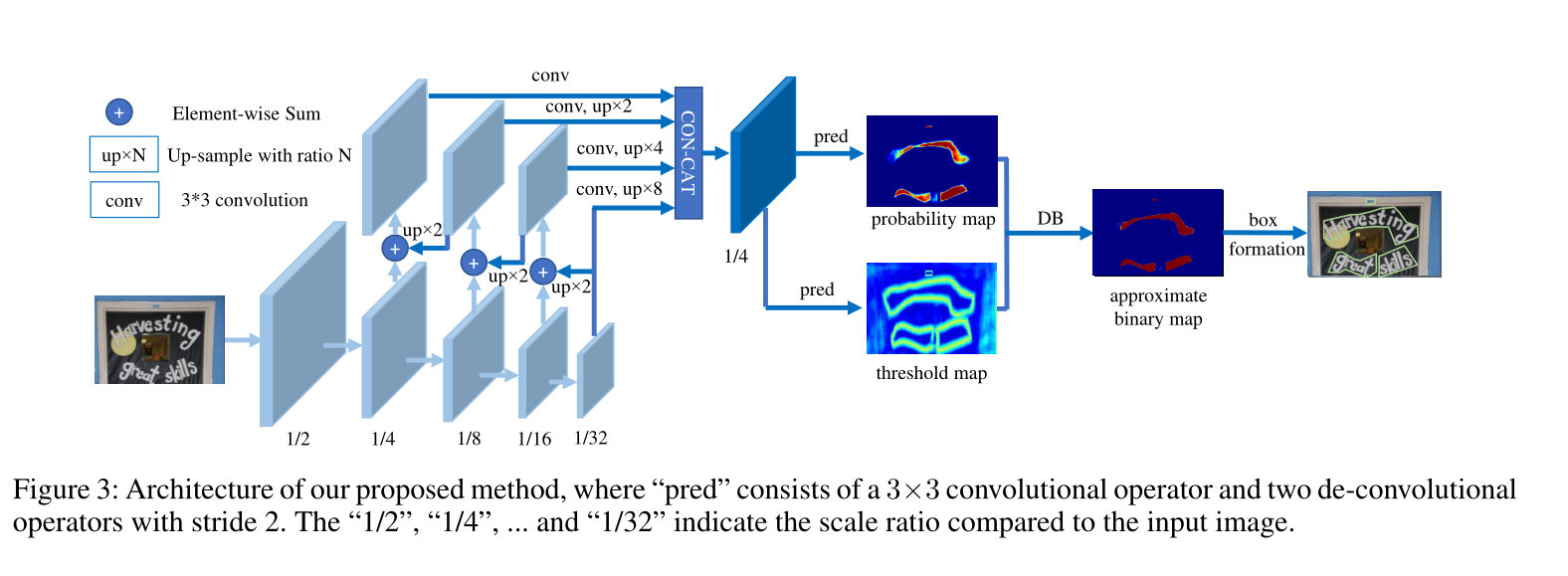
MakeBorderMap的输入是polygon,输出是“threshold_map”和对应的“threshold_mask”。
threshold_map 是由polygon进行内外延展而来,shrink_ratio设置得越大,延展得越小。
threshold_map 越靠近polygon的数值越高,threshold_map 中数值最小为thresh_min,数值最大为thresh_max。
threshold_mask 是覆盖polygon外延展后的mask区域。
下图的threshold_map 0.4 ,是当shrink_ratio 设置为0.4 出来的图,此时内外延展是比设置为0.9要大的。
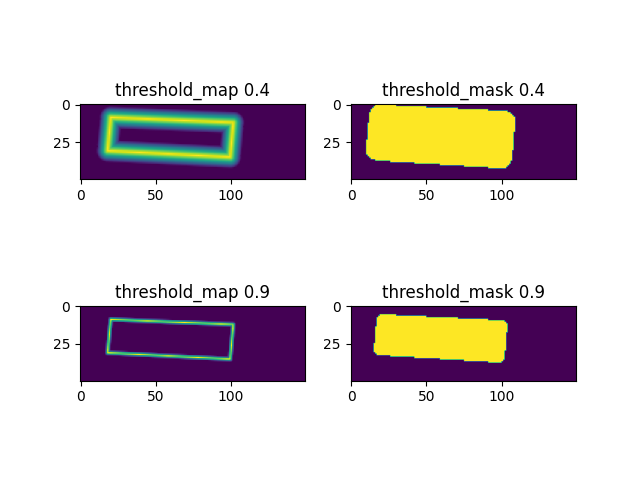
下面是测试的代码:
import cv2
import numpy as np
import pyclipper
from shapely.geometry import Polygon
class MakeBorderMap(object):
def __init__(self,
shrink_ratio=0.4,
thresh_min=0.3,
thresh_max=0.7,
**kwargs):
self.shrink_ratio = shrink_ratio
self.thresh_min = thresh_min
self.thresh_max = thresh_max
def __call__(self, polygon):
data = {}
canvas = np.zeros((640, 640), dtype=np.float32)
mask = np.zeros((640, 640), dtype=np.float32)
self.draw_border_map(polygon, canvas, mask=mask)
canvas = canvas * (self.thresh_max - self.thresh_min) + self.thresh_min
data['threshold_map'] = canvas
data['threshold_mask'] = mask
return data
def draw_border_map(self, polygon, canvas, mask):
polygon = np.array(polygon)
assert polygon.ndim == 2
assert polygon.shape[1] == 2
polygon_shape = Polygon(polygon)
if polygon_shape.area <= 0:
return
distance = polygon_shape.area * (
1 - np.power(self.shrink_ratio, 2)) / polygon_shape.length
subject = [tuple(l) for l in polygon]
padding = pyclipper.PyclipperOffset()
padding.AddPath(subject, pyclipper.JT_ROUND, pyclipper.ET_CLOSEDPOLYGON)
padded_polygon = np.array(padding.Execute(distance)[0])
cv2.fillPoly(mask, [padded_polygon.astype(np.int32)], 1.0)
xmin = padded_polygon[:, 0].min()
xmax = padded_polygon[:, 0].max()
ymin = padded_polygon[:, 1].min()
ymax = padded_polygon[:, 1].max()
width = xmax - xmin + 1
height = ymax - ymin + 1
polygon[:, 0] = polygon[:, 0] - xmin
polygon[:, 1] = polygon[:, 1] - ymin
xs = np.broadcast_to(np.linspace(0, width - 1, num=width).reshape(1, width), (height, width))
ys = np.broadcast_to(np.linspace(0, height - 1, num=height).reshape(height, 1), (height, width))
distance_map = np.zeros((polygon.shape[0], height, width), dtype=np.float32)
for i in range(polygon.shape[0]):
j = (i + 1) % polygon.shape[0]
absolute_distance = self._distance(xs, ys, polygon[i], polygon[j])
distance_map[i] = np.clip(absolute_distance / distance, 0, 1)
distance_map = distance_map.min(axis=0)
xmin_valid = min(max(0, xmin), canvas.shape[1] - 1)
xmax_valid = min(max(0, xmax), canvas.shape[1] - 1)
ymin_valid = min(max(0, ymin), canvas.shape[0] - 1)
ymax_valid = min(max(0, ymax), canvas.shape[0] - 1)
canvas[ymin_valid:ymax_valid + 1, xmin_valid:xmax_valid + 1] = np.fmax(
1 - distance_map[ymin_valid - ymin:ymax_valid - ymax + height,
xmin_valid - xmin:xmax_valid - xmax + width],
canvas[ymin_valid:ymax_valid + 1, xmin_valid:xmax_valid + 1])
def _distance(self, xs, ys, point_1, point_2):
'''
compute the distance from point to a line
ys: coordinates in the first axis
xs: coordinates in the second axis
point_1, point_2: (x, y), the end of the line
'''
height, width = xs.shape[:2]
square_distance_1 = np.square(xs - point_1[0]) + np.square(ys - point_1[
1])
square_distance_2 = np.square(xs - point_2[0]) + np.square(ys - point_2[
1])
square_distance = np.square(point_1[0] - point_2[0]) + np.square(
point_1[1] - point_2[1])
cosin = (square_distance - square_distance_1 - square_distance_2) / (
2 * np.sqrt(square_distance_1 * square_distance_2))
square_sin = 1 - np.square(cosin)
square_sin = np.nan_to_num(square_sin)
result = np.sqrt(square_distance_1 * square_distance_2 * square_sin /
square_distance)
result[cosin <
0] = np.sqrt(np.fmin(square_distance_1, square_distance_2))[cosin
< 0]
return result
def extend_line(self, point_1, point_2, result, shrink_ratio):
ex_point_1 = (int(
round(point_1[0] + (point_1[0] - point_2[0]) * (1 + shrink_ratio))),
int(
round(point_1[1] + (point_1[1] - point_2[1]) * (
1 + shrink_ratio))))
cv2.line(
result,
tuple(ex_point_1),
tuple(point_1),
4096.0,
1,
lineType=cv2.LINE_AA,
shift=0)
ex_point_2 = (int(
round(point_2[0] + (point_2[0] - point_1[0]) * (1 + shrink_ratio))),
int(
round(point_2[1] + (point_2[1] - point_1[1]) * (
1 + shrink_ratio))))
cv2.line(
result,
tuple(ex_point_2),
tuple(point_2),
4096.0,
1,
lineType=cv2.LINE_AA,
shift=0)
return ex_point_1, ex_point_2
if __name__ == '__main__':
polygon = [[151.24755859, 187.33417013],
[69.91391389, 183.99514903],
[67.82457435, 205.93434209],
[149.14115574, 210.08720066]]
shrink_ratio = 0.4
thresh_min = 0.3
thresh_max = 0.7
MakeBorderMapI = MakeBorderMap(shrink_ratio, thresh_min, thresh_max)
data = MakeBorderMapI(polygon)
canvas = data['threshold_map']
mask = data['threshold_mask']
canvas1 = canvas[175:225, 50:200]
mask1 = mask[175:225, 50:200]
shrink_ratio = 0.9
MakeBorderMapI = MakeBorderMap(shrink_ratio, thresh_min, thresh_max)
data = MakeBorderMapI(polygon)
canvas = data['threshold_map']
mask = data['threshold_mask']
canvas2 = canvas[175:225, 50:200]
mask2 = mask[175:225, 50:200]
import matplotlib.pyplot as plt
plt.subplot(221)
plt.title('threshold_map 0.4')
plt.imshow(canvas1)
plt.subplot(222)
plt.title('threshold_mask 0.4')
plt.imshow(mask1)
plt.subplot(223)
plt.title('threshold_map 0.9')
plt.imshow(canvas2)
plt.subplot(224)
plt.title('threshold_mask 0.9')
plt.imshow(mask2)
plt.show()
本文内容由网友自发贡献,版权归原作者所有,本站不承担相应法律责任。如您发现有涉嫌抄袭侵权的内容,请联系:hwhale#tublm.com(使用前将#替换为@)