C# 调用WebService的方式汇总
- 方式一:根据提供的webservice地址,用VS自带工具生成cs文件,添加到项目中使用即可。
- 方式二:根据webservice地址,动态在项目中生成代理类文件,通过反射调用即可
- 方式三:通过 HttpWebRequest 直接调用webservice中的函数
方式一:根据提供的webservice地址,用VS自带工具生成cs文件,添加到项目中使用即可。
工具:
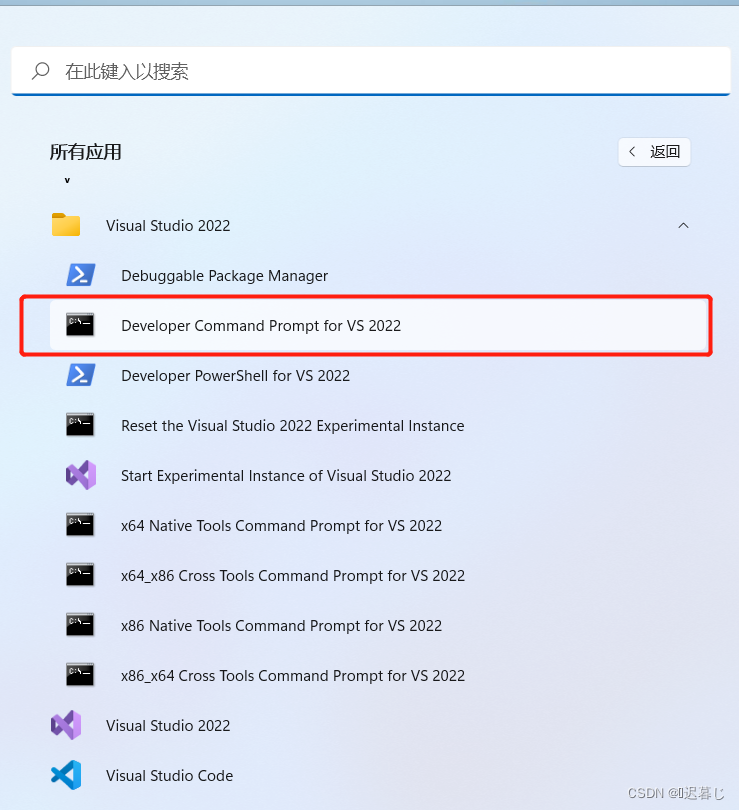
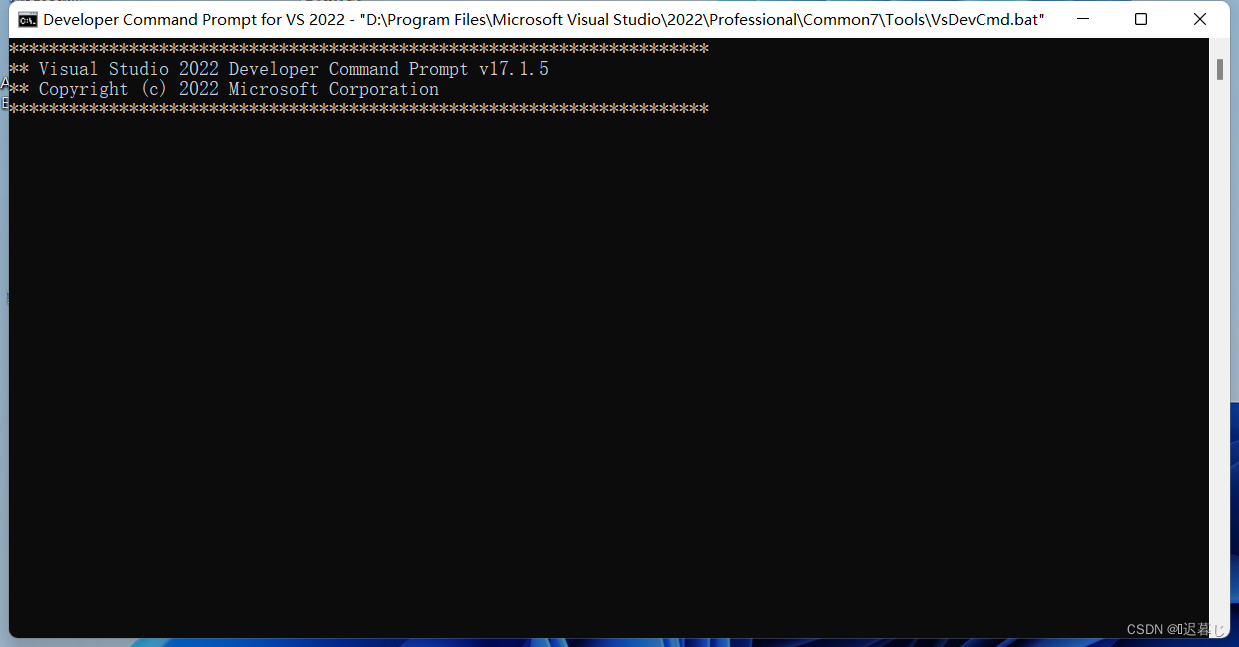
步骤一:如果本地访问不了webservice地址,则通过远端生成wsdl文件,拷贝到本地用工具生成cs类文件,导入项目中。
1.获取webservice地址;
2.在浏览器中访问webservice地址,此处访问需要在地址后面加上 ?wsdl
例如:原地址:http://42.123.92.137:1010/interface/Hospital_Interface.asmx
访问时:http://42.123.92.137:1010/interface/Hospital_Interface.asmx?wsdl
3.将网页中的数据另存为 (xxx.wsdl)文件,
3.用VS自带工具将上一步生成的(xxx.wsdl)文件通过命令生成 (xxx.cs) 类文件。
(1)直接通过wsdl文件位置生成。公式:wsdl + wsdl文件路径 + /out:+ cs生成文件路径
例:wsdl E:\service.wsdl /out:E:/WebServicetest.cs
(2)生成带有命名空间的cs文件。公式:wsdl + wsdl文件路径 + /n:命名空间名称 +/out:+ cs生成文件路径
例:wsdl E:\service.wsdl /n:test /out:E:/WebServicetest.cs
步骤二:如果本地可以直接访问到webservice地址,直接通过VS工具生成
生成公式:wsdl /n:+命名空间名称+ /out:+cs类文件路径+文件名称.cs+ webservice地址+?wsdl (注意公式里面空格的地方)
例:wsdl /n:xx /out:D:/web.cs http://192.168.0.222/WebSite/Service.asmx?wsdl
方式二:根据webservice地址,动态在项目中生成代理类文件,通过反射调用即可
1.将webservice地址存放到配置文件中,代码中通过读取地址生成代理类。
public static int InitCreatClass(string url)
{
try
{
XmlTextReader reader = new XmlTextReader(url + "?wsdl");
ServiceDescription serviceDes = ServiceDescription.Read(reader);
ServiceDescriptionImporter sdi = new ServiceDescriptionImporter();
sdi.AddServiceDescription(serviceDes, "", "");
CodeNamespace cnspace = new CodeNamespace(@namespace);
CodeCompileUnit ccUnit = new CodeCompileUnit();
ccUnit.Namespaces.Add(cnspace);
sdi.Import(cnspace, ccUnit);
CSharpCodeProvider cscProvider = new CSharpCodeProvider();
ICodeCompiler icc = cscProvider.CreateCompiler();
CompilerParameters copParameters = new CompilerParameters();
copParameters.GenerateExecutable = false;
copParameters.GenerateInMemory = true;
copParameters.OutputAssembly = "TestWebService.dll";
copParameters.ReferencedAssemblies.Add("System.dll");
copParameters.ReferencedAssemblies.Add("System.XML.dll");
copParameters.ReferencedAssemblies.Add("System.Web.Services.dll");
copParameters.ReferencedAssemblies.Add("System.Data.dll");
CompilerResults comResults = icc.CompileAssemblyFromDom(copParameters, ccUnit);
if (true == comResults.Errors.HasErrors)
{
System.Text.StringBuilder sb = new System.Text.StringBuilder();
foreach (System.CodeDom.Compiler.CompilerError ce in comResults.Errors)
{
sb.Append(ce.ToString());
sb.Append(System.Environment.NewLine);
}
throw new Exception(sb.ToString());
}
}
catch (Exception ex)
{
throw new Exception(ex.Message);
}
return 0;
}
2.访问代理类,调用类中的函数
public static object InvokeWebService(string methodname, object[] args)
{
try
{
System.Reflection.Assembly asm = System.Reflection.Assembly.LoadFrom("TestWebService.dll");
Type t = asm.GetType(classname);
object o = Activator.CreateInstance(t);
System.Reflection.MethodInfo method = t.GetMethod(methodname);
return method.Invoke(o, args);
}
catch (Exception ex)
{
throw new Exception(ex.InnerException.Message, new Exception(ex.InnerException.StackTrace));
}
}
private void TestInvoke()
{
string[] ReqParms = new string[4];
ReqParms[0] = "参数1";
ReqParms[1] = "参数2";
ReqParms[2] = "参数3";
ReqParms[3] = "参数4";
string result = InvokeWebServiceD("Func_Name", ReqParms).ToString();
}
方式三:通过 HttpWebRequest 直接调用webservice中的函数
构建httprequest帮助类
public partial class HttpHelper
{
private static HttpHelper m_Helper;
public static HttpHelper Helper
{
get { return m_Helper ?? (m_Helper = new HttpHelper()); }
}
public string GetResponseString(string strUrl, ERequestMode requestMode, Dictionary<string, string> parameters, Encoding requestCoding, Encoding responseCoding, int timeout = 300)
{
string url = VerifyUrl(strUrl);
HttpWebRequest webRequest = (HttpWebRequest)WebRequest.Create(new Uri(url));
HttpWebResponse webResponse = null;
switch (requestMode)
{
case ERequestMode.Get:
webResponse = GetRequest(webRequest, timeout);
break;
case ERequestMode.Post:
webResponse = PostRequest(webRequest, parameters, timeout, requestCoding);
break;
}
if (webResponse != null && webResponse.StatusCode == HttpStatusCode.OK)
{
using (Stream newStream = webResponse.GetResponseStream())
{
if (newStream != null)
using (StreamReader reader = new StreamReader(newStream, responseCoding))
{
string result = reader.ReadToEnd();
return result;
}
}
}
return null;
}
private HttpWebResponse GetRequest(HttpWebRequest webRequest, int timeout)
{
try
{
webRequest.Accept = "text/html, application/xhtml+xml, application/json, text/javascript, */*; q=0.01";
webRequest.Headers.Add("Accept-Language", "zh-cn,en-US,en;q=0.5");
webRequest.Headers.Add("Cache-Control", "no-cache");
webRequest.UserAgent = "DefaultUserAgent";
webRequest.Timeout = timeout;
webRequest.Method = "GET";
HttpWebResponse webResponse = (HttpWebResponse)webRequest.GetResponse();
return webResponse;
}
catch (Exception ex)
{
return null;
}
}
private HttpWebResponse PostRequest(HttpWebRequest webRequest, Dictionary<string, string> parameters, int timeout, Encoding requestCoding)
{
try
{
string postStr = string.Empty;
if (parameters != null)
{
parameters.All(o =>
{
if (string.IsNullOrEmpty(postStr))
postStr = string.Format("{0}={1}", HttpUtility.UrlEncode(o.Key), HttpUtility.UrlEncode(o.Value));
else
postStr += string.Format("&{0}={1}", HttpUtility.UrlEncode(o.Key), HttpUtility.UrlEncode(o.Value));
return true;
});
}
byte[] byteArray = requestCoding.GetBytes(postStr);
webRequest.Accept = "text/html, application/xhtml+xml, application/json, text/javascript, */*; q=0.01";
webRequest.Headers.Add("Accept-Language", "zh-cn,en-US,en;q=0.5");
webRequest.Headers.Add("Cache-Control", "no-cache");
webRequest.UserAgent = "DefaultUserAgent";
webRequest.ContentType = "application/x-www-form-urlencoded";
webRequest.ContentLength = byteArray.Length;
webRequest.Method = "POST";
using (Stream newStream = webRequest.GetRequestStream())
{
newStream.Write(byteArray, 0, byteArray.Length);
newStream.Close();
}
HttpWebResponse webResponse = (HttpWebResponse)webRequest.GetResponse();
return webResponse;
}
catch (Exception ex)
{
return null;
}
}
private string VerifyUrl(string url)
{
if (string.IsNullOrEmpty(url))
throw new Exception("URL 地址不可以为空!");
if (url.StartsWith("http://", StringComparison.CurrentCultureIgnoreCase))
return url;
return string.Format("http://{0}", url);
}
}
public enum ERequestMode
{
Get,
Post
}
调用方式
private void TestInvoke()
{
Dictionary<string, string> parameters = new Dictionary<string, string>();
parameters.Add("CantonCode", PersonInfo.RegionNumber);
parameters.Add("CardCode", PersonInfo.CardNo);
parameters.Add("Name", txtName.Text);
parameters.Add("CompHosCode", SystemConfigData.HospitalCode);
string _result = HttpHelper.Helper.GetResponseString(url, ERequestMode.Post, parameters, Encoding.Default, Encoding.UTF8);
}
以下是对上面三种方式进行总结。
方式一、
优点:利用地址直接生成cs类,操作方便快捷,发生错误机率小,调用简单;
缺点:如果webservice地址或者内容发生改变,项目必须重新生成cs文件,导致项目维护性差;
采用场景:webservice接口已经趋于稳定,不会有变动的情况下可使用这种方式;
方式二、
优点:将webservice地址放在配置文件中,一旦地址放生变化,只需要修改配置文件即可,项目维护性好;
缺点:对webservice接口要求比较高,不规范的webservice接口,生成代理类时会发生错误;
采用场景:webservice接口规范,且变动小时,可采用此种方式;
方式三、
优点:添加帮助类,然后调用即可,方便快捷,webservice地址放置在配置文件中,易于维护;
缺点:暂时未发现较大缺点;
采用场景:任何webservice接口,均可采用此种方式;
使用推荐:方式三 > 方式一 > 方式二
本文内容由网友自发贡献,版权归原作者所有,本站不承担相应法律责任。如您发现有涉嫌抄袭侵权的内容,请联系:hwhale#tublm.com(使用前将#替换为@)