
/// <param name="pfile"></param>
void AddFile(GoldFile* pfile)
{
mChildFile.push_back(pfile);
}
/// <summary>
/// 目录中可以增加其他目录
/// </summary>
/// <param name="pdir"></param>
void AddDir(GoldDir* pdir)
{
mChildDir.push_back(pdir);
}
/// <summary>
/// 显示目录名,同时也要负责其下面的文件和目录名的显示工作
/// </summary>
/// <param name="lvlstr"></param>
void ShowName(string lvlstr) //lvlstr:为了显示层次关系的缩进字符串内容
{
//(1)输出本目录名
cout << lvlstr << "+" << mSname << endl;//显示"+"代表是一个目录,其中会包含其他内容
//(2)输出所包含的文件名
lvlstr += " "; //本目录中的文件和目录的显示,要缩进一些来显示
for (auto iter = mChildFile.begin(); iter != mChildFile.end(); ++iter)
{
(*iter)->ShowName(lvlstr); //显示文件名
}
//(3)输出所包含的目录名
for (auto iter = mChildDir.begin(); iter != mChildDir.end(); ++iter)
{
(*iter)->ShowName(lvlstr); //显示目录名,这里涉及到了递归调用
}
}
private:
/// <summary>
/// 目录名
/// </summary>
string mSname;
/// <summary>
/// 目录中包含的文件列表,记得在源文件上面增加代码 #include <list>
/// </summary>
list<GoldFile*> mChildFile;
/// <summary>
/// 目录中包含的子目录列表
/// </summary>
list<GoldDir*> mChildDir;
};
}
#endif
/*****************************************************************//**
* \file GoldFile.h
* \brief 组合模式CompositePattern 亦称: 对象树、Object Tree、Composite c++14
* 2023年5月28日 涂聚文 Geovin Du Visual Studio 2022 edit.
* \author geovindu
* \date May 2023
*********************************************************************/
#pragma once
#ifndef GOLDFILE_H
#define GOLDFILE_H
#include <iostream>
#include <sstream>
#include <vector>
#include <list>
using namespace std;
/**
* @brief 类库空间名
* geovindu edit
*
*/
namespace DuCompositePattern
{
/// <summary>
/// 文件相关类
/// </summary>
class GoldFile
{
public:
/// <summary>
/// 构造函数
/// </summary>
/// <param name="name"></param>
GoldFile(string name) :mSname(name) {}
/// <summary>
/// 显示文件名
/// </summary>
/// <param name="lvlstr"></param>
void ShowName(string lvlstr)
{
cout << lvlstr << "-" << mSname << endl; //显示"-"代表是一个文件,属末端节点(不会再有子节点)
}
private:
string mSname; //文件名
};
}
#endif
/*****************************************************************//**
* \file PearFile.h
* \brief 组合模式CompositePattern 亦称: 对象树、Object Tree、Composite c++14
* 2023年5月28日 涂聚文 Geovin Du Visual Studio 2022 edit.
* \author geovindu
* \date May 2023
*********************************************************************/
#pragma once
#ifndef PEARFILE_H
#define PEARFILE_H
#include <iostream>
#include <sstream>
#include <vector>
#include <list>
#include "PearFileSystem.h"
using namespace std;
/**
* @brief 类库空间名
* geovindu edit
*
*/
namespace DuPearCompositePattern
{
/// <summary>
/// 文件相关类
/// </summary>
class PearFile:public PearFileSystem
{
public:
/// <summary>
/// 构造函数
/// </summary>
/// <param name="name"></param>
PearFile(string name) :mSname(name) {}
/// <summary>
///
/// </summary>
/// <param name="level"></param>
void ShowName(int level)
{
for (int i = 0; i < level; ++i) { cout << " "; } //显示若干个空格用与对齐
cout << "-" << mSname << endl;
}
/// <summary>
///添加
/// </summary>
/// <param name="pfilesys"></param>
/// <returns></returns>
virtual int Add(PearFileSystem* pfilesys)
{
//文件中其实是不可以增加其他文件或者目录的,所以这里直接返回-1,无奈的是父类中定义了该接口,所以子类中也必须实现该接口
return -1;
}
/// <summary>
/// 移除
/// </summary>
/// <param name="pfilesys"></param>
/// <returns></returns>
virtual int Remove(PearFileSystem* pfilesys)
{
//文件中不包含其他文件或者目录,所以这里直接返回-1
return -1;
}
private:
/// <summary>
/// 文件名
/// </summary>
string mSname;
};
}
#endif
/*****************************************************************//**
* \file PearDir.h
* \brief 组合模式CompositePattern 亦称: 对象树、Object Tree、Composite c++14
* 2023年5月28日 涂聚文 Geovin Du Visual Studio 2022 edit.
* \author geovindu
* \date May 2023
*********************************************************************/
#pragma once
#ifndef PEARDIR_H
#define PEARDIR_H
#include <iostream>
#include <sstream>
#include <vector>
#include <list>
#include "PearFileSystem.h"
using namespace std;
/**
* @brief 类库空间名
* geovindu edit
*
*/
namespace DuPearCompositePattern
{
/// <summary>
/// 目录相关类
/// </summary>
class PearDir:public PearFileSystem
{
public:
/// <summary>
/// 构造函数
/// </summary>
/// <param name="name"></param>
PearDir(string name) :mSname(name) {}
/// <summary>
/// 显示名字
/// </summary>
/// <param name="level"></param>
void ShowName(int level)
{
//(1)显示若干个空格用与对齐
for (int i = 0; i < level; ++i) { cout << " "; }
//(2)输出本目录名
cout << "+" << mSname << endl;
//(3)显示的层级向下走一级
level++;
//(4)输出所包含的子内容(可能是文件,也可能是子目录)
//遍历目录中的文件和子目录
for (auto iter = mChild.begin(); iter != mChild.end(); ++iter)
{
(*iter)->ShowName(level);
}//end for
}
/// <summary>
/// 增加
/// </summary>
/// <param name="pfilesys"></param>
/// <returns></returns>
virtual int Add(PearFileSystem* pfilesys)
{
mChild.push_back(pfilesys);
return 0;
}
/// <summary>
/// 移除
/// </summary>
/// <param name="pfilesys"></param>
/// <returns></returns>
virtual int Remove(PearFileSystem* pfilesys)
{
mChild.remove(pfilesys);
return 0;
}
private:
/// <summary>
/// 文件名
/// </summary>
string mSname;
/// <summary>
/// 目录中包含的文件或其他目录列表
/// </summary>
list<PearFileSystem*> mChild; //
};
}
#endif
/*****************************************************************//**
* \file PearFileSystem.h
* \brief 组合模式CompositePattern 亦称: 对象树、Object Tree、Composite c++14
* 2023年5月28日 涂聚文 Geovin Du Visual Studio 2022 edit.
* \author geovindu
* \date May 2023
*********************************************************************/
#pragma once
#ifndef PEARFILESYSTEM_H
#define PEARFILESYSTEM_H
#include <iostream>
#include <sstream>
#include <vector>
#include <list>
using namespace std;
/**
* @brief 类库空间名
* geovindu edit
*
*/
namespace DuPearCompositePattern
{
/// <summary>
/// 抽象父类FileSystem(抽象接口)
/// </summary>
class PearFileSystem
{
public:
/// <summary>
///
/// </summary>
/// <param name="level"></param>
virtual void ShowName(int level) = 0; //显示名字,参数level用于表示显示的层级,用于显示对齐
/// <summary>
/// 向当前目录中增加文件或子目录
/// </summary>
/// <param name="pfilesys"></param>
/// <returns></returns>
virtual int Add(PearFileSystem* pfilesys) = 0;
/// <summary>
/// 从当前目录中移除文件或子目录
/// </summary>
/// <param name="pfilesys"></param>
/// <returns></returns>
virtual int Remove(PearFileSystem* pfilesys) = 0;
/// <summary>
/// 做父类时析构函数应该为虚函数
/// </summary>
virtual ~PearFileSystem() {}
};
}
#endif
/*****************************************************************//**
* \file JadeFile.h
* \brief 组合模式CompositePattern 亦称: 对象树、Object Tree、Composite c++14
* 2023年5月28日 涂聚文 Geovin Du Visual Studio 2022 edit.
* \author geovindu
* \date May 2023
*********************************************************************/
#pragma once
#ifndef JADEFILE_H
#define JADEFILE_H
#include <iostream>
#include <sstream>
#include <vector>
#include <list>
#include "JadeFileSystem.h"
using namespace std;
/**
* @brief 类库空间名
* geovindu edit
*
*/
namespace DuJadeCompositePattern
{
/// <summary>
/// 文件相关类
/// </summary>
class JadeFile :public JadeFileSystem
{
public:
/// <summary>
/// 构造函数
/// </summary>
/// <param name="name"></param>
JadeFile(string name) :mSname(name) {}
/// <summary>
/// 显示名字
/// </summary>
/// <param name="level"></param>
void ShowName(int level)
{
for (int i = 0; i < level; ++i) { cout << " "; } //显示若干个空格用与对齐
cout << "-" << mSname << endl;
}
public:
virtual int countNumOfFiles()
{
return 1; //文件节点,做数量统计时按1计算
}
private:
/// <summary>
/// 文件名
/// </summary>
string mSname;
};
}
#endif
/*****************************************************************//**
* \file JadeDir.h
* \brief 组合模式CompositePattern 亦称: 对象树、Object Tree、Composite c++14
* 2023年5月28日 涂聚文 Geovin Du Visual Studio 2022 edit.
* \author geovindu
* \date May 2023
*********************************************************************/
#pragma once
#ifndef JADEDIR_H
#define JADEDIR_H
#include <iostream>
#include <sstream>
#include <vector>
#include <list>
#include "JadeFileSystem.h"
using namespace std;
/**
* @brief 类库空间名
* geovindu edit
*
*/
namespace DuJadeCompositePattern
{
/// <summary>
///
/// </summary>
class JadeDir:public JadeFileSystem
{
//该类内容基本没变,把Add和Remove成员函数前面的virtual修饰符去掉即可。
public:
/// <summary>
/// 构造函数
/// </summary>
/// <param name="name"></param>
JadeDir(string name) :mSname(name) {}
/// <summary>
/// 显示名字
/// </summary>
/// <param name="level"></param>
void ShowName(int level)
{
//(1)显示若干个空格用与对齐
for (int i = 0; i < level; ++i) { cout << " "; }
//(2)输出本目录名
cout << "+" << mSname << endl;
//(3)显示的层级向下走一级
level++;
//(4)输出所包含的子内容(可能是文件,也可能是子目录)
//遍历目录中的文件和子目录
for (auto iter = mChild.begin(); iter != mChild.end(); ++iter)
{
(*iter)->ShowName(level);
}//end for
}
/*virtual*/
int Add(JadeFileSystem* pfilesys)
{
mChild.push_back(pfilesys);
return 0;
}
/*virtual*/
int Remove(JadeFileSystem* pfilesys)
{
mChild.remove(pfilesys);
return 0;
}
public:
virtual JadeDir* ifCompositeObj() { return this; }
public:
virtual int countNumOfFiles()
{
int iNumOfFiles = 0;
for (auto iter = mChild.begin(); iter != mChild.end(); ++iter)
{
iNumOfFiles += (*iter)->countNumOfFiles();//递归调用
}
return iNumOfFiles;
}
private:
/// <summary>
/// 文件名
/// </summary>
string mSname;
/// <summary>
///
/// </summary>
list<JadeFileSystem*> mChild;
};
}
#endif
/*****************************************************************//**
* \file JadeFileSystem.h
* \brief 组合模式CompositePattern 亦称: 对象树、Object Tree、Composite c++14
* 2023年5月28日 涂聚文 Geovin Du Visual Studio 2022 edit.
* \author geovindu
* \date May 2023
*********************************************************************/
#pragma once
#ifndef JADEFILESYSTEM_H
#define JADEFILESYSTEM_H
#include <iostream>
#include <sstream>
#include <vector>
#include <list>
using namespace std;
/**
* @brief 类库空间名
* geovindu edit
*
*/
namespace DuJadeCompositePattern
{
class JadeDir;
/// <summary>
///
/// </summary>
class JadeFileSystem
{
public:
/// <summary>
/// 显示名字,参数level用于表示显示的层级,用于显示对齐
/// </summary>
/// <param name="level"></param>
virtual void ShowName(int level) = 0;
/// <summary>
/// 做父类时析构函数应该为虚函数
/// </summary>
virtual ~JadeFileSystem() {}
public:
/// <summary>
///
/// </summary>
/// <returns></returns>
virtual JadeDir* ifCompositeObj() { return nullptr; }
public:
/// <summary>
///
/// </summary>
/// <returns></returns>
virtual int countNumOfFiles() = 0; //统计目录下包含的文件个数
};
}
#endif
/*****************************************************************//**
* \file GeovinDu.h
* \brief 组合模式CompositePattern 亦称: 对象树、Object Tree、Composite c++14
* 2023年5月28日 涂聚文 Geovin Du Visual Studio 2022 edit.
* \author geovindu
* \date May 2023
*********************************************************************/
#pragma once
#ifndef GEOVINDU_H
#define GEOVINDU_H
#include <iostream>
#include <sstream>
#include <vector>
#include <list>
#include "GoldFile.h"
#include "GoldDir.h"
#include "PearFileSystem.h"
#include "PearDir.h"
#include "PearFile.h"
#include "JadeDir.h"
#include "JadeFile.h"
#include "JadeFileSystem.h"
using namespace std;
using namespace DuPearCompositePattern;
using namespace DuJadeCompositePattern;
/**
* @brief 类库空间名
* geovindu edit
*
*/
namespace DuCompositePattern
{
/// <summary>
///
/// </summary>
class GeovinDu
{
private:
public:
/// <summary>
///示例
/// </summary>
void displaySimple();
/// <summary>
///
/// </summary>
void displayPearSimple();
/// <summary>
///
/// </summary>
void displayJadeSimple();
};
}
#endif
/*****************************************************************//**
* \file GeovinDu.cpp
* \brief 组合模式CompositePattern 亦称: 对象树、Object Tree、Composite c++14
* 2023年5月28日 涂聚文 Geovin Du Visual Studio 2022 edit.
* \author geovindu
* \date May 2023
*********************************************************************/
#include "GeovinDu.h"
using namespace std;
using namespace DuPearCompositePattern;
using namespace DuJadeCompositePattern;
/**
* @brief 类库空间名
* geovindu edit
*
*/
namespace DuCompositePattern
{
/// <summary>
/// 示例
/// </summary>
void GeovinDu::displaySimple()
{
//(1)创建各种目录,文件对象
DuCompositePattern::GoldDir* pdir1 = new DuCompositePattern::GoldDir("root");
//-------
DuCompositePattern::GoldFile* pfile1 = new DuCompositePattern::GoldFile("common.mk");
DuCompositePattern::GoldFile* pfile2 = new DuCompositePattern::GoldFile("config.mk");
DuCompositePattern::GoldFile* pfile3 = new DuCompositePattern::GoldFile("makefile");
//-------
DuCompositePattern::GoldDir* pdir2 = new DuCompositePattern::GoldDir("app");
DuCompositePattern::GoldFile* pfile4 = new DuCompositePattern::GoldFile("nginx.c");
DuCompositePattern::GoldFile* pfile5 = new DuCompositePattern::GoldFile("ngx_conf.c");
//-------
DuCompositePattern::GoldDir* pdir3 = new DuCompositePattern::GoldDir("signal");
DuCompositePattern::GoldFile* pfile6 = new DuCompositePattern::GoldFile("ngx_signal.c");
//-------
DuCompositePattern::GoldDir* pdir4 = new DuCompositePattern::GoldDir("_include");
DuCompositePattern::GoldFile* pfile7 = new DuCompositePattern::GoldFile("ngx_func.h");
DuCompositePattern::GoldFile* pfile8 = new DuCompositePattern::GoldFile("ngx_signal.h");
//(2)构造树形目录结构
pdir1->AddFile(pfile1);
pdir1->AddFile(pfile2);
pdir1->AddFile(pfile3);
//-------
pdir1->AddDir(pdir2);
pdir2->AddFile(pfile4);
pdir2->AddFile(pfile5);
//-------
pdir1->AddDir(pdir3);
pdir3->AddFile(pfile6);
//-------
pdir1->AddDir(pdir4);
pdir4->AddFile(pfile7);
pdir4->AddFile(pfile8);
//(3)输出整个目录结构,只要调用根目录的ShowName方法即可,每个子目录都有自己的ShowName方法负责自己旗下的文件和目录的显示
pdir1->ShowName("");//缩进字符刚开始可以为空
//(4)释放资源
delete pfile8;
delete pfile7;
delete pdir4;
//-------
delete pfile6;
delete pdir3;
//-------
delete pfile5;
delete pfile4;
delete pdir2;
//-------
delete pfile3;
delete pfile2;
delete pfile1;
delete pdir1;
}
/// <summary>
///
/// </summary>
void GeovinDu::displayPearSimple()
{
//(1)创建各种目录,文件对象
DuPearCompositePattern::PearFileSystem* pdir1 = new DuPearCompositePattern::PearDir("root");
//-------
DuPearCompositePattern::PearFileSystem* pfile1 = new DuPearCompositePattern::PearFile("common.mk");
DuPearCompositePattern::PearFileSystem* pfile2 = new DuPearCompositePattern::PearFile("config.mk");
DuPearCompositePattern::PearFileSystem* pfile3 = new DuPearCompositePattern::PearFile("makefile");
//-------
DuPearCompositePattern::PearFileSystem* pdir2 = new DuPearCompositePattern::PearDir("app");
DuPearCompositePattern::PearFileSystem* pfile4 = new DuPearCompositePattern::PearFile("nginx.c");
DuPearCompositePattern::PearFileSystem* pfile5 = new DuPearCompositePattern::PearFile("ngx_conf.c");
//-------
DuPearCompositePattern::PearFileSystem* pdir3 = new DuPearCompositePattern::PearDir("signal");
DuPearCompositePattern::PearFileSystem* pfile6 = new DuPearCompositePattern::PearFile("ngx_signal.c");
//-------
DuPearCompositePattern::PearFileSystem* pdir4 = new DuPearCompositePattern::PearDir("_include");
DuPearCompositePattern::PearFileSystem* pfile7 = new DuPearCompositePattern::PearFile("ngx_func.h");
DuPearCompositePattern::PearFileSystem* pfile8 = new DuPearCompositePattern::PearFile("ngx_signal.h");
//(2)构造树形目录结构
pdir1->Add(pfile1);
pdir1->Add(pfile2);
pdir1->Add(pfile3);
//-------
pdir1->Add(pdir2);
pdir2->Add(pfile4);
pdir2->Add(pfile5);
//-------
pdir1->Add(pdir3);
pdir3->Add(pfile6);
//-------
pdir1->Add(pdir4);
pdir4->Add(pfile7);
pdir4->Add(pfile8);
//(3)输出整个目录结构,只要调用根目录的ShowName方法即可,每个子目录都有自己的ShowName方法负责自己旗下的文件和目录的显示
pdir1->ShowName(0);
//(4)释放资源
delete pfile8;
delete pfile7;
delete pdir4;
//-------
delete pfile6;
delete pdir3;
//-------
delete pfile5;
delete pfile4;
delete pdir2;
//-------
delete pfile3;
delete pfile2;
delete pfile1;
delete pdir1;
}
/// <summary>
///
/// </summary>
void GeovinDu::displayJadeSimple()
{
//(1)创建各种目录,文件对象
DuJadeCompositePattern::JadeDir* pdir1 = new DuJadeCompositePattern::JadeDir("root");
//-------
DuJadeCompositePattern::JadeFileSystem* pfile1 = new DuJadeCompositePattern::JadeFile("common.mk");
DuJadeCompositePattern::JadeFileSystem* pfile2 = new DuJadeCompositePattern::JadeFile("config.mk");
DuJadeCompositePattern::JadeFileSystem* pfile3 = new DuJadeCompositePattern::JadeFile("makefile");
//-------
DuJadeCompositePattern::JadeDir* pdir2 = new DuJadeCompositePattern::JadeDir("app");
if (pdir2->ifCompositeObj() != nullptr)
{
//是个组合对象,可以向其中增加单个对象和其它组合对象
}
if (dynamic_cast<DuJadeCompositePattern::JadeDir*>(pdir2) != nullptr)
{
//是个组合对象,可以向其中增加单个对象和其它组合对象
}
DuJadeCompositePattern::JadeFileSystem* pfile4 = new DuJadeCompositePattern::JadeFile("nginx.c");
DuJadeCompositePattern::JadeFileSystem* pfile5 = new DuJadeCompositePattern::JadeFile("ngx_conf.c");
//-------
DuJadeCompositePattern::JadeDir* pdir3 = new DuJadeCompositePattern::JadeDir("signal");
DuJadeCompositePattern::JadeFileSystem* pfile6 = new DuJadeCompositePattern::JadeFile("ngx_signal.c");
//-------
DuJadeCompositePattern::JadeDir* pdir4 = new DuJadeCompositePattern::JadeDir("_include");
DuJadeCompositePattern::JadeFileSystem* pfile7 = new DuJadeCompositePattern::JadeFile("ngx_func.h");
DuJadeCompositePattern::JadeFileSystem* pfile8 = new DuJadeCompositePattern::JadeFile("ngx_signal.h");
//(2)构造树形目录结构
//这部分内容基本没变,
pdir1->Add(pfile1);
pdir1->Add(pfile2);
pdir1->Add(pfile3);
//-------
pdir1->Add(pdir2);
pdir2->Add(pfile4);
pdir2->Add(pfile5);
//-------
pdir1->Add(pdir3);
pdir3->Add(pfile6);
//-------
pdir1->Add(pdir4);
pdir4->Add(pfile7);
pdir4->Add(pfile8);
//(3)输出整个目录结构,只要调用根目录的ShowName方法即可,每个子目录都有自己的ShowName方法负责自己旗下的文件和目录的显示
pdir1->ShowName(0);
cout << "文件的数量为:" << pdir1->countNumOfFiles() << endl;
//(4)释放资源
//这部分内容基本没变,
delete pfile8;
delete pfile7;
delete pdir4;
//-------
delete pfile6;
delete pdir3;
//-------
delete pfile5;
delete pfile4;
delete pdir2;
//-------
delete pfile3;
delete pfile2;
delete pfile1;
delete pdir1;
}
}
调用:
/*****************************************************************//**
* \file ConsoleDuCompositePattern.cpp
* \brief 组合模式CompositePattern 亦称: 对象树、Object Tree、Composite c++14
* 2023年5月28日 涂聚文 Geovin Du Visual Studio 2022 edit.
* \author geovindu
* \date May 2023
*********************************************************************/
// ConsoleDuCompositePattern.cpp : 此文件包含 "main" 函数。程序执行将在此处开始并结束。
//2023年5月22日 涂聚文 Geovin Du Visual Studio 2022 edit.
#define _UNICODE
#include <iostream>
#include <vector>
#include <list>
#include "GeovinDu.h"
#ifdef _DEBUG //只在Debug(调试)模式下
#ifndef DEBUG_NEW
#define DEBUG_NEW new(_NORMAL_BLOCK,__FILE__,__LINE__) //重新定义new运算符
#define new DEBUG_NEW
#endif
#endif
using namespace std;
using namespace DuCompositePattern;
int main()
{
_CrtSetDbgFlag(_CRTDBG_ALLOC_MEM_DF | _CRTDBG_LEAK_CHECK_DF);//程序退出时检测内存泄漏并显示到“输出”窗口
std::cout << "Hello World!涂聚文 Geovin Du\n";
GeovinDu geovin;
geovin.displaySimple();
cout << "*********" << endl;
geovin.displayPearSimple();
cout << "*********" << endl;
geovin.displayJadeSimple();
system("pause");
return 0;
}
// 运行程序: Ctrl + F5 或调试 >“开始执行(不调试)”菜单
// 调试程序: F5 或调试 >“开始调试”菜单
// 入门使用技巧:
// 1. 使用解决方案资源管理器窗口添加/管理文件
// 2. 使用团队资源管理器窗口连接到源代码管理
// 3. 使用输出窗口查看生成输出和其他消息
// 4. 使用错误列表窗口查看错误
// 5. 转到“项目”>“添加新项”以创建新的代码文件,或转到“项目”>“添加现有项”以将现有代码文件添加到项目
// 6. 将来,若要再次打开此项目,请转到“文件”>“打开”>“项目”并选择 .sln 文件
#define UNICODE
输出:
/*****************************************************************//**
* \file ConsoleDuCompositePattern.cpp
* \brief 组合模式CompositePattern 亦称: 对象树、Object Tree、Composite c++14
* 2023年5月28日 涂聚文 Geovin Du Visual Studio 2022 edit.
* \author geovindu
* \date May 2023
*********************************************************************/
// ConsoleDuCompositePattern.cpp : 此文件包含 "main" 函数。程序执行将在此处开始并结束。
//2023年5月22日 涂聚文 Geovin Du Visual Studio 2022 edit.
#define _UNICODE
#include <iostream>
#include <vector>
#include <list>
#include "GeovinDu.h"
#ifdef _DEBUG //只在Debug(调试)模式下
#ifndef DEBUG_NEW
#define DEBUG_NEW new(_NORMAL_BLOCK,__FILE__,__LINE__) //重新定义new运算符
#define new DEBUG_NEW
#endif
#endif
using namespace std;
using namespace DuCompositePattern;
int main()
{
_CrtSetDbgFlag(_CRTDBG_ALLOC_MEM_DF | _CRTDBG_LEAK_CHECK_DF);//程序退出时检测内存泄漏并显示到“输出”窗口
std::cout << "Hello World!涂聚文 Geovin Du\n";
GeovinDu geovin;
geovin.displaySimple();
cout << "*********" << endl;
geovin.displayPearSimple();
cout << "*********" << endl;
geovin.displayJadeSimple();
system("pause");
return 0;
}
// 运行程序: Ctrl + F5 或调试 >“开始执行(不调试)”菜单
// 调试程序: F5 或调试 >“开始调试”菜单
// 入门使用技巧:
// 1. 使用解决方案资源管理器窗口添加/管理文件
// 2. 使用团队资源管理器窗口连接到源代码管理
// 3. 使用输出窗口查看生成输出和其他消息
// 4. 使用错误列表窗口查看错误
// 5. 转到“项目”>“添加新项”以创建新的代码文件,或转到“项目”>“添加现有项”以将现有代码文件添加到项目
// 6. 将来,若要再次打开此项目,请转到“文件”>“打开”>“项目”并选择 .sln 文件
#define UNICODE
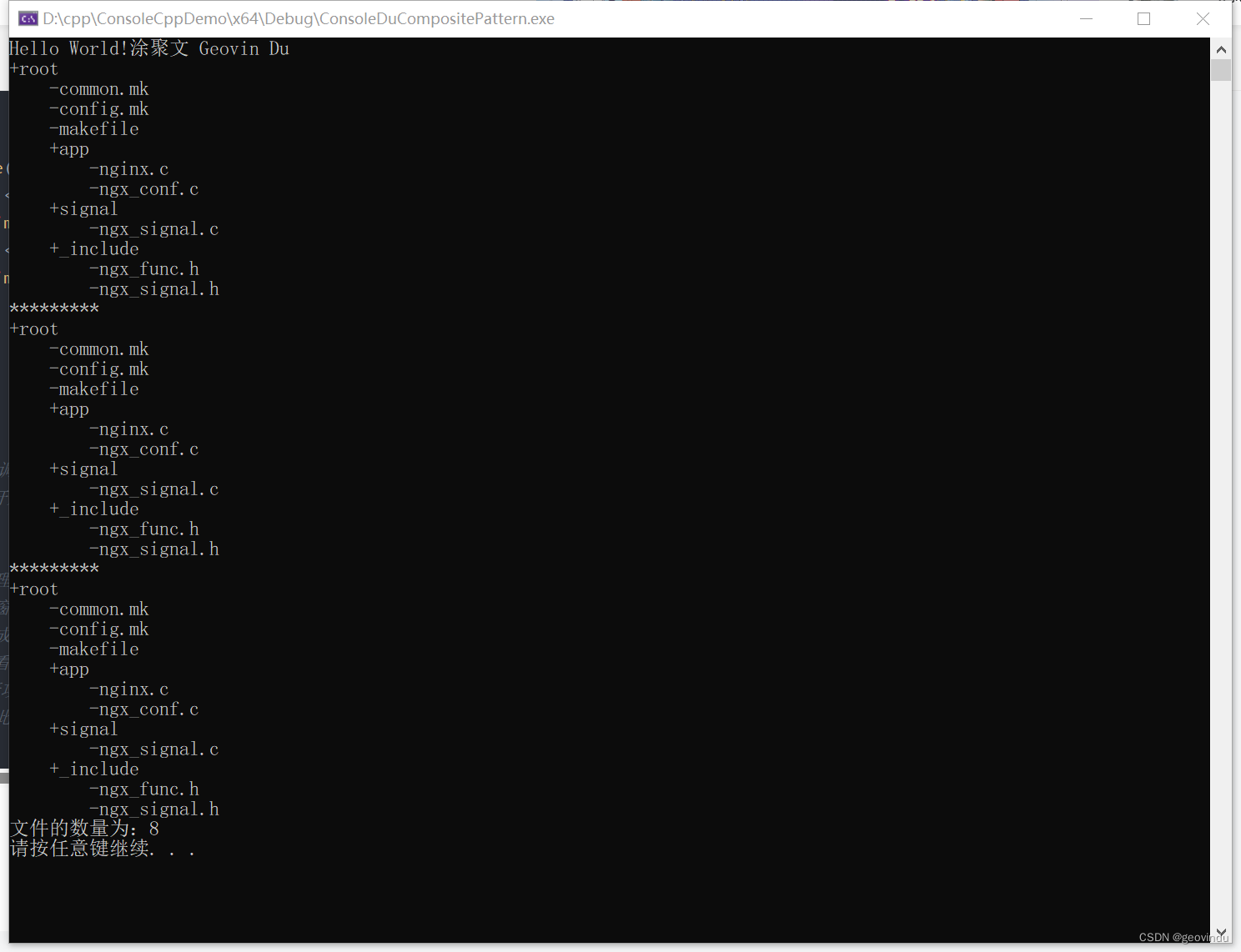