String to Integer (atoi)
Implement atoi
which converts a string to an integer.
The function first discards as many whitespace characters as necessary until the first non-whitespace character is found. Then, starting from this character, takes an optional initial plus or minus sign followed by as many numerical digits as possible, and interprets them as a numerical value.
The string can contain additional characters after those that form the integral number, which are ignored and have no effect on the behavior of this function.
If the first sequence of non-whitespace characters in str is not a valid integral number, or if no such sequence exists because either str is empty or it contains only whitespace characters, no conversion is performed.
If no valid conversion could be performed, a zero value is returned.
Note:
- Only the space character
' '
is considered as whitespace character. - Assume we are dealing with an environment which could only store integers within the 32-bit signed integer range: [−231, 231 − 1]. If the numerical value is out of the range of representable values, INT_MAX (231 − 1) or INT_MIN (−231) is returned.
Example 1:
Input: "42"
Output: 42
Example 2:
Input: " -42"
Output: -42
Explanation: The first non-whitespace character is '-', which is the minus sign.
Then take as many numerical digits as possible, which gets 42.
Example 3:
Input: "4193 with words"
Output: 4193
Explanation: Conversion stops at digit '3' as the next character is not a numerical digit.
Example 4:
Input: "words and 987"
Output: 0
Explanation: The first non-whitespace character is 'w', which is not a numerical
digit or a +/- sign. Therefore no valid conversion could be performed.
Example 5:
Input: "-91283472332"
Output: -2147483648
Explanation: The number "-91283472332" is out of the range of a 32-bit signed integer.
Thefore INT_MIN (−231) is returned.
solution1
public int myAtoi1(String str) {
boolean converting = false;
final int len = str.length();
int i = 0;
boolean neg = false;
char c = 0;
while (i < len && ' ' == str.charAt(i)) {
i ++;
}
if (i == len) return 0;
c = str.charAt(i);
if ('-' == c) {
neg = true;
i ++;
} else if ('+' == c) {
i ++;
}
int result = 0;
while (i < len) {
c = str.charAt(i++);
if (c < '0' || c > '9') {
break;
}
int digit = c - '0';
if (result < Integer.MIN_VALUE/10 || result == Integer.MIN_VALUE/10 && digit == 9) {
return neg? Integer.MIN_VALUE:Integer.MAX_VALUE;
}
result = result*10 - digit;
}
if (neg) {
return result;
}
return result == Integer.MIN_VALUE? Integer.MAX_VALUE:-result;
}
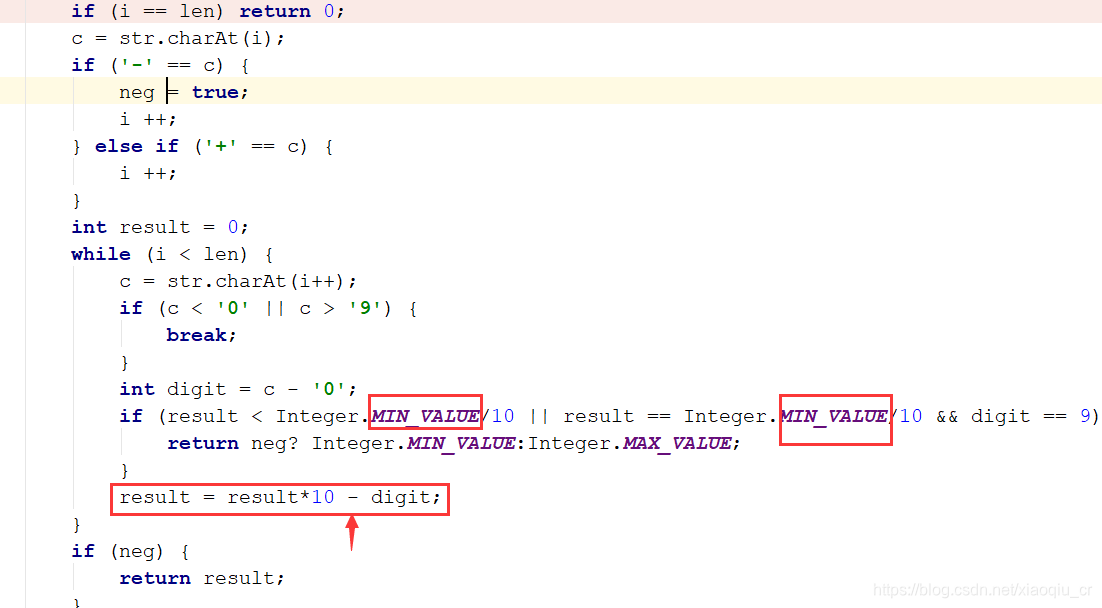
solution2
public int myAtoi(String str) {
if (str.isEmpty()) return 0;
int sign = 1, base = 0, i = 0, n = str.length();
while (i < n && str.charAt(i) == ' ') ++i;
if (i < n && (str.charAt(i) == '+' || str.charAt(i) == '-')) {
sign = (str.charAt(i++) == '+') ? 1 : -1;
}
while (i < n && str.charAt(i) >= '0' && str.charAt(i) <= '9') {
if (base > Integer.MAX_VALUE / 10 || (base == Integer.MAX_VALUE / 10 && str.charAt(i) - '0' > 7)) {
return (sign == 1) ? Integer.MAX_VALUE : Integer.MIN_VALUE;
}
base = 10 * base + (str.charAt(i++) - '0');
}
return base * sign;
}
solution3(精简)
public int myAtoi(String str) {
if (str == null || str.length() <= 0)
return 0;
int flg = 1;
int ret = 0;
int i = 0;
str = str.trim();
int len = str.length();
if (i < len && (str.charAt(i) == '+' || str.charAt(i) == '-')) {
flg = (str.charAt(i++) == '+') ? 1 : -1;
}
while (i < len && str.charAt(i) >= '0' && str.charAt(i) <= '9') {
if (ret > Integer.MAX_VALUE / 10 || (ret == Integer.MAX_VALUE / 10 && str.charAt(i) - '0' > 7)) {
return (flg == 1) ? Integer.MAX_VALUE : Integer.MIN_VALUE;
}
ret = 10 * ret + (str.charAt(i++) - '0');
}
return ret * flg;
}
wrong solution
class Solution {
public int myAtoi(String str) {
if(str == null)
return 0;
String newStr = str.trim();
if(newStr == null)
return 0;
int ret = 0;
int flg = 1;
if(newStr.length() >= 1){
char c = newStr.charAt(0);
int n = (int)newStr.charAt(0) - '0';
if(c != '+' && c != '-'){
if( n < 0 || n > 9 ){
return 0;
}
}
}
int j = 0;
if(newStr.length() >= 1){
if(newStr.charAt(0) == '+' || newStr.charAt(0) == '-'){
if(newStr.charAt(0) == '-'){
flg = -1;
j = 1;
}
if(newStr.charAt(0) == '+'){
flg = 1;
j = 1;
}
}
}
while(j < newStr.length() && (int)newStr.charAt(j) - '0' >= 0 && (int)newStr.charAt(j) - '0' <= 9 ){
if(flg == 1 && ret*flg >= Integer.MAX_VALUE / 10 && j == 9 && newStr.charAt(j) - '0' >= 8 ){
return Integer.MAX_VALUE;
}
if(flg == -1 && ret*flg <= (Integer.MIN_VALUE / 10 ) && j == 10 && newStr.charAt(j) - '0' >= 9){
return Integer.MIN_VALUE;
}
ret =ret * 10 + newStr.charAt(j) - '0';
if(ret*flg <= Integer.MIN_VALUE )
return Integer.MIN_VALUE;
if(ret*flg >= Integer.MAX_VALUE)
return Integer.MAX_VALUE;
j++;
}
ret = ret * flg;
if(ret <= Integer.MIN_VALUE )
return Integer.MIN_VALUE;
if(ret >= Integer.MAX_VALUE)
return Integer.MAX_VALUE;
return ret ;
}
}
wrong solution
class Solution {
public int myAtoi(String str) {
if(str == null || str.length <= 0)
return 0;
int ret = 0;
str = str.trim();
boolean isNeg = false;
int startIndex = 0;
if(str.charAt(0) == '+'){
startIndex++;
}
if(str.charAt(0) == '-'){
isNeg = true;
startIndex++;
}
for(int startIndex = 0; startIndex < str.length(); i++){
int digitNum = str.charAt(startIndex) - '0';
ret = ret * 10 + digitNum;
}
if(isNeg)
ret = -ret;
if(ret < Integer.MIN_VALUE)
return Integer.MIN_VALUE;
if(ret > Integer.MAX_VALUE)
return Integer.MAX_VALUE;
return ret;
}
}
本文内容由网友自发贡献,版权归原作者所有,本站不承担相应法律责任。如您发现有涉嫌抄袭侵权的内容,请联系:hwhale#tublm.com(使用前将#替换为@)