模型中,最为关键的步骤是,在生产者回调函数中,未生产之前,消费者回调函数是阻塞的,阻塞方式是条件变量。
那么不使用条件变量,如何使用“信号量”实现阻塞呢?
答案是因为调用 sem_wait,当 sem == 0 时候,该线程就会阻塞。因此:生产者对应一个信号量 :sem_t produce;消费者对应一个信号量 :sem_t customer。
sem_init(&produce, 0, 2);
sem_init(&customer, 0, 0); 消费者 value = 0,表示被阻塞
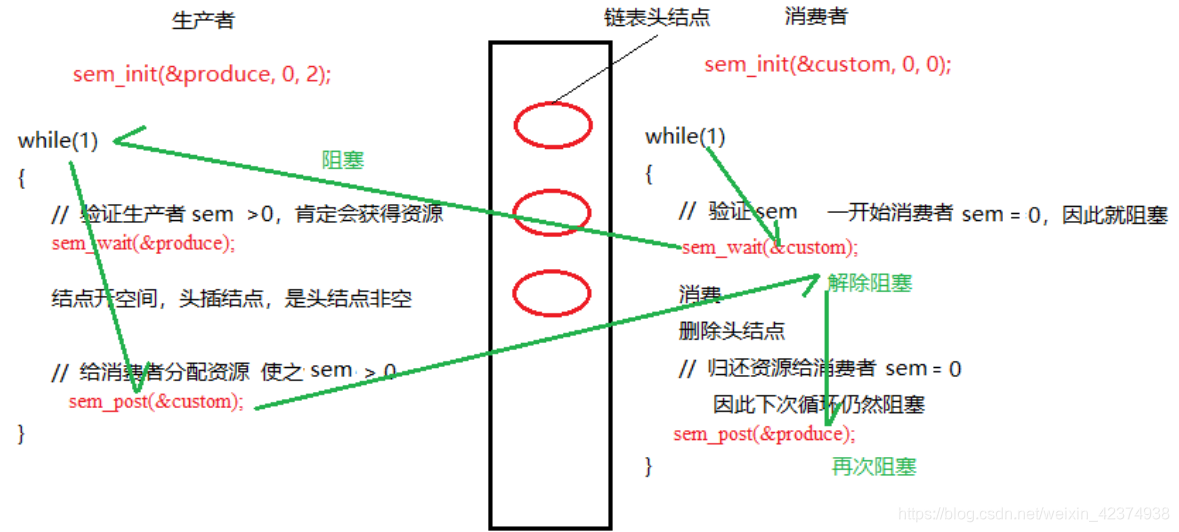
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <pthread.h>
#include <semaphore.h>
#include <time.h>
sem_t produce;
sem_t custom;
typedef struct node
{
int data;
struct node* next;
}Node;
Node* phead = NULL;
void* produce_fun()
{
while(1)
{
sem_wait(&produce);
Node* node = (Node*)malloc(sizeof(Node));
node->data = rand() % 1000;
node->next = phead;
phead = node;
printf("生产者:%lu, 产品:%d\n", pthread_self(), node->data);
sem_post(&custom);
sleep(1);
}
return NULL; }
void* custom_fun()
{
while(1)
{
sem_wait(&custom);
Node* del = phead;
phead = phead->next;
printf("消费者: %lu, 消费: %d\n", pthread_self(), del->data);
free(del);
sem_post(&produce);
sleep(1);
}
return NULL; }
int main()
{
srand(time(NULL));
sem_init(&produce, 0, 2);
sem_init(&custom, 0, 0);
pthread_t p1, p2;
pthread_create(&p1, NULL, produce_fun, NULL);
pthread_create(&p2, NULL, custom_fun, NULL);
pthread_join(p1, NULL);
pthread_join(p2, NULL);
sem_destroy(&produce);
sem_destroy(&custom);
return 0;
}
本文内容由网友自发贡献,版权归原作者所有,本站不承担相应法律责任。如您发现有涉嫌抄袭侵权的内容,请联系:hwhale#tublm.com(使用前将#替换为@)