首先创建Controller和resource
php artisan make:controller ProductsController -r
php artisan make:resource ProductResource
将ProductsController.php改为:
<?php
namespace App\Http\Controllers;
use App\Http\Resources\ProductResource;
use App\Models\Products;
use Illuminate\Http\Request;
class ProductsController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
return Products::all();
}
/**
* Show the form for creating a new resource.
*
* @return \Illuminate\Http\Response
*/
public function create()
{
//
}
/**
* Store a newly created resource in storage.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(Request $request)
{
$productName = $request->input('name');
$productPrice = $request->input('price');
$productDescription = $request->input('description');
$product = Products::create([
'name' => $productName,
'price' => $productPrice,
'description' => $productDescription,
]);
return response()->json([
'data' => new ProductResource($product)
], 201);
}
/**
* Display the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function show(Products $product)
{
return new ProductResource($product);
}
/**
* Show the form for editing the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function edit($id)
{
//
}
/**
* Update the specified resource in storage.
*
* @param \Illuminate\Http\Request $request
* @param int $id
* @return \Illuminate\Http\Response
*/
public function update(Request $request, Products $product)
{
$productName = $request->input('name');
$productPrice = $request->input('price');
$productDescription = $request->input('description');
$product->update([
'name' => $productName,
'price' => $productPrice,
'description' => $productDescription
]);
return response()->json([
'data' => new ProductResource($product)
], 200);
}
/**
* Remove the specified resource from storage.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function destroy(Products &$product)
{
$product->delete();
return response()->json(null, 204);
}
}
PrductResource.php
<?php
namespace App\Http\Resources;
use Illuminate\Http\Resources\Json\JsonResource;
class ProductResource extends JsonResource
{
/**
* Transform the resource into an array.
*
* @param \Illuminate\Http\Request $request
* @return array|\Illuminate\Contracts\Support\Arrayable|\JsonSerializable
*/
public function toArray($request)
{
return [
'id' => $this->id,
'productName' => $this->name,
'discountedPrice' => "$" . ($this->price * 0.8),
'discount' => "$" . ($this->price * 0.2),
'productDescription' => $this->description,
];
}
}
这里需要在routes/api.php中添加代码,不用在web.php中添加
<?php
use App\Http\Controllers\ProductsController;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Route;
/*
|--------------------------------------------------------------------------
| API Routes
|--------------------------------------------------------------------------
|
| Here is where you can register API routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| is assigned the "api" middleware group. Enjoy building your API!
|
*/
Route::middleware('auth:sanctum')->get('/user', function (Request $request) {
return $request->user();
});
Route::get('products', [ProductsController::class, 'index'])->name('products.index');
Route::get('products/{product}', [ProductsController::class, 'show'])->name('products.show');
Route::post('products', [ProductsController::class, 'store'])->name('products.store');
Route::put('products/{product}', [ProductsController::class, 'update'])->name('products.update');
Route::delete('products/{product}', [ProductsController::class, 'destroy'])->name('products.destroy');
运行截图如下:
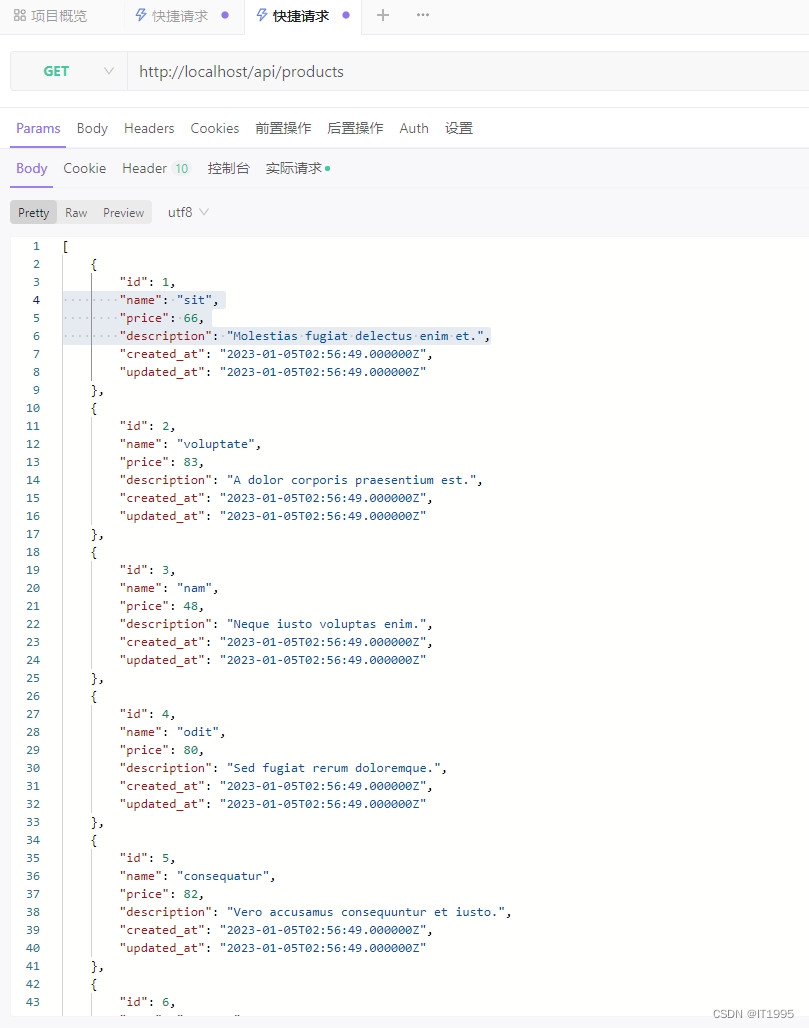
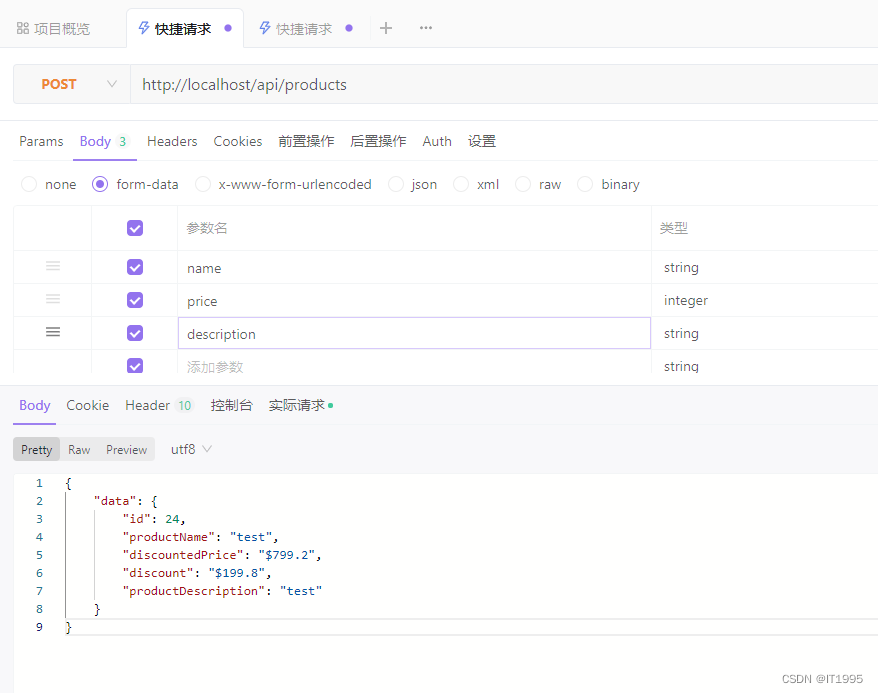
本文内容由网友自发贡献,版权归原作者所有,本站不承担相应法律责任。如您发现有涉嫌抄袭侵权的内容,请联系:hwhale#tublm.com(使用前将#替换为@)