这篇文章主要是本人学习springboot时一个简单的测试demo,仅供参考。
springboot的介绍:由Pivotal团队提供的全新框架,设计的目的是简化spring新应用搭建的过程,使用特定方式进行配置,无需开发人员进行过多配置。也就是只需要简单的配置就可以进行项目的快速开发,下面的例子是进行一个对外接口的开发。
构建数据库:创建springboot数据库,写sql脚本,然后运行即可:
use springboot;
drop table if exists `t_user`;
create table `t_user`(
`id` int(11) NOT NULL AUTO_INCREMENT COMMENT 'id',
`name` varchar(10) default null comment '姓名',
`age` int(11) default null comment '年龄',
primary key (`id`)
)engine=InnoDB auto_increment=1 default charset=utf8;
项目结构如下:
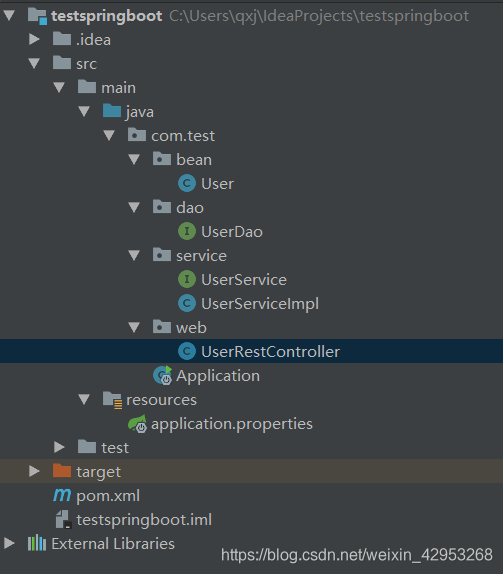
com.test.bean 实体层
com.test.dao 数据操作层(DAO)
com.test.web Controller层
com.test.service 业务逻辑层
Application.java 项目启动类
application.properties 项目运行时加载的配置
maven依赖:
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.1.1.RELEASE</version>
<relativePath/>
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<java.version>1.8</java.version>
<mybatis-spring-boot>1.2.0</mybatis-spring-boot>
<mysql-connector>5.1.39</mysql-connector>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<!-- Spring Boot Mybatis 依赖 -->
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>${mybatis-spring-boot}</version>
</dependency>
<!-- MySQL 连接驱动依赖 -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>${mysql-connector}</version>
</dependency>
</dependencies>
<build>
<plugins>
<!--运用SpringBoot 插件 使用spring-boot-devtools模块的应用,当classpath中的文件有改变时,会自动重启! -->
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<configuration>
<fork>true</fork>
</configuration>
</plugin>
</plugins>
</build>
application.properties自定义配置:
##数据源配置
spring.datasource.url=jdbc:mysql://localhost:3306/springboot?useUnicode=true&characterEncoding=utf8
spring.datasource.username=root
spring.datasource.password=
spring.datasource.driver-class-name=com.mysql.jdbc.Driver
##Mybatis 配置
#配置为 com.test.bean 指向实体类包路径
mybatis.typeAliasesPackage=com.test.bean
#配置为 classpath 路径下 mapper 包下,* 代表会扫描所有 xml 文件。
mybatis.mapperLocations=classpath\:mapper/*.xml
实体类:
package com.test.bean;
public class User {
private int id;
private String name;
private int age;
public User(){
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
数据操作类:
package com.test.dao;
import com.test.bean.User;
import org.apache.ibatis.annotations.*;
import org.springframework.stereotype.Repository;
import java.util.List;
/**
* Title:UserDao
* Description:用户数据处理接口
* @author ty
* @date 2019/4/17
*/
@Repository
@Mapper//表示接口基于此注解实现CRUD
public interface UserDao {
/**
*
*用户增加
**/
@Insert("insert into t_user(id,name,age) values (#{id},#{name},#{age})")
void addUser(User user);
/**
*用户数据修改
*
*
* @param user
* */
@Update("update t_user set name=#{name},age=#{age} where id=#{id}")
void updateUser(User user);
/**
* 用户数据删除
* @param id
*/
@Delete("delete from t_user where id=#{id}")
void deleteUser(int id);
/***
* 根据名称查询用户信息
*/
@Select("selete id,name,age FROM t_user where name=#{name}")
User findByName(@Param("username")String username);
/**
* 查询所有
*/
@Select("Select id,name,age FROM t_user")
List<User> findAll();
}
业务逻辑:
package com.test.service;
import com.test.bean.User;
import java.util.List;
/***
*
* Title:UserService
* Description:用户接口
* @author ty
* @date 2019/4/17
*
*/
public interface UserService {
/**
* 增加用户
* @param user
* @retuen
*
*/
boolean addUser(User user);
/***
* 修改用户信息
* @param user
* @return
*
*/
boolean updateUser(User user);
/**
* 删除用户
* @param user
* @return
*
*/
boolean deleteUser(int user);
/**
* 根据用户名字查询用户
* @param username
* @return
*/
User findUserByName(String username);
/**
* 查询所有
* @return
*
*/
List<User> findAll();
}
业务逻辑实现类:
package com.test.service;
import com.test.bean.User;
import com.test.dao.UserDao;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
/**
* Title:UserServiceImpl
* Description:用户操作实现类
* @author ty
* @date 2019/4/17
*
*/
@Service
public class UserServiceImpl implements UserService {
@Autowired
private UserDao userDao;
@Override
public boolean addUser(User user) {
boolean flag=false;
try {
userDao.addUser(user);
flag=true;
}catch(Exception e){
e.printStackTrace();
}
return flag;
}
@Override
public boolean updateUser(User user) {
boolean flag=false;
try {
userDao.updateUser(user);
flag=true;
}catch(Exception e){
e.printStackTrace();
}
return flag;
}
@Override
public boolean deleteUser(int user) {
boolean flag=false;
try {
userDao.deleteUser(user);
flag=true;
}catch(Exception e){
e.printStackTrace();
}
return flag;
}
@Override
public User findUserByName(String username) {
return userDao.findByName(username);
}
@Override
public List<User> findAll() {
return userDao.findAll();
}
}
控制层代码:
package com.test.web;
import com.test.bean.User;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import com.test.service.UserService;
import java.util.List;
@RestController
@RequestMapping(value = "/api")
public class UserRestController {
@Autowired
private UserService userService;
@RequestMapping(value = "/user",method = RequestMethod.POST)
public boolean addUser(User user){
System.out.println("开始新增用户");
return userService.addUser(user);
}
@RequestMapping(value = "/user",method =RequestMethod.PUT )
public boolean updateUser(User user){
System.out.println("开始更新用户");
return userService.updateUser(user);
}
@RequestMapping(value = "/user",method = RequestMethod.DELETE)
public boolean delete(@RequestParam(value = "id",required = true) int id){
System.out.println("开始删除用户");
return userService.deleteUser(id);
}
@RequestMapping(value = "/user",method = RequestMethod.GET)
public User findByUserName(@RequestParam(value = "userName",required = true) String userName){
System.out.println("开始根据用户名字进行查找");
return userService.findUserByName(userName);
}
@RequestMapping(value = "/findall",method = RequestMethod.GET)
public List<User> findAll(){
System.out.println("开始查找所有用户");
return userService.findAll();
}
}
启动类:
package com.test;
import org.mybatis.spring.annotation.MapperScan;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
/**
* Title:com.test.Application
* Description:程序启动类
* @author ty
* @date 2019/4/17
*/
@SpringBootApplication
@MapperScan("com.test.dao")
public class Application {
public static void main(String args[]){
SpringApplication.run(Application.class,args);
System.out.println("程序在运行");
}
}
完成后运行启动类,在postman中进行测试即可,测试结果如下:
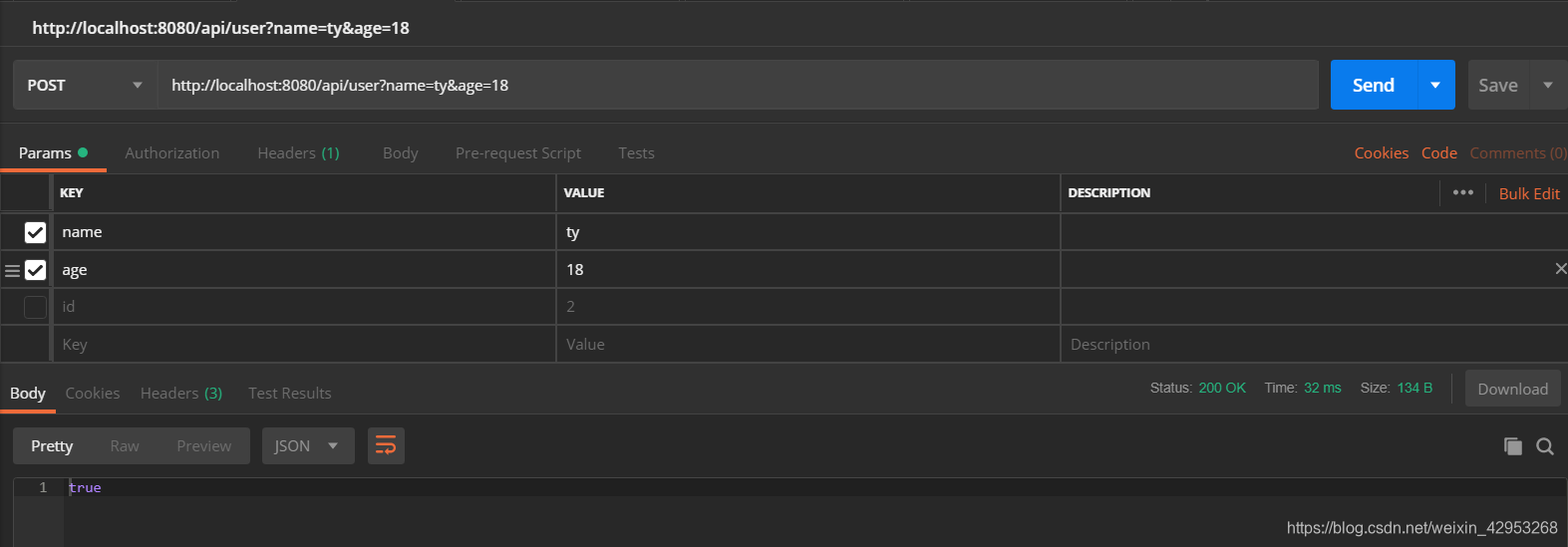
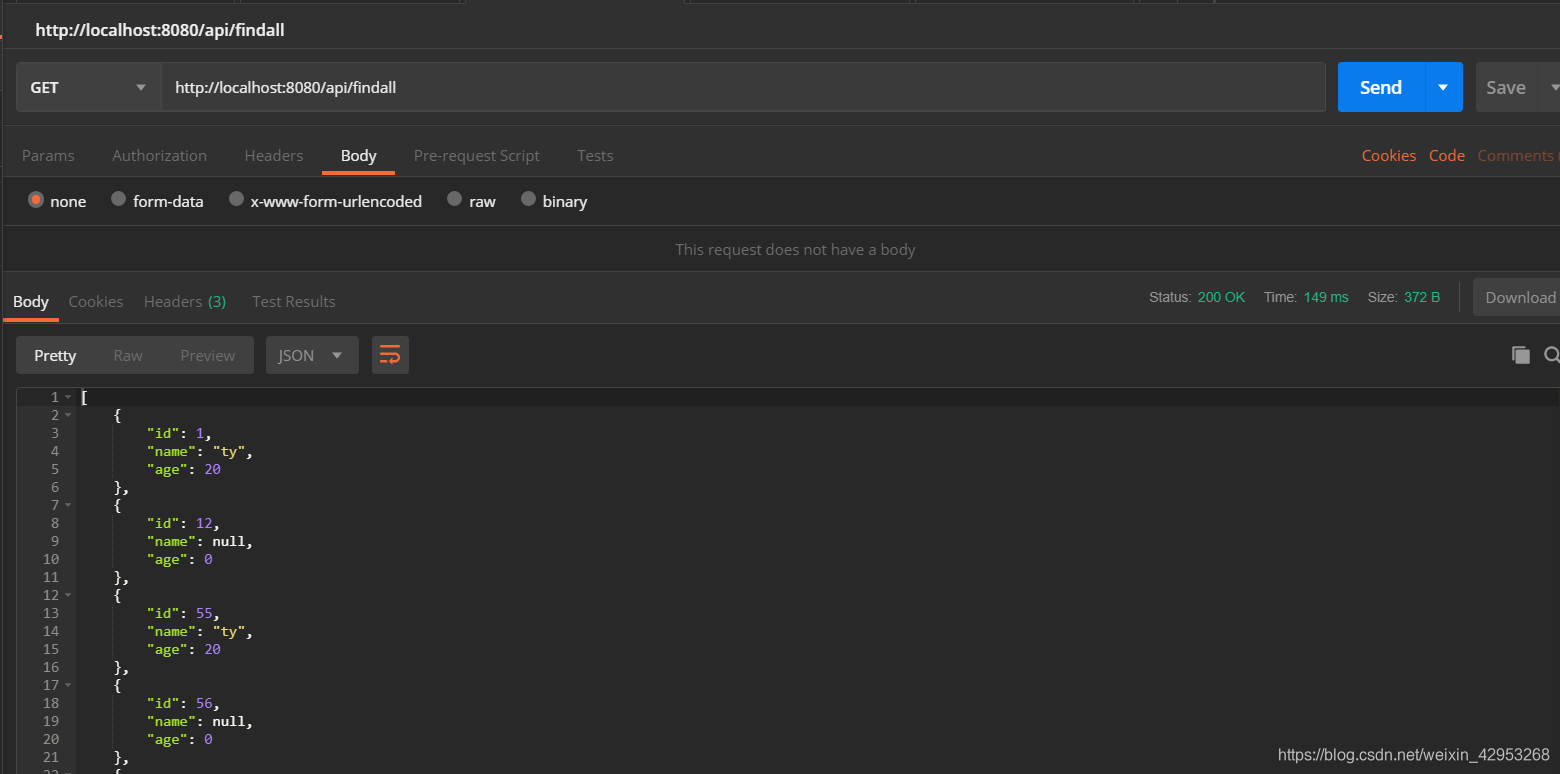
前往数据库查看结果也可以。
本文内容由网友自发贡献,版权归原作者所有,本站不承担相应法律责任。如您发现有涉嫌抄袭侵权的内容,请联系:hwhale#tublm.com(使用前将#替换为@)