末尾获取源码
开发语言:python
框架:Django
数据库:MySQL5.7
开发软件:pycharm
目录
一、项目简介
二、系统功能
三、系统项目截图
3.1前台首页
3.2后台管理
四、核心代码
4.1登录相关
4.2文件上传
4.3封装
一、项目简介
新生入学管理系统利用网络沟通、计算机信息存储管理,有着与传统的方式所无法替代的优点。比如计算检索速度特别快、可靠性特别高、存储容量特别大、保密性特别好、可保存时间特别长、成本特别低等。在工作效率上,能够得到极大地提高,延伸至服务水平也会有好的收获,有了网络,新生入学管理系统的各方面的管理更加科学和系统,更加规范和简便。
二、系统功能
本新生入学管理系统主要包括两大功能模块,即用户功能模块和管理员功能模块。
(1)管理员模块:专业管理、班级管理、学生管理、商品分类管理、商品信息管理、新生签到管理、交流论坛、系统管理、订单管理。
(2)学生:商品信息、交流论坛、报到指南、个人中心、后台管理、购物车。
三、系统项目截图
3.1前台首页
前台注册页面,未有账号的学生可在该页面进行注册并且登录。
登录到前台我们可以看到有以下图片的相关功能模块
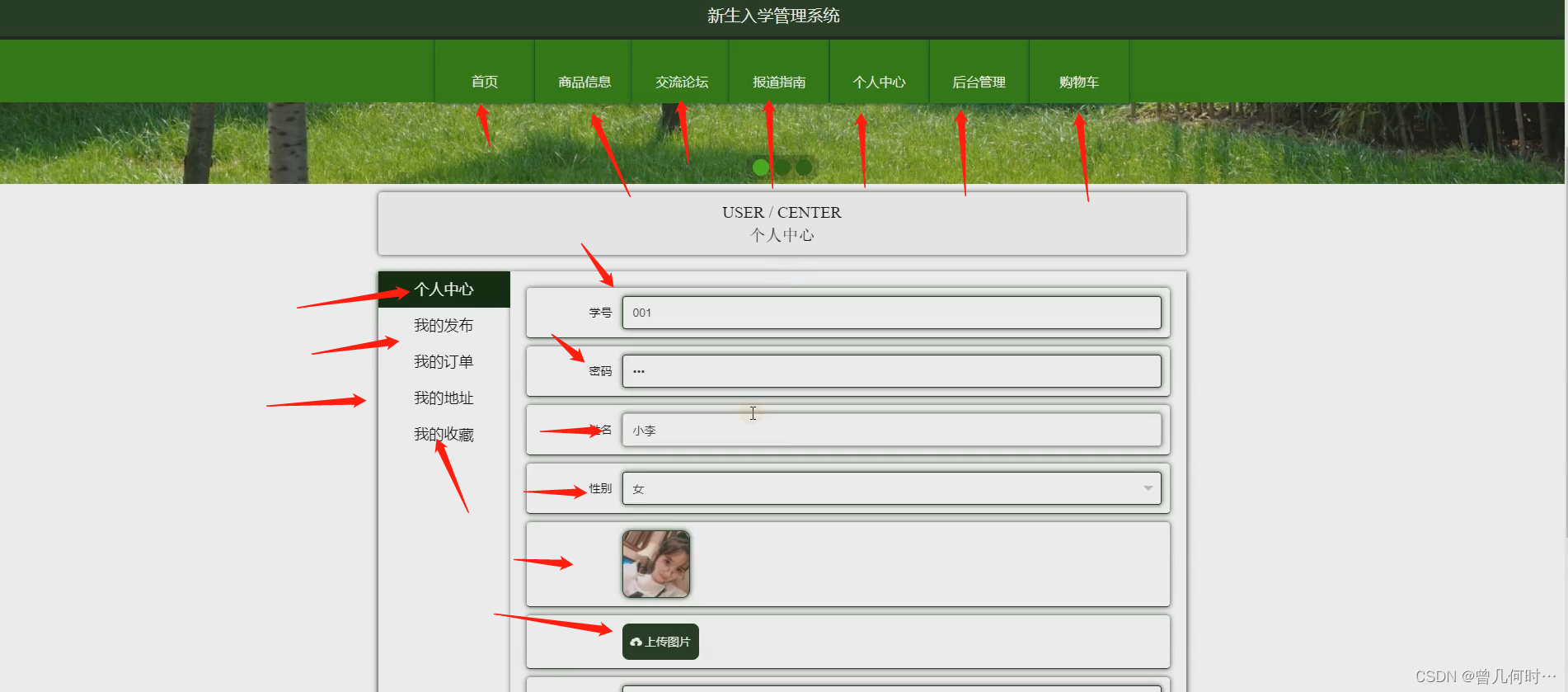
在报到指南我们可以查看学校发布的一些入校指南文章
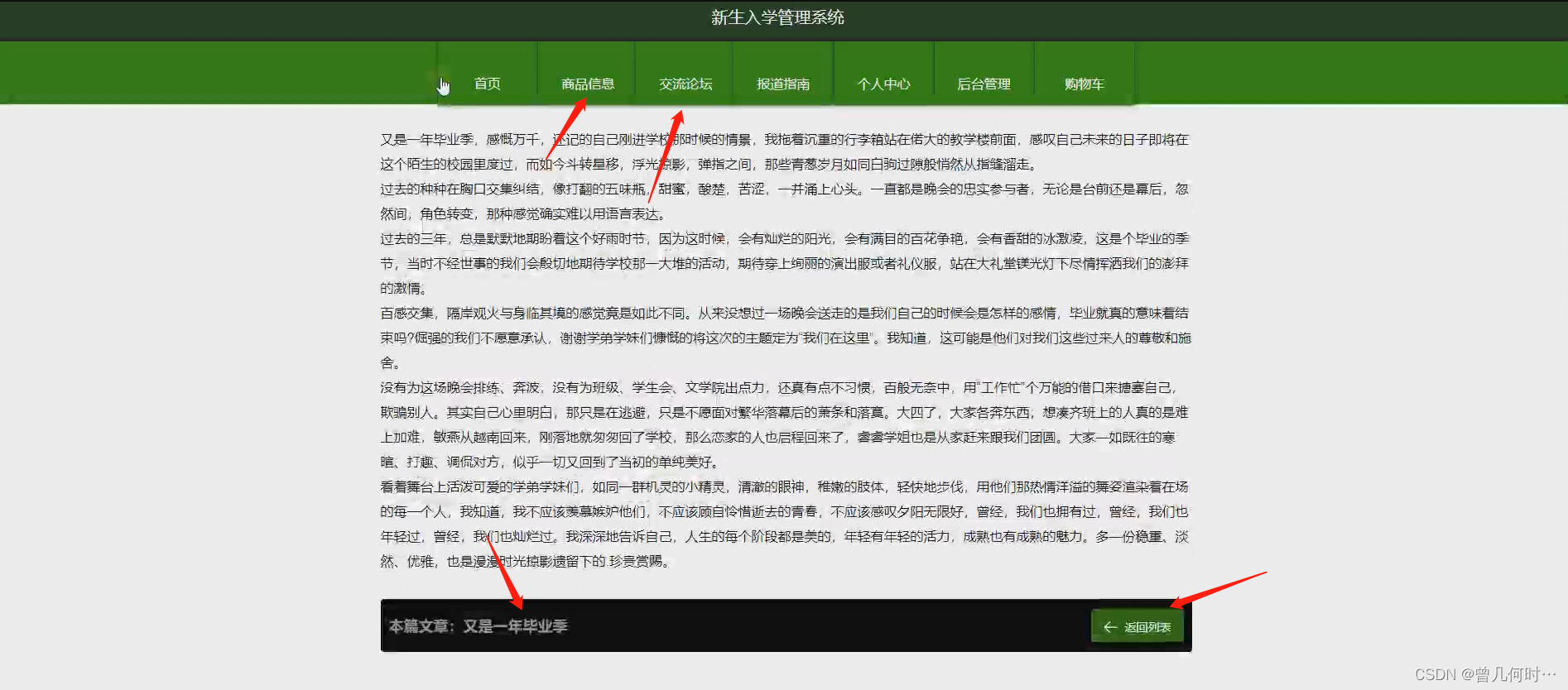
选择想看的入校指南文章即可查看
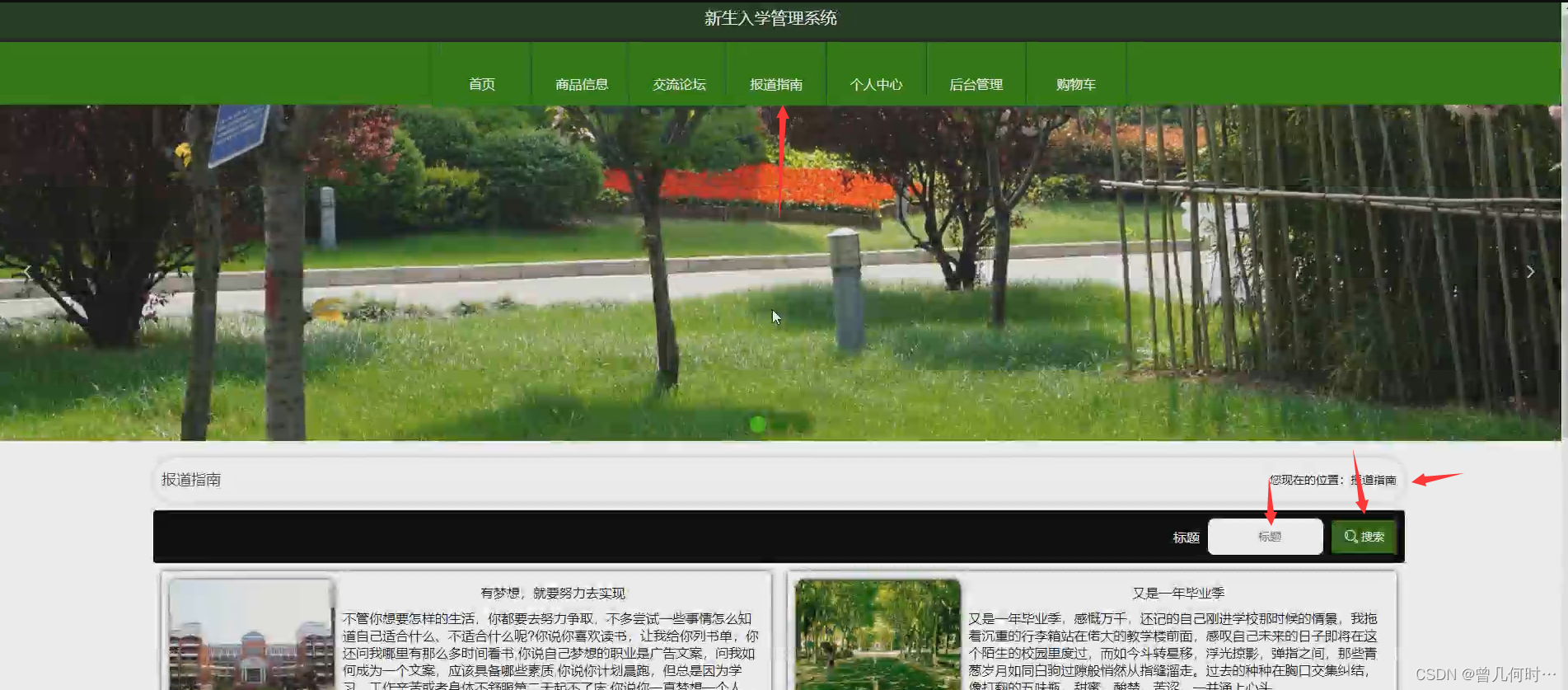
入校我们可能需要一些商品,可以选择自己的生活所需品可购买下单
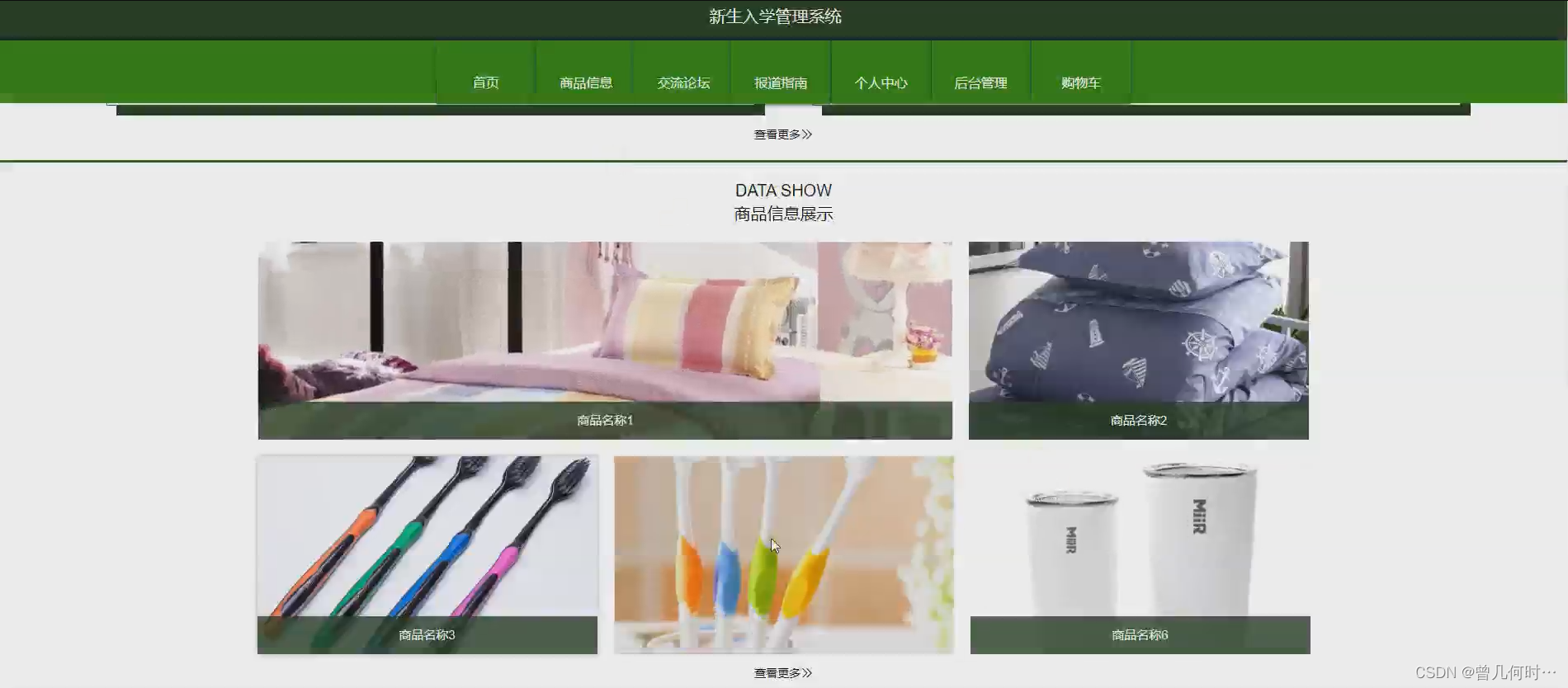
点击相关的商品我们可以查看商品的信息和价格 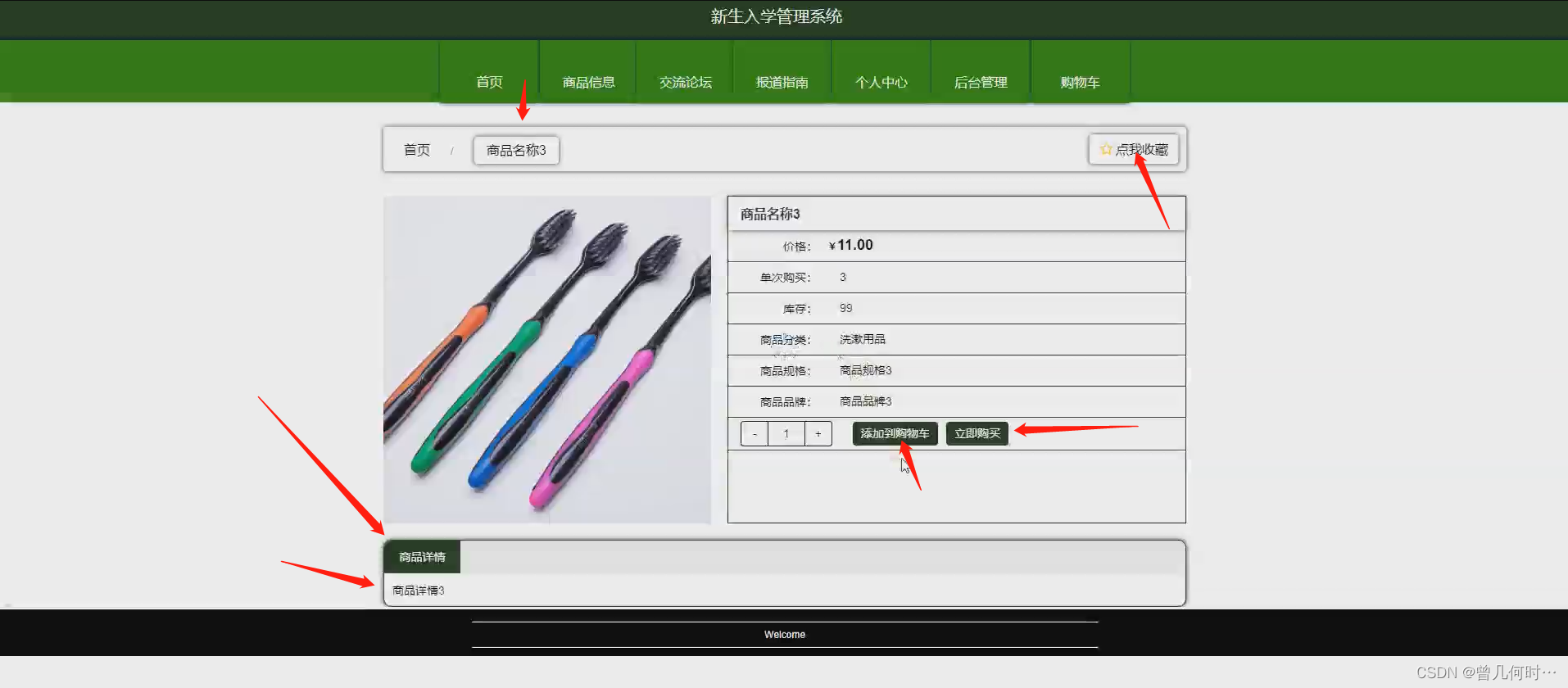
下单我们可以选择地址然后点击支付
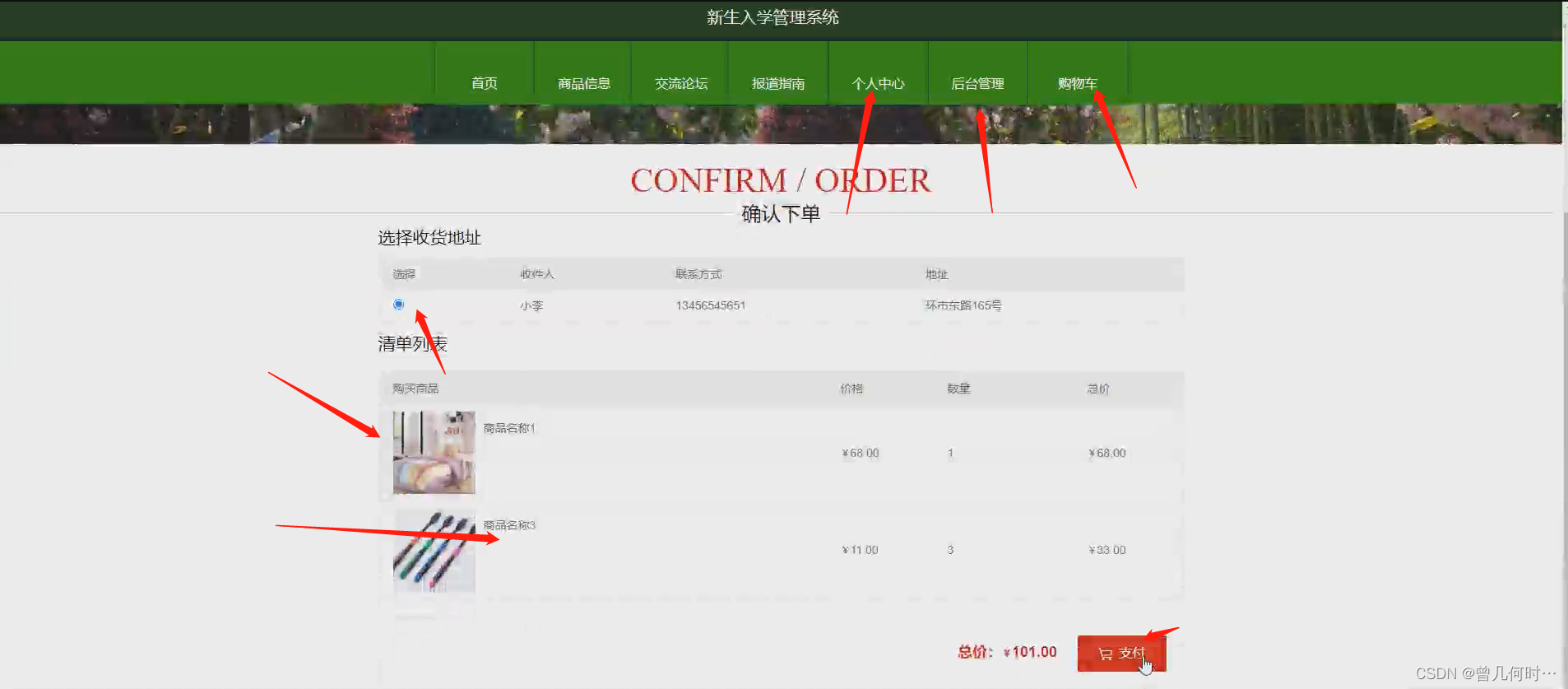
3.2后台管理
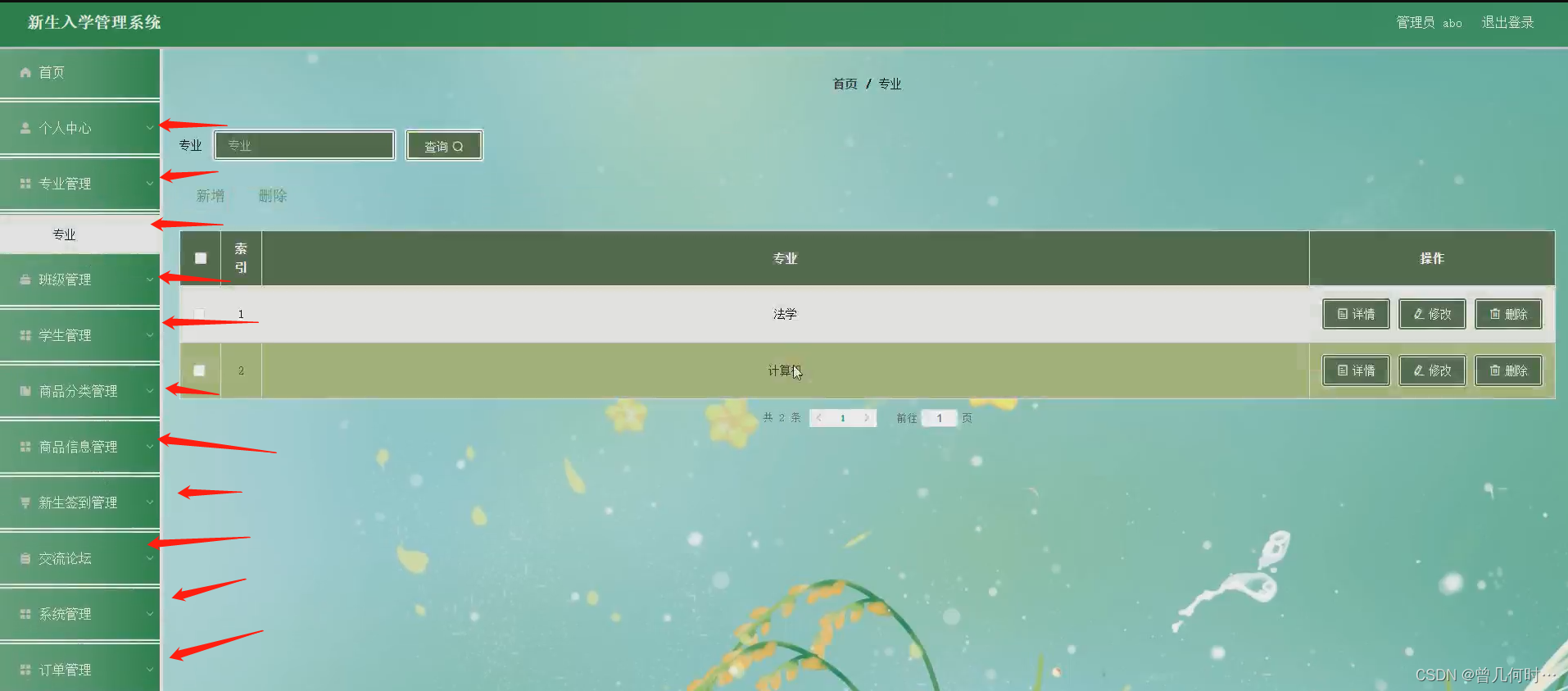
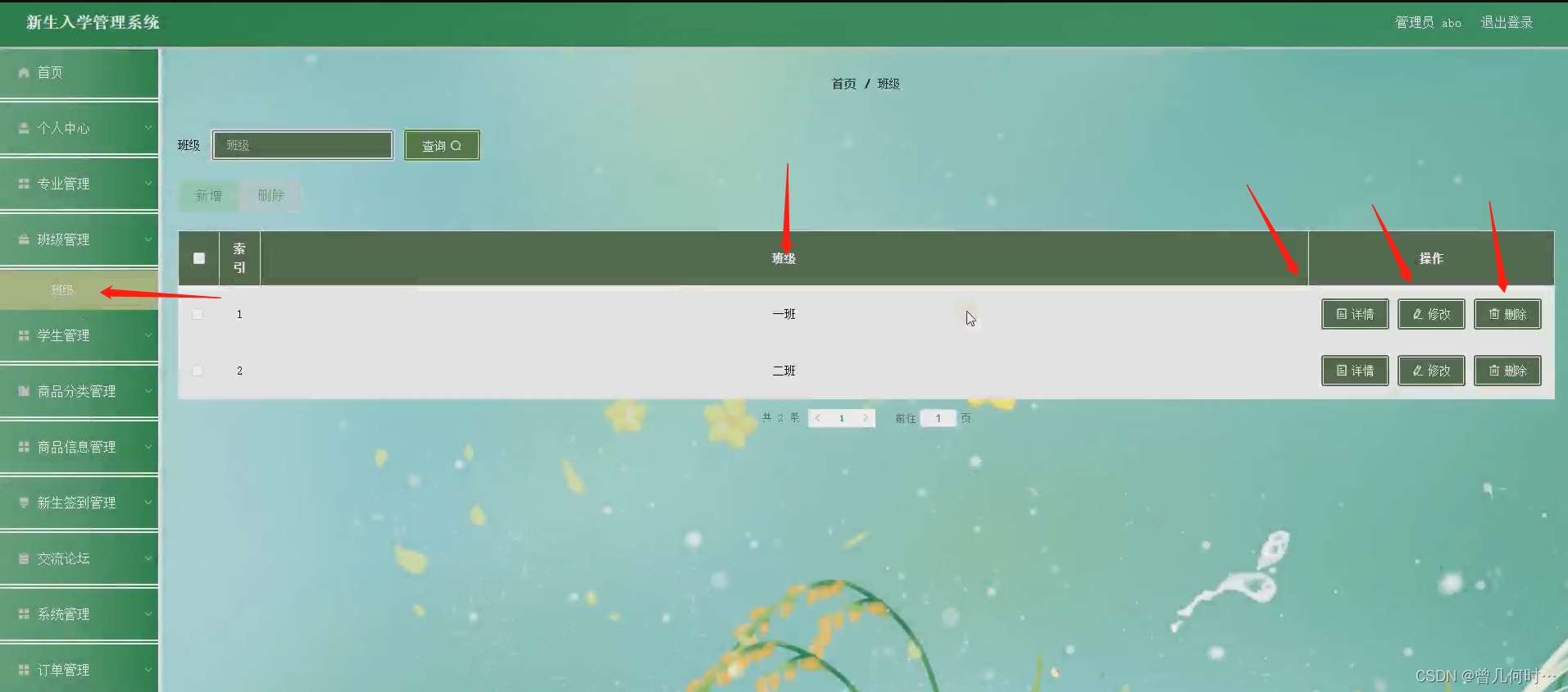
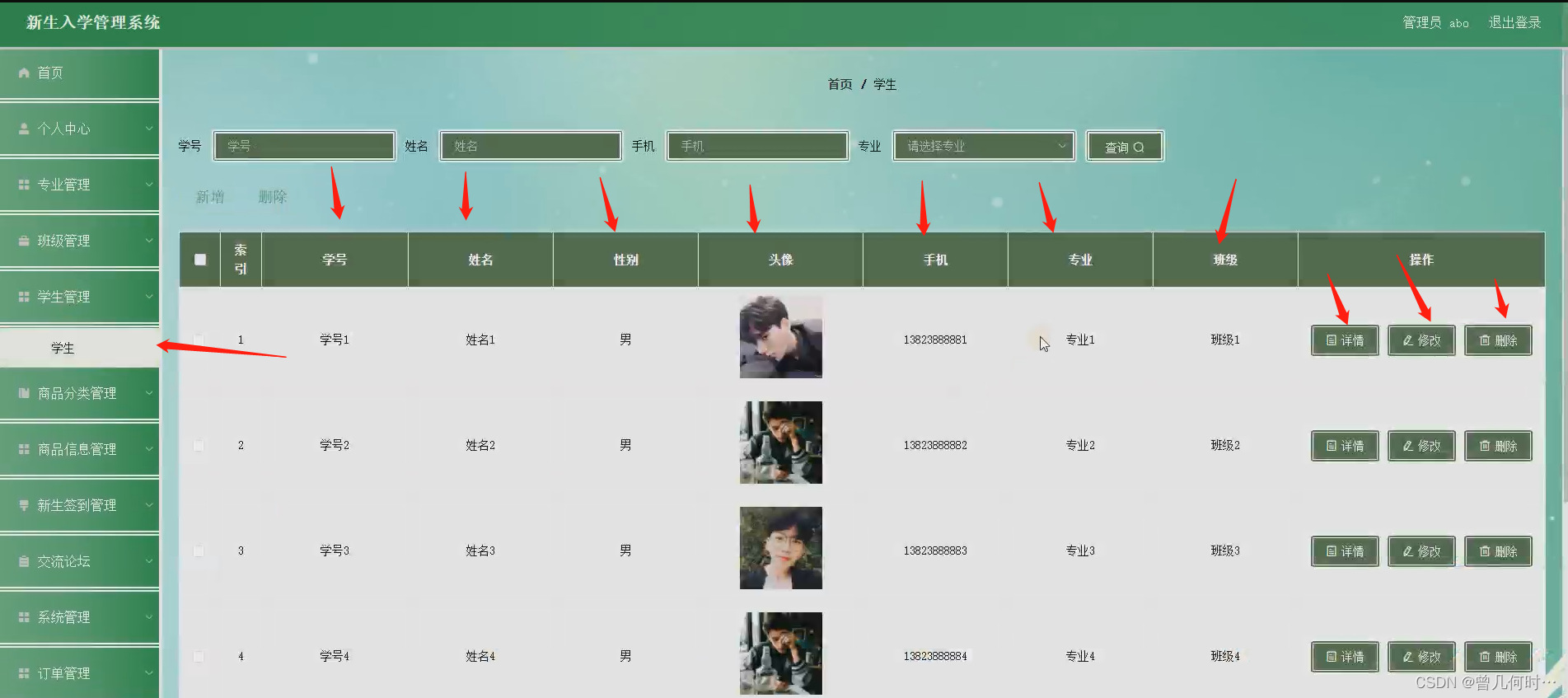
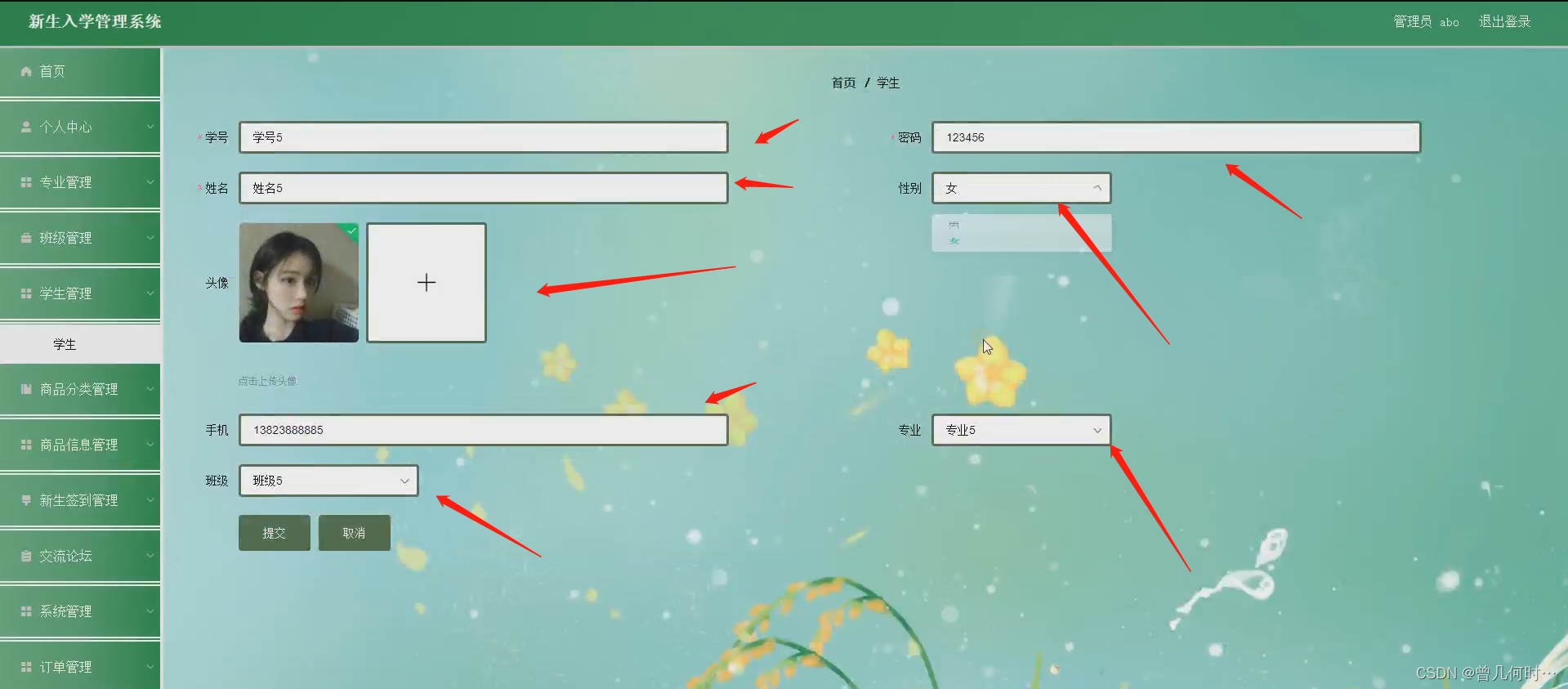
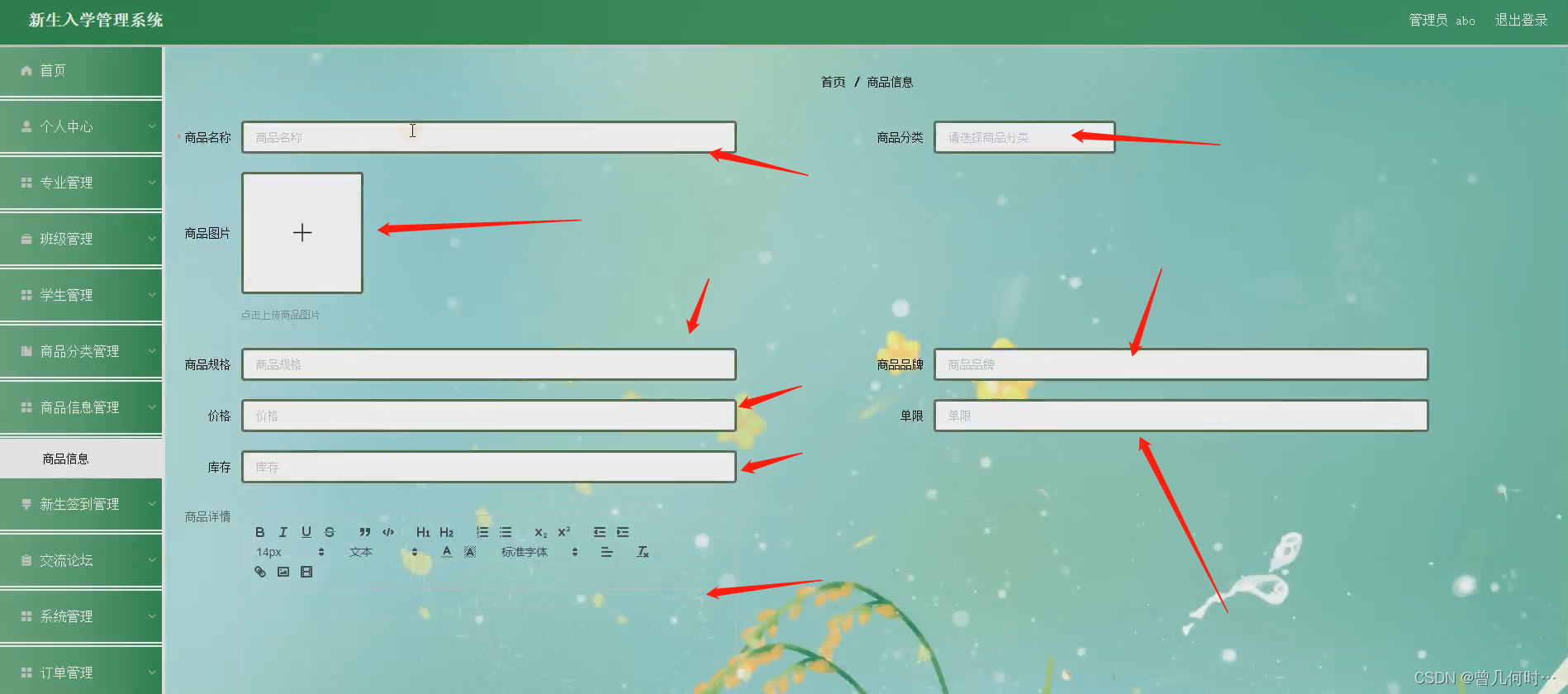
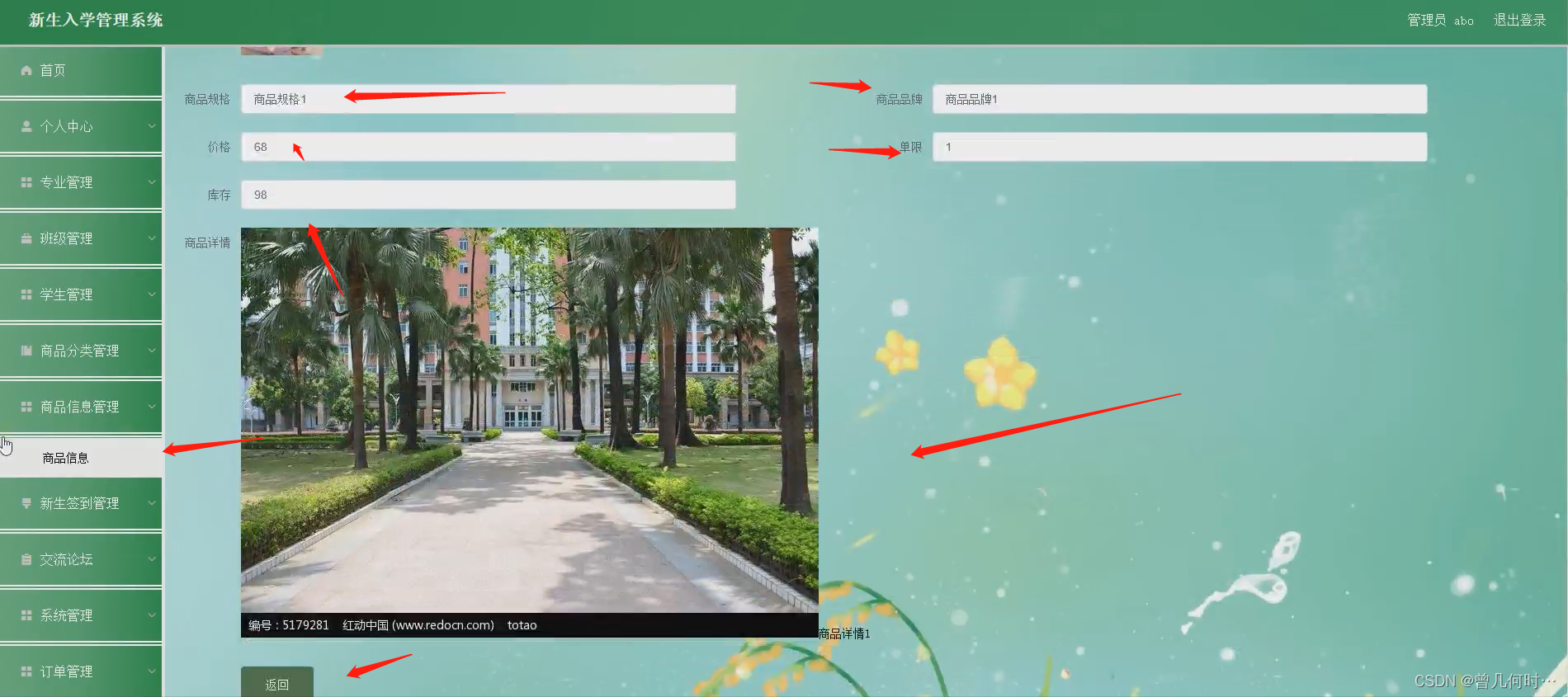
四、核心代码
4.1登录相关
package com.controller;
import java.util.Arrays;
import java.util.Calendar;
import java.util.Date;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.bind.annotation.RestController;
import com.annotation.IgnoreAuth;
import com.baomidou.mybatisplus.mapper.EntityWrapper;
import com.entity.TokenEntity;
import com.entity.UserEntity;
import com.service.TokenService;
import com.service.UserService;
import com.utils.CommonUtil;
import com.utils.MD5Util;
import com.utils.MPUtil;
import com.utils.PageUtils;
import com.utils.R;
import com.utils.ValidatorUtils;
/**
* 登录相关
*/
@RequestMapping("users")
@RestController
public class UserController{
@Autowired
private UserService userService;
@Autowired
private TokenService tokenService;
/**
* 登录
*/
@IgnoreAuth
@PostMapping(value = "/login")
public R login(String username, String password, String captcha, HttpServletRequest request) {
UserEntity user = userService.selectOne(new EntityWrapper<UserEntity>().eq("username", username));
if(user==null || !user.getPassword().equals(password)) {
return R.error("账号或密码不正确");
}
String token = tokenService.generateToken(user.getId(),username, "users", user.getRole());
return R.ok().put("token", token);
}
/**
* 注册
*/
@IgnoreAuth
@PostMapping(value = "/register")
public R register(@RequestBody UserEntity user){
// ValidatorUtils.validateEntity(user);
if(userService.selectOne(new EntityWrapper<UserEntity>().eq("username", user.getUsername())) !=null) {
return R.error("用户已存在");
}
userService.insert(user);
return R.ok();
}
/**
* 退出
*/
@GetMapping(value = "logout")
public R logout(HttpServletRequest request) {
request.getSession().invalidate();
return R.ok("退出成功");
}
/**
* 密码重置
*/
@IgnoreAuth
@RequestMapping(value = "/resetPass")
public R resetPass(String username, HttpServletRequest request){
UserEntity user = userService.selectOne(new EntityWrapper<UserEntity>().eq("username", username));
if(user==null) {
return R.error("账号不存在");
}
user.setPassword("123456");
userService.update(user,null);
return R.ok("密码已重置为:123456");
}
/**
* 列表
*/
@RequestMapping("/page")
public R page(@RequestParam Map<String, Object> params,UserEntity user){
EntityWrapper<UserEntity> ew = new EntityWrapper<UserEntity>();
PageUtils page = userService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.allLike(ew, user), params), params));
return R.ok().put("data", page);
}
/**
* 列表
*/
@RequestMapping("/list")
public R list( UserEntity user){
EntityWrapper<UserEntity> ew = new EntityWrapper<UserEntity>();
ew.allEq(MPUtil.allEQMapPre( user, "user"));
return R.ok().put("data", userService.selectListView(ew));
}
/**
* 信息
*/
@RequestMapping("/info/{id}")
public R info(@PathVariable("id") String id){
UserEntity user = userService.selectById(id);
return R.ok().put("data", user);
}
/**
* 获取用户的session用户信息
*/
@RequestMapping("/session")
public R getCurrUser(HttpServletRequest request){
Long id = (Long)request.getSession().getAttribute("userId");
UserEntity user = userService.selectById(id);
return R.ok().put("data", user);
}
/**
* 保存
*/
@PostMapping("/save")
public R save(@RequestBody UserEntity user){
// ValidatorUtils.validateEntity(user);
if(userService.selectOne(new EntityWrapper<UserEntity>().eq("username", user.getUsername())) !=null) {
return R.error("用户已存在");
}
userService.insert(user);
return R.ok();
}
/**
* 修改
*/
@RequestMapping("/update")
public R update(@RequestBody UserEntity user){
// ValidatorUtils.validateEntity(user);
userService.updateById(user);//全部更新
return R.ok();
}
/**
* 删除
*/
@RequestMapping("/delete")
public R delete(@RequestBody Long[] ids){
userService.deleteBatchIds(Arrays.asList(ids));
return R.ok();
}
}
4.2文件上传
package com.controller;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.util.Arrays;
import java.util.Date;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Random;
import java.util.UUID;
import org.apache.commons.io.FileUtils;
import org.apache.commons.lang3.StringUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpStatus;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.util.ResourceUtils;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.multipart.MultipartFile;
import com.annotation.IgnoreAuth;
import com.baomidou.mybatisplus.mapper.EntityWrapper;
import com.entity.ConfigEntity;
import com.entity.EIException;
import com.service.ConfigService;
import com.utils.R;
/**
* 上传文件映射表
*/
@RestController
@RequestMapping("file")
@SuppressWarnings({"unchecked","rawtypes"})
public class FileController{
@Autowired
private ConfigService configService;
/**
* 上传文件
*/
@RequestMapping("/upload")
public R upload(@RequestParam("file") MultipartFile file,String type) throws Exception {
if (file.isEmpty()) {
throw new EIException("上传文件不能为空");
}
String fileExt = file.getOriginalFilename().substring(file.getOriginalFilename().lastIndexOf(".")+1);
File path = new File(ResourceUtils.getURL("classpath:static").getPath());
if(!path.exists()) {
path = new File("");
}
File upload = new File(path.getAbsolutePath(),"/upload/");
if(!upload.exists()) {
upload.mkdirs();
}
String fileName = new Date().getTime()+"."+fileExt;
File dest = new File(upload.getAbsolutePath()+"/"+fileName);
file.transferTo(dest);
FileUtils.copyFile(dest, new File("C:\\Users\\Desktop\\jiadian\\springbootl7own\\src\\main\\resources\\static\\upload"+"/"+fileName));
if(StringUtils.isNotBlank(type) && type.equals("1")) {
ConfigEntity configEntity = configService.selectOne(new EntityWrapper<ConfigEntity>().eq("name", "faceFile"));
if(configEntity==null) {
configEntity = new ConfigEntity();
configEntity.setName("faceFile");
configEntity.setValue(fileName);
} else {
configEntity.setValue(fileName);
}
configService.insertOrUpdate(configEntity);
}
return R.ok().put("file", fileName);
}
/**
* 下载文件
*/
@IgnoreAuth
@RequestMapping("/download")
public ResponseEntity<byte[]> download(@RequestParam String fileName) {
try {
File path = new File(ResourceUtils.getURL("classpath:static").getPath());
if(!path.exists()) {
path = new File("");
}
File upload = new File(path.getAbsolutePath(),"/upload/");
if(!upload.exists()) {
upload.mkdirs();
}
File file = new File(upload.getAbsolutePath()+"/"+fileName);
if(file.exists()){
/*if(!fileService.canRead(file, SessionManager.getSessionUser())){
getResponse().sendError(403);
}*/
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_OCTET_STREAM);
headers.setContentDispositionFormData("attachment", fileName);
return new ResponseEntity<byte[]>(FileUtils.readFileToByteArray(file),headers, HttpStatus.CREATED);
}
} catch (IOException e) {
e.printStackTrace();
}
return new ResponseEntity<byte[]>(HttpStatus.INTERNAL_SERVER_ERROR);
}
}
4.3封装
package com.utils;
import java.util.HashMap;
import java.util.Map;
/**
* 返回数据
*/
public class R extends HashMap<String, Object> {
private static final long serialVersionUID = 1L;
public R() {
put("code", 0);
}
public static R error() {
return error(500, "未知异常,请联系管理员");
}
public static R error(String msg) {
return error(500, msg);
}
public static R error(int code, String msg) {
R r = new R();
r.put("code", code);
r.put("msg", msg);
return r;
}
public static R ok(String msg) {
R r = new R();
r.put("msg", msg);
return r;
}
public static R ok(Map<String, Object> map) {
R r = new R();
r.putAll(map);
return r;
}
public static R ok() {
return new R();
}
public R put(String key, Object value) {
super.put(key, value);
return this;
}
}