什么是BOM?
BOM(browser object model)浏览器对象模型
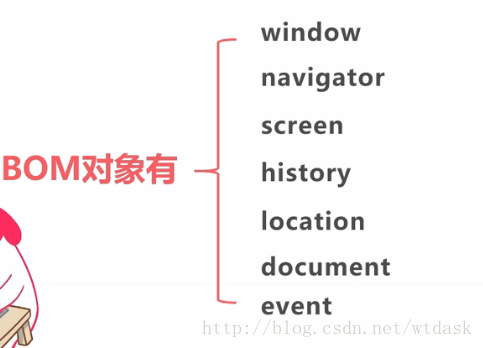
window对象
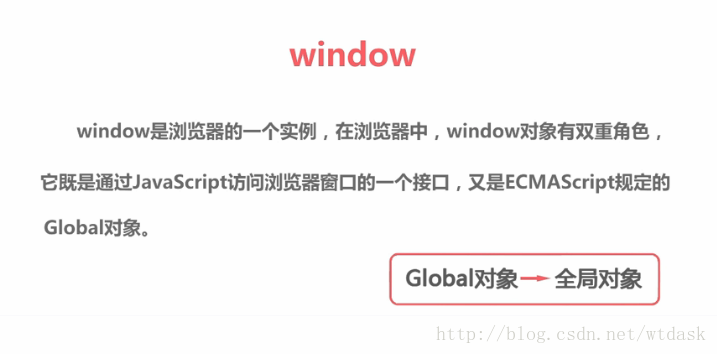
全局对象
说明:所有的全局变量和全局方法都被归为window上
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title></title>
</head>
<body>
<script>
var age=15;
function sayAge(){
alert('我'+window.age);
}
//声明一个全局变量
window.username="marry";//var username="marry";
//声明一个全局方法
window.sayName=function(){
alert("我是"+this.username);
}
sayAge();
window.sayName();
</script>
</body>
</html>
alert-confirm-prompt
语法:window.alert(“content”);
功能:显示带有一段消息和一个确认按钮的警告框。
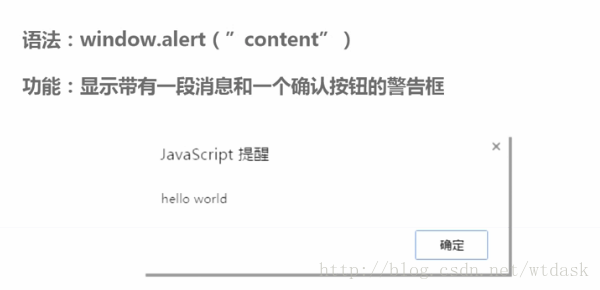
语法:window.confirm(“message”);
功能:显示一个带有指定消息和OK及取消按钮的对话框。
返回值:布尔型
如果用户点击确定按钮,则confirm()返回true
如果用户点击取消按钮,则confirm()返回false
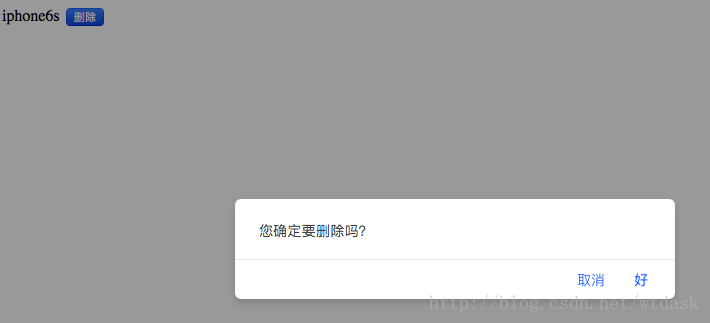
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title></title>
</head>
<body>
<div id="box">
<span>iphone6s</span>
<input type="button" value="删除" id="btn" />
</div>
<script>
//confirm
//获取按钮,绑定事件
var btn =document.getElementById("btn");
btn.onclick=function(){
//弹出确认对话框
var result=window.confirm("您确定要删除吗?该信息\n将不可恢复!");
console.log(result);
if(result){
document.getElementById("box").style.display="none";
}
}
</script>
</body>
</html>
语法:window.prompt(“text”,”defaultText”)
参数说明:
text:要在对话框中显示的纯文本(而不是HTML格式的文本)
defaultText:默认的输入文本
返回值:
如果用户点击提示框的取消按钮,则返回null
如果用户单击确认按钮,则返回输入字段当前显示的文本
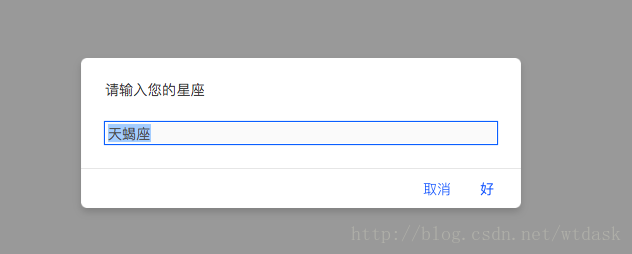
open和close
语法:window.open(pageURL,name,parameters)
功能:打开一个新的浏览器窗口或查找一个已命名的窗口
参数说明:
pageURL:子窗口路径
name:子窗口句柄(name声明了新窗口的名称,方便后期通过name对子窗口进行引用)
parameters:窗口参数(各参数用逗号分隔)
语法:window.close()
功能:关闭浏览器窗口
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>open</title>
</head>
<body>
<input type="button" value="退出" id="quit" />
<script>
window.onload=function(){
//打开子窗口,显示newWindow.html
window.open("newWindow.html","newWindow","width=400,height=200,left=0,tap=0,toolbar=no,menubar=no,scrollbars=no,location=no,status=no");
}
var quit =document.getElementById("quit");
//点击关闭当前窗口
quit.onclick=function(){
window.close();
}
</script>
</body>
</html>
定时器setTimeout:超时调用
超时调用:
语法:setTimeout(code,millisec)
功能:在指定的毫秒数后调用函数或计算表达式
参数说明:
1、code:要调用的函数或要执行的JavaScript代码串
2、millisec:在执行代码前需等待的毫秒数
说明:setTimeout方法返回一个ID值,通过它取消超时调用
setTimeout()只执行code一次。如果要多次调用,可以让code自身再次调用setTimeout()
清除超时调用
语法:clearTimeout(id_of_settimeout)
功能:取消由setTimeout()
方法设置的timeout
参数说明:
1、id_of_settimeout:由setTimeout()返回的ID值,该值标识要取消的延迟执行代码块
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title></title>
</head>
<body>
<script>
// setTimeout("alert('hello')",4000 );
var timeout1= setTimeout(function() {
alert("hello")
}, 4000);
var fnCall = function() {
alert("world");
}
// setTimeout(fnCall,6000);
//取消setTimeout
clearTimeout(timeout1);
</script>
</body>
</html>
定时器setInterval:间歇调用
语法:setInterval(code,millisec)
功能:每隔指定的时间执行一次代码
参数说明:
1、code:要调用的函数或要执行的代码串
2、millisec:周期性执行或调用code之间的时间间隔,以毫秒计
清除间歇调用
语法:clearInterval(id_of_setinterval)
功能:取消由setInterval()方法设置的interval
参数说明:
1、id_of_setinterval:由setInterval()返回的ID值,该值标识要取消的延迟执行代码块
<script>
var intervalId= setInterval(function(){
console.log("您好");
},1000);
//10秒之后停止打印
setTimeout(function(){
clearInterval(intervalId);
},10000);
</script>
说明:JavaScropt是单线程语言,单线程就是所执行的代码必须按照顺序。
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title></title>
</head>
<body>
<script>
// var intervalId= setInterval(function(){
// console.log("您好");
// },1000);
//
// //10秒之后停止打印
// setTimeout(function(){
// clearInterval(intervalId);
// },10000);
var num=1,
max=10,
timer=null;//null-释放内存
//每隔1秒钟num递增一次,直到num的值等于max清除
// timer=setInterval(function(){
// console.log(num);
// num++;
// if(num>max){
// clearInterval(timer);
// }
// },1000);
//使用超时调用实现
function increamentNum(){
console.log(num);
num++;
if(num<=max){
setTimeout(increamentNum,1000);
}else{
clearTimeout(timer);
}
}
timer=setTimeout(increamentNum,1000);
</script>
</body>
</html>
loaction对象
loaction对象提供了与当前窗口中加载的文档相关的信息,还提供了一些导航的功能,它既是window对象的属性,也是document对象的属性。
location对象的常用属性
功能:返回当前加载页面的完整URL
语法:loaction.href
说明:location.href与window.location.href等价
功能:返回URL中的hash(#号后跟零或多个字符),如果不包含则返回空字符串。
语法:location.hash
功能:返回服务器名称和端口号(如果有)
语法:location.host
功能:返回不带端口号的服务器名称
语法:location.hostname
功能:返回URL中的目录和(或)文件名
语法:location.pathname
功能:返回URL中指定的端口号,如果没有,返回空字符串
语法:location.port
功能:返回页面使用的协议
语法:location.protocol
功能:返回URL 的查询字符串,这个字符串以问号开头
语法:location.search
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title></title>
<style>
.box1{
height: 400px;
background: #ccc;
}
.box2{
height: 600px;
background: #666;
}
</style>
</head>
<body>
<div class="box1" id="top"></div>
<div class="box2"></div>
<input type="button" id="btn" value="返回顶部" />
<script>
// console.log(location.href); //返回当前加载页面的完整URL
// console.log(location.hash); //返回URL中的hash(#号后跟零或多个字符),如果不包含则返回空字符串。多用于锚点
var btn=document.getElementById("btn");
btn.onclick=function(){
location.hash="#top";
}
console.log(location.host);//返回服务器名称和端口号
console.log(location.hostname);//返回不带端口号的服务器名称
console.log(location.pathname);//返回URL中的目录和(或)文件名
console.log(location.port); //返回URL中指定的端口号,如果没有,返回空字符串
console.log(location.protocol);//返回页面使用的协议
console.log(location.search);//返回URL 的查询字符串,这个字符串以问号开头
</script>
</body>
</html>
location对象方法
位置操作
改变浏览器位置的方法:
location.href属性
location对象其他属性也可以改变URL:
location.hash
location.search
location.reaplace():重新定向URL
说明:使用location.replace不会在历史记录中生成新记录
location.reload():重新加载当前显示的页面。
说明:
* location.reload()有可能从缓存中加载
* location.reload(true)从服务器重新加载
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title></title>
</head>
<body>
<input type="button" value="刷新" id="reload" />
<script>
// setTimeout(function(){
location.href="BOM4.html";//留下浏览记录
// //window.location='BOM4.html'
// location.replace("BOM4.html");
// },2000);
document.getElementById("reload").onclick=function(){
location.reload(true);
}
</script>
</body>
</html>
history对象
history对象保存了用户在浏览器中访问页面的历史记录。
History历史对象
功能:回到历史记录的上一步
语法:history.back()
说明:相当于使用history.go(-1)
功能:回到历史记录的下一步
语法:location.forward()
说明:相当于使用了history.go(1)
功能:回到历史记录的前n步
语法:history.go(-n)
功能:回到历史记录的后n步
语法:history.go(n)
screen对象
Screen对象包含有关客户端显示屏幕的信息
语法:screen.availWidth
功能:返回可用的屏幕宽度
语法:screen.availHeight
功能:返回可用的屏幕高度
—获取窗口文件显示区的高度和宽度,可以使用innerHeight和innerWidth
<body>
<script>
console.log("页面宽:"+screen.availWidth);
console.log("页面高:"+screen.availHeight);
console.log("pageWidth:"+window.innerWidth);
console.log("pageHeight:"+window.innerHeight);
</script>
</body>
navigator对象
Navigator对象的userAgent属性:用来识别浏览器名称,版本,引擎以及操作系统等信息的内容
var explorer = navigator.userAgent;
alert(explorer);
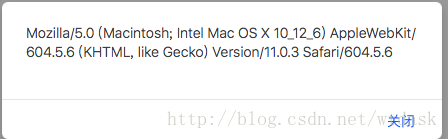
判断浏览器的类型
如何判断设备的终端是移动还是PC
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title></title>
</head>
<body>
<script>
//检测浏览器类型
function getBrowser(){
//获取userAgent属性
var explorer = navigator.userAgent.toLocaleLowerCase(),browser;
//indexOf()方法返回某个指定的字符串值在字符串首次出现的位置,如果没有出现过,返回-1
if(explorer.indexOf("msie")>-1){
browser="IE";
}else if(explorer.indexOf("chrome")>-1){
browser="chrome";
}else if(explorer.indexOf("opera")>-1){
browser="opera";
}else if(explorer.indexOf("safari")>-1){
browser="safari";
}
return browser;
}
var explorer = getBrowser();
console.log("您当前试用的是:"+explorer);
</script>
</body>
</html>