前言
串口编程分为这么几类:
<1> 普通的TTL:一对一通信
<2> RS232:一对一通信
<3> RS485:一对多通信
通信模式目前最好的是主从方式,即主机向从机发送一段数据,从机接收到主机的数据之后执行相应的动作,把结果返回给主机。
一、包含串口编程必要的头文件?
示例:pandas 是基于NumPy 的一种工具,该工具是为了解决数据分析任务而创建的。
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <termios.h>
#include <errno.h>
#include <getopt.h>
#include <string.h>
二、串口初始化
static int fd;
static char *device="/dev/ttySTM3";
fd = open_port(device);
三、串口写数据
write(fd, xmit, strlen(xmit));
四、串口阻塞读取数据
这边用到了Select系统调用,监测fd是否可读,不可读,系统阻塞,阻塞的时间是timeout结构体决定的,当timeout的时间消耗完,或者串口有数据,select函数执行结束。
注意,这里有一个坑,就是select函数通过指针调用timeout结构体,改版timeout的值,所以每次调用select的时候要重新初始化timeout结构体。
fd_set rset;
int rv = -1 ,i=0;
int nread=0;
struct timeval timeout;
timeout.tv_sec=sec;
timeout.tv_usec=0;
FD_ZERO(&rset);
FD_SET(fd, &rset);
rv = select(fd+1, &rset, NULL, NULL, &timeout);
if(rv < 0)
{
printf("select() failed: %s\n", strerror(errno));
return 0;
}
nread = read(fd, buffer, len);
五、RS485与RS232编程和TTL的区别
可以先看大神的文章,讲的比较好。
链接: https://blog.csdn.net/zhejfl/article/details/78638833.、
我这里总结一下。
RS485与RS232使用的电平与TTL不同,需要电平转换芯片,有一类的需要软件控制读写方向,有二类的读写方向由硬件自动控制,如下面:
第一类:需要芯片端引出一个GPIO控制RS485的RE/DE
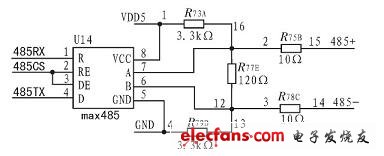
第二类:硬件根据电平变换,自动控制读写方向。
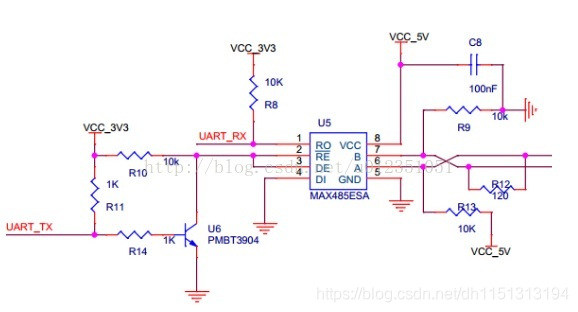
软件上的区别:
RS485,RS232
写数据:
- gpio引脚设置
- 调用write函数
读数据:
- gpio引脚设置
- 调用串口读函数
六、完整代码
完整代码:
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <termios.h>
#include <errno.h>
#include <getopt.h>
#include <string.h>
#include <usart.h>
#define FALSE 1
#define TRUE 0
char *recchr="We received:\"";
void print_usage (FILE *stream, int exit_code);
static int fd;
static char *device="/dev/ttySTM2";
static int speed=2400;
int speed_arr[] = {
B921600, B460800, B230400, B115200, B57600, B38400, B19200,
B9600, B4800, B2400, B1200, B300,
};
int name_arr[] = {
921600, 460800, 230400, 115200, 57600, 38400, 19200,
9600, 4800, 2400, 1200, 300,
};
void set_speed(int fd, int speed)
{
int i;
int status;
struct termios Opt;
tcgetattr(fd, &Opt);
for ( i= 0; i < sizeof(speed_arr) / sizeof(int); i++) {
if (speed == name_arr[i]) {
tcflush(fd, TCIOFLUSH);
cfsetispeed(&Opt, speed_arr[i]);
cfsetospeed(&Opt, speed_arr[i]);
status = tcsetattr(fd, TCSANOW, &Opt);
if (status != 0)
perror("tcsetattr fd1");
return;
}
tcflush(fd,TCIOFLUSH);
}
if (i == 12){
printf("\tSorry, please set the correct baud rate!\n\n");
}
}
/*
*@brief 设置串口数据位,停止位和效验位
*@param fd 类型 int 打开的串口文件句柄*
*@param databits 类型 int 数据位 取值 为 7 或者8*
*@param stopbits 类型 int 停止位 取值为 1 或者2*
*@param parity 类型 int 效验类型 取值为N,E,O,,S
*/
int set_Parity(int fd,int databits,int stopbits,int parity)
{
struct termios options;
if ( tcgetattr( fd,&options) != 0) {
perror("SetupSerial 1");
return(FALSE);
}
options.c_cflag &= ~CSIZE ;
switch (databits) /*设置数据位数*/ {
case 7:
options.c_cflag |= CS7;
break;
case 8:
options.c_cflag |= CS8;
break;
default:
fprintf(stderr,"Unsupported data size\n");
return (FALSE);
}
switch (parity) {
case 'n':
case 'N':
options.c_cflag &= ~PARENB; /* Clear parity enable */
options.c_iflag &= ~INPCK; /* Enable parity checking */
break;
case 'o':
case 'O':
options.c_cflag |= (PARODD | PARENB); /* 设置为奇效验*/
options.c_iflag |= INPCK; /* Disnable parity checking */
break;
case 'e':
case 'E':
options.c_cflag |= PARENB; /* Enable parity */
options.c_cflag &= ~PARODD; /* 转换为偶效验*/
options.c_iflag |= INPCK; /* Disnable parity checking */
break;
case 'S':
case 's': /*as no parity*/
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
break;
default:
fprintf(stderr,"Unsupported parity\n");
return (FALSE);
}
/* 设置停止位*/
switch (stopbits) {
case 1:
options.c_cflag &= ~CSTOPB;
break;
case 2:
options.c_cflag |= CSTOPB;
break;
default:
fprintf(stderr,"Unsupported stop bits\n");
return (FALSE);
}
/* Set input parity option */
if (parity != 'n')
options.c_iflag |= INPCK;
options.c_cc[VTIME] = 150; // 15 seconds
options.c_cc[VMIN] = 0;
options.c_lflag &= ~(ECHO | ICANON);
tcflush(fd,TCIFLUSH); /* Update the options and do it NOW */
if (tcsetattr(fd,TCSANOW,&options) != 0) {
perror("SetupSerial 3");
return (FALSE);
}
return (TRUE);
}
/**
*@breif 打开串口
*/
int OpenDev(char *Dev)
{
int fd = open( Dev, O_RDWR ); //| O_NOCTTY | O_NDELAY
if (-1 == fd) { /*设置数据位数*/
perror("Can't Open Serial Port");
return -1;
} else
return fd;
}
int usartinit(void)
{
fd = OpenDev(device);
if (fd > 0) {
set_speed(fd, speed);
} else {
fprintf(stderr, "Error opening %s: %s\n", device, strerror(errno));
exit(1);
}
if (set_Parity(fd,8,1,'E')== FALSE) {
fprintf(stderr, "Set Parity Error\n");
close(fd);
exit(1);
}
}
unsigned char usartread(int fd,char *buffer,int len,unsigned char sec)
{
fd_set rset;
int rv = -1;
int nread=0;
struct timeval timeout;
timeout.tv_sec=sec;
timeout.tv_usec=0;
FD_ZERO(&rset);
FD_SET(fd, &rset);
rv = select(fd+1, &rset, NULL, NULL, &timeout);
if(rv < 0)
{
printf("select() failed: %s\n", strerror(errno));
return 0;
}
nread = read(fd, buffer, len);
return nread;
}
int usartgetdate(char *CMD,unsigned char CMDlen,char* RES,char RESlen,unsigned char sec)
{
int nread=0;
printf("%s SEND: %s\n",device,CMD);
write(fd, CMD, CMDlen);
nread=usartread(fd,RES, RESlen,sec);
if (nread > 0) {
RES[nread] = '\0';
printf("%s RES[%d]: %s\n", device, nread, RES);
}else{
printf("%s receive timeout\n",device);
}
return nread;
}