一、ST7789v介绍
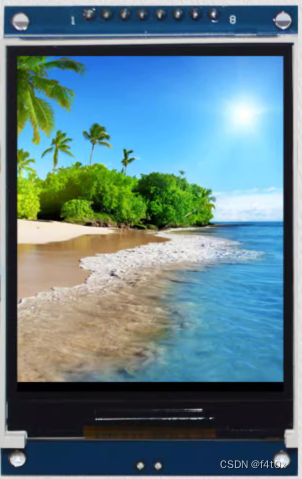
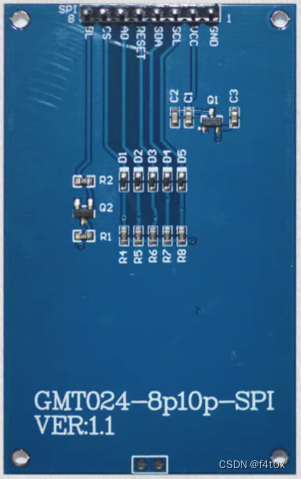
ST7789v是小尺寸液晶中常用的驱动芯片,作者手里的是网上买的一块2.4寸液晶模组,接口
为SPI接口。
网上能找到这个芯片的micropython驱动,这不是本文的重点。
本文的重点是如何利用这个驱动,并使用字库的方法来显示汉字。
我曾经有一篇文章,介绍如何使用unicode字模库在屏幕上显示汉字,如果你对这个方法还不是很了解,可以看一下我之前这篇文章。使用micropython(ESP8266、ESP32)驱动SES 2.66寸墨水屏显示中文_esp8266驱动墨水屏_f4t0x的博客-CSDN博客
二、st7789v原驱动
这个驱动可以在网上找到,我把源码贴下来。
"""
Copyright (c) 2020, 2021 Russ Hughes
This file incorporates work covered by the following copyright and
permission notice and is licensed under the same terms:
The MIT License (MIT)
Copyright (c) 2019 Ivan Belokobylskiy
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in
all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
THE SOFTWARE.
The driver is based on devbis' st7789py_mpy module from
https://github.com/devbis/st7789py_mpy.
This driver adds support for:
- 320x240, 240x240 and 135x240 pixel displays
- Display rotation
- Hardware based scrolling
- Drawing text using 8 and 16 bit wide bitmap fonts with heights that are
multiples of 8. Included are 12 bitmap fonts derived from classic pc
BIOS text mode fonts.
- Drawing text using converted TrueType fonts.
- Drawing converted bitmaps
"""
import time
from micropython import const
import ustruct as struct
# commands
ST7789_NOP = const(0x00)
ST7789_SWRESET = const(0x01)
ST7789_RDDID = const(0x04)
ST7789_RDDST = const(0x09)
ST7789_SLPIN = const(0x10)
ST7789_SLPOUT = const(0x11)
ST7789_PTLON = const(0x12)
ST7789_NORON = const(0x13)
ST7789_INVOFF = const(0x20)
ST7789_INVON = const(0x21)
ST7789_DISPOFF = const(0x28)
ST7789_DISPON = const(0x29)
ST7789_CASET = const(0x2A)
ST7789_RASET = const(0x2B)
ST7789_RAMWR = const(0x2C)
ST7789_RAMRD = const(0x2E)
ST7789_PTLAR = const(0x30)
ST7789_VSCRDEF = const(0x33)
ST7789_COLMOD = const(0x3A)
ST7789_MADCTL = const(0x36)
ST7789_VSCSAD = const(0x37)
ST7789_MADCTL_MY = const(0x80)
ST7789_MADCTL_MX = const(0x40)
ST7789_MADCTL_MV = const(0x20)
ST7789_MADCTL_ML = const(0x10)
ST7789_MADCTL_BGR = const(0x08)
ST7789_MADCTL_MH = const(0x04)
ST7789_MADCTL_RGB = const(0x00)
ST7789_RDID1 = const(0xDA)
ST7789_RDID2 = const(0xDB)
ST7789_RDID3 = const(0xDC)
ST7789_RDID4 = const(0xDD)
COLOR_MODE_65K = const(0x50)
COLOR_MODE_262K = const(0x60)
COLOR_MODE_12BIT = const(0x03)
COLOR_MODE_16BIT = const(0x05)
COLOR_MODE_18BIT = const(0x06)
COLOR_MODE_16M = const(0x07)
# Color definitions
BLACK = const(0x0000)
BLUE = const(0x001F)
RED = const(0xF800)
GREEN = const(0x07E0)
CYAN = const(0x07FF)
MAGENTA = const(0xF81F)
YELLOW = const(0xFFE0)
WHITE = const(0xFFFF)
_ENCODE_PIXEL = ">H"
_ENCODE_POS = ">HH"
_DECODE_PIXEL = ">BBB"
_BUFFER_SIZE = const(256)
_BIT7 = const(0x80)
_BIT6 = const(0x40)
_BIT5 = const(0x20)
_BIT4 = const(0x10)
_BIT3 = const(0x08)
_BIT2 = const(0x04)
_BIT1 = const(0x02)
_BIT0 = const(0x01)
# Rotation tables (width, height, xstart, ystart)[rotation % 4]
WIDTH_320 = [(240, 320, 0, 0),
(320, 240, 0, 0),
(240, 320, 0, 0),
(320, 240, 0, 0)]
WIDTH_240 = [(240, 240, 0, 0),
(240, 240, 0, 0),
(240, 240, 0, 80),
(240, 240, 80, 0)]
WIDTH_135 = [(135, 240, 52, 40),
(240, 135, 40, 53),
(135, 240, 53, 40),
(240, 135, 40, 52)]
# MADCTL ROTATIONS[rotation % 4]
ROTATIONS = [0x00, 0x60, 0xc0, 0xa0]
def color565(red, green=0, blue=0):
"""
Convert red, green and blue values (0-255) into a 16-bit 565 encoding.
"""
try:
red, green, blue = red # see if the first var is a tuple/list
except TypeError:
pass
return (red & 0xf8) << 8 | (green & 0xfc) << 3 | blue >> 3
def _encode_pos(x, y):
"""Encode a postion into bytes."""
return struct.pack(_ENCODE_POS, x, y)
def _encode_pixel(color):
"""Encode a pixel color into bytes."""
return struct.pack(_ENCODE_PIXEL, color)
class ST7789():
"""
ST7789 driver class
Args:
spi (spi): spi object **Required**
width (int): display width **Required**
height (int): display height **Required**
reset (pin): reset pin
dc (pin): dc pin **Required**
cs (pin): cs pin
backlight(pin): backlight pin
rotation (int): display rotation
- 0-Portrait
- 1-Landscape
- 2-Inverted Portrait
- 3-Inverted Landscape
"""
def __init__(self, spi, width, height, reset=None, dc=None,
cs=None, backlight=None, rotation=0):
"""
Initialize display.
"""
if height != 240 or width not in [320, 240, 135]:
raise ValueError(
"Unsupported display. 320x240, 240x240 and 135x240 are supported."
)
if dc is None:
raise ValueError("dc pin is required.")
self._display_width = self.width = width
self._display_height = self.height = height
self.xstart = 0
self.ystart = 0
self.spi = spi
self.reset = reset
self.dc = dc
self.cs = cs
self.backlight = backlight
self._rotation = rotation % 4
#self.hard_reset()
#self.soft_reset()
self.sleep_mode(False)
self._set_color_mode(COLOR_MODE_65K | COLOR_MODE_16BIT)
time.sleep_ms(50)
self.rotation(self._rotation)
self.inversion_mode(True)
time.sleep_ms(10)
self._write(ST7789_NORON)
time.sleep_ms(10)
if backlight is not None:
backlight.value(1)
self.fill(0)
self._write(ST7789_DISPON)
time.sleep_ms(500)
def _write(self, command=None, data=None):
"""SPI write to the device: commands and data."""
if self.cs:
self.cs.off()
if command is not None:
self.dc.off()
self.spi.write(bytes([command]))
if data is not None:
self.dc.on()
self.spi.write(data)
if self.cs:
self.cs.on()
def hard_reset(self):
"""
Hard reset display.
"""
if self.cs:
self.cs.off()
if self.reset:
self.reset.on()
time.sleep_ms(50)
if self.reset:
self.reset.off()
time.sleep_ms(50)
if self.reset:
self.reset.on()
time.sleep_ms(150)
if self.cs:
self.cs.on()
def soft_reset(self):
"""
Soft reset display.
"""
self._write(ST7789_SWRESET)
time.sleep_ms(150)
def sleep_mode(self, value):
"""
Enable or disable display sleep mode.
Args:
value (bool): if True enable sleep mode. if False disable sleep
mode
"""
if value:
self._write(ST7789_SLPIN)
else:
self._write(ST7789_SLPOUT)
def inversion_mode(self, value):
"""
Enable or disable display inversion mode.
Args:
value (bool): if True enable inversion mode. if False disable
inversion mode
"""
if value:
self._write(ST7789_INVON)
else:
self._write(ST7789_INVOFF)
def _set_color_mode(self, mode):
"""
Set display color mode.
Args:
mode (int): color mode
COLOR_MODE_65K, COLOR_MODE_262K, COLOR_MODE_12BIT,
COLOR_MODE_16BIT, COLOR_MODE_18BIT, COLOR_MODE_16M
"""
self._write(ST7789_COLMOD, bytes([mode & 0x77]))
def rotation(self, rotation):
"""
Set display rotation.
Args:
rotation (int):
- 0-Portrait
- 1-Landscape
- 2-Inverted Portrait
- 3-Inverted Landscape
"""
rotation %= 4
self._rotation = rotation
madctl = ROTATIONS[rotation]
if self._display_width == 320:
table = WIDTH_320
elif self._display_width == 240:
table = WIDTH_240
elif self._display_width == 135:
table = WIDTH_135
else:
raise ValueError(
"Unsupported display. 320x240, 240x240 and 135x240 are supported."
)
self.width, self.height, self.xstart, self.ystart = table[rotation]
self._write(ST7789_MADCTL, bytes([madctl]))
def _set_columns(self, start, end):
"""
Send CASET (column address set) command to display.
Args:
start (int): column start address
end (int): column end address
"""
if start <= end <= self.width:
self._write(ST7789_CASET, _encode_pos(
start+self.xstart, end + self.xstart))
def _set_rows(self, start, end):
"""
Send RASET (row address set) command to display.
Args:
start (int): row start address
end (int): row end address
"""
if start <= end <= self.height:
self._write(ST7789_RASET, _encode_pos(
start+self.ystart, end+self.ystart))
def _set_window(self, x0, y0, x1, y1):
"""
Set window to column and row address.
Args:
x0 (int): column start address
y0 (int): row start address
x1 (int): column end address
y1 (int): row end address
"""
self._set_columns(x0, x1)
self._set_rows(y0, y1)
self._write(ST7789_RAMWR)
def vline(self, x, y, length, color):
"""
Draw vertical line at the given location and color.
Args:
x (int): x coordinate
Y (int): y coordinate
length (int): length of line
color (int): 565 encoded color
"""
self.fill_rect(x, y, 1, length, color)
def hline(self, x, y, length, color):
"""
Draw horizontal line at the given location and color.
Args:
x (int): x coordinate
Y (int): y coordinate
length (int): length of line
color (int): 565 encoded color
"""
self.fill_rect(x, y, length, 1, color)
def pixel(self, x, y, color):
"""
Draw a pixel at the given location and color.
Args:
x (int): x coordinate
Y (int): y coordinate
color (int): 565 encoded color
"""
self._set_window(x, y, x, y)
self._write(None, _encode_pixel(color))
def blit_buffer(self, buffer, x, y, width, height):
"""
Copy buffer to display at the given location.
Args:
buffer (bytes): Data to copy to display
x (int): Top left corner x coordinate
Y (int): Top left corner y coordinate
width (int): Width
height (int): Height
"""
self._set_window(x, y, x + width - 1, y + height - 1)
self._write(None, buffer)
def rect(self, x, y, w, h, color):
"""
Draw a rectangle at the given location, size and color.
Args:
x (int): Top left corner x coordinate
y (int): Top left corner y coordinate
width (int): Width in pixels
height (int): Height in pixels
color (int): 565 encoded color
"""
self.hline(x, y, w, color)
self.vline(x, y, h, color)
self.vline(x + w - 1, y, h, color)
self.hline(x, y + h - 1, w, color)
def fill_rect(self, x, y, width, height, color):
"""
Draw a rectangle at the given location, size and filled with color.
Args:
x (int): Top left corner x coordinate
y (int): Top left corner y coordinate
width (int): Width in pixels
height (int): Height in pixels
color (int): 565 encoded color
"""
self._set_window(x, y, x + width - 1, y + height - 1)
chunks, rest = divmod(width * height, _BUFFER_SIZE)
pixel = _encode_pixel(color)
self.dc.on()
if chunks:
data = pixel * _BUFFER_SIZE
for _ in range(chunks):
self._write(None, data)
if rest:
self._write(None, pixel * rest)
def fill(self, color):
"""
Fill the entire FrameBuffer with the specified color.
Args:
color (int): 565 encoded color
"""
self.fill_rect(0, 0, self.width, self.height, color)
def line(self, x0, y0, x1, y1, color):
"""
Draw a single pixel wide line starting at x0, y0 and ending at x1, y1.
Args:
x0 (int): Start point x coordinate
y0 (int): Start point y coordinate
x1 (int): End point x coordinate
y1 (int): End point y coordinate
color (int): 565 encoded color
"""
steep = abs(y1 - y0) > abs(x1 - x0)
if steep:
x0, y0 = y0, x0
x1, y1 = y1, x1
if x0 > x1:
x0, x1 = x1, x0
y0, y1 = y1, y0
dx = x1 - x0
dy = abs(y1 - y0)
err = dx // 2
ystep = 1 if y0 < y1 else -1
while x0 <= x1:
if steep:
self.pixel(y0, x0, color)
else:
self.pixel(x0, y0, color)
err -= dy
if err < 0:
y0 += ystep
err += dx
x0 += 1
def vscrdef(self, tfa, vsa, bfa):
"""
Set Vertical Scrolling Definition.
To scroll a 135x240 display these values should be 40, 240, 40.
There are 40 lines above the display that are not shown followed by
240 lines that are shown followed by 40 more lines that are not shown.
You could write to these areas off display and scroll them into view by
changing the TFA, VSA and BFA values.
Args:
tfa (int): Top Fixed Area
vsa (int): Vertical Scrolling Area
bfa (int): Bottom Fixed Area
"""
struct.pack(">HHH", tfa, vsa, bfa)
self._write(ST7789_VSCRDEF, struct.pack(">HHH", tfa, vsa, bfa))
def vscsad(self, vssa):
"""
Set Vertical Scroll Start Address of RAM.
Defines which line in the Frame Memory will be written as the first
line after the last line of the Top Fixed Area on the display
Example:
for line in range(40, 280, 1):
tft.vscsad(line)
utime.sleep(0.01)
Args:
vssa (int): Vertical Scrolling Start Address
"""
self._write(ST7789_VSCSAD, struct.pack(">H", vssa))
def _text8(self, font, text, x0, y0, color=WHITE, background=BLACK):
"""
Internal method to write characters with width of 8 and
heights of 8 or 16.
Args:
font (module): font module to use
text (str): text to write
x0 (int): column to start drawing at
y0 (int): row to start drawing at
color (int): 565 encoded color to use for characters
background (int): 565 encoded color to use for background
"""
for char in text:
ch = ord(char)
if (font.FIRST <= ch < font.LAST
and x0+font.WIDTH <= self.width
and y0+font.HEIGHT <= self.height):
if font.HEIGHT == 8:
passes = 1
size = 8
each = 0
else:
passes = 2
size = 16
each = 8
for line in range(passes):
idx = (ch-font.FIRST)*size+(each*line)
buffer = struct.pack(
'>64H',
color if font.FONT[idx] & _BIT7 else background,
color if font.FONT[idx] & _BIT6 else background,
color if font.FONT[idx] & _BIT5 else background,
color if font.FONT[idx] & _BIT4 else background,
color if font.FONT[idx] & _BIT3 else background,
color if font.FONT[idx] & _BIT2 else background,
color if font.FONT[idx] & _BIT1 else background,
color if font.FONT[idx] & _BIT0 else background,
color if font.FONT[idx+1] & _BIT7 else background,
color if font.FONT[idx+1] & _BIT6 else background,
color if font.FONT[idx+1] & _BIT5 else background,
color if font.FONT[idx+1] & _BIT4 else background,
color if font.FONT[idx+1] & _BIT3 else background,
color if font.FONT[idx+1] & _BIT2 else background,
color if font.FONT[idx+1] & _BIT1 else background,
color if font.FONT[idx+1] & _BIT0 else background,
color if font.FONT[idx+2] & _BIT7 else background,
color if font.FONT[idx+2] & _BIT6 else background,
color if font.FONT[idx+2] & _BIT5 else background,
color if font.FONT[idx+2] & _BIT4 else background,
color if font.FONT[idx+2] & _BIT3 else background,
color if font.FONT[idx+2] & _BIT2 else background,
color if font.FONT[idx+2] & _BIT1 else background,
color if font.FONT[idx+2] & _BIT0 else background,
color if font.FONT[idx+3] & _BIT7 else background,
color if font.FONT[idx+3] & _BIT6 else background,
color if font.FONT[idx+3] & _BIT5 else background,
color if font.FONT[idx+3] & _BIT4 else background,
color if font.FONT[idx+3] & _BIT3 else background,
color if font.FONT[idx+3] & _BIT2 else background,
color if font.FONT[idx+3] & _BIT1 else background,
color if font.FONT[idx+3] & _BIT0 else background,
color if font.FONT[idx+4] & _BIT7 else background,
color if font.FONT[idx+4] & _BIT6 else background,
color if font.FONT[idx+4] & _BIT5 else background,
color if font.FONT[idx+4] & _BIT4 else background,
color if font.FONT[idx+4] & _BIT3 else background,
color if font.FONT[idx+4] & _BIT2 else background,
color if font.FONT[idx+4] & _BIT1 else background,
color if font.FONT[idx+4] & _BIT0 else background,
color if font.FONT[idx+5] & _BIT7 else background,
color if font.FONT[idx+5] & _BIT6 else background,
color if font.FONT[idx+5] & _BIT5 else background,
color if font.FONT[idx+5] & _BIT4 else background,
color if font.FONT[idx+5] & _BIT3 else background,
color if font.FONT[idx+5] & _BIT2 else background,
color if font.FONT[idx+5] & _BIT1 else background,
color if font.FONT[idx+5] & _BIT0 else background,
color if font.FONT[idx+6] & _BIT7 else background,
color if font.FONT[idx+6] & _BIT6 else background,
color if font.FONT[idx+6] & _BIT5 else background,
color if font.FONT[idx+6] & _BIT4 else background,
color if font.FONT[idx+6] & _BIT3 else background,
color if font.FONT[idx+6] & _BIT2 else background,
color if font.FONT[idx+6] & _BIT1 else background,
color if font.FONT[idx+6] & _BIT0 else background,
color if font.FONT[idx+7] & _BIT7 else background,
color if font.FONT[idx+7] & _BIT6 else background,
color if font.FONT[idx+7] & _BIT5 else background,
color if font.FONT[idx+7] & _BIT4 else background,
color if font.FONT[idx+7] & _BIT3 else background,
color if font.FONT[idx+7] & _BIT2 else background,
color if font.FONT[idx+7] & _BIT1 else background,
color if font.FONT[idx+7] & _BIT0 else background
)
self.blit_buffer(buffer, x0, y0+8*line, 8, 8)
x0 += 8
def _text16(self, font, text, x0, y0, color=WHITE, background=BLACK):
"""
Internal method to draw characters with width of 16 and heights of 16
or 32.
Args:
font (module): font module to use
text (str): text to write
x0 (int): column to start drawing at
y0 (int): row to start drawing at
color (int): 565 encoded color to use for characters
background (int): 565 encoded color to use for background
"""
for char in text:
ch = ord(char)
if (font.FIRST <= ch < font.LAST
and x0+font.WIDTH <= self.width
and y0+font.HEIGHT <= self.height):
each = 16
if font.HEIGHT == 16:
passes = 2
size = 32
else:
passes = 4
size = 64
for line in range(passes):
idx = (ch-font.FIRST)*size+(each*line)
buffer = struct.pack(
'>128H',
color if font.FONT[idx] & _BIT7 else background,
color if font.FONT[idx] & _BIT6 else background,
color if font.FONT[idx] & _BIT5 else background,
color if font.FONT[idx] & _BIT4 else background,
color if font.FONT[idx] & _BIT3 else background,
color if font.FONT[idx] & _BIT2 else background,
color if font.FONT[idx] & _BIT1 else background,
color if font.FONT[idx] & _BIT0 else background,
color if font.FONT[idx+1] & _BIT7 else background,
color if font.FONT[idx+1] & _BIT6 else background,
color if font.FONT[idx+1] & _BIT5 else background,
color if font.FONT[idx+1] & _BIT4 else background,
color if font.FONT[idx+1] & _BIT3 else background,
color if font.FONT[idx+1] & _BIT2 else background,
color if font.FONT[idx+1] & _BIT1 else background,
color if font.FONT[idx+1] & _BIT0 else background,
color if font.FONT[idx+2] & _BIT7 else background,
color if font.FONT[idx+2] & _BIT6 else background,
color if font.FONT[idx+2] & _BIT5 else background,
color if font.FONT[idx+2] & _BIT4 else background,
color if font.FONT[idx+2] & _BIT3 else background,
color if font.FONT[idx+2] & _BIT2 else background,
color if font.FONT[idx+2] & _BIT1 else background,
color if font.FONT[idx+2] & _BIT0 else background,
color if font.FONT[idx+3] & _BIT7 else background,
color if font.FONT[idx+3] & _BIT6 else background,
color if font.FONT[idx+3] & _BIT5 else background,
color if font.FONT[idx+3] & _BIT4 else background,
color if font.FONT[idx+3] & _BIT3 else background,
color if font.FONT[idx+3] & _BIT2 else background,
color if font.FONT[idx+3] & _BIT1 else background,
color if font.FONT[idx+3] & _BIT0 else background,
color if font.FONT[idx+4] & _BIT7 else background,
color if font.FONT[idx+4] & _BIT6 else background,
color if font.FONT[idx+4] & _BIT5 else background,
color if font.FONT[idx+4] & _BIT4 else background,
color if font.FONT[idx+4] & _BIT3 else background,
color if font.FONT[idx+4] & _BIT2 else background,
color if font.FONT[idx+4] & _BIT1 else background,
color if font.FONT[idx+4] & _BIT0 else background,
color if font.FONT[idx+5] & _BIT7 else background,
color if font.FONT[idx+5] & _BIT6 else background,
color if font.FONT[idx+5] & _BIT5 else background,
color if font.FONT[idx+5] & _BIT4 else background,
color if font.FONT[idx+5] & _BIT3 else background,
color if font.FONT[idx+5] & _BIT2 else background,
color if font.FONT[idx+5] & _BIT1 else background,
color if font.FONT[idx+5] & _BIT0 else background,
color if font.FONT[idx+6] & _BIT7 else background,
color if font.FONT[idx+6] & _BIT6 else background,
color if font.FONT[idx+6] & _BIT5 else background,
color if font.FONT[idx+6] & _BIT4 else background,
color if font.FONT[idx+6] & _BIT3 else background,
color if font.FONT[idx+6] & _BIT2 else background,
color if font.FONT[idx+6] & _BIT1 else background,
color if font.FONT[idx+6] & _BIT0 else background,
color if font.FONT[idx+7] & _BIT7 else background,
color if font.FONT[idx+7] & _BIT6 else background,
color if font.FONT[idx+7] & _BIT5 else background,
color if font.FONT[idx+7] & _BIT4 else background,
color if font.FONT[idx+7] & _BIT3 else background,
color if font.FONT[idx+7] & _BIT2 else background,
color if font.FONT[idx+7] & _BIT1 else background,
color if font.FONT[idx+7] & _BIT0 else background,
color if font.FONT[idx+8] & _BIT7 else background,
color if font.FONT[idx+8] & _BIT6 else background,
color if font.FONT[idx+8] & _BIT5 else background,
color if font.FONT[idx+8] & _BIT4 else background,
color if font.FONT[idx+8] & _BIT3 else background,
color if font.FONT[idx+8] & _BIT2 else background,
color if font.FONT[idx+8] & _BIT1 else background,
color if font.FONT[idx+8] & _BIT0 else background,
color if font.FONT[idx+9] & _BIT7 else background,
color if font.FONT[idx+9] & _BIT6 else background,
color if font.FONT[idx+9] & _BIT5 else background,
color if font.FONT[idx+9] & _BIT4 else background,
color if font.FONT[idx+9] & _BIT3 else background,
color if font.FONT[idx+9] & _BIT2 else background,
color if font.FONT[idx+9] & _BIT1 else background,
color if font.FONT[idx+9] & _BIT0 else background,
color if font.FONT[idx+10] & _BIT7 else background,
color if font.FONT[idx+10] & _BIT6 else background,
color if font.FONT[idx+10] & _BIT5 else background,
color if font.FONT[idx+10] & _BIT4 else background,
color if font.FONT[idx+10] & _BIT3 else background,
color if font.FONT[idx+10] & _BIT2 else background,
color if font.FONT[idx+10] & _BIT1 else background,
color if font.FONT[idx+10] & _BIT0 else background,
color if font.FONT[idx+11] & _BIT7 else background,
color if font.FONT[idx+11] & _BIT6 else background,
color if font.FONT[idx+11] & _BIT5 else background,
color if font.FONT[idx+11] & _BIT4 else background,
color if font.FONT[idx+11] & _BIT3 else background,
color if font.FONT[idx+11] & _BIT2 else background,
color if font.FONT[idx+11] & _BIT1 else background,
color if font.FONT[idx+11] & _BIT0 else background,
color if font.FONT[idx+12] & _BIT7 else background,
color if font.FONT[idx+12] & _BIT6 else background,
color if font.FONT[idx+12] & _BIT5 else background,
color if font.FONT[idx+12] & _BIT4 else background,
color if font.FONT[idx+12] & _BIT3 else background,
color if font.FONT[idx+12] & _BIT2 else background,
color if font.FONT[idx+12] & _BIT1 else background,
color if font.FONT[idx+12] & _BIT0 else background,
color if font.FONT[idx+13] & _BIT7 else background,
color if font.FONT[idx+13] & _BIT6 else background,
color if font.FONT[idx+13] & _BIT5 else background,
color if font.FONT[idx+13] & _BIT4 else background,
color if font.FONT[idx+13] & _BIT3 else background,
color if font.FONT[idx+13] & _BIT2 else background,
color if font.FONT[idx+13] & _BIT1 else background,
color if font.FONT[idx+13] & _BIT0 else background,
color if font.FONT[idx+14] & _BIT7 else background,
color if font.FONT[idx+14] & _BIT6 else background,
color if font.FONT[idx+14] & _BIT5 else background,
color if font.FONT[idx+14] & _BIT4 else background,
color if font.FONT[idx+14] & _BIT3 else background,
color if font.FONT[idx+14] & _BIT2 else background,
color if font.FONT[idx+14] & _BIT1 else background,
color if font.FONT[idx+14] & _BIT0 else background,
color if font.FONT[idx+15] & _BIT7 else background,
color if font.FONT[idx+15] & _BIT6 else background,
color if font.FONT[idx+15] & _BIT5 else background,
color if font.FONT[idx+15] & _BIT4 else background,
color if font.FONT[idx+15] & _BIT3 else background,
color if font.FONT[idx+15] & _BIT2 else background,
color if font.FONT[idx+15] & _BIT1 else background,
color if font.FONT[idx+15] & _BIT0 else background
)
self.blit_buffer(buffer, x0, y0+8*line, 16, 8)
x0 += font.WIDTH
def text(self, font, text, x0, y0, color=WHITE, background=BLACK):
"""
Draw text on display in specified font and colors. 8 and 16 bit wide
fonts are supported.
Args:
font (module): font module to use.
text (str): text to write
x0 (int): column to start drawing at
y0 (int): row to start drawing at
color (int): 565 encoded color to use for characters
background (int): 565 encoded color to use for background
"""
if font.WIDTH == 8:
self._text8(font, text, x0, y0, color, background)
else:
self._text16(font, text, x0, y0, color, background)
def bitmap(self, bitmap, x, y, index=0):
"""
Draw a bitmap on display at the specified column and row
Args:
bitmap (bitmap_module): The module containing the bitmap to draw
x (int): column to start drawing at
y (int): row to start drawing at
index (int): Optional index of bitmap to draw from multiple bitmap
module
"""
bitmap_size = bitmap.HEIGHT * bitmap.WIDTH
buffer_len = bitmap_size * 2
buffer = bytearray(buffer_len)
bs_bit = bitmap.BPP * bitmap_size * index if index > 0 else 0
for i in range(0, buffer_len, 2):
color_index = 0
for _ in range(bitmap.BPP):
color_index <<= 1
color_index |= (bitmap.BITMAP[bs_bit // 8]
& 1 << (7 - (bs_bit % 8))) > 0
bs_bit += 1
color = bitmap.PALETTE[color_index]
buffer[i] = color & 0xff00 >> 8
buffer[i + 1] = color_index & 0xff
to_col = x + bitmap.WIDTH - 1
to_row = y + bitmap.HEIGHT - 1
if self.width > to_col and self.height > to_row:
self._set_window(x, y, to_col, to_row)
self._write(None, buffer)
# @micropython.native
def write(self, font, string, x, y, fg=WHITE, bg=BLACK):
"""
Write a string using a converted true-type font on the display starting
at the specified column and row
Args:
font (font): The module containing the converted true-type font
s (string): The string to write
x (int): column to start writing
y (int): row to start writing
fg (int): foreground color, optional, defaults to WHITE
bg (int): background color, optional, defaults to BLACK
"""
buffer_len = font.HEIGHT * font.MAX_WIDTH * 2
buffer = bytearray(buffer_len)
fg_hi = (fg & 0xff00) >> 8
fg_lo = fg & 0xff
bg_hi = (bg & 0xff00) >> 8
bg_lo = bg & 0xff
for character in string:
try:
char_index = font.MAP.index(character)
offset = char_index * font.OFFSET_WIDTH
bs_bit = font.OFFSETS[offset]
if font.OFFSET_WIDTH > 1:
bs_bit = (bs_bit << 8) + font.OFFSETS[offset + 1]
if font.OFFSET_WIDTH > 2:
bs_bit = (bs_bit << 8) + font.OFFSETS[offset + 2]
char_width = font.WIDTHS[char_index]
buffer_needed = char_width * font.HEIGHT * 2
for i in range(0, buffer_needed, 2):
if font.BITMAPS[bs_bit // 8] & 1 << (7 - (bs_bit % 8)) > 0:
buffer[i] = fg_hi
buffer[i + 1] = fg_lo
else:
buffer[i] = bg_hi
buffer[i + 1] = bg_lo
bs_bit += 1
to_col = x + char_width - 1
to_row = y + font.HEIGHT - 1
if self.width > to_col and self.height > to_row:
self._set_window(x, y, to_col, to_row)
self._write(None, buffer[:buffer_needed])
x += char_width
except ValueError:
pass
def write_width(self, font, string):
"""
Returns the width in pixels of the string if it was written with the
specified font
Args:
font (font): The module containing the converted true-type font
string (string): The string to measure
"""
width = 0
for character in string:
try:
char_index = font.MAP.index(character)
width += font.WIDTHS[char_index]
except ValueError:
pass
return width
其中,st7789.text()方法就是显示ASCII字符了,你可能也发现了,遗憾的是,这个驱动只支持8*8和16*16的字符,并且不支持中文。
下面我们在这个驱动下面增加中文字符显示功能。
三、中文显示
如我之前那篇文章所述,显示中文得有字模库,取到字模库中要显示得汉字字模后,采用blit的方法显示出来。
我们注意到,前面那个驱动里,也正好有blit这个方法。
1.取字模库
依然采用前述那篇文章中的软件,提取unicode字模库。
要注意的是,为了编程方便,我们把unicode从0x0000-0xFFFF全部取出来,我用的SD卡存储字模库,所以没那么在乎存储空间的问题,如果你在意的话,只取中文部分0x4E00开始也没问题,只需在取到中文unicode码之后减掉0x4E00再去fileseek()即可。
这个是取模软件截图。
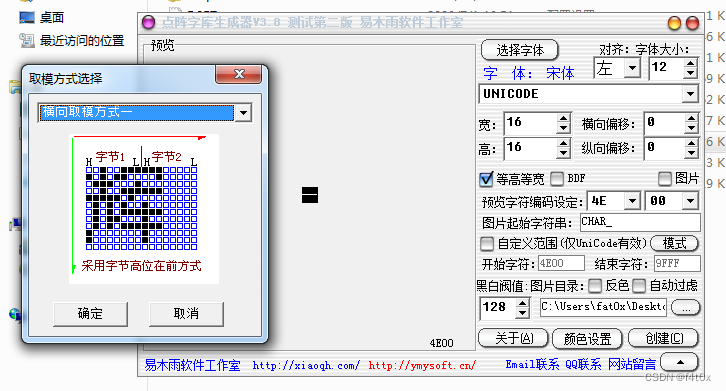
取好字模库后,保存到flash或SD卡备用。
2.处理字模库
关于这一步,其实开始我也是不知道的,因为取模直接显示出来的中文字符出现了问题:
字符左右半边各自镜像于正常字符。比如下边这个“辉”字,中间为界,左半边和右半边各自左右翻转了。
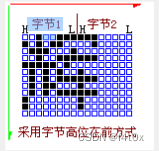
显示成了:
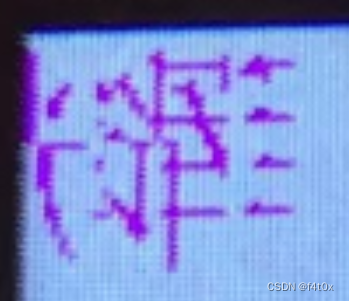
不难看出,是取模方式的问题。横向取模是对的,但是每个字节高低位是反的。图中的字节H和L是反的,但是取模软件横向取模只有这一种方式,没有其他方式可选,那就只能自己处理下字模文件了。
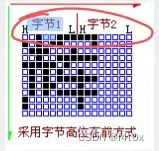
所以就有了下面这个小程序(随手写的,有些逻辑问题,不影响结果):
取模完成后,用程序把字模文件的每个字节高低顺序对调。
f=open('e:/fonts-unicode(0000-00FF)-w16-h32-b24.dzk','rb')
f1=open('e:/fonts-unicode(0000-00FF)-w16-h32-b24-inv8.dzk','wb')
buff=f.read(1)
a=buff[0]
cnt=0
writeBuff=bytearray(1)
while buff is not None:
b=0x00
#print("a=",a)
for i in range(8):
b=(b&0xfe) | (0x01 & a)
if i<7:
b=b<<1
a=a>>1
#print("b=",b)
writeBuff[0]=b
f1.write(writeBuff)
cnt+=1
#print("cnt=",cnt)
try:
buff=f.read(1)
a=buff[0]
except:
break
print("cnt=",cnt)
f1.flush()
f.close()
f1.close()
print("done")
处理完成后的字模库文件终于可以用了。
3.显示中文
经过一番准备,终于可以干正事了。
ST7789v液晶屏幕上,一个像素点需要使用2个字节来表示该点的颜色,即RGB565,毕竟是彩色屏幕嘛。
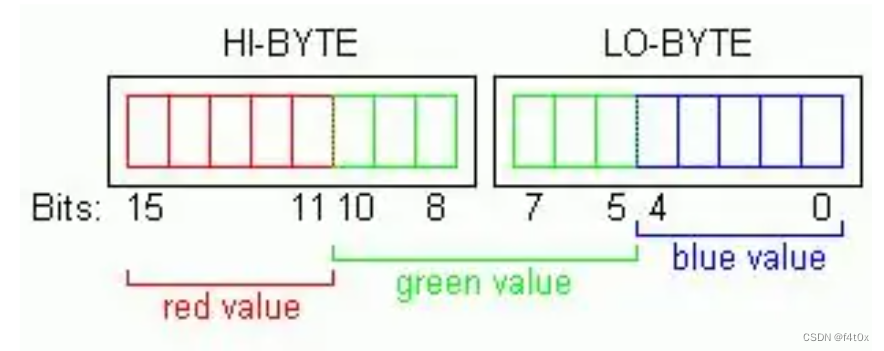
我们在字模库中取出的字模,实际是黑白像素字模,一个位对应一个像素点。这就需要我们做一个转换。字模库中取出一个位,我们判断是1的话,就用文字颜色(RGB565的2个字节)填充;如果是0的话,就用背景色填充。
原理清楚了,就可以直接上代码了。
注意程序中对中文字符和ASCII字符做了区别处理。这是因为在同等高度,比如都是high=32的情况下,ASCII字符不需要占用到32,只需要16就可以了,否则ASCII字符就周围空白很多就不好看了。所以中文字符和ASCII字符用了不同的字模库。
这段程序支持16和32两种字符。
encode_get_utf8_size和encode_utf8_to_unicode是用来把中文转为unicode编码的,之前那篇文章提到过。
def stringCN(self,string, x0, y0, high=32,color=WHITE, background=BLACK):
import gc
gc.collect()
high=high
if high==16:
fontFileCN=open('/sd/fonts-unicode(0000-FFFF)-w16-h16-b12-inv8.dzk','rb')
fontFileEN=open('/sd/fonts-unicode(0000-00FF)-w8-h16-b12-inv8.dzk','rb')
widthCN=16
widthEN=8
elif high==32:
fontFileCN=open('/sd/fonts-unicode(0000-FFFF)-w32-h32-b24.dzk','rb')
fontFileEN=open('/sd/fonts-unicode(0000-00FF)-w16-h32-b24-inv8.dzk','rb')
widthCN=32
widthEN=16
else:
print("unsupported font!")
return
#输入字符串长度,即字符数,一个中文/英文字符均算作1个
i=0#i是string通过string=bytes(string,'utf-8')转换成字节串之后在每个循环中处理的位置
scrPos=0#要显示的某个字符在屏幕X轴方向的位置
string=bytes(string,'utf-8')
#此时string的len已经和输入参数中的len不一样了,因为中文在输入时len为1,转换后len为3
while i<len(string):
utfbytes=self.encode_get_utf8_size(string[i])#单个字符的所占字节数,一般英文为1,中文为3
#print(i,string[i],utfbytes,string[i:i+utfbytes])
tmp=self.encode_utf8_to_unicode(string[i:i+utfbytes])#字符转码为unicode编码,0x0000~0xffff,常用中文在0x4e00~0x9fff,英文在0x0000~0x00ff
#print(type(tmp),tmp)
i+=utfbytes
if utfbytes==1:
width=widthEN
fontFile=fontFileEN
elif utfbytes==3:
width=widthCN
fontFile=fontFileCN
else:
print("utfbytes error,utfbytes=",utfbytes)
return
fontFile.seek(tmp*int(high*width/8))
zimo=fontFile.read(int(high*width/8))
buffer=bytearray(0)
for pixByteIndex in range(int(high*width/8)):
#print(pixByteIndex)
for bitCounter in range(8):
#print(bitCounter)
#RGB565,一个像素点2字节,如果字模中像素点为0,就填充背景色,为1就填充color色
if (zimo[pixByteIndex] & 0x01<<bitCounter):
buffer.append(~((color&0xff00)>>8))
buffer.append(~(color&0x00ff))
else:
buffer.append(~((background & 0xff00)>>8))
buffer.append(~(background & 0x00ff))
self.blit_buffer(buffer, x0+scrPos, y0, width=width, height=high)
scrPos+=width
def encode_get_utf8_size(self,utf):
if utf < 0x80:
return 1
if utf >= 0x80 and utf < 0xC0:
return -1
if utf >= 0xC0 and utf < 0xE0:
return 2
if utf >= 0xE0 and utf < 0xF0:
return 3
if utf >= 0xF0 and utf < 0xF8:
return 4
if utf >= 0xF8 and utf < 0xFC:
return 5
if utf >= 0xFC:
return 6
def encode_utf8_to_unicode(self,utf8):
utfbytes = self.encode_get_utf8_size(utf8[0])
if utfbytes == 1:
unic = utf8[0]
if utfbytes == 2:
b1 = utf8[0]
b2 = utf8[1]
if ((b2 & 0xE0) != 0x80):
return -1
unic = ((((b1 << 6) + (b2 & 0x3F)) & 0xFF) << 8) | (((b1 >> 2) & 0x07) & 0xFF)
if utfbytes == 3:
b1 = utf8[0]
b2 = utf8[1]
b3 = utf8[2]
if (((b2 & 0xC0) != 0x80) or ((b3 & 0xC0) != 0x80)):
return -1
unic = ((((b1 << 4) + ((b2 >> 2) & 0x0F)) & 0xFF) << 8) | (((b2 << 6) + (b3 & 0x3F)) & 0xFF)
if utfbytes == 4:
b1 = utf8[0]
b2 = utf8[1]
b3 = utf8[2]
b4 = utf8[3]
if (((b2 & 0xC0) != 0x80) or ((b3 & 0xC0) != 0x80) or ((b4 & 0xC0) != 0x80)):
return -1
unic = ((((b3 << 6) + (b4 & 0x3F)) & 0xFF) << 16) | ((((b2 << 4) + ((b3 >> 2)
& 0x0F)) & 0xFF) << 8) | ((((b1 << 2) & 0x1C) + ((b2 >> 4) & 0x03)) & 0xFF)
if utfbytes == 5:
b1 = utf8[0]
b2 = utf8[1]
b3 = utf8[2]
b4 = utf8[3]
b5 = utf8[4]
if (((b2 & 0xC0) != 0x80) or ((b3 & 0xC0) != 0x80) or ((b4 & 0xC0) != 0x80) or ((b5 & 0xC0) != 0x80)):
return -1
unic = ((((b4 << 6) + (b5 & 0x3F)) & 0xFF) << 24) | (((b3 << 4) + ((b4 >> 2) & 0x0F) & 0xFF) << 16) | ((((b2 << 2) + ((b3 >> 4) & 0x03)) & 0xFF) << 8) | (((b1 << 6)) & 0xFF)
if utfbytes == 6:
b1 = utf8[0]
b2 = utf8[1]
b3 = utf8[2]
b4 = utf8[3]
b5 = utf8[4]
b6 = utf8[5]
if (((b2 & 0xC0) != 0x80) or ((b3 & 0xC0) != 0x80) or ((b4 & 0xC0) != 0x80) or ((b5 & 0xC0) != 0x80) or ((b6 & 0xC0) != 0x80)):
return -1
unic = ((((b5 << 6) + (b6 & 0x3F)) << 24) & 0xFF) | (((b5 << 4) + ((b6 >> 2) & 0x0F) << 16) & 0xFF) | ((((b3 << 2) + ((b4 >> 4) & 0x03)) << 8) & 0xFF) | ((((b1 << 6) & 0x40) + (b2 & 0x3F)) & 0xFF)
return unic
OK,最终显示效果:
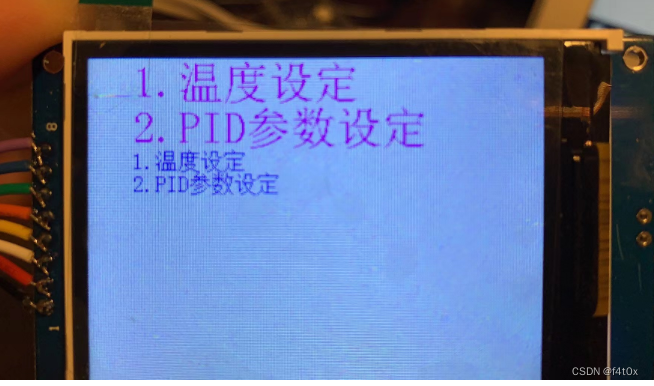
最后说一句,原驱动程序中使用了大量color if font.FONT[idx] & _BITx else background这种语句,个人感觉太笨重了,可以优化成循环语句,不过我也懒得去修改了。