Flask 基础知识
基本框架结构
from flask import Flask
from sqlalchemy import create_engine
from sqlalchemy.orm import sessionmaker
from database_setup import Base, Restaurant, MenuItem
app = Flask(__name__)
engine = create_engine('sqlite:///restaurantmenu.db')
Base.metadata.bind = engine
DBSession = sessionmaker(bind=engine)
session = DBSession()
@app.route('/')
@app.route('/hello')
def HelloWorld():
restaurant = session.query(Restaurant).first()
items = session.query(MenuItem).filter_by(restaurant_id=restaurant.id)
output = ''
for i in items:
output += i.name
output += '</br>'
output += i.price
output += '</br>'
output += i.description
output += '</br>'
output += '</br>'
return output
if __name__ == '__main__':
app.debug = True
app.run(host='0.0.0.0', port=5000)
路由 route
Flask Documentation on Routing :
http://flask.pocoo.org/docs/0.10/quickstart/#routing
flask路由的格式为/path/<type:value>/path
其中,type可以为string, int, path
模板 template
HTML Character Escaping is a way of writing special characters inside of HTML code, this is a different concept from the HTML escaping with python code
注意模板文件都应该放入名为templates的文件夹。
URL-Buiding : url_for
url_for 用来将模板里的超链接转化为flask定义的后端接口函数。例如:
./templates/menu.html
<h1>{{restaurant.name}}</h1>
{% for i in items %}
<br>
<h2>{{i.name}}</h2>
{{i.description}}
<br>
{{i.price}}
<a href={{url_for('edit_menu_item', restaurant_id=restaurant.id, menu_id=i.id)}}>Edit</a>
<a href={{url_for('delete_menu_item', restaurant_id=restaurant.id, menu_id=i.id)}}>Delete</a>
<br>
<br>
{% endfor %}
./flask_server.py
import flask
from flask import Flask
from sqlalchemy import create_engine
from sqlalchemy.orm import sessionmaker
from database_setup import Base, Restaurant, MenuItem
app = Flask(__name__)
engine = create_engine('sqlite:///restaurantmenu.db')
Base.metadata.bind = engine
DBSession = sessionmaker(bind=engine)
session = DBSession()
@app.route('/')
@app.route('/hello')
def HelloWorld():
restaurant = session.query(Restaurant).first()
items = session.query(MenuItem).filter_by(restaurant_id=restaurant.id)
output = ''
for i in items:
output += i.name
output += '</br>'
output += i.price
output += '</br>'
output += i.description
output += '</br>'
output += '</br>'
return output
@app.route("/restaurant/<int:restaurant_id>/new")
def new_menu_item(restaurant_id):
return "create new menu item for the Restaurant(id={})".format(restaurant_id)
@app.route("/restaurant/<int:restaurant_id>/edit/<int:menu_id>")
def edit_menu_item(restaurant_id, menu_id):
return "edit the Menu(id={}) in Restaurant(id={})".format(menu_id, restaurant_id)
@app.route("/restaurant/<int:restaurant_id>/delete/<int:menu_id>")
def delete_menu_item(restaurant_id, menu_id):
return "delete the Menu(id={}) in Restaurant(id={})".format(menu_id, restaurant_id)
@app.route("/restaurant/<int:restaurant_id>/menus")
def menus(restaurant_id):
restaurant = session.query(Restaurant).filter_by(id=restaurant_id).first()
if restaurant:
menus = session.query(MenuItem).filter_by(restaurant_id=restaurant_id).all()
return flask.render_template('menu.html', items=menus, restaurant=restaurant)
return "you come to a wrong page!"
if __name__ == '__main__':
app.debug = True
app.run(host='0.0.0.0', port=8080)
Form Requests and Redirects 表单提交和跳转
常用的三个函数
from flask import url_for, redirect, request
url_for 用于与响应函数绑定
redirect用于重定向
request用于获取form表单中的值
import flask
from flask import Flask, url_for, redirect, request
from sqlalchemy import create_engine
from sqlalchemy.orm import sessionmaker
from database_setup import Base, Restaurant, MenuItem
app = Flask(__name__)
engine = create_engine('sqlite:///restaurantmenu.db')
Base.metadata.bind = engine
DBSession = sessionmaker(bind=engine)
session = DBSession()
@app.route('/')
@app.route('/hello')
def HelloWorld():
restaurant = session.query(Restaurant).first()
items = session.query(MenuItem).filter_by(restaurant_id=restaurant.id)
output = ''
for i in items:
output += i.name
output += '</br>'
output += i.price
output += '</br>'
output += i.description
output += '</br>'
output += '</br>'
return output
@app.route("/restaurant/<int:restaurant_id>/new")
def new_menu_item(restaurant_id):
return "create new menu item for the Restaurant(id={})".format(restaurant_id)
@app.route("/restaurant/<int:restaurant_id>/edit/<int:menu_id>", methods=['GET', 'POST'])
def edit_menu_item(restaurant_id, menu_id):
menu = session.query(MenuItem).filter_by(id=menu_id).first()
if request.method == 'POST':
if request.form['name']:
menu.name = request.form['name']
session.add(menu)
session.commit()
return redirect(url_for('menus', restaurant_id=restaurant_id))
else:
return flask.render_template('editmenu.html', item=menu)
@app.route("/restaurant/<int:restaurant_id>/delete/<int:menu_id>")
def delete_menu_item(restaurant_id, menu_id):
return "delete the Menu(id={}) in Restaurant(id={})".format(menu_id, restaurant_id)
@app.route("/restaurant/<int:restaurant_id>/menus")
def menus(restaurant_id):
restaurant = session.query(Restaurant).filter_by(id=restaurant_id).first()
if restaurant:
menus = session.query(MenuItem).filter_by(restaurant_id=restaurant_id).all()
return flask.render_template('menu.html', items=menus, restaurant=restaurant)
return "you come to a wrong page!"
if __name__ == '__main__':
app.debug = True
app.run(host='0.0.0.0', port=8080)
message flashing
message flashing 用于与用户进行交互,反映用户操作产生的结果。
from flask import flash
app.secret_key = ‘super_secret_key’ 用来创建用户相关的会话(session)事务
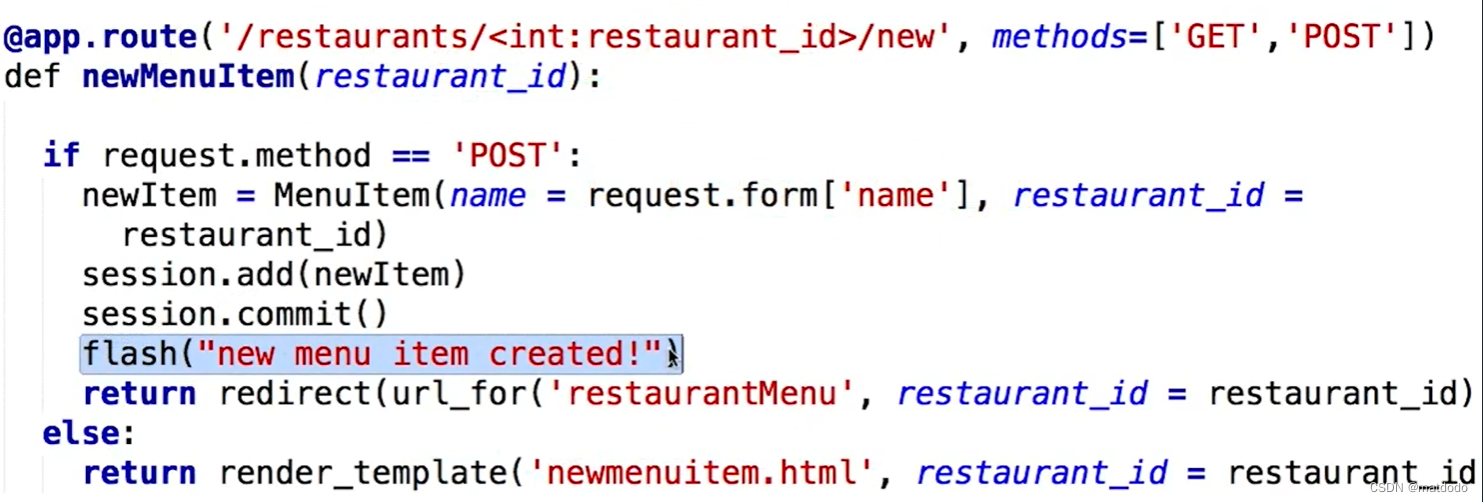
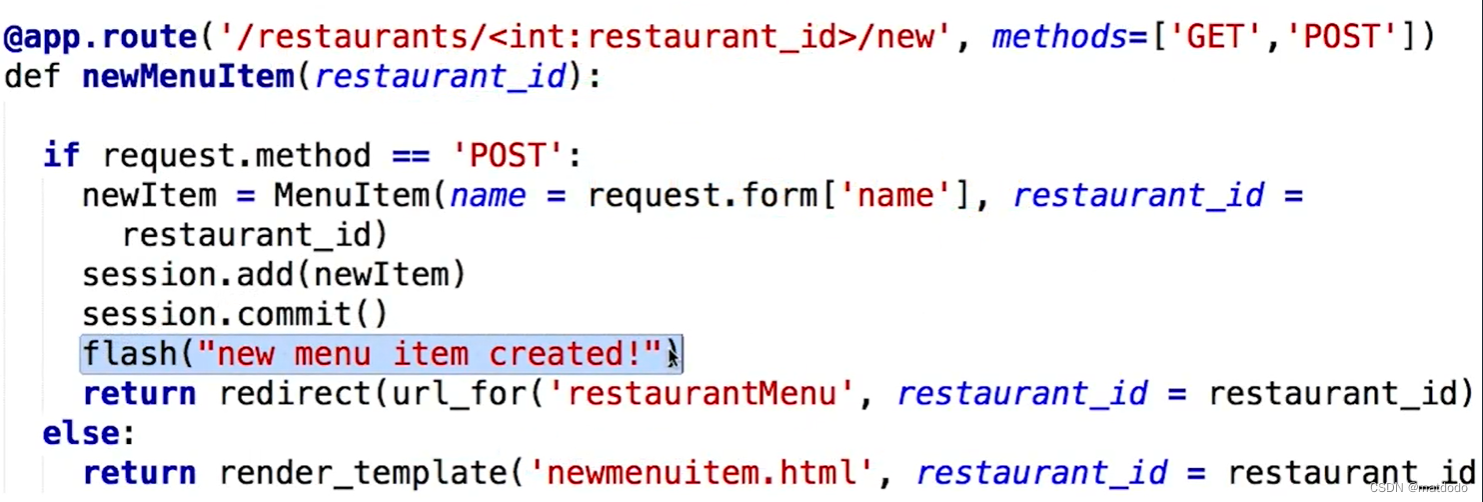
from crypt import methods
import flask
from flask import Flask, url_for, redirect, request, flash
from sqlalchemy import create_engine
from sqlalchemy.orm import sessionmaker
from database_setup import Base, Restaurant, MenuItem
app = Flask(__name__)
engine = create_engine('sqlite:///restaurantmenu.db')
Base.metadata.bind = engine
DBSession = sessionmaker(bind=engine)
session = DBSession()
@app.route('/')
@app.route('/hello')
def HelloWorld():
restaurant = session.query(Restaurant).first()
items = session.query(MenuItem).filter_by(restaurant_id=restaurant.id)
output = ''
for i in items:
output += i.name
output += '</br>'
output += i.price
output += '</br>'
output += i.description
output += '</br>'
output += '</br>'
return output
@app.route("/restaurant/<int:restaurant_id>/new", methods=['GET', 'POST'])
def new_menu_item(restaurant_id):
restaurant = session.query(Restaurant).filter_by(id=restaurant_id).first()
if restaurant:
if request.method == 'GET':
return flask.render_template('createmenuitem.html', restaurant=restaurant)
else:
menu_item_name = request.form['name']
if menu_item_name:
session.add(MenuItem(name=menu_item_name, restaurant_id=restaurant_id))
session.commit()
flash("successfully created a new Menu Item!")
return redirect(url_for('menus', restaurant_id=restaurant_id))
return "you come to a wrong page"
@app.route("/restaurant/<int:restaurant_id>/edit/<int:menu_id>", methods=['GET', 'POST'])
def edit_menu_item(restaurant_id, menu_id):
menu = session.query(MenuItem).filter_by(id=menu_id).first()
if request.method == 'POST':
if request.form['name']:
menu.name = request.form['name']
session.add(menu)
session.commit()
flash("one Menu item has been editted")
return redirect(url_for('menus', restaurant_id=restaurant_id))
else:
return flask.render_template('editmenu.html', item=menu)
@app.route("/restaurant/<int:restaurant_id>/delete/<int:menu_id>", methods=['GET','POST'])
def delete_menu_item(restaurant_id, menu_id):
menu = session.query(MenuItem).filter_by(id=menu_id).first()
if menu:
if request.method == 'POST':
session.delete(menu)
session.commit()
flash("one menu item has been deleted")
else:
return flask.render_template('deletemenu.html', item=menu)
return redirect(url_for('menus', restaurant_id=restaurant_id))
@app.route("/restaurant/<int:restaurant_id>/menus")
def menus(restaurant_id):
restaurant = session.query(Restaurant).filter_by(id=restaurant_id).first()
if restaurant:
menus = session.query(MenuItem).filter_by(restaurant_id=restaurant_id).all()
return flask.render_template('menu.html', items=menus, restaurant=restaurant)
return "you come to a wrong page!"
if __name__ == '__main__':
app.secret_key = 'super_secret_key'
app.debug = True
app.run(host='0.0.0.0', port=8080)
对象序列化便于传递数据
class MenuItem(Base):
__tablename__ = 'menu_item'
name =Column(String(80), nullable = False)
id = Column(Integer, primary_key = True)
description = Column(String(250))
price = Column(String(8))
course = Column(String(250))
restaurant_id = Column(Integer,ForeignKey('restaurant.id'))
restaurant = relationship(Restaurant)
@property
def serialize(self):
return {
'name' : self.name,
'description' : self.description,
'id' : self.id,
'price' : self.price,
'course' : self.course,
}
from flask import jsonify
上面的函数serialize将被jsonify调用进行对象序列化
@app.route('/restaurants/<int:restaurant_id>/menu/JSON')
def restaurantMenuJSON(restaurant_id):
restaurant = session.query(Restaurant).filter_by(id=restaurant_id).one()
items = session.query(MenuItem).filter_by(
restaurant_id=restaurant_id).all()
return jsonify(MenuItems=[i.serialize for i in items])
本文内容由网友自发贡献,版权归原作者所有,本站不承担相应法律责任。如您发现有涉嫌抄袭侵权的内容,请联系:hwhale#tublm.com(使用前将#替换为@)