webservice的 发布一般都是使用WSDL(web service descriptive language)文件的样式来发布的,在WSDL文件里面,包含这个webservice暴露在外面可供使用的接口。今天搜索到了非常好的 webservice provider列表
http://www.webservicex.net/WCF/default.aspx
这上面列出了70多个包括很多方面的free webservice provider,utilities->global weather就可以获取全球的天气预报。
下面我们来看Java如何通过WSDL文件来调用这些web service:
注意,以下的代码并没有经过真正的测试,只是说明这些情况,不同版本的Axis相差很大,大家最好以apache网站上的例子为准,这里仅仅用于说明其基本用法。
1,直接AXIS调用远程的web service
我觉得这种方法比较适合那些高手,他们能直接看懂XML格式的WSDL文件,我自己是看不懂的,尤其我不是专门搞这行的,即使一段时间看懂,后来也就忘记了。直接调用模式如下:
import java.util.Date;
import java.text.DateFormat;
import org.apache.axis.client.Call;
import org.apache.axis.client.Service;
import javax.xml.namespace.QName;
import java.lang.Integer;
import javax.xml.rpc.ParameterMode;
public class caClient {
public static void main(String[] args) {
try {
String endpoint = "http://localhost:8080/ca3/services/caSynrochnized?wsdl";
// 直接引用远程的wsdl文件
// 以下都是套路
Service service = new Service();
Call call = (Call) service.createCall();
call.setTargetEndpointAddress(endpoint);
call.setOperationName("addUser");// WSDL里面描述的接口名称
call.addParameter("userName",
org.apache.axis.encoding.XMLType.XSD_DATE,
javax.xml.rpc.ParameterMode.IN);// 接口的参数
call.setReturnType(org.apache.axis.encoding.XMLType.XSD_STRING);// 设置返回类型
String temp = "测试人员";
String result = (String) call.invoke(new Object[] { temp });
// 给方法传递参数,并且调用方法
System.out.println("result is " + result);
} catch (Exception e) {
System.err.println(e.toString());
}
}
}
2,直接SOAP调用远程的webservice
这种模式我从来没有见过,也没有试过,但是网络上有人贴出来,我也转过来
import org.apache.soap.util.xml.*;
import org.apache.soap.*;
import org.apache.soap.rpc.*;
import java.io.*;
import java.net.*;
import java.util.Vector;
public class caService {
public static String getService(String user) {
URL url = null;
try {
url = new URL(
"http://192.168.0.100:8080/ca3/services/caSynrochnized");
} catch (MalformedURLException mue) {
return mue.getMessage();
}
// This is the main SOAP object
Call soapCall = new Call();
// Use SOAP encoding
soapCall.setEncodingStyleURI(Constants.NS_URI_SOAP_ENC);
// This is the remote object we're asking for the price
soapCall.setTargetObjectURI("urn:xmethods-caSynrochnized");
// This is the name of the method on the above object
soapCall.setMethodName("getUser");
// We need to send the ISBN number as an input parameter to the method
Vector soapParams = new Vector();
// name, type, value, encoding style
Parameter isbnParam = new Parameter("userName", String.class, user,
null);
soapParams.addElement(isbnParam);
soapCall.setParams(soapParams);
try {
// Invoke the remote method on the object
Response soapResponse = soapCall.invoke(url, "");
// Check to see if there is an error, return "N/A"
if (soapResponse.generatedFault()) {
Fault fault = soapResponse.getFault();
String f = fault.getFaultString();
return f;
} else {
// read result
Parameter soapResult = soapResponse.getReturnValue();
// get a string from the result
return soapResult.getValue().toString();
}
} catch (SOAPException se) {
return se.getMessage();
}
}
}
3,使用wsdl2java把WSDL文件转成本地类,然后像本地类一样使用,即可。
这是像我这种懒人最喜欢的方式,仍然以前面的global weather report为例。
首先 java org.apache.axis.wsdl.WSDL2Java http://www.webservicex.net/globalweather.asmx.WSDL
原本的网址是http://www.webservicex.net/globalweather.asmx?WSDL,中间个各问号,但是Linux下面它不能解析,所以去掉问号,改为点号。
那么就会出现4个文件:
GlobalWeather.java
GlobalWeatherLocator.java
GlobalWeatherSoap.java
GlobalWeatherSoapStub.java
其中GlobalWeatherSoap.java是我们最为关心的接口文件,如果你对RMI等SOAP实现的具体细节不感兴趣,那么你只需要看接口文件即可,
在使用的时候,引入这个接口即可,就好像使用本地类一样。
一:webService介绍
1.什么是webService
webService是一种使用http传输SOAP协议数据的远程调用技术
2.webService三要素
SOAP:规范XML标签
WSDL:服务端的使用说明书
UDDI:目录
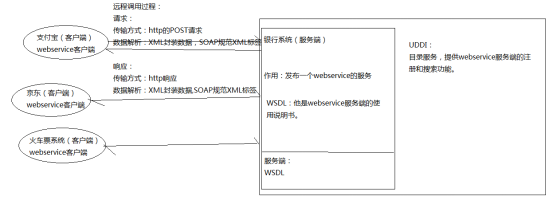
二:webService入门小程序
1.服务端
(1)、开发步骤
A、创建接口
package com.webservice.jaxws;
public interface WeatherService {
//查询天气的方法
public String queryWeather(String cityName);
}
B、创建实现类,在实现类上加入@WebService注解,该注解的作用是标识该实现类是webservice的服务类,发布该实现类中的public方法
package com.webservice.jaxws;
import javax.jws.WebService;
/**
* 天气查询的实现类
* @author Administrator
*
*/
@WebService
public class WeatherServiceImpl implements WeatherService {
//查询天气
public String queryWeather(String cityName) {
System.out.println(cityName + "天气是:晴天");
return "晴";
}
}
C、发布服务,使用EndPoint类中的publish()方法发布,参数分别为服务访问的地址和服务的实现类
package com.webservice.jaxws;
import javax.jws.WebService;
/**
* 天气查询的实现类
* @author Administrator
*
*/
@WebService
public class WeatherServiceImpl implements WeatherService {
//查询天气
public String queryWeather(String cityName) {
System.out.println(cityName + "天气是:晴天");
return "晴";
}
}
D、测试服务是否发布成功,阅读使用说明书,确认要调用的类、方法、参数等
● WSDL访问地址:
http://localhost:12345/weather?wsdl
● WSDL说明书阅读方式:从下往上阅读
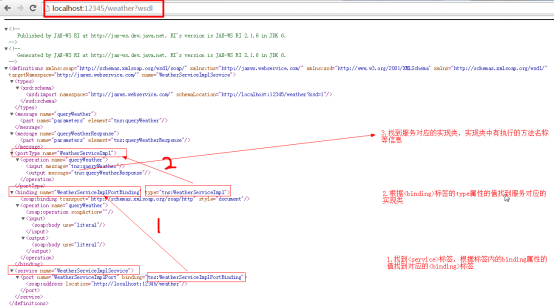
E、如何发布SOAP1.2版本的服务端
● 引入第三方jar包
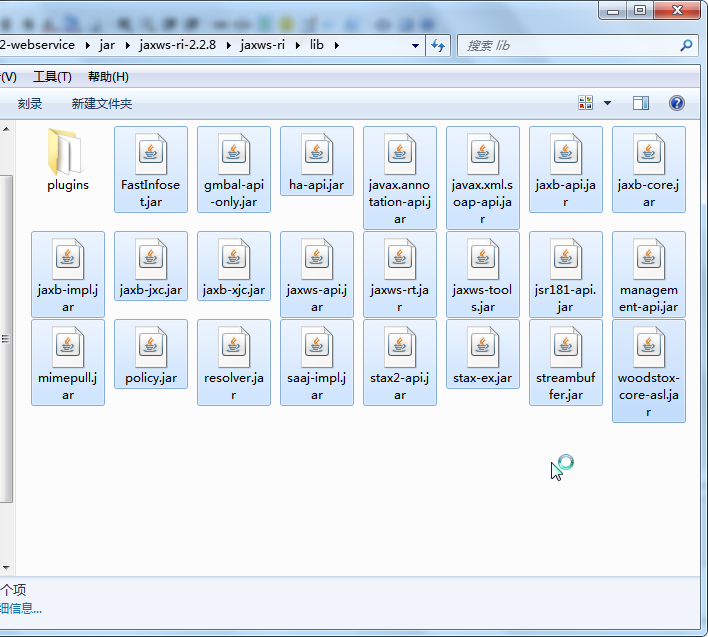
● 在服务实现类上加入注解 @BindingType(SOAPBinding.SOAP12HTTP_BINDING)
package com.webservice.jaxws;
import javax.jws.WebService;
import javax.xml.ws.BindingType;
import javax.xml.ws.soap.SOAPBinding;
/**
* 天气查询的实现类
* @author Administrator
*
*/
@WebService
@BindingType(SOAPBinding.SOAP12HTTP_BINDING)
public class WeatherServiceImpl implements WeatherService {
//查询天气
public String queryWeather(String cityName) {
System.out.println(cityName + "天气是:晴天");
return "晴";
}
}
2.客户端
(1)、开发步骤
A、在工作空间创建用于存放使用wsimport命令生成的客户端代码的java工程
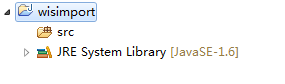
B、使用jdk提供的wsimport命令生成客户端代码
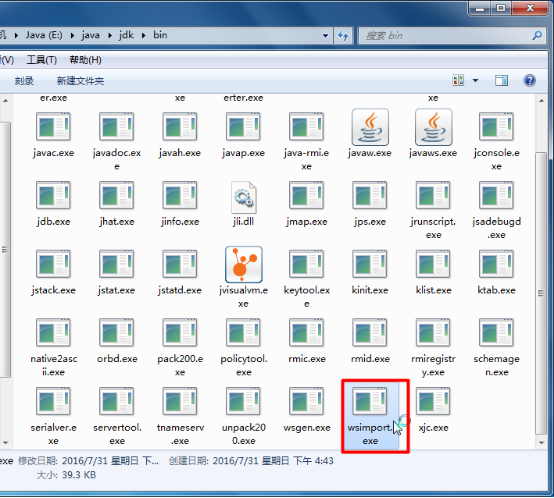
● wsimport命令是jdk提供的,作用是根据使用说明书生成客户端代码,wsimport只支持SOAP1.1客户端的生成
● wsimport常用参数
-d:默认参数,用于生成.class文件
-s:生成.java文件
-p:指定生成java文件的包名,不指定则为WSDL说明书中namespace值得倒写

C、在doc窗口进入java工程项目的src目录,执行wsimport命令
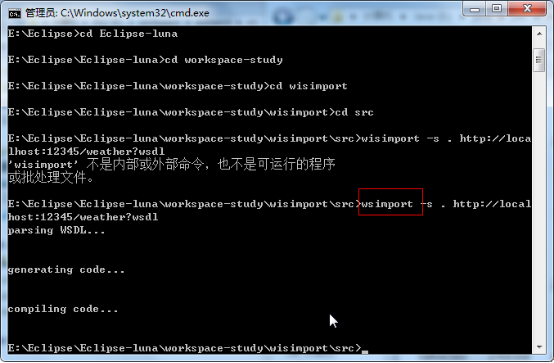
D、在Eclipse中刷新java项目,将生成的客户端代码copy到客户端工程中
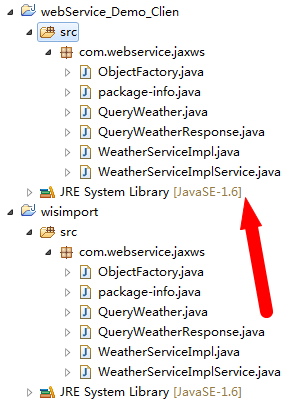
E、创建服务视图,类名从<service>标签的name属性获取

F、获取服务实现类,视图实例调用getProt()方法,实现类的类名从portType的name属性获取
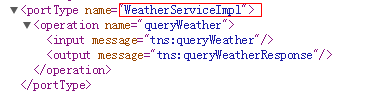
G、调用查询方法,方法名从portType下的operation标签的name属性获取
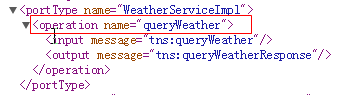
package com.webservice.client;
import com.webservice.jaxws.WeatherServiceImpl;
import com.webservice.jaxws.WeatherServiceImplService;
public class Client {
public static void main(String[] args) {
//创建视图
WeatherServiceImplService wsis = new WeatherServiceImplService();
//获取服务实现类
WeatherServiceImpl wsi = wsis.getPort(WeatherServiceImpl.class);
//调用查询方法
String weather = wsi.queryWeather("北京");
System.out.println(weather);
}
}
三:webService三要素详解
1.WSDL
(1)、定义
WSDL即web服务描述语言,它是服务端的使用说明书,是XML格式的文档,说明服务地址、服务类、方法、参数和返回值,是伴随服务发布成功,自动生成的
(2)、文档结构
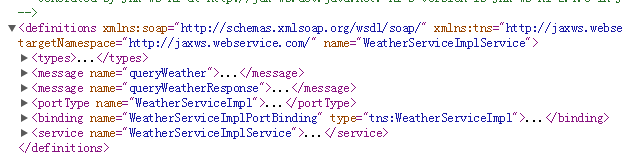
● <service> 服务视图,webservice的服务结点,它包括了服务端点
● <binding> 为每个服务端点定义消息格式和协议细节
● <portType> 服务端点,描述 web service可被执行的操作方法,以及相关的消息,通过binding指向portType
● <message> 定义一个操作(方法)的数据参数(可有多个参数)
● <types> 定义 web service 使用的全部数据类型
2.SOAP
(1)、定义
SOAP即简单对象访问协议(Simple Object Access Protocol),使用http发送XML格式的数据,他不是webservice的专有协议
(2)、结构 SOAP = HTTP + XML
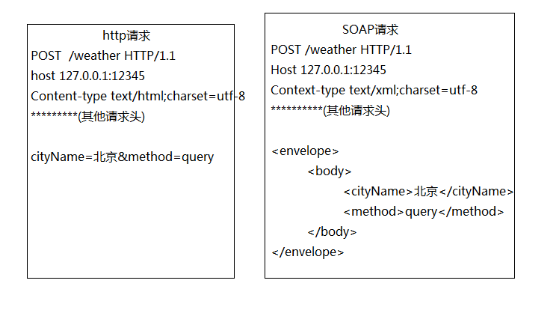
(3)、协议的格式
Envelope:必须有,此元素将整个 XML 文档标识为一条SOAP消息
Header:可选元素,包含头部信息
Body:必须有,包含所有调用和响应信息
Fault:可选元素,提供有关在处理此消息时所发生的错误信息
(4)、版本
A、SOAP1.1
● 请求
POST /weather HTTP/1.1
Accept: text/xml, multipart/related
Content-Type: text/xml; charset=utf-8
SOAPAction: "http://jaxws.ws.itcast.cn/WeatherInterfaceImpl/queryWeatherRequest"
User-Agent: JAX-WS RI 2.2.4-b01
Host: 127.0.0.1:54321
Connection: keep-alive
Content-Length: 211
<?xml version="1.0" ?>
<S:Envelope xmlns:S="http://schemas.xmlsoap.org/soap/envelope/">
<S:Body><ns2:queryWeather xmlns:ns2="http://jaxws.ws.itcast.cn/"><arg0>北京</arg0></ns2:queryWeather>
</S:Body>
</S:Envelope>
● 响应
HTTP/1.1 200 OK
Transfer-encoding: chunked
Content-type: text/xml; charset=utf-8
Date: Fri, 04 Dec 2015 03:45:56 GMT
<?xml version="1.0" ?>
<S:Envelope xmlns:S="http://schemas.xmlsoap.org/soap/envelope/">
<S:Body>
<ns2:queryWeatherResponse xmlns:ns2="http://jaxws.ws.itcast.cn/"><return>晴</return></ns2:queryWeatherResponse>
</S:Body>
</S:Envelope>
B、SOAP1.2
● 请求
POST /weather HTTP/1.1
Accept: application/soap+xml, multipart/related
Content-Type: application/soap+xml; charset=utf-8;
action="http://jaxws.ws.itcast.cn/WeatherInterfaceImpl/queryWeatherRequest"
User-Agent: JAX-WS RI 2.2.4-b01
Host: 127.0.0.1:54321
Connection: keep-alive
Content-Length: 209
<?xml version="1.0" ?>
<S:Envelope xmlns:S="http://www.w3.org/2003/05/soap-envelope">
<S:Body><ns2:queryWeather xmlns:ns2="http://jaxws.ws.itcast.cn/"><arg0>北京</arg0></ns2:queryWeather>
</S:Body>
</S:Envelope>
● 响应
HTTP/1.1 200 OK
Transfer-encoding: chunked
Content-type: application/soap+xml; charset=utf-8
Date: Fri, 04 Dec 2015 03:55:49 GMT
<?xml version='1.0' encoding='UTF-8'?>
<S:Envelope xmlns:S="http://www.w3.org/2003/05/soap-envelope">
<S:Body>
<ns2:queryWeatherResponse xmlns:ns2="http://jaxws.ws.itcast.cn/"><return>晴</return></ns2:queryWeatherResponse>
</S:Body>
</S:Envelope>
C、SOAP1.1 和 SOAP1.2的区别
● 相同点
请求方式都是POST
协议格式都一样,都有envelope和body
● 不同点
①、数据格式不同
SOAP1.1:text/xml;charset=utf-8
SOAP1.2:application/soap+xml;charset=utf-8
②、命名空间不同
四:webservice客户端的四种调用方式
1.生成客户端调用方式
(1)、开发步骤
A、wisimport生成客户端代码
B、创建服务视图
C、获取实现类
D、调用查询方法
2.service编程实现调用
(1)、开发步骤
A、wisimport生成客户端代码
B、使用serivce类创建服务视图
C、获取服务实现类
D、调用查询方法
import java.io.IOException;
import java.net.MalformedURLException;
import java.net.URL;
import javax.xml.namespace.QName;
import javax.xml.ws.Service;
import cn.itcast.mobile.MobileCodeWSSoap;
/**
*
* <p>Title: ServiceClient.java</p>
* <p>Description:Service编程实现客户端</p>
*/
public class ServiceClient {
public static void main(String[] args) throws IOException {
//创建WSDL地址,不是服务地址
URL url = new URL("http://webservice.webxml.com.cn/WebServices/MobileCodeWS.asmx?wsdl");
//创建服务名称
//1.namespaceURI - 命名空间地址
//2.localPart - 服务名称
QName qname = new QName("http://WebXml.com.cn/", "MobileCodeWS");
//Service创建视图
//参数:
//1.wsdlDocumentLocation - 使用说明书地址
//2.serviceName - 服务名称
Service service = Service.create(url, qname);
//获取实现类
MobileCodeWSSoap mobileCodeWSSoap = service.getPort(MobileCodeWSSoap.class);
//调用查询方法
String result = mobileCodeWSSoap.getMobileCodeInfo("188888888", "");
System.out.println(result);
}
}
特点:方便管理,是一个标准的开发方式
3.HttpURLConnection调用方式
(1)、开发步骤
A、创建服务地址
B、打开服务地址的一个连接
C、设置连接参数
● 注意
a、POST必须大写,如果小写会出如下异常:

b、如果不设置输入输出,会报如下异常:

D、组织SOAP协议数据,发送给服务器
E、接收服务端的响应
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
/**
*
* <p>Title: HttpClient.java</p>
* <p>Description:HttpURLConnection调用方式</p>
*/
public class HttpClient {
public static void main(String[] args) throws IOException {
//1:创建服务地址
URL url = new URL("http://webservice.webxml.com.cn/WebServices/MobileCodeWS.asmx");
//2:打开到服务地址的一个连接
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
//3:设置连接参数
//3.1设置发送方式:POST必须大写
connection.setRequestMethod("POST");
//3.2设置数据格式:Content-type
connection.setRequestProperty("content-type", "text/xml;charset=utf-8");
//3.3设置输入输出,新创建的connection默认是没有读写权限的,
connection.setDoInput(true);
connection.setDoOutput(true);
//4:组织SOAP协议数据,发送给服务端
String soapXML = getXML("1866666666");
OutputStream os = connection.getOutputStream();
os.write(soapXML.getBytes());
//5:接收服务端的响应
int responseCode = connection.getResponseCode();
if(200 == responseCode){//表示服务端响应成功
InputStream is = connection.getInputStream();
InputStreamReader isr = new InputStreamReader(is);
BufferedReader br = new BufferedReader(isr);
StringBuilder sb = new StringBuilder();
String temp = null;
while(null != (temp = br.readLine())){
sb.append(temp);
}
System.out.println(sb.toString());
is.close();
isr.close();
br.close();
}
os.close();
}
/**
* <?xml version="1.0" encoding="utf-8"?>
<soap:Envelope xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="http://www.w3.org/2001/XMLSchema" xmlns:soap="http://schemas.xmlsoap.org/soap/envelope/">
<soap:Body>
<getMobileCodeInfo xmlns="http://WebXml.com.cn/">
<mobileCode>string</mobileCode>
<userID>string</userID>
</getMobileCodeInfo>
</soap:Body>
</soap:Envelope>
* @param phoneNum
* @return
*/
public static String getXML(String phoneNum){
String soapXML = "<?xml version=\"1.0\" encoding=\"utf-8\"?>"
+"<soap:Envelope xmlns:xsi=\"http://www.w3.org/2001/XMLSchema-instance\" xmlns:xsd=\"http://www.w3.org/2001/XMLSchema\" xmlns:soap=\"http://schemas.xmlsoap.org/soap/envelope/\">"
+"<soap:Body>"
+"<getMobileCodeInfo xmlns=\"http://WebXml.com.cn/\">"
+"<mobileCode>"+phoneNum+"</mobileCode>"
+"<userID></userID>"
+"</getMobileCodeInfo>"
+" </soap:Body>"
+"</soap:Envelope>";
return soapXML;
}
}
4.Ajax调用方式
<!doctype html>
<html lang="en">
<head>
<title>Ajax调用方式</title>
<script type="text/javascript">
function queryMobile(){
//创建XMLHttpRequest对象
var xhr = new XMLHttpRequest();
//打开链接
xhr.open("post","http://webservice.webxml.com.cn/WebServices/MobileCodeWS.asmx",true);
//设置content-type
xhr.setRequestHeader("content-type","text/xml;charset=utf-8");
//设置回调函数
xhr.onreadystatechange=function(){
//判断客户端发送成功&&服务端响应成功
if(4 == xhr.readyState && 200 == xhr.status){
alert(xhr.responseText);
}
}
//组织SOAP协议数据
var soapXML = "<?xml version=\"1.0\" encoding=\"utf-8\"?>"
+"<soap:Envelope xmlns:xsi=\"http://www.w3.org/2001/XMLSchema-instance\" xmlns:xsd=\"http://www.w3.org/2001/XMLSchema\" xmlns:soap=\"http://schemas.xmlsoap.org/soap/envelope/\">"
+"<soap:Body>"
+"<getMobileCodeInfo xmlns=\"http://WebXml.com.cn/\">"
+"<mobileCode>"+document.getElementById("phoneNum").value+"</mobileCode>"
+"<userID></userID>"
+"</getMobileCodeInfo>"
+" </soap:Body>"
+"</soap:Envelope>";
alert(soapXML);
//发送请求
xhr.send(soapXML);
}
</script>
</head>
<body>
手机号归属地查询:<input type="text" id="phoneNum" /><input type="button" value="查询" οnclick="javascript:queryMobile();"/>
</body>
</html>
五、深入开发:用注解修改WSDL内容
1.WebService的注解都位于javax.jws包下:
@WebService-定义服务,在public class上边
targetNamespace:指定命名空间
name:portType的名称
portName:port的名称
serviceName:服务名称
endpointInterface:SEI接口地址,如果一个服务类实现了多个接口,只需要发布一个接口的方法,可通过此注解指定要发布服务的接口。
@WebMethod-定义方法,在公开方法上边
operationName:方法名
exclude:设置为true表示此方法不是webservice方法,反之则表示webservice方法
@WebResult-定义返回值,在方法返回值前边
name:返回结果值的名称
@WebParam-定义参数,在方法参数前边
name:指定参数的名称
作用:
通过注解,可以更加形像的描述Web服务。对自动生成的wsdl文档进行修改,为使用者提供一个更加清晰的wsdl文档。
当修改了WebService注解之后,会影响客户端生成的代码。调用的方法名和参数名也发生了变化,必须重新生成客户端代码
示例:
import javax.jws.WebMethod;
import javax.jws.WebParam;
import javax.jws.WebResult;
import javax.jws.WebService;
import javax.xml.ws.BindingType;
import javax.xml.ws.soap.SOAPBinding;
/**
*
* <p>Title: WeatherInterfaceImpl.java</p>
* <p>Description:SEI实现类</p>
*/
@WebService(
targetNamespace="http://service.itcast.cn",
name="WeatherWSSoap",
portName="WeatherWSSoapPort",
serviceName="WeatherWS"
)
@BindingType(SOAPBinding.SOAP12HTTP_BINDING)
public class WeatherInterfaceImpl implements WeatherInterface {
@WebMethod(
operationName="getWeather",
exclude=false
)
@Override
public @WebResult(name="result")String queryWeather(@WebParam(name="cityName")String cityName) {
System.out.println("from client..."+cityName);
String weather = "晴";
return weather;
}
}