原创 visualfc
在LUA中进行GUI程序设计,可以选择的GUI库一般有wxLua和IupLua。wxLua具备典型的面向对象风格,功能相对强大。而IUP的LUA绑定则非常简洁易用。本文主要介绍IupLua。
IUPLUA目前稳定版本为2.7.1,最新版本为3.0beta1。
IUP项目主页为:http://sourceforge.net/projects/iup
在IupLua程序设计中,主要使用表来设计应用程序界面,表的键值则为GUI部件的属性,通过回调函数完成窗口消息的获取。下面给出一个完整的例子。
1
require "iuplua"
2

3
text_location = iup.text{expand="HORIZONTAL", id="text_location"}
4
btn_browse = iup.button{title="Browse", rastersize="x22",id="btn_browse"}
5
dlg = iup.dialog
6
{
7
iup.vbox
8
{
9
iup.label{title="Location:"},
10
iup.hbox
11
{
12
text_location,
13
btn_browse
14
; margin="0x0"
15
},
16
iup.label{title="Text:"},
17
iup.multiline{expand="YES"},
18
}
19
;title="iuplua sample", size="200x100", margin="10x10"
20
}
21

22
function btn_browse:action()
23
local dlg = iup.filedlg{dialogtype="DIR"}
24
dlg:popup()
25
if dlg.status == "0" then
26
text_location.value = dlg.value
27
end
28
end
29

30
dlg:show()
31

32
iup.MainLoop()
33
上面的例子通过hbox和vbox进行界面的自动调整。程序很简单,如下图所示:
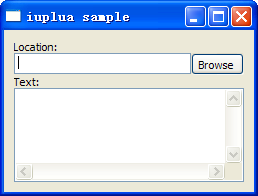
其中22行的fuctnion btn_browse:action即为回调函数,相比wxLua的Bind方法,这种用法更为简洁。
另外我们也可以看到在此程序中我们需要对text_location和btn_browse进行声明,而后在dialog的box中进行引用,我们也可以使用类似HTML控制中的ID值来进行设计而无需事先声明。如下:
1
require "iuplua"
2
require "iupid"
3

4
dlg = iup.dialog
5
{
6
iup.vbox
7
{
8
iup.label{title="Location:", id="ok"},
9
iup.hbox
10
{
11
iup.text{expand="HORIZONTAL", id="text_location"},
12
iup.button{title="Browse", rastersize="x22", id="btn_browse"}
13
; margin="0x0"
14
},
15
iup.label{title="Text:"},
16
iup.multiline{expand="YES"},
17
}
18
;title="iuplua sample", size="200x100", margin="10x10"
19
}
20

21
function btn_browse:action()
22
local dlg = iup.filedlg{dialogtype="DIR"}
23
dlg:popup()
24
if dlg.status == "0" then
25
text_location.value = dlg.value
26
end
27
end
28

29
dlg:show()
30

31
iup.MainLoop()
32
如上所示,使用id值的方式GUI设计代码显得更为一致。那么如何做到这一点呢,在LUA中实现起来很简单,使用upvalue就可以做到。
1
function iup_id(func)
2
return function(o)
3
if o.id ~= nil then
4
_G[o.id] = func(o)
5
return _G[o.id]
6
else
7
return func(o)
8
end
9
end
10
end
然后对所需部件进行声明。
--standard
iup.button = iup_id(iup.button)
iup.text = iup_id(iup.text)

这样就可以使用id的方式来直接引用GUI部件了,另外需要注意的是各个GUI部件要取不同的id值。
下面给出了我写的iupcd.lua的完整源代码以供参考。
require "iuplua"
require "iupluacontrols"


function iup_id(func)
return function(o)
if o.id ~= nil then
_G[o.id] = func(o)
return _G[o.id]
elseif o.ID ~= nil then
_G[o.ID] = func(o)
return _G[o.ID]
else
return func(o)
end
end
end

--standard
iup.button = iup_id(iup.button)
iup.canvas = iup_id(iup.canvas)
iup.frame = iup_id(iup.frame)
iup.multiline = iup_id(iup.multiline)
iup.progressbar = iup_id(iup.progressbar)
iup.spin = iup_id(iup.spin)
iup.tabs = iup_id(iup.tabs)
iup.val = iup_id(iup.val)
iup.toggle = iup_id(iup.toggle)
iup.radio = iup_id(iup.radio)
iup.text = iup_id(iup.text)
iup.list = iup_id(iup.list)
iup.label = iup_id(iup.label)
--dialog
iup.dialog = iup_id(iup.dialog)
iup.filedlg = iup_id(iup.filedlg)
iup.messagedlg = iup_id(iup.messagedlg)
iup.colordlg = iup_id(iup.colordlg)
iup.fontdlg = iup_id(iup.fontdlg)
iup.alarm = iup_id(iup.alarm)
iup.getfile = iup_id(iup.getfile)
iup.gettext = iup_id(iup.gettext)
iup.listdialog = iup_id(iup.listdialog)
iup.message = iup_id(iup.message)
iup.scanf = iup_id(iup.scanf)
iup.getcolor = iup_id(iup.getcolor)
iup.getparam = iup_id(iup.getparam)
--layout
iup.fill = iup_id(iup.fill)
iup.vbox = iup_id(iup.vbox)
iup.hbox = iup_id(iup.hbox)
iup.zbox = iup_id(iup.zbox)
iup.cbox = iup_id(iup.cbox)
iup.sbox = iup_id(iup.cbox)
--additional
iup.cells = iup_id(iup.cells)
iup.colorbar = iup_id(iup.colorbar)
iup.colorbrowser = iup_id(iup.colorbrowser)
iup.dial = iup_id(iup.dial)
iup.gauge = iup_id(iup.gauge)
iup.tabs = iup_id(iup.tabs)
iup.matrix = iup_id(iup.matrix)
iup.tree = iup_id(iup.tree)
iup.glcanvas = iup_id(iup.glcanvas)
iup.pplot = iup_id(iup.pplot)
iup.olecontrol = iup_id(iup.olecontrol)
iup.speech = iup_id(iup.speech)
本文内容由网友自发贡献,版权归原作者所有,本站不承担相应法律责任。如您发现有涉嫌抄袭侵权的内容,请联系:hwhale#tublm.com(使用前将#替换为@)