一、效果演示
利用html,css,js制作出飞机大战的简易版
需要代码的小伙伴可以在文章末尾资源自行下载,下载打开运行在浏览器即可游戏,本篇资源分享分为两个版本,一个是键盘版本(awsd移动和k攻击),一个是鼠标移动版
设计思路
-
在游戏进行界面中,通过鼠标操控自己方飞机(仅一架),在初始化时就进行无限制的匀速发射子弹。
-
在游戏进行界面中,左上方有得到的分数显示,敌方飞机有三种类型(小,中,大),他们的形状,大小,击落的分数各不同,以合适的速度控制它们的数量,以及运动的速度。
-
在进行游戏的过程中点击鼠标左键会进入游戏暂停界面,有重新开始,回到主页,继续三个选项。当游戏结束时,会弹出游戏分数框,有继续按钮,点击回到主页。
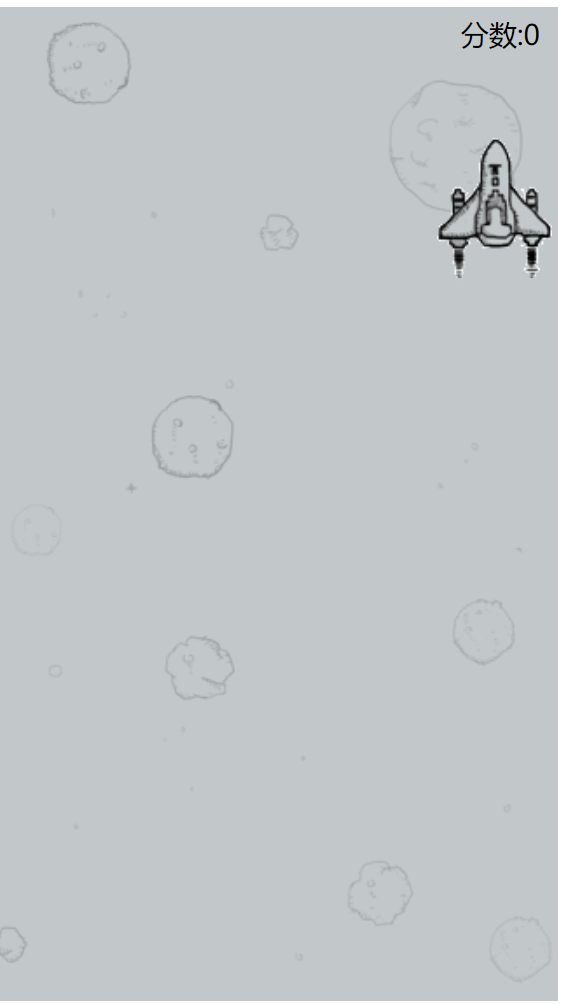
二、鼠标版飞机大战代码展示
1.HTML结构代码
<body>
<div id="plane">
<span>分数:<strong style="font-weight: normal;">0</strong></span>
</div>
<script type="text/javascript" src="js.js"></script>
<script type="text/javascript" src="plane.js"></script>
</body>
2.CSS样式代码
<style type="text/css">
*{
padding:0px;
margin:0px;
}
#plane{
width: 320px;
height: 568px;
background: url(img/background.png);
position: relative;
margin:50px auto 0;
cursor: none;
overflow: hidden;
}
#plane span{
position: absolute;
right:10px;
top:5px;
}
</style>
3.JavaScript代码
js.js文件
//缓冲运动
function getStyle(obj, attr) {
if (obj.currentStyle) {
return obj.currentStyle[attr];
} else {
return getComputedStyle(obj)[attr];
}
}
function startMove(obj, json, fn) {
var cur = 0;
var timer = null;
var speed = null;
clearInterval(obj.timer)
obj.timer = setInterval(function() {
var bstop = true;
for (var attr in json) {
if (attr == 'opacity') {//求初始值
cur = Math.round(getStyle(obj, attr) * 100);
} else {
cur = parseInt(getStyle(obj, attr));
}
speed = (json[attr] - cur) / 8;
speed = speed > 0 ? Math.ceil(speed) : Math.floor(speed);
if (cur != json[attr]) {
bstop = false;
if (attr == 'opacity') {
obj.style[attr] = (cur + speed) / 100;
obj.style.filter = 'alpha(opacity=' + (cur + speed) + ')';
} else {
obj.style[attr] = cur + speed + 'px';
}
}
}
if (bstop) {
clearInterval(obj.timer);
fn && fn();
}
}, 30);
}
//通过类名获取元素
function getClass(oClass, oParent) {
var oP = oParent || document;
var arr = [];
var aEle = oP.getElementsByTagName('*');
var reg = new RegExp('\\b' + oClass + '\\b');
for (var i = 0; i < aEle.length; i++) {
if (reg.test(aEle[i].className)) {
arr.push(aEle[i]);
}
}
return arr;
}
//取任意的随机数,范围是min-max之间
function getrandom(min,max){
return Math.floor(Math.random()*(max-min+1))+min;
}
plane.js文件
//我方飞机构造函数
var planeBox = document.getElementById('plane');
var planescore = document.getElementsByTagName('strong')[0];
var zscore = 0;
function Myplane(w, h, imgsrc, boomsrc) {
this.w = w;
this.h = h;
this.imgsrc = imgsrc;
this.boomsrc = boomsrc;
this.createMyplane();
}
//创建我方飞机
Myplane.prototype.createMyplane = function() {
this.plane = document.createElement('img');
this.plane.src = this.imgsrc;
this.plane.style.cssText = 'width:' + this.w + 'px;height:' + this.h + 'px;position:absolute;left:' + (planeBox.offsetWidth - this.w) / 2 + 'px;top:' + (planeBox.offsetHeight - this.h) + 'px;';
planeBox.appendChild(this.plane);
this.move();
this.shoot();
};
//我方飞机移动
Myplane.prototype.move = function() {
var that = this;
document.onmousemove = function(ev) {
var ev = ev || window.event;
that.oLeft = ev.clientX - plane.offsetLeft - that.w / 2;
that.oTop = ev.clientY - plane.offsetTop - that.h / 2;
if (that.oLeft < 0) {
that.oLeft = 0;
} else if (that.oLeft >= plane.offsetWidth - that.w) {
that.oLeft = plane.offsetWidth - that.w
}
if (that.oTop < 0) {
that.oTop = 0;
} else if (that.oTop >= plane.offsetHeight - that.h) {
that.oTop = plane.offsetHeight - that.h;
}
that.plane.style.left = that.oLeft + 'px';
that.plane.style.top = that.oTop + 'px';
return false;
}
};
//我方飞机发射子弹
Myplane.prototype.shoot = function() {
var that = this;
document.onmousedown = function(ev) {
var ev = ev || window.event;
if (ev.which == 1) {
bulletshoot();
that.timer = setInterval(bulletshoot, 200);
function bulletshoot() {
var bullet = new Bullet(that.plane.offsetLeft + that.w / 2 - 3, that.plane.offsetTop - 14, 6, 14, 'img/bullet.png');
}
}
return false;
}
document.onmouseup = function(ev) {
var ev = ev || window.event;
if (ev.which == 1) {
clearInterval(that.timer);
}
}
document.oncontextmenu = function() {
return false;
}
};
//子弹的构造函数
function Bullet(x, y, w, h, imgsrc) {
this.x = x; //位置
this.y = y;
this.w = w; //尺寸
this.h = h;
this.imgsrc = imgsrc;
this.createBullet();
}
//创建子弹
Bullet.prototype.createBullet = function() {
this.bullet = document.createElement('img');
this.bullet.src = this.imgsrc;
this.bullet.style.cssText = 'width:' + this.w + 'px;height:' + this.h + 'px;position:absolute;left:' + this.x + 'px;top:' + this.y + 'px;';
planeBox.appendChild(this.bullet);
this.move();
}
//子弹移动
Bullet.prototype.move = function() {
var that = this;
this.timer = setInterval(function() {
that.y -= 5;
if (that.y <= -that.h) {
clearInterval(that.timer);
planeBox.removeChild(that.bullet);
}
that.bullet.style.top = that.y + 'px';
that.hit();
}, 20);
};
Bullet.prototype.hit = function() {
var allEnemy = document.getElementsByClassName('planeEnemy');
for (var i = 0; i < allEnemy.length; i++) {
if ((this.x + this.w) >= allEnemy[i].offsetLeft && this.x <= (allEnemy[i].offsetLeft + allEnemy[i].offsetWidth) && this.y <= (allEnemy[i].offsetTop + allEnemy[i].offsetHeight)) {
clearInterval(this.timer);
try{
planeBox.removeChild(this.bullet);
}catch(e){
}
allEnemy[i].hp--;
allEnemy[i].checkhp();
}
}
};
//敌方飞机的构造函数
function Enemyplane(x, y, w, h, imgsrc, boomsrc, speed, hp, score) {
this.x = x; //位置
this.y = y;
this.w = w; //尺寸
this.h = h;
this.imgsrc = imgsrc;
this.boomsrc = boomsrc;
this.speed = speed;
this.hp = hp;
this.score = score;
this.createEnemy();
}
//创建敌机
Enemyplane.prototype.createEnemy = function() {
var that = this;
this.enemy = document.createElement('img');
this.enemy.src = this.imgsrc;
this.enemy.className = 'planeEnemy'; //通过设置类名,方便后面获取
this.enemy.style.cssText = 'width:' + this.w + 'px;height:' + this.h + 'px;position:absolute;left:' + this.x + 'px;top:' + this.y + 'px;';
planeBox.appendChild(this.enemy);
this.enemy.hp = this.hp; //变化的
this.enemy.score = this.score; //变化的
this.enemy.checkhp = function() { //this==this.enemy
if (this.hp <= 0) {
clearInterval(this.timer);
this.className = '';
this.src = that.boomsrc;
setTimeout(function() {
planeBox.removeChild(that.enemy);
}, 1000);
zscore += this.score
planescore.innerHTML = zscore;
}
}
this.move();
}
Enemyplane.prototype.move = function() {
var that = this;
this.enemy.timer = setInterval(function() {
that.y += that.speed;
if (that.y >= planeBox.offsetHeight) {
clearInterval(that.enemy.timer);
planeBox.removeChild(that.enemy);
}
that.enemy.style.top = that.y + 'px';
that.enemyhit();
}, 50);
}
Enemyplane.prototype.enemyhit = function() {
if ((this.y + this.h) >= myplane.oTop && this.y <= (myplane.oTop + myplane.h) && (this.x + this.w) > myplane.oLeft && this.x <= (myplane.oLeft + myplane.w)) {
var allEnemy = document.getElementsByClassName('planeEnemy');
for (var i = 0; i < allEnemy.length; i++) {
clearInterval(allEnemy[i].timer);
}
clearInterval(timer);
document.onmousedown = null;
document.onmousemove = null;
myplane.plane.src = myplane.boomsrc;
setTimeout(function() {
alert('game over!!');
window.location.reload(); //重刷新
}, 3000);
}
}
var myplane = new Myplane(66, 80, 'img/myplane.gif', 'img/myplaneBoom.gif');
for (var i = 0; i < getrandom(1, 2); i++) { //随机创建各类飞机
var timer = setInterval(function() {
var num = getrandom(1, 20); //1-15 小飞机 16-20 中飞机 20打飞机
if (num >= 1 && num < 15) {
var enemy = new Enemyplane(getrandom(0, planeBox.offsetWidth - 34), -24, 34, 24, 'img/smallplane.png', 'img/smallplaneboom.gif', getrandom(3, 5), 1, 1);
} else if (num >= 15 && num < 20) {
var enemy = new Enemyplane(getrandom(0, planeBox.offsetWidth - 46), -60, 46, 60, 'img/midplane.png', 'img/midplaneboom.gif', getrandom(2, 4), 3, 5);
} else if (num == 20) {
var enemy = new Enemyplane(getrandom(0, planeBox.offsetWidth - 110), -164, 110, 164, 'img/bigplane.png', 'img/bigplaneboom.gif', getrandom(1, 2), 10, 10);
}
}, 1000)
}
三、键盘版飞机大战代码展示
1.HTML结构代码
<body>
<div class="gamebox">
<span>分数:<em>0</em></span>
</div>
<script type="text/javascript" src="plane.js"></script>
</body>
2.CSS样式代码
<style type="text/css">
* {
padding: 0px;
margin: 0px;
}
.gamebox{
width: 320px;
height:568px;
background: url(img/background.png) repeat-y;
margin:20px auto;
position: relative;
cursor: none;
overflow: hidden;
}
.gamebox span{
position: absolute;
right:10px;
top:10px;
}
.gamebox span em{
font-style: normal;
}
</style>
3.JavaScript代码
;
(function() {
var gamebox = document.querySelector('.gamebox');
var oEm = document.querySelector('em');
var zscore = 0;
//1.让背景运动起来
var bgposition = 0;
var bgtimer = setInterval(function() {
bgposition += 2;
gamebox.style.backgroundPosition = '0 ' + bgposition + 'px';
}, 30);
//2.我方飞机的构造函数
function Myplane(w, h, x, y, imgurl, boomurl) { //w,h宽高 x,y位置 imgurl和boomurl我方飞机的图片路径
this.w = w;
this.h = h;
this.x = x;
this.y = y;
this.imgurl = imgurl;
this.boomurl = boomurl;
this.createmyplane()
}
//2.1创建我方飞机
Myplane.prototype.createmyplane = function() {
this.myplaneimg = document.createElement('img');
this.myplaneimg.src = this.imgurl;
this.myplaneimg.style.cssText = `width:${this.w}px;height:${this.h}px;position:absolute;left:${this.x}px;top:${this.y}px;`;
gamebox.appendChild(this.myplaneimg);
//飞机创建完成,执行运动和发射子弹
this.myplanemove();
this.myplaneshoot();
}
//2.2键盘控制我方飞机移动
Myplane.prototype.myplanemove = function() {
var that = this;
//方向定时器
var uptimer = null,
downtimer = null,
lefttimer = null,
righttimer = null;
var uplock = true,
downlock = true,
leftlock = true,
rightlock = true;
document.addEventListener('keydown', movekey, false); //movekey:事件处理函数
function movekey(ev) { //W:87 A:65 S:83 D:68 K:75
var ev = ev || window.event;
switch (ev.keyCode) {
case 87:
moveup(); // 上
break;
case 83:
movedown(); // 下
break;
case 65:
moveleft(); // 左
break;
case 68:
moveright(); // 右
break;
}
function moveup() {
if (uplock) {
uplock = false;
clearInterval(downtimer);
uptimer = setInterval(function() {
that.y -= 4;
if (that.y <= 0) {
that.y = 0;
}
that.myplaneimg.style.top = that.y + 'px';
}, 30);
}
}
function movedown() {
if (downlock) {
downlock = false;
clearInterval(uptimer);
downtimer = setInterval(function() {
that.y += 4;
if (that.y >= gamebox.offsetHeight - that.h) {
that.y = gamebox.offsetHeight - that.h;
}
that.myplaneimg.style.top = that.y + 'px';
}, 30);
}
}
function moveleft() {
if (leftlock) {
leftlock = false;
clearInterval(righttimer);
lefttimer = setInterval(function() {
that.x -= 4;
if (that.x <= 0) {
that.x = 0;
}
that.myplaneimg.style.left = that.x + 'px';
}, 30);
}
}
function moveright() {
if (rightlock) {
rightlock = false;
clearInterval(lefttimer);
righttimer = setInterval(function() {
that.x += 4;
if (that.x >= gamebox.offsetWidth - that.w) {
that.x = gamebox.offsetWidth - that.w;
}
that.myplaneimg.style.left = that.x + 'px';
}, 30);
}
}
}
document.addEventListener('keyup', function(ev) {
var ev = ev || window.event;
if (ev.keyCode == 87) {
clearInterval(uptimer);
uplock=true;
}
if (ev.keyCode == 83) {
clearInterval(downtimer);
downlock=true;
}
if (ev.keyCode == 65) {
clearInterval(lefttimer);
leftlock=true;
}
if (ev.keyCode == 68) {
clearInterval(righttimer);
rightlock=true;
}
}, false);
}
//2.3我方飞机发射子弹
Myplane.prototype.myplaneshoot = function() {
var that = this;
var shoottimer = null;
var shootlock = true;
document.addEventListener('keydown', shootbullet, false);
function shootbullet(ev) {
var ev = ev || window.event;
if (ev.keyCode == 75) {
if (shootlock) {
shootlock = false;
function shoot() {
new Bullet(6, 14, that.x + that.w / 2 - 3, that.y - 14, 'img/bullet.png');
}
shoot();
shoottimer = setInterval(shoot, 200);
}
}
}
document.addEventListener('keyup', function(ev) {
var ev = ev || window.event;
if (ev.keyCode == 75) {
clearInterval(shoottimer);
shootlock = true;
}
}, false);
}
//3.子弹的构造函数
function Bullet(w, h, x, y, imgurl) { //w,h宽高 x,y位置 imgurl图片路径
this.w = w;
this.h = h;
this.x = x;
this.y = y;
this.imgurl = imgurl;
//创建子弹
this.createbullet();
}
//3.1创建子弹
Bullet.prototype.createbullet = function() {
this.bulletimg = document.createElement('img');
this.bulletimg.src = this.imgurl;
this.bulletimg.style.cssText = `width:${this.w}px;height:${this.h}px;position:absolute;left:${this.x}px;top:${this.y}px;`;
gamebox.appendChild(this.bulletimg);
//子弹创建完成,执行运动。
this.bulletmove();
}
//3.2子弹运动
Bullet.prototype.bulletmove = function() {
var that = this;
this.timer = setInterval(function() {
that.y -= 4;
if (that.y <= -that.h) { //让子弹消失
clearInterval(that.timer);
gamebox.removeChild(that.bulletimg);
}
that.bulletimg.style.top = that.y + 'px';
that.bullethit();
}, 30)
}
Bullet.prototype.bullethit = function() {
var enemys = document.querySelectorAll('.enemy');
for (var i = 0; i < enemys.length; i++) {
if (this.x + this.w >= enemys[i].offsetLeft && this.x <= enemys[i].offsetLeft + enemys[i].offsetWidth && this.y + this.h >= enemys[i].offsetTop && this.y <= enemys[i].offsetTop + enemys[i].offsetHeight) {
clearInterval(this.timer);
try {
gamebox.removeChild(this.bulletimg);
} catch (e) {
return;
}
//血量减1
enemys[i].blood--;
//监听敌机的血量(给敌机添加方法)
enemys[i].checkblood();
}
}
}
//4.敌机的构造函数
function Enemy(w, h, x, y, imgurl, boomurl, blood, score, speed) {
this.w = w;
this.h = h;
this.x = x;
this.y = y;
this.imgurl = imgurl;
this.boomurl = boomurl;
this.blood = blood;
this.score = score;
this.speed = speed;
this.createenemy();
}
//4.1创建敌机图片
Enemy.prototype.createenemy = function() {
var that = this;
this.enemyimg = document.createElement('img');
this.enemyimg.src = this.imgurl;
this.enemyimg.style.cssText = `width:${this.w}px;height:${this.h}px;position:absolute;left:${this.x}px;top:${this.y}px;`;
gamebox.appendChild(this.enemyimg);
this.enemyimg.className = 'enemy'; //给每一架创建的敌机添加类
this.enemyimg.score = this.score; //给每一架创建的敌机添加分数
this.enemyimg.blood = this.blood; //给每一架创建的敌机添加自定义的属性--血量
this.enemyimg.checkblood = function() {
//this==>this.enemyimg
if (this.blood <= 0) { //敌机消失爆炸。
this.className = ''; //去掉类名。
this.src = that.boomurl;
clearInterval(that.enemyimg.timer);
setTimeout(function() {
gamebox.removeChild(that.enemyimg);
}, 300);
zscore += this.score;
oEm.innerHTML = zscore;
}
}
//子弹创建完成,执行运动。
this.enemymove();
}
//4.2敌机运动
Enemy.prototype.enemymove = function() {
var that = this;
this.enemyimg.timer = setInterval(function() {
that.y += that.speed;
if (that.y >= gamebox.offsetHeight) {
clearInterval(that.enemyimg.timer);
gamebox.removeChild(that.enemyimg);
}
that.enemyimg.style.top = that.y + 'px';
that.enemyhit();
}, 30);
}
//4.3敌机碰撞我方飞机
Enemy.prototype.enemyhit = function() {
if (!(this.x + this.w < ourplane.x || this.x > ourplane.x + ourplane.w || this.y + this.h < ourplane.y || this.y > ourplane.y + ourplane.h)) {
var enemys=document.querySelectorAll('.enemy');
for (var i = 0; i < enemys.length; i++) {
clearInterval(enemys[i].timer);
}
clearInterval(enemytimer);
clearInterval(bgtimer);
ourplane.myplaneimg.src = ourplane.boomurl;
setTimeout(function() {
gamebox.removeChild(ourplane.myplaneimg);
alert('game over!!');
location.reload();
}, 300)
}
}
var enemytimer = setInterval(function() {
for (var i = 0; i < ranNum(1, 3); i++) {
var num = ranNum(1, 20); //1-20
if (num < 15) { //小飞机
new Enemy(34, 24, ranNum(0, gamebox.offsetWidth - 34), -24, 'img/smallplane.png', 'img/smallplaneboom.gif', 1, 1, ranNum(2, 4));
} else if (num >= 15 && num < 20) {
new Enemy(46, 60, ranNum(0, gamebox.offsetWidth - 46), -60, 'img/midplane.png', 'img/midplaneboom.gif', 3, 5, ranNum(1, 3));
} else if (num == 20) {
new Enemy(110, 164, ranNum(0, gamebox.offsetWidth - 110), -164, 'img/bigplane.png', 'img/bigplaneboom.gif', 10, 10, 1);
}
}
}, 3000);
function ranNum(min, max) {
return Math.round(Math.random() * (max - min)) + min;
}
//实例化我方飞机
var ourplane = new Myplane(66, 80, (gamebox.offsetWidth - 66) / 2, gamebox.offsetHeight - 80, 'img/myplane.gif', 'img/myplaneBoom.gif');
})();
四、代码资源分享
本文内容由网友自发贡献,版权归原作者所有,本站不承担相应法律责任。如您发现有涉嫌抄袭侵权的内容,请联系:hwhale#tublm.com(使用前将#替换为@)