转自:https://blog.csdn.net/weistin/article/details/78839804
OCR图像识别技术的JAVA实现
最近有个需求需要用图像识别,学习记录一下。
目前网络上的开源的图像识别技术有很多,例如 OCRE(OCR Easy)、Clara OCR、OCRAD、TESSERACT-OCR 等等,今天本blog将记录下tesseract-ocr的JAVA实现,后面会记录研究下Android的使用:
1、TESSERACT-OCR安装
首先下载EXE安装包进行安装,我安装的版本是“tesseract-ocr-setup-3.05.01.exe”,建议直接安装在默认路径。安装完毕后,目录下:
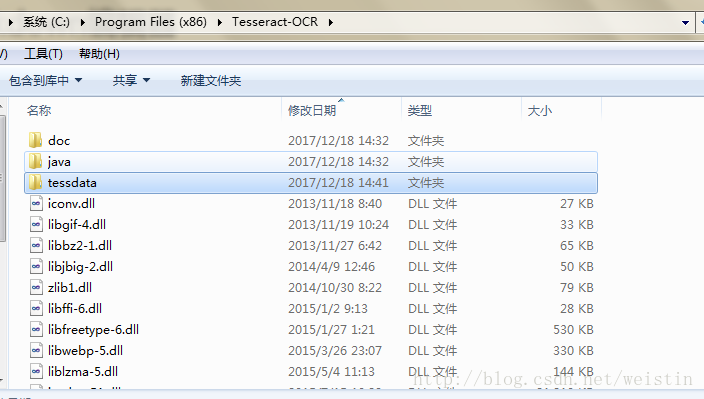
其中,文件夹“tessdata”用于放置语言库。
程序实现
上一步已经安装好了TESSERACT-OCR,下面直接贴代码。
核心代码 OCRHelper.java:
package com.liping.test;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileInputStream;
import java.io.InputStreamReader;
import java.util.ArrayList;
import java.util.List;
/**
* 图文识别帮助类
*
* @author Felix Li
* @create 2017-12-19-9:12
*/
public class OCRHelper {
private final String LANG_OPTION = "-l";
private final String EOL = System.getProperty("line.separator");
/**
* Tesseract-OCR的安装路径
*/
private String tessPath = "C://Program Files (x86)//Tesseract-OCR";
//private String tessPath = new File("tesseract").getAbsolutePath();
/**
* @param imageFile 传入的图像文件
* @param imageFormat 传入的图像格式
* @return 识别后的字符串
*/
public String recognizeText(File imageFile) throws Exception {
/**
* 设置输出文件的保存的文件目录
*/
File outputFile = new File(imageFile.getParentFile(), "output");
StringBuffer strB = new StringBuffer();
List<String> cmd = new ArrayList<String>();
cmd.add(tessPath + "\\tesseract");
cmd.add("");
cmd.add(outputFile.getName());
cmd.add(LANG_OPTION);
cmd.add("chi_sim");
//cmd.add("eng");
ProcessBuilder pb = new ProcessBuilder();
/**
*Sets this process builder's working directory.
*/
pb.directory(imageFile.getParentFile());
cmd.set(1, imageFile.getName());
pb.command(cmd);
pb.redirectErrorStream(true);
long startTime = System.currentTimeMillis();
System.out.println("开始时间:" + startTime);
Process process = pb.start();
// tesseract.exe 1.jpg 1 -l chi_sim
//不习惯使用ProcessBuilder的,也可以使用Runtime,效果一致
// Runtime.getRuntime().exec("tesseract.exe 1.jpg 1 -l chi_sim");
/**
* the exit value of the process. By convention, 0 indicates normal
* termination.
*/
// System.out.println(cmd.toString());
int w = process.waitFor();
if (w == 0)// 0代表正常退出
{
BufferedReader in = new BufferedReader(new InputStreamReader(
new FileInputStream(outputFile.getAbsolutePath() + ".txt"),
"UTF-8"));
String str;
while ((str = in.readLine()) != null) {
strB.append(str).append(EOL);
}
in.close();
long endTime = System.currentTimeMillis();
System.out.println("结束时间:" + endTime);
System.out.println("耗时:" + (endTime - startTime) + "毫秒");
} else {
String msg;
switch (w) {
case 1:
msg = "Errors accessing files. There may be spaces in your image's filename.";
break;
case 29:
msg = "Cannot recognize the image or its selected region.";
break;
case 31:
msg = "Unsupported image format.";
break;
default:
msg = "Errors occurred.";
}
throw new RuntimeException(msg);
}
new File(outputFile.getAbsolutePath() + ".txt").delete();
return strB.toString().replaceAll("\\s*", "");
}
}
测试类Test.java:
package com.liping.test;
import java.io.File;
import java.io.IOException;
/**
* 测试主类
*
* @author Felix Li
* @create 2017-12-19-9:17
*/
public class Test {
public static void main(String[] args) {
try {
//图片文件:此图片是需要被识别的图片
File file = new File("C://Program Files (x86)//Tesseract-OCR//shouye.png");
String recognizeText = new OCRHelper().recognizeText(file);
System.out.print(recognizeText + "\t");
} catch (IOException e) {
e.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
}
}
}
以上就是TESSERACT-OCR的JAVA使用。
注意事项
图片读出来有的字符可能会乱码,这是由于识别语言库的原因,可以训练语言库,来提升识别准确率与识别速度,这个以后再讲。
有问题的欢迎留言讨论!