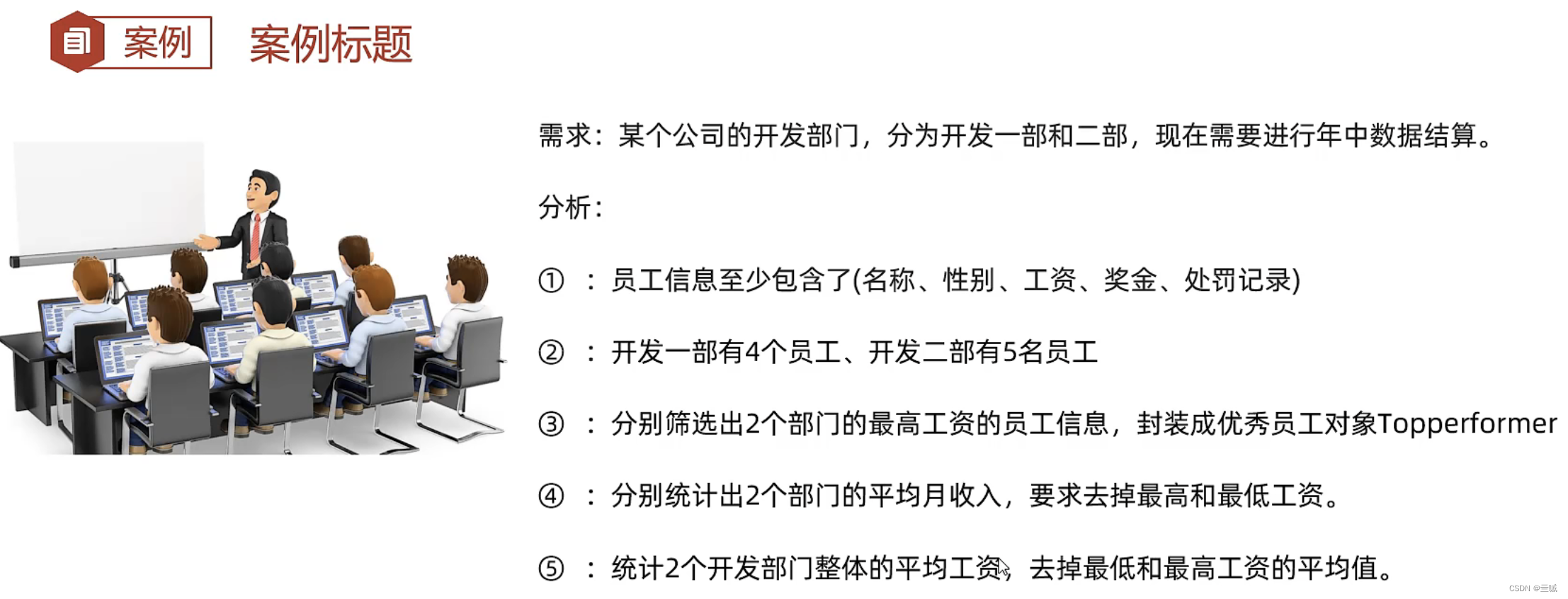
员工属性类:
package Java_project_1;
public class Employee {
private String name;//姓名
private char sex;//性别
private double salary;//薪水
private double bonus;//奖金
private String punish;//处罚
@Override
public String toString() {
return "Employee{" +
"name='" + name + '\'' +
", sex=" + sex +
", salary=" + salary +
", bonus=" + bonus +
", punish='" + punish + '\'' +
'}';
}
public Employee() {
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public char getSex() {
return sex;
}
public void setSex(char sex) {
this.sex = sex;
}
public double getSalary() {
return salary;
}
public void setSalary(double salary) {
this.salary = salary;
}
public double getBonus() {
return bonus;
}
public void setBonus(double bonus) {
this.bonus = bonus;
}
public String getPunish() {
return punish;
}
public void setPunish(String punish) {
this.punish = punish;
}
public Employee(String name, char sex, double salary, double bonus, String punish) {
this.name = name;
this.sex = sex;
this.salary = salary;
this.bonus = bonus;
this.punish = punish;
}
}
优秀员工属性类:
package Java_project_1;
public class Topperformer {
private String name;
private double money;//月薪
public Topperformer(String name, double money) {
this.name = name;
this.money = money;
}
public String getName() {
return name;
}
@Override
public String toString() {
return "Topperformer{" +
"name='" + name + '\'' +
", money=" + money +
'}';
}
public void setName(String name) {
this.name = name;
}
public double getMoney() {
return money;
}
public void setMoney(double money) {
this.money = money;
}
public Topperformer() {
}
}
Stream流体系类:
package Java_project_1;
import java.math.BigDecimal;
import java.math.RoundingMode;
import java.util.ArrayList;
import java.util.List;
import java.util.stream.Stream;
public class StreamTest {
public static double allMoney;
public static double allMoney2;
public static void main(String[] args) {
//导入员工数据
List<Employee>one=new ArrayList<>();
one.add(new Employee("猪八戒",'男',30000,25000,null));
one.add(new Employee("孙悟空",'男',25000,1000,"顶撞上司"));
one.add(new Employee("沙僧",'男',20000,20000,null));
one.add(new Employee("小白龙",'男',20000,25000,null));
List<Employee> two = new ArrayList<>();
two.add(new Employee("武松",'男',15000,9000,null));
two.add(new Employee("李逵",'男',20000,10000,null));
two.add(new Employee("西门庆",'男',50000,100000,"被打"));
two.add(new Employee("潘金莲",'男',3500,1000,"被打"));
two.add(new Employee("武大郎",'男',20000,0,"下毒"));
//取最高工资员工的信息
//指定max规则实现后封装
Topperformer t=one.stream().max((e1,e2)->
Double.compare(e1.getSalary()+e1.getBonus(), e2.getSalary()+ e2.getBonus()))
.map(e->new Topperformer(e.getName(), e.getSalary()+e.getBonus())).get();
System.out.println(t);
//统计平均工资,去掉最高和最低工资
one.stream().sorted((e1,e2)->Double.compare(e1.getSalary()+ e1.getBonus(), e2.getSalary()+ e2.getBonus()))
.skip(1).limit(one.size()-2).forEach(e->{
allMoney+=(e.getSalary()+e.getBonus());
});
System.out.println("开发一部的平均工资"+allMoney/(one.size()-2));
//合并2个集合流
Stream<Employee>s1=one.stream();
Stream<Employee>s2=two.stream();
Stream<Employee>s3= Stream.concat(s1,s2);
s3.sorted((e1,e2)->Double.compare(e1.getSalary()+ e1.getBonus(), e2.getSalary()+ e2.getBonus()))
.skip(1).limit(one.size()+ two.size()-2).forEach(e->{
allMoney2+=(e.getSalary()+e.getBonus());
});
//给予精确值
BigDecimal a=BigDecimal.valueOf(allMoney2);
BigDecimal b=BigDecimal.valueOf(one.size()+ two.size()-2);
System.out.println("开发部的平均工资"+a.divide(b,2, RoundingMode.HALF_UP));
}
}