QML项目开发过程中,有时候需要对控件大小和位置‘进行人为调整,因此设计该组件。该组件鼠标置于边框和顶点位置时鼠标样式对应改变,拖动边框可修改该方向组件大小,拖动顶点可修改组件处横纵向组件大小。对控件拖拽效果如图:
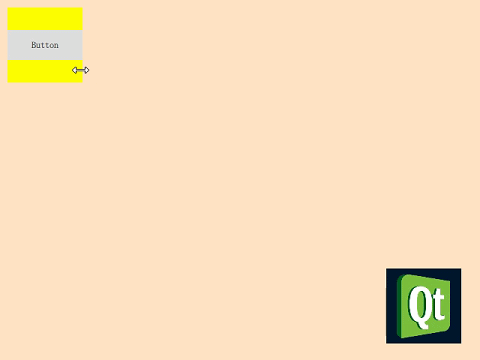
新增了对拖拽对象为主窗口的支持,效果图:
属性:
property var resizeTarget: 需要控制调整的目标组件(传入对象ID)
property int minimumWidth: 组件可调整到的最小宽度
property int minimumHeight: 组件可调整到的最小高度
property int mouseRegion: 接收鼠标调整区域范围
使用时,将该组件文件ResizableRectangle.qml引入项目中,设置resizeTarget为需要控制的组件即可,如:
import QtQuick 2.1
import QtQuick.Window 2.1
import QtQuick.Controls 2.1
Window {
width: 640
height: 480
visible: true
title: qsTr("Resizable Widget")
Rectangle {
id: rectangle
x: 10
y: 10
width: 100
height: 100
color: "yellow"
Button {
text: qsTr("Button")
anchors.verticalCenter: parent.verticalCenter
anchors.horizontalCenter: parent.horizontalCenter
onClicked: {
text = "Yellow Clicked!"
}
}
ResizableRectangle{
resizeTarget: rectangle//设置调整目标ID
}
}
}
当拖拽对象为系统主窗体时:
import QtQuick 2.15
import QtQuick.Controls 2.15
ApplicationWindow {
id:appWindow
width: 640
height: 480
visible: true
title: qsTr("GIS")
visibility:"Maximized"
flags:Qt.FramelessWindowHint
ResizableRectangle{
anchors.fill: parent
resizeTarget: appWindow
}
}
Demo示例Github:
https://github.com/zjgo007/QmlDemo/tree/master/ResizableRectangle
https://github.com/zjgo007/QmlDemo/tree/master/ResizableRectangleDemo示例CSDN:
https://download.csdn.net/download/zjgo007/84806016
https://download.csdn.net/download/zjgo007/84806016
ResizableRectangle.qml完整代码:
import QtQuick 2.0
import QtQuick.Window 2.15
Item {
id:rect
property int minimumWidth: 50
property int minimumHeight: 50
property int mouseRegion: 5
property var resizeTarget: rect
anchors.fill: resizeTarget
MouseArea {
id:leftX
width: mouseRegion
anchors.left: parent.left
anchors.top: parent.top
anchors.bottom: parent.bottom
anchors.leftMargin: 0
anchors.bottomMargin: 0
anchors.topMargin: 0
cursorShape: Qt.SizeHorCursor
property int xPosition: 0
onPressed: {
xPosition = mouse.x
}
onPositionChanged: {
var xOffset = mouse.x-xPosition
if(rect.resizeTarget.x+xOffset>0 && rect.resizeTarget.width-xOffset>minimumWidth){
rect.resizeTarget.x = rect.resizeTarget.x+xOffset
rect.resizeTarget.width = rect.resizeTarget.width-xOffset
}
}
}
MouseArea{
id:rightX
width: mouseRegion
anchors.right: parent.right
anchors.top: parent.top
anchors.bottom: parent.bottom
anchors.bottomMargin: 0
anchors.topMargin: 0
anchors.rightMargin: 0
cursorShape: Qt.SizeHorCursor
property int xPosition: 0
onPressed: {
xPosition = mouse.x
}
onPositionChanged: {
var xOffset = mouse.x-xPosition
var xWidth = rect.resizeTarget.width+xOffset
var availableWidth = rect.resizeTarget.parent ? rect.resizeTarget.parent.width:Screen.desktopAvailableWidth
if(xWidth+rect.resizeTarget.x<availableWidth && xWidth>minimumWidth){
rect.resizeTarget.width = xWidth
}
}
}
MouseArea{
id:topY
height: mouseRegion
anchors.left: parent.left
anchors.right: parent.right
anchors.top: parent.top
anchors.rightMargin: 0
anchors.leftMargin: 0
anchors.topMargin: 0
cursorShape: Qt.SizeVerCursor
property int yPosition: 0
onPressed: {
yPosition = mouse.y
}
onPositionChanged: {
var yOffset = mouse.y-yPosition
if(rect.resizeTarget.y+yOffset>0 && rect.resizeTarget.height-yOffset>minimumHeight){
rect.resizeTarget.y = rect.resizeTarget.y+yOffset
rect.resizeTarget.height = rect.resizeTarget.height-yOffset
}
}
}
MouseArea{
id:bottomY
height: mouseRegion
anchors.left: parent.left
anchors.right: parent.right
anchors.bottom: parent.bottom
anchors.rightMargin: 0
anchors.leftMargin: 0
anchors.bottomMargin: 0
cursorShape: Qt.SizeVerCursor
property int yPosition: 0
onPressed: {
yPosition = mouse.y
}
onPositionChanged: {
var yOffset = mouse.y-yPosition
var yHeight = rect.resizeTarget.height+yOffset
var availableHeight = rect.resizeTarget.parent ? rect.resizeTarget.parent.height : Screen.desktopAvailableHeight
if(yHeight+rect.resizeTarget.y<availableHeight && yHeight>minimumHeight){
rect.resizeTarget.height = yHeight
}
}
}
MouseArea{
width: mouseRegion
height: mouseRegion
anchors.left: parent.left
anchors.top: parent.top
anchors.topMargin: 0
anchors.leftMargin: 0
cursorShape: Qt.SizeFDiagCursor
property int xPosition: 0
property int yPosition: 0
onPressed: {
xPosition = mouse.x
yPosition = mouse.y
}
onPositionChanged: {
var xOffset = mouse.x-xPosition
if(rect.resizeTarget.x+xOffset>0 && rect.resizeTarget.width-xOffset>minimumWidth){
rect.resizeTarget.x = rect.resizeTarget.x+xOffset
rect.resizeTarget.width = rect.resizeTarget.width-xOffset
}
var yOffset = mouse.y-yPosition
if(rect.resizeTarget.y+yOffset>0 && rect.resizeTarget.height-yOffset>minimumHeight){
rect.resizeTarget.y = rect.resizeTarget.y+yOffset
rect.resizeTarget.height = rect.resizeTarget.height-yOffset
}
}
}
MouseArea{
width: mouseRegion
height: mouseRegion
anchors.right: parent.right
anchors.top: parent.top
anchors.topMargin: 0
anchors.rightMargin: 0
cursorShape: Qt.SizeBDiagCursor
property int xPosition: 0
property int yPosition: 0
onPressed: {
xPosition = mouse.x
yPosition = mouse.y
}
onPositionChanged: {
var xOffset = mouse.x-xPosition
var xWidth = rect.resizeTarget.width+xOffset
var availableWidth = rect.resizeTarget.parent ? rect.resizeTarget.parent.width:Screen.desktopAvailableWidth
var availableHeight = rect.resizeTarget.parent ? rect.resizeTarget.parent.height : Screen.desktopAvailableHeight
if(xWidth+rect.resizeTarget.x<availableWidth && xWidth>minimumWidth){
rect.resizeTarget.width = xWidth
}
var yOffset = mouse.y-yPosition
if(rect.resizeTarget.y+yOffset>0 && rect.resizeTarget.height-yOffset>minimumHeight){
rect.resizeTarget.y = rect.resizeTarget.y+yOffset
rect.resizeTarget.height = rect.resizeTarget.height-yOffset
}
}
}
MouseArea{
width: mouseRegion
height: mouseRegion
anchors.left: parent.left
anchors.bottom: parent.bottom
anchors.leftMargin: 0
anchors.bottomMargin: 0
cursorShape: Qt.SizeBDiagCursor
property int xPosition: 0
property int yPosition: 0
onPressed: {
xPosition = mouse.x
yPosition = mouse.y
}
onPositionChanged: {
var xOffset = mouse.x-xPosition
var availableWidth = rect.resizeTarget.parent ? rect.resizeTarget.parent.width:Screen.desktopAvailableWidth
var availableHeight = rect.resizeTarget.parent ? rect.resizeTarget.parent.height : Screen.desktopAvailableHeight
if(rect.resizeTarget.x+xOffset>0 && availableWidth-xOffset>minimumWidth){
rect.resizeTarget.x = rect.resizeTarget.x+xOffset
rect.resizeTarget.width = rect.resizeTarget.width-xOffset
}
var yOffset = mouse.y-yPosition
var yHeight = rect.resizeTarget.height+yOffset
if(yHeight+rect.resizeTarget.y<availableHeight && yHeight>minimumHeight){
rect.resizeTarget.height = yHeight
}
}
}
MouseArea{
width: mouseRegion
height: mouseRegion
anchors.right: parent.right
anchors.bottom: parent.bottom
anchors.rightMargin: 0
anchors.bottomMargin: 0
cursorShape: Qt.SizeFDiagCursor
property int xPosition: 0
property int yPosition: 0
onPressed: {
xPosition = mouse.x
yPosition = mouse.y
}
onPositionChanged: {
var xOffset = mouse.x-xPosition
var xWidth = rect.resizeTarget.width+xOffset
var availableWidth = rect.resizeTarget.parent ? rect.resizeTarget.parent.width:Screen.desktopAvailableWidth
var availableHeight = rect.resizeTarget.parent ? rect.resizeTarget.parent.height : Screen.desktopAvailableHeight
if(xWidth+rect.resizeTarget.x<availableWidth && xWidth>minimumWidth){
rect.resizeTarget.width = xWidth
}
var yOffset = mouse.y-yPosition
var yHeight = rect.resizeTarget.height+yOffset
if(yHeight+rect.resizeTarget.y<availableHeight && yHeight>minimumHeight){
rect.resizeTarget.height = yHeight
}
}
}
}
本文内容由网友自发贡献,版权归原作者所有,本站不承担相应法律责任。如您发现有涉嫌抄袭侵权的内容,请联系:hwhale#tublm.com(使用前将#替换为@)