目录
一,问题背景
二,解决方法
1,POST增
2,DELETE删
3,PUT改
4,参考源代码
一,问题背景
最开始接触web后端开发时,使用的请求大多是通过自定义的一些请求名称,比如update.do、delete.do等,现在为了规范化开发,要求请求需要符合RESTful风格。
这里以一个简单的web项目为例,需要在它基础之上将其修改为RESTful风格的交互方式。
二,解决方法
1,SpringBoot2.0+默认不支持restful请求,需要在application.properties/yml中设置对应的属性将他打开。
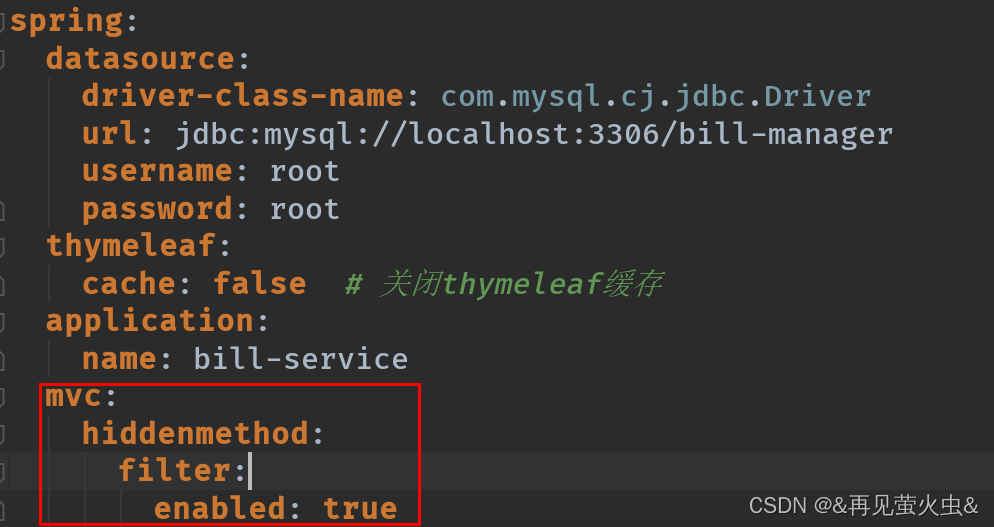
2,在form表单中添加一个input框,对应设置的属性有所要求才能被识别
<form id="delete" method="post">
<input type="hidden" value="DELETE" name="_method">
</form>
3,在controll中,接受对应请求时指明具体的method
@RequestMapping(value = "{id}", method = RequestMethod.DELETE)
public String delete(@PathVariable("id") Long id) {
billService.delete(id);
return "redirect:/bill/list-page";
}
1,POST增
1,在原先的html页面的form表单下添加隐藏input标签
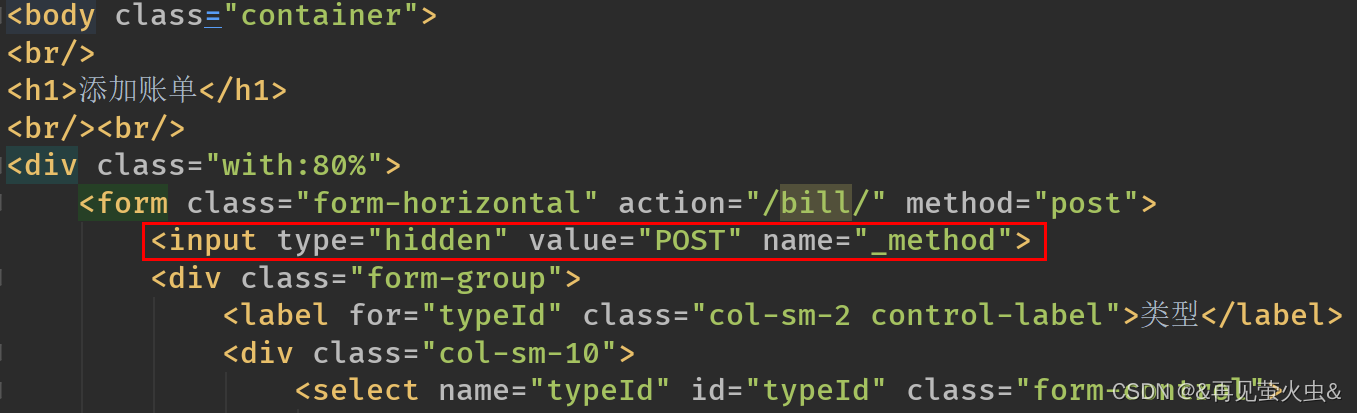
2,controller中指明对应的请求方式

3,效果如下

2,DELETE删
1,删除原先是通过a标签嵌入href的方式通过GET方式实现,这里保留了原先的样式,通过添加javascript和隐藏表单来实现a标签发送POST请求

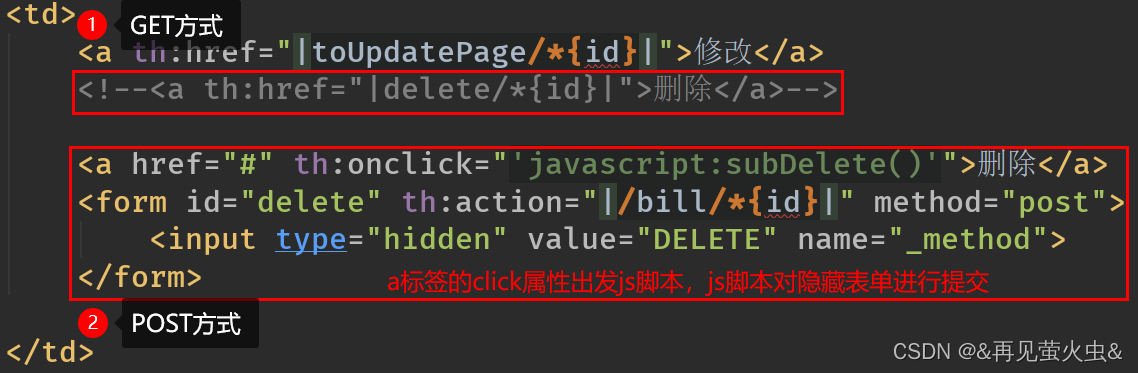
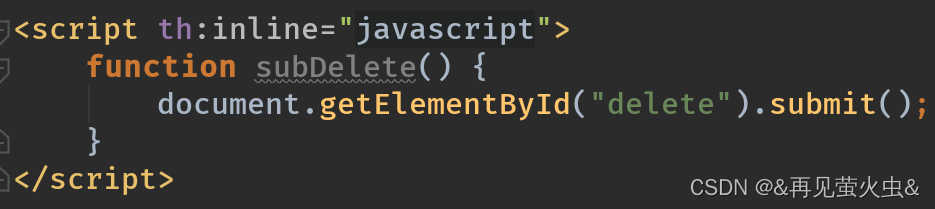
2,controller中指明对应的请求方式

3,PUT改
1,点击a标签后通过href属性设置的请求跳转到更新页面,回显需要更新的数据
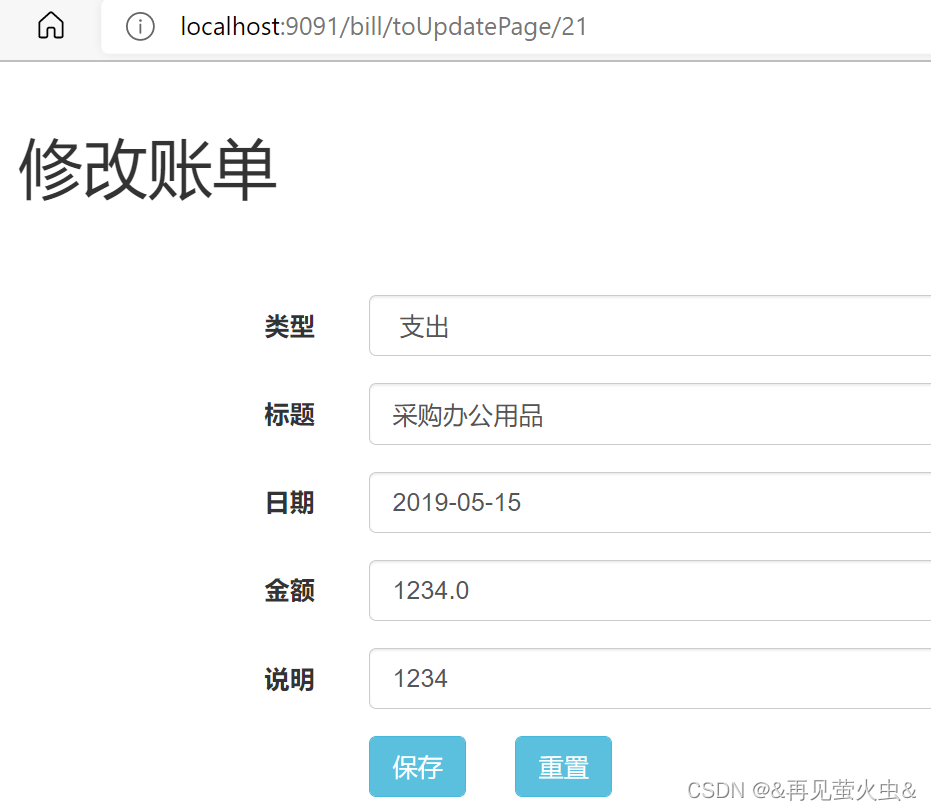
2,在更新html页面的form表单下添加隐藏input标签

3,controller中指明对应的请求方式

4,参考源代码
BillController.java
import com.github.pagehelper.PageInfo;
import com.xxy.entity.Bill;
import com.xxy.entity.BillType;
import com.xxy.service.BillService;
import com.xxy.service.TypeService;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import javax.annotation.Resource;
import java.util.List;
@Controller
@RequestMapping("bill")
public class BillController {
@Resource
private BillService billService;
@Resource
private TypeService typeService;
@RequestMapping("list-page")
public String listPage(@RequestParam(defaultValue = "1") int pageNum,
@RequestParam(defaultValue = "5") int pageSize,
Bill bill,
Model model) {
List<BillType> types = typeService.selectAll();
model.addAttribute("types", types);
PageInfo<Bill> page = billService.listPage(bill, pageNum, pageSize);
model.addAttribute("page", page);
return "/bill/list-page";
}
@RequestMapping("toAddPage")
public String toAdd(Model model) {
List<BillType> billTypes = typeService.selectAll();
model.addAttribute("types", billTypes);
return "/bill/add";
}
@RequestMapping(value = "", method = RequestMethod.POST)
public String add(Bill bill) {
billService.insert(bill);
return "redirect:/bill/list-page";
}
@RequestMapping(value = "{id}", method = RequestMethod.DELETE)
public String delete(@PathVariable("id") Long id) {
billService.delete(id);
return "redirect:/bill/list-page";
}
@RequestMapping("toUpdatePage/{id}")
public String update(@PathVariable("id") Long id, Model model) {
List<BillType> billTypes = typeService.selectAll();
model.addAttribute("types", billTypes);
Bill bill = billService.selectByPrimaryKey(id);
model.addAttribute("bill", bill);
return "/bill/update";
}
@RequestMapping(value = "", method = RequestMethod.PUT)
public String update(Bill bill) {
billService.updateByPrimaryKey(bill);
return "redirect:/bill/list-page";
}
}
list-page.html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8"/>
<title>userList</title>
<link rel="stylesheet" th:href="@{/css/bootstrap.css}"></link>
<script type="text/javascript" th:src="@{/js/My97DatePicker/WdatePicker.js}"></script>
<script type="text/javascript" src="/js/My97DatePicker/lang/zh-cn.js"></script>
<script type="text/javascript" th:src="@{/js/jquery/jquery-1.10.2.min.js}"></script>
</head>
<body class="container">
<br/>
<h1>账单列表</h1>
<br/><br/>
<div class="with:80%">
<form class="form-inline" id="qf" th:action="@{/bill/list-page}" method="post">
<!-- 隐藏对象PageNum(当前页数)和PageSize(每页行数) -->
<input type="hidden" name="pageNum" id="pageNum" th:value="${page.pageNum}">
<input type="hidden" name="pageSize" id="pageSize" th:value="${page.pageSize}">
<div class="form-group">
<label for="typeId" class="control-label">类型</label>
<select name="typeId" id="typeId" class="form-control">
<option value="">全部</option>
<option th:each="type: ${types}" th:value="${type.id}" th:text="${type.name}" th:selected="${type.id} + '' == ${#strings.trim(param.typeId)}"></option>
</select>
</div>
<div class="form-group">
<label for="date1" class="control-label" >开始时间</label>
<input type="text" class="form-control" name="date1" id="date1" placeholder="开始时间" onclick="WdatePicker()"/>
</div>
<div class="form-group">
<label for="date2" class="control-label">结束时间</label>
<input type="text" class="form-control" name="date2" id="date2" placeholder="结束时间" onclick="WdatePicker()"/>
</div>
<div class="form-group">
<input type="submit" value="查询" class="btn btn-info" />
<input type="reset" value="重置" class="btn btn-info" />
<a href="/bill/toAddPage" th:href="@{/bill/toAddPage}" class="btn btn-info">添加</a>
</div>
</form>
</div>
<br/>
<div class="with:80%">
<table class="table table-striped table-bordered">
<thead>
<tr>
<th>#</th>
<th>标题</th>
<th>时间</th>
<th>金额</th>
<th>类别</th>
<th>说明</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<tr th:each="bill, status : ${page.list}" th:object="${bill}" th:style="${status.even} ? 'backgroundcolor: grey'">
<td th:text="${bill.id}">1</td>
<td th:text="*{title}">1</td>
<td th:text="*{billTime} ? ${#dates.format(bill.billTime, 'yyyy-MM-dd')}">time</td>
<td th:text="*{price}">price</td>
<td th:text="*{typeName}">typename</td>
<td th:text="*{explain}">explain</td>
<td>
<a th:href="|toUpdatePage/*{id}|">修改</a>
<!--<a th:href="|delete/*{id}|">删除</a>-->
<a href="#" th:onclick="'javascript:subDelete()'">删除</a>
<form id="delete" th:action="|/bill/*{id}|" method="post">
<input type="hidden" value="DELETE" name="_method">
</form>
</td>
</tr>
</tbody>
</table>
</div>
<!-- 分页工具类-->
<ul class="pagination">
<li><button class="btn btn-default" id="first">第一页</button></li>
<li><button class="btn btn-default" id="prev">上一页</button></li>
<li th:each="p:${page.navigatepageNums}">
<button class="btn btn-default" id="pn" name="pn" th:text="${p}" th:disabled="(${p} == ${page.pageNum})"></button>
</li>
<li><button class="btn btn-default" id="next">下一页</button></li>
<li><button class="btn btn-default" id="last">最后页</button></li>
</ul>
<script th:inline="javascript">
function subDelete() {
document.getElementById("delete").submit();
}
</script>
<script>
<!-- 分页的js代码-->
$(function () {
// 通过内联的js得到分页相关数据
var pageNum = [[${page.pageNum}]];// 当前页
var pageCount = [[${page.pages}]];
var hasNextPage = [[${page.hasNextPage}]];
var hasPreviousPage = [[${page.hasPreviousPage}]];
// 绑定按钮事件
$("#next").click(function () {
$("#pageNum").val(pageNum + 1);
$("#qf").submit();// 提交表单
});
$("#prev").click(function () {
$("#pageNum").val(pageNum - 1);
$("#qf").submit();// 提交表单
});
$("#first").click(function () {
$("#pageNum").val(1);
$("#qf").submit();// 提交表单
});
$("#last").click(function () {
$("#pageNum").val(pageCount);
$("#qf").submit();// 提交表单
});
// 点击页面跳转
$("button[name='pn']").click(function () {
$("#pageNum").val($(this).html());
$("#qf").submit();// 提交表单
});
// 控制按钮状态
if (!hasNextPage) {
$("#next").prop("disabled", true);
$("#last").prop("disabled", true);
}
if (!hasPreviousPage) {
$("#prev").prop("disabled", true);
$("#first").prop("disabled", true);
}
});
</script>
</body>
</html>
add.html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8"/>
<title>user</title>
<link rel="stylesheet" th:href="@{/css/bootstrap.css}"></link>
<script type="text/javascript" th:src="@{/js/My97DatePicker/WdatePicker.js}"></script>
<script type="text/javascript" src="/js/My97DatePicker/lang/zh-cn.js"></script>
</head>
<body class="container">
<br/>
<h1>添加账单</h1>
<br/><br/>
<div class="with:80%">
<form class="form-horizontal" action="/bill/" method="post">
<input type="hidden" value="POST" name="_method">
<div class="form-group">
<label for="typeId" class="col-sm-2 control-label">类型</label>
<div class="col-sm-10">
<select name="typeId" id="typeId" class="form-control">
<!-- TODO 回显账单类型 -->
<option th:each="type: ${types}" th:value="${type.id}" th:text="${type.name}"></option>
</select>
</div>
</div>
<div class="form-group">
<label for="title" class="col-sm-2 control-label" >标题</label>
<div class="col-sm-10">
<input type="text" class="form-control" name="title" id="title" placeholder="标题"/>
</div>
</div>
<div class="form-group">
<label for="billTime" class="col-sm-2 control-label">日期</label>
<div class="col-sm-10">
<input type="text" class="form-control" name="billTime" id="billTime" placeholder="日期" onclick="WdatePicker()"/>
</div>
</div>
<div class="form-group">
<label for="price" class="col-sm-2 control-label">金额</label>
<div class="col-sm-10">
<input type="text" class="form-control" name="price" id="price" placeholder="金额"/>
</div>
</div>
<div class="form-group">
<label for="explain" class="col-sm-2 control-label">说明</label>
<div class="col-sm-10">
<input type="text" class="form-control" name="explain" id="explain" placeholder="说明"/>
</div>
</div>
<div class="form-group">
<div class="col-sm-offset-2 col-sm-10">
<input type="submit" value="保存" class="btn btn-info" />
<input type="reset" value="重置" class="btn btn-info" />
</div>
</div>
</form>
</div>
</body>
</html>
update.html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8"/>
<title>user</title>
<link rel="stylesheet" th:href="@{/css/bootstrap.css}"></link>
<script type="text/javascript" th:src="@{/js/My97DatePicker/WdatePicker.js}"></script>
<script type="text/javascript" src="/js/My97DatePicker/lang/zh-cn.js"></script>
</head>
<body class="container">
<br/>
<h1>修改账单</h1>
<br/><br/>
<div class="with:80%">
<!-- TODO 回显数据 -->
<form class="form-horizontal" action="/bill/" method="post" th:object="${bill}">
<input type="hidden" value="PUT" name="_method">
<!--注意这里要添加隐藏对象,用来标记修改对象的id-->
<input type="hidden" name="id" th:value="*{id}">
<div class="form-group">
<label for="typeId" class="col-sm-2 control-label">类型</label>
<div class="col-sm-10">
<select name="typeId" id="typeId" class="form-control">
<!-- TODO 回显账单类型 -->
<option th:each="type: ${types}" th:value="${type.id}" th:text="${type.name}" th:selected="${type.id} + '' == *{typeId}"></option>
</select>
</div>
</div>
<div class="form-group">
<label for="title" class="col-sm-2 control-label" >标题</label>
<div class="col-sm-10">
<input type="text" th:value="*{title}" class="form-control" name="title" id="title" placeholder="标题"/>
</div>
</div>
<div class="form-group">
<label for="billTime" class="col-sm-2 control-label">日期</label>
<div class="col-sm-10">
<input type="text" th:value="*{#dates.format(billTime, 'yyyy-MM-dd')}" class="form-control" name="billTime" id="billTime" placeholder="日期" onclick="WdatePicker()"/>
</div>
</div>
<div class="form-group">
<label for="price" class="col-sm-2 control-label">金额</label>
<div class="col-sm-10">
<input type="text" th:value="*{price}" class="form-control" name="price" id="price" placeholder="金额"/>
</div>
</div>
<div class="form-group">
<label for="explain" class="col-sm-2 control-label">说明</label>
<div class="col-sm-10">
<input type="text" th:value="*{explain}" class="form-control" name="explain" id="explain" placeholder="说明"/>
</div>
</div>
<div class="form-group">
<div class="col-sm-offset-2 col-sm-10">
<input type="submit" value="保存" class="btn btn-info" />
<input type="reset" value="重置" class="btn btn-info" />
</div>
</div>
</form>
</div>
</body>
</html>